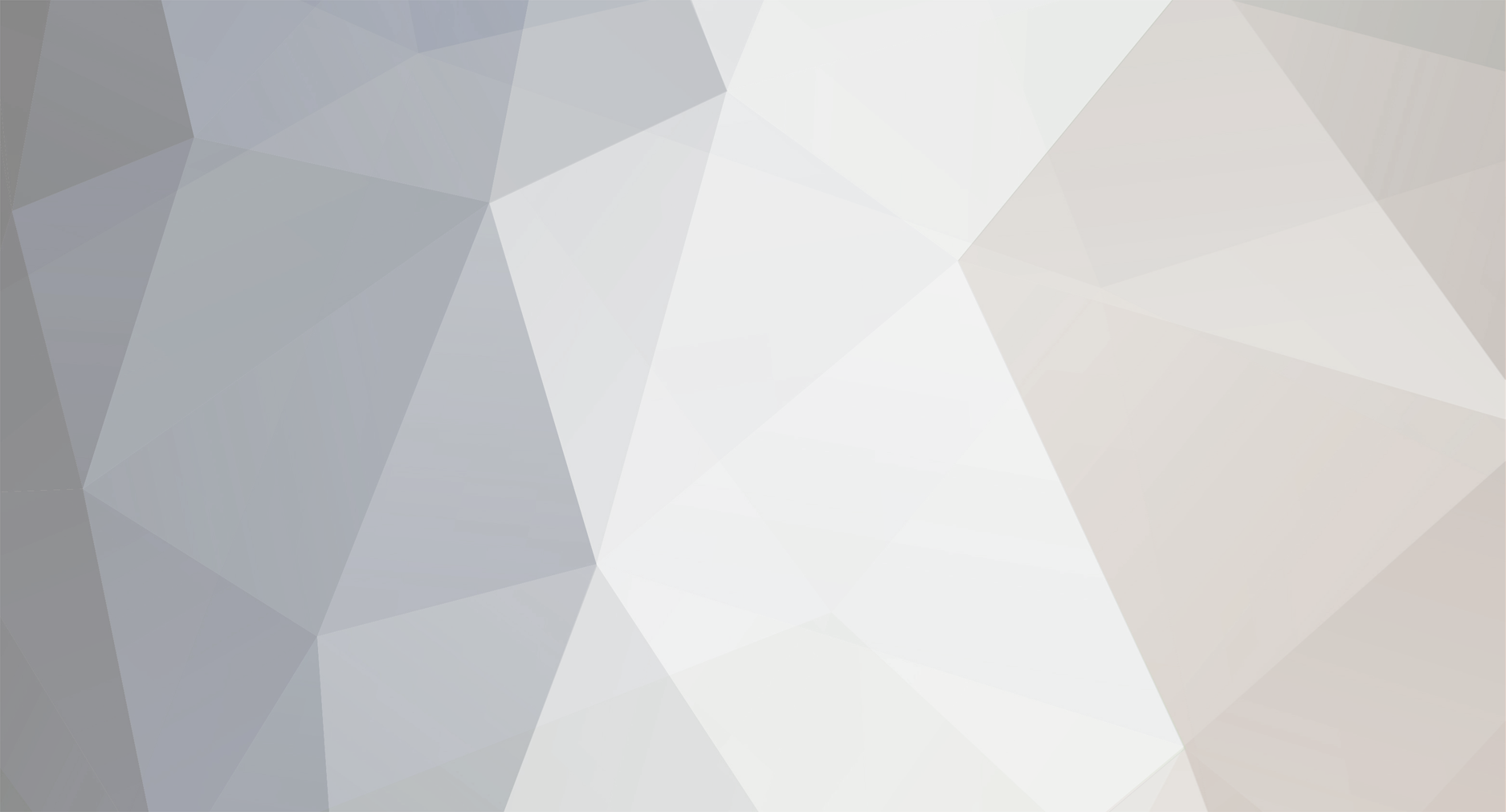
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
you don't have to pass the form, you can just pass the textarea element like this
[code]
onclick="addtext(this)";
[/code]
[code]
addtext(txt){
var Article = txt.value;
}
[/code]
This way you don't have to worry about names.
