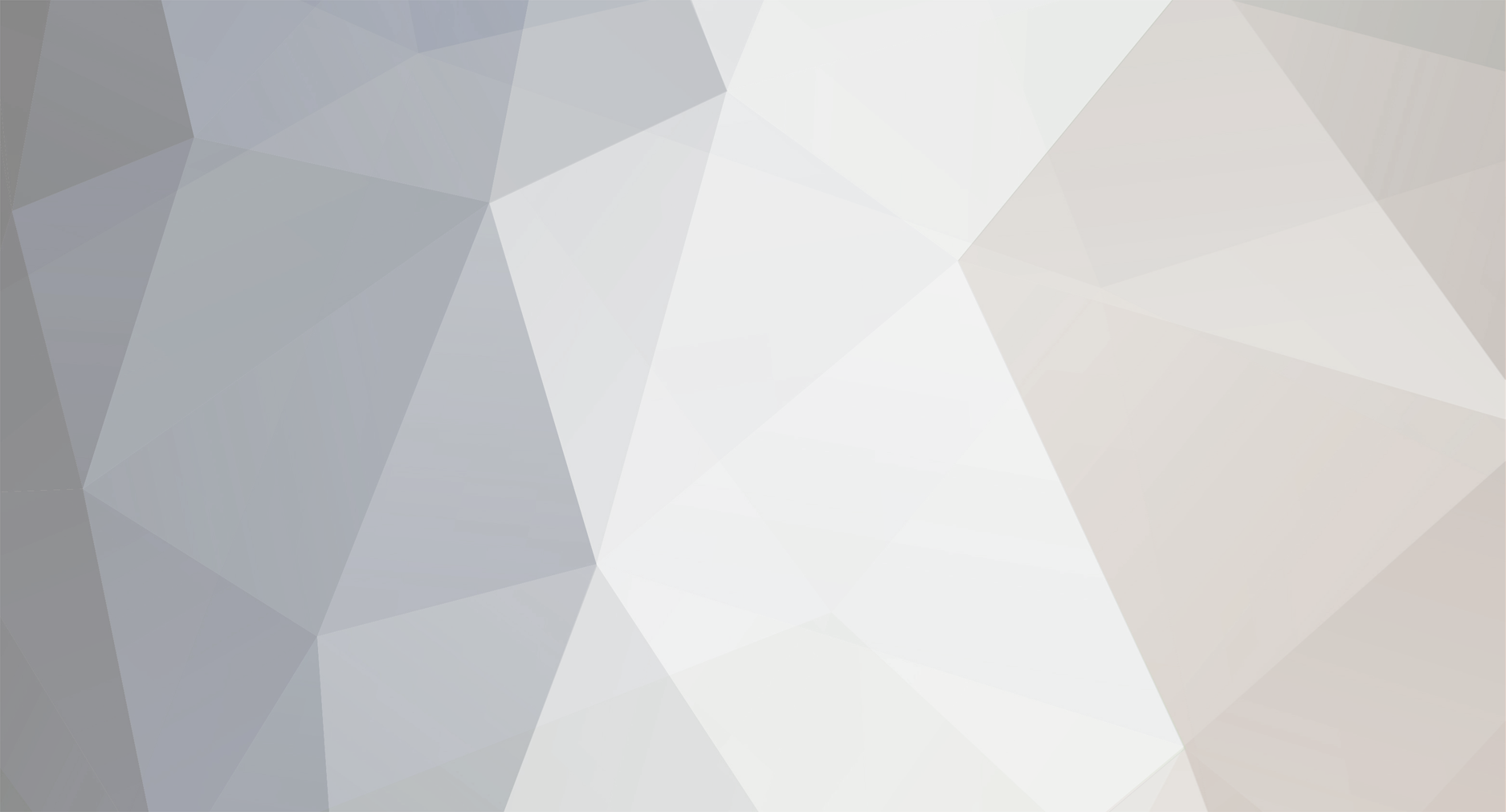
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
http://dev.mysql.com/doc/refman/4.1/en/date-and-time-functions.html#function_date-add
-
Does this help: http://www.php.net/shuffle
-
I'm going to guess that your problem stems from the line: if( $rank = 1 ){ Someone else had a problem constructing an if statement and I jumped in with a lengthy explanation. Rather than retype it, here is a link to the thread: http://www.phpfreaks.com/forums/index.php/topic,110618.0.html Note the part where I talk about the difference between = and ==
-
For those unfamiliar with the concept, if you have only a single PC and want to test IE6 and IE7 concurrently, you can do so with a virtual machine. It's a little slower but definitely cheaper than putting up a second PC. I use VMWare.
