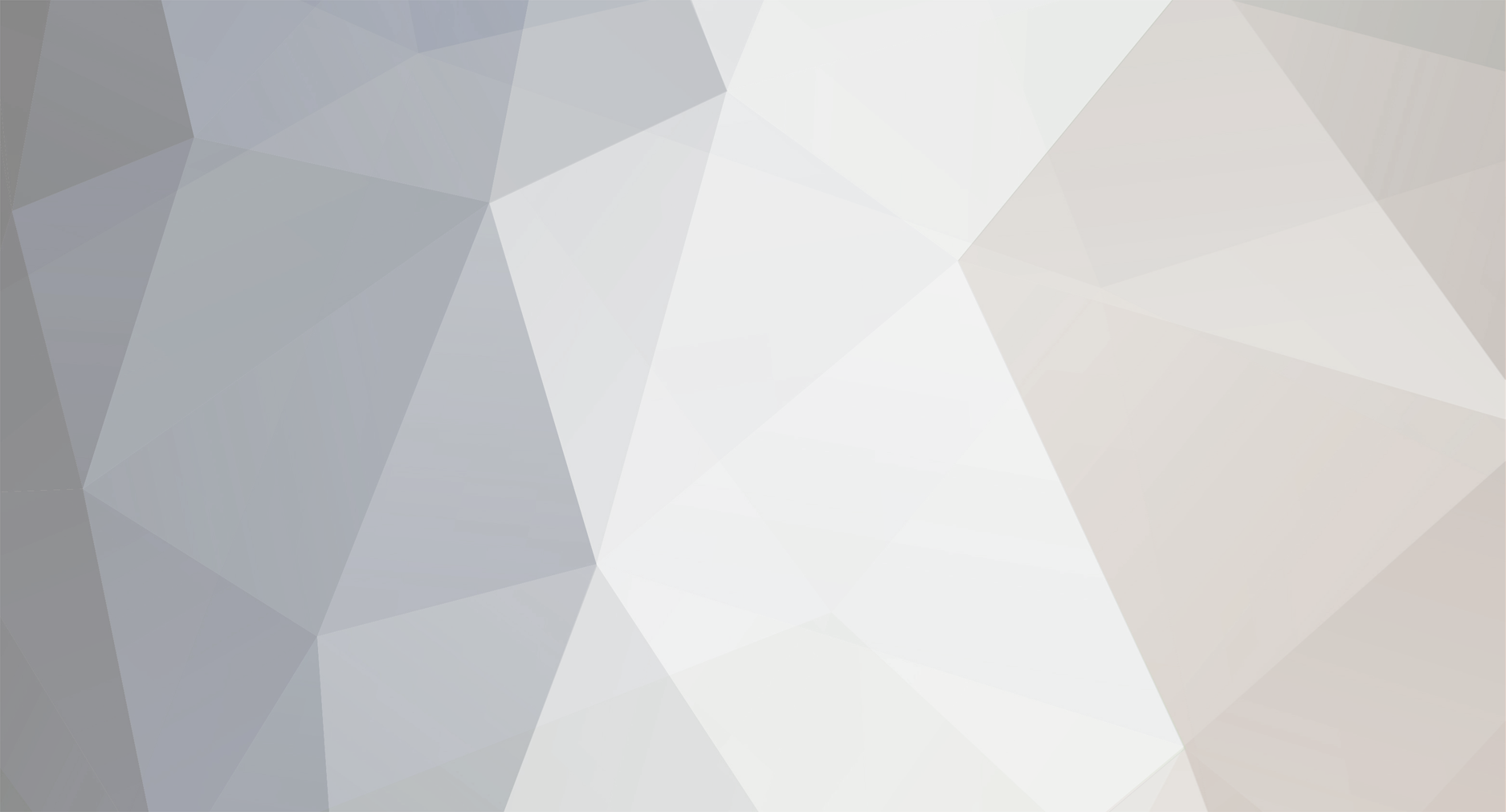
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Computer Guess Num Game | Computer Guesses Your Number
Psycho replied to xxplosions's topic in PHP Coding Help
This will do the computer guesses all in one shot - i.e. one page load - and display all the guesses and results. You can store the guesses in SESSION variables as suggested above and make the user click a button to see each guess one at a time. <?php $min = 1; $max = 100; $output = ''; function guessNumber($min, $max) { return round(($min+$max) / 2); } if($_SERVER['REQUEST_METHOD']=='POST') { $number = intval($_POST['number']); if($number<$min || $number>$max) { $output = "<span style='color:red;'>You must enter a number from {$min} to {$max}.</span>"; } else { $output = "Your number was {$number}.<br><br>\n"; $min_guess = $min; $max_guess = $max; $guessNo = 1; $guess = guessNumber($min_guess, $max_guess); while($guess != $number) { if($guess < $number) { $guessResult = 'low'; $min_guess = $guess + 1; //Set guess as new minimum } else { $guessResult = 'high'; $max_guess = $guess - 1; //Set guess as new maximum } $output .= "Guess number {$guessNo} was {$guess}. That was too {$guessResult}.<br>\n"; $guess = guessNumber($min_guess, $max_guess); $guessNo++; } $output .= "Guess number {$guessNo} was {$guess}. Correct!<br>\n"; } } ?> <html> <head></head> <body> <form action="" method="post"> Enter a number between <?php echo $min; ?> and <?php echo $max; ?>: <input type="text" name="number" /> <button type="submit">Submit</button> </form> <br><br> <?php echo $output; ?> </body> </html> As you requested this will be the most efficient method for finding the number by using the 50% rule. But, that's no fun for the user since any given number will always return the exact same guesses each time. I think it would be more entertaining for the user to add some variability. Plus, it would not be hard for the user to see what the computer is doing and to easily find numbers that would take longer for the computer to guess. There are two things you could do: 1. Make at least the first guess a random umber between the minimum and the maximum. So change the line before the while loop to this $guess = rand($min, $max); 2. Or, you could make each guess a random between the "current" minimum and the "current" maximum. If a guess is to low, it becomes the new current minimum. So, if 15 was 'guessed' and found to be too low, the logic would never try to then guess a number that was 15 or less. It will continue to get closer to the number on each guess, but the guess would still be random. You could easily do this with the previous code, just change the function to this function guessNumber($min, $max) { return rand($min, $max); } -
Bovine scatology detection meter shot off the scale again @White_Lily: Stop posting this nonsense, which you've also posted previously and were advised it was not accurate.
-
