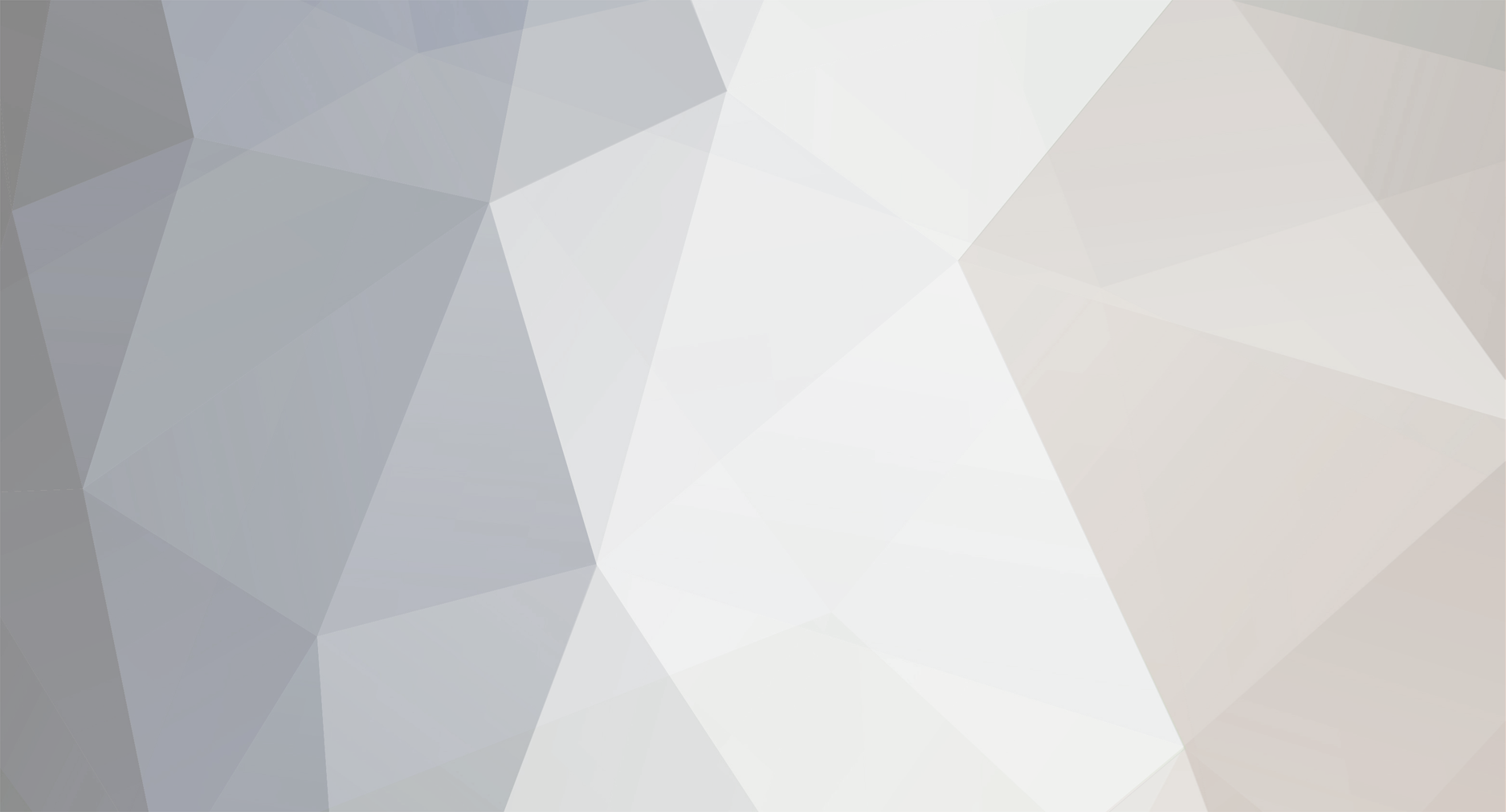
Psycho
Moderators-
Posts
12,164 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
First, STOP using the mysql_ functions. They are no longer supported in current versions of PHP. Instead use the mysqli_ functions or, better yet, PDO for database operations. Second, learn how to use prepared statements instead of trying to manipulate the data before running the query using things such as mysql_real_escape_string(). Prepared statements will prevent SQL Injection if done properly.
