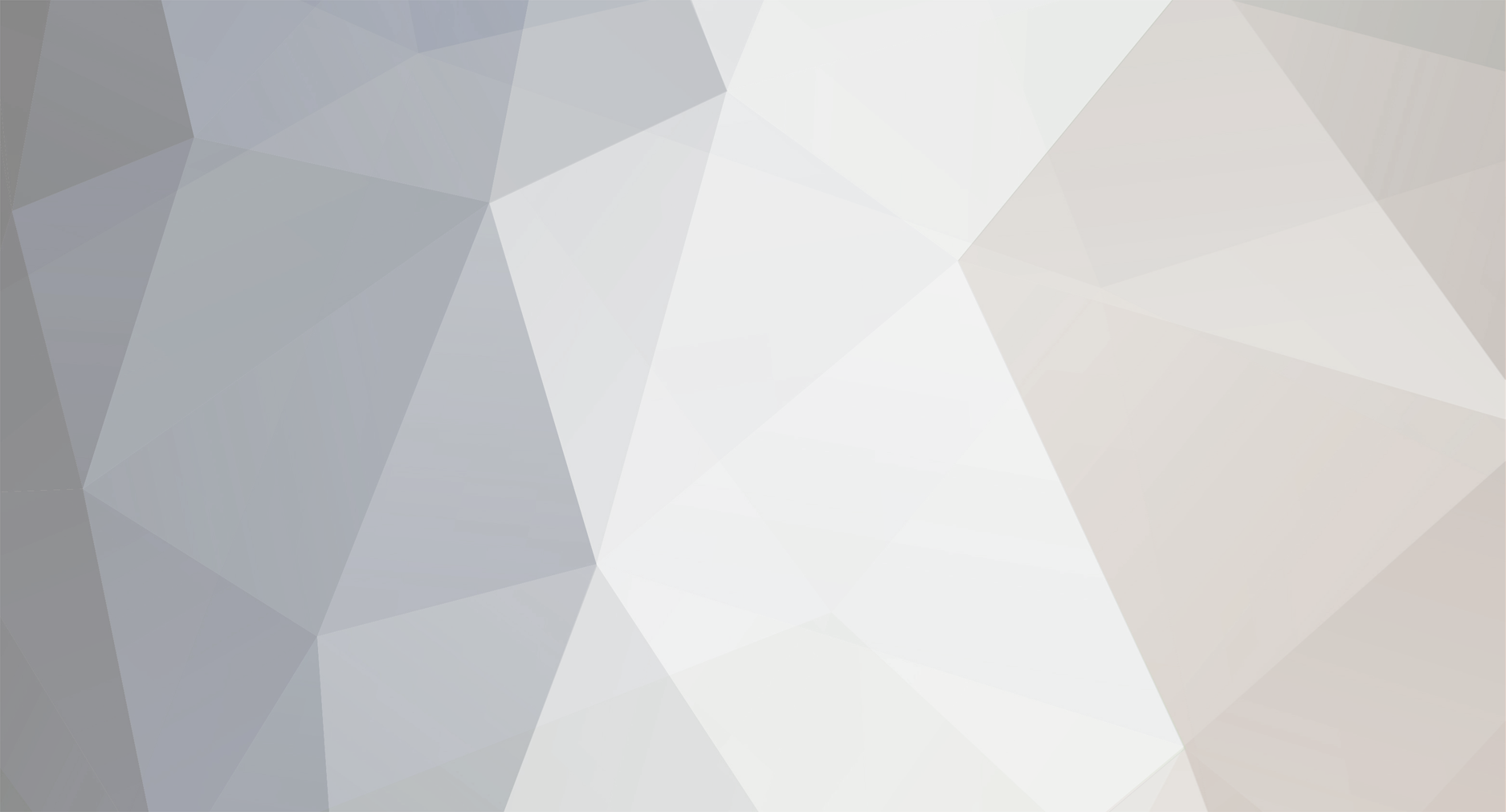
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
If you check the NoGray JavaScript library, you'll find a whole set of animation options. Also there is a set opacity function.