Exactly. @NewCastleFan, Why not have multiple fields in one table for the three different prices? You are making this way more difficult than it should be. You can still update all three prices at once or individually. And if price3 was simply another field in the main table you can still do the same thing. Let's say the table structure is something like this: Products: prid_id, prod_name, prod_description, price1, price2, price3 You can have a process to set price1 AND automatically set price2 and price3 using the percentage logic using UPDATE products SET price1 = $price, price2 = $price*.9, price3 = $price*.8 WHERE prod_id = $prodID Then you can also update individual prices just as easily UPDATE products SET price3 = $special WHERE prod_id = $prodID
-
Some notes first: 1. The FONT tags have been deprecated for many, many years! 2. Simplify your logic by determining the values to display at the beginning of the loop and then do the output after. To add the row numbers, just add a variable and increment and output it on each iteration. $rowNo = 0; while($res = mysql_fetch_array($result)) { $rowNo++; //Increment Row Number $date = date("D, j M, Y", $res['time']); $center = strtoupper($res['center']); if($res['approval'] == "Yes") { $approvalText = "Approved": $approvalColor = '#CDE861'; } else if($res['approval'] == "No") { $approvalText = "Rejected": $approvalColor = '#F28598'; } else { $approvalText = "Pending": $approvalColor = '#EDA553'; } echo "<tr align='center' bgcolor='{$approvalColor}'>\n"; echo " <td style='color:black;'>{$rowNo}</td>"; echo " <td style='color:black;'>{$res['randid']}</td>"; echo " <td style='color:black;'>{$center}</td>"; echo " <td style='color:black;'>{$res['customer_name']}</td>"; echo " <td style='color:black;'>{$res['home_phone']}</td>"; echo " <td style='color:black;'>{$date}</td>"; echo " <td>{$approvalText}</td>"; echo " <td><a href ='view.php?id={$res['id']}'><center>Click to View </center></a></td>\n"; //And continue on for other rows echo "</tr>\n"; }
-
The terminology used in this thread is misleading. PHP variables are NOT used in Javascript. PHP and Javascript are independent of one another and do not work together. There are two main ways where there is a connection between PHP and Javascript: 1. Using AJAX, Javascript can make requests to a PHP page. And the PHP page can return a result to the Javascript. 2. PHP can be used to write dynamic Javascript code to the page. But, once the page is complete and sent to the user the PHP code is done - there is no more execution. What this thread seems to be about is item #2. So, talking about "PHP variables working in Javascript" is incorrect. The PHP variables can be used to write out Javascript just as PHP can be used to write out HTML. This problem is a Javascript problem. You simply need to take your working HTML page and then create PHP code to dynamically write out the content to match the same format. Writing code with code can be confusing. So, if it is not working, then view the HTML source and see what is off and make changes till it is as it should be.
