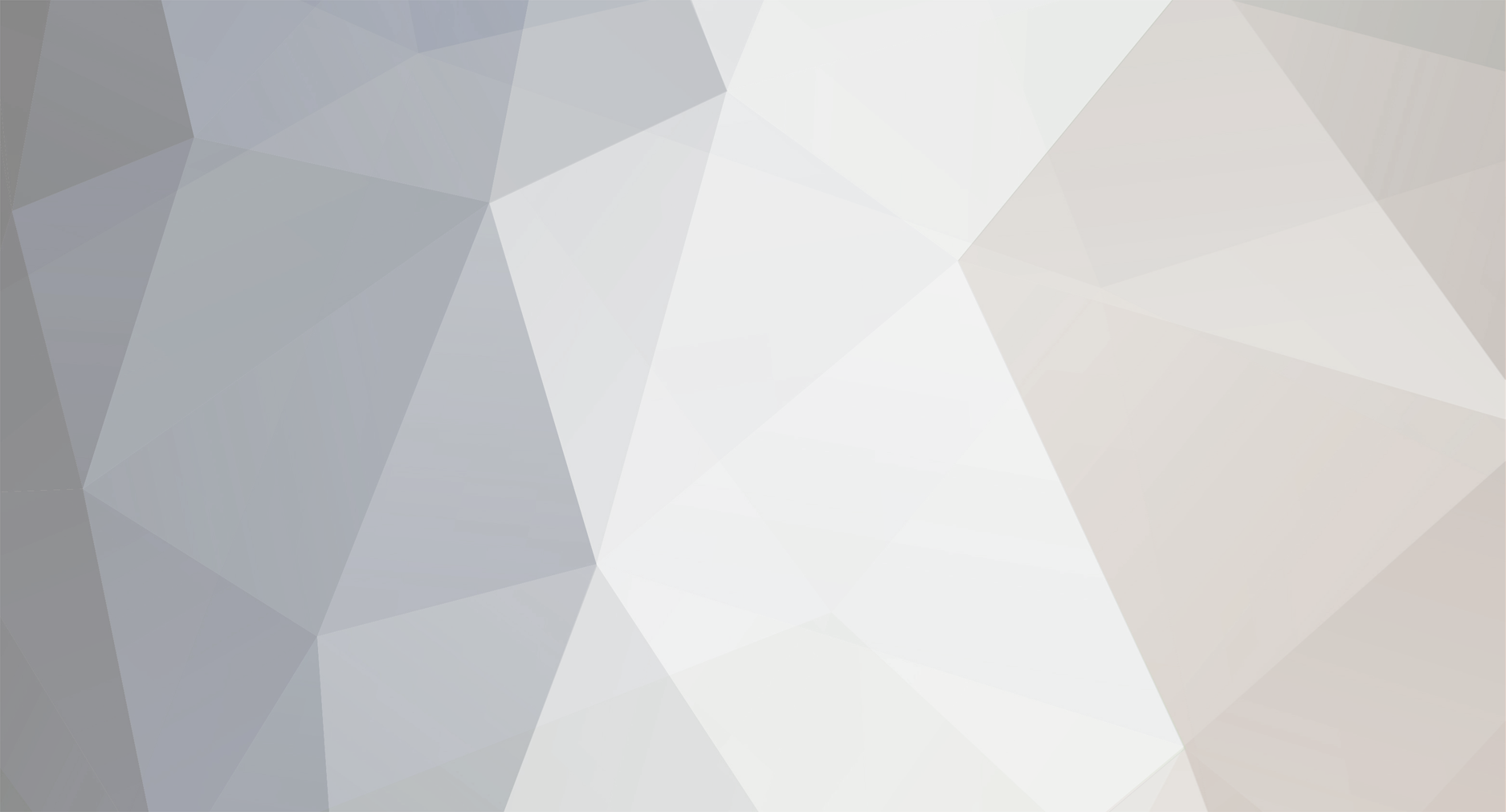
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
You have two problems: 1. The child elements are INSIDE the parent elements. So, when you click on the child you are also clicking on the parent. If you put an alert in the function you will see it popup multiple times based upon which child element you select. 2. The child elements will inherit the styles of the parent elements. The easy fix is to not have nested divs.
-
I think you missed the point. You were using $rememberme = "on" with one equal sign, which is an assignment operator - instead of two equal signs which is the comparison operator. Besides the usual way to identify if a checkbox was checked is simply isset(). In many cases (such as this one) the value of the checkbox really is not important.
