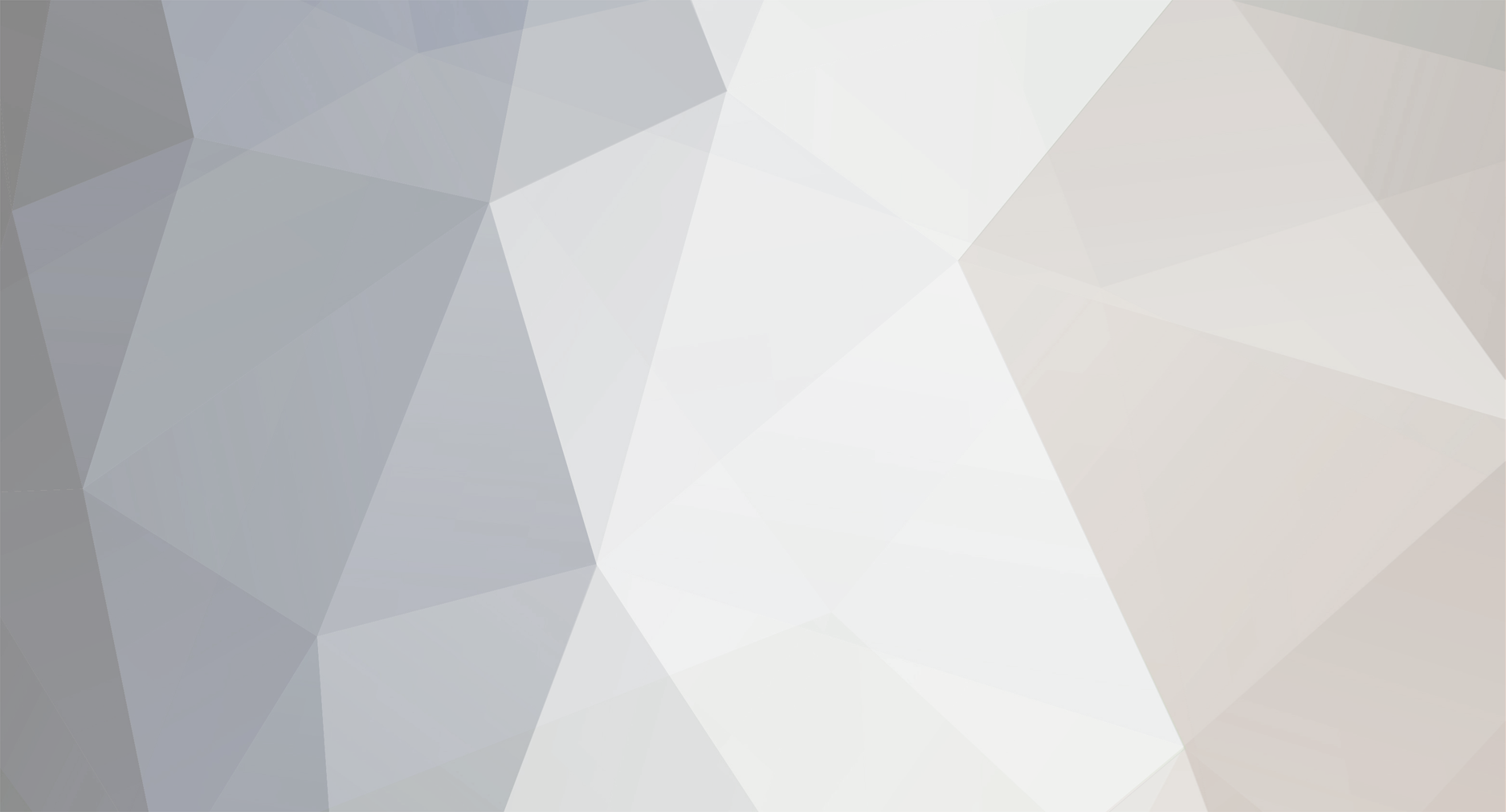
Psycho
Moderators-
Posts
12,164 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Change echo "$row<br>"; To echo "{$d[$row][0]}<br>";
-
modify date('W') range from specific day of a week
Psycho replied to misiek's topic in PHP Coding Help
This is ugly, but it works. I would likely go through and refactor make it more efficient. I'm sure it could be done in a simpler fashion, but I had to change direction multiple times. It works on the same logic as the ISO logic. For any weeks that span Dec/Jan, the week will belong to the prior year or the next year based on which year has the most days in that week (i.e. at least 4). Note; There is debugging code at the bottom that shows the results for the days at the end/beginning of each year to validate the results <?php function weekNo($dateTs) { //Create return variable and set initial 'year' value $result = array(); $result['year'] = date('Y', $dateTs); //Get Jan 1 for the provided timestamp and the DOW $janFirst = strtotime(date("1/1/Y", $dateTs)); $janFirstDow = date('N', $janFirst); //Determine first day of first week if($janFirstDow == 3) { //First day of first week is Jan 1 $firstDayOfFirstWeek = $janFirst; } elseif(in_array($janFirstDow, [3,4,5,6])) { //First day of first week is Wed prior to Jan 1 $firstDayOfFirstWeek = strtotime('last Wednesday', $janFirst); } else { //First day of first week is Wed following Jan 1 $firstDayOfFirstWeek = strtotime('next Wednesday', $janFirst); } //Calculate number of weeks since first day of first week $startDate = new DateTime(date('m/d/Y', $firstDayOfFirstWeek)); $currDate = new DateTime(date('m/d/Y', $dateTs)); $daysDiff = $currDate->diff($startDate)->format("%a"); //Calculate the Week NO $result['week'] = ceil( ($daysDiff+1) / 7); //Handle the first three days or last three days of the year //if they should fall in the adjoining year's first/last week //If selected date is Jan 1 - 3 if(date('m', $dateTs)==1 && date('j', $dateTs)<=3) { //If Jan 1st falls on Sun/Mon/Tue AND selected date is on Sun/Mon/Tue if(in_array($janFirstDow, [7,1,2]) && in_array(date('N', $dateTs), [7,1,2])) { //Get year/week no for the last week of prior year $result = weekNo(strtotime(date("m/d/Y", $dateTs).' -3 days')); //Increment year & set to week 1 } } //If selected date is Dec 29 - 31 if(date('m', $dateTs)==12 && date('d', $dateTs)>=29) { //If 12-31 falls on Wed/Thu/Fri AND selected date is on Wed/Thu/Fri if(in_array(date('N', strtotime(date('12/31/Y', $dateTs))), [3,4,5]) AND in_array(date('N', $dateTs), [3,4,5])) { //Increment year & set to week 1 $result['year']++; $result['week'] = 1; } } //Return result return $result; } //Debugging code echo "<pre>"; for($year=2005; $year<=2015; $year++) { $startDate = "12/15/{$year}"; $noOfDays = 30; echo "<h1>{$year}</h1>"; for($day=0; $day<$noOfDays; $day++) { $timeStamp = strtotime("{$startDate} + {$day} days"); //$isoWeek = date("W", $timeStamp); $weekNo = weekNo($timeStamp); $dow = date('N',$timeStamp); if( date('N',$timeStamp) == 3 ) { echo "<br>\n"; } echo "<b>" . date('D, m/d/Y', $timeStamp) . "</b> -- Year: {$weekNo['year']}, Week: {$weekNo['week']}\n"; } } echo "</pre>"; ?> -
modify date('W') range from specific day of a week
Psycho replied to misiek's topic in PHP Coding Help
Without determining the FIRST week of the year, you cannot know the logic for determining the "week" for any other dates in the year. I've already got a solution - except it doesn't handling the "remaining" days at the end of the year that should belong to week one. Should have a complete solution shortly. -
modify date('W') range from specific day of a week
Psycho replied to misiek's topic in PHP Coding Help
I think that would cause scenarios where the "first week" of the year, will be mis-calculated. Take the following example where Jan 1 starts on a Friday Mon Tue Wed Thu Fri Sat Sun DEC 28 29 30 31 1 2 3 JAN 4 5 6 7 8 9 10 - First week For a standard ISO week, the first week of the year is the one beginning on Jan 4, because it has at least four days in the current year. Using the logic above, the 4th and 5th of January would be counted as the last week of the previous year. For a Wed - Tue week, the first week for that same year should begin on Dec 30th (of the prior year). Wed Thu Fri Sat Sun Mon Tue DEC 30 31 1 2 3 4 5 - First week JAN 6 7 8 9 10 -
modify date('W') range from specific day of a week
Psycho replied to misiek's topic in PHP Coding Help
OK, how will the first week of the year be determined and how are you going to determine how many weeks there are in a year (hint: it is not always 52)? You can't simply "shift" the results of "W" to get the right answer (well depends on what your rules are I guess). The "W" format is for specifying the ISO-8601 week number. Here are the "rules" for determining the first and last week for that model So, determine your rules for determining how you will decide the first and last week - THEN we can help write code for it. -
Here's a class to get the duration of an MP3 file from the header information. http://www.zedwood.com/article/php-calculate-duration-of-mp3
-
Using file size of a bunch of MP3 files to determine the total play length seems a bit clunky. How many files are there? Why not get the actual play length of the files directly from the file headers?
