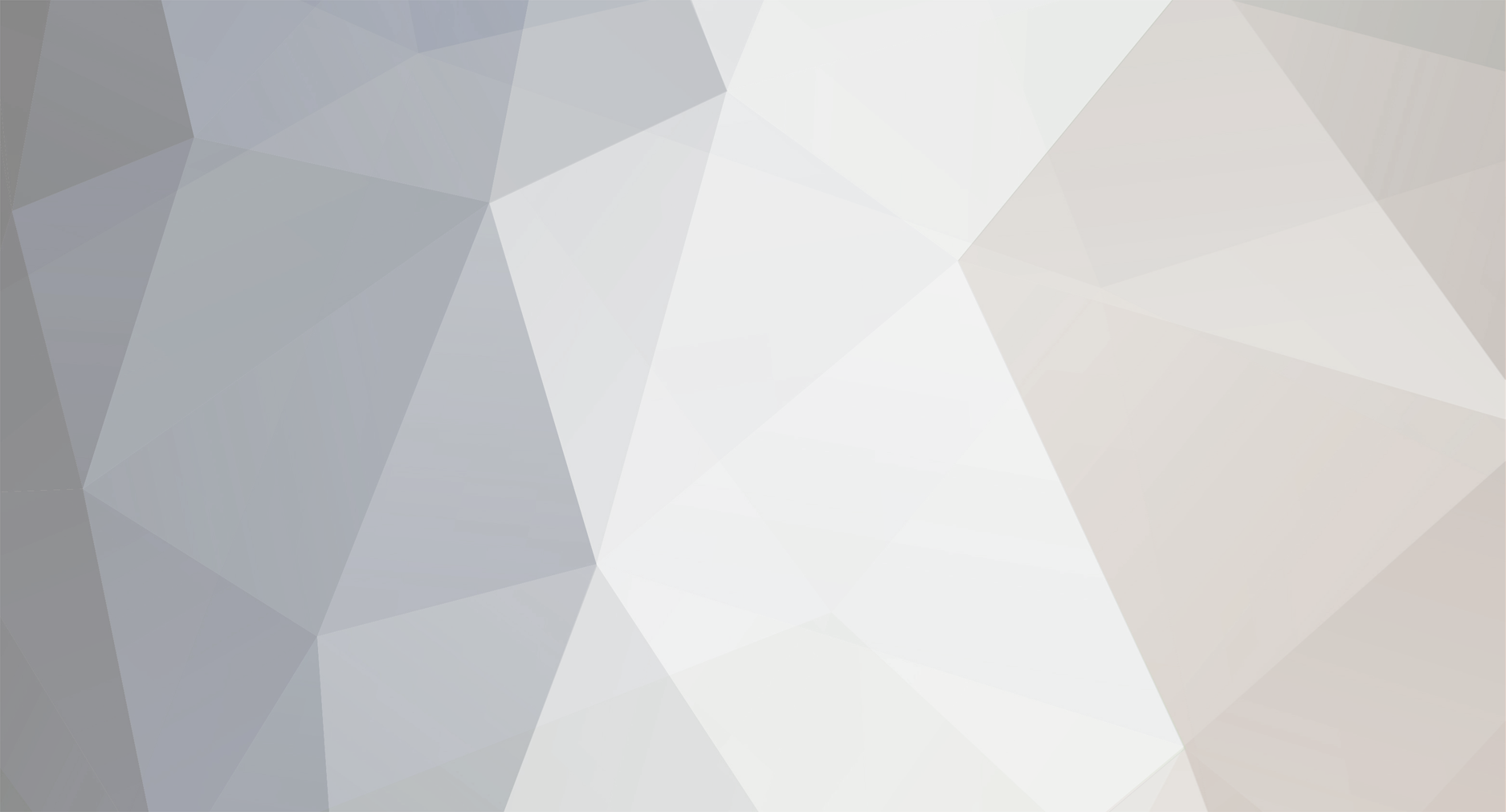
Psycho
Moderators-
Posts
12,164 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Yeah, you need to provide clarification. I';ve read your code and your request and am not sure what you are trying to accomplish. I see that you are creating some "random" records to specify "seat" which include three properties: section, row and seat no. Are you wanting to condense these so you can show a series of seats in the same section/row? E.g. "Section 4, Row 8, Seats 6-10"? Or are you just wanting to output each record in a human readable format: "Section 4, Row 8, Seats 6", "Section 4, Row 8, Seats 7", "Section 4, Row 8, Seats 8", etc.? If it is the former, I would structure the array differently. If the latter, then just loop over the array foreach($arrayVar as $seat) { echo "Section: {$seat['section_name']}, Row: {$seat['row_name']}, Seat: {$seat['seat_name']}<br>"; }
-
OK, so Google does have a product that you might be able to tie into: Vision AI. I saw in a forum post that there is an enhancement request to detect image orientation, but I don't know if it is implemented yet. It does have face recognition and I would assume that would return a rectangle. So, if there are faces in a photo, the correct orientation should have the long sides of the rectangle(s) be vertical. But, you could inadvertently turn the picture upside down. In any event, trying to explain how to use such a service is beyond the scope of a forum post. Good luck.
