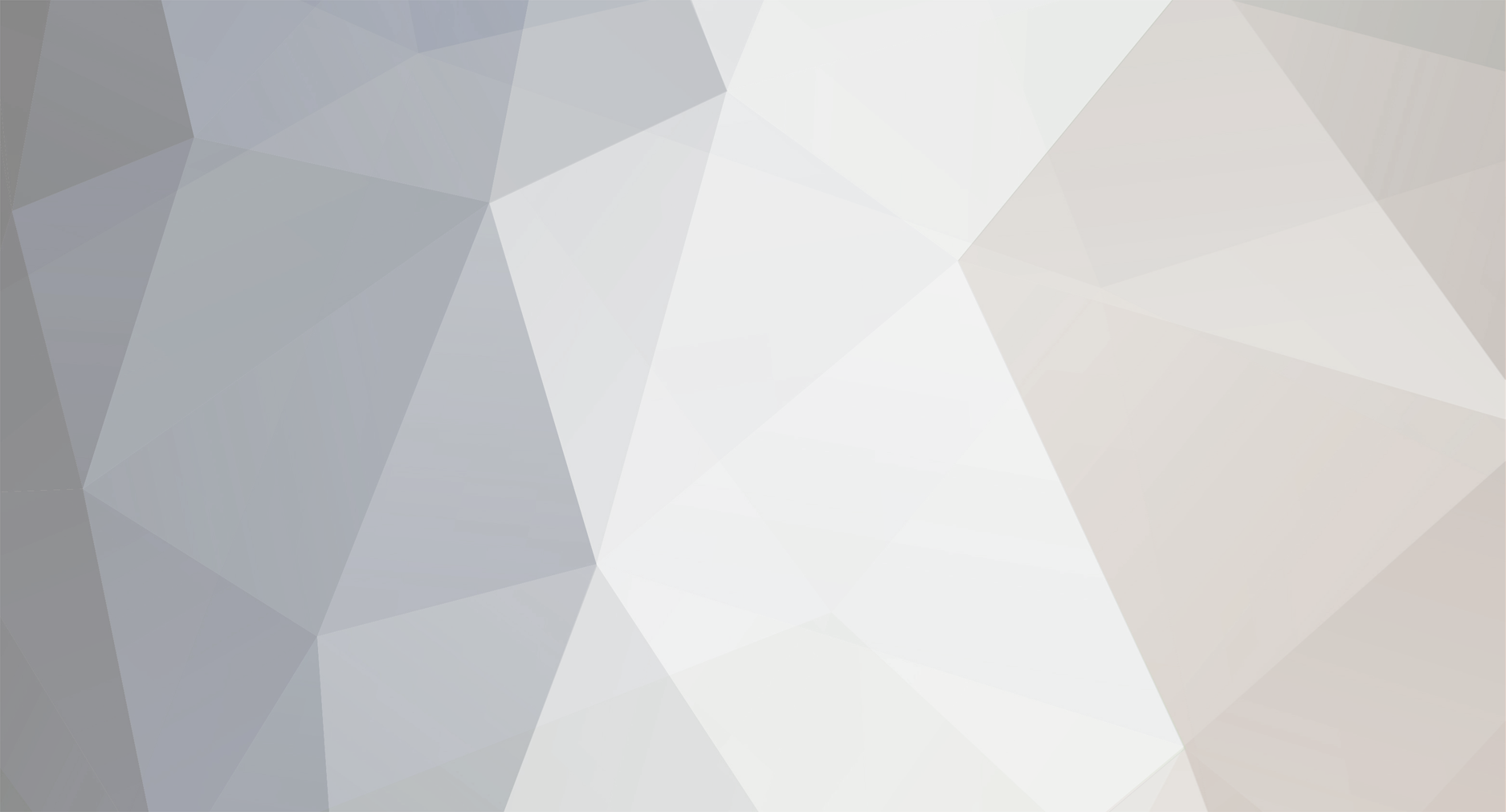
Psycho
Moderators-
Posts
12,164 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Adding additional logic to existing triggers is a pretty simple process. You could add the logic in the current function that is called or create an additional function. However, you have provided no code as to what is currently occurring. So, it's kind of hard to provide any suggestions. We'd need to see how the onchange trigger is implemented (in line, onload, etc.) and what is currently getting passed. For example, if the function that is called has the "value" of the clicked option, then you could simply call another function from within that one to do the additional work //Existing function function registrationPrice(registrationType) { //Call new function to show appropriate fields showField(registrationType); //... rest of code for registration price } //new function function showFields(registrationType) { switch(registrationType) { case 1: //Show hide relevant fields break; case 2: //Show hide relevant fields break; case 3: //Show hide relevant fields break; default: //Fail-safe condition. Hide all fields break; } } However, you may have a bigger issue based on the above. Any price calculation on the client side should be strictly cosmetic and not used to send to the server. Any important calculations should always be calculated on the server based on the parameters that are received by the user. It is a trivial task for a user to change any values submitted by a form whether they are select lists, hidden fields, etc. So, it is fine to have client-side logic to provide the user additional information and usability, but never rely upon JavaScript to enforce business logic. E.g. If option B is disabled when option A is selected. A user could very easily pass a form with both options selected. Even though it is a good idea to disable options based on other input from a usability stand point, the server-side logic should check for ALL error conditions and reject invalid data (with appropriate messaging to the user).