-
"Which" custom error is being presented? I assume the custom error should prevent the record from being inserted. So, I further assume that your problem is that you believe the custom error is being triggered erroneously. But, that is a lot of assuming on my part. How about we change this around and you actually tell us the problem instead of us playing 20 questions? I know that when you are working on a problem, all these things seem "obvious" to you. But, when you need help, you need to step back and determine the relevant information to provide. A good rule of thumb would be to follow a normal bug report: 1. What are the steps to reproduce the issue 2. What is the expected result 3. What is the actual result
-
What "problem" are you having - exactly. What IS getting saved in the database? Or, are you getting errors? If so, what are they? Here are a few things you should verify: 1. Do a View Source on the form page and inspect the Select Options are properly formatted and that their values are the IDs that you expect. 2. Run this in the code the handles the form submission to verify the complete and correct data is being submitted echo "<pre>".print_r($_POST, 1)."</pre>"; 3. echo the query tot he page to ensure it is correct. Also, you can copy/paste the derived query into your database management console (e.g. PHPMyAdmin) to verify it is valid and see if there are any errors. Pro tip: Create and test your queries in a management console first (with hard coded data). Once you have them working as you want - then put them in your code replacing with the dynamic values. NOTE 1: Your code is wide open to SQL injection. You should be using prepared statements. NOTE 2: Who are you to say what characters may be in a person's name? What about a person with a hyphentated name "Julie Brown-Smith" or what about someone with diacritic characters in their name: "Robert Muñoz"
-
Any ideas why code is inserting just one character of each value?
Psycho replied to sonnieboy's topic in PHP Coding Help
Well, that's my fault. I use PDO and would just use the $sname in the execute statements instead of defining the values as defined variables. In my haste I threw in the list() function not thinking it only worked for numerically indexed arrays. -
Any ideas why code is inserting just one character of each value?
Psycho replied to sonnieboy's topic in PHP Coding Help
Change your form field names to this structure name="employee[<?=$id?>][employeename]" name="employee[<?=$id?>][ttitle]" name="employee[<?=$id?>][email]" . . . etc. Then on your form processing code you can do this $sql = "INSERT INTO table_name (employeename1, ttitle, email) VALUES(?, ?, ?)"; $sth = mysqli_prepare($conn,$sql mysqli_stmt_bind_param($sth, 'sssssss', $employeename, $ttitle, $email); //Iterate through each RECORD in the post data foreach($_POST['employee'] as $employee) { //Extract array fields into variables for prepared statement list($employeename, $ttitle, $email) = $employee; //Execute the query mysqli_stmt_execute($sth) } Note, I've left off a lot of error handling on this for brevity, but you should be able to see the concept., -
Pretty much what I was going to say. You just need an array of the database names along with a single connection. Then you can dynamically build the UNION queries something like this: //Create a query "mask" with placeholder for DB name $queryMask = "SELECT field1, field2, feild3 FROM [DB_NAME].tablename"; //Iterate over each DB creating the clause //for each DB replacing the mask $unionClauses = array(); foreach($databases as $db_name) { $unionClauses[] = str_replace('[DB_NAME]', $db_name); } //Cobine all the clauses with a UNION $query = implode("\nUNION\n", $unionClauses); //Using line break '\n' to make the query readable if you need to echo it out
-
The issue, is that you post a question and then a few minutes later you post that you've resolved it. That's great. You will learn much more by solving your problem yourself than someone just telling you what the problem is. But, there are two reasons why seeing such behavior will make me not want to post a response: 1) It would seem that the OP did not take the time to at least attempt to solve their problem before posting. Providing help to such people would only encourage them to not try to solve their own problems. 2) If I take the time to research the issue and post a response, the OP may already find the solution before I get a chance to reply. Nobody gets paid to provide help here. We do this because we like helping people. So, the fact that someone was not really "stuck" and was just posting a "drive-by" question while they complete their due diligence makes me feel like they do not value my time. I'm happy to help, really I am. But, if I find that my time is being wasted, I will be less apt to reply. You are free to post however you like (as long as it meets the forum rules), I am just providing some constructive criticism that I believe will help you get more and better responses.