-
Then you should post in the Javascript forum. Moving.
-
<?php $letters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; // Alpha characters $numbers = "123456789"; //Numeric characters $characters = str_repeat($letters, 4) . str_repeat($numbers, 9); $multiplier = 5; $length = 15; $separator = "<br>\n"; $codes = array(); for($i=0; $i<$multiplier; $i++) { $rand_chars = str_shuffle($characters); $codes[] = substr($rand_chars, 0, $length); } print implode($separator, $codes); print "<br>\n"; ?>
-
Sorry, if this is a stupid question, but I've read through your posts here and I'm wondering why you need separate tables. In fact, do you need to even store price2 and price3 at all? First, why are you needing to store these in separate tables? Why not store price2 and price3 in the same table in different fields? Then you don't have to duplicate the data across the tables. There might be a valid reason for doing this but, if not, you will save yourself a ton of problems by putting them in the same table. Second, if price2 and prioce3 will always be the same percentage off from price1 then you don't need to store price2 and price3 at all. Just hard-code or have a configuration on what the offset for price2 and price3 should be. Then dynamically change the price based upon your need. Example: //Config vars for the offsets $price1 = 1; $price2 = .9; $price3 = .8; //Then in your code have some logic to determine the offset switch($user_type) { case 'Big Buyer': $price_offset = $price3; break; case 'Frequent Buyer': $price_offset = $price2; break; case 'Normal Buyer': Default: $price_offset = $price1; break; } //Then use that offset in any queries to get price $query = "SELECT name, (price * $price_offset) as price FROM products";
-
Here's a rewrite in what I think is a more logical workflow that isn't as complicated: <?php //Set error message for form $error_msg = ''; //Set variable for populating form field values //Used to make fields sticky in case of errors $nameHTML = ''; $usernameHTML = ''; $emailHTML = ''; if($_POST["submitReg"]) { //Preprocess the inputs $regName = trim($_POST["name"]); $regUsername = trim($_POST["username"]); $regPassword = trim($_POST["password"]); $regREpassword = trim($_POST["rePassword"]); $regEmail = trim($_POST["email"]); //Run field validations $errors = array(); //Array to hold errors //Validate name if($regName == '') { $errors[] = "Name is required."; } else { $regUsernameSQL = mysql_real_escape_string($regUsername); $check = select("COUNT(*)", "members", "username = '$regUsernameSQL'"); if(mysql_result($check, 0) !== 0) { $errors[] = "That username has been taken, pick another."; } } //Validate email if($regEmail == '') { $errors[] = "Email is required."; } elseif(!preg_match("^[a-zA-Z0-9_.-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$", $regEmail)) { $errors[] = "Email is not valid."; } //Validate passwords if($regPassword == '' || $regREpassword == '') { $errors[] = "Password and confirmation are required."; } elseif($regPassword != $regREpassword) { $errors[] = "The password fields didn't match."; } //If validations passed, attempt to create records if(!count($errors)) { //Validations passed $regNameSQL = mysql_real_escape_string($regName); $emailSQL = mysql_real_escape_string($email); $regPasswordSQL = sha1($regPassword); $id = uniqid(); $register = insert("members", "name, email, username, password, user_level, id, ban", "'$regNameSQL', '$emailSQL', '$regUsernameSQL', '$regPasswordSQL', 1, '$id', 0"); if(!$register) { $errors[] = "Sorry, we could not register your account details, if this persists contact the webmaster."; } } //If member was created, attempt to send email if(!count($errors)) { $to = $email; $subject = "Fusion Forums - Account Confirmation"; $message = "Hello! You have recently registered to Fusion Forum's.<br><br>"; $message.= "This is a confirmation email, below you will find your account details along with a Unique ID.<br><br>"; $message.= "In order to activate your account you must first enter the ID into the text field that follows the link at the end of this email. details are as follows:<br><br>"; $message.= "<table>\n"; $message.= "<tr><td><strong>Name:</strong></td><td>{$regName}</td></tr>\n"; $message.= "<tr><td><strong>Email:</strong></td><td>{$email}</td></tr>\n"; $message.= "<tr><td><strong>Username:</strong></td><td>{$regUsername}</td><tr>\n"; $message.= "<tr><td><strong>Unique ID:</strong></td><td>{$id}</td><tr>\n"; $message.= "</table><br><br>"; $message.= "Please follow this link in order to activate your account (opens in your default browser):<br>"; $message.= "<a href='http://www.janedealsart.co.uk/activate.php?id=".$id."'