Or you can do use the set opacity as a stand alone with a setTimeout to hide and show the divs.
// pass the object to the function using // something like document.getElementById() // IE might need the object to have absolut positioning function setOpacity(obj, val){ // checking if the browser is Opera var is_Opera = (window.navigator.userAgent.search("Opera") != -1); // checking if the browser is IE var is_IE = ((window.navigator.userAgent.search("MSIE") != -1) && !is_Opera); if (is_IE){ obj.style.filter ="progid:DXImageTransform.Microsoft.BasicImage(Opacity="+val+")"; } else { obj.style.MozOpacity = val; } obj.style.opacity = val; }
The val should be between 0 to 1
-
You need to initiate two Date variables to get the different times, here is an example
<script language="javascript"> function do_something(){ // start timer var st_time = new Date(); // do stuff var foo = 0; for (i=0; i<1000; i++){ foo += foo * i; } // end timer var en_time = new Date(); alert(st_time.getTime()+" "+en_time.getTime()); } do_something(); </script>
-
just add an id to the text field
<input name=number id=number type=text value=1 > <input type=\"button\" value=\"ADD TO CART\" onclick=\"window.location.href='cart.php?id=1&number='+document.getElementById('number').value\";>
-
Check this out
http://developer.yahoo.com/yui/menu/
Click on the examples (there is an animated example there too) to see how it works.
-
You only can read pages from the same domain for security reasons. If you want to capture the code, you'll need to make a server side script (like PHP or ASP) that will grap the site content and display it on your domain.
-
<body onload="setTimeout('location.href=location.href', 2*60*1000);">
-
I haven't tested this, but you'll need to set a boolean type variable on the body element. When the mouse is down, you set the variable value will be true and when the mouse is over an object, you can check the variable value.
-
change this
IF (document.FormName.fCardType = 'Visa' || 'Mastercard')
to
if ((document.FormName.fCardType == 'Visa') || (document.FormName.fCardType == 'Mastercard'))
-
Here is a few function that will help you select any option based on the value, text or index
<script language="javascript"> // select an option based on value function selSelectValue(sel, val){ var op = sel.childNodes; var selIndex = 0; for(i=0; i<op.length; i++){ if (op[i].tagName == "OPTION"){ if (op[i].value == val) { sel.selectedIndex = selIndex; i = op.length+1; } selIndex++; } } } // select an option based on text function selSelectText(sel, val){ var op = sel.childNodes; var selIndex = 0; for(i=0; i<op.length; i++){ if (op[i].tagName == "OPTION"){ if (op[i].innerHTML == val) { sel.selectedIndex = selIndex; i = op.length+1; } selIndex++; } } } // select an option based on index function selSelectIndex(sel, val){ sel.selectedIndex = val; } </script> <select id="sel" name="sel"> <option VALUE="value1">Hotmail</option> <option VALUE="value2">Gmail</option> <option VALUE="value3">Yahoo</option> </select> <br /> Text:<br /> <input type="text" id="val" /> <br /><br /> <button onclick="selSelectValue(document.getElementById('sel'), document.getElementById('val').value);">Select by Value</button> enter value1, value2 or value3<br /> <button onclick="selSelectText(document.getElementById('sel'), document.getElementById('val').value);">Select by Text</button> enter Hotmail, Gmail, Yahoo<br /> <button onclick="selSelectIndex(document.getElementById('sel'), document.getElementById('val').value);">Select by Index</button> enter 0, 1, 2
-
If you need any javascript or ajax help, I can help with anything. Just drop me a quick email.
P.S. I can create a JS library to match your PHP classes if you're using OOP with easy to use syntax.
-
You gotta wait until the second page loads before you access it's elements. You can use the script inside the whatever.html page to get the code from the parent window once it loaded.
in whatever.html <script language="javascript"> function ini_page(){ document.getElementById('theDiv').innerHTML = opener.getConent(); } </script> <body onload="ini_page();">
in the main page function getContent(){ return theTable; }
Just make sure theTable is a global variable.
Code untested.