-
I would take that a step further. If the value for id is passed - but is NOT the value you think it is, the logic would still attempt to perform the query, but nothing would be returned if there is no matching id. Also, no need to include the id in the select list of values and assign it to a value - since you already have the id. I would suggest trying this: //Debug lines echo "Var dump of POST['id']: " var_dump($_POST['id']); echo "<br>\n"; //Trim the id - if passed, else false $id = isset($_POST['id']) ? trim($_POST['id']) : false; if(empty($_POST['id'])){ echo 'ID is missing'; } else { # set form input fields $sql = 'SELECT id, name, email FROM author WHERE id = :id'; $query = $dbConnection->prepare($sql); $query->bindValue(':id', $_POST['id']); $query->execute(); $row = $query->fetch(); $name = $row['name']; $email = $row['email']; }
-
What I see in that code is that it appears to be creating three TD elements and changing which one in which the one containing the button element will exist based on the value of $rand. So, what is your problem? Since the other two TDs are empty it's difficult to know how the page would look without knowing the rest of the definition of the table. But, my guess is that the value of $rand is not what you think it is. Plus, that code is poorly written. Never copy/paste code when you want the same general results with a small variation (i.e. the button in a diff position). You could very easily have a typo in one scenario and not see it unless you adequately test every possible scenario. But, all that code does is change the position of the button in the table - it won't function any differently. EDIT: It was my understanding that the JQuery AJAX components were built to prevent the issues with caching and the need to pass a new parameter. I may be wrong though. EDIT #2: From the JQuery documentation for ajax() However, it looks like that parameter is not supported in the $.get() method for JQuery, so you should go back to the $.ajax() method and use this parameter instead of creating your own method to prevent caching.
-
Check if variable is a two element unassociated array
Psycho replied to NotionCommotion's topic in PHP Coding Help
I would also add to Jacques last post that readability of code is not a trivial concern. Using good practices when writing code saves many, many hours of wasted hours in varying ways. From least to most beneficial, I would rank it as follows: 1. When actively writing new code you don't have to try and "think up" new acronyms to use for variable/function names that don't conflict with others that may already exist that you can't remember. If I need to define a variable for the first day of the current month for a reporting module, using $fom is a bad choice, whereas $firstDayOfCurrentMonth is a much better choice. The amount of extra disk space or execution is too small to even consider. 2. If you need to revise or debug existing code you will spend an inordinate amount of time trying to understand what you wrote before because the names are not intuitive. If you have a problem with a function with parameters such as $ac, $fuj, $tor, you will have to go back to where the function is called to understand what the values are that are passed to the function - which may require you to go back even further to assess the logic on how the values were derived. 3. Most importantly, when anyone else has to review/revise your code they won't spend hours/days/weeks trying to figure things out. Heck, if you are properly naming and using comments you will be able to copy/paste a section of code into this forum with a description of what your problem is and what you want to achieve and get a good answer quickly. I.e. no need for multiple back-and-forth posts to try and figure out what the heck your code is trying to do. Lastly, don't be afraid to use comments liberally. If some code you write was not very simple and took some thought to develop, chances are you won't remember how or why you ended up with the final code. If for example, you have to fix a bug where a division by zero error occurs - then spell it out where you implement that fix. -
One reason why you question makes no sense is that "128" can be a valid float value. var_dump(floatval(128)); //Output: float(128) If you must have the value "formatted" in a way that "looks" like a float (which seems doubtful) you can force the format using something like number_format(); $number = intval(128); echo number_format ($number, 1); //Output: 128.0 EDIT: Since you need to do that with array values you could apply that using array_walk() to all the values as needed.