-
OK, let's break this down. You state you want to automatically change the orientation of an image when it is submitted. First of all, I don't see anything in that code related to image submissions. I would expect to see data relate to $_POST/$_FILES arrays on a page that handles submissions. OK, but let's say we have that code. How are you planning to determine the correct orientation to change to automatically? Functionally, it is not 'difficult' to change the orientation of a photo in PHP, but there is no functionality in PHP to "look" at a photo and know how to correct it. What you could do is allow a user to upload a photo and then present the photo back to them so they can determine if the orientation is correct. If not, give them the ability to "fix" it. This could be as simple as providing three buttons: rotate 90, 180, 270 which would just perform that one action OR you could provide some robust javascript front-end tools to 'manipulate' the image on screen and allow them to submit those changes. That would require PHP code to actually implement all the options you allow on the front-end. Here is the PHP manual for the image rotation function. But, as stated above, you have to give the code some way to "know" how to rotate it correctly. I'm sure there are some third party applications (for $$$) that you could implement. Or, Google may have something for free you could tie into to try and determine the correct orientation. But, none of that would be simple.
-
What you are wanting to do is "spoof" the from address. While there can be some legitimate business needs to do this, it can create problems that are difficult/impossible to resolve. Spoofing the from address is rather simple and is something that spammers/scammers have been doing for many years now. E.g. you might be sent an email from [email protected] as a phishing attempt. The fact that the from address looks to be a legitimate email from your bank gives the email some credibility. Because of this, there are an array of different protections that can be in place to prevent/hinder this. The crux of the issue is that you want to send an email from "[email protected]" but it is being sent though your form which is going to send it through the email server that you have configured for your form - in this case gmail.com and using the credentials of a gmail account. Generally, an email should be sent through the SMTP server that is responsible for the domain of the sending user (or through an SMTP server that has been identified as an authoritative server for that domain). You cannot control the authoritative servers for domains you do not own. Then, there can be protections on the receiving end: either in the SMTP servers or in third-party services. When an email comes in the system may do a reverse-lookup to ensure the email came from an authoritative server. If not, it gets dropped. To put it simply, you can try it. It may not work for all emails (especially if they are being sent to different domains) and there is no guarantee that it won't stop working one day because you are performing the same action as a scammer would. Having said all that, when sending an email you can specify the sender information within the headers. Here is an example of the header in a sample script of mine using PHP's mail function (this uses a "friendly" name :in addition to a specified from email address $to = '[email protected]'; $subject = "Subject of the email"; $message = "Here is body of the email message"; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; //From info $headers .= 'From: Bob Smith <[email protected]>' . "\r\n"; $headers .= 'Reply-To: Bob Smith <[email protected]>' . "\r\n"; $headers .= 'X-Mailer: PHP/' . phpversion(); $message = "Here is the message"; mail($to, $subject, $message, $headers); For your function, I suspect you would do it like this: $headers = array( 'From' => $_POST['EmailAddress'], 'To' => $to, 'Subject' => $subject ); But, for the reasons stated above, I would not advise this. "System" emails should be coming from the system/application. There are other ways to allow the recipient to respond to the requester.