-
looping results from query and limit amount per row
Psycho replied to jacko_162's topic in PHP Coding Help
This is unnecessary // complete a partial row $count = count($chunk); if($count < $items_per_row) { echo str_repeat("<td> </td>\n", $items_per_row - $count); } All you need is this // complete a partial row echo str_repeat("<td> </td>\n", $items_per_row - count($chunk)); If the chunk contains 5 items (or the same number as $items_per_row, the multiplier will be 0 and no empty cells will be output. -
I'm not sure, but you are using the SELECT statement to populate a temporary table. I don't think it is going to return the data. I would think you would then need to run a second query against that temporary table to retrieve the data.
-
How/What is the correct way to create a function for MS SQL Queries.
Psycho replied to kat35601's topic in PHP Coding Help
Yes, you do have a target. You are creating it with a more complicated logic using datediff() and getdate(). I just gave a high level example, if you wanted records for the current month it would look like WHERE DATEPART(yyyy, tdate) = DATEPART(yyyy, getdate()) AND DATEPART(mm, tdate) = DATEPART(mm, getdate()) But, per your original request you asked So, I provided how I would do it. You never asked about getting totals for the periods. If you want totals for particular periods you would use GROUP BY using similar logic to what I provided. As stated before, the ISO_WEEK will work if your weeks align with the start/end of ISO_WEEK. Else, you are going to have to create some logic to appropriately group the weeks as you want them. -
How/What is the correct way to create a function for MS SQL Queries.
Psycho replied to kat35601's topic in PHP Coding Help
For records in a particular month, I would use something like YEAR(datefield) = targetYear AND MONTH(datefield) = targetMonth For records within a particular week, you can do something similar if your weeks are aligned with ISO_WEEKS -
When using UNION, the queries MUST have the same number of fields and the same field types (in the same order). The two queries you are trying to UNION fail just from the first fields. The first field in the first query is a team name, but the first field in the second query is a count. And, from what I deduce you should not be using UNION. It is used to combine records from multiple queries where each record is independent. I think you mean to use a JOIN. But, it appears you are trying to calculate data and store in another table. That is a bad practice. Just get the calculated data from the source tables when you need the data.
-
What I would suggest is to go through code a line at a time. For each line see what function(s) are used and/or the data. Look up the functions in the manual and understand what is happening on that line of code. Then go to the next line. Sometimes you will have to "go back" to previous code to understand the current line you are on. I think it really helps to learn logic that way (assuming the code has good logic). However, if you have someone that has a line of code that does 10 different things, it can be hard to decipher into something meaningful
-
Some notes: The function randColor() is used to create a random color. As Barand explained, when defining colors in an HTML page, they are composed of RGB (Red Green Blue) values, with each component having a value from 0 to 255. FYI: The same RGB process is used in many different contexts to define color. The function defines three variables for those three components with a random value from 0 to 255 using the rand() function. The function then takes those three values and composes them into a string format which can be used in the HTML output. A variable ($tdata) is defined to store the output for the table (i.e. 'tdata' is the table data). There are two loops that use a for() loop. The for loops 1) set an arbitrary variable ($i or $j) to 0, 2) Create a condition to continue the loop as long as the variable is less than a certain value, and 3) increment the variable on each iteration of the loop. for ($i=0; $i<10; $i++) { The first iteration of this loop will set $i equal to 0. The loop will continue as long as the condition of $i being less than 10 is true. $i will be incremented by 1 on each iteration of the loop. Therefore the loop will run 10 times. The outer loop is for the rows of the table and the inner loop is for the columns in the rows (there will be 10 rows). The outer loop adds an opening row tag to the variable to store the output. The inner loop creates the individual cells for the row (there will be 20 cells/columns in each row). First, it calls the function described above to get a new random color for each cell. That color is used in creating the content for the cell that is also stored in the output variable. After each completion of the inner loop a closing row tag is added to the output at the end of the outer loop. Finally, after all the PHP processing is complete, the variable $tdata holds the entirety of the output that was produced. After the PHP code comes the actual HTML output that will be sent to the user. The majority of this is simply a hard coded HTML page. But, the variable with the dynamic content created above is then inserted into the HTML table element. The result of all of this is that on every call to this page an HTML table will be displayed. It will have 10 rows and 20 columns. Each cell in that table will display a random color.
