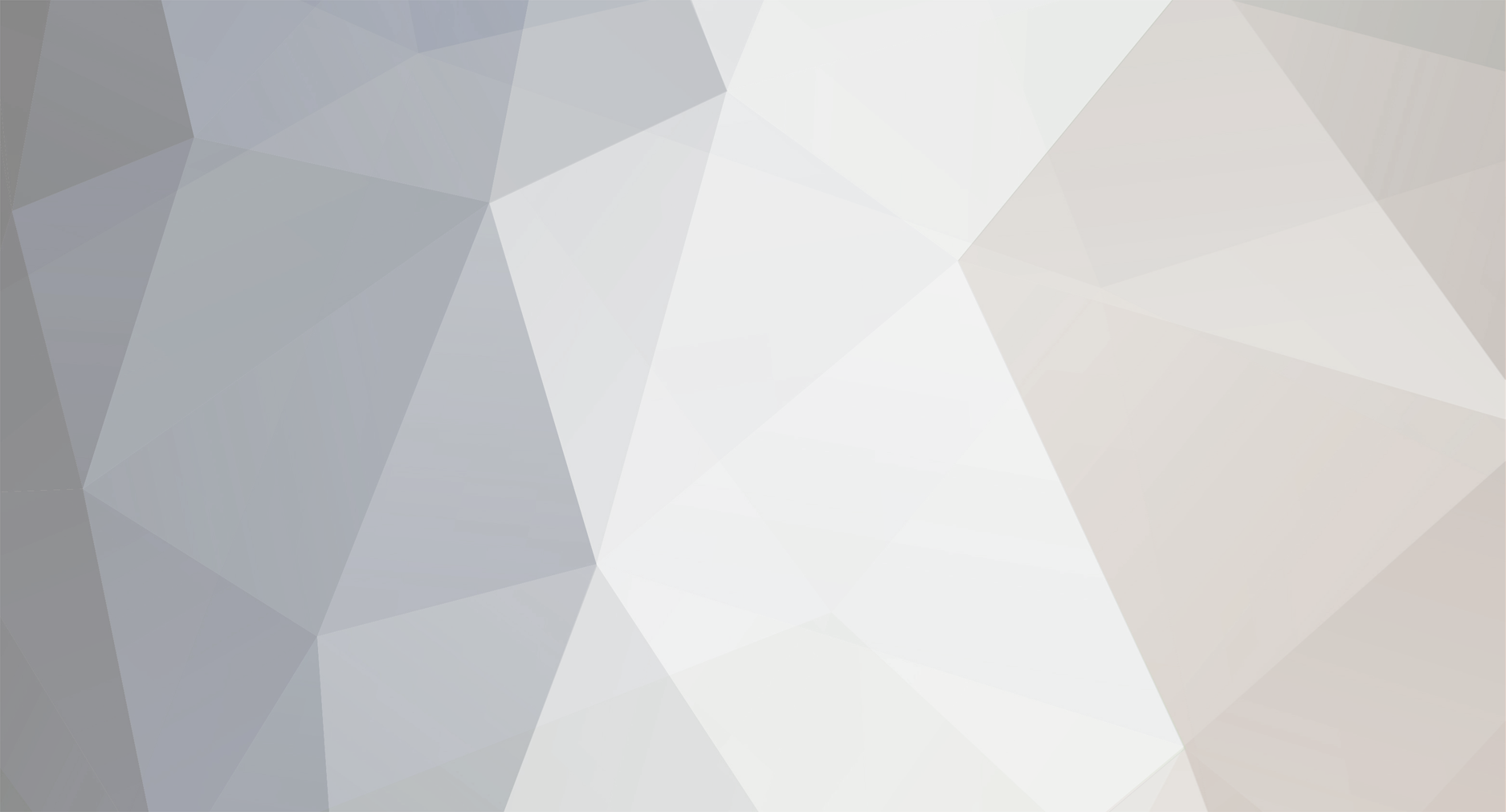
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I am not familiar with Word Press and so I'm not sure what part of that code is core to WP or what the best ways are to accomplish this in that framework. But, it would seem to me that the best solution would be to modify the get_terms() function to only return the categories you want. That function currently takes one parameter - the string "portfoliocategory". I don't know what that function does with that string and how it gets the categories from that. If it is pulling categories based upon a DB attribute then one solution is to change categories 1-7 to have the attribute "portfoliocategory1" and categories 6 & 7 to have the attribute "portfoliocategory2". Then just pass "portfoliocategory1" or "portfoliocategory2" based on which sets of categories you want.