-
So, now you are going to post the REAL code? How nice of wasting people's time. You still haven't done what has been suggested multiple times - output your variables so you can SEE what they are. Instead of putting your query string directly in the prepare() statement, create it as a variable. Also, the full query that you are now showing us could be the problem. Even if there is matching data an error in the JOIN criteria could prevent any results. Replace this for the relevant section in your code and look at the results to see if the query and param values are what you think they are. // // FINDTOTAL RECORDS IN SEARCH RESULTS // $placeholderStr = join(",", $placeholders); $query = "SELECT COUNT(*) as tot FROM ( SELECT id FROM ( SELECT v.id , v.title , GROUP_CONCAT(tag SEPARATOR ' ') as alltags FROM product v JOIN product_tag t ON v.id = t.product_id GROUP BY v.id ) v JOIN product_tag t ON v.id = t.product_id WHERE tag IN ({$placeholderStr}) GROUP BY id HAVING COUNT(t.tag) = ? ) found;"; echo "<b>Query:</b><pre>{$query}</pre><br>\n"; echo "<b>Params:</b><pre>".print_r($params, true)."</pre><br>\n"; $res = $db->prepare($query); $res->execute($params);
-
First, I would strongly suggest that you first use more appropriately named variables. Don't create a generic variable and then try and reuse it. What does "$params" represent? Parameters obviously, but parameters for "what"? In this case $params is an array that keeps getting additional values appended to it - and those values are logically different "types" of values. Also, a prepared query will expect an array with the same number of parameters as placeholder in the query. Second, output your variables to the page to "see" what they contain. $params does not contain what you think it does when you are using it.
-
//I broke out the code into multiple lines for readability and maintainability function insertReferences($text) { $regEx = "#(\d{3})\.(\w)#is"; $format ='<span class="btn btn-link" data-target="section${1}" onclick="showPage('section${1}')" data-parent="#page">${1}.${2}</span>'; return preg_replace($regEx, $format, $text); }
-
I think the best solution is RegEx [specifically preg_replace() or preg_match()], but to provide a solution would require the "specs" for the article references. You gave one example where the reference was three digits + period + the letter 'f'. Do they always start with a series of digits? If so, what is the minimum/maximum number of digits? Are the digits always followed by a period? Is the period always followed by a letter? If so, what are the valid letters and are they always lower case? Also, how is that reference supposed to be modified to a URL? I.e. what would the URL look like for your example of '105.f'? Here is an example function insertReferences($text) { return preg_replace("#(\d{3})\.(\w)#is", '<a href="displayArticle.php?id=${1}&type=${2}">${1}.${2}</a>', $text); } //Text from DB $articleText = 'Beginning text and then a reference 105.f to another article'; //Modify text to include hyperlinks $outputText = insertReferences($articleText); //Output the result echo $outputText Output: Beginning text and then reference <a href="displayArticle.php?id=105&type=f">105.f</a> to another article
-
Please read requinix's earlier response. If you are still getting the full output with your code above, that is because the function wpjm_the_job_description() is outputting the content to the page and not returning it. E.g. wpjm_the_job_description() { $value = "Get some text from some process or source to be displayed"; echo $value; //The function is directly outputting text to the page return; //Nothing is returned from the function for you to modify it } You will either need to see if there is a function to get the string rather than outputting it or you can try modifying that function directly.
-
How to split a search strings and put it into a prepare statement?
Psycho replied to bbmak's topic in PHP Coding Help
If you want to do this with mysqli, there is a comment in the documentation with an example class: http://www.php.net/manual/en/mysqli-stmt.bind-param.php#109256 But, PDO is the way to go.- 6 replies
-
- search string
- split
-
(and 1 more)
Tagged with:
-
Select & checkbox values don’t show up in submitted form
Psycho replied to ThatGuyRay's topic in PHP Coding Help
To add to benanamen's response, it is not valid HTML markup to have multiple elements with the same id as you have with the checkboxes. The id for each element must be unique. <select id="choose" name="choose"> $vehicles = implode(', ', $_POST['vehicles'] ); $choose = $_POST['choose']; -
Where do you put opening curly braces when defining a method?
