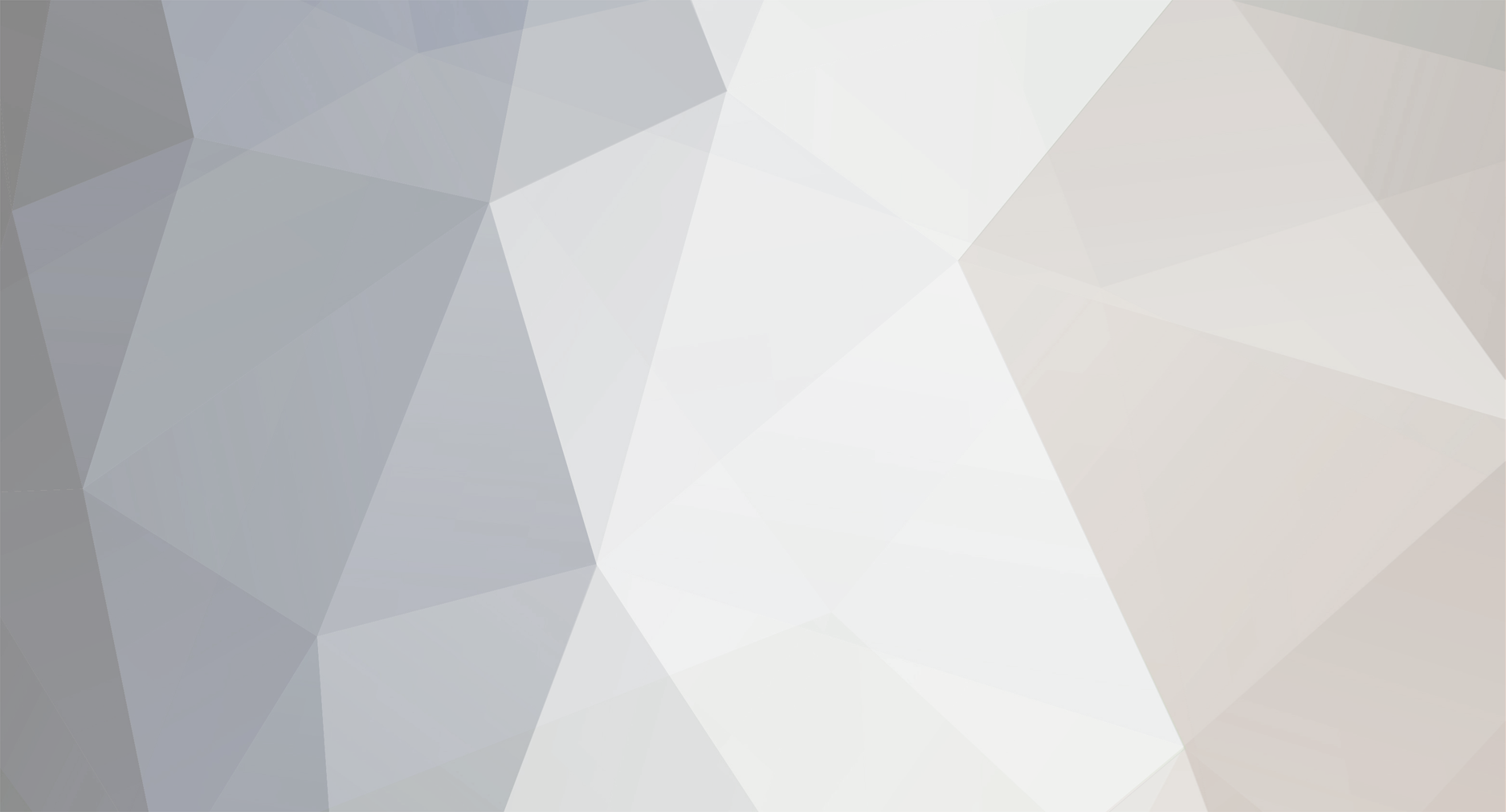
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
add an alert(results); before you compare to see what the actuall value of results.
Also, results is a string, so your comparisson should be
if (results == "1")
with quotes around the one
finally, you can use
if (results == "1") { ... }
else { ... }
-
I am not sure if you looked over the whole thing, but that's exactly what the Yahoo UI will do, here is an example
http://developer.yahoo.com/yui/examples/menu/topnavfrommarkupwithanim.html
-
make sure you call the right function check or checkAll
-
try this
delete winBody;
-
PHP and JavaScript can't see each other. so, the "php code here" should be the output of the php. You can use ajax to call a php page and get the response.
-
try to put all your attrbuite values in quotes
<form method="POST" action="blahblah" .... <a href="javascript:document.view$ff_draft_team_name.submit();" ...
