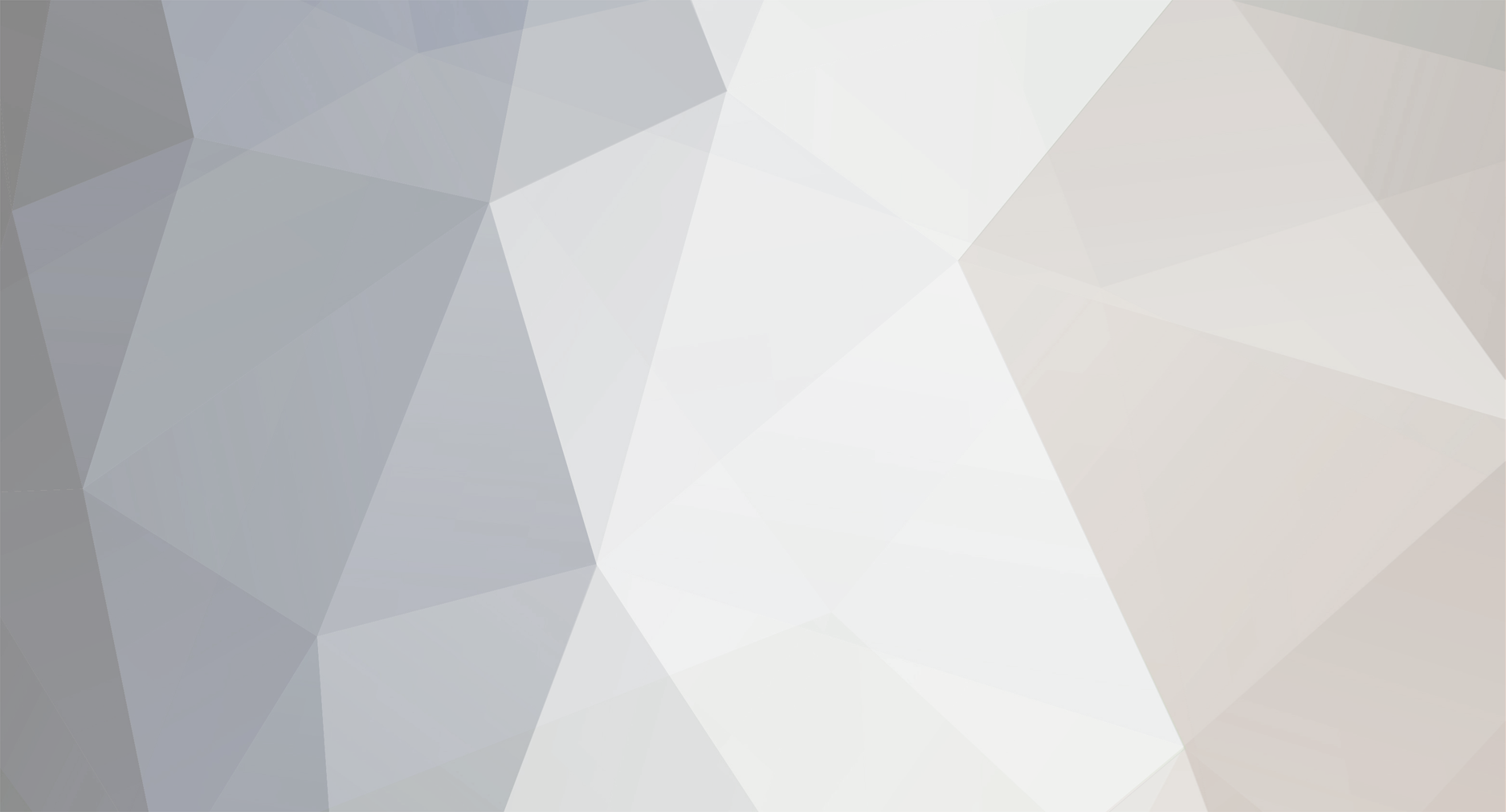
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Beginner In Php Need Help With A Music Playlist App
Psycho replied to zenmind's topic in PHP Coding Help
There is nothing wrong with that ^^^. He didn't end the foreach loop by closing the PHP tag. In fact, he actually never ended the loop. Although I abhore opening and closing PHP tags multiple times in my script there is nothing wrong with doing that. -
Beginner In Php Need Help With A Music Playlist App
Psycho replied to zenmind's topic in PHP Coding Help
You should be getting errors based upon that code - but you chose not to include them? When you are writing code, especially as a beginning, do one thing and verify it works before doing the next thing. In this case you should verify that you are getting the results of the files. So, I wouldn't even use a foreach() loop to produce output, just use a print_r() on the array. Also, don't put too much onto one line. Here is your problem: foreach (scandir(C:\Users\test\Desktop\Main\xampp\htdocs\mp3)("./mp3/*.mp3") as $song) So, don't do a foreach() on the function results. Instead put the function results into a variable and use that. But, look at the directory path you are using for scan directory. You are supposed to pass a string to the function. You should either set the path as a variable OR you need to enclose the string in quote marks: $directory = 'C:\Users\test\Desktop\Main\xampp\htdocs\mp3'; $files = scandir($directory); // print_r($files); //Uncomment to debug foreach($files as $file) { //Do something } -
Personally, I don't think the DB type has any bearing on the question. It all depends on "how" the POST value is to be used. Personally, I use an isset check before referencing ANY POST values. I may have the same backend code that works with different inputs. So, if the value is not included in the POST data I will set to false or an empty string - again based upon how I will use the value in the code later. $long_name = isset($_POST['long_name']) ? $_POST['long_name'] : '';
-
Php Mysqli -- Database Connection Failure
Psycho replied to nodirtyrockstar's topic in PHP Coding Help
Open the connection once, then run all your queries before closing the connection. In fact, you don't really need to close the connection at all - that will be done automatically when the script ends. -
Php Mysqli -- Database Connection Failure
Psycho replied to nodirtyrockstar's topic in PHP Coding Help