-
I'm not going to read through all of that and try and decipher, but it looks like you are using an array to compile the errors. So, the solution should be pretty simple. For whatever code you do not want to process when there are errors (i.e. the code to send an email) wrap it in an if() conditional based upon whether that array has any elements. if(!count($errors)) { //Send the email only if there are no elements in $errors array }
-
Displaying database data in three vertical columns
Psycho replied to wchamber22's topic in PHP Coding Help
I think this is a little more strait-forward. include_once "connect_to_mysql.php"; $result = mysql_query("SELECT plantID, botanicalName FROM plants ORDER BY botanicalName"); $numrows = mysql_num_rows($result); $max_cols = 3; $max_rows = ceil($numrows / $max_cols); $count = 0; $tdData = array(); while ($row = mysql_fetch_array($result)) { $colIdx = floor($count/$max_rows); $rowIdx = $count - ($colIdx * $max_rows); $tdData[$rowIdx][$colIdx] = "<td><a href=\"plant_details.php?plantID={$row['plantID']}\" id=\"plantLink_{$row['plantID']}\">{$row['botanicalName']}</a></td>\n"; $count++; } $dyn_table = '<table width="750" cellpadding="0" cellspacing="0" border="0">'; foreach ($tdData as $rowAry) { $dyn_table .= "<tr>\n" . implode("", $rowAry) . "</tr>\n"; } $dyn_table .= '</table>'; Note: I changed the output to make the ID parameter of the anchor tags unique. You are not supposed to have multiple elements on a page with the same ID. -
"Electronic signatures" is a general term that applies to any means for a person to electronically indicate that they accept a document. What you will need to implement will be based upon the jurisdiction that you are in and the legal requirements for maintaining/applying the digital signatures. In other words, I think this really needs to start as a legal question (not appropriate for this forum). once you have the requirements, then you can pose the implementation question.
-
Your code is pretty messy, any there are some things that just don't make sense (for example you use variables that are never defined). So, I can't guarantee this code will work, but it should point you in the right direction. You are wide open to SQL Injection attacks. I wasn't sure on the field types for all your variables, so I didn't add logic to handle all of them. Your logic for determining if the record is existing or new is flawed. You should not need to query the DB to determine if the user is attempting to edit or create a new record. Typically, I set an "action" field int he form to make that determination. Alternatively, I assume you have an auto-increment column in the database for the primary key. So, you should be able to check to see if that field has a value. If so, the process should attempt an update. Otherwise, it should perform a new. It seems the length of the script to run is based upon the email part. First off there is no need to define the variables for the email in the loop. Only the email address is changing so redefining the content and headers of the email in the loop is inefficient. Instead of creating individual emails for each person you could simply add all the addresses together and send one email. Put the addresses as BCC if you don't want the users to see the others receiving the email. <?PHP if(isset($_POST['submit']) && $_POST['type']<>"") { $poster_id = $_SESSION['id']; $ID = intval($_GET['ID']) $short = $_POST['short']; $location = $_POST['location']; $type = isset($_POST['type']) ? intval($_POST['type']) : false; $starthour = $_POST['starthour']; $startmin = $_POST['startmin']; $endhour = $_POST['endhour']; $endmin = $_POST['endmin']; $reminders = mysql_real_escape_string($_POST['reminders']); $view = isset($_POST['view']) ? $_POST['view'] : false; $val = isset($_POST['val'])) ? $_POST['val'] : false; //Determine if this is a new record or one to be edited if($ID) { //Create and run query to update record $query = "UPDATE bl_calender SET datecotent='$reminders', location='$location', type='$type', starthour='$starthour', startmin='$startmin', endhour='$endhour', endmin='$endmin', short='$short', viewable='$view' WHERE dateclass='$ID' AND viewable='1' AND poster_id = $logOptions_id"; $result = mysql_query($query); if(!$result) { $msgToUser = "Error running Query: {$query}<br>Error: " . mysql_error(); } elseif(!mysql_affected_rows()) { // you are not the owner $msgToUser = '<br /><br /><font color="#FF0000">Sorry but only the owner can change the event details.