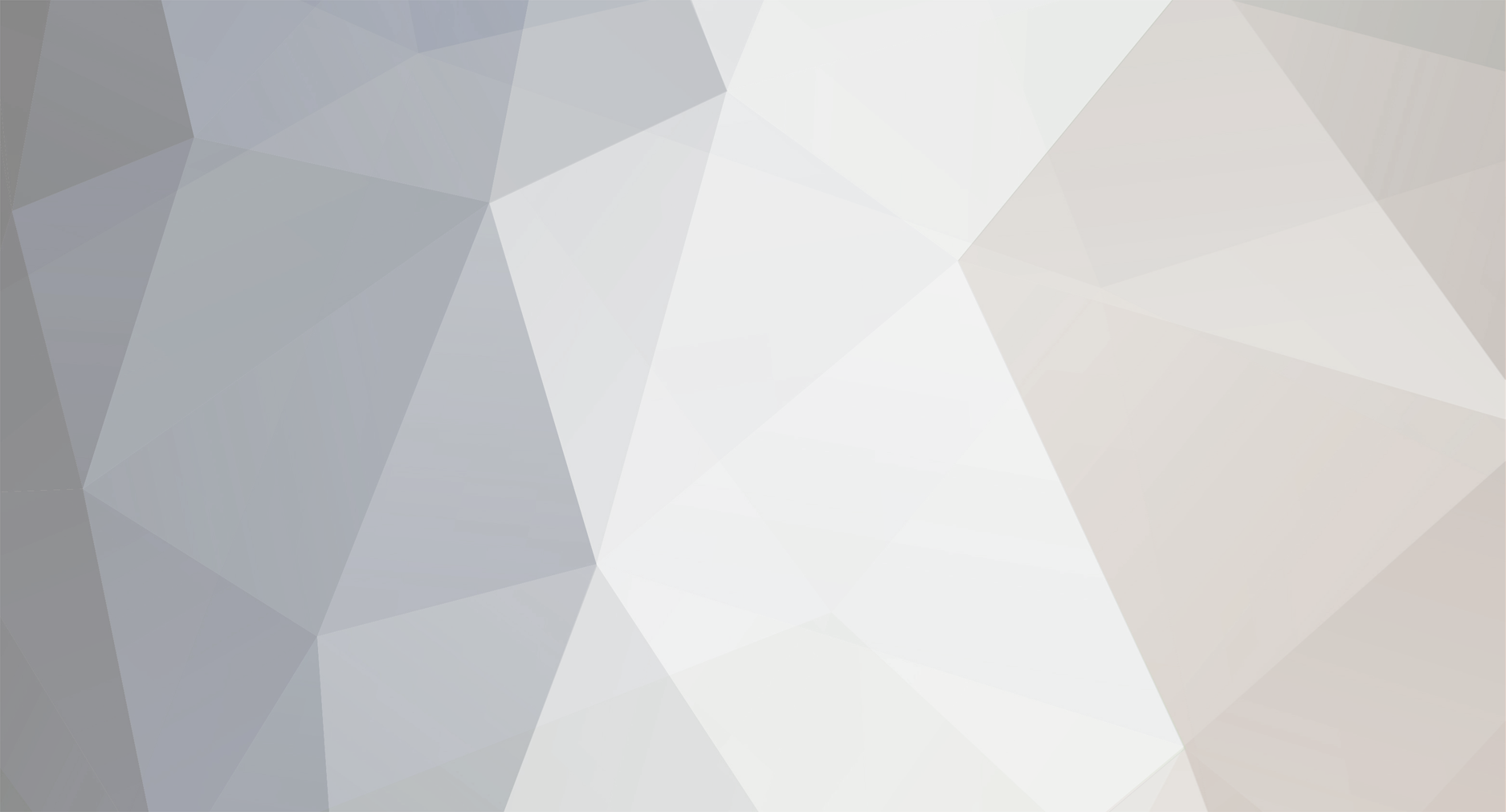
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
ohhh, got it.
Since all your elements inside the div tag are floated, you'll need to clear them. otherwise, they will go over the div, and the div won't show up.
You'll need to add this to the style
[code]
.clear_float{clear:both;
font-size:1px;
overflow:hidden;}
[/code]
and before you close your div
[code]
.... your code....
