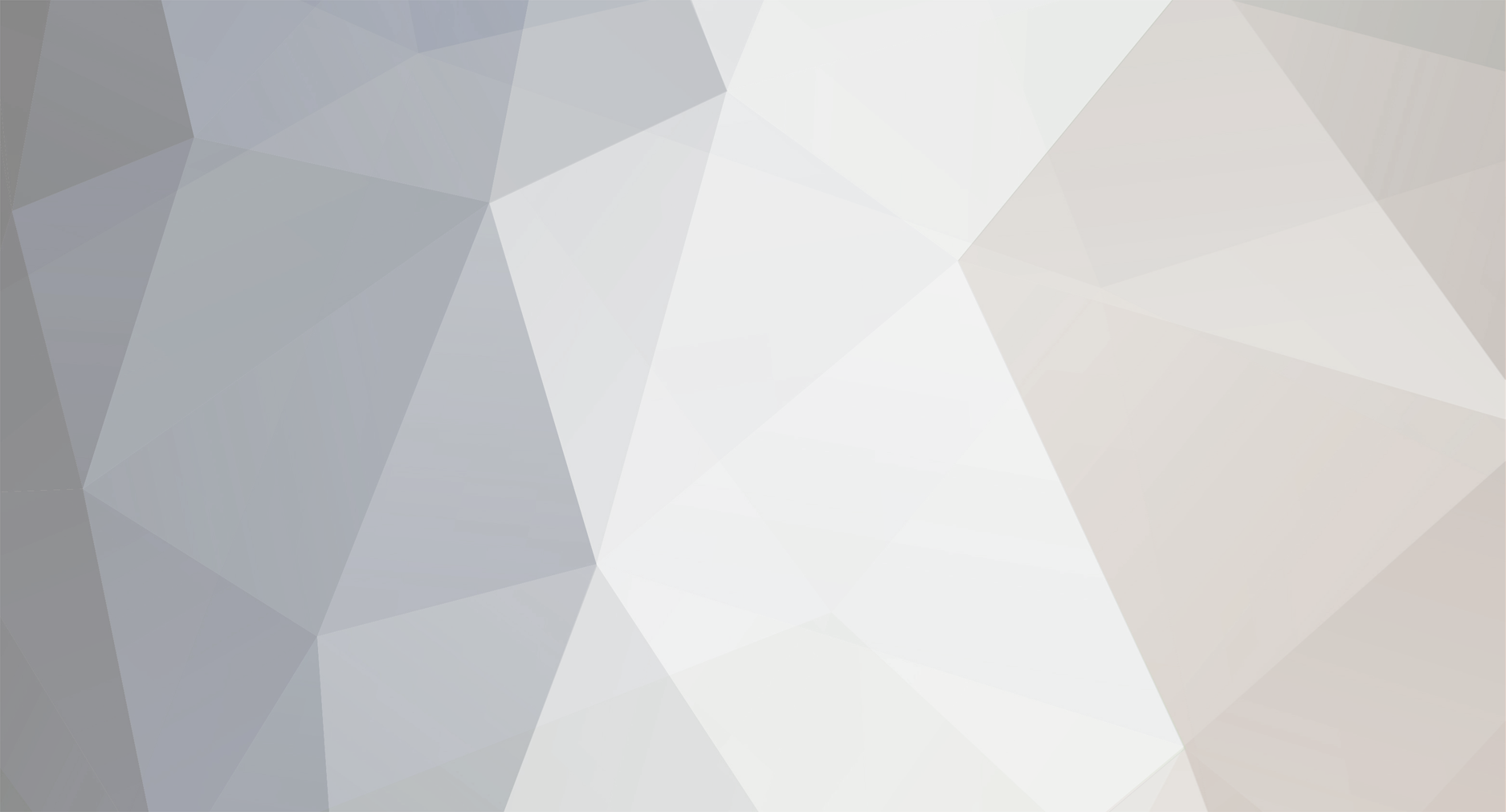
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, that will be very problematic because you have no way to know when a user has "logged off" on one machine in order to allow them to log in on another machine. You ahve two choices: 1) When a user logs in, save the session ID to the data base and have a field to set their "last access" time. Then do not allow another machine to log in as that user as long as the last access time is less than some time limit. For example, you could set the time limit to 20 minutes. So, if you do not see any activity from a user within that time frame you allow them to log in from another machine for the same account. Then when you update the session ID in the table, the first machine will be considered "logged out" so, using the value in the database, you would kill the first machine' session. 2) Using a similar approach to above you could do the same thing but don't use a time limit. So, whenever an authentication attempt is made, if there is an existing session for that user, you would destroy the session for the first machine before completing the authentication of the second machine.