Psycho replied to NotionCommotion's topic in PHP Coding Help
Someone asked a similar question a long time back and someone pointed them toward the PSR coding standards. So, that's another option: http://www.php-fig.org/psr/psr-2/ -
What IS the problem? What are you expecting the code to do and what is is actually doing? One potential problem is that you have a scenario in which no value would be defined. But, since I have no clue on how your conditions are generated I have no idea if that is your problem. As to your code, my first suggesting is to stop injecting a mass of PHP logic into the HTML like that. Put your PHP logic at the top of your script and generate the dynamic content into a variable. Then output that variable in the HTML. EDIT: I do see a couple possible errors in your code. Look at the comments in the below revision example Example: <?php //Create code to determine value of the input field if (isset($_SESSION['user'])) { //This scenario ASSUMES that fname_bill is set, but the condition only tested //to see if user index in the Session is set. $firstnameValue = $curd->word($userRow['fname_bil'], 'UTF-8'); } elseif (!empty($_SESSION['auto_fill']['bil_adr'])) { //The condition is checking if the array index is NOT SET, but then //you try to reference that array index in generating the value $firstnameValue = $crud->word($_SESSION['auto_fill']['bil_adr']['cos_fis'], 'UTF-8'); } else { //Your original code had no logic to set the value based on neither //of the above two above conditions being true $firstnameValue = "?????"; } ?> <html> <body> <input type="Text" name="sen_add_dat_bil_fir" maxlength="200" required="True" placeholder="Firstname" value="<?php echo $firstnameValue; ?>"> </body> </html>
-
<?php $recordsPerPage = 24; $adPosition = 12; $limitStart = $recordsPerPage * ($pagenumber - 1); $sql = "SELECT * FROM content WHERE `live` LIKE '0' AND '{$today}' > `date` ORDER BY date DESC LIMIT {$limitStart}, {$recordsPerPage}"; $result=mysql_query($sql); $recordCount = 0; while($rows=mysql_fetch_array($result)) { //Increment the counter $recordCount++; //Code to display the current record goes here ## ## //Check if this is the 12th record if($recordCount == $adPosition) { //Code to display the ad goes here ## ## } } ?>
-
I agree with Jacques1. You really need to put some time into learning better practices. This is all very basic stuff. But, I'll be very generous and point out some of the problems. 1. Do not use the mysql_ extensions. They are no longer supported. You should be using mysqli_ or, better yet, PDO for database operations. 2. You should be using prepared statements for your queries with placeholders for any variable values in the query. This will prevent SQL injection (such as you are having). NEVER put user entered data directly into a query 3. You appear to be storing the password as plain text. Could you please provide me a list of any websites that you work on now and in the future so I can be sure to never sign up on them? </sarcasm>. You need to store the password as a hash. Then at login, hash the user input password and compare it to the stored hash. Do not use a simple MD5() or other hash. Use the built in PHP functions [password_hash() and password_verify()] or a properly vetted framework such as phpass. 4. I don't even know what this line is supposed to do. It should produce an error and even if it didn't the intent is unclear. I think you are trying to store a session value related to the password. There is no good reason to do this. hash($_SESSION["pass"] = $row['pass']; //<== Where's the closing paren??? 5. The is_array() check is meaningless. An empty result set would still return an (empty) array. You shoudl instead check if there was a record returned, Here is a resource to get you started on using the PDO extension and prepare queries: https://phpdelusions.net/pdo
-
You have duplicate data for 'text' and 'value' in each record for your example array, so I'm not sure which one you are wanting to use. Modify as needed foreach($productArray as $idx => $product) { echo $product['text'] . "<br>\n"; }
-
I'm sure you could do it with a query, but I think it would be complicated. Similar to determining a "rank", but you would have to keep track of win counts and start end dates. How many records will be applicable to any one request? If it is not really huge, just pull all the relevant records and determine the win streaks when iterating over the results. Not tested, but I think the logic is sound //Run query for all the records ordering by date // - 'win' will be a Boolean (1 or 0) $query = "SELECT date, (pointsfor > pointsagainst) as win FROM table_name ORDER BY `date` ASC"; $sth = $dbh->prepare($query); $sth->execute(); $result = $sth->fetchAll(); //Variable to hold the longest streak data $longestStreakCount = 0; $longestStreakStart = ''; $longestStreakEnd = ''; //Variable to track 'current' streak counts $currentStreakCount = 0; foreach($result as $row) { //Check if current record IS a win if($row['win']) { ## CURRENT RECORD IS A WIN //If first win, set start date for current streak if($currentStreakCount==0) { $currentStreakStart = $row['date']; } //Increment the win count for current streak $currentStreakCount++; //Set a 'lastWinDate' (used to determine the end of streak after 1st loss) $lastWinDate = $row['date']; } else { ## CURRENT RECORD IS NOT A WIN //Check if $current Streak is greater than the longest streak so far if($currentStreakCount > $longestStreakCount) { //Set new values for longest streak $longestStreakCount = $currentStreakCount; $longestStreakStart = $currentStreakStart; $longestStreakEnd = $lastWinDate; } //Reset current streak count $currentStreakCount = 0; } }
-
Join two tables, but only get single results from other table
Psycho replied to Strahan's topic in MySQL Help
