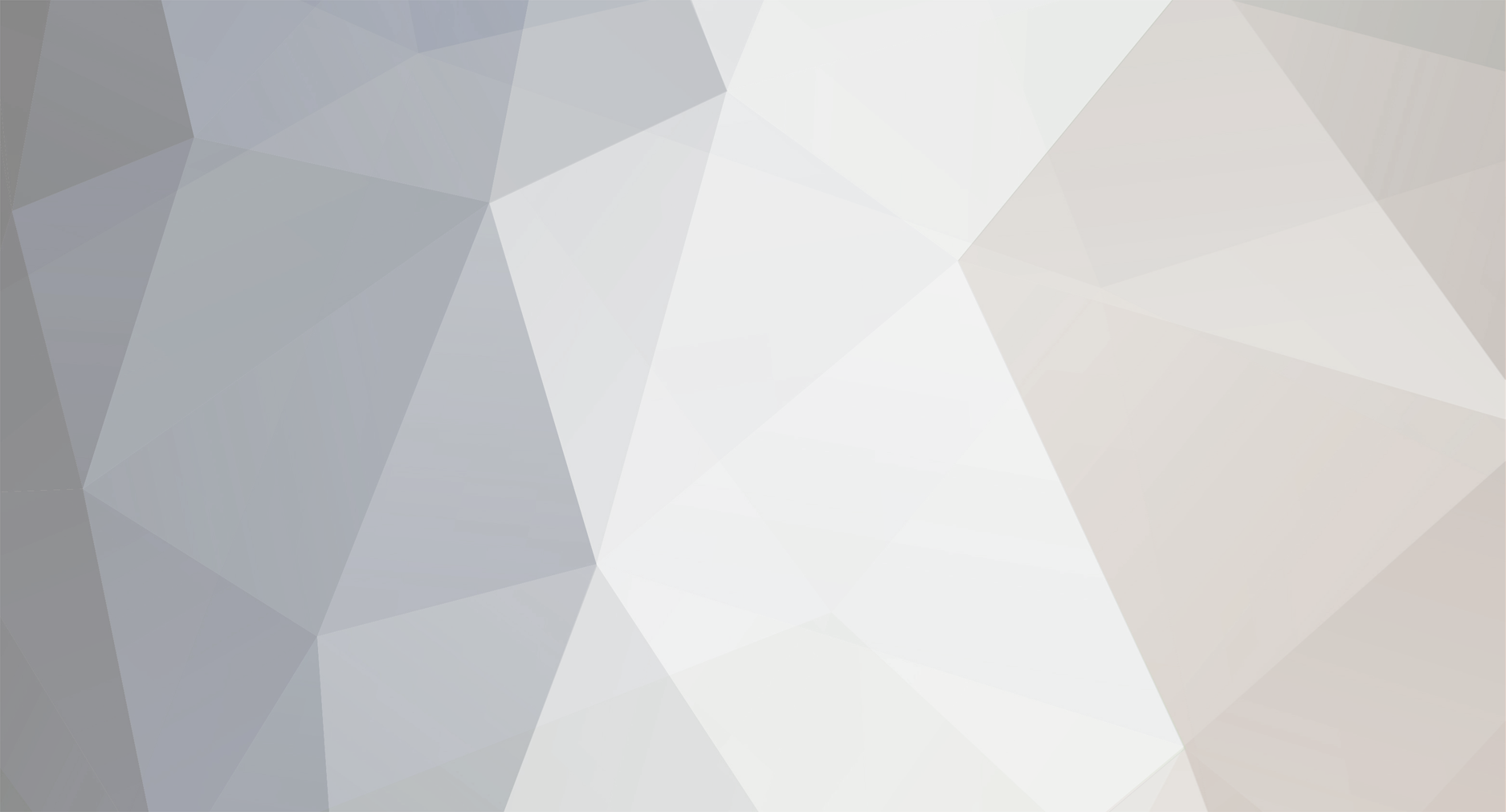
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[SOLVED] alright i was wrong cant figure it out
roopurt18 replied to thewooleymammoth's topic in PHP Coding Help
Why don't you replace 'error' with mysql_error() and get some output that's actually useful. Web servers will send what's called header information to a browser before any of the actual web page is sent. Headers basically warn the browser what the content it's about to receive actually is. For example, if the web server is sending a basic HTML file, it will warn the browser that the incoming content is of type text/html. If instead the web server were about to send a PDF file, it would warn the browser that the incoming content is of type application/pdf so that the browser knows to open it with a PDF reader. This is an oversimplified explanation. The catch with headers is they must come before any of the actual page or document. Certain PHP functions, set_cookie() being one of them, modify header information. Therefore when you have any sort of echo, print, print_r, etc. statement and then call set_cookie, PHP is going to complain at you. Now you tried to fix it by placing $makecookie=setcookie ('auth','$user'); at the top of your script. What this does is calls the function and stores the function's return value in a variable $makecookie. This does not save the statement into a variable to be executed later; it calls the function immediately. Therefore, in conjunction with your first if statement: if($_COOKIE['auth']) The cookie will always be set! The way you have structured your code the first if block should always execute; that is how I knew it was your die() statement that was printing the "error" string. It was impossible for your code to execute the other statement that outputs "error." I suggest you read up a little more in the PHP manual about functions, return values, and how to make use of them. As for the actual statement: $makecookie=setcookie ('auth','$user'); This will set a cookie named auth with the value $user and not the contents of the variable $user. For example, if $user holds the value "bob", the value of your cookie will not be "bob;" it will be "$user." Variables are only replaced with their values inside of double quoted strings: $makecookie=setcookie ('auth',"$user"); // Note the double quotes! But since there is no other string inside the double quotes, why not just use the variable? $makecookie=setcookie ('auth',$user); // No quotes Now let's