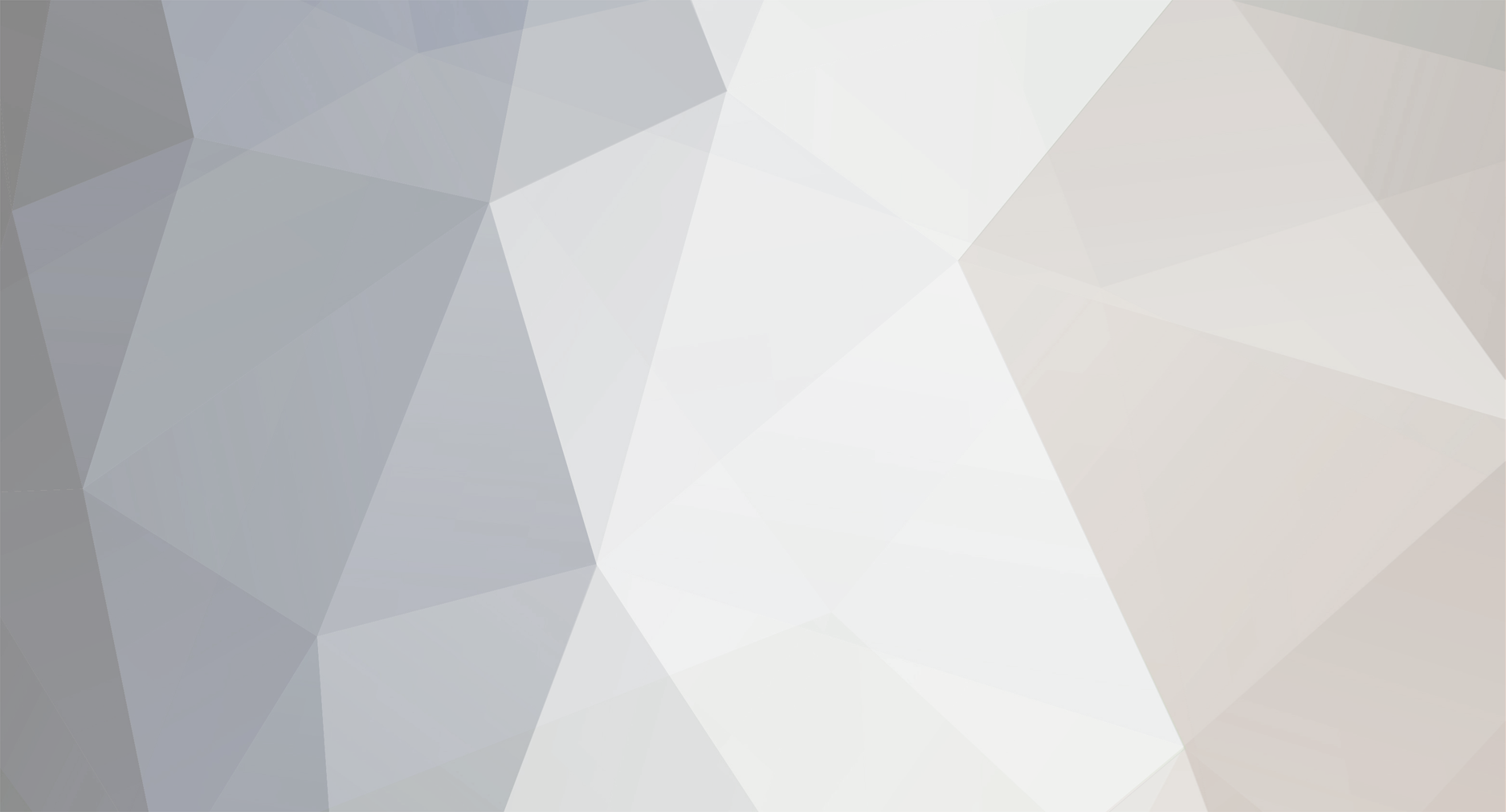
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I wrote myself a small debugging library. It basically gives me three functions: debug_add() : add a debug message to an array debug_echo() : echo a debug message immediately debug_dump() : dump the array populated with debug_add() Everything is dumped inside of pre tags to keep the nice formatting of print_r. Hasn't failed me yet. I started programming with MS Visual products so I always had debuggers with break points, call stacks, and variable watching. However, almost all of my programming in college was done in telnet, those wonderful black and white 80x23 (not sure on the height) windows; in that environment the only debugging tool I ever used was logging or printf statements. After a year and a half of this I finally went into one of the computer labs where everyone was using nice IDEs with debuggers and they still couldn't get their programs to run properly. Yet there I was with my 5 telnet windows chugging away at programs that worked and they looked at me like I was the crazy one. Anyways, if you need a call stack in PHP, look into debug_backtrace(): http://www.php.net/debug_backtrace Combined with echo and pre tags it has everything you need. P.S. I'm not harping on visual debuggers, I think they're great tools. But I've seen too many programmers that don't know how to troubleshoot their programs without one; it makes me a sad panda.
