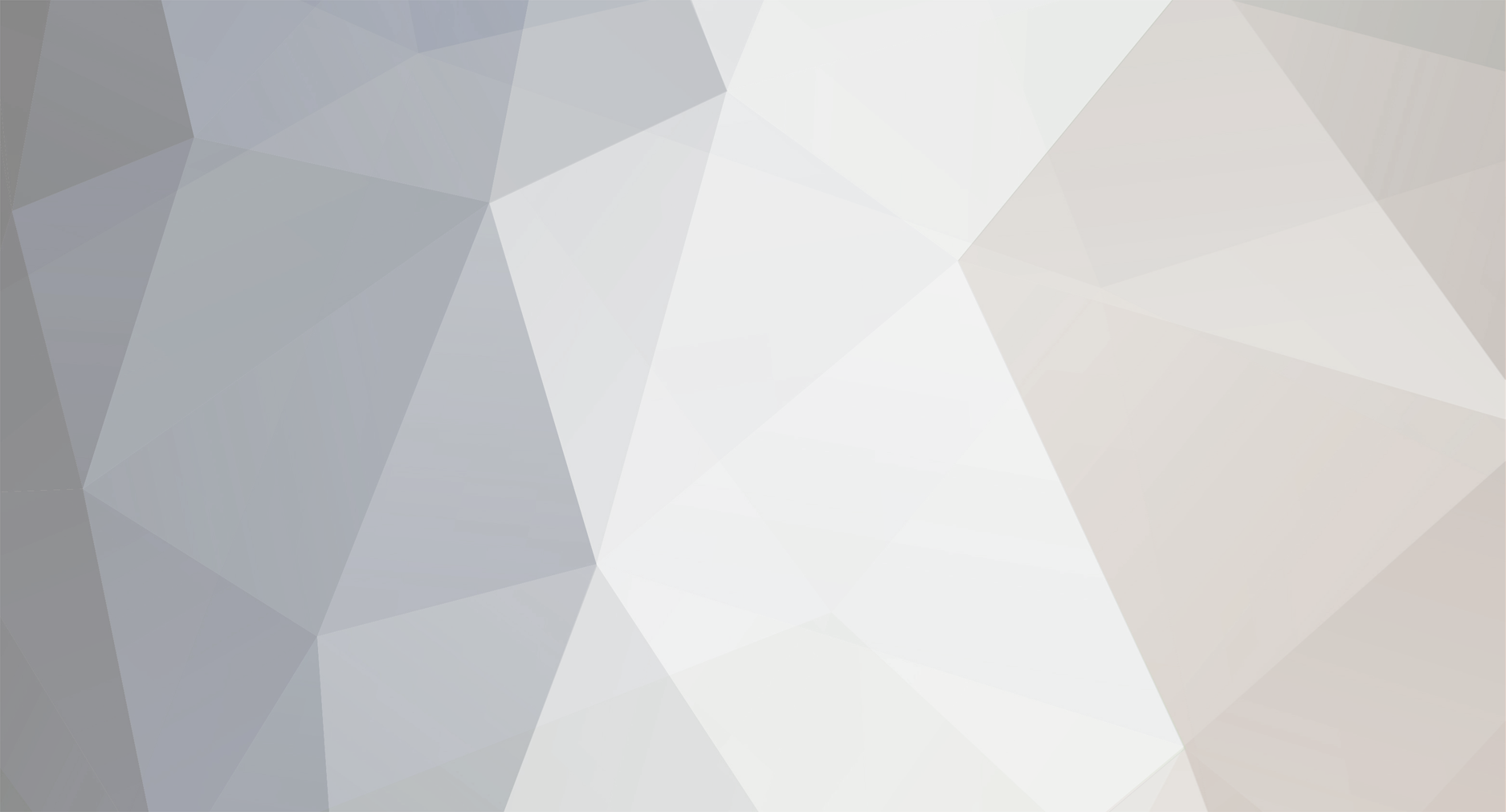
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
If you anticipate working with databases other than MySQL, I would consider a purely abstract base DB class that exposes the most common DB interactions: select, insert, update, delete, etc. If you only need functionality with MySQL databases, then you can skip the abstract class and just create a class exposing those functions: I personally just pass a complete SQL statement into any DB class that I create and use an additional layer to build the SQL statements. For example: <?php class TopicDAO{ // This DAO object would have a member pointing at an instance of the DB class /** * getTopics * Returns an array of topic information. * string $category Topic category */ function getTopics($category = null){ $Clean = Array(); $Clean["Cat"] = $this->m_db->sanitize($category); // If category is set, we will have a WHERE clause $Wheres = Array(); if(isset($category)){ $Wheres[] = "cat={$Clean['Cat']}"; } // Build the SQL $sql = "SELECT * FROM topics"; if(count($Wheres)){ $sql .= " " . implode(" AND ", $Wheres); } $sql .= " ORDER BY name"; return $this->m_db->select($sql); } } ?> Using a system like this it is very easy to convert optional or extra function parameters into part of the WHERE clause. An optional $order parameter could also be specified. The database error checking should occur in the DB class and not the DAO class. This way the application can just request data from DAO objects and not worry about the underlying database structure. If the underlying DB structure or engine is changed, the application code doesn't need to be modified. Just the code within the DAO layer of the affected areas. In my current project, the DB class is really just a wrapper for mysql_query() calls. Each of the functions select(), insert(), update(), etc. just accepts a full SQL statement and performs the proper checking on the value returned by mysql_query() to determine success. Since the application layer shouldn't have any real knowledge about how the underlying database, all of the DAO retrieval operations return arrays. In order to accomplish this, the DB class's select() function has to loop over the returned resource at least once and typically the application will loop over it again. This is fine for small return sets but horribly inefficient for large return sets. On my next project I plan to develop the DB class to return an iterator object that allows iteration over the records as if they were an array but still provide a way for the application layer to not care about what database engine is under the hood.