Also, beside giving the form a name, give it an id too
<form id="formID" ....
after that, you can use document.getElementById() function
-
just add style="display:none;" to the div tags
-
change the exit statment to something like this
exit("<script language=\"javascript\">location.href=\"company1.html\";</script>If you are not redirected in 2 seconds, please <a href=\"company1.htmt\">click here</a>");
-
-
Change the text inside the link to
and add
style="display:none;"
to the div
-
change the document.style. in your functions to obj.style (since you're passing the object to the function)
-
Just hide and show the buttons as well
<a href="#" onclick="hideStuff('deals'); hideStuff('hide_link); showStuff('show_link'); return false;" id="hide_link"><img src="images/hide.png" width="10" height="10" border="0"></a> <a href="#" onclick="showStuff('deals'); hideStuff('show_link); showStuff('hide_link'); return false;" id="show_link"><img src="images/show.png" width="10" height="10" border="0"></a>
-
to preserve the image proportions just enlarge the width or height (not both), the browser should take care of the rest.
-
The open_one() function works fine, you have an load_config() function on the onload, maybe it's causing the error.
-
something like this will do
<select name="test" id="test"> <option value="1">1</option> <option value="2">2</option> </select> <a href="#" onclick="window.open('pop_up.html?test='+document.getElementById('test').value, '', 'width=500, height=500'); return false;">Click here</a>
-
You can change the format on these two lines
line 535 p_format = "YYYY-MM-DD";
line 554 p_format = "YYYY-MM-DD";
-
You only need to write your functions in the javascript once (don't write the function in the while loop)
And you mixing javascript and PHP when writing the image tag (id=imgId). This will make all your images have one id "imgId".
Also, the while loop shouldn't have a ; after it
It should be
while (statment) { do something }
You can change your image tag to look like the following
<img src=$d2 border=0 width=$width height=$height onmouseover=imgEnlarge(this) onmouseout=imgShrink(this)>
and your functions to this
function imgEnlarge(obj){ obj.height += 100; obj.width += 100; } function imgShrink(obj){ obj.height -= 100; obj.width -= 100; }
-
Try to use the NoGray javascript library, there is a getStyle function that will return the current style of the object.
Since the documentations are a bit outdated, here is a quick example
<html> <head> <title>getStyle Example</title> <!-- including the base.js --> <script language="javascript" src="nogray_lib/base.js" type="text/javascript"></script> <script language="javascript"> function ini_page(){ // this function will assign the NoGray libraray methods // to the object // _obj() is the same as document.getElementById() make_NoGray(_obj('wdiv')); // getting the style attributes // you need to use a full attribute for example // backgroundColor rather than background var txt = "Background color: "+_obj('wdiv').getStyle("backgroundColor")+"\n"; txt += "Font family: "+_obj('wdiv').getStyle("fontFamily")+"\n"; txt += "Height: "+_obj('wdiv').getHeight()+"\n"; txt += "Width: "+_obj('wdiv').getWidth(); alert(txt); } </script> </head> <body onLoad="ini_page();"> <div id="wdiv">Hello World</div> </body> </html>
-
Well, you can use a "try and catch" statment. It's not a perfect solution but it works.
<script language="javascript"> function checkID(){ try { var objID = document.getElementById(document.getElementById('input1').value).id; alert("ID is already in use"); return true; } catch(e){ alert("There is no object with this ID"); return false; } } </script> <div id="div1">First Div</div> <input type="text" id="input1" value="div1" /> <button onclick="checkID();">Check if ID</button>
-
What happens is the thumb nail library you're using will look at the HTML (not javascript) code when the page loads to assign the onclick events to them. Since your drop down uses JavaScript to generate the links, you'll need to assign the onclick event from the library after the links are generated.
Or the easiest way is to create a hidden div with the link inside (as html) and use the javascript to show and hide that div rather than creating the whole thing.
-
The first script will run the Javascript before the browser writes the div tag, so it will look for the div tag but can't find (error, the object haven't loaded)
The second one will run the script after.
To be safe, always make sure your page loads before trying to access element. You can add an onload even in the body tag and run a function from there
<head> <script type="text/javascript"> function write_UN(){ document.getElementById('login_button').innerHTML = "George"; } </script> </head> <body onload="write_UN();">
Also, This.value should be this.value (lower case letters)
-
You can't call the addslashes inside the echo statment
change it to something like this
echo " <script language = \"Javascript\" type = \"text/javascript\"> var elemId = ".addslashes($d1)."; ...
-
Try this function
function deleterow(rvalue) { var answer = confirm("Are you sure you want to delete?"); var values = rvalue.split("|"); if(answer){ document.getElementById('ch'+values[0]).innerHTML = "CH1"; document.getElementById('bh'+values[0]).innerHTML = "BH1"; } else { alert(values[0] + '-' + values[1] + '-' + values[2] + '-' + values[3]); } }
-
Client side peripherals
in Javascript Help
Posted
The magstripe/smartcard readere should operate as an input device (keyboard) if it's connect through usb (I think).
Try to make a textarea and focus on it, than slid the card.
Also, this might come in handy http://www.acmetech.com/blog/magnetic-track-data-parsers/