Yes, you can make many queries to the DB on a single script execution. Are you sure you aren't closing the connection prematurely or referencing the wrong link to the DB? For the single page execution that would never happen. One query would finish before the next is executed. -
You change the VALUE of the button. The button doesn't have a NAME. But, I wouldn't use the submit button as a check anyway. Just use if ($_SERVER['REQUEST_METHOD']=="POST") I think I got the names right
-
Value Is Posted Definitly But Isset() Says No....
Psycho replied to vishalonne's topic in PHP Coding Help
Based upon your last post you have two functions: formhash1() and formhash2(). The first function is dynamically creating a field with the name "p1" whereas the second function is dynamically creating a field with the name "p". If those two functions are used for forms 1 and 2 respectively then that explains why form 1 does not have a POST value for "p". But, this is a terrible implementation. You should not be doing any hashing on the client side. -
Delete Multiple *selected* Fields/columns/rows
Psycho replied to White_Lily's topic in PHP Coding Help
Why do you say that? POST is no safer/secure than GET if that is what you were insinuating. It is a trivial process to modify POST data outside of the form. -
Delete Multiple *selected* Fields/columns/rows
Psycho replied to White_Lily's topic in PHP Coding Help
@The Little Guy, That really is overcomplicated don't you think? All you are doing it creating a custom function that does the exact same thing as intval().I provided what is arguable a more full-featured solution, but if all you want to do is force the values to an integer then this would be much simpler: $values = implode(",", array_map('intval', $_POST["imAnArray"])); No need for the sanitize function, no need to create the dynamic function, use the bult @White_Lily, Yes, this would be the easiest approach. Just create your checkboxes as an array using the record ID as the value. Then run a single delete query as shown. Just be sure to provide any validation needed. For example if these are associated with a user and users should only be able to delete their own images then make sure the user ID is for the person logged in (in fact, you would want to have the user id as a session variable rather than have it passe din the form data if that is the case) -
Creating Dashboard Messages - Seeking Best Practices?