-
Either you didn't make all the changes Barand mentioned or you are not testing on an actual web server. If you have an HTML page that you are opening through your file system, the AJAX will fail (at least I know it does in Chrome) - for security reasons. You need both the user page and the server page to be on a web server. Or, the URL for the request is not correct. You can also simplify things with the $.get method Client page <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script> <script> $(document).ready(function() { $("button[name=data]").on( "click", function() { $.get("http://localhost/test.php?mid=1&random=2040187", function(data, status){ $('#summary').html(data); }); } ); }); </script> </head> <body> <form method="get"> <button name="data" type="button" onclick="getData()">Click</button> </form> <div id="summary"></div> </body> </html> Server page <?php $order = $_GET['mid']; echo $order; exit(); ?>
-
Here's some revised code. Note that I created a new onchange process for the select list. The onchange is hard-coded to that specific select list, but the code is written to be adaptable to other select lists (which I assume you might have for different radio button options). If that is the case, change the identifier for the onchange event to apply to all of the select lists that you may want the same functionality for. Also, I made the first option in the select field enabled as it may have been the cause of part of your problem depending on the browser. I would either enable the "instruction" option or remove it. It was working for me when disabled (it would default to 1 when first clicking the radio button option), but I could see where there could be a browser specific issue. <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script> <script> //Displaying select box when type radio button is clicked $(document).ready(function() { $("input[name=vertical]").on( "change", function() { var fieldGroupName = $(this).val(); var fieldGroupObj = $("#"+fieldGroupName); var selectListObj = $("#"+fieldGroupName+"_select"); fieldGroupObj.show(); $('#Pricelist_select').trigger("change"); } ); $("select[name=Pricelist]").on( "change", function() { var selectID = $(this).attr('id'); var selectValue = $(this).val(); if(selectValue != "") { $.cookie(selectID, selectValue); } } ); }); </script> <style> #Pricelist { display: none; } </style> </head> <body> <div class="row"> <div class="col-md-6"> <div class="radio clip-radio radio-primary"> <input type="radio" id="radio3" name="vertical" value="Pricelist" /> <label for="radio3">Pricelist</label> </div> <div id="Pricelist" class="desc"> <div class="form-group"> <select class="cs-select cs-skin-elastic" name="Pricelist" id="Pricelist_select"> <option value="" select="selected">Select pricelist</option> <option value="1">1</option> <option value="2">2</option> </select> </div> </div> </div> </div> </body> </html>
-
Check if variable is a two element unassociated array
Psycho replied to NotionCommotion's topic in PHP Coding Help
What is the source of the data? Why are you concerned that the returned data may have indexes other than 0 and 1? And, if it does get returned with indexes other than 0/1 is there a possibility it is correct, yet the process changed to send back different indexes? Why would you want your application to break if that happens? Why aren't you more concerned with the values in the array? FYI: There is no reason to use the strict comparison for the array length count($a)===2. It's impossible for that function to return a string of "2". Just check that the the value is a two-dimensional array and force the indexes. Then, more importantly, validate the values in the array before you use them. Plus, you can make the length configurable so you can re-purpose, if needed. function checkArray($arr, $len=2) { if(!is_array($arr) || count($arr)!=$len) { return false; } //Validate the values . . . } -
There are several problems in that code that make no sense. You've only implemented an onChange event on the radio button. When selected it: 1. Hides the "desc" element 2. Dynamically shows the select list based on the value passed frm the clicked radio button 3. Makes a call for the value of the select list (using hard-coded id) - but does nothing with the value, so that line has no purpose 4. Triggers an onChange event for the select list (using hard-coded id). 5. Does an alert of the selected value (again using a hard-coded id). 6. Sets a cookie using the selected value of the select list (again using a hard-coded id). Here are the problems: 1. The function starts by using the value of the passed radio button to dynamically show the select option, but then all the remaining code has hard-coded identifiers. The code should be dynamic or hard-coded - not both. 2. Not sure why you make a call for the value of the select list, but don't do anything with it. That line has no functional purpose 3. There is no onChange function defined for the select list. So, the fact that you trigger an onchange for it has no purpose. 4. Since the above is executed when the radio button is clicked, the initial value of the select list will be the default value which, in this case, is an empty string for a disabled option. This is what is getting saved to the cookie. Create a separate onchange event for the select list and use THAT to save the value to a cookie.