-
Creating New Array Inside A Foreach Loop And Returning Its Value
Psycho replied to deadliver's topic in PHP Coding Help
OK, here you go. using the sample file you have provided this should do most everything you need. All you need to pass to the function is the location of the file or even the url to the page with the content to be parsed. The function will then return an array of all the data. function getUserData($source_location) { $source = file_get_contents($source_location); if(!$source) { return false; } $results = array(); $major_cats = array( "Battles Participated", "Victories", "Defeats", "Battles Survived", 'Hit Ratio', "Destroyed", "Detected", "Hit Ratio", "Damage", "Capture Points", "Defense Points"); foreach($major_cats as $cat) { $pattern = "<td class=\"\"> {$cat}: </td>.*?<td class=\"td-number-nowidth\">([^<]*)"; if(preg_match("#{$pattern}#is", $source, $match)) { $results[$cat] = trim($match[1]); } } $minor_cats = array('Targets Destroyed', 'Targets Detected'); foreach($minor_cats as $cat) { $pattern = "<td><span>{$cat}</span></td>[^<]*"; $pattern .= "<td class=\"right value\">([^<]*)</td>"; if(preg_match("#{$pattern}#is", $source, $match)) { $results[$cat] = trim($match[1]); } } $vehicles = array('Type 59', 'IS-3', 'IS-8', 'IS-7', 'T-150', 'IS'); foreach($vehicles as $veh) { $pattern = "<a class=\"b-gray-link\" href=\"/encyclopedia/vehicles/[^\"]*\">{$veh}</a>[^<]*"; $pattern .= "</td>[^<]*"; $pattern .= "<td class=\"right value\">([^<]*)</td>[^<]*"; $pattern .= "<td class=\"right value\">([^<]*)"; if(preg_match("#{$pattern}#is", $source, $match)) { $results['vehicles'][$veh]['total'] = trim($match[1]); $results['vehicles'][$veh]['wins'] = trim($match[2]); } } return $results; } $source_file = 'wotsource.txt'; $userData = getUserData($source_file); print_r($userData); Using the sample file you provided, here is what the content of the array looks like: Array ( [battles Participated] => 10 338 [Victories] => 4 963 (48%) [Defeats] => 5 166 (50%) [battles Survived] => 2 325 (22%) [Hit Ratio] => 61% [Destroyed] => 7 042 [Detected] => 12 848 [Damage] => 8 409 237 [Capture Points] => 13 725 [Defense Points] => 5 562 [Targets Destroyed] => 7 042 [Targets Detected] => 12 848 [vehicles] => Array ( [Type 59] => Array ( [total] => 1 083 [wins] => 539 ) [iS-3] => Array ( [total] => 588 [wins] => 252 ) [iS-8] => Array ( [total] => 563 [wins] => 257 ) [iS-7] => Array ( [total] => 379 [wins] => 178 ) [T-150] => Array ( [total] => 353 [wins] => 140 ) [iS] => Array ( [total] => 346 [wins] => 153 ) ) ) -
Aside from the problems noted above, but to your problem: If you want to restrict login based upon failed attempts you need to query the record by ONLY the username. Also, I would add a new field to the table called "last_login_failure". Then when the user attempts to login follow this approach: 1. If no record is returned then the username is unknown so just fail login. You can't really set a value for any user since you don't know which user to apply it to. 2. Assuming a record was returned, check the last_login_failure time (if it exists). If that time was less than 5 minutes fail the login. But do not update the last_login_failure. If you do, then if the user waitetd 4:50 seconds thinking the 5 minutes had expired the clock would be reset. 3. Assuming the last_login_failure was NULL or more than 5 minutes ago, then you check if the password is correct. If not, fail the login and set the last_login_failure datetime value. If the password is correct, then just log the user in. Using the above method you only need to set a value for last_login_failure when a username/password match is incorrect. You don't need to remove the value after a successful login.