Psycho replied to Lostnode's topic in Application Design
I'm in agreement with Spiderwell. From the logic you are wanting, it doesn't seem that there is really any specific "read" event other than the message was displayed to the user. And since you want to display new notifications upon login you don't need to track which notifications have been read - you only need to display the notifications created since the user's last login. Also, no need to muddy the waters with AJAX - at least initially. Build the core functionality first. Then, you can modify using AJAX later if needed. But, in this case - as Spideerwell stated - you may not even need AJAX. Just populate the notifications into a DIV and provide a button to hide/remove the notifications. That can all take place on the client-side. Now, the one trick to this is that when a user logs in you need to store their previous last login (such as in a session variable) before updating their record with the current login datetime. Otherwise, if you were to use the last login time from their user record it would always be after all the notifications. Here is a rough outline of some code to get you started <?php //Get any new notifications created after prev login $query = "SELECT date_created, notification FROM notifications WHERE date_created > {$_SESSION['prev_login']}"; $result = mysql_query($query); //Create notifications output $notifications = ''; if(mysql_num_rows($result)) { //Opening DIV container $notifications = "<div id='notifications'>"; while($row = mysql_fetch_assoc($result)) { //Individual notification DIV $notifications .= "<div>{$row['date_created']}: $row['notification']<div>"; } //Link to close/hide notification DIV $notifications .= "<a href='#' onclick=\"document.getElementById('notifications').style.display='none'\">Close</a>"; //Closing DIV container $notifications .= "</div>"; } ?> <html> <body> <?php echo $notifications; ?> </body> </html> -
I'm in agreement with Kicken - using exceptions for something such as an invalid username is clunky at best. There should be a distinction between a "system error" one that prevents the script from completing and a "user error" - script can complete but there are errors to display to the user. But, since the OP specifically mentioned using classes I'd like to offer a suggestion of how I like to handle user errors within a function. I'll typically create an $error property for a class. Then if there are any user type errors within any of the methods I will simply set an appropriate error message in that property and return false from the method. class user_class { public $error; public function isValidUsername($uname) { if (!preg_match("/^[a-zA-Z0-9]+$/" )) { $this->error = "The entered username contains invalid characters."; return false; } $query = mysql_query("SELECT COUNT(*) as num FROM users WHERE username = '$uname'"); $user_count = mysql_result($query, 0); if ($user_count>0) { $this->error = "The entered username is already taken."; return false; } return true; } } if (isset($_POST['username'])) { $userObj = new user_class(); if(!$userObj->isValidUsername(($_POST['username'])) { echo $userObj->error; } else { echo 'The entered username is valid.'; } }
-
Split String And Create Array With Named Values
Psycho replied to matthewtbaker's topic in PHP Coding Help
-- marking solved based upon OPs last response -- -
Delete Multiple *selected* Fields/columns/rows
Psycho replied to White_Lily's topic in PHP Coding Help
@The Little Guy, That won't work. array_walk() applies the function results to the array elements - i.e. it modifies the variable. It returns either true or false. So, the implode above is trying to implode a true/false as returned from array_walk() - not the actual array. Instead, array_map() would be a more appropriate solution since it returns an array with the modified values. I was also going to suggest just using intval() as the callback function, but by using a custom function it makes it easier to modify as needed later on. But, I would also add a process to remove empty/false values from the resulting array function parseIntIDs($idsArray) { //Force values to INTs $idsArray = array_map('intval', $idsArray); //Filter out false/empty values $idsArray = array_filter($idsArray); return $idsArray; } $valuesList = implode(', ', parseIntIDs($_POST["imAnArray"])); $query = "DELETE FROM my_table WHERE record_id in ($valuesList)"; mysql_query($query); -
OK, I've studies the code and I'm having some trouble understanding it all - the varaible variables definitly seem an over complication. I *think* this may work, but I'm sure there is a simpler solution if I really understood all the data and how it is used. Anyway, this will only echo the resulting single INSERT query. So, you can verify that it is generating the query before you actually execute it if(!isset($_POST['submit'])) { echo "Error!"; } else { //Read CSV file into array $file = $_POST['filepath']; $handle = fopen($file, 'r'); $fileop = fgetcsv($handle, 2000, ','); //read only headers (first row) $csvData = array(); while(($csvLine = fgetcsv($handle,10000,",")) !== false) { $csvData[] = $csvLine; } // build and run qry to select data from assessment, assessmenttype tables $query = "SELECT assessmenttype.AssessType_Code, Assess_Num FROM assessment JOIN assessmenttype ON assessmenttype.AssessType_Code = assessment.AssessType_Code WHERE Offer_ID = '$offerId';"; $result = mysql_query($query) or die('Query failed: ' . mysql_error()); $c = 1; //Process query results into INSERT values $values = array(); while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $assessNum = $row['Assess_Num']; $assessCode = $row['AssessType_Code']; $mark = $fileop[$_POST["lstAssess$c"]]; //Loop through csv data to create insert values associated with this record foreach($csvData as $line) { $values[] = "('$line[2]', '$offerId', '$assessNum', '$assessCode', '$mark')"; } $c++; } //Create insert query $query = "INSERT INTO gradebook (Stud_ID, Offer_ID, Assess_Num, AssessType_Code, GB_Mark) VALUES " . implode(",\n", $vaules); //Debug line echo $query; //Execution line //$result = mysql_query($query) or die('Query failed: ' . mysql_error()); } //close database connection mysql_close($conn);
-
Your two loops are as follows: while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) while((${"fileop$i"} = fgetcsv($handle,10000,",")) !== false) Now, let's step through the logic. On the first iteration of the outer loop, the first record from $result is extracted. Then the inner loop will start by getting a line from the csv file. That inner loop continues as long as another line can be extracted from the csv file. Now, once the inner loop completes (i.e. no more lines can be read from the csv file the execution breaks out to the outer loop. So, on the second iteration of the outer loop the next record from $result is extracted and execution continues. But, now when the execution hits the second while loop it will skip it entirely since all the lines were already read from the file! If you are wanting to create records for each line int he CSV file but have them duplicated for each record in the first query results you shoudl definitley take jesi's advice and build up a SINGLE query to create the records.