-
iframe and parent, how can I "refresh" the parent
roopurt18 replied to weemikey's topic in Javascript Help
Keep in mind that if an iframe refreshes it's container the iframe will itself be refreshed and likely take you back to the first page of the iframe. To prevent this, you would likely have to host the top and side bars in frames with borders turned off and have the content iframe refresh the menu and top iframes. I think you're better off accomplishing this first on the server-side with PHP as Karl33to suggested; any solution that requires Javascript is guaranteed to break as soon as someone browses your site with Javascript turned off. -
Your other option would be to use a throttle. In the onkeyup event, set a timer to a lambda function that does the actual work with a timeout of 500ms, or half a second. On each keyup event, cancel the timer if it is already present. Essentially what you'd be doing is on every keyup telling JS to run a function half a second from now, but the only way it'd actually run is if the user stopped typing for at least half a second.
-
Here's the PHP side of it. <?php // We have two sections of the final output that are dynamic and depend on // the contents of our database. We will build each of those sections in // this top portion and then insert them into the final output. $Options = Array(); // The select item's options $JS_OLN = Array(); // The Javascript object literal notations while($row = mysql_fetch_array($result)){ // $row will have the following keys: // id, itemname, UPC, instock, price $Options[] = "<option value=\"{$row['id']}\">{$row['itemname']}</option>"; $instock = $row['instock'] ? "true" : "false"; $JS_OLN[] = "window.mysqlData.rec_{$row['id']} = {" . "id : {$row['id']}, " . "name : \"{$row['itemname']}\", " . "upc : \"{$row['UPC']}\", " . "inStock : {$instock}, " . "price : {$row['price']} " . "};"; } ?> <script type="text/javascript"> window.mysqlData = new Object(); // Create a generic object // Now we want to create a property in our mysqlData object for each record in // the mysql database. We will use object literal notation because it's cool, // convenient, and allows us to work with named values rather than integer-based // array indexes. <?=implode("\n", $JS_OLN)?> function changeItems(oEvent){ // This is our event handler for the select box's onChange event. oEvent = oEvent || window.event; // Distinguish between DOM L2 or IE event models var oNode = oEvent.srcElement || oEvent.target; // DOM Node that triggered event // First we retrieve the value of the selected item var selVal = oNode.options[oNode.selectedIndex].value; // Now we access the corresponding property in window.mysqlData, remember to // add the 'rec_' prefix var obj = window.mysqlData["rec_" + selVal]; // Now we can access the object any way we desire, you should modify this to use // the data however it is you intend alert( "id: " + obj.id + "\n" + "name: " + obj.name + "\n" + "upc: " + obj.upc + "\n" + "inStock: " + obj.inStock + "\n" + "price: " + obj.price + "\n" ); } </script> <select id="selItems" name="selItem"> <?=implode("\n", $Options)?> </select> <script type="text/javascript"> // Add an onchange event handler to our select control try{ var el = document.getElementById("selItems"); if(document.addEventListener){ // DOM L2 method el.addEventListener("change", changeItems, false); }else if(document.attachEvent){ // IE method el.attachEvent("onchange", changeItems); } }catch(e){ alert("Error: Old browser!"); } </script>
-
This topic is an overlap of MySQL and design so I'm placing it here, feel free to move it to the MySQL board if it's more appropriate there. Following is a bit of setup to the problem, if you want to jump directly to the problem scroll down to the section labeled "The point of this thread." The software I develop allows our clients to build a survey that captures vital and demographic data. Vital data is that data of interest to all of our clients, items such as first and last names, address information, contact information, etc. Demographic data are those types of questions that are multiple choice, open-ended responses, etc. that differ from client to client. Since there's no way for me to predict which questions our clients would want to include on their surveys, I built an interface where they can create their own questions. <Side note> I have a beta environment set up where you can test this if you're curious. The login credentials are beta1 / beta1; there is a top-left menu