-
Yahoo UI has this made at
http://developer.yahoo.com/yui/button/
click on examples to see how they set radio buttons.
-
If you notice in your code
txt['Bungler's1']
You can't have the single quote ' in the array index, change it
txt['Bunglers1']
There are more than one, this is just an example.
-
use a more common AJAX approch to avoid any problems. The XMLHttpRequest works in IE 7 and other browsers, while ActiveXObject("Msxml2.XMLHTTP") only works in IE 6 and below
something like this will be a better choice
var xmlHttp; try{ // Firefox, Opera 8.0+, Safari xmlHttp=new XMLHttpRequest(); } catch (e) { // Internet Explorer try{ xmlHttp=new ActiveXObject("Msxml2.XMLHTTP"); } catch (e){ try { xmlHttp=new ActiveXObject("Microsoft.XMLHTTP"); } catch (e){ alert("Your browser does not support AJAX!"); return false; } } }
-
Here is an example on how to access the selected option value. You cannot have any events on the option directly.
I used a javascript array for the data, but most likely you would wanna use ajax if you have a gain list.
good luck
<script language="javascript"> var txt = new Array(); txt['option 1 value'] = "option 1 description"; txt['option 2 value'] = "option 2 description"; txt['option 3 value'] = "option 3 description"; txt['option 4 value'] = "option 4 description"; txt['option 5 value'] = "option 5 description"; function show_txt(val){ if (val != "") document.getElementById('description').innerHTML = txt[val]; else document.getElementById('description').innerHTML = ""; } </script> <select onchange="show_txt(this.value);"> <option value="">Select</option> <optgroup label="Hello"> <option value="option 1 value">Option 1</option> <option value="option 2 value">Option 2</option> <option value="option 3 value">Option 3</option> </optgroup> <option value="option 4 value">Option 4</option> <option value="option 5 value">Option 5</option> </select> <div id="description"></div>
-
use document.getElementById(id) instead of document.all
-
The problem is the lightboxes out there only look at the HTML code when the page loads, so if you using AJAX to load the page, the lightbox won't know it has to look for the links, therefore not working. To fix that, look at your lightbox script and find the initiating function and find what events the script add to the links. You can call that function when the AJAX page loads as well.
-
somewhere in the javascript (outside the functions) create a variable called folder
var folder = "/foldername/";
Inside the function, just use that variable
... img.src = folder+'image_assets/expand.gif'; }
-
If you running the script from your own pc, make it an HTA file (HTML application)
more on hta http://msdn2.microsoft.com/en-us/library/ms536496.aspx
This will give you the security clearness to write to your own pc (if you have Norton antivirous, you'll get a warrning).
If it's live, than you can't write using front end script, you can use JScript.net, but thats works on the server side as well.
The issue is if someone gained access to your page that write the files, they can add a script to the URL query to comprimize your site.
-
You need to print the array outside the echo statment and add signle quotes around the javascript variables
<img src=\"del.gif\" onClick=\"confirmDelete('".$post[id]."', '".$post[topic]."');\" border=0>
Also, make sure your $post doesn't have any quotes or signle quotes.
-
-
you can add a "Check if unique" link next to the field, so when they finish typing, they can click on that and a small pop-up (or ajax) will check if the username is unique.
Also, you can submit the form to a hidden iframe that will either process the form and than redirect the parent window, or will print out a javascript alert with the errors (this way they don't leave the form after they submit unless it's valid).
-
The filesystemobject is only an IE object and only works on .hta (HTML application) file.
You can't write to the server using JavaScript because it's a huge security issue (anyone can hack your site by writing server side files).
User a server side script to write the file such as PHP or ASP,
-
both these scripts work fine, make sure you don't have an error somewhere else in your code that stops the script from working. In firefox, after you get the error click on "Tools" from the top menu (next to File, Edit, View) and select "Error Console" (or Javascript Console if you have an older version).
This should let you know where is your error.
Not sure if this is the right forum but how do i do this?
in Javascript Help
Posted
check this out
http://www.walterzorn.com/tooltip/tooltip_e.htm