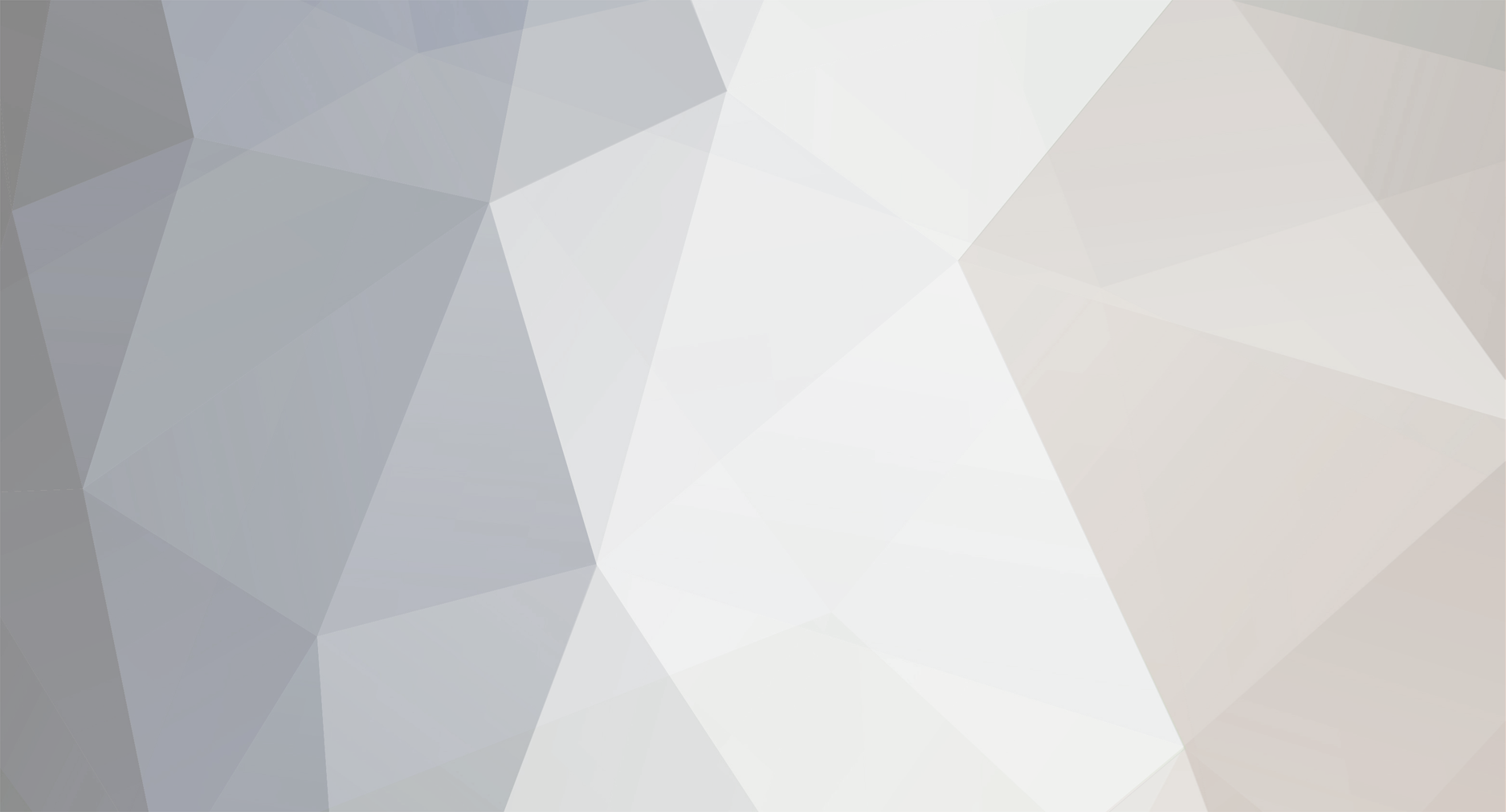
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Post your PHP script.