<br> Close this window.</font><p></p>'; } else { $msgToUser = '<br /><br /><font color="#FF0000">Your event has been updated. Close this window.</font><p></p>'; } } else { //it's a new event we want to add $query = "INSERT INTO bl_calender (poster_id, dateclass, starthour, startmin, endhour, endmin, location, type, short, datecotent, viewable ) VALUES ('$poster_id', '$ID', '$starthour', '$startmin', '$endhour', '$endmin', '$location', '$type', '$short', '$reminders', '1')"; $result = mysql_query($query); if(!$result) { $msgToUser = "Error running Query: {$query}<br>Error: " . mysql_error(); } else { $msgToUser = '<br /><br /><font color="#FF0000">Your event has been created, Close this window.</font><p></p>'; // query the members who want an email $query = "SELECT email, email_work, firstname, lastname FROM myMembers WHERE notification_calendar = '1'"; $result = mysql_query($query); if(!$result) { $msgToUser = "Error running Query: {$query}<br>Error: " . mysql_error(); } elseif(mysql_num_rows($result)) { //Collect email addresses $addresses = array(); while($row = mysql_fetch_array($result)) { if(!empty($row['email'])) { $addresses[] = "{$row['firstname']} {$row['lastname']}<{$row['email']}>"; } elseif(!empty($row['email_work'])) { $addresses[] = "{$row['firstname']} {$row['lastname']}<{$row['email_work']}>"; } } $to = implode(', ', $addresses); //Set email parameters $webmaster = "KAI-DEFAT@minbuza.nl"; $headers = "From: MAAC Webmaster<$webmaster>"; $subject = "A new message has been posted in the MAAC Calendar."; $message = "A new event has been posted in the MAAC Calendar.\n"; $message .= "Goto the MAAC website to get the details.\n"; $message .= "Click here to view the Calendar $dyn_www/Web_Intersect/calen.php\n"; // send email mail($to, $subject, $message, $headers); } } } include_once 'msgToUser2.php'; } ?>
-
If you want a loading image you need to display that BEFORE you actually submit the data. Typically this is done when using AJAX enabled forms. Otherwise, once you submit the form the page will not display until it is done processing. A better solution is to identify WHY the page is taking so long to process and solve that. I see that you are running queries within a loop. This is a very bad idea and can cause huge performance issues. You have a SELECT query that then (within the loop to process those results) performs UPDATE queries. You should be able to accomplish that with one single query. If I get some time I'll analyze further to see if I can provide the appropriate query/code
-
LOL, I saw you used a flag variable ($first) and I was going to comment that I originally took that approach but made a last minute change in my code and instead used a temp variable to hold the content of the first cell (which I cleared after the first iteration of each type). Then I noticed that I still had the line $first = true; in my code which no longer had any purpose. Too funny. Not only did we go with the same approach, but we used the same exact variable name/value combination. As they say, great minds think alike.
-
Generally, you would want to use htmlentities() on any user supplied content when using that content in the HTML markup of a page. However, there are limitless scenarios and that is not the correct process for all of them. That is your job to determien what, if anything, needs to be done - and do it.
-
Typically, you want to store the data in its original format. Then, translate the data at the time you are going to output it. Here are a couple reasons why this is a better approach, IMO: 1. If you run the input through htmlentities(), or any other translation, you will expand the size of the content. This can cause problems when you are trying to define the size of your database fields. E.g. if you have a field that you only want to allow the user to enter 20 characters you will have to make the database field much larger to accommodate the content after it is run through htmlentities(). It would be uncommon for a user to enter A LOT of characters that would need to be converted. But, how many do you account for? To cover the absolute worst case scenario you would have to make the DB field at least 120 characters! 2. If you ever want to output the data into something other than an HTML page you will have to try and reverse the process of htmlentities(). By keeping the content in its original form you can easily translate the content for any output type you need.