-
Help to get Json data to a comma delimited file
Psycho replied to techboy992's topic in PHP Coding Help
Updated solution from Barand // create array from json data $arr = json_decode(file_get_contents('result.txt'),1); // remove empty records $arr = array_filter($arr); // open output file $fp = fopen('result.csv','w'); foreach ($arr as $record) { // trim the values $rec = array_map('trim', $record); // skip records that only contain " " if($rec[0]==' ') { continue; } // remove non-numeric characters from amount $rec[2] = preg_replace("#[^\d]#", "", $rec[2]); // replace character code with apostrophe $rec[3] = str_replace(''', "'", $rec[3]); // save in output file in csv format fputcsv($fp, $rec); } // close output file fclose($fp); -
Is a single JSON MySQL retrieval faster than individual rows?
Psycho replied to DeX's topic in MySQL Help
Also, all the fields used to JOIN the tables should be indexed. Pretty much anything with "id" int he field name in your case. I was trying to rewrite the query, but without knowing the schema on all the tables it was getting confusing. I *think* your DB design is flawed. The "match_quote_product" appears to be the table to list out the products included in a quote. So, why is there a field for purchase_order_id? Are there different purchase orders for different items int he same quote? There should be a general "purchase_order" table that contains the header data (e.g. date, customer, PO, etc.) Then the details table should include all the items in the quote that reference back to the main record. There also seem to be some circular references that could be a performance issue as well. But, here is my attempt at rewriting the query. But, it may not work. I wouodl have to really understand all the relationships to be sure which would take more time than I am willing to invest. And even then, I would probably have to make changes to the schema as well. SELECT mqp.product_id mqp.price_per_k, mqp.board_feet, mqp.location, mqp.power, mqp.locking_handle_side, mqp.unit_price, mqp.total_price_calculation_id, mqp.quantity, width, length, height, colours.name, total_price FROM match_quote_product mqp JOIN building_products bp ON mqp.product_id = bp.id JOIN match_quote_colour mqc ON mqp.colour_type_id = mqc.colour_type_id AND mqp.quote_id = mqc.quote_id JOIN colours c ON mqc.colour_id = c.id WHERE match_quote_product.quote_id = 50000 AND building_products.purchase_order_id = 5 ORDER BY purchase_order_order ASC -
Is a single JSON MySQL retrieval faster than individual rows?
Psycho replied to DeX's topic in MySQL Help
To add some context to why requinix is asking for this information: There are things that can/should be done to configure a database schema and/or queries to be efficient. But HOW you accomplish that directly depends on how the data is to be used. With respect to the database schema, you will typically want to index any fields that are used for JOINing tables, used in frequent filter conditions, or used in similar contexts. Also, in the actual queries it is possible to write two different queries to return the same results where one is exponentially faster than the other. It is impossible to explain all the ins and outs of how to work with a database efficiently in a forum post. There are whole books written on the subject. So, we need to see the actual details of your DB schema and queries to provide specific advice. -
That can't work. If it looks like it is working - it isn't. This is similar to the earlier problem about the href parameter. You need to understand that computer programs require explicit instructions. Humans are very good at deciphering things that may not be in context, but computers are not. When you define a string such as this: $content = "test"; The computer sees that you want to define a variable. Then it sees the first double quote which tells it that the following content should be assigned to the variable. When it sees the next double quote it takes that as the end of the content to be assigned. You can also use single quotes to define a string (but there are some different rules on how the content is handled). In your code above you start with a double quote. But then, you have a double quote IN THE CONTENT at the end of the src attribute. So, that is the end of the content that would be assigned to the variable. If you start a string with a double quote, use single quotes inside the string or escape any double quotes in the string. Either of these would work $content .= "<td> <a href='$varcomplete'><img src='wp-content/custom/imageicon.png' alt='imageicon' border='0'></a> </td>"; $content .= "<td> <a href=\"$varcomplete\"><img src=\"wp-content/custom/imageicon.png\" alt=\"imageicon\" border=\"0\"></a> </td>";
-