<div class="clear_float"> </div>
</div>
[/code] -
try to change the onsubmit to "return matchpwd();"
-
try to take the single quotes out of the background image in the css
background: url(http://www.allinthissite.co.uk/include/images/bar.gif); -
This is more of an HTML issue, so if you can post the HTML output of the script rather then the PHP code.
like <div>text and more text</div>
for the text to be justified, it has to be longer then one line (with no line breaks <br />) or it will look normal -
this looks like an FCKEditor variable, check it at http://www.fckeditor.net/
-
You can set your original select without any events, and attach the events to the new select menus. I am posting a sample to show how you can attach a simple function on onchange event (Also, changing the name and id of the select object) and a more advance attach for the onclick event to show how to get the object (using "this" refrence) and the event object as well.
[code]
<script language="javascript">
// checking if the browser is Opera
var is_Opera = (window.navigator.userAgent.search("Opera") != -1);
// checking if the browser is IE
var is_IE = ((window.navigator.userAgent.search("MSIE") != -1) && !is_Opera);
function add_sel(){
var new_sel = document.getElementById('sel_menu').cloneNode(true);
new_sel.name = "New_select";
new_sel.id = "new_select_id";
new_sel.onchange = function (){ alert('I am here'); }
new_sel.onclick = show_me;
document.getElementById('holder').appendChild(new_sel);
}
function show_me(evt){
if (is_IE) evt = event;
alert(this.tagName);
alert(evt);
alert(this.name + " " + this.id);
}
</script>
<select id="sel_menu">
<option value="1">a</option>
<option value="2">b</option>
</select>
<div id="holder">
</div>
<button onClick="add_sel();">Clone</button>
[/code]
if that doesn't work, you can always get the inner HTML of the holder div, replace the "<select" with "<select event event evetn" and then replacing the inner HTML of the holder div with the new HTML code. -
The error is in the ajax part, I copied your code and removed the ajax part and seems to work (not sure if it's right, but the error problem is gone).
One of the problem may be in this statement
[code]
document.getElementById(UPDATE[0]+'_container').innerHTML = "";
if(trimString(document.getElementById(UPDATE[0]+'_container').value) != ""){
dispSubmit(UPDATE[0],'remove');
}
[/code]
I don't think you need the _container in the getElementById(UPDATE[0]+'_container').value since you checking the field and not the container. -
[quote]
it seems to me as though it is the same as $_SERVER[HTTP_USER_AGENT]. Is it not?
[/quote]
It uses the $_SERVER[HTTP_USER_AGENT] to get the browser info, but it also uses a database to fill in all the details, Here is a sample output
[quote]
Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.7) Gecko/20040803 Firefox/0.9.3
Array
(
[browser_name_regex] => ^mozilla/5\.0 (windows; .; windows nt 5\.1; .*rv:.*) gecko/.* firefox/0\.9.*$
[browser_name_pattern] => Mozilla/5.0 (Windows; ?; Windows NT 5.1; *rv:*) Gecko/* Firefox/0.9*
[parent] => Firefox 0.9
[platform] => WinXP
[browser] => Firefox
[version] => 0.9
[majorver] => 0
[minorver] => 9
[css] => 2
[frames] => 1
[iframes] => 1
[tables] => 1
[cookies] => 1
[backgroundsounds] =>
[vbscript] =>
[javascript] => 1
[javaapplets] => 1
[activexcontrols] =>
[cdf] =>
[aol] =>
[beta] => 1
[win16] =>
[crawler] =>
[stripper] =>
[wap] =>
[netclr] =>
)
[/quote]
as you can see, you'll get all the browser details such as the name, version, support for css, frames, javascript, java, etc etc.... (the support part is if the browser is capable, and not if the user have it turned on or off). -
you just use cloneNode(true) and that should clone all the childern of the elements
Here is an example
[code]
<script language="javascript">
function add_sel(){
var new_sel = document.getElementById('sel_menu').cloneNode(true);
document.getElementById('holder').appendChild(new_sel);
}
</script>
<select id="sel_menu">
<option value="1">a</option>
<option value="2">b</option>
</select>
<div id="holder">
</div>
<button onClick="add_sel();">Clone</button>
[/code]
I am not sure why Opera change it though!! -
Instead of looping through everytime you need to fill the menu, you don't you make a pre-filled select menu, and then clone it when you need. Should run a bit faster.