-
<html> <head> <script type="text/javascript"> function disp_confirm(){ var msg = "Do you really want to delete this?"; if (confirm(msg)){ window.location.href = "http://www.yahoo.com"; } return false; } </script> </head> <body> <a href='' onclick='return disp_confirm();'>delete</a> </body> </html>
-
Try this: function disp_confirm(){ var msg = "Do you really want to delete this?"; if (confirm(msg)){ window.location("delete.php?id=$data[id_cat]"); return false; } else{ return true; } }
-
You don't call window.location as if it's a function. Do you see any difference at all between the following? // wrong! window.location("delete.php?id=$data[id_cat];") // correct! window.location.href = "http://www.yahoo.com";
-
[SOLVED] shorter way of selecting 1 specific thing from DB?
roopurt18 replied to scarhand's topic in PHP Coding Help
<?php $sql = "SELECT name, value FROM styles WHERE name IN ('mainbgcolor', 'maintextcolor', 'mainbordercolor')"; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ ${$row["name"]} = $row["value"]; } } ?> -
A core file is a memory dump created when a compiled program crashes. So yes, they're bad as in they indicate something isn't working somewhere. I couldn't begin to predict what process on your server is creating it or tell you how to figure that out.
-
[SOLVED] I need to export query results to a .txt file.
roopurt18 replied to JSHINER's topic in PHP Coding Help
You have to place \n inside of double quotes. -
[SOLVED] I need to export query results to a .txt file.
roopurt18 replied to JSHINER's topic in PHP Coding Help
If the .txt file is being called up in a web browser, why not just change the link to the file to a .php script. Then do the following: <?php header("Content-type: text/plain"); $sql = "SELECT ..."; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ echo "{$row['col1']},{$row['col2']}"; } } ?> -
[SOLVED] I need to export query results to a .txt file.
roopurt18 replied to JSHINER's topic in PHP Coding Help
How exactly is this text file "called up?" Through the web browser? -
I tend to limit the amount of data in my sessions to the username / id and if they're logged in or not. I don't think there's any extra security gained from this, but it allows me to only have to worry about updating information in one place. Here's a hypothetical situation for you. You have an admin user that is abusing their privileges. The super-user logs in and demotes them to non-admin. Now, if each page load is reading the user's status from the DB then they immediately lose their privileges. If you have their role / status stored in a session then it won't take affect until they log out, which means they can continuously abuse their privileges even though the super-user has revoked them.
-
You're taking shots in the dark at optimizing your database; which is to say there are areas you suspect are problems and want to fix them without any actual proof. You need to gather actual data about performance before you run off and willy-nilly start changing database design and structure. Assuming you are calling a wrapper function for your database calls this shouldn't be difficult to implement. Before and after every single DB call, check the current time and compare the two results. If any query runs longer than X seconds, where X is an arbitrary number decided by you, log the following into a separate DB table: the query, how long it took to run, the return value of debug_backtrace(), and the time that this occurred. Set this up so you can easily disable / enable logging; you don't want it to always be on as it will affect performance. After a week or so of periodic logging you should be able to determine which queries are bringing your site down and from where they're being called.
-
Well now you know your script isn't terminating early without throwing an error, which can happen. Are you sure that your DB connection is successfully made and that your query is actually returning results?
-
Well, throw an echo statement into your script as the last thing. echo "hi"; ?> If you see a 'hi' on your browser it finished executing; if you see nothing the problem is something else.
-
The id attribute of page elements has to be unique, so you can't have multiple images with the same id. The best way around this is to assign all of the images the same class attribute; then you can grab all of the images on the page, loop over them, and assign the event handler to any that have that class. <img class="specialImg" alt="blah blah" src="path/to/image"/> <script type="text/javascript"> var imgs = document.getElementsByTagName("img"); var max = imgs.length; for( var i = 0; i < max; i++ ){ var img = imgs[i]; if(img.className != "specialImg"){ continue; } if(img.addEventListener){ img.addEventListener("click", doSomething, false); }else if(img.attachEvent){ img.attachEvent("onclick", doSomething); } } Is there a relationship between the thumbnail src and the regular image src? Usually I name mine the same thing but prepend a th_ onto my thumbnails. If the img is inside an a tag that points to the image you want to display there is another approach I can help you with.
