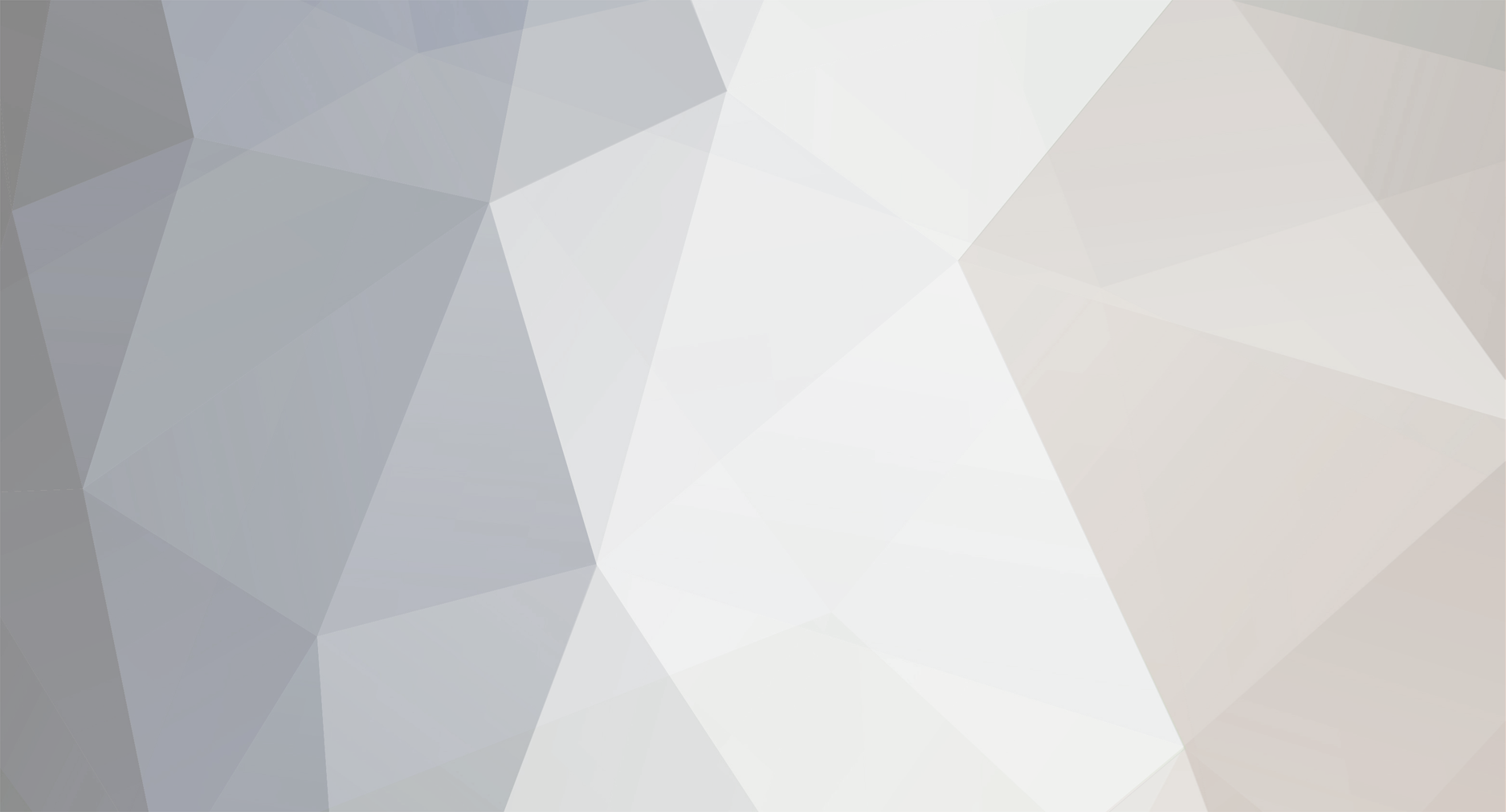
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
Make sure you dont have another element with the id "submitForm", the form you posted seems to work just as excepected.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <form action='index.php' method='post' name='submitForm' id='submitForm'> <select name='campaign' id='campaign' onchange="javascript: document.getElementById('submitForm').submit();"> <option value='1'>1</option> <option value='2'>2</option> </select> </form> </body> </html>
-
define the x outside the function
var x=0; function Update() { document.write(x); x++; clockID = setTimeout("Update()", 100); }
-
better option is to make a function to get the value, so you dont have to worry about variable scope or page load
function getVale(obj){ return document.form[obj].value; } function checkForm(){ // first checks that required fields aren't empty if((getVale('f_name')=="") ............ }
Not tested
-
-
We, first make sure you use parseInt for the value so JavaScript covert it from a string into a integer.