labeled InterestView. The sub-menu Demographic Setup allows users to build their demographic questions; the sub-menu Preview allows a preview of the entire survey as it would appear to users. URL: http://www.ibswebview.com/wv/beta/admin </Side note> iview_demo_setup id int Demographic question ID order int Display order of the question; i.e. 1 means first question, 2 means second question, etc. setup text Serialized PHP array that contains the question setup; see note below. <note> There are 5 basic question types that can be created; consistent to all of them are the prompt, type, extras and possibly a few more fields. extras is itself an associative array whose members depends on the question's type. For instance, extras in multiple choice questions includes details about the choices while in a rating scale question it includes details about the ratings to display. I chose to store the questions' setup parameters as serialized arrays because it facilitates easier expansion of question types and it is unlikely I will have to run queries such as "Which questions are multiple choice and include 'Apples' as a choice?" Which is to say I see a great deal of complexity in trying to represent a demographic question's setup with multiple DB tables and very, very little gain. </note iview_response id int Response ID entered datetime Timestamp of when response was received exported tinyint 1 if exported to our server software, 0 otherwise For each response submitted through the form, a master record is created in iview_response. Currently, only the vital information (first name, last name, address, phone, e-mail, etc.) is being saved (elsewhere in the database). The point of this thread: I now need to store the answers to the demographic questions in the database and I will later need to perform statistical analysis on the responses. My initial thoughts are the following table design: iview_demo_short_ans - Used for short answers, i.e. multiple choice & rating scale responseID int Which response record this answer belongs to questionID int Which question this answer belongs to answer varchar() The user's answer iview_demo_long_ans - Used for long answers, i.e. open-ended text questions responseID int Which response record this answer belongs to questionID int which question this answer belongs to answer text The user's answer For multiple choice and rating scale questions, likely statistics would be frequency of choices or for people who answered question X as A, how did they answer question Y? For the open-ended text responses, I'm thinking some sort of word frequency statistics. For example, how many responses contained words associated with dissatisfaction, a positive experience, etc. I'm curious if anyone else has dealt with this scenario, if you think I'm headed in the correct direction, or any alternative ideas that can be thought of.
-
Your question is a little unclear so I'm going to try and restate it, let me know if this is correct: You want a method of passing data in a MySQL database into Javascript. You then want to display a drop down list and when the user selects an item from the list you want to choose the corresponding data you sent from MySQL. Brief Tutorial // Creating a global variable in Javascript, I use window. to specify this specifically as global window.someVar = 5; // Now there exists a global variable named someVar // Creating a generic object window.someObj = new Object(); // Now there exists a global object named someObj, but it // has no properties or methods // Creating some properties in our object window.someObj.aProperty = "Hello, World!"; window.someObj.bProperty = "Goodbye, World!"; // Displaying those values alert(window.someObj.aProperty); // Popup displays: "Hello, World!" alert(window.someObj.bProperty); // Popup displays: "Goodbye, World!" // When displaying the properties above, we explicitly stated (in code) which // property to display. We can also access a property through a variable. var tmp = "aProperty"; // This is the property name alert(window.someObj[tmp]); // equivalent to: alert(window.someObj.aProperty); tmp = "bProperty"; // This is the property name alert(window.someObj[tmp]); // equivalent to: alert(window.someObj.bProperty); // Last but not least is "Object Literal Notation," search this on google for a more // in-depth explanation. Briefly, we can enclose an object definition in curly braces { }, // in the curly braces are comma-separated property-value pairs. window.literalObj = { // <-- Starts