-
Creating New Array Inside A Foreach Loop And Returning Its Value
Psycho replied to deadliver's topic in PHP Coding Help
OK, I created some sample data by working backwards from the processes you were using. Not sure if it is accurate or not - but it should be close. I then created a small function to extract the data you are apparently after. Here was the sample text I used for $curlstr <tr><td class="">Battles Participated</td> <td class="td-number-nowidth">99</td> </tr> <tr><td class="">Victories</td> <td class="td-number-nowidth">55</td> </tr> <tr><td class="">Defeats</td> <td class="td-number-nowidth">44</td> </tr> <tr><td class="">Battles Survived</td> <td class="td-number-nowidth">35</td> </tr> Here is the code I created to process that text: function GetStats($curlstr, $statList) { $results = array(); foreach($statList as $stat) { $pattern = "#<td class=\"\">{$stat}</td>.*?<td class=\"td-number-nowidth\">([^<]*)#is"; echo $pattern . "<br>\n"; if(preg_match($pattern, $curlstr, $match)) { $results[$stat] = $match[1]; } } return $results; } $CategoriesMajor = array( "Battles Participated", "Victories", "Defeats", "Battles Survived", "Destroyed", "Detected", "Hit Ratio", "Damage", "Capture Points", "Defense Points"); print_r(GetStats($text, $CategoriesMajor)); Here is the output of the print_r() for the results: Array ( [battles Participated] => 99 [Victories] => 55 [Defeats] => 44 [battles Survived] => 35 ) A couple notes: 1) If the array of categories is not used anywhere else I would probably define them inside the function. But, if the categories you need are the only patterns in the content that you need. You probably don't need to define the categories at all and can have the RegEx pattern just grab all the relevant data. For example, this gives the same result as above with my test data without having to define the categories: function GetStats($curlstr, $statList) { $results = array(); $pattern = "#<td class=\"\">([^<]*)</td>.*?<td class=\"td-number-nowidth\">([^<]*)#is"; if(preg_match_all($pattern, $curlstr, $matches, PREG_SET_ORDER)) { foreach($matches as $match) { $results[$match[1]] = $match[2]; } } return $results; } print_r(GetStats($text, $CategoriesMajor)); -
Creating New Array Inside A Foreach Loop And Returning Its Value
Psycho replied to deadliver's topic in PHP Coding Help
You accept you are a novice - and there is nothing wrong with that, we all have to start somewhere. So, with that understanding it is best, IMO, to lay out what you are really trying to accomplish. You may be taking the wrong approach entirely and we can help point you into a more efficient and elegant solution. Can you please provide some details such as: Sample of the originating data ($curlstr). I assume you are reading a single file and then reading parts of it based upon the "tags" in the array. You are taking multiple steps to get that data. So, it would be helpful to see a sample of the data showing the "tag" as well as the specific data piece you are trying to get. Although string functions are typically faster than regek - I think this might be a perfect problem for a regex solution. -
Php, Sometime A Strange Thing Is Happen When Get Data From Db
Psycho replied to pascal_22's topic in PHP Coding Help
No, it's not a good choice. There are many reasons but the one that I think is the most important is that by separating the "values" from the "data" makes the application much more difficult to maintain. You apparently have a list of eye colors, for example. In the database you are storing a 1, 2, etc. for the eye color. Then when you want to display the data you have to translate that ID into the value against the array. You also need to use the array when creating the form fields. Now, let's say you need to modify the list in the future. You have to make sure to keep the array absolutely in sync with the data in the database. Also, it makes it impossible to understand the data int he database without the arrays. Since it appears you are dealing with different languages here would be my recommendation: Create a separate table for each attribute description (e.g. "attr_eye_colors", "attr_hair_color", etc.). In these tables you would have a column for the id and then multiple columns for each language you support. I would name these columns using the standardized language code: either "fr" for french or if you want to be more specific "fr-ca" for french Canadian. Then when you need to get a user's data your query might look something like this: $query = "SELECt Profils.id, Profils.name, att_eye_color.$lang as eye_color, att_hair_color.$lang as hair_color FROM Profils AS u LEFT JOIN attr_eye_color ON Profils.eye_color_id = attr_eye_color.eye_color_id LEFT JOIN attr_hair_color ON Profils.hair_color_id = attr_hair_color.hair_color_id WHERE Profils.NomUsager='$NomUsager'"; $lang would be a variable that you set for the user (I'd probably store as a session variable) with the value of the appropriate language code for the user. -