Parameter values (like the href parameter for an anchor tag) should be within quotes so the browser knows where the value starts and ends. If the value is not within quotes it assumes that the first space is the end of the value $content .= "<td> <a href='$varcomplete'>test</a> </td>";
-
As mac_gyver states you have no error handling. You may have other problems, but the only thing I can "see" wrong in your code is the field name "INT". That is a reserved word in MySQL, so you can use it normally as a field name like that. You can either change the field name or you need to enclose the filed name in backticks to tell the MySQL engine that you are referring to the field name and not using the INT command. $query = "INSERT INTO vehicles (VehID, Color1, Color2, PosX, PosY, PosZ, VW, `INT`) VALUES ($vehicleid, $color1, $color2, $posX, $posY, $posZ, $vw, $int)";
-
I would also add that it would be a better idea to handle the timestamps using MySQL than in the PHP code. Also, I think it makes more sense to make the timestamd represent the last activity of the user instead of some arbitrary time in the future. It give you a lot more flexibility - e.g. select the users active within the last 5 minutes or 10 minutes or whatever. Don't use "SELECT *" when you don't really need all the columns. There are performance and security reasons why it is not a best practice. Plus, never run queries within loops - it is a huge resource hog. Learn to do proper JOINs as Jacques1 suggest. Your current loop is running a query for every record in the prior result set, but it pulling the data for the current logged in user - not the user in each record of the result set.
-
@ginerjm, the OP will not have an image for every possible number value. E.g. there will be no "525.jpg" for the number 525. So, the correct process is to iterate over each character in the numeric string. @Jacques1, you are correct. That approach removes the necessity for splitting the string into an actual array. It's never seemed logical to me to reference characters in a string as if it was an array, so that did not come to mind when providing a solution.
-
Um, no. You are incorrect. The "working example" on that page is PHP code that defines an hard-coded array for testing purposes instead of getting the values from a DB. The author even includes the DB logic commented out showing that is how it would really be done. Plus, the way an array is defined looks different than when you output an array using print_r(). The print_r() function is really more of a debugging tool to output an array showing the structure and the values. Expecting that to look exactly like the definition of the array is foolish. The only difference between the hard-coded array in that example and the array built from the code I provided is that the array in that example code has numerically based indexes (i.e. 0, 1, 2) rather than named indexes as will be present from a DB query. I don't know if the values of the indexes will matter or not. But, if they do need to be numerical indexes, then convert the array to remove the named indexes and replace with numerical ones. I would show you how to do that, but apparently you know what you are doing, and don't need any assistance from us. EDIT: And just to be 100% clear. The "JSON Array" code that you are pointing to is not JSON. It is simply the definition of a PHP array. It would still need to be converted to JSON (if that's really what you need).
-
1. Create/download 10 different image files for each digit. 2. Create a function to take a numeric string value and return the HTML with an image for each digit The function could go something like this: function convertToImage($numberStr) { //Check that input only has numeric characters if(!ctype_digit()) { return false; } //Split string into an array of each character $numbersAry = str_split($numberStr); //Loop through each character and create HTML for image $output = ""; foreach($numbersAry as $number) { $output .= "<img src='1.jpg' alt='1'>"; } //Return results return $output; }
-
You can't "echo" an array (it will just output the word Array as you have seen. You either need to iterate through the array elements and echo the values or you can use print_r() to output the whole array. Also, do the string concatenation in the query instead of the php logic. Try this <?php $mysqli = mysqli_connect("localhost", "root", "", "plods"); $sql_locations = "SELECT CONCAT(address1, ', ', address2) as address, Longitude, Latitude FROM southdowns"; $rs_locations = mysqli_query($mysqli, $sql_locations); while( $rs_location = $rs_locations->fetch_assoc() ) { $markers[] = $rs_location; } echo "<pre>".print_r($markers, 1)."</pre>"; ?>
-
I really don't understand what you are trying to do, nor what your problem is. What IS the code doing now and what do you WANT it to do differently? EDIT: What is the "numberoflines" variable supposed to do? Is it supposed to make the "tree" wider, taller, or what?