object literal notation aProperty : "Hello, World!", // <-- a property-value pair bProperty : "Goodbye, World!" // <-- another property-value pair }; <-- Ends object literal notation // We can access this object just like the first one alert(window.literalObj.aProperty); // Popup with "Hello, World!" Understand the above before proceeding; once you do, we can create an outline of what our finished product will be: <-- This is a layout of the final HTML we want output to the browser --> <script type="text/javascript"> window.mysqlData = new Object(); // Create a generic object // Now we want to create a property in our mysqlData object for each record in // the mysql database. We will use object literal notation because it's cool, // convenient, and allows us to work with named values rather than integer-based // array indexes. window.mysqlData.rec_1 = { id : 1, name : "Peanut Butter", upc : "SomeString", inStock : true, price : 1.49 }; window.mysqlData.rec_2 = { id : 2, name : "Jelly", upc : "SomeString", inStock : false, price : 1.69 }; function changeItems(oEvent){ // This is our event handler for the select box's onChange event. oEvent = oEvent || window.event; // Distinguish between DOM L2 or IE event models var oNode = oEvent.srcElement || oEvent.target; // DOM Node that triggered event // First we retrieve the value of the selected item var selVal = oNode.options[oNode.selectedIndex].value; // Now we access the corresponding property in window.mysqlData, remember to // add the 'rec_' prefix var obj = window.mysqlData["rec_" + selVal]; // Now we can access the object any way we desire, you should modify this to use // the data however it is you intend alert( "id: " + obj.id + "\n" + "name: " + obj.name + "\n" + "upc: " + obj.upc + "\n" + "inStock: " + obj.inStock + "\n" + "price: " + obj.price + "\n" ); } </script> <select id="selItems" name="selItem"> <option value="1">Peanut Butter</option> <option value="2">Jelly</option> </select> <script type="text/javascript"> // Add an onchange event handler to our select control try{ var el = document.getElementById("selItems"); if(document.addEventListener){ // DOM L2 method el.addEventListener("change", changeItems, false); }else if(document.attachEvent){ // IE method el.attachEvent("onchange", changeItems); } }catch(e){ alert("Error: Old browser!"); } </script> The above code is fairly straightforward once you become accustomed to it, but it's a lot to take at a single sitting. I'm going to make a follow up post to help with the PHP side of it shortly.
-
I wrote a quick my_round function that seems to work fairly well. <html> <head> <title>Javascript Round Function</title> <script type="text/javascript"> function my_round(num, dec){ dec = dec === undefined ? 2 : dec; if(typeof num == "number" && typeof dec == "number"){ return num.toFixed(dec); } return Number.NaN; } </script> </head> <body> <script type="text/javascript"> alert(my_round(5)); alert(my_round(21.15, 7)); alert(my_round(60.80, 10)); alert(my_round(21.15 + 60.80,); </script> </body> </html>
-
For humans this is true, but for computers it's not always the case. The problem arises in that there are a limited number of bits to represent any value in a computer but there are infinite floating point numbers. For example, there are infinite values between 0.00001 and 0.00002. However, most computers only have 32 or 64 bits to represent all of those possible values. Thus, floating point numbers in computers are almost always approximations to their real values and you can almost never count on them being exact. Taking our example from this thread, if we do: 21.15 + 60.80 = 81.95 it is fairly straight-forward. However, if we try the following in a computer: if( (21.15 + 60.80) == 81.95 ){ alert("true"); } We may not see an alert box! This is because either of the two operands may be an approximation to the real value, which means the computer might actually be performing: 21.149999999999999 + 60.79999999999999 or something similar. If you want to use floating point numbers in comparisons that are not equivalent to integers (i.e. numbers that are not equivalent to 0.00, 1.00, 2.00), you might have to do something like this: var aFloat = 21.15 + 60.80; if( aFloat >= 81.94 && aFloat <= 81.96 ){ alert("true"); } You can change the values 81.94 and 81.96 to be more precise if necessary. The thing to remember is that when performing floating point calculations you are almost always dealing with approximations and not exact numbers.