-
I am not sure if you notice this part
[quote]
Note: In order for this to work, your [url=http://us2.php.net/manual/en/ref.misc.php#ini.browscap]browscap[/url] configuration setting in php.ini must point to the correct location of the browscap.ini file on your system.
browscap.ini is not bundled with PHP, but you may find an [url=http://browsers.garykeith.com/downloads.asp]up-to-date php_browscap.ini[/url] file here.
[/quote]
if the get_browser() doesn't work on your server, you can use an alternative at http://alexandre.alapetite.net/doc-alex/php-local-browscap/
-
There is a [url=http://us2.php.net/manual/en/function.get-browser.php]get_browser()[/url] function in php
Just look it up in php.net -
If you want opacity in IE, you gotta set the position to absolute, and try to use their filters as descriped in http://msdn.microsoft.com/library/default.asp?url=/workshop/author/filter/filters.asp
[code]
<div style="position:absolute; filter:progid:DXImageTransform.Microsoft.BasicImage(opacity='0.7');">
70% opacity
</div>
[/code]
If your site doesn't work in IE, you really gotta fix it. It's not because IE is better or FF is better, it's because IE have over 85% of the web users... which could be over 85% of your future customers or clients.
and Microsoft urge their employees to use alternatives to IE (like FF or Opera) to see what other people use and what can be added to IE in the future. -
you can use [url=http://us2.php.net/manual/en/function.implode.php]implode()[/url] to do this
[code]
echo implode("<br />", $test);
[/code] -
This would be just normal HTML tags in your message
image example
[code]
<img src="http://www.domain.com/images/image.gif" border="0" alt="" />
[/code]
Make sure you use a full path (http://....)
for different font, just change the style
[code]
<div style="font-family:arial; font-size:9pt;">
text
</div>
[/code] -
most likely the page is cached and that's why it display the same image.
Try to hit "CTRL+SHIFT" and "Refresh" to see if the image reload in IE. If it works it's a cache problem.
You can fix it by adding
[code]
header("Cache-Control: no-cache, must-revalidate"); // HTTP/1.1
header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); // Date in the past
[/code]
to your php file. -
I am not sure if your include_once("header_inc.php"); print out anything or not. But header function must not have any output before it (no html, alert, text).
-
just replace this line
sDate.setDate(sDate.getDate()+1);
with this
sDate.setDate(sDate.getDate()+count); -
IE does ignore Extra Spaces (that's extra) if you have a line break after the image, that will be considered a space. You can use a line break (<br />) after the image or just close the td
If you want to width to work (using width="##") you gotta set the table width and the other cell width as well. Otherwise you can use css which works better. -
Check this site out,
http://www.maani.us/charts/index.php
-
something like this will work,
[code]
<script language="javascript">
function hide(){
document.getElementById('option1').style.display = "none";
document.getElementById('option2').style.display = "none";
}
function show_div(wdiv){
hide();
document.getElementById(wdiv).style.display = "block";
}
</script>
<input type="radio" onClick="show_div(this.value)" value="option1" name="options" /> Option 1<br />
<input type="radio" onClick="show_div(this.value)" value="option2" name="options" /> Option 2<br />
<div id="option1" style="display: none;">
Div 1 Text
</div>
<div id="option2" style="display: none;">
Div 2 Text
</div>
[/code] -
I would go with the PHP solution to write out the <script> tags, but if you want javascript only, you can make an importer file like this
[code]
var inc = new Array("file1.js","file2.js","file3.js");
var sc = "script";
for(i=0; i<inc.length; i++){
document.write("<"+sc+" language='javascript' src='"+inc[i]+"'></"+sc+">");
}
[/code]
and include that file in the header section. -
If you have more than one button, you gotta make sure they all have unique ids (like signed_1, signed_2, signed_3)
You can set a counter to assign the different ids for each button. But this is a guess since I don't have your HTML output.
If you can post the output for three rows, I can pin point the issue.
-
Oh, I thought the onclick event was in the textarea (dauhhh)
what you can do is access the textarea by id instead of name, this way you can either have the same id or define it in the JS in the beginning
[code]
onclick="addtext()";
[/code]
[code]
var Article = document.getElementById('ID_HERE').value;
[/code]
[code]
<textarea id="ID_HERE"></textarea>
[/code]
P.S. the id doesn't have to be the same as the name, but have to be unique.
my page works with ie and aol explorer but not with firefox and opera
in CSS Help
Posted