-
Mail Merge with PHP and Microsoft Word
roopurt18 replied to beginneratphp's topic in PHP Coding Help
We have a server application based on Visual FoxPro that performs mail merge with MS Word where I work. My boss recently told me he'd like our clients to be able to export their data to our web site and perform the mail merge there. My boss and the other programmer immediately became engrossed in how to do the actual merge on the server. "Do we have the client upload a word document? How will they know the fields when they're creating it? etc. etc." Then I asked a profound question: "Why do we need to use MS Word?" "So they can format it." "That's it?" "Yah, basically." "Then our problem is solved. We don't need MS Word at all. We can put a rich text control on the server and allow them to create their merge documents right there on the server. We just allow them to save each as a template and when they perform their mailings they can type up a new body or select from an existing template. Simple." -
How to pass session id in URL if and only if cookies are disabled?
roopurt18 replied to Jessica's topic in PHP Coding Help
And all of the URIs are relative? Sorry to ask such mundane questions; I just know how easy it is when frustrated by something to overlook the minor details (that are usually right in front of my face). -
Of course it causes overhead; any sort operation creates overhead. I think what Andy was getting at was exactly how much overhead is it causing you? 1 ms? 4 s? As another solution, if this is a set of data that doesn't change often, you could always run a cron job in the morning to create a table with the rows in the order you want them and just select from there.
-
Is there any better method to validate $result= mysql_query($q1);
roopurt18 replied to sayedsohail's topic in PHP Coding Help
The short answer to your question is you use mysql_affected_rows() just like with UPDATE. The long answer is there is no clear cut way to determine if a query was successful as the definition of success varies. For example, say you run an update query that can potentially update multiple rows. All of the following could be considered successful depending on what you're doing: * The query updated all of the rows expected * The query updated some of the rows expected * The query updated zero rows The same is true with insert. The best thing you could do is follow this link: http://php.net/mysql Scroll down to the area labeled Table of Contents; this is a list of all the mysql_* functions you can use in your program. Read the names and the descriptions and look for code examples. mysql_affected_rows will return -1 if the previous query failed so this check can erroneously report success. // The most general way to test for success if(mysql_affected_rows() >= 0){ } -
Most of the time I just save the data as-is and only perform substitutions when re-displaying it. In either case: $txt = str_replace(";", "<br>", $txt);
-
How to pass session id in URL if and only if cookies are disabled?
roopurt18 replied to Jessica's topic in PHP Coding Help
Just curious if you noticed this in the documentation and made sure your PHP was configured correctly: -
Is there any better method to validate $result= mysql_query($q1);
roopurt18 replied to sayedsohail's topic in PHP Coding Help
If the query is only expected to update a single row, this is fine. But what happens when the update works on more than one row? -
Maybe this will help you: http://www.igvita.com/blog/2007/08/20/pseudo-reverse-indexes-in-mysql/
-
In addition, ronald feels that you should just [moderated] google it! (Sorry to speak on your behalf ron!)
