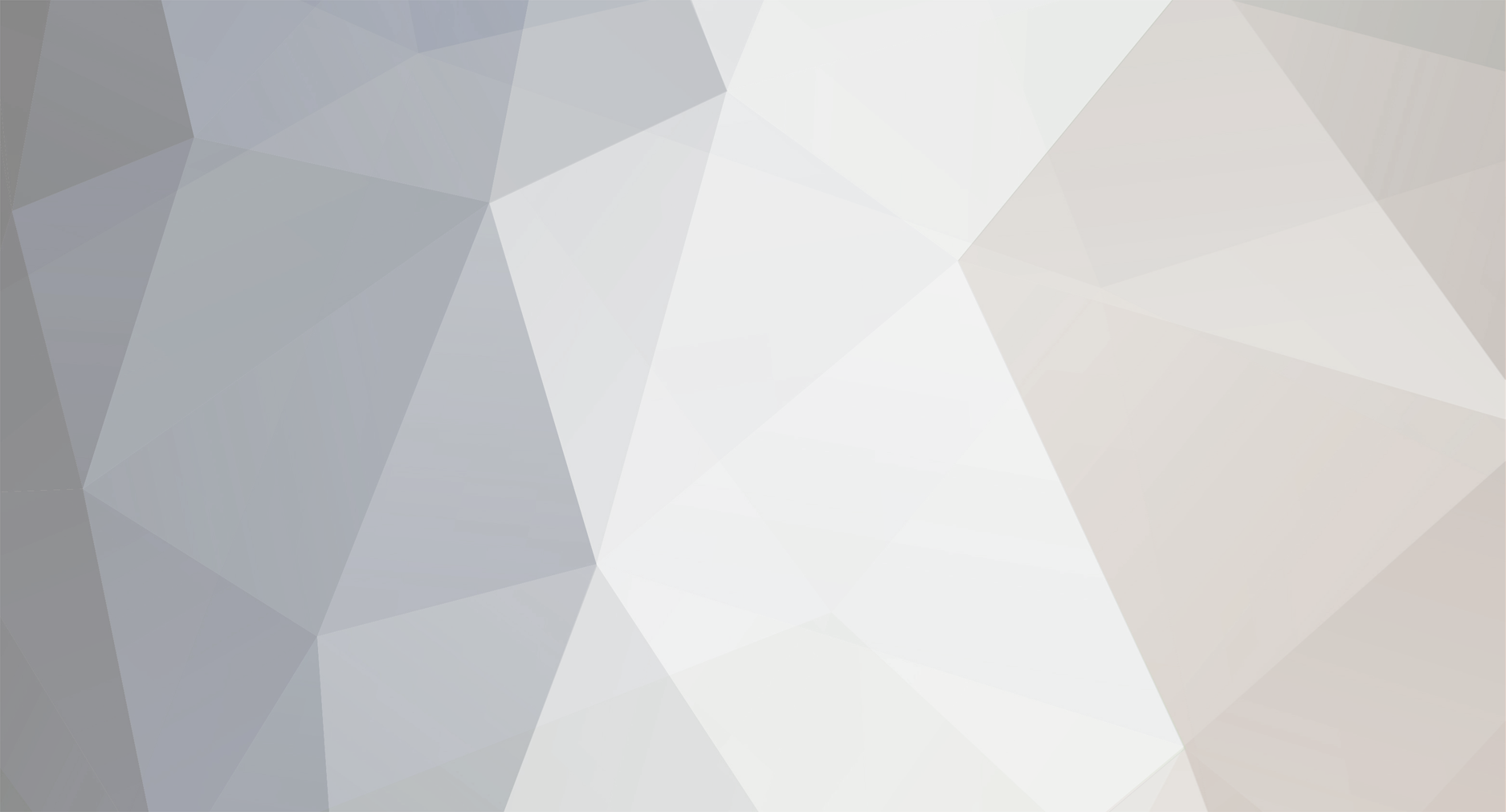
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I'm trying to do something like: select * from ( case 1 when 1 then ( select distinct p.pro_code, p.project from wsproj p, wsjob j, webopthd o where p.pro_code=j.pro_code AND j.job_code=o.job order by p.project ) else ( select pro_code, project from wsproj order by project ) end case ) as t Basically I need to run one of two queries based on a parameter. This is done in a report writer so I can't use programming logic to choose one query or the other.
-
I agree about avoiding synchronous requests, however I have used them in minor places when they're expected to be quick. One place where I'll usually use a synchronous request is on saving data. I don't want the client to click off the page during a save so that they miss the status message for the request. I could create an iframe and post the results into there so that even if they leave the page they're notified of the save status; but I doubt my boss would want me to take the time to implement that and then go back and upgrade the few areas that need it. Bleh!