-
NO, the explode is not exploding as expected. It must be using something other than a space to separate the values. You will need to inspect the actual characters to know what to explode the data with. It might be a tab character ("\t") or some other white-space character. Output the variable $output to the page and then inspect the HTML to see if you can ascertain the actual character used as the delimiter. Post the actual HTML source here.
-
I have similar questions to Barand. Not sure what the query to get the last_action from the users table is for. It look slike you want to use that to determine who was active in the last "year" from that date. If your application/game is going to have long periods of inaction by any users, maybe it isn't successful? I would just query using all users where their last action was within one year from the current date. Also, you state that last_active is set using time(). I assume you mean the PHP function time that returns a complete timestamp (which also identified a date) as opposed to the MySQL function that only returns a time (irrespective of date). If so, you are doing it wrong. Let MySQL do the work for you. Set the field up as a timestamp in the database with an onchange trigger to update the field automatically when making changes to the records. But, that means the table should only be used for tracking user activity since making any changes to a record (an admin updating banned status would update that field). So, the activity tracking should be in its own table. But, if you want to use the users table, then you should update that value using the MySQL function NOW() as the value for the last_active field. As I interpret your code, your current query would be returning all records where the last active date is less than (?) the "year" for the date you grabbed in the first query which, as Barand pointed out, has no relevance. You should probably be using something like this: SELECT COUNT(id) as userCount, SUM(money) as totalMoney, SUM(exp) as totalExp FROM users WHERE banned = 'n' AND active = 'y' AND last_action >= DATE_SUB(NOW(), INTERVAL 1 YEAR)
-
But, your question was about the CODE. You first need to determine what the user experience will be, THEN determine how it should be coded. I don't know what the images are for, how often users would be expected to be adding/changing/removing them, how the users will "use" the images, etc. etc. So, I cannot provide any advise on what the user experience should be.
-
AzeS, I think you need to start by understanding what the intended actions are for the user - then build accordingly. What I believe I understand from the prior posts is that users are going to be updating their profile and that the profile can apparently have multiple pictures. What is not clear is whether the number of pictures is dynamic or fixed. For example, if you allow a user to have an avatar, a logo and a signature image, that is a fixed number of images. But, if you allow the user to upload multiple avatars that they can then cycle though, that would be a dynamic number. How you let the user manage those could be very different. If you have very specific entities for images (1 avatar, 1 profile, etc), then the form for the user profile should just have a single file field for each image entity. You could then have a single class to process any form submissions for user profiles. In that class it would check each of those file fields and (if anything was passed) run through the code to upload the image. If the upload succeeds, then include the image reference at the end of the class when running a SINGLE update query for the user profile to include the input fields as well as the images. However, for images, if the user does not pass a file for any image you should keep the existing entry (if there is one). You should provide a separate process for removing images. If you are going to allow an arbitrary number of images, then you need to figure out the workflow from the user's perspective. Do you want to allow them to upload multiple images at one time or only one image at a time. This might be a scenario where you have a special area to manage images. I can't really say since I don't know what you are doing.
-
Based on your first response you are getting a string with 6 pieces of data separates by spaces. There are three pairs of data (IP and MAC). Step 1: Split the data into an array of six elements $deviceData = explode(' ', $output); Step 2: Convert the array into separate elements for each device by 'chunking' it $devices = array_chunk($deviceData, 2); You should now have a multi-dimensional array with each sub-array representing a single device. Each sub-array would have two elements, the IP and the MAC address. Step 3: Now you can loop over those elements and validate that the pieces of data are valid and insert/update into your DB foreach($devices as $device) { $deviceIP = $device[0]; $deviceMAC = $device[1]; //Validate the values and then (if valid) insert/update into DB }
-
That is not what print_r() would output. Do you actually have an array? If so, we need to see the structure. Or, are you getting a string (which is what it looks like by what you posted)?
-
You have to be very careful about any calculations during the changes due to daylight savings to guard against tearing a hole in the space time continuum. That is why you would want to use a timestamp (not a datetime) field. A timestamp is the number of seconds since Dec. 1(?), 1970. The "date" you might see in the database is simply the extrapolation of that timestamp into a representative value based on the servers timezone setting. So, if you do have a timestamp from the data, you are good, else you have no way of knowing which record is for which hour when the clocks go back. Although, if there is one record per hour (or a set number) I suspect you may be able to assume the first record was the first hour and the second was from the hour after the clocks were set back.