look at your line: $makecookie; Since $makecookie stores the result of a function call, in this case either true or false, this statement is equivalent to one of the following (depending on what the function returned) // this one true; // or this one false; Both of them are valid PHP statements AFAIK, but neither does anything useful. As for actually figuring out what's wrong with your code, here are some steps you can follow. First, insert the following at the bottom of your file. This function will allow you to dump useful information about your data. After all, you know what the data should be, so make sure it's what you're expecting. function dbg_echo($val){ echo '<pre style="text-align: left;">' . print_r($val, true) . '</pre>'; } Next, change the following: $connectmysql=mysql_connect('localhost',$username,$password) or die(mysql_error()); $selectdb=mysql_select_db($database) or die(mysql_error()); $query=mysql_query("SELECT*FROM $table WHERE username='$user'"); $result=mysql_fetch_assoc($query) or die('error'); to // You do not need either the $connectmysql or $selectdb variables // you had. You only need to save values in variables if you are // planning to use them again. Your script makes use of neither. mysql_connect('localhost', $username, $password) or die("connect: " . mysql_error()); mysql_select_db($database) or die("select_db: " . mysql_error()); // Here you do need the $query value because you are planning // to use it $query = mysql_query("SELECT*FROM $table WHERE username='$user'") or die("select: " . mysql_error()); $result = mysql_fetch_assoc($query); dbg_echo($result); -
I've found that the preg_* functions seem to crash when the string you're applying the regexp gets large. Try using the regexp on a smaller string first and see if it succeeds.
-
That's pretty inefficient if all of the records are coming from the same row in the source table. INSERT INTO tblDest (dest1, dest2, dest3) SELECT src1, src2, src3 FROM tblSrc WHERE conditions
-
[SOLVED] alright i was wrong cant figure it out
roopurt18 replied to thewooleymammoth's topic in PHP Coding Help
How do you know the string "error" that you're seeing is not from this line: $result=mysql_fetch_assoc($query) or die('error'); You also have this line: $makecookie=setcookie ('auth','$user'); You should probably drop the single quotes around the variable $user. What are you trying to accomplish with the line: $makecookie; Also, you have spelled combination wrong. -
Filtering out phone numbers from a string...