-
Have a look here; use your browser's "View -> HTML Source" http://ns2271.serverpowered.net/test/test.html
-
What would you love to see changed or add to PHP?
roopurt18 replied to tibberous's topic in Miscellaneous
Not necessarily. In most cases, the needle is a scalar and the haystack is an array. In that case, you just change the function implementation to check the type of the first and second arguments. Then you change the documentation to reflect whatever. That should handle 99% of it. -
Weird! I'll look into it.
-
Just make sure you trim it before checking the length or the following will pass: " "
-
If you want to force the user to enter a value in a text box, you should check the length. You have to watch out for people entering malicious content as well, such as HTML / Javascript. if( !(isset($_POST["first_name"]) && strlen(trim($_POST["first_name"])) > 0) ){ $errs[] = "You must provide a first name"; }
-
This is a Javascript issue. HTML is just a formatting language, it doesn't provide any event handling or programming functions. The quick and dirty way, which I whole heartedly do not recommend: <input type="submit" id="btnSubmit" value="Submit"> <script type="text/javascript"> document.getElementById("btnSubmit").onclick = function(){ this.value = "Uploading..."; } </script> That should be more or less it, or get you part of the way there. You should google <i>Javascript event model</i> and <i>Javascript DOM</i> to learn more.
-
I'm wondering if anyone would like to fill this small form out. I just need some seed data to practice creating reports on. I don't care if the information is phony, as long as it's clean. http://ns2271.serverpowered.net/wv/demo/guest/InterestView
-
I'd call it a lowly hack in that it will not prevent you from running into problems. Also, you must remember that most browsers will only hold two connections to a server open at a time. You have two options here. 1) Implement a Javascript priority queue. Instead of enacting the request right away, place it on the queue to be executed later when a request is available. You can only ever have two requests happening at once, so bear that in mind before allowing the queue to launch another request. Also, you should implement age-based priority to prevent a low-priority request from sitting in the queue forever. 2) Reduce the number of AJAX requests. I had a situation in my own Javascript program where it would send a request to the server, wait for the response, and based on the response send another request to the server. The second request was dependent on the first request, so I used a synchronous request in the first request, which would lock the UI until it returned. Eventually I realized I only needed one request. Example of two requests (pseudo code) request1 = send_sync_ajax_request() if(request1 == ONE_VALUE){ request2 = send_async_ajax_request() }else{ request2 = send_other_async_ajax_request() } alert( request2 ); Here we have two AJAX requests when we really only need one. Instead of performing the logic of testing the result of request1 on the client, why not just test the result on the server and invoke the second request from the server? Reducing requests down to 1 (pseudo code) // Javascript request = send_ajax_request(); alert( request ); // PHP Script on the server $result1 = perform some action based on $_POST / $_GET parameters if($result1 == ONE_VALUE){ $result2 = perform one thing }else{ $result2 = perform another thing } $JSON = Array( "result1" => $result1, "result2" => $result2 ); echo Array2JSON($JSON); A real world example would be the user enters a coupon code at a shop; if the coupon is valid you return a list of items it applies to, if the coupon is invalid you return a list of items that are still bargains and similar to the items the coupon would have applied to. You must validate the coupon on the server so the client can't bypass it. So with two requests you'd do the validation, then send another request based on the validation. But really you could send the coupon code, determine if it's valid, and return the proper list from the server right from the beginning, thus using a single request.
-
Once you have the event object, either passed to the function with the DOM L2 model or through the global window.event object in IE, you can access the node that triggered the event. function handler(oEvent){ oEvent = oEvent || window.event; // Get the proper event object var oNode = oEvent.target || oEvent.srcElement; // Get the proper node object oNode.value = "Hello, World!"; // Or if it's a list box alert(oNode.options[oNode.selectedIndex].value); } If you use the event models correctly, you don't need the this keyword. In addition, the node object will have all the properties of the this keyword in addition to the DOM methods as well. Your statement about having to handle multiple models being a pain is correct, but that doesn't absolve you from being a good programmer and working with what is the current standard. My suggestion: Learn how to use the event models and get your Javascript out of your HTML; it just makes it ugly.