-
you can try the [url=http://us3.php.net/manual/en/function.eval.php]eval[/url] function
[code]
echo eval($some_info.";");
[/code] -
If you want IE to center you div by using the margins, you need to include the document declartion
[code]
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
[/code]
If you floating HTML elements, you'll need to clear them before closing the holding div
So, something like this in the style
[code]
.clear_float {clear:both;
height:1px;
overflow:hidden;
[/code]
and you middel section will look like this
[code]
<div id="middle">
middle
<div id="middle-top">
middle top
</div>
<div id="left">
left
</div>
<div id="right">
right
</div>
<div class="clear_float"> </div>
</div>
[/code] -
Just google "Web bug" and you'll get tons of way of doing it.
Basically you create a server side file (php, asp, or whatever) that will record if the email was open and return a small image (could be just a spacer).
Using a web bug will increase your spam score with many email providers (but not much) -
a recursive is just like any other function instead it calls itself.
So basically it works kinda like this
function,
return match if true,
return function if false,
return match if true
return function if false.
etc etc....
so, after looping so and so times, each function call must return a result until it reach the top function which will return the final result. A bit odd at first, but it's simple when you get it. -
If it's one file message will be seprated by two line breaks and the From email@domain.com
some the file might look like this
From someone@somewhere ......
... more headers ....
message
From someone@somewhere ......
... more headers ....
message
From someone@somewhere ......
... more headers ....
message
usually the first section is the setting of the email account, so you can split the text file using "\n\nFrom" as the sperator and check each if for whatever you want it to match (the email, subject, etc)
To add the message to another account, you can just place it in the bottom of that file (just make sure it has \n\n before it and after) you should include the headers for it to show up in the email manager (I think).
You'll need to make some tests and test some more for this to work like you want it. -
try to add mysq_error() to your query to find out if you have any errors
[code]
$result=mysql_query($query) or die(mysql_error());
[/code] -
You need to use javascript to do that.
check this page for details
http://www.w3schools.com/js/tryit.asp?filename=tryjs_breakout -
You are forgeting the limit in the query
[code]
keywords LIKE '%$find%' limit ".(($page-1) * $pageSize).','.$pageSize
[/code] -
I think you are missing a return in the else part.
return findParentID($userID, $Row["parentID"]);
this just a guess, but give it a shot. -
It depends how your server is set up to store the mail, most servers will store each account (and folder) in a text file. Some will store each message in a folder for that email account. If you have access to your mail folder, you can read the files using PHP and extracting any message you want. You can also place it in another email account as well.
-
you set the position to absolute and not the top and left, top and left will be set to auto (meaning they'll appear at the same location). Or you can set them to auto as well.
Now, since they are in a table cell, I set the height and width of the table cell itself. This way the space is used and the images will show up on top of it. Finally set the valign of the table cell to the top and the text align to the left.
and there is nothing standrad about HTML, as long as it works, it should be ok. -
ok, I see the error. You'll need to use ids instead of names.
change your calc function to
[code]
function calc(f, e){
one = document.getElementById(f).value;
two = document.getElementById(e).value;
document.getElementById('total1').value = (one * 1) + (two * 1);
}
[/code]
Notice you'll always use total1 in this function.
and change your HTML to
[code]
<td><input name="a<?=$i?>" id="a<?=$i?>" type="text" onFocus="calc(<?=$fn?>, <?=$ef?>);"></td>
<td><input name="b<?=$i?>" id="b<?=$i?>" type="text" onFocus="calc('<?=$fn?>', '<?=$ef?>');"></td>
<td><input name="total<?=$i?>" id="total<?=$i?>" type="text"></td>
[/code] -
try to use absolut positioning instead, this way you don't have to worry about negative margins and top.
[code]
<table border="1">
<tr><td style="width:275px; height:347px; text-align:left;" valign="top">
<div id="Layer1" style="position:absolute; width:275px; height:347px; z-index:1;"><img src="images/commercial.jpg"></div>
<div id="Layer2" style="position:absolute; width:275px; height:347px; z-index:2; opacity: 0; -moz-opacity: 0; -khtml-opacity: 0; filter: alpha(opacity=0);"><img src="images/commercial_alt2.jpg"></div></td></tr></table>
[/code] -
Try to print out your $_SERVER['HTTP_REFERER'] in the second page so you know exactly how it looks like (it could be http://domain.com or http://www.domain.com, etc)
-
You need to include the div names in single quotes
like this
onFocus="calc('<?=$fn?>', '<?=$ef?>');" -
change this line
$headers .= "Content-type: text/plain; charset=iso-8859-1\n";
to
$headers .= "Content-type: text/html; charset=iso-8859-1\n";
and your message should show html -
You can do this in different ways, one is to set 2 arrays, one for the new values and one for the old values. Then you loop around them and if the values are different, you just change add the new value to the query.