-
I wanted to pop back in and explain what I did and mark this as solved just in case someone runs into a similar issue in the future. I created a page with a form that has two fields. One field is a drop down of the possible columns in the table, such as fname, lname, addr1, etc; The drop down displays user friendly descriptions but maps to those values behind the scenes. The other field is a drop down with the choices "Exact" or "Sounds Like." Each time this form is submitted it runs the query, which I'll talk about in a minute, but then redisplays itself with two new drop downs to select another field-match type combination. So for example, when first presented with the form I can specify to find duplicates where the states are exact matches. I can then further clarify that I want cities that are exact matches. Lastly, I can add a filter where the last names or phone numbers or whatever sound similar. I've tested this on data generated by the data generator in the sticky on this board and some manual entries as well; it seems to work well but it hasn't faced any real-world scenarios yet. As for the query itself, I'm actually running quite a few. As I stated before, I have three tables: iview_response, iview_vitals, iview_emails. iview_response is the master record created whenever a user submits a form. iview_vitals contains information such as first name, last name, address, and contact information. I chose to store the emails in a separate table with fields "local_part" and "domain" so that users could enter multiple e-mail addresses per response. The query suggested by Barand is fairly straight forward when the results are stored in a single table but becomes more complicated with my table setup. So here is what I do with the database: 1) Create a temporary table based off of iview_vitals. 2) Alter the temp table and add an "email" column with type TINYTEXT, since an e-mail can be longer than 255 characters. 3) Do an INSERT ... SELECT into the temp table, selecting from iview_vitals and iview_emails. In this step, the e-mail is converted back into a "local_part@domain" form. However, if someone enters multiple e-mail addresses, the temp table will have duplicate rows where only the "email" field differs; we must make a note of this. 4) Add a FULLTEXT index on the email column in the temp table. The query we intend to run has to include the same table twice; however, temporary tables can appear only a single time in a query, so we... 5) Create a second temp table and immediately select from the first temp table; now we have two identical temporary tables. 6) Run a form of the query suggested by Barand. It's important to note that we do not want to compare the same record to itself, which would always report a duplicate. We also only want to compare the records in a single direction, i.e. once we compare record 1 to record 2, we don't need to compare record 2 to record 1. This is why Barand included: WHERE a.id < b.id in his query. My temp tables contain a column "response_id" which is the master record the vitals / emails belong to. I stated earlier we had to take note that the temp table would have duplicate rows where users entered multiple e-mail addresses. To ensure that records are compared in a single direction and that vitals belonging to the same response weren't compared with each other, I changed my WHERE part to be: WHERE a.response_id < b.response_id I seeded my tables with 200 records, which is far less than most of our clients will have for quite some time as far as I can tell, and it runs quickly. I'm hoping it scales.
-
Thanks! I'll get to try that out on Monday.
-
You're stuck in a very common problem, which is to generate a multi-page form. The form has to be created by the server but you can't create the entire form without some initial input from the user. Each time a form is submitted you can access the user's responses in PHP via $_POST. All HTML form elements have a way of specifying a default value. So this should really be a no-brainer. 1) You display a form to the user. 2) The user fills out some info and submits 3) You re-display the form to the user, adding additional fields, and set the default values of the previously entered fields using what was in $_POST from the previous submition 4) You continue in this fashion until all the information is supplied <?php // Display a form in three steps // Check if form is submitted and we have everything if(isset($_POST["field1"]) && isset($_POST["field2"]) && isset($_POST["field2"]){ // All three exist, so we process the form and then exit ProcessForm(); exit(); } // Get defaults $field1 = isset($_POST["field1"]) ? $_POST["field1"] : null; $field2 = isset($_POST["field2"]) ? $_POST["field2"] : null; $field3 = isset($_POST["field3"]) ? $_POST["field3"] : null; ?> <form method="post" action=""> <input type="text" name="field1" value="<?=$field1?>" /> <?php if($field1 !== null){ ?> <input type="text" name="field2" value="<?=$field2?>" /> <?php if($field2 !== null){ ?> <input type="text" name="field3" value="<?=$field3?>" /> <?php } ?> <?php } ?> <input type="submit" name="submit" value="Submit" /> </form>
-
If you have short-tags enabled: <?php $price1 = "150.00" ; ?> <script> var price=<?=$price1?>; </script>
-
You need to add a hidden span to the document containing the text you'd like to pop up. Use CSS to style the span so that it has an absolute position and give it a width, height, font-color, border, background color, etc. Then you add event handlers to the onmouseout and onmouseover for the image. The onmouseover handler should display and position the span; the onmouseout should hide the span.