-
1. When you explode() a string it is automatically converted into an array. You do not need to convert it to an array type as you did on the second line. 2. You want to use a foreach() loop to iterate through an array, not a while loop. $ingredientsAry = explode(',', $row_rs_recipes['ingredients']); foreach($ingredientsAry as $ingredient) { echo "<li>$ingredient</li>"; } In fact you can just do a foreach() loop on the explode() value foreach(explode(',', $row_rs_recipes['ingredients']) as $ingredient) { echo "<li>$ingredient</li>"; }
-
I think the problem you may be having is that the first row needs to include the type, but the subsequent ones do not. So, you need some conditional logic to handle the first row of each recipe differently than the rest. <?php $sushi = array( 'Fresh Tuna Sushi' => array ( 'Fresh Tuna Fillet' => '8 ounces', 'Sushi Rice' => '2 cups', 'Wasabi Paste' => '2 tablespoons' ), 'Shrimp Sushi' => array ( 'Large Shrimps' => '10 pieces', 'Vinegar' => '2 tablespoons', 'Sushi Rice' => '2 cups', 'Wasabi Paste' => '2 tablespoons', ), 'Nori Crab Sushi' => array ( 'Nori Seaweeds' => '4 sheets', 'Crabmeat' => '8 ounces', 'Cream Cheese' => '8 ounces', 'Worcestershire Sauce' => '3/4 teaspoon', 'Garlic Salt' => '1/4 teaspoon' ), 'Vegetarian Sushi' => array ( 'Rice Vinegar' => '1/2 cup', 'Sesame Oil' => '2 tablespoons', 'Nori Seaweeds' => '4 sheets', 'Sushi Rice' => '2 cups', 'Cucumber Strips' => '1 cup', 'Sweet Potatoes' => '1 cup', 'Sesame Seeds' => '2 tablespoon' ), 'California Roll Sushi' => array ( 'Sushi Rice' => '1 cup', 'Nori Seaweeds' => '8 sheets', 'Sesame Seeds' => '2 tablespoons', 'Crabmeat' => '1/2 cup', 'Mayonnaise' => '3 tablespoons', 'Avocado' => '1 piece', 'Cucumber Strips' => '1/2 cup' ) ); $recipe_output = ""; foreach ($sushi as $recipe => $ingredientsAry) { $ingredientCount = count($ingredientsAry); $typeCell = "<td rowspan='{$ingredientCount}'>{$recipe}</td>\n"; $first = true; foreach($ingredientsAry as $ingredient => $amount) { $recipie_output .= "<tr>\n"; if($typeCell != false) { $recipe_output .= $typeCell; $typeCell = false; } $recipe_output .= "<td>{$ingredient}</td>\n"; $recipe_output .= "<td>{$amount}</td>\n"; $recipe_output .= "</tr>\n"; } } ?> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title></title> </head> <body> <table border="1"> <tr> <th>Sushi</th> <th colspan="2">Ingredients</th> </tr> <?php echo $recipe_output; ?> </table> </body> </html>
-
Put the content into an array and then implode() the array with the commas. Before the foreach() loop define a temp array $subforums = array(); Then, inside the foreach() loop generate the content like this: $subforums[] = "<a href=\"?z={$data['id']}\">{$data['name']}</a>"; Lastly, after the foreach() loop, implode() the temp array values using a comma and assign to $forum_data['subforums'] $forum_data['subforums'] = implode(', ', $subforums);
-
Why do you need a separate submission page? The only thing you should be submitting is the ID. You should never include variables such as price as part of your submission since you already have that data in your database. The page that you submit to (i.e. the processing page) should take the product ID, query the DB for additional data that you need and then perform whatever processes you need.
-
No, it doesn't. You can't put "input" fields into the options of a select list. Besides,you are going about this entirely wrong. You should not put information such as price into a hidden field and use that in your processing logic. It is too easy for someone to modify those values. So, for example, a user could place an order for a product and submit the page such that the price value is $0.01. Instead you should only need to pass the ID of the product. Then, when you process the form you can get the price (or any other information for the product) from your database. That way you are certain to be using the correct information. So, based upon what you have above you would only want to query the name and id of the products (don't use * in your SELECT statements) and then use those two values for the value and label of the options.
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=353189.0
-
I was responding to your previous post, was I not? I'm finished with this thread.
-
You simply need to tap the breaks a bit and slow down. You are not understanding what has been stated multiple times. Or, you are simply trying to be difficult. No it would NOT. As long as the data is "Sanitized" using prepared statements or something like mysql_real_escape_string, then that input would do absolutely no harm. It would simply set the name as the literal string "a';DROP TABLE users;". THAT is the whole point of Sanitizing the data. It ensures that the data is safe for use in a query. Fair enough, but to me, they are interrelated. Related, maybe, but they have very different purposes. You should ALWAYS sanitize the input and the methods for doing so are pretty standard. But, "validation" is a whole other can of worms for which there is no standard. The validations will be based on your particular requirements. As stated before, you may decide to require that phone numbers contain 10 digits, that emails are in a proper format, that a date is of a certain format, etc. etc. I've already answered your question multiple times. Any regex you implement is not adding any security. If you want to go to the trouble of excluding certain content for the name field, or any other, go ahead. That is your perogative.