-
[SOLVED] Edit entry and overwrite existing string
roopurt18 replied to FridayRain's topic in PHP Coding Help
Remove the single quotes from around NOW(). -
[SOLVED] Edit entry and overwrite existing string
roopurt18 replied to FridayRain's topic in PHP Coding Help
Insert the new entry and set that column to the value of NOW(). -
[SOLVED] Edit entry and overwrite existing string
roopurt18 replied to FridayRain's topic in PHP Coding Help
From the MySQL documentation: http://dev.mysql.com/doc/refman/5.0/en/update.html UPDATE [LOW_PRIORITY] [iGNORE] tbl_name SET col_name1=expr1 [, col_name2=expr2 ...] [WHERE where_condition] [ORDER BY ...] [LIMIT row_count] (Note the comma!) -
So what you're saying is that the user should have to fill out and submit this form before every page request? A config file is meant to be included(), which means no one is posting to it. You need to learn how to do the following. A) Create a form and process it on the server. B) Create and write to a file in PHP, the functions fopen(), fwrite(), fclose() will be helpful here. C) Combine your knowledge from A and B to process the form and rewrite the existing config.php based on what the user submitted in the form.
-
Wouldn't a proper index help with ORDER BY?
-
Got it. You were on MySQL 4.1.x if I recall, so I'm not sure if you can do this. But assuming you can run sub-queries and use the UNION statement, this should get you everything you need using one call to mysql_query(). SELECT `team`, SUM(IF(`result`='Win',1,0)) AS `wins`, SUM(IF(`result`='Loss',1,0)) AS `losses` FROM ( SELECT `home_team` AS `team`, `home_results` AS `result` FROM `sports_football` WHERE LENGTH(`home_results`)<>0 UNION ALL SELECT `away_team` AS `team`, `away_results` AS `result` FROM `sports_football` WHERE LENGTH(`away_results`)<>0 ) AS `t` GROUP BY `team` ORDER BY `wins` DESC, `team`
-
Due to the table structure I don't think there is any way to do this in a single query. The reason being that you have two separate columns representing teams that we'd like to combine into a single output column. Perhaps there is a way to do that, but I don't know what it is. My solution involves inserting into a temporary table and then selecting from there. I would opt for this solution over one that uses PHP to accomplish this task for a few reasons: 1) It's more portable 2) You can use the database to order the rows, which if combined with indexes will be faster than doing so in PHP 3) It let's the DB engine do the work, which let's your PHP code look cleaner Create the temporary table CREATE TEMPORARY TABLE IF NOT EXISTS tmp_team_records ( `team` VARCHAR(100), `win` TINYINT(1), `loss` TINYINT(1) ) Insert home team results INSERT INTO tmp_team_records (`team`, `win`, `loss`) SELECT `home_team`, IF(`home_results`='Win',1,0), IF(`home_results`='Loss',1,0) FROM `sports_football` WHERE LENGTH(`home_results`)<>0 Insert away team results INSERT INTO tmp_team_records (`team`, `win`, `loss`) SELECT `away_team`, IF(`away_results`='Win',1,0), IF(`away_results`='Loss',1,0) FROM `sports_football` WHERE LENGTH(`away_results`)<>0 Select the data you want SELECT `team`, SUM(`win`) AS `wins`, SUM(`loss`) AS `losses` FROM tmp_team_records GROUP BY `team` ORDER BY `wins` DESC, `team` drop the temporary table, this will occur automatically if you don't do it DROP TEMPORARY TABLE IF EXISTS tmp_team_records That's the best I could do! (edit) I also wanted to point out that while Oldiesmann's solution only uses two queries, it executes one of them for each team, so it could run many times. Also, I think I may have hit another way of possibly doing this with sub-queries. I'll try that out...