-
You need to slow down. All of these drive-by posts are going to make people (i.e. me) decide not to help. If you get an error - read it. What do you think the error means? Then look at the code in question and determine what may be going on. An "array to string conversion" is pretty self explanatory. You are referencing a variable that is an array and you are performing some function on it that is intended for a string type value. Note: Many times when you get a parsing error, the error is actually before the line on which the error actually exists. PHP will report the line oin which it reached a place where it cannot understand what to do. For example, if you forget to close a string variable with double quotes, the PHP parser will think the lines of code following that line is part of the string you are defining. It isn't until it sees a closing quote mark that it will identify the error. FYI echo "<input type='hidden' name='employeename[]' value='".$sourcename1."' />"; echo "<input type='hidden' name='ttitle[]' value='".$sourceaddress1."' />"; echo "<input type='hidden' name='sourcename1[]' value='".$income1."' />"; When defining string with double quotes, there is no need to exit the quotes to append a variable. You can do this echo "<input type='hidden' name='employeename[]' value='{$sourcename1}' />"; echo "<input type='hidden' name='ttitle[]' value='{$sourceaddress1}' />"; echo "<input type='hidden' name='sourcename1[]' value='{$income1}' />";
-
A parse error will typically provide more information than that, such as You are trying to use the "rowIDs" POST value as an array on the foreach line. You have defined two fields as "rowID" on your form - but they are not an array. They are instead two separate single entity fields. What will happen in the post data is that the second field's value will overwrite the first field's value. If you want an array to be returned you need to define the fields with array names (note the "[]" in the field name). <!--reseed attribute IDs in case of gap resulting from deletions --> <input type="hidden" name="rowIDs[]" value="{{rowNumber}}" /> Since you are having problems with the POST data names/indexes and the logic to use them, add this on the action page (for debugging purposes) so you can SEE what is being passed. echo "DEBUG POST DATA: <pre>".print_r($_POST, 1)."</pre>";
-
What is the exact error? Please copy/paste it here. EDIT: FYI, are you aware that you are using the exact same variable for all form fields ($nameError) for determining field level errors? <?php if($nameError != '') { ?> <span class="error"><?=$nameError;?></span> <?php } ?> Also, Instead of littering the markup (HTML) with PHP logic, create PHP variables at the top for the error messages, then just output those variables in the markup. E.g. //PHP code at top of script (hint, you could create a function to pass the error message to and have it return back the formatted HTML). $nameErrorOutput = ($nameError != '') ? "<span class='error'>{$nameError}</span>" : ''; $otherfield1ErrorOutput = ($otherfield1Error != '') ? "<span class='error'>{$otherfield1Error}</span>" : ''; $otherfield2ErrorOutput = ($otherfield2Error != '') ? "<span class='error'>{$otherfield1Error}</span>" : ''; //HTML markup at bottom of script <div class="form-group"> <label for="employeename">Employee Name</label><br> <input type="text" name="employeename" id="employeename" style="width:375px;" placeholder="your name..." class="form-control" value="" class="required requiredField" /> <?=$nameErrorOutput?> </div>
-