Creating New Array Inside A Foreach Loop And Returning Its Value
Psycho replied to deadliver's topic in PHP Coding Help
Ridicule? I have no clue what you are ultimately trying to accomplish. I saw something that doesn't look right, but for all I know it is what you need. Rather than provide a lengthy explanation that may or may not apply I simply asked the question for the purpose of you to possibly take a second look to see if it is, in fact, what you intended. What did I mean by it? You have a loop that is extracting the index and the value of each element of the array into the varaibles $key and $value foreach($array as $key =>$value) Then within that loop you are apprently doign some processing of the $value variable which is then stored in a separate variable called $str, correct? Then at the end of the loop you are taking that processed value ($str) and putting it into a new array. But, you are using the value from the variable $value (i.e. the unprocessed, original value) as the key $Stat[$value] = $str; Based upon the processing that you are doing to get the result for $str, the variable $value contains HTML code. That would seem like odd content to be using as the index for an array element. But again, how the hell am I to know what you are ultimately using the data for? So, I simply asked the question. Also, you're welcome for me finding one of the two errors causing the underlying problem, Barand found the other. -
Php, Sometime A Strange Thing Is Happen When Get Data From Db
Psycho replied to pascal_22's topic in PHP Coding Help
So, you are getting values from the DB and then using some logic to determine the correct text to display, correct? And, we have gone through 15 posts in this thread before you felt the need to share that information? :-\ If you ran the debugging code I provided above and the results were consistently as expected then you should look into the logic to determine the correct text based on language. Don't know why we didn't suspect it was something like this before Oh wait, I did. -
Creating New Array Inside A Foreach Loop And Returning Its Value
Psycho replied to deadliver's topic in PHP Coding Help
Why you are using the full, unprocessed $value as the key when building array makes no sense to me, but whatever. As to your problem, take a closer look at the last few lines of the function and see if you can spot the problem. $Stat[$value] = $str; } return $Stats; } Hint "Stats" != "Stat" -
I try to refrain from using derogatory comments towards others (at least in this forum), but you are a flaming idiot if you think this is acceptable in any way shape or form. You have NO control over what servers an email will pass through from the origination to the destination. All it takes is one server that has been compromised by someone running a sniffer looking for patterns such as CC numbers. And the CC info for every single customer could be compromised.
-
Php, Sometime A Strange Thing Is Happen When Get Data From Db
Psycho replied to pascal_22's topic in PHP Coding Help
Um, if ANY parameters in the query change - then the query IS dynamic. Why would you think that performance would be improved by creating new variables for data you already have stored in another variable. Using the array $profile would be better in terms of performance, but any performance benefit would be miniscule. I just see no reason to create new variables as it only creates code bloat. I still think the problem has something to do with the code you are using to output the results and NOT the query. But, since you have not shared that info I'm not sure what else we can do to help. -
You should NOT be sending credit card information in ANY emails! That's crazy. If you are going to do that please tell me what your site is so I can be sure to never go there and to tell everyone I know not to go there. But, to your question I really don't know what the confusion is: $message1 = "This is the message for the first email"; $message2 = "This is the message for the second email"; $subject = "(Order Confirmation) - somesite.com!"; //Send first email mail($recipient1, $subject, $message1, $headers1); //Send second email mail($recipient2, $subject, $message2, $headers2); You would, of course, want to define the recipient and headers separately as well if they are going to two different recipients.