-
You should not be generating any PHP code on the fly. Research "data driven design."
-
You will want to review fopen(), fread(), fwrite() in the PHP manual, although usually you store information in a database.
-
Using Try...Catch versus nested IF statements
roopurt18 replied to dshevnock's topic in Javascript Help
I think it's more a matter of preference. Try doing it one way and if you like how it looks and it works, great. If it works but you don't like how it looks, try it the other way. I imagine you're doing some sort of form validation prior to submittal to the server and you want to cancel it if the e-mail fields do not match. Here is a simple example: function formSubmit(){ var valid = true; var errs = new Array(); var eml1 = document.getElementById("eml1"); var eml2 = document.getElementById("eml2"); // Force user to enter an e-mail, you said it's optional, so this test doesn't really apply // Note that you should really trim appending / trailing white space as well if(eml1.value.length == 0 || eml2.value.length == 0){ valid = false; errs.push("You must enter an e-mail address."); } // E-mail fields have to be equal if(eml1.value != eml2.value){ valid = false; errs.push("Your e-mail fields must match."); } // More validating... // Now we can check if the form is valid if(!valid){ // Form is not valid, stop form submittal. // Optionally, we can take the text strings in our errs variable and create an // UL element and place it at the top of our form } } -
We run a similar operation where I work. Our clients are able to sync the data from our server software, which is Visual Foxpro and uses dbase, to our MySQL software hosted elsewhere. We built a routine into our Foxpro product that builds a series of DBF files to export, zips them up, and then places them into a client directory on the server where the MySQL DB is located. We have a cron script that runs and looks for new data from our clients and then imports the data. Your #2 approach is closest to what I would do in the ideal world. Which would be to create a script / program on the server with the originating DB that creates a .sql file, zips it, sends it to the destination server, and then just executes mysql from the shell and redirects stdio to come from the .sql file. You already said you encountered a permission error, I'd look into resolving that. Another thing to consider is the sensitivity of this information. As a last note, I wasn't working at my current job when they devised the scheme of importing data from our Foxpro product to the Web product. Depending on what you're doing, it becomes very difficult to keep the same data in sync in two places, especially if it can be modified in either place. The best scenario is one in which you only import from one DB into another a single time and then use the new DB from that point forward. No one from a business stand-point wants to do that because it means abandoning a product for one that may not have as many features. But IMO, the time spent dealing with database sync issues could be better spent developing the new product. But I didn't major in business, so what do I know.
-
Eclipse Editor w/PHP Plugin
roopurt18 replied to per1os's topic in Editor Help (PhpStorm, VS Code, etc)
I recently saw the link to eclipse in your signature in another thread and it prompted me to download and try it since I've also been looking for a good editor to use. I've had small segments of time to look at it here and there but not a lot of time to really dig into it. So far what I love about it is the ability to create projects dependent on each other. I like to think of a website I'm working on as an entire project, but it would be nice to divide it into sub-projects based on functionality of the files contained within and to even have the same file be present in multiple projects. Most editors I've seen don't provide that, or they provide it in a clumsy manner. I would love the auto-completion except for one thing, which I've posted about here: http://www.phpfreaks.com/forums/index.php/topic,149353.0.html What really excites me about eclipse is the potential to find plug-ins for the other types of files I need to work with, such as Javascript, CSS, SQL, etc. -
I would like to configure eclipse so that if the incoming character is from a specific set of characters and the character following the cursor matches the incoming character that it doesn't insert any character but instead moves the cursor ahead one position. For example, if the cursor is in front of a double-quote and I type a double-quote, then I would just like eclipse to move the cursor after the existing double-quote. I only want this to occur for some characters: double-quote, single-quote, square and curly brackets, etc. I use Zend at work and this feature combined with auto-complete is wonderful, but I had to turn off eclipse's auto-complete at home because I don't like moving my hand to the arrow keys. I've been searching for the "magical" editor that does everything I want and I'm hoping eclipse is it. I really like how you can manage your projects, make them dependent on each other, and find plug-ins to add desired functionality. The thing that's killing it the most for me is the topic of this thread.