-
searching a range when only high/low in database
Psycho replied to turpentyne's topic in PHP Coding Help
You really need to redo your table. By storing the values as "12 cm" you lose the ability to use a lot of the database functions. I would suggest you do as jcbones suggested and have one record for each entry with a high and low value. I would also suggest you store the values as numeric fields in the smallest unit. So, if you have "6 cm" save it as 6000. Then create a couple of functions to convert microns into an appropriate length and to convert lengths into the numeric value for microns. -
Fair enough, but to me, they are interrelated. Well, then, you would be wrong. Sanitizing/Escaping an input to prevent SQL Injection or other types of errors is very different from validating that the input is of the format that you expect. A value such as "D33bb13" would have absolutely no security risk with respect to a name value. YOU keep changing what you are talking about. Figure out what you are trying to achieve and stay on point. As long as your input is being properly escapes the RegEx is doing nothing to make the input more or less safe. It is an arbitrary validation that you are implementing. If you feel that it is necessary, then by all means use it. But, it doesn't do anything to improve security. Validation such as those can be necessary/warranted - such as the input for a phone number to ensure it contains 10 digits. That will work. But, I personally hate putting PHP logic inside my markup. My preference would be to simply define $firstName in the "logic" of my code and then simply output it within the HTML markup. In the logic of the code $firstName = isset($firstName) ? htmlspecialchars($firstName, ENT_QUOTES) : ''; $firstNameError = (!empty($errors['firstName'])) ? "<span class='error'>{$errors['firstName']}</span>" : ''; In the display/output of the code <!-- First Name --> <li> <label for="firstName"><b>*</b>First Name:</label> <input id="firstName" name="firstName" type="text" maxlength="20" value="<?php echo $firstName; ?>" /> <!-- Sticky Field --> <?php echo $firstNameError; ?> </li> makes the code much more readable, IMHO. But, use whatever works for you.
-
Per the manual for $_SERVER Your if() condition simply checks to see if the request method was 'POST'. There are three other options, so in the else{} block you cannot definitively assume that the request method was 'GET'. But, for most purposes it will be 'GET'
-
^^ That is what I would use. 0 is treated as the logical 'false', so if 0 records are returned that condition will be true.
-
Who said anything about JavaScript? My point was simply that the requirements for this changed in the thread from implementing a security measure to preventing "obnoxious" names. If the requirement is to prevent SQL Injection, properly escaping the input (either via mysql_real_escape_string(), prepared statements, etc.) will prevent SQL Injection attacks. Adding logic to prevent certain inputs does not improve security (with regard to SQL Injection). If the requirement is to prevent "obnoxious" names then more detailed requirements need to be defined to create appropriate code. However, you also need to consider "how" the input will be used. If the input is stored and then later display int he HTML content, then you will need to run the input through htmlspecialchars() when generating the output. Otherwise, you are at risk of XSS attacks - which is an entirely different thing than SQL Injection.
-
Before you code anything you need to know what the requirements are. You are changing the requirements between posts. The escaping of the input prevents any security risk (which is what you were first asking about). But, now you are saying you want to prevent names that you feel are obnoxious. Only YOU can define the specific rules for what YOU feel is an obnoxious name. The main thing to keep in mind with creating such a process is that you have the risk of creating false positives, i.e. excluding the real name of a person. Regex operations are typically a costly operation and should only be used when necessary. If you really feel that you need to restrict names then state what your rules are. FYI: Your current expression would find many valid names as invalid - most notably accented characters such as รค. The US is FULL of people from around the world who would have such names. Personally, I would not go to the trouble to try and do these validations as it, in my opinion, is just a waste of time. If someone wants their name to be Zippity Do Da on my site, what do I care? Of course, if they are making a CC purchase they will have to provide the valid name for the account.
-
Not sure what you mean. The loop WILL continue to the next value on each iteration. Based upon the code you posted (assuming sequential, numerically based indexes) if the values for key ($K) 3 meet the criteria, $data[0][3] will be unset and the loop will start over with $k being equal to 4. I think you are leaving out some pertinent information. however, I will add that I think you might want to look into using array_filter() with a custom function.