-
OK, I decided to extend the function a bit (I'm sure there is probably already pre-written ones to do this, but I had a few minutes to kill). The function takes three parameters. 1) $startTimeStr: The start time (as a string) for the period to be measured 2) $allUnits [Optional]: Determines if only the largest measurement is returned or the largest and all others with a non-zer0 value 3) $endTimeStr [Optional]: The end time of the period to be measured. If not passed (or false) will default to current timestamp. //Function to determine a textual representation of the time between two events function time_since ($startTimeStr, $allUnits=false, $endTimeStr=false) { //Set start and end times in seconds $endTimeInt = (!$endTimeStr) ? time() : strtotime($endTimeStr); $startTimeInt = strtotime($startTimeStr); if($endTimeInt===false || $startTimeInt===false) { return false; } //Calculate the difference $timeDiff = $endTimeInt - $startTimeInt; //Get the difference in seconds $measurements = array ( 'year' => 31536000, 'month' => 2592000, 'week' => 604800, 'day' => 86400, 'hour' => 3600, 'minute' => 60, 'second' => 1 ); $returnValues; foreach ($measurements as $label => $measurement) { if ($timeDiff >= $measurement) { $units = floor($timeDiff/$measurement); $plural = ($units>1) ? 's' : ''; $returnString = $units . ' ' . $label . $plural; if(!$allUnits) { return $returnString; } $timeDiff -= $units * $measurement; $returnValues[] = $returnString; } } if(!count($returnValues)) { return false; } return implode(', ', $returnValues); } ## USAGE $postedDateTime = '2012-09-24 13:14:02'; echo 'This post was updated ' . time_since($postedDateTime) . ' ago'; // This post was updated 2 days ago echo 'This post was updated ' . time_since($postedDateTime, true) . ' ago'; // This post was updated 2 days, 19 minutes, 33 seconds ago
-
There is a fundamental misunderstanding in this thread. Some believe that what is wanted is a concatenated output of multiple units (e.g. "1 year, 5 months, 3 days ago). However, that is not what I understand what the OP wants. I believe the OP just wants the LARGEST until measurement. So, if the time since is ~15 days then the output should be "2 weeks ago". I believe the problem the OP is having with the code is that he is testing with a FUTURE date/time which results in a negative value - which fouls up the entire logic. I have no idea what timezone the OP is in - so I can't be sure, but the test value used in his code $date = '2012-09-26 17:25:02'; has not passed yet for the US/Canada timezones I have rewritten the code in what I felt was a more logical format. function time_since ($startTimeStr, $endTimeStr=false) { //Set start and end times in seconds $endTimeInt = (!$endTimeStr) ? time() : strtotime($endTimeStr); $startTimeInt = strtotime($startTimeStr); //Calculate the difference $timeDiff = $endTimeInt - $startTimeInt; //Get the difference in seconds $tokens = array ( 'year' => 31536000, 'month' => 2592000, 'week' => 604800, 'day' => 86400, 'hour' => 3600, 'minute' => 60, 'second' => 1 ); foreach ($tokens as $label => $measurement) { if ($timeDiff >= $measurement) { $units = floor($timeDiff/$measurement); $plural = ($units>1) ? 's' : ''; return $units . ' ' . $label . $plural; } } return false; } $postedDateTime = '2012-09-26 12:25:02'; echo 'Mr. Tharanga added his tutor profile ' . time_since($postedDateTime) . ' ago';
-
Scratch that
-
Php, Sometime A Strange Thing Is Happen When Get Data From Db
Psycho replied to pascal_22's topic in PHP Coding Help
In addition to the above suggestion, I would also have the record ID added to the output for debugging purposes. But, if the query IS the same one being run each time then you should be getting back the same record. Well, unless you have some code that is modifying the "NomUsager" value for your records. Or, there could be some logic in the output code that is suppressing the display of certain fields. You have provided no code aside from the dynamic query - so all of this is just hypothetical guesses. You need to implement some debugging techniqes to see what is really happening. In addition to echo'ing the query I would do something such as this: $strSql = "select * from Profils inner join ........... where Profils.NomUsager='" . $NomUsager . "'"; $MonProfil = mysql_query($strSql,$cn) or die(mysql_error()); $profile = mysql_fetch_assoc($MonProfil); echo "Query: {$strSql}<br>\n"; echo "Record Count: " . mysql_num_rows($MonProfil) . "<br>\n"; echo "Date:<pre>\n" . print_r($profile, 1) . "</pre><br>\n"; Run your code and pay close attention to the data that is output. See what changes when the problem occurs. -