Here is an example using switch (but you can use if as well)
<html> <head> <title>Kayak Wars Submission Form</title> <script type="text/javascript"> function calc_points(){ var val = parseInt(document.getElementById("species").value); var species1 = 0; switch (val) { case 0: species1 = 0; break; case 1: case 2: case 5: case 10: species1 = 10; break; case 3: case 4: case 6: case 8: case 12: species1 = 20; break; case 7: case 9: species1 = 40; break; case 11: case 13: case 14: case 16: species1 = 50; break; case 15: case 17: case 18: case 19: species1 = 100; break; case 20: species1 = 200; break; default: species1 = 0; } var total_points = (species1 * parseInt(document.getElementById('quantity').value)); document.getElementById("points").value = total_points; } </script> </head> <body> <form id="form1" name="form1" method="POST" action=""> <input type="hidden" name="submit_id" value="NULL"> <select name="species" id="species"> <option value="0">Select Species</option> <option value="1">Red Drum</option> <option value="2">Trout</option> <option value="3">Snook</option> <option value="4">Shark</option> <option value="5">Black Drum</option> <option value="6">Snapper</option> <option value="7">King Mackerel</option> <option value="8">Grouper</option> <option value="9">Cobia (Ling)</option> <option value="10">Flounder</option> <option value="11">Tarpon</option> <option value="12">Tripletail</option> <option value="13">Permit</option> <option value="14">Blackfin Tuna</option> <option value="15">Yellowfin Tuna</option> <option value="16">Barracuda</option> <option value="17">Bonefish</option> <option value="18">Dorado</option> <option value="19">Wahoo</option> <option value="20">Billfish</option> </select> <span class="style2"> <label>Quantity</label> </span> <label> <select name="quantity" id="quantity" onChange="calc_points();"> <option value="0">Select Quantity</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> <option value="9">9</option> <option value="10">10</option> <option value="11">11</option> <option value="12">12</option> <option value="13">13</option> <option value="14">14</option> <option value="15">15</option> </select> </label> <span class="style2"> <label>Total Points</label> </span> <label> <input name="points" id="points"> </body> </html>
-
-
You can't access the document element until the page has loaded, If you put this before the </body> tag it should work.
-
add this function to your main window
function removeWin(user_ID){ delete wins[user_ID]; }
and on the opended window, add an onunload event
<body onunload="opener.removeWin(user_ID);"...
Just make sure to use the same user_ID
Not tested. Both pages has to be in the same domain
-
Can you try this and see if it works?
<img src='http://www.mysite.com/image.jpg'width="45" onclick="javascript:window.open('http://www.google.com','','width=210,height=210,toolbar=no,status=no,scrollbars=no,resizable=no,menubar=no,location=no,direction=no');" style="cursor:pointer">
-
Try to avoid eval (search for "eval is evil")
You can do the same with using a simple JS object
<script language="javascript"> var wins = {}; function open_win(user_ID){ if (!wins[user_ID]){ wins[user_ID] = window.open("http://www.google.com", "", ""); } } </script> <input type="button" onclick="open_win(2);" value="Open" />
-
Just make sure the window name is vaild "$v.option[$ke].option_name" If you don't have one, just remove it and it should work.
-
Here is a small script that will do something similar, you just need to fine tune it.
<script language="javascript"> var base_val = 100; var opts = {}; opts.Memory =0; opts.Size = 0; opts.Warranty = 0; function addValues(){ var total = base_val; for (p in opts){ total += opts[p]; } document.getElementById('total').innerHTML = total; } function updateOptions(opt, value){ opts[opt] = value; addValues(); } </script> Memory: <select onchange="if (this.value == '256 MB') updateOptions('Memory', 0); else if (this.value == '512 MB') updateOptions('Memory', 50); else updateOptions('Memory', 100);"> <option value="256 MB">256 MB</option> <option value="512 MB">512 MB</option> <option value="1024 MB">1024 MB</option> </select> <br /> Memory: <select onchange="if (this.value == '50 GB') updateOptions('Size', 0); else if (this.value == '100 GB') updateOptions('Size', 50); else updateOptions('Size', 100);"> <option value="50 GB">50 GB</option> <option value="100 GB">100 GB</option> <option value="150 GB">150 GB</option> </select> <br /> Warranty: <input type="checkbox" onclick="if (this.checked) updateOptions('Warranty', 200); else updateOptions('Warranty', 0);" /> <div id="total" style="font-weight:bold;">100</div>
-
change the "return true" to "return false" on your onclick event, otherwise the page will reload
-
Can post the output HTML, this is the smarty code and it's not clear what's your output will look like. Just visit your page and right click anywhere empty and select "view source"
-
the event should have an object to hold the shift key status
event.shiftKey
event.shiftLeft
http://msdn2.microsoft.com/en-us/library/ms536939(VS.85).aspx
-
You better use some math and loops for making the options. Writing a gaint select menu with innerHTML is impractical.