I don't think this will work: $del_tim_holder_1[count($del_tim_holder_1)] Assuming a zero based index, an array with a count of '3' would have indexes of '0', '1', & '2'. There would be no value with an index of '3'. You could instead use array_pop or, instead of having to use split/explode first to create an array, just use string functions (see example below). Also, rather than only put in the logic for the interval in the function, I would suggest just passing the $del_tim_holder_0 value and have the function do all the logic to return the applicable date string. private function calculateDate($intervalStr) { //Get value following last ':' and ensure a number $inervalWeeks = intval(substr(strrchr($intervalStr, ":"), 1)); //Convert number to weeks $intervalDays = $inervalWeeks * 7; //Create a date intervale $interval = new DateInterval("P{$intervalDays}D"); //Create current date object $currDate = new DateTime(date("d.m.Y")); //Apply the interval and convert to string $newDate = $currDate->add($interval)->format('d.m.Y'); return $newDate; } $del_tim_holder_0 = "15:18:21"; $this->date = $this->calculateDate($del_tim_holder_0); // $this->date = '13.07.2017'
-
It depends on the user workflow. When there are multiple fields needing validation, I would find it more preferable to do the validation on the form submission as opposed to triggering an error on each individual field separately.
-
I'll just say that what you are doing is probably not needed and (if you don't have similar validation on the back end) completely useless. However, your problem is that your current "test" is trying to see if the value contains the entire string of "!\"·$%&/()=?¿@#¬". You need to create a regular expression patter to look for any character. Plus, I suspect one of your other lines in the failure scenario is failing, probably this one document.signup.char_name.focus(); You should pass the form element by reference to the function instead of using getElementById() Also, you should use the onchange event - otherwise the logic will get caught in an infinite loop. When the alert is displayed, the act of the user clicking the OK will initiate another onblur event. function checkInput(inputObj) { var user_input = inputObj.value; var notgood = /[!"·$%&\/()=?¿@#¬]/; if ( !notgood.test(user_input) ) { document.getElementById('submitlink').removeAttribute('disabled'); document.getElementById('badInput').innerHTML = ''; return true; } else { document.getElementById('submitlink').setAttribute('disabled','disabled'); document.getElementById('badInput').innerHTML = 'Bad Input'; alert('Cannot use that character'); inputObj.focus(); return false; } } <input type="text" name="char_name" value="" id="char_name" required="" onchange="checkInput(this);"/>
-
Because the code is creating a new table in the loop for each record. Start the table before your loop. In the loop, output each row. Then after the loop, close the table. As this appears to be an assignment, I will leave it to you to make the modifications.
-
Read the documentation. There is no "one way" that an SDK is used. Nine times out of 10 the SDK file (or the help content/site) will contain a working example.
-
OK, after taking a look afterwards, here is another way without needing to create new objects for each team. Team 1 ID: $matchup->team[0]->attributes()->id OR $matchup->team[0]['id'] Team 2 ID: $matchup->team[1]->attributes()->id OR $matchup->team[1]['id']
-
I think your confusion stems from the fact that the "teams" are separate objects within an array of the match. There may be another way, but it will work if you assign those two array elements of the main object to two new variables/objects, then extract the data there. Also, you can reference the attributes as you have above OR via array indexes which is a little simpler, IMHO. //Load the xml content into object $xmlObj = simplexml_load_file('test.xml'); //Output the week echo "NFL Scheduled Week: {$xmlObj->attributes()->week}<br><br>\n"; foreach($xmlObj as $matchup) { //Display the matchup attributes echo "<b><u>Matchup attributes</u></b><br>"; echo "Kickoff: {$matchup->attributes()->kickoff}<br>\n"; //Object property echo "Game Seconds Remaining: {$matchup['gameSecondsRemaining']}<br><br>\n"; //Array index //Get the team objects from the team array $team1 = $matchup->team[0]; $team2 = $matchup->team[1]; //Display team1 attributes as object properties echo "<b>Team 1:</b><br>\n"; echo "</ul>"; echo "<li>ID: {$team1->attributes()->id}</li>\n"; echo "<li>isHome: {$team1->attributes()->isHome}</li>\n"; echo "<li>score: {$team1->attributes()->score}</li>\n"; echo "<li>rushDefenseRank: {$team1->attributes()->rushDefenseRank}</li>\n"; echo "<li>passDefenseRank: {$team1->attributes()->passDefenseRank}</li>\n"; echo "<li>rushOffenseRank: {$team1->attributes()->rushOffenseRank}</li>\n"; echo "<li>passOffenseRank: {$team1->attributes()->passOffenseRank}</li>\n"; echo "<li>hasPossession: {$team1->attributes()->hasPossession}</li>\n"; echo "<li>inRedZone: {$team1->attributes()->inRedZone}</li>\n"; echo "<li>spread: {$team1->attributes()->spread}</li>\n"; echo "</ul><br>"; //Display team2 attributes as array indexes echo "<b>Team 2:</b><br>\n"; echo "</ul>"; echo "<li>ID: {$team2['id']}</li>\n"; echo "<li>isHome: {$team2['isHome']}</li>\n"; echo "<li>score: {$team2['score']}</li>\n"; echo "<li>rushDefenseRank: {$team2['rushDefenseRank']}</li>\n"; echo "<li>passDefenseRank: {$team2['passDefenseRank']}</li>\n"; echo "<li>rushOffenseRank: {$team2['rushOffenseRank']}</li>\n"; echo "<li>passOffenseRank: {$team2['passOffenseRank']}</li>\n"; echo "<li>hasPossession: {$team2['hasPossession']}</li>\n"; echo "<li>inRedZone: {$team2['inRedZone']}</li>\n"; echo "<li>spread: {$team2['spread']}</li>\n"; echo "</ul><br><hr>\n"; } Output
-
ScoobyDont, Asmac_gyver is alluding to, you are not outputting the HTML correctly to have a "selected" option. It would look like this <option value ="1" selected="selected">Option 1</option> So, you need to get the currently selected value and determine which option to add that parameter to. IMO, this is easiest when the option list is dynamically created. If you have a table to define the options in the DB, then you can use that. Else, you can define an array. Then create a function to create the option list passing the list of available options and the selected option. Also, you can use the same data (DB or array) to validate that the user passed a valid value. function createOptions($options, $selectedValue=false) { $optionsHTML = ''; foreach($options as $value => $label) { $selected = ($value == $selectedValue ) ? ' selected="selected"' : ''; $optionsHTML .= "<option vaue='{$value}'{$selected}>{$label}</option>\n"; } return $optionsHTML; } Usage <?php //In head of document $jobStatuses = array( 'offsite' => 'Off Site', 'onsite' => 'In On Site', 'allocated' => 'Allocated' ); //Get the selected value $selectedJobStatus = //Some code to retrieve selected value //Create job status option list $jobStatusOptions = createOptions($jobStatuses, $selectedJobStatus); ?> <select name="team" class="formfields"> <?php echo $jobStatusOptions; ?> </select>
-
requinex beat me to it, but I'll post the sample code I was working on: <form action="" method="post"> <br/><br/> <h2>Please select issue</h2><br/> <input type="image" src="testing/images/need_toner.png" name="toner"/> <input type="image" src="testing/images/paper_jam.png" name="paper-jam"/><br> <input type="image" src="testing/images/printer_fault.png" name="printer-fault"/> <input type="image" src="testing/images/other.png" name="other"/> </form> if(isset($_POST['toner_x'])) { echo "Perform the Toner action"; } if(isset($_POST['paper-jam_x'])) { echo "Perform the Paper jam action"; } if(isset($_POST['printer-fault_x'])) { echo "Perform the Printer Fault action"; } if(isset($_POST['other_x'])) { echo "Perform the Other action"; } Replace the echo statements with the relevant code for each option. Also, the two "fields" that are returned when clicking a button (fieldName_x 7 fieldName_y) will have values for the x & y positions that the mouse clicked on the relevant image. Put this on your page to "see" how it works: echo "<pre>" . print_r($_POST, 1) . "</pre>";