Use MAX() to get the last date alone with a GROUP BY clause SELECT m.fileid, m.filename, MAX(w.watched) as last_watched FROM media m LEFT OUTER JOIN watchlog w ON m.fileid = w.parent WHERE m.parent = 6816 GROUP BY m.fileid ORDER BY filename -
There was a typo. User this: $selector = (isset($_GET['selector'])) ? $_GET['selector'] : false;
-
mac_gyver's solution is a good one too. One benefit of that solution is that you can easily change the options without having to touch the code/logic. You could even put the data in a separate (included) file. One assumption that he made in his example is that the passed value will necessarily be the value at the end of the URL and that all the URL will have the same base content. I would not make that assumption. Here is a revision of his code with some comments. // Define the choices in a data structure // This array can be defined at the top // of the page or in an included file $choices = array( 'airflow' => array( 'href' => '/folding-beds/browse-by/airflow', 'text' => 'Beds with Airflow Fibre Mattresses' ), 'deluxe' => array( 'href' => '/folding-beds/browse-by/deluxe', 'text' => 'Deluxe Collection' ), 'supreme' => array( 'href' => '/folding-beds/browse-by/supreme', 'text' => 'Supreme Collection' ) ); //Define the selection based on passed url parameter (or false if not set) $selector = isset($_GET['selector']) ? $_GET['selector'] : false; //Check if there is a value in the defined array for the $selector //If not, define the first key as the selector if(!isset($choices[$selector])) { $selector = key($choices); } //Define the text and href using the selector $href = $choices[$selector]['href']; $text = $choices[$selector]['text'];
-
Use this $label = trim($label['name']); Code should be separated based on its logical function/purpose. I would first start by separating the logic (i.e. PHP code) and the presentation (i.e. HTML content) into separate files. The presentation should just have code to output PHP variables that were generated within the logic. For new/casual programmers it is easiest to first start with what I proposed above - putting all the logic at the top of the script. In my opinion, people new to programming find it difficult to conceptualize the entire process when dealing with multiple, independent files and it is easier to work with a single field that creates the complete page. As you gain experience you should start moving any logic that is used on multiple pages into separate file(s). For example, if you make database calls, you should have a single file that creates the database connection. If you create multiple select lists dynamically, I would create a function that accepts tow parameters (an array with the value/label and (optionally) the currently selected value) and that function can build and return the HTML content for those select options. Once you have a better understanding of using multiple files, classes, etc in development then you can start using an MVC framework or something similar.
-
Better is subjective. If you are only going to have a couple of options then a single if/else condition is fine. You don't need two conditions for 'airflow' and '' since you want them to do the same thing. But, if there will be more options - or if you think it may grow, a switch() condition may be better. However, one problem I do see is that there is nothing defined in the case where 'selector' is not passed or if it does not equal one of those three values. Examples below Option 1 (only two end results): if(isset($_GET['selector']) && $_GET['selector']=='supreme') { $href = '/folding-beds/browse-by/supreme'; $text = 'Supreme Collection'; } else { //Values if 'selector' not set or does not equal 'supreme' $href = '/folding-beds/browse-by/airflow'; $text = 'Beds with Airflow Fibre Mattresses'; } Option 2 (if there will be multiple results): //Need to define value conditionally on if it is set to prevent //error referencing undefined value $selector = (isset($_GET['selector']) ? isset($_GET['selector'] : false; //Switch statement to define different conditions based on $selector value switch($selector) { case 'supreme': $href = '/folding-beds/browse-by/supreme'; $text = 'Supreme Collection'; break; case 'deluxe': $href = '/folding-beds/browse-by/deluxe'; $text = 'Deluxe Collection'; break; //If $select = 'airflow' or default: if did not meet any previous conditions case 'airflow': default: $href = '/folding-beds/browse-by/airflow'; $text = 'Beds with Airflow Fibre Mattresses'; break; }
-
His point is that (if the application is properly built) exposing the ID should not pose a security threat. If so, the application should be fixed rather than relying upon obfuscation as security.
-
What is this supposed to be doing? $lender_list = ($lender_list); Pro tip: create your logic (i.e. the core PHP code at the top of the script. Then output within the HTML at the bottom of the script. <?php //PHP Code at the TOP of the script //Create variable to hold the lender options $lenderOptions = ''; //Iterate through the array of lenders foreach($lender_list as $value => $label) { //Trim the value $label = trim($label); //If label is empty skip this record if(empty($label)) { continue; } //Create new option for the current lender $lenderOptions .= "<option value='{$value}'>{$label}</option>\n"; } ?> <html> . . . . <select name="some_field_name"> <?php echo $lenderOptions; ?> </select> </html>
-
So, is the scenario that the user selects to edit a record, but then decides they don't want to edit that record and wants to go back to the list of records? For that scenario, I typically implement a "Cancel" button on the edit form that will take the user back to the selection screen. Since your selection list is apparently based on a prior criteria input you could save that input as a session value. Then, clicking the cancel button will pull the search value from session and regenerate the selection list.