-
validating multiple file input elements
roopurt18 replied to horseatingweeds's topic in Javascript Help
In your input_formCheck.js: // ... }else if (form.fileImg1.value.length > 0){ alert(''); }else{ returnValue = true; } Perhaps if you actually checked the values of form.fileImg2.value.length and form.fileImg3.value.length you could validate them. Also, I noticed you're looking at file extensions to determine MIME type; that's considered bad practice. -
I have the following table structure for a web form that captures user information: CREATE TABLE IF NOT EXISTS `iview_response`( `id` INT NOT NULL AUTO_INCREMENT PRIMARY KEY, `entered` DATETIME NOT NULL, `exported` TINYINT(1) NOT NULL DEFAULT 0 ); CREATE TABLE IF NOT EXISTS `iview_vitals`( `id` INT NOT NULL AUTO_INCREMENT PRIMARY KEY, `response` INT NOT NULL DEFAULT 0, `fname` VARCHAR(24) NOT NULL DEFAULT '', `lname` VARCHAR(24) NOT NULL DEFAULT '', `addr1` VARCHAR(32) NOT NULL DEFAULT '', `addr2` VARCHAR(32) NOT NULL DEFAULT '', `city` VARCHAR(32) NOT NULL DEFAULT '', `state` VARCHAR(2) NOT NULL DEFAULT '', `zip` VARCHAR(5) NOT NULL DEFAULT '', `hphone` VARCHAR(17) NOT NULL DEFAULT '', `wphone` VARCHAR(17) NOT NULL DEFAULT '', `cphone` VARCHAR(17) NOT NULL DEFAULT '', `fax` VARCHAR(17) NOT NULL DEFAULT '' ); CREATE TABLE IF NOT EXISTS `iview_emails`( `id` INT NOT NULL AUTO_INCREMENT PRIMARY KEY, `response` INT NOT NULL DEFAULT 0, `lpart` VARCHAR(128) NOT NULL DEFAULT '', `domain` VARCHAR(255) NOT NULL DEFAULT '', UNIQUE( `response`, `lpart`, `domain` ) ); iview_emails exists as a separate table to allow people to enter as many e-mail addresses as they'd like when filling out a form. These three tables are intended to capture marketing information so users can potentially fill out the form multiple times. I can't place any unique constraints on the fields because marketing being marketing, they don't want to reject any information. So I have to build a "deduping" report that displays similar records that scales relatively well. Anyone have any recommendations on the best way to check for similar, but not exact, records within a table?
-
There's nothing automatic that I know of to help you with this. Usually I just use the DOM explorer in the Web Developer toolbar for Firefox.
-
I grew up fishing at a local lake that they stock with trout every winter and already has a population of bass, sunfish, catfish, bluegill, etc. I taught my fiance how to fish and she loves it because she's Filipino. She performs all the gruesome stuff herself too, such as getting the hook out of their stomach and cleaning them. It's been a long time since we've gone. Also, I've never been ocean fishing; maybe some day!
-
When I wrote my first site a friend recommended Web Host Freaks so I signed up there. That site is still up and still hosted by WHF, although I no longer manage it. I do host my personal site (in my sig) with them. For both of those sites I never had much need for technical support but up-time has always been good. For work we needed more control over our server so I moved us to a VPS with Server Powered and the uptime and service has been great. I actually developed in our live site for quite some time before I convinced my boss it was time to have a development server; when it came time to set one up I went with Server Powered again. Never had a problem with them. Unless an unfortunate incident arises or they just don't fit the requirement, I plan to use them for any and all of my hosting.
-
I predict that office productivity is about to shoot through the roof.
-
OOP sounds incredibly simple until you actually try to apply it and this is the step that you're on now. You know what objects are, you have some vague ideas about how it may be useful, but then you sit down to write code and you don't know what should be an object and what shouldn't. Unfortunately, this part of OOP is something people learn through experience. There are no set rules into when you should develop classes, when you should make a base class, etc. It's something you develop a "feeling" for over time. My advice is to just try and write anything and do it with objects. Evaluate how you did when you finish. You may realize halfway through that if you had create Base Class A it would have saved you a lot of typing; you may also realize that the Base Class B you made isn't really all that useful. A great mini-project to help with OOP is to create a dungeon sort of game where you travel through rooms, gather items, and encounter monsters.