-
Of course you can. Create an object with a different property for each 'config' name. (EDIT: Note that it does not have to be the same object used to get the data. Instead create a 'standard' dynamic class in which to populate the data and return to the caller) Not tested, but the logic should be sound class Config { private $dbObj; public function __construct($db) { //Set db object $this->dbObj = $db; //Call get method and return result return $this->get(); } public function get() { //Run query to get data $query = 'SELECT config, value FROM config'; $select = $this->dbObj->query($query); //Create object and populate with query results $configObj = new stdClass(); while ($row = $select->fetch(PDO::FETCH_OBJ)) { $configObj->$row->config = $row->value; } //Return object return $configObj; } } $config = new Config($db); echo $config->test; //Where 'test' is the name of any 'config' field from the DB results
-
Yes, I changed some names half way through. As I said - not tested. Add some debugging to validate what IS returned from the query. Updated code: <?php //Create an run the query to get unread message count $queryStr = "SELECT COUNT(*) as unreadCount FROM `inbox` WHERE `to` = :username AND `read` = 0"; $inboxQry = $db->prepare($queryStr); $inboxQry->bindParam(":username", $Username); $inboxQry->execute(); //Get the results & create the output $inbox = $inboxQry->fetch(PDO::FETCH_ASSOC); $inboxCountHtml = ($inbox['unreadCount']) ? "{$inbox['unreadCount']} New" : "0"; //Debug lines echo "Query result: "; var_dump($inboxQry); echo "Fetched Row: "; var_dump($inbox); ?> <div class="FooterText"><a href="Inbox.php">Inbox</a> (<?php echo $inboxCountHtml; ?>)
-
First, a few pointers: 1. Don't use "SELECT *". In this case you only want to know if the user has any unread messages. So, you shouldn't be selecting records and instead just query the count. But, even when you do need data, using * is a bad practice for several reasons which I won't go into right now. 2. As stated above, you just need to do a "SELECT COUNT(*)". That will be much more efficient. 3. Add comments! Even when working on small pieces of code it will save you time in the long run. In regards to your problem. the rowCount() method is supposed to be used on the database result (e.g. "$UsersInbox"). The code above is trying to use it on the the first row from the result set. Additionally, that will be a Boolean FALSE if there were no rows returned. I'm also curious why you are using the hard-coded unread variable since the intent of the query is to get the unread messages. I can't think of a reason why you would want to get only the read messages. Try this (untested) <?php //Create an run the query to get unread message count $queryStr = "SELECT COUNT(*) as unreadCount FROM `inbox` WHERE `to` = :username AND `read` = 0"; $inboxQry = $db->prepare($queryStr); $inboxQry->bindParam(":username", $Username); $inboxQry->execute(); //Get the results $inbox = $inboxQry->fetchObject(); $inbox->unreadCount; //Prepare the output based on results $inboxCountHtml = ($inbox->unreadCount) ? "{$userInbox->unreadCount} New" : "0"; ?> <div class="FooterText"><a href="Inbox.php">Inbox</a> (<?php echo $inboxCountHtml; ?>)
-
Well, I wrote the code based on his initial post which stated But, looking at his last post he magically changed the requirement from dashes to underscores.
-
//Create function to be called using array_map() function updateDataArray($value) { //Check if any letters exist if(preg_match("#[a-z]#i", $value)) { //Contains a letter replace "-" with space return str_replace("-", " ", $value); } //Does not contain letter, return unchanged value return $value; } $original_data = array( 'abcd-efgh', 'xyz-abc', 'alpha-lima-lima', 'bravo-charlie-charlie', '100-500', '1000-5200', '100000-800000' ); //Call the function above as a parameter in the array_map function $modified_data = array_map('updateDataArray', $original_data); echo "<b>Before</b><pre>" . print_r($original_data, true) . "</pre>"; echo "<b>After</b><pre>" . print_r($modified_data, true) . "</pre>"; Before Array ( [0] => abcd-efgh [1] => xyz-abc [2] => alpha-lima-lima [3] => bravo-charlie-charlie [4] => B-B-D [5] => 100-500 [6] => 1000-5200 [7] => 100000-800000 ) After Array ( [0] => abcd efgh [1] => xyz abc [2] => alpha lima lima [3] => bravo charlie charlie [4] => B B D [5] => 100-500 [6] => 1000-5200 [7] => 100000-800000 )