-
That's why I wrapped it up in my own Javascript events interface that creates a single client interface despite the browser being used. All of my Javascript is much cleaner and more concise. function someHandler(oEvent){ alert(oEvent.node.value); } Events.addEvent( document, "load", function(oEvent){ // I can add events two ways, with the element or with it's ID var el = document.getElementById("some_textbox"); Events.addEvent( el, "change", someHandler ); Events.addEvent( "other_textbox", "change", someHandler ); });
-
[SOLVED] Are you a programmer? Read this, it's VERY important.
roopurt18 replied to jscix's topic in Miscellaneous
According to one of the more reliable links, the legislation fell by the wayside after 9/11 and Congress has only now gotten back around to it. As the article points out, I think it was the businessweek one, the problem is not piracy but the media industries inability and / or refusal to change its business models for a new type of distribution. So far, the big proponents are the music and movie industries, so the first targets will be digital entertainment media. The concept is to lock out media at the hardware level, rather than the software level. In that regard, this proposes no danger at all to open-source code, which is what the OP was originally warning about. This legislation is about protecting copyrighted material, I really don't see how it could possibly stop the open-source community. <rant> IMO, the movie industry is full of itself. "People aren't rushing out to the theaters anymore to watch our movies, they _MUST_ be pirating them!" I'm sure it has nothing to do with what most of Hollywood producing being total crap, the cost of a ticket being $10, and a !@#$%^& popcorn and soda costing $8 dollars. Let's just add on the wonderfully clean bathrooms and the nanometer thin toilet paper that every theater seems to have. Anyone remember when you had to wait almost a year for a movie to go from theater to the video store? Now it takes about 3 to 6 months at the most. Ready for the killer, Hollywood, here it is. Home entertainment centers that cost only a few grand. Let's assume a family that frequently likes to watch movies. Average trip to the theater is going to run $40 to $50 for a movie that is most likely going to suck. For the amount of money saved by not going to the theater for a single year, you can afford a moderate big screen and surround sound in addition to a monthly rental subscription at the video store. Not only that, you can buy cheap candy from the supermarket and if you have to take a crap, you can pause the movie, crap at your leisure, and be sure that your finger isn't going to pop through the paper. But "Nooooooooooooo." Consumers _MUST_ be pirating everything. It couldn't possibly be that we've been getting the shaft for years, reasonable alternatives are now available, and consumers are making wiser spending choices. What you're experiencing Hollywood, is not the effects of piracy; it's the same thing the cable and phone companies went through, and that is your "clients" giving you a big, gigantic, F U. </rant> -
What would you love to see changed or add to PHP?
roopurt18 replied to tibberous's topic in Miscellaneous
The needle / haystack flip-flop is probably my #1 reason for consulting the manual. -
That will work correctly with multiple users.
-
Unless I'm mistaken, if the users are on different connections to the DB then last_insert_id will return the ID for the user calling it and not other users.
-
OK. I've asked the host about upgrading the memory on the VPS; I will have to move to a dedicated server. We are currently paying ~$170 / month in hosting fees for two VPS, one of which is managed. I'm thinking I can go with their cheapest P4 package, $159 / month with 512MB RAM, with a 512 MB RAM upgrade, another $19 / month. That would put my company at ~$180 / month for the dedicated and another ~$70 / month for the VPS we use as a test environment. However, I think it would be best to just create a second user account on the dedicated server and use it as the development area. Basically, use a single dedicated in place of both our VPS packages. Does this sound like a good route to go to anyone else?
-
I suppose I can ask the host; although when I signed up I asked them about expanding the allocated disk space at some point in the future. Their response was that, at least for the HDD, I'd need to move from VPS to dedicated. So I sort of expect the same in regards to memory. Also, I found a lot of threads where people were getting the "Could not allocate heap memory blah blah blah" inside of a VPS. The problem arises from the JVM detecting the full system's resources and not those allocated to the VPS, so even if they did up my memory, I might still run into issues.
-
I have a VPS package with 512MB of RAM and plenty of bandwidth and HDD space. Along with the regular LAMP services I want to install Tomcat so PHP can interface with a Java report writer. The server sits at approximately 100 to 120MB of free memory and every time I consistently get this output: @ java -version Error occurred during initialization of VM Could not reserve enough space for object heap Could not create the Java virtual machine. In another forum, it was suggested to limited the memory java tried to use: @ java -Xms4m -Xmx8m -version Error occurred during initialization of VM Could not reserve enough space for object heap Could not create the Java virtual machine. But I still get the same error. However, if I first kill apache and then try the above again, it works: @ apachectl stop /usr/local/sbin/apachectl stop: httpd stopped @ java -Xms4m -Xmx8m -version java version "1.5.0_12" Java(TM) 2 Runtime Environment, Standard Edition (build 1.5.0_12-b04) Java HotSpot(TM) Server VM (build 1.5.0_12-b04, mixed mode) I haven't even set tomcat up yet and it appears I'm hitting memory limits. Is it time to jump up to a dedicated host with 1GB+ of RAM or do you think I can tweak the server until all is running? Once I get this running I have no idea how many resources actually generating reports might use and I expect a fair amount of report generation to occur. Server is CentOS 4.5 and I'm currently trying to get a "proof of concept" going on a local CentOS installation using VMWare. I'm guessing a dedicated host might be necessary, although I'm trying to save as much money for my company as possible because our budget is currently pretty tight. Thanks for any input / suggestions.