-
You'll want to use two queries. It might be able to be done in one, but I think it would get overly complicated. Query #1 will be to get the number of records that match the condition SELECT COUNT(miles) as limit_amt FROM mpg WHERE vehicle_id='$id' Then take the value from that query, subtract 2, and assign it to a variable (let's say $limit_amt). Now run this query to get the average of the records except the oldest two SELECT AVG(miles) AS av_miles FROM (SELECT miles FROM mpg WHERE vehicle_id='$id' ORDER BY date DESC LIMIT $limit_amt) as data_set
-
If they are numbers store them as numbers. An int of x number of characters would take less memory than a varchar of the same number of characters.
-
How do I run a cron job? and what is the code for a cron job? A cron job is just a scheduled process where you can specify a PHP file to run at a certain period/interval. How you set that up would be dependant on the type of server you are running. If you are using a host look into the tools your host provides. Also, be aware that the code provided below is only "example" code. You would need a delete query for all tables that you need to delete from and those tables must have a field where you are already tracking the date created for the data. Additionally, you need to take foreign key references into consideration. You should not delete records if those records are used as a foreign key reference in another table. Lastly, if you are capturing the date created for records (which you must do in order to implement this), then you probably don't need to delete the records anyway! If you want to display a list of records that were created in the last 30 days, then just format your queries to do that instead of deleting the old records. You need to be very sure that you don't want data before deleting it.
-
Absolutely not! Never run queries in loops. Why would you run a SELECT query to get all the records that are older than 30 days only to loop through each of the records in the result to perform a DELETE. You can simply run ONE query to delete all the records older than 30 days. Example DELETE FROM table_name WHERE date_created < DATE_SUB(curdate(), INTERVAL 30 DAY)
-
The problem must be in the code you have in the database. There is likely a tag that was not properly closed or there are closing tags that shouldn't be there. View the page source code and check the results.
-
You should NEVER run queries in loops!!! As to your last question. As requinix stated you have a value $i which goes from 0 to 99, so just use $i + 1 and display next to each record. But, you should really modify that code so you are not running queries in a loop. I'll take a look and see if I can provide you something. EDIT: Where is the query that provides the first result set $rs? EDIT #2: Font tags? Really? Those have been deprecated for years.
-
If you are using PHPMYADMIN, on the form to set up a DB field there is a column for "Default Value" if the "type" of field is "timestamp" the deafult clumn will have a checkbox titled "CURRENT_TIMESTAMP". Check it. Or you can run a query such as this: ALTER TABLE `table_name` ADD `date_created` TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
-
No idea what you are talking about. What field input?
-
After looking at your two queries I see that there is some unnecessary logic in the method get_parent_job_list(). Your WHERE clause is as follows where (par_job_id is null and ((name like '%PJ%') or (name like '%PD%'))) OR (par_job_id is null) That is logically the same as where par_job_id is null Those LIKE portions do nothing because the part before the last OR matches all records that par_job_id is null AND the name matches those conditions. But the part after the last OR matches ALL the records where par_job_id is null. Anyway, create the following method: function get_full_job_list() { $sql = "SELECT p.job_id as parent_id, p.name as parent_name, c.job_id as child_id, c.name as child_name FROM job AS p JOIN job as c ON c.parent_id = p.job_id WHERE p.par_job_id is null ORDER BY p.name, c.name"; $rs = get_rs_array("db", $sql); return $rs; } Note: I created the method to sort the results based on names as that would make sense to the user. You can change if needed Then call the method and format the results in a hierarchical array. $full_job_list = get_full_job_list(); $job_list = array(); foreach($full_job_list as $idx => $job) { if(!isset($job_list[$idx])) { $job_list[$idx] = array('p_id' => $job[0], 'p_name' => $job[1], 'jobs' => array()); } $job_list[$idx]['jobs'][] = array('c_id' => $job[2], 'c_name' => $job[3], } Lastly, change your smarty code to correspond to the hierarchical array. {section name=par loop=$job_list} <optgroup label="{$job_list[par]['p_id']}, {$job_list[par]['p_name']}"> {section name=job loop=$job_list[par]['jobs']} <option value="{$job_list[par]['jobs'][job]['c_id']}">{$job_list[par]['jobs'][job]['c_id']}, {$job_list[par]['jobs'][job]['c_name']}</option> {/section} </optgroup> {/section}
-
OK, so you provided how the lists are currently constructed, but you didn't state how you wanted them changed. I like to help, but I want to see that you at least put forth some effort. You will probably want to create a mufti-dimensional array. You could do it using the two methods you currently have - but that would mean running multiple queries to get all the child records. That is inefficient. You should instead have a new method to get ALL the data with a single query. Then create the mufti-dimensional array, then change your template code accordingly. Let me take a look and see what I can come up with and I'll post back.
