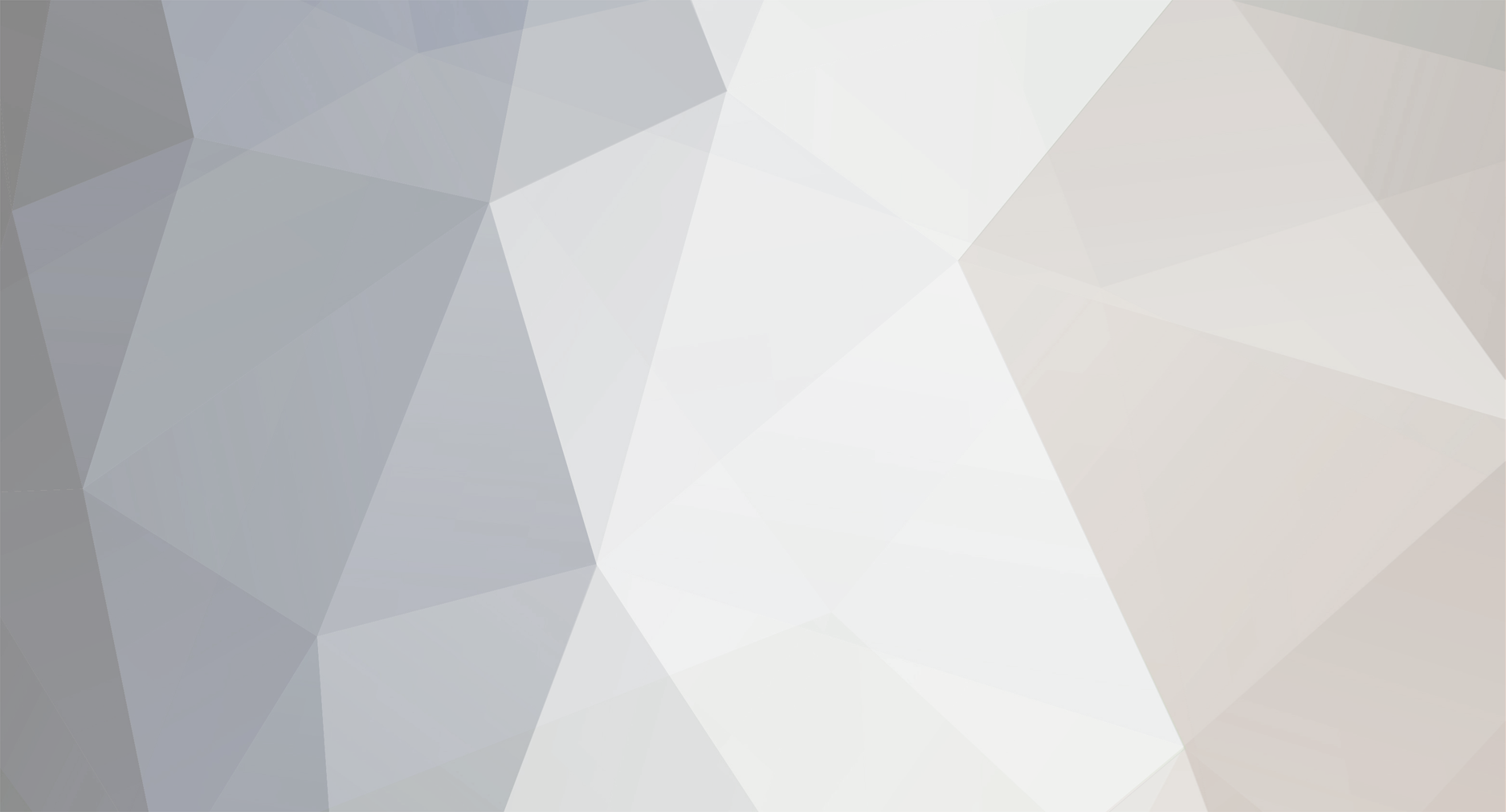
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Question about Array/Other functions to compare data.