roopurt18 replied to nevynev's topic in PHP Coding Help
I have to wonder why you wouldn't want people to be able to post phone numbers or emails on a social networking site? Besides, once you get it down all I have to do is post with: I can be reached at $1,444,555,123.4 e123 -
Unless you're running Google, Yahoo, YouTube, MySpace, etc. then the performance gained by such a "tweak" would be almost negligible. The process for programming web pages which interact with a database should always, IMO, be: 1) Write your code such that it is readable and maintainable 2) Execute your code in a real world environment, with real data 3) Monitor your page load times, if a page loads slowly do the following: 3a) Check all of your queries being executed, update DB indexes as appropriate 3b) Make any changes to actual code next, typically in the form of refactoring or modifying your algorithm / process 4) Build your site such that you can turn on performance logging. When this feature is enabled you're site should notify you of any page or query that takes longer than X seconds to load or execute. This will help you spend your time optimizing code where it is needed.
-
If you run a company that sells tangible products, you expect to lose a certain amount of revenue due to theft or damaged goods. You accordingly budget this into your business strategy so that you can cope with the loss. You should take the same approach with your game. Instead of spending many resources trying to prevent it altogether, you should modify your game design such that the impact of cheating is negligible. How you do this depends on your game though and putting the idea into practice can be difficult. Here are some ideas: Use a system of diminishing returns. For example, when you first attack someone you earn the full amount of reward if successful. On the second attack, you are limited to 50% of reward from the previous attack. In conjunction with the above rule, the player is not able to obtain 100% reward until either: 1) They attack a certain number of different enemies, like 10 2) A certain amount of time passes, like 24 or 48 hours You can limit the amount of gold, items, etc. that a player can hold at any one time. It's hard to say more without knowing about your particular game.
-
convert mysql date format "2007-04-01" to "April 2007" output
roopurt18 replied to scotchegg78's topic in PHP Coding Help
If a mod feels I'm out of line, by all means edit this post, but this response was way out of line. You asked a question, someone gave you the information to solve your problem with two different methods. In the PHP documentation, all it took was a ctrl + f -> "mysql" and you get this code: // format MySQL DateTime (YYYY-MM-DD hh:mm:ss) using date() function datetime($syntax,$datetime) { $year = substr($datetime,0,4); $month = substr($datetime,5,2); $day = substr($datetime,8,2); $hour = substr($datetime,11,2); $min = substr($datetime,14,2); $sec = substr($datetime,17,2); return date($syntax,mktime($hour,$min,$sec,$month,$day,$year)); } The link to the MySQL documentation contains six examples less then half a page down. Both of them were obtainable by you in less than 15 seconds, yet you decided to take that time to reply and throw help back in someone's face? It's irrelevant that the date is already returned in a query as you can always modify the query to return different or additional information. As thorpe pointed out, it's almost always more efficient to have the database engine perform the work for you. I suggest you learn some basic manners and how to take a little initiative before you attempt to do much programming; if you do not learn how to apply a general example to your specific problem you will extremely hamper your ability to become a proficient programmer and problem solver. -
Send data submitted by user in form to address bar
roopurt18 replied to e1seix's topic in PHP Coding Help
What thorpe said is probably the best approach, I just wanted to point out you can only use variables inside of double quoted strings and not single quoted strings like in your code. -
The only Javascript book I've ever read, likely I'll never need another. http://www.amazon.com/JavaScript-Definitive-Guide-David-Flanagan/dp/0596101996/ref=pd_bbs_sr_1/104-4895521-9474323?ie=UTF8&s=books&qid=1188432087&sr=8-1
-
mysql_fetch_assoc is updating an internal record pointer so that after the first full loop is finished, it will appear empty. Check out this function: http://www.php.net/manual/en/function.mysql-data-seek.php As a side note, if your result sets are large you will find that looping over it many times is slow an inefficient. You should try and organize your code so that you loop over it only once.