-
preg_replace takes a regular expression that is properly formatted with delimiters. Plus, I think you need to escape the forward slashes with backslashes. Kinda hard to verify if the expression is even close without seeing an example of the code to be replaced.
-
Adding Next And Previous Function To An Edit Form
Psycho replied to RLAArtistry's topic in PHP Coding Help
That would not work. ALL the records in the table would match one of those three conditions. Using LIMIT 3 would not pull the three records you want - it would pull the first three records by whatever the sort order it - if none is given then it is usually by the order the records were created. Therefore, that query would always pull the first three records regardless of the value of $thisID- 6 replies
-
- php next code
- previous code
-
(and 2 more)
Tagged with:
-
You are the one asking for help. It is your responsibility to provide the information we need to provide that help. If you can't even be bothered to post accurate code then why ask for help? Look at it this way. You ask for help providing nothing for us to provide that help. Then when you finally decide to post some code and we point out problems in that code you come back and state that we should just ignore that error. Why are you wasting our time when we are only trying to help? Let's go back to your original post Provide the content of $returned that you say is not working with that str_replace(). As shown in the example Barand provided with some mock content of $returned it worked perfectly fine.
-
Really? the function seems to work perfectly fine for everyone - and has for years. But, you are not getting the results you expect so youjump to the conclusion that the function is broken. As Christian F. alluded to, in the code you finally provided you aren't doing anything with the returned value of str_replace() str_replace('securimage/securimage_show.php', '[url="http://www.serverlist.us/securimage/securimage_show.php"]http://www.serverlis...rimage_show.php[/url]' $returned); str_replace() does not modify the original value (if that is what you were thinking) - it only returns the modified string. You need to do something with that returned value (otherwise why run it in the first place).
-
PHP is executed on the server. Once the script COMPLETES then the entire thing is sent to the browser. The way to achieve what you are wanting is with Javascript - not PHP.
-
Ok, you make a call to the function getBanner() which is supposed to return either the user's banner or the default. And you're saying that the default is always returned right? So, here is that function: function getBanner($rel) { $query = select("*", "users", "username = '$loggedUser'"); $fetch = mysql_fetch_assoc($query); $bannerImg = $fetch["bannerImage"]; $link = "<a href='".$GLOBALS["siteUrl"]."/images/banners/".$bannerImg."' rel='".$rel."'>"; $Img = "<img src='".$GLOBALS["siteUrl"]."/images/banners/".$bannerImg."' />"; $close = "</a>"; $default = "<img src='".$GLOBALS["defaultImage"]."' />"; $acutalImg = $link.$Img.$close; if($actualImg) { return $actualImg; }else{ return $default; } } I see a few problems with that function. Even if you fixed the problem it would still not work as you want. Let's go through it line by line: $query = select("*", "users", "username = '$loggedUser'"); Looks like you have a custom function for running a SELECT query? Not sure why, but OK. 1) why are you selecting * when you only need the image? 2) $loggedUser has not been defined in the function so that value would always be empty. 3) Not sure why you are using the username instead of the userID which you should have in the table. $fetch = mysql_fetch_assoc($query); You should do a check to see if any record was even returned before you try and fetch the data. $bannerImg = $fetch["bannerImage"]; Yeah, you need to check if any record was returned first. $link = "<a href='".$GLOBALS["siteUrl"]."/images/banners/".$bannerImg."' rel='".$rel."'>"; $Img = "<img src='".$GLOBALS["siteUrl"]."/images/banners/".$bannerImg."' />"; $close = "</a>"; $default = "<img src='".$GLOBALS["defaultImage"]."' />"; $GLOBALS ?!?!? Really? That's very poor form. $acutalImg = $link.$Img.$close; Interesting name for that variable, don't you think? if($actualImg) { return $actualImg; }else{ return $default; } First of all, that condition is checking the wrong variable - you misspelled it when you set it previously. Second, that will ALWAYS return true since you just set the value before you did that check. You need to check if the returned value (if there was one) had a non-null value to determine whether to show the default or not. $actualImg will always have a value as you have coded it. I have rewritten the function below. You need to now pass the values for $loggedUser and $rel. Ideally I would also pass the values you are referencing using $GLOBAL, but I was lazy This should get you closer to what you want //Banner Image(s) function getBanner($loggedUser, $rel) { //Define return value as default $banner = "<img src='{$GLOBALS['defaultImage']}' />"; //Run query $query = select('bannerImage', 'users', "username='$loggedUser'"); //Determine if record was returned if($query != false && mysql_num_rows($query)>0) { $bannerImg = mysql_result($query, 0); //Determine if value was empty if(!empty($bannerImg)) { //Redefine return value $imgURL = "{$GLOBALS['siteUrl']}/images/banners/{$bannerImg}"; $banner = "<a href='{$imgURL}' rel='{$rel}'><img src='{$imgURL}' /></a>"; } } return $banner; }
-
Um, yeah. I have no idea what I was thinking.