-
You should not be placing any Javascript directly into your HTML. If you read up on the proper way to use the JS event model you can determine the DOM node that caused the event without passing anything to the event handler. I don't feel like typing up an extended example right now, but this should get you started: http://www.quirksmode.org/js/introevents.html There are two basic event models you should support, the IE model and the DOM Level 2 model. At this stage of the game I think you can safely ignore the Level 0 model.
-
The quick version: I'd like to provide reporting in our PHP software, lots of it. On our server product (MS Visual Foxpro), we provide a standard set of reports. We frequently receive requests for new reports or slight variations on existing reports, but these requests typically come in one client at a time. Basically, Client A sees report Y, which was created for Client B; Client A says "Report Y would be great if only it provided ..." So we create a new report Z for Client A and release it into the software. All reporting in our Foxpro product has to be done through programming, which means a lot of hours spent writing reports and less hours improving or maintaining the software. This is what I'm trying to avoid in our PHP application, which is used by the same clients with the same frequent demand for new or modified reports. I could spend a significant amount of time programming a report writer in PHP, but why reinvent the wheel? Searching the web I found BIRT, an eclipse based open-source reporting solution. This looks like the ideal candidate to solve this problem. I can create the reports locally, upload them to the server, and then expose them to our clients through a PHP interface. The down-side is BIRT is Java-based so I have to set up a Java application server on our VPS. I started this thread on that topic: http://www.phpfreaks.com/forums/index.php/topic,151873.0.html I'm running heap memory errors which are causing the JVM to be unable to start. Looking into that, it appears there can be some issues in trying to run a JVM on a VPS. According to the BIRT installation instructions, I can have PHP interact with the Java classes directly (possibly through PHP - Java bridge), or I can make requests to an application server such as Tomcat. In either case, I need the JVM to start. So unless Buyocat or anyone else has an alternative solution to the problem, it may be time to order a dedicated host or to run a second VPS without apache / mysql / etc and use it as the application server.
-
PHP-JAVA - What remoting protocol to use? Best practice?
roopurt18 replied to mazaka's topic in Application Design
I am currently working on a similar issue. I found an open-source eclipse based reporting software that I'm hoping I can use to quickly build reports for our clients. It's Java-based so my initial plan is to install a Tomcat server for the reporting and request reports through PHP. However, I think I'm running into memory constraints on our VPS so I'm not sure how it's going to turn out. I'd be curious to hear how you go about solving this issue or what anyone else has to offer. -
I just wanted to chime in with an alternate approach that you might want to look into after you get the basics working. Your example is you want to continuously update part of the page with the most recent contents from the server, which I assume are changing from input elsewhere. Let's say that on average the part that is being updated consists of 2KB of text. If you are refreshing this every 1 second that is 120KB of text every minute. If you're site has a mere 10 users they will consume 1MB of bandwidth every minute, where each minute could be inactivity on their part. This could be seem as extremely wasteful. Let's say the file you want to refresh is stats.php. The best approach is to store a timestamp on the server, possibly in your database, of the last time the stats were changed. When you initially feed the page to your clients, they'll have the most up to date version of stats.php. In addition to what you're already sending them, send them the server's timestamp as well. Then you set up Javascript on the client to continuously poll the server using the timestamp it received as an argument. The server will check the timestamp passed by the client and if it is older than the timestamp of the last change to stats.php, you can send the new stats page; otherwise you send a value indicating to the client that no change has been made. Essentially, the conversation looks like this: client: Server, I'd like to view home.php, which has embedded within it stats.php server: Here is home.phpp along with stats.php; the last time stats.php was updated was 3PM today. [LOOP] client: Server, have the stats changed since 3PM today? server: Yes, here are the new stats. <Client updates that portion of the page> ~OR~ server: No <Client does nothing> [/LOOP] The goal in this type of application is to cut down computation and response time as well as bandwidth.
-
Liam, would it kill you to read the previous posts before responding? I could understand if there were already 20-something replies and you didn't want to wade through them all and accidentally reposted the same thing as someone else. But Jesus H. Christ, repeating a previous post in a thread 4 posts long where each post has an average length of 1 sentence is just silly.