-
Where would I find a list of the mysql query syntax?
roopurt18 replied to ballhogjoni's topic in PHP Coding Help
You might also try the MySQL manual: http://dev.mysql.com/doc/refman/4.1/en/index.html -
Well, users shouldn't have to change their security settings for it to work. The idea is to make this easy on the users, not more difficult. Well it appears that since the cookie is coming from an iframe and a different domain, IE treats it as a third party cookie and rejects it depending on the security settings. So I found this link which explains how to correctly set up data privacy information on your site, which I think will stop IE from blocking the cookie. http://www.w3.org/P3P/usep3p.html
-
I just had a friend try it with IE7 and he also got in just fine. I went into my IE settings and changed some security stuff and it's working for me now also. I don't know which setting it was yet, but it's partway solved.
-
I'm trying to allow our clients to embed my site into their own home pages via an iframe. I thought the only problem I'd encounter was using Javascript to resize the iframe to be the same height as the content. Once I had that working though, I found a peculiar problem with IE 6 & 7. I have this sample page set up: http://www.rbredlau.com/test/ponderosa.html The login page is the iframe pointing at a different host. When I log in with FF, I have no problems. Everything works as expected. When I log in with IE, the page redirects to the correct login page but for some reason $_SESSION["LoggedIn"] loses it's value and I receive a site-generated message: "You do not have permission to view this page." For the life of me I can't figure this one out. Username: admin Password: admin The page within the iframe can be accessed at: http://ns2271.serverpowered.net/wv/demo
-
Did you try #apache?
-
I refer to online documentation and google on a daily basis which, IMO, is better than someone who doesn't. The 30 seconds I spend in the PHP manual going, "Is there already a function that does this?" is better than the other programmer who spends 10 minutes writing a custom function and then an accumulative hour debugging it over time. (EDIT) I think it was Einstein who inquired why should we remember anything we can write down.
-
My first experience with PHP was creating test cases in a software design class. The class was terribly mismanaged though. Most of us didn't even know we were using PHP. So anyways, we spent the first couple weeks going over test cases we'd come up with, but some groups were having trouble. So I was like "Ef this..." and took off for 6 weeks. I come back, thinking I'm horribly behind and going to fail and the class is still doing test cases.
-
There's no way your proposal was worse than mine.
-
You mean better than the one we already have? You might try IRC.
-
[SOLVED] IE6 blank page problem, running out of hair
roopurt18 replied to roopurt18's topic in PHP Coding Help
Thanks for the heads up JayBachatero, adding the following to an .htaccess within the site seems to clear it up: mod_gzip_on Off However, now nothing in the site will be served with compression. O.o -
[SOLVED] IE6 blank page problem, running out of hair
roopurt18 replied to roopurt18's topic in PHP Coding Help
Well here's whats funny about that. I originally had IE7 on my system and it worked flawlessly. So I've uninstalled back to IE6 to troubleshoot the problem. Now when I originally started working on it I had the same issue. Then I threw an echo statement near the top of my script, which created an error message due to a later call to header(). However, the rest of the page output as expected. I then removed the echo statement and the page continued to work. I had to delete all local files, cookies, etc. in IE6 in order to get the problem to repeat itself. I still have no idea what's causing this and I don't even know if its an IE6 setting / add-on on the local machine or something that's happening on the server. -
[SOLVED] IE6 blank page problem, running out of hair
roopurt18 replied to roopurt18's topic in PHP Coding Help
carterhost & clanstyles, you're both saying you can open the link the first time from the ponderosa website? O.o -
[SOLVED] IE6 blank page problem, running out of hair
roopurt18 replied to roopurt18's topic in PHP Coding Help