-
I was going to add that you can prevent repeating code by wrapping it in a function. Then at most you're repeating one line, i.e. the function call.
-
You need to set the headers to indicate an incoming file to the browser. I don't have a working example in front of me, but try going here: http://php.net/header Scroll down to where it says Example 1578. Download dialog. Remember to change the content-type and see if that doesn't give you a step in the right direction.
-
I think most wireless routers come with a set of wired jacks as well.
-
I hate counting; discrete math is a beast.
-
Wouldn't it be 120 combinations?
-
[SOLVED] how do I show if something is new?
roopurt18 replied to acidglitter's topic in PHP Coding Help
That echo statement I gave you is your best friend when debugging. Program not working? Dump the data you have, compare it with the data you should have, fix and repeat as necessary. Glad you got it resolved! -
Well, what I mean is as an expression tree: x * x +-- x | * --+ | +-- x If you differentiate each branch of the tree separately it'll become: +-- 1 | * --+ | +-- 1 Then when you evaluate that you arrive at 1, instead of 2x. So I revise my approach to: 1) Accept user's input 2) Break into expression tree 3) Simplify expression 4) Break simplified expression into tree 5) Create the derivative function of the tree 6) Evaluate the derivative function Steps 3 and 6 are really the same function, namely one that evaluates and simplifies the expression. It should change things like 2 * (x + x) into 4x
-
One more thing to consider, you might need to pass the initial user input through an expression simplifier. For example, if the user enters: x * x + 5 You want to make sure that your program outputs 2x rather than 1
-
Might want to remove this one:
-
i was not aware that you can chain methods in php
roopurt18 replied to emehrkay's topic in Miscellaneous
It's pretty simple. Let's say you have a class Manager that can return an instance of your DB object: <?php $db = Manager->getDB(); $db->query($sql); ?> Since you know that the getDB method of Manager will return a DB object, you don't really need the $db variable. <?php Manager->getDB()->query($sql); // Due to operator associativity, the above is executed as if it were: // (Manager->getDB())->query($sql) // The (Manager->getDB()) is representative of the $db var in the // first example ?> You see this syntax more often in Javascript, Java, and C++. For example, if you wanted the body element in Javascript: document.getElementsByTagName("body")[0]->appendChild(someNode); I'm not sure about the implications in Java or Javascript, but if you were going to reuse the returned object multiple times I'd just store it in a variable. Using the first example again: <?php $db = Manager->getDB(); $db->query($sql); echo $db->successMsg(); ?> I consider that a little more efficient (as far as PHP is concerned) than: <?php Manager->getDB()->query($sql); echo Manager->getDB()->successMsg(); ?> The reason is that in the second example here we have to call Manager->getDB twice to get the same value; this is slightly inefficient, but will still run really fast. However, in a language like C++ you don't have this problem. You can return a const reference to the object (yes I'm aware you can return references in PHP) and you can also declare the function as inline. Hope that helps to clarify! -
[SOLVED] how do I show if something is new?
roopurt18 replied to acidglitter's topic in PHP Coding Help
Add this to your loop: echo "<pre style=\"text-align: left;\">" . print_r($row, true) . "</pre>"; -
[SOLVED] how do I show if something is new?
roopurt18 replied to acidglitter's topic in PHP Coding Help
You might try putting NOW() - INTERVAL 1 WEEK inside of parens. -
If you're storing this in a database, please use a DATETIME field.
-
[SOLVED] how do I show if something is new?
roopurt18 replied to acidglitter's topic in PHP Coding Help
Hmmm. The syntax should be ok, I ran this and came up with results: SELECT NOW( ) , NOW( ) - INTERVAL 1 WEEK, IF( NOW( ) - INTERVAL 1 WEEK < NOW( ) , 1, 0 ) AS date FROM Asset WHERE 1 LIMIT 1 I can only think of two things. 1) date is a MySQL keyword, try enclosing it in backticks 2) Your version of MySQL doesn't support this feature (would seem unlikely)