-
It would be hard to say what's going on without seeing some more of your code. Or you can try changing the line: window.location("delete.php?id=$data[id_cat];") to window.location.href="http://www.yahoo.com/" Just to make sure it's redirecting correctly when you press OK.
-
You should call exit() after making a call to header where you set the location. Headers are basically "hidden" information that is sent between the browser and web server; it's hidden in the sense that you have to make extra effort to view it in most browsers. Header information gives the browser clues on what to expect, such as what type of file the server is about to send or if it should set a cookie or not. The way the HTTP protocol works headers have to be sent before any other form of output, such as the actual HTML. The moment your script spits out any output, even a white space, you are no longer allowed to set any header information.
-
Ok, so when the event handler is invoked there is an event object. In IE the event object is global (window.event) and in other browsers it is passed to the event handler. In both cases, the event object will have a property that refers to the object that triggered the event; in your case it will be the image. In IE this property is named srcElement and in other browsers it is named target; you might find this resource helpful: http://www.javascriptkit.com/domref/domevent.shtml <html> <head> <script type="text/javascript"> function doSomething(e) { var posx = 0; var posy = 0; if (!e) var e = window.event; var node = window.event ? e.srcElement : e.target; alert(node.src); if (e.pageX || e.pageY) { posx = e.pageX; posy = e.pageY; } else if (e.clientX || e.clientY) { posx = e.clientX + document.body.scrollLeft + document.documentElement.scrollLeft; posy = e.clientY + document.body.scrollTop + document.documentElement.scrollTop; } alert(posx + ", " + posy); } </script> </head> <body> <BR><BR><BR><BR> <img id="theDiv" alt="Logo" src="path/to/image"/> <script type="text/javascript"> var div = document.getElementById("theDiv"); if(div.addEventListener){ div.addEventListener("click", doSomething, false); }else if(div.attachEvent){ div.attachEvent("onclick", doSomething); } </script> </body> </html>
-
Why are you trying to delay the loading of the Javascript?
-
Try tacking an or die(mysql_error()) onto the call to mysql_query.
-
Where is the image in relation to the object being clicked on? Or are you attaching the event handler to the image?
-
Unless your client is on a really, and I mean really, tight budget, they should look into upgrading their host. You can get a hosting plan that has at least one database for as little as $3 / month AFAIK. The amount of money they'll spend just paying you to write code to piss around with flat-file storage would probably cover the hosting cost for 6 to 12 months.
-
Cookie information is sent with headers and you can't echo anything to the browser without sending all of the headers. You don't have error reporting turned on, but if you did you would get a message about headers already sent, unable to blah blah blah. Either queue up all of your output into a variable and echo it a single time at the end, turn on output buffering, or set your cookie before you echo anything.
-
So place the file in a directory that can't be accessed by a browser. Or when you upload files via FTP does it automatically stick them in public_html?
-
<html> <head> <script type="text/javascript"> function doSomething(e) { var posx = 0; var posy = 0; if (!e) var e = window.event; if (e.pageX || e.pageY) { posx = e.pageX; posy = e.pageY; } else if (e.clientX || e.clientY) { posx = e.clientX + document.body.scrollLeft + document.documentElement.scrollLeft; posy = e.clientY + document.body.scrollTop + document.documentElement.scrollTop; } alert(posx + ", " + posy); } </script> </head> <body> <BR><BR><BR><BR> <div id="theDiv">asdasd</div> <script type="text/javascript"> var div = document.getElementById("theDiv"); if(div.addEventListener){ div.addEventListener("click", doSomething, false); }else if(div.attachEvent){ div.attachEvent("onclick", doSomething); } </script> </body> </html>
-
What does the URL look like after you click the delete link and then click OK to confirm?