Psycho replied to Travis1128's topic in MySQL Help
Had you mentioned that "p_id" was being passed and was needed in the query you would have saved us both a lot of time. That explains why you are getting multiple entries - the foreign keys in the "Product_Categories" table have duplicates. You should just need to modify the query. I assume there is only one entry for each category in the "Product_Categories" table associated with any particular "p_id". <?php $p_id = int_val($_GET['p_id']); $query = "SELECT C.ID, C.Name, IF(C.ID = PC.Cat_ID, 1, 0) AS selected FROM Categories AS C LEFT JOIN Product_Categories AS PC ON C.ID = PC.Cat_ID AND PC.Product_ID = $p_id ORDER BY C.Name"; $result = mysql_query($query); //Create options list $catOptions = ''; while($option = mysql_fetch_assoc($result)) { $selected = ($option['selected']==1) ? ' selected="selected"' : ''; $catOptions .= "<option value=\"{$option['ID']}\">{$option['Name']}</option>\n"; } ?> <select name="p_categories"> <?php echo $catOptions; ?> </select> If this doesn't work, then start with the query. Test the results to see if they make sense. If not, then we need to adjust the query. If they do make sense then there may be a problem in the PHP code that parses the results -
Question about Array/Other functions to compare data.
Psycho replied to Travis1128's topic in MySQL Help
Too complicated? No, I don't think so. Trying to put a bunch of IF statements for each and every option is unnecessary. I already provided that logic in the code I posted previously using the ternary operator. I asked YOU to display the code you were using and provided some "sample" code. I didn't say it would work out of the box because I have no idea what you are doing in the code since you have provided absolutely no code. Based upon the details you have provided the logic I have provided will work if implemented correctly. I explained what the results of the query I provided should return. Did you test the query and check the results to see if they are valid for waht you need, or did you simply copy/paste because you are too lazy to think for yourself? The only way that the options in the select list would be duplicated is if there are duplicates returned from the query (which shouldn't occur if they are unique records in the Categories table) OR if you left in hard coded values in that list. Why don't you start by running the query and pasting the results here. BY the way, what is "p_id"? I presume the product id, but you did not refer to that anywhere previously. based upon your first post you wanted a select list of every category and if the category was referenced in the Product_Categories table, then you wanted it selected. -
Question about Array/Other functions to compare data.
Psycho replied to Travis1128's topic in MySQL Help
Where is the code you are using to generate the select list? The query I provided would return two fields: 1) the name from the category table and 2) a dynamic field "selected" that will be a 1 or 0. You need to use that second field to determine whether the record is selected or not (using the correct HTML format). Also, that query will need to be changed to also get the category id. Sample code <?php $query = "SELECT C.ID, C.Name, IF(C.ID = PC.Cat_ID,1, 0) AS selected FROM Categories AS C LEFT JOIN Product_Categories AS PC ON C.ID = PC.Cat_ID ORDER BY C.Name"; $result = mysql_query($query); //Create options list $catOptions = ''; while($option = mysql_fetch_assoc($result)) { $selected = ($option['selected']==1) ? ' selected="selected"' : ''; $catOptions .= "<option value=\"{$option['ID']}\">{$option['Name']}</option>\n"; } ?> <select name="p_categories"> <?php echo $catOptions; ?> </select> -
No, you just need to do a COUNT() on a single field (do not include other fields in the SELECT statement) and remove the GROUP BY. Using your original query: SELECT COUNT(number) FROM `test` WHERE number LIKE '%1%' AND number LIKE '%2%' AND number LIKE '%3%' That will return a single result of the number of records matching the WHERE clause.
-
Question about Array/Other functions to compare data.