-
What happens if I go back to pages I "liked" for him previously and remove the like?
-
My users always have to refresh multiple times after deployment
Psycho replied to DeX's topic in Javascript Help
I've used the same hack(s) for css files as well. -
My users always have to refresh multiple times after deployment
Psycho replied to DeX's topic in Javascript Help
It sounds as if your main problem is with Javascript files. Are you storing "data" in those files? If so, the data should be captured dynamically (e.g. AJAX call) instead of hard-coded in a JS file. Also, while Requinix is giving you the right answer, there is a very simple "hack" that will solve this problem. Change the extension of your JavaScript include files to a file type that the server will know needs to be retrieved every time - e.g. php, aspx, etc. Those pages should not be cached. A Javascript fiel included with a script tag does not have to have a js extension - it can be anything. <script src="myjavascript.php"></script> However, it also means those pages will be sent to the parsing engine each time. Not exactly efficient, but for an internal site it probably would have no impact. Another hack, which may work, is to put a random parameter at the end of the URL <script src="myjavascript.js?randomkey=12345"></script> Again, these are hacks to provide a quick and dirty temporary solution until you can configure and test the server settings. -
I can't see that that error would have anything to do with defining a two element JSON array. Based on a couple quick searches it could be caused by an infinite loop in the JavaScript or the redefining of elements within a loop. Just like you were trying to output the array on each iteration of the PHP loop, check that you aren't doing something similar in the JS code.
-
So, what's the problem? That is correct JSON format. If your issue is with the empty fields (lat & lng) those were already empty in your previous output, so converting to JSON will not put values there. Either the fields (event_lat & event_lng) are empty in those records or those are not the correct field names. Here's that same data in a more readable format [ { "lat":"", "lng":"", "name":"Lobster Louie's Truck", "address":"300 Pine, San Francisco, CA 94104", "place":"300 Pine", "hours":"8:00am - 10:00am", "location":"Located at the corner of pine and 3rd." }, { "lat":"", "lng":"", "name":"Lobster Louie's Truck", "address":"Terry Francois Blvd, San Francisco, CA", "place":"The Yard at Mission Rock", "hours":"11:00am - 3:00pm", "location":"Located at the Yard" } ]
-
And what is the current output with the above code?
-
What you are after is a JSON encoded array. http://php.net/manual/en/function.json-encode.php Plus, it is showing three entries because you have the print_r() within the loop that is creating the records. So, on the first iteration you add the first element to the array and output the array (with just that one element). Then on the second iteration of the loop, you add a second element to the array and output the array again (which now holds two elements). 1 + 2 = 3. move the print_r() outside the loop to see the final contents of the array.
-
Opinion: strip_tags on field that will be encrypted in the database
Psycho replied to LLLLLLL's topic in PHP Coding Help
There is a banking site I have to use that is tied to my health insurance. When creating my security questions, one was "What's your favorite band?". I provided the answer "U2" and went along my way. Fast forward many months to when I needed to reset my password. They provided that question above and when I entered the answer and submitted it I was greeted with the error message that my answer was too short. I am in total agreement with Jacques1. You should never change a user's input. Plus, in the vast majority of instances, it isn't necessary to disallow certain characters for 'security' at all. If you are doing that, it's probably because the code isn't written securely to begin with. Sort of like creating a lock that could be jimmied with a pencil and proclaiming no one may have a pencil - instead of just fixing the lock. -
Not sure what ON DUPLICATE would help with since you are updating records. Your original title was about INSERTS, but you asked to have the title changed about UPDATES (which I did). So, the "ON DUPLICATE" wouldn't apply. Creating a massive UPDATE query with case statements would make problems very hard to debug. I would stick with a single prepared statement (make sure the emulate prepares parameter is set to false when creating the connection to the DB). That way if any individual updates fail, you can log and take any action needed. Breaking it up into chunks is a good idea though. However, if all of that doesn't work, I can think of another option - depending on the population of the values to be updated. If the possible values to be updated are not huge you could create a process that updates all the records with a C1 value of "1", then all the C1 values of "2", etc. Then, do the same for the C2 values. This assumes you have the data in a way that can be easily manipulated to determine those groups of records. Run something like this in a loop, changing the c1 value and the list of primary keys. UPDATE t SET c1 = 1 WHERE pk IN (2,4,15, 17, 22, 34, 66)