-
Then you need to show the structure of the arrays that are created and state how you want the structure changed. From what you are saying the arrays are being created, just not in the format you need them. But, all you've shown (of PHP code) is a couple of lines that apparently call some custom methods that return data. How the hell are we supposed to know what format the data is returned or how you want that format to be?
-
No, I do not get what you meant. You can't overwrite content you have already generated (well, technically you can, but that is not appropriate here). Looking at your mock page you have a section for three "featured" videos and another section for up to 24 videos. You simply need to run the appropriate queries to get UP TO the number of videos you want. If you only want the newest videos then only get the 24 most recent videos. As to how to output them, you simply have a series of divs - so just output the content in divs. Also, if you don't have it, create a new field in the table for the creation date. You can set it up so the value is automatically set when creating a new record. Sorting by the id to indicate date added is not correct methodology. Example $query = "SELECT * FROM `G4V_Videos` ORDER BY `date_added` DESC LIMIT 24"; $result = mysql_query($query); if(!$result) { echo "Error running query: " . mysql_error(); } else { while ($row = mysql_fetch_assoc($result)) { echo "<div id='Video_thumbnail'>"; echo "ID-nummer: {$row['id']} - Name: {$row['navn']} - "; echo "<a href='/Video.php?id={$row['id']}'>"; echo "<img src='http://i.ytimg.com/vi/{$row['link']}/hqdefault.jpg' width='200' height='160' /></a>"; echo "<br>"; echo "<a href='/Video.php?={$row['id']}'>"; echo "</div>"; } }
-
That isn't PHP code. Wrong forum.
-
Don't use short tags. This line is completely wrong $sql=("SELECT * FROM user_alerts WHERE user_id = '".($_SESSION['user_id'])."'") or die (mysql_error()); That is simply assigning a string to the variable $sql and if that fails would attempt to show the mysql error. You need to be checking if the execution of the query fails! Also, do not use 'NO' for your database value. Because then you have to do comparisons on the value to see if it is true or not. For boolean values use a 1 (true) or 0 (false). Then you don't need to do string comparisons. I wrote the code below using the 'NO' value - but it is really poor implementation. Lastly there is no reason to do a query of all the records for the user when you are only interested in the unread ones. SO, include additional criteria on the WHERE clause so you don't have to process unnecessary records. Not tested require'xxxxxxxxxxxxxxxxx.php'; mysql_select_db("membership") or die( "Unable to select database"); $query = "SELECT COUNT(user_id) FROM user_alerts WHERE user_id = '{$_SESSION['user_id']}' AND messageRead = 'NO'"; $result = mysql_query($query); if(!$result) { echo "Error running query: $query<br>Error: " . mysql_error(); } else { $mail_count = mysql_result($result, 0); if($mail_count>0) { echo "<div>\n"; echo "<img src='xxxxxxxxxxxxxx.png' width='32' height='32' alt='unread mail' />\n"; echo "<a href='http://www.xxxxxxxxxxxx/account=account'>You have {$mail_count} Messages!</a>\n"; echo "<div id='boxes'>\n"; echo "<div style='top: 199.5px; left: 551.5px; display: none;' id='dialog' class='window'>\n"; echo "<h2>You have new mail!</h2>\n"; echo "<br />\n"; echo "<p>Visit your account page to read it.</p>\n"; echo "<br>\n"; echo "<a href='#' class='close'>Close Window</a>\n"; echo "</div>\n"; echo "<!-- Mask to cover the whole screen -->\n"; echo "<div style='width: 1478px; height: 602px; display: none; opacity: 0.8;' id='mask'></div>\n"; echo "</div>\n"; echo "</div>\n"; } }
-
No idea. It would be based on how efficient you make it (which doesn't sound too efficient at the moment), the server specs and the available bandwidth. You would need to do some load testing to find how many users you could support. Your messages should have a timestamp of when they are created. So, you use the timestamp to get all new messages using a query such as SELECT message FROM message_table WHERE create_time > '$timestamp' ORDER BY create_time
-
No. Since this will be checking for updates frequently you want to keep the amount of data being sent and returned from the server to an absolute minimum. So, one solution is to use a timestamp. In the PHP script you would get the timestamp of the time you first grab results for a user. And, in addition to sending those results to the client you would also send the timestamp. Then when the client does a check to get new content it ONLY sends the timestamp. The server checks to see if there is any new content since the timestamp passes and sends the results and a new timestamp. The JavaScript code will append the new content to the existing content and store the timestamp for the next request to the server. So, when there is no new content, the only thing you are passing back and forth is the timestamp. Yeah you did. But I like to make things break. For what it's worth your didn't break. But, you should be running the content through htmlentities() or htmlspecialcharacters() before sending back to the client.