-
There is no difference between POST and GET data from a security standpoint. Do not thing that because the user does not see the value in the query string that they cannot modify the data. If you have a select list - do not assume that the value passed for that field was one of the ones that you defined for the list. Do not assume that a value you put into a hidden field is not going to be modified by the user. It is very easy for someone to create a modified form to send whatever data they want. There are some tricks that I've seen used to try and prevent form submissions from external sources, but that only adds complexity with no real benefit. There are free tools available that allow a user to 'trap' http data being send from their computer and modify it before continuing on to the web server. You need to validate and sanitize ALL data coming from the user: POST, GET, COOKIE, etc. Don't trust any of it. There is no way to cover absolutely every aspect of what you need to consider. You just need to stop and think about HOW each piece of data will be used and what you should expect the value to be. Here are some examples of the things I would consider: With a select list the displayed label typically corresponds to an ID which will be the actual value passed and it is very common for that ID to correspond to an auto-increment integer in the DB. For any values that should be a integer ID you should always check for an integer or force the value to be an integer. Again, it depends on how it will be used. SCENARIO #1 If the value is used to SELECT some data from the database (e.g. a search/filter) I may just force the value to be an integer by typecasting it as such or using intval(). If the value isn't even a number it will typically be converted to a 0. Auto-increment IDs always start as 1 by default. So, if the value is converted to a 0 (or some other integer that doesn't exist in the DB) then the worst that would happen is that the user would get no results or possible some invalid results - but since they purposefully tried to cheat the system I find that acceptable. SCENARIO #2 Ok, let's say the value is used to SELECT data as above - but the ID is in a list of approved values that the user can select. In other words, there are values we don't want the user to be able to select. In that case (after forcing the value to an integer) I would want to do one of two things. 1) I could verify the value first by running a query to see if the passed value is in the list of approved IDs for the user. Or 2) I could adjust the query used to search the data to do that verification internally. I'm sure there are better ways, but here is a quick example: Instead of this SELECT * FROM data_table WHERE id = '$submittedID' You could use this SELECT * FROM data_table JOIN approved_ids ON data_table.id = approved_ids.id AND approved_ids.user = '$thisUser' WHERE id = '$submittedID' Again, if the user submitted a non-approved ID then they just wouldn't get a result SCENARIO #3: Lastly, let's say that the value passed is used in the creation of a record, the value is required for the records and you must ensure it matches one of a list of existing IDs. For example, the record is for a car and the field is to select the make of the car. Then, in addition to checking or forcing it to be an integer you would want to do a DB check on the value to verify it is in the list of available IDs for makes of cars before trying to insert the record. All of the above is just "off the top of my head" thoughts and does not represent everything to be considered or all possibilities. It is only meant to provide some advice on the thought process you would go through to determine what the right process is for your particular needs
-
file_get_contents(); But, yeah, what you are asking to do sounds very problematic. At the very least it is going to introduce a slight delay in processing. At worst it could prevent the application from running if there are connectivity problems
-
Am I the only one that sees that there are three quote marks? The one right after car1 ends the string so the part after it is interpreted as . . . I have no idea. I can't believe there aren't errors reported. Should be $howmanycarpics = glob("/garage/uploads/cars/15/thumbs/car1/*.*");