>Activate Account</a>"; $from = "noreply@janedealsart.co.uk"; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers.= "From: {$from}"; if(!mail($to, $subject, $message, $headers)) { $errors[] = "There was a problem sending your confirmation email."; } } //If user registration passed, add forum message if(!count($errors)) { $newsTitle = "New member registered!"; $cont = "{$regUsername} has just joined Fusion Forums!<br>"; $cont.= "Check out his/her profile:<br><br>"; $cont.= "View Profile"; $newsCont = $cont; $newMem = insert("news", "news_title, news_content, username", "'$newsTitle', '$newsCont', '$regUsername'"); //If this fails the user registration still completed. No need to provide error to user. } //If there were errors, create error message for form if(count($errors)) { $error_msg = "<div class='error'>"; $error_msg .= "The following error(s) occured:\n"; $error_msg .= "<ul>\n"; foreach($errors as $error) { $error_msg .= "<li>$error</li>\n"; } $error_msg .= "</ul>\n"; $error_msg .= "</div>"; //Set the form field values to be repopulated $nameHTML = htmlentities($name); $usernameHTML = htmlentities($username); $emailHTML = htmlentities($email); } else { //Include page with register success message include('register_success.php'); //$done = "You have successfully registered to Fusion Fourm's.<br>"; //$done .= "We have sent you an email with a confirmation code on it,"; //$done .= " when you go to confirm your account you will need this code in order to be able to access the forum's."; exit(); } } ?> <html> <head><title>Registration Form</title></head> <body> <?php echo $error_msg; ?> <form action="" method="POST"> <label>Full Name:</label> <input type="text" class="field" name="name" value="<?php echo $nameHTML; ?>" /> <div class="clear"></div> <label>Email:</label> <input type="text" class="field" name="email" value="<?php echo $emailHTML; ?>" /> <div class="clear"></div> <label>Username:</label> <input type="text" class="field" name="username" value="<?php echo $usernameHTML; ?>" /> <div class="clear"></div> <label>Password:</label> <input type="password" class="field" name="password" /> <div class="clear"></div> <label>Again:</label> <input type="password" class="field" name="rePassword" /> <div class="clear"></div> <input type="submit" class="button" name="submitReg" value="Register" /> <div class="clear"></div> </form> </BODY> </HTML>
-
@White_Lilly: The new forum software doesn't do a good job of keeping the formatting for code when posting. So, I don't know if what you posted is representative if the formatting you actually had. But, as snowdog posted you would do yourself a great favor by adding formatting (especially indenting) to provide a visual representation of the logical flow of your code. However, I would also suggest that if you have a very deep nest of logic that you should probably take a second look to see if you can reduce how deep that nesting goes. I do see an error in the code snowdog posted where another ending brace was removed that shouldn't have. That might not have happened with simpler structure. But there are other problems as well: You are running all of the input through htmlspecialchars() and mysql_real_escape_string() BEFORE you validate the data. You should validate the data first THEN transform the data as needed. Using the method you have, valid data could fail the validation. Plus, if I am reading the coed right yu are running the POST values through that same logic twice - once in the foreach loop and again right after that. Also, I would advise not using htmlspecialchars() on the data you save to the database. You should make that change as you echo the content to the page. If you were to ever use the data for something other than directly outputting onto an HTML page the data would be messed up. if($regPassword != $regREpassword || $regREpassword != $regPassword) Huh? Regarding the nested logic, here is an example of something that can be simplified. Instead of this if(preg_match("^[a-zA-Z0-9_.-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$", $_POST["email"]) === 0) { $msg3 .= "Please enter a valid email."; } else { if($regPassword != $regREpassword || $regREpassword != $regPassword) { $msg3 .= "The password fields didn't match."; } else { You could have if(preg_match("^[a-zA-Z0-9_.-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$", $_POST["email"]) === 0) { $msg3 .= "Please enter a valid email."; } elseif($regPassword != $regREpassword || $regREpassword != $regPassword) { $msg3 .= "The password fields didn't match."; } else { But, I don't like logic where the script stops on the first error. It should check for ALL errors then provide the user a list of what failed.