I wasn't suggesting you were asking for someone to build it for you. Only that the person building such a system would have to analyze the requirements before determining the database structure. If you want to "know how it works" then I suggest finding some resources regarding database normalization.
-
Inserting And Striping Html From User Comments
Psycho replied to freemancomputer's topic in PHP Coding Help
Yes I did mention that: There could be several examples where the result would not be as expected. The reason I do not always give a specific example is that an OP might think that the example given is the only problematic one and might dismiss it as not being relevant. If someone would like specifics they can ask and I will be happy to provide more details. -
Php, Sometime A Strange Thing Is Happen When Get Data From Db
Psycho replied to pascal_22's topic in PHP Coding Help
From what you provided it appears you are only getting the data for one record. Why, then, are you using a while() loop to extract the record? That aside, there is nothing from the code you have provided that would explain the behavior you described. One possibility is that the data is changing between page loads (e.g. someone added a height value), but I assume you are wortking from "known" data. Other than that, there has to be some other aspect to the functionality that you have not shared that is causing this. Are you using any data that is stored in session or cookie data? Are you sure that you are getting the data for the record you expect and that the data between the two page loads isn't really from two different records? One other possibility is that it is a cachng issue in the browser. -
Inserting And Striping Html From User Comments
Psycho replied to freemancomputer's topic in PHP Coding Help
You don't say how you want to apply the <p> and <br> tags in the output. If it is around the content then there is no problem. If it is inside the content that is a different matter. It is simple enough to add line breaks using nl2br(), but if you want <p> tags inside you many have to build some functionality. But, here's my position. There's absolutely no reason you have to use strip_tags() - especially for a comment. You should be very, very sure about removing any part of a user's input without them knowing. It could result in problems. The important thing is to ensure that the content is not treated as HTML code. For example if I add <b>bold</b> into my post here it doesn't appear bold. So, my opinioin is that the only data transformation you should do before saving the content is to trim it. Then when outputtng the data use htmlspecialcharacters() or htmlentities() to prevent the content from being interpreted as HTML. Plus, you should us nl2br() to add any line breaks. -
The answer is "It depends". It cannot be answered without knowing what data would need to be stored for that complext system. I think you are missing the point of how to detemine the database structure. The data to be stored and the logical association of that data should determine what the structure is. So, you can't just say something like "I want to build a shopping cart" and ask for someone to give you the appropriate database design. Sure there might be some basic elements, but YOU need to determine the requiremetns which would then determine the structure needed.
-
The solution will be based upon the features you want to support in this messaging system. If all you need is a "basic" system with sender/receiver ids, text of the message, date sent, and possibly a flag to determine if it has been viewed/read, then a single table is perfectly apprpriate and probably the best approach.