<?php // Page.php // Defines the basic outline for all pages created in Webview. // Expects vars: // $Client - An instance of our client object // $Sess - An instance of the session object // $Title - Page title // $CSS - Extra CSS <links> or <style> tags // $Javascript - Extra <script> tags // $Content - Main page content // $NavMenu - The navigation menu // Sets the following vars before displaying markup // $Header - The page header $IFrame = $Sess->isIFrame(); // $Header if(!$IFrame){ $params = Array(); $params['Client'] = $Client; $Header = LoadComponent("General/Header", $params); }else{ $Header = ""; } ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html;charset=utf-8" /> <title>Webview - <?=$Title?></title> <link href="/CSS/layout.css" rel="stylesheet" type="text/css" media="all" /> <link href="/CSS/text.css" rel="stylesheet" type="text/css" media="all" /> <link href="/CSS/nav_menu.css" rel="stylesheet" type="text/css" media="all" /> <link href="/CSS/tooltips.css" rel="stylesheet" type="text/css" media="all" /> <link href="/CSS/form.css" rel="stylesheet" type="text/css" media="all" /> <link href="/CSS/legacy.css" rel="stylesheet" type="text/css" media="all" /> <?=$CSS?> <script src="/JS/Objects/CCookie.js" type="text/javascript"></script> <script src="/JS/Objects/CUserInterface.js" type="text/javascript"></script> <script src="/JS/common.js" type="text/javascript"></script> <script src="/JS/Objects/CNavMenu.js" type="text/javascript"></script> <script src="/JS/ajax.js" type="text/javascript"></script> <script src="/JS/htmlDom.js" type="text/javascript"></script> <script src="/JS/ieSucks.js" type="text/javascript"></script> <script src="/JS/Events.js" type="text/javascript"></script> <script src="/JS/Singletons/MsgQueueSidebar.js" type="text/javascript"></script> <script src="/JS/Singletons/StandardTable.js" type="text/javascript"></script> <?=$Javascript?> </head> <body class="NormTxt"> <!-- HEADER --> <?php if(!$IFrame){ ?> <div id="PageHeader" class="SmallTxt"> <?=$Header?> </div> <?php } ?> <!-- CONTENT --> <table id="PageContent" cellpadding="0" cellspacing="0"> <tr> <td id="ColNav"> <?php if($Sess->isAdmin()){ ?> <div id="MsgQSidebar"></div> <?php } ?> <?=$NavMenu?> <?php if(!$Sess->isAdmin()){ ?> <div id="MsgQSidebar"></div> <?php } ?> </td> <td id="ColDisp"> <input id="btnHideNav" class="button" type="button" value="<<<" style="display: <?=$Sess->isAdmin() ? "" : "none"?>;" onclick="window.wvNavMenu.toggle();" /> <?=$Maintenance?> <?=$Content?> <?=rDebug_dump(false)?> </td> </tr> </table> <!-- FOOTER --> <?php if(!$IFrame){ ?> <div id="PageFooter" class="TinyTxt"> Copyright © 2002 - 2006 Pharaoh Information Services. All rights reserved. </div> <?php } ?> </body> </html> I did some testing where I echoed some text if the remote address stored in $_SERVER was equal to my IP. I placed several echo statements directly before the ?>. if($_SERVER["..."] == "my ip"){ echo "component: Page.php<br/>"; echo "<pre>" . print_r(htmlentities($Client), true) . "</pre>"; echo "<pre>" . print_r(htmlentities($Sess), true) . "</pre>"; // ... for each of the variables expected by the page } ?> Whats weird is all of a sudden the page worked. But if I commented out all of the echo statements except the "component: Page.php" one, I'd get a blank page with just that text on it. -
A page on a client's website, which is not managed by me: http://www.ponderosahomes.com/buy/optionspreview.php At the bottom of this page is a link to a site I do manage: In order to access the site, click here. When clicking on this link in IE6, a new window opens and displays a blank page. Refreshing the page will cause the proper login screen to appear. This behavior appears to only occur in IE6. I have been working on this for about 6 hours now and becoming somewhat frustrated. I have tried numerous solutions on a test machine which entail reinstalling IE6, using regsrvr32 to re-register DLLs, etc. I have added calls to header() on the server to no avail. Any suggestions?
-
I wasn't sure if this was the best place for this thread, so please move it to the appropriate area if necessary. I manage a site using a PHP / MySQL back end that tracks user authentication via sessions, nothing magical about that. A design company is creating a new website for one of our clients and they'd like to have access to our user database so that the mutual client's users would be able to use the same user authentication on each site. In addition, they'd like to provide the functionality that if a user logs into the new website and clicks the link to our website that they'd enter our website as already authenticated. I'm reluctant to provide any outside access to our database, although in reality I'd only be granting "SELECT" access on a single table for a user that had to come from a specific host, which is easy enough. It's the second part about passing authentication from one server to the next that confuses me. Is this even possible? I've thought about it a little and can think of one, maybe two ways in which it might be accomplished, but they both seem unlikely.
-
Congrats and remember there's no luck, it's all skill!
-
You are taking raw data directly from $_POST and $_GET and inserting it into your database. $update = "UPDATE guestbook SET actual_time='".$_POST['actual_time'] . "..."; What happens if I enter the following in your actual_time field: NULL; DELETE * FROM guestbook WHERE 1; Your SQL query then becomes: UPDATE guestbook SET actual_time=NULL; DELETE * FROM guestbook WHERE 1; It would take some experimenting and knowledge about your site to get that far, but careless programming makes that kind of information easy to retrieve.