Psycho replied to Travis1128's topic in MySQL Help
SELECT C.Name`, IF(C.ID = PC.Cat_ID,1, 0) AS selected FROM Categories AS C LEFT JOIN Product_Categories AS PC ON C.ID = PC.Cat_ID EDIT: Hold on, I don't think that's right. Let me do some checking. EDIT #2: Nope, it works. -
You are being presumptuous silkfire. While your solution would work with data exactly like that shown it would not work with data that has different numbers of characters. For example, if the numbers being searched are "1", "2" and "3" and there is a number in the database such as "1123" your code would match that and mine would not. Which is right? Neither of us knows. The OP didn't give very explicit requirements, so I over-compensated in my solution to account for multiple scenarios. And, you don't need a GROUP BY to get the count if you are only using COUNT against one field as Muddy_Funster showed.
-
If you are always going to be comparing three digit numbers, then I would suggest you do this in the query itself by determining the possible combinations and counting the results that match. $match = "123"; //create combinations for where clause $combos = array(); $combos[] = "`field` = $match[0].$match[1].$match[2]"; $combos[] = "`field` = $match[0].$match[2].$match[1]"; $combos[] = "`field` = $match[1].$match[0].$match[2]"; $combos[] = "`field` = $match[1].$match[2].$match[0]"; $combos[] = "`field` = $match[2].$match[0].$match[1]"; $combos[] = "`field` = $match[2].$match[1].$match[0]"; //Create query to count mathching values $query = "SELECT COUNT(*) FROM table_name WHERE " . implode(' OR ', $combos); The resulting query would look something like this SELECT COUNT(*) FROM table_name WHERE `field` = 123 OR `field` = 132 OR `field` = 213 OR `field` = 231 OR `field` = 312 OR `field` = 321
-
I did not test the code I provided previously. I just created a test database and after fixing a minor syntax error it works. To fix the syntax error, change this if(isset($_SESSION['username']) To this if(isset($_SESSION['username']))
-
OK, I see the problem. I didn't specify a method on the form and it defaulted to the GET method, but the code was looking for the selected value in the POST method. Change the form to <form name="menuform" method="post">
-
This is more of a suggestion, but the logic is backwards. You should have the code for the form at the end of the script. That way if the user did not submit credentials OR if login fails you can display the form and repopulate the user id. Also, regarding ZulfadlyAshBurn's update there is a problem with this: $submit = $_POST['submit']; That can cause errors to be displayed depending on the error reporting level. Plus, later in the code there is a check using if(!$submit) { A non value will be interpreted as false, but that is a sloppy method. I would suggest this isset($_POST['submit']) As for your script you are not setting the session value anywhere - only trying to display it. Also, the FONT tag has been deprecated for YEARS - stop using it. You were using it wrong anyway - you have three opening font tags and only one closing tag. You can put multiple parameters into one opening tag. Don't use PHP_SELF - it is not safe. Just leave the action parameter empty or do some research on the proper way to set the value. Lastly, you are using the password in plain text in the database. You should be hashing the password (preferably with a salt). I didn't do anything with the password in the script below because you would have to create the hashing process to also implement in the script that creates the user records. Here is a complete rewrite fixing many different problems and providing a more logical flow. <?php session_start(); $con = mysql_connect("localhost","username","password"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("database_name", $con) or die('Could not select db: ' . mysql_error());; //Create text var to hold any error messages $errorMsg = ''; if(isset($_POST['submit'])) { $username = mysql_real_escape_string(trim($_POST['username'])); $password = mysql_real_escape_string(trim($_POST['password'])); if(empty($username) || empty($password)) { //Username and/or password is empty $errorMsg = "Username and password are required."; } else { //Create and run query to validate credentials $query = "SELECT userid FROM users WHERE username='$username' and password='$password'"; $result = mysql_query($query); if(mysql_num_rows($result)!=1) { //Validation failed $errorMsg = "Error! Invalid username and password combination."; } else { //Validation passed $_SESSION['username'] = trim($_POST['username']); ##Ideally you should redirect to a welcome page using a header() after setting the session vars. ##For illustrative purposes we will display