-
You need to start by renaming your fields so they are "logically" associated into arrays. Then you can easily create code to process the data without a lot of work. Trying to explain how to do this would take more time than just showing you. Here is a working script using a form just like the one you showed that dynamically creates the form and processes it. Try it out and then implement as you need it. <?php if(isset($_POST['name'])) { //Include separate php script to process the form //Putting here only for brevity $email .= "\n\nName: {$_POST['name']}\n"; $email .= "Date: {$_POST['date']}\n"; foreach($_POST['time'] as $time) { //Assuming Job No is required, only process records were job not empty $jobNo = trim($time['job_no']); if(!empty($jobNo)) { $desc = trim($time['desc']); $st = intval($time['st']); $ot = intval($time['ot']); $dt = intval($time['dt']); $email .= "\nJob Number: {$jobNo} -- {$desc} -- {$st} -- {$ot} -- {$dt}"; } } echo "<pre>{$email}</pre>"; echo "<a href=''>Go back to form</a>"; exit(); } //Create the duplicated form fields $records = 8; $formFieldsHTML = ''; for($i=0; $i<$records; $i++) { $formFieldsHTML .= "<tr>\n"; $formFieldsHTML .= "<td><input type='text' name='time[$i][job_no]' class='job_no' /></td>\n"; $formFieldsHTML .= "<td><input type='text' name='time[$i][desc]' class='desc' /></td>\n"; $formFieldsHTML .= "<td><input type='text' name='time[$i][st]' class='st' /></td>\n"; $formFieldsHTML .= "<td><input type='text' name='time[$i][ot]' class='ot' /></td>\n"; $formFieldsHTML .= "<td><input type='text' name='time[$i][dt]' class='dt' /></td>\n"; $formFieldsHTML .= "</tr>\n"; } ?> <html> <style> label, th { font-weight: bold; color: blue; } .job_no { width: 100px; } .desc { width: 300px; } .st, .ot, .dt { width: 40px; } </style> <body> <form action="" method="post"> <div style="float:left;"> <label for="name">NAME</lablel><br> <input type="text" name="name" id="name" /> </div> <div> <label for="date">DATE</lablel><br> <input type="text" name="date" id="date"/> </div> <br> <table border="1" id="timesheet"> <tr> <th>JOB NO.</th> <th>DESCRIPTION</th> <th>ST</th> <th>OT</th> <th>DT</th> </tr> <?php echo $formFieldsHTML; ?> </table> <button type="submit">Submit timesheet</button> <button type="reset">Reset timesheet</button> </form> </body> </html>
-
Yes, I am 100% in agreement with jesirose - your database schema is flawed. You should use a numeric type field to store the data. not sure what your intention of '-' is for. Do you interpret that as a 0.00 price or perhaps it means No Price? If you mean for that to be no price then you should change the field to a numeric type and allow nulls. Then set those fields to NULL. You're creating more problems for yourself later by doing what you are currently doing.
-
Security question. Hide files and folders under PHP's root directory
Psycho replied to Jagand's topic in PHP Coding Help
You should look at this thread: http://forums.phpfreaks.com/index.php?topic=357293.0 You can restrict access to files by using an htaccess file or you can put the files into a folder that is not in the web root. However, you stated that you wanted to do this for css files? CSS, images, JavaScript includes, etc are all requested from the browser. If you do not make them directly accessible you have to build functionality to make it work. So, if you have this in your HTML file <link rel="stylesheet" type="text/css" href="somefolder/style.css" /> . . . and the folder the file in is not accessible directly it will not be loaded by the browser. What you could do though is set the href to something like <link rel="stylesheet" type="text/css" href="getstyle.php?id=3" /> Then you need to build that script to take t he additional parameter, find the correct style sheet, read it into memory, and then spit it back to the client. But, that URL sill has to be web accessible - so you aren't gaining anything. YOu could make some more complicated solutions to ensure the request is coming from a logged in session or something like that, but ultimately CSS, Images, etc are always downloaded to the users PC anyway. It makes perfect sense to restrict direct access to PHP/Config type files that are included in the pages that are directly accessed. But, pages that are accessed because they are included via the HTML page does not make sense.