Here is a full working version
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <script language="javascript"> function daysInMonth(mnt, yr){ var mnt = mnt-1; var dt = new Date(yr, mnt, 28); for (i=28; i<=32; i++){ dt.setDate(i); if (mnt != dt.getMonth()){ return i-1; } } } function popSelectMenus(dt, mn, yr){ var st_yr = 1990; var en_yr = 2010; var sel = document.getElementById('year'); sel.innerHTML = ""; sel.onchange = function(){updateDates(document.getElementById('date').value);}; var opt = document.createElement('option'); opt.innerHTML = "Year"; sel.appendChild(opt); for(i=st_yr; i<=en_yr; i++){ opt = document.createElement("option"); opt.value = i; opt.innerHTML = i; if (i == yr) opt.selected = "selected"; sel.appendChild(opt); } sel = document.getElementById('month'); sel.innerHTML = ""; sel.onchange = function(){updateDates(document.getElementById('date').value);}; opt = document.createElement('option'); opt.innerHTML = "Month"; sel.appendChild(opt); for(i=1; i<=12; i++){ opt = document.createElement("option"); opt.value = i; opt.innerHTML = i; if (i == mn) opt.selected = "selected"; sel.appendChild(opt); } updateDates(dt); } function updateDates(dt){ var mn = document.getElementById('month').value; var yr = document.getElementById('year').value; if (!dt) var dt = document.getElementById('date').value; if (mn == "") days = 31; else if(yr == "") days = daysInMonth(mn, new Date().getFullYear()); else days = daysInMonth(mn, yr); var sel = document.getElementById('date'); sel.innerHTML = ""; var opt = document.createElement('option'); opt.innerHTML = "Date"; sel.appendChild(opt); for(i=1; i<=days; i++){ opt = document.createElement("option"); opt.value = i; opt.innerHTML = i; if (i == dt) opt.selected="selected"; sel.appendChild(opt); } } </script> </head> <body onload="popSelectMenus(5, 8, 2002);"> <select name="date" id="date"></select> <select name="month" id="month"></select> <select name="year" id="year"></select> </body> </html>
-
You better using DOM when working with AJAX chat or similar scripts, basiclly, you'll need to remove the old divs after a while by set an interval that checks the lenght of comments.
For example
function removeOldDivs(){ var maxMessages = 20; var msg_divs = document.getElementById('chat').getElementsByTagName('div'); var numMessages = msg_divs.length; if (numMessages > maxMessages){ var loop = numMessages - maxMessages; for (var i=0; i<loop; i++){ document.getElementById('chat').removeChild(msg_divs[i]); } } }; setInterval("removeOldDivs()", 5*60*1000); //every 5 minutes
-
-
-
Your write about the name attribute; I didn't mean to leave that one in there, but the rest of the attributes should work fine for all images in your page.
Not sure if you're familar with collections and single elements. Here are some details about it getElementsByTagName
http://developer.mozilla.org/en/docs/DOM:element.getElementsByTagName
Notice it returns a node list which doesn't support any of the attriubtes you listed above.
Try to save your code as HTML and test it
-
I don't know how you call your ajax, but here is how it should work
Ajax.request(....) // function that parse the results function ajax_return(txt){ // do stuff with text (ajax return) // select first drop down update_second_dropdown(); }
-
First, you need to escape your strings
shopzilla="<script language=\"JavaScript\"
this works the same way as in PHP
second, you can't have </script> in the middle of your script anywhere
so you'll need to write it like this
...type=\"text/javascript\"></s"+"cript>";
no point of putting a noscript tag in the middle of your JS
<noscript> and everything between it
last thing, make sure you don't have any line breaks in your strings
var something = "hello world
bye world"; is wrong
do this instead
var sometimg = "hello world\nbye world";
or
var something = "hello world\n";
something += "bye world";
-
First you're onsubmit event needs to return the validation function
onSubmit=\"return logincheck()\">
Second, after you alert the error, make sure you return false as well
alert("You never filled in the username.");
return false;
-
getElementsByTagName will return a collection of images, so you can't set the name attribute to it directly.
If you want to add attributes to your images, you can access them using something like this
var imgs = document.getElementsByTagName('img'); var i=0, loop=imgs.length; for (i=0; i<loop; i++){ imgs[i].id = "image_"+i; }
Mail with document attachment from form
in PHP Coding Help
Posted
using the
in your attachment is a security risk since you exposing the temporary saved file name. If someone uploads a php file with malicious code, they can run it directly in your server.
You'll need to move the uploaded file (after you varifiy it's safe) to another folder in your site, and than send that file. More details on how to upload a file can be found here
http://us3.php.net/manual/en/features.file-upload.php