-
First of all, if you are looking for people to beta test your project, there is a forum and procedures for that. As for the specific issues you are having, what steps have you taken to debug the problems and what were your results. I for one am not willing to download and read through all your files to try and figure out what the problems might be. It is your program and you should be able to know what decision points in the logic that could cause your problems. You need to add debugging code to see what the values are compared to what you expect them to be. Then you can isolate the exact cause of the problem. How often you check the server for updates its up to you. 1 second sounds reasonable. Will that crash your server? Not likely. But, I don't know what the specs are for your server or the traffic it gets. You absolutely should be using a timestamp in order to ONLY get new content instead of all the content for the thread when you make a call though. SO, in most cases the client will make a request of the server, the server then finds there was no new content and simply returns a '0' (to indicate false) or some other very small piece of data.
-
Start with an empty style sheet. Then add one section. Test. If everything works add another section. Test. Continue this process until the scroll bar breaks. Then check the code and see what may be causing it and try modifying that code to get what you want using different parameters.
-
What is the purpose for this? The ultimate use for the font could have implications on what solution you should be going after. It also might affect what type of web server you should be using Windows vs. Linux. I used to work for a company that developed web-based solutions for the printing industry - most notably the ability to create print-ready files through a web application. Fonts was probably one of the most problematic aspects.
-
That logic is flawed in the manner you implemented it. $query="SELECT email FROM users WHERE email='$email'"; $row=mysql_query($query); $result=mysql_fetch_row($row); if ($email==$result[0]) { return 1; } else { return 0; } If the email doesn't exist int he DB then $result will be the Boolean false - not an array. So, $result[0] doesn't exist either. The point is you only need to query the DB for records matching the value - then check if there were any results. You don't need to extract the results and compare them.
-
I don't think ROLLUP will get you what you want. Your request is kind of misleading, but after reading it a couple of times and looking at your code I think I understand what you want. You want a result set of every team and for every team you want 1) that teams total score and 2) that teams total for the last five games. I think you are going to need to use a sub-query and I don't know how efficient this query would be. I'll try some things out and see if I can come up with a solution then post back.
-
Your query is likely failing. You'll need to view the error to know why. Also, give your variables appropriate names to prevent confusion. In the example above you assign the "result" of the query to a variable named $row. You should use $result to hold the result of the query and $row as the variable to hold the data from a record (or row) from the result set. This is pretty much a standard. Plus, there is no reason to do a test of the value and return a 1 or 0 - the result of the comparison will do that for you. Lastly, the logic of the above does not make sense. You are pulling the email address where email address equals $email. Then you are doing a comparison between the retrieved value and $email. Except for differences in letter case they would always be the same value. If you are trying to see if that email email already exists you should be checking the count of records returned. $query = "SELECT COUNT(email) FROM users WHERE email='$email'"; $result = mysql_query($query) or die(mysql_error()) //This will return 1 if the email exists, 0 otherwise return(mysql_num_rows($result);
-
You don't have a closing curly brace after the second function: error_for(). The last curly brace closes the if() statement.
-
Well, looking at one of your earlier posts (http://www.phpfreaks.com/forums/index.php?topic=345762.msg1632361#msg1632361) it looks like the real problem is how you are storing the data. You should not store multiple pieces of data into a single field. Instead of taking some time to learn the proper way to work with this data (associated tables) you are building an unsustainable solution.