-
I see why this is being thrown off. We were assuming that only games that were actually played were present within the table when what you have here is a full schedule. The games that haven't been played yet are also present. I'll play with it for a few minutes and see what I can do.
-
Is it possible for you to use phpMyAdmin to export the table into a .sql file and post that? That would make it easy for me to recreate it on my end and I could play with it myself.
-
You have to change table b to reflect your table. The query I gave you uses the same table twice.
-
Substitute table in the query with the proper table name and tell me how this does: SELECT a.`home_team` AS `team`, SUM(IF(a.`home_results`='Win',1,0)) + SUM(IF(b.`away_results`='Win',1,0)) AS `Wins`, SUM(IF(a.`home_results`='Loss',1,0)) + SUM(IF(b.`away_results`='Loss',1,0)) AS `Losses` FROM table a LEFT JOIN table b ON ( a.home_team=b.away_team ) GROUP BY a.`home_team` ORDER BY `Wins`
-
You use it to access class functions and members statically, where statically means you don't have an instance of the object. However, you have to be careful how you use it. If your class is designed correctly in the first place, you shouldn't be directly setting or reading the values of class properties (variables). These should only be set and read through accessor functions. For example: <?php class a { public $property = null; // Rest of class follows... } $var = new a(); // The next two lines of code are pretty bad practice IMO $a->property = "Hello, World!" echo $a->property; ?> Realistically, the member $property should be private or protected and only settable / readable through methods (functions). Now, let's change our definition of the class slightly above. <?php class a { public static $static_value = null; } // We can access the static value like so a::$static_value = "Hi there!"; echo a::$static_value; ?> (edit) Doh! PHP Does support consts! (/edit) The same examples can be applied to functions within a class definition. You can call a function within a class using the static syntax: my_class::my_function(). You have to be careful using this with class functions though; if the function uses the $this keyword it will not be defined and your code will crash. The reason $this is not defined is simple: $this refers to the instance of the class but you're calling the function without an instance, so there is no $this to refer to! IMO, the best use of calling functions in this manner in PHP is to simulate namespaces, which is a short way of saying it allows you to avoid naming collisions between functions that perform similar functions on different types of data.
-
[SOLVED] POST to mysql database and email results?
roopurt18 replied to theredking's topic in PHP Coding Help
You realize this is a lot like asking, "How can I put on my shoes and tie them too? I can put on my shoe. I can also tie my shoe if it's already on my foot. But I just can't seem to combine them." Basically, you create a form. On the page that processes it you add code that puts it in the database. Then you add more code that emails the results. -
Since playing online games I don't have the attention span to play anything single player. The only single player games I've actually finished since 1998 are: Half Life 1 & 2 Final Fantasy X Hmmm...wow...I think that's all of them. I had fun with lots of games, GTA, Thief, Oblivion (although it's a buggy turd) and some RTS games come to mind; but I can't stomach playing any single player game for more than a week at an hour or two per day. So for the large part I haven't really been into any consoles since 2000 or so. So now you can play console games online but trying to play an FPS with those terrible controllers makes my head implode. Keyboard + Mouse dominates any other form of control where quick reflexes are key, sports games being the only exception. Mario Party 8 on wii is loads of fun but its hard to justify buying a wii just for that. My poisons are FPS, RTS, and MMORP games. In that area, console just doesn't offer anything over PC. On a side note, I've heard a few people here and there bring up the physical activity induced by playing the wii because of it's motion sensor controllers. While I agree that it at least gets people up and moving a bit, playing the wii is hardly a substitute for exercise. Anyone who thinks otherwise is seriously deluding themselves IMO.
-
The only method I know of in MySQL that allows you to order anything is ORDER BY, so I don't really see how you can escape using it if you want to accomplish this in MySQL. You could, however, use a combination of mysqli_data_seek() and mysqli_ num_ rows() to loop over the record set in reverse.