for example, you html form can look like this
[code]
<form method="post" action="php_file.php">
<input type="hidden" name="id" value="1" />
<input type="text" name="new[field_name]" />
<input type="text" name="new[another_field]" />
<input type="submit" />
</form>
[/code]
and your php file
[code]
<?PHP
// connect to db here
$result = mysql_query("select * from `Table` where `id` = '".$_POST['id']."' limit 1") or die("couldn't select " . mysql_error());
$old = mysql_fetch_array($result);
$new = $_POST['new'];
foreach($new as $key=>$val){
if ($old[$key] != $val){
$query .= "`$key` = '".mysql_real_escape_string($val)."', ";
}
}
$query = "update `Table` set ".trim($query, ", ")." where `id` = '".$_POST['id']."'";
$result = mysql_query($query);
?>
[/code]
I haven't tested this, just typed it right now, so you may have to adjust it a bit. -
let's say the date variable is called "dt"
[code]
document.getElementById("link").href = "link1.php?datecompleted="+dt;
[/code] -
The easiest way is to use flash, works anywhere ;) and with some ActionScript (which I know nothing about) you can make the pictures dynamic.
-
There are a lot of ways to promote a site, but it takes some time and effort.
The best way to get conversions is in search engine marketing because you can target the actual keyword and ads for people who search. This is pretty easy and conversion rate is high, but it cost a lot for a lot of keywords (like insurance, cash advance, etc..)
You can find these at
[url=http://adwords.google.com]http://adwords.google.com[/url]
[url=http://adcenter.msn.com]http://adcenter.msn.com[/url]
[url=http://www.overture.com]http://www.overture.com[/url]
Second is contextual ads, this is a bit cheaper and you can place your ads in sites that are relevant to your topic. Usually the conversion rate is lower and it's cheaper than search engine marketing.
some sites
[url=http://www.adbrite.com]http://www.adbrite.com[/url]
[url=http://www.clicksor.com]http://www.clicksor.com[/url]
there are tons of them, just google it ;)_
Third is Email Marketing. This method have a very low conversion rate and can be very cheap. But there is too many things to take care of such as the mailing list, the canspam laws, spam filters, etc...
Fourth, Web Traffic (expire domain redirecting, pop ups, etc) This is the cheapest way to get traffic to the site, and usually conversion rate is 1 in 100000. Kinda like wasting money.
Fifth, banner placement on banners network (like double click). Banners usually get low traffic and the conversion rate vary from one campaign to another. It works best when the banner is placed in a highly trafficed site that is relevant to your site, but these sites are a bit pricey too.
==================================
Other than paid advertisement, you can do some of these stuff.
SEO, very important, but takes a lot of work and a long time. You'll need to optimize your site so it'll rank higher in search engines for your terms. When you get good placement, you'll get a lot of qualified traffic for free. A lot of details can be found at
[url=http://www.seochat.com]http://www.seochat.com[/url]
Posting in forums (like PHPFreaks)
Post and answer as many questions as you can in forums related to your site. Include you site link in the signature, people who read the forum and find your answers helpful will visit your site sometimes.
Submit your site to all directories you can find like yahoo directory and dmoz.org
I think this covers most online strategies, but you also can go offline and put a small ad in the paper or on the radio (if you got the extra cash) -
you got the blendtrans for IE, for firefox, you'll need to set a javascript function to set the opacities of both objects and blend them that way. You'll need to use setInterval() to change the opacity like this
object.style.MozOpacity = ##
## is between 0.0 and 1.0
You can do the same for Opera 9.0 and higher (the newest version) by changing the CSS3 opacity property
object.style.opacity = ## -
If the table cell is empty (like <td></td>) It won't have any borders or background. You'll need to have at least a space (like <td> </td> or <td>& n b s p;</td> without the spaces).
-
for JS you gotta build the url-encode yourself using the replace() function. it should be easy since you only need to replace the & character.
How can I show a message when the script is loading?
in Javascript Help
Posted
[code]
<body onload="document.getElementById('calc').style.display = 'none';">
<div id="calc">Calc....</div>
</body>
[/code]