-
That's not really a good solution as it is not precise. If the records with the same criteria were created at the same time it's possible that they would have the same timestamp and the above logic would delete multiple records. Besides, a sub-query isn't necessary.
-
So, what exactly is your question? If you are asking about "how" you would do it, the back-end logic (i.e. PHP) would be nearly identical to a pagination script except instead of SELECTing X number of records using a LIMIT based upon the selected page you would instead SELECT all the records for the selected day. That's actually easier than a basic pagination script. However, if your question is about how to "display" it then that really isn't a programming question. It's more appropriate for the HTML and/or CSS forums.
-
So, you are saying that there are multiple records in that table with the same cart_id and code and you want to delete only one of those records? Well, based upon your initial query it is as simple as putting a LIMIT on the query DELETE FROM holiday_cart WHERE cart_id='$cid' AND code='$code' LIMIT 1 However, now you are saying you only want to delete the most recent matching record based upon a timestamp field. So, in addition to the ORDER BY you would also want to specify the ORDER DELETE FROM holiday_cart WHERE cart_id='$cid' AND code='$code' ORDER BY date_field DESC LIMIT 1
-
Paginate A Table In Multiple Databases (Php/mysql)
Psycho replied to FishSword's topic in PHP Coding Help
There is really no good way to do this that I know of. I don't believe you can do a JOIN or UNION across databases. So, you would have to query ALL the data from each table from each database, dump the results into a temporary table and then query that. Or alternatively, query all the tables and then do all the logic in the PHP code. Either way would take quite a bit of time and resources to complete. If the data doesn't need to be real-time you could have an automated process to grab the data from each table in the separate databases to updated the temp table. Then each page load could just use that data. But, I guess the bigger question is are the different databases really needed? -
Am I Going About This The Right Way Or Is There A Better Way
Psycho replied to far2slow's topic in PHP Coding Help
I would check how long it takes to get the information from Xbox Live. Based on what I know you can only check the online status of your friends. If these people are all your friends on Xbox Line then it would only take one scrape to get all of their online statuses. If you are going to do multiple scrapes to get the data then my answer below might change. Based upon my understanding this is the process I would follow. When page loads where you want to display online status: 1. Script should first check if a cache file exists (e.g. xbox_online.txt). 2a. If the file does not exist then create it. 2b. If the file exists, check the time that the file was last updated. 3. If 2a was true OR if the last updated time was > the specified value (e.g. 60 minutes) then run a screen scrape and put the results into the cache file 4. Use the data in the cache file to display the online status of the users Using the above process you will only get a new online status if a user visits the page and the cache data is > 60 minutes (or whatever value you set). I would not think that scraping the page would take so long that it would be an issue. I suggest the cache file with the assumption that the data is only going to be used for displaying the online status. In fact, I would create the cache file already formatted as HTML code. So, you would only need to include() it int he output of the page. However, if you have other purposes for this data, then you should put it in the database. -
Searching, And Removing Next Page Link On Last Results Page.
Psycho replied to strago's topic in PHP Coding Help
There are a ton of tutorials out there on pagination and yours is missing many of the very basics. The code below solves almost all of the issues you asked about except how to simplify the search. Searching multiple fields using the same search term is a basic part of what is known as "full text" searching. To do that requires setting up the database table in a specific way and changing the queries. Do a Google search for "mysql full text search" and see what you will need to do. There is too much to explain to try and explain in a forum post. <?php $records_per_page = 10; mysql_connect("localhost", "root", "PASSWORD") or die(mysql_error()); mysql_select_db("DATABASENAME") or die(mysql_error()); //Get the search string $search = isset($_GET['q']) ? mysql_real_escape_string(trim($_GET['q'])) : ''; if(!empty($search)) { //Create the BASE query $FROM = "FROM memes WHERE `title` LIKE '%{$query}%' OR `source` LIKE '%{$query}%' OR `category` LIKE '%{$query}%' OR `body` LIKE '%{$query}%' OR `facebook` LIKE '%{$query}%'"; //Create and execute query to get total count of records $query = "SELECT COUNT(*) {$FROM}"; $result = mysql_query($query) or die(mysql_error()); $total_records = mysql_result($result, 0); //Determine total pages for search term $total_pages = ceil($total_records / $records_per_page); //Get selected page (or set to page 1) $page = isset($_GET['page']) ? intval($_GET['page']) : 1; //Ensure page is between 1 and total pages $page = max(min($total_pages, $page), 1); //Calc limit start value $limit = ($page - 1) * $records_per_page; //Create and execute query to get current page's records $query = "SELECT id, title, source {$FROM} ORDER by `title` LIMIT {$limit}, {$records_per_page}"; $result = mysql_query($query) or die(mysql_error()); //Determine if there were results if(!mysql_num_rows($result)) { echo "No results"; } else { //Create results output while ($row = mysql_fetch_assoc($result)) { $results[]="<a href='file.php?id={$row['id']}'>{$row['title']}</a> - {$row['source']}"; } echo implode("<br>\n", $results); //Create navigation links echo "<p>"; if ($page > 1) { $prev = $page - 1; echo "<p><a href='file.php?q={$search}&page={$prev}'>Back</a>"; } if($page > 1 && $page < $total_pages) { echo " - "; } if($page < $total_pages) { $next = $page + 1; echo " <a href='file.php?q={$search}&page={$next}'>Next</a>"; } } } ?> -
Can't really answer since you have not posted any code. But, you should definitely do a couple things: 1. Update the code to properly validate/escape the content before running it in a query 2. You could set up a very simple password check. Just hard code it into the page. Set a session value once the password check is done. Then only display/execute the form if that valule is set.
-
I believe he wants to be able to send a message to a friend on facebook using his own page/script. @Mancent, you should first check to see if doing this is within the terms of service for facebook. If it is, check to see if they have an SDK for it. If they don't then go download a program called fiddler. It allows you to capture all traffic back and forth from your machine and internet sites. You can then send a test message on FB and see exactly what parameters are being passed.
-
You should log into the database using PhpMyAdmin (or whatever management application you have) to verify what the actual database name is.
-
Why are you assuming the database is named playerSearch because of a file? In my local MySql installation each database has it's own folder and each table has several files.
-
The rules for subtraction are (Reference: http://www.factmonst...a/A0769547.html) Several rules apply for subtracting amounts from Roman numerals: a. Only subtract powers of ten (I, X, or C, but not V or L) For 95, do NOT write VC (100 – 5). DO write XCV (XC + V or 90 + 5) b. Only subtract one number from another. For 13, do NOT write IIXV (15 – 1 - 1). DO write XIII (X + I + I + I or 10 + 3) c. Do not subtract a number from one that is more than 10 times greater (that is, you can subtract 1 from 10 [iX] but not 1 from 20—there is no such number as IXX.) For 99, do NOT write IC (C – I or 100 - 1). DO write XCIX (XC + IX or 90 + 9)
-
Ah, I missed that you had the enctype parameter in the TD tags, but I saw you did have it in the form tag. It probably doesn't do anything to have it in the TD tags since there is no enctype parameter for those. The problem is you are not referencing the uploads correctly - they are not in the $_POST global, they are in the . . . wait for it . . . $_FILES global. But, please read that tutorial because they are in sub-arrays - not like normal POST values.
-
You don't reference file upload fields in the same manner as other form inputs. Take a look at this short tutorial: http://www.tizag.com.../fileupload.php
-
Have you echo'd the query to the page to verify what it actually contains? EDIT: Also, what are you trying to accomplish using MATCH() in the select part of the query? My assumption is that MATCH would return either a 0 or 1 based upon whether there was a "match". But, since you are using the same MATCH clause in the WHERE condition all of the records returned would be a match. So, there is no need for that in the WHERE clause - if I am understanding it right. Nevermind, MATCH can be used in the SELECT query to get the "value" of the match. I think the error is that count(*) is an implied GROUP BY, but the MATCH() in the select list is not using a SUM(), COUNT() or other type of GROUP BY condition. So, the two values you are trying to get in the SELECT list are not of the same type - singular and plural Since your query also has an ORDER BY I will assume you really want all the matching records and not the COUNT()? $query = "SELECT *, MATCH (title, keywords) AGAINST('{$keyword}') as score FROM $tbl_name1 WHERE MATCH (title, keywords) AGAINST ('{$keyword}') ORDER BY score DESC";
-
+1 To expand on Barands' response let me provide an illustration of why you should validate first. Let's say you have a field where you want to enforce a minimum character length of 6. If the user inputs "o'kay" (5 characters) and you sanitize first (using mysql_real_escape_string() or something similar), the value will pass the validation because the apostrophe will be escaped and the result will be "o\'kay" (6 characters). By the same token, if you have a field where you want to allow an apostrophe but not the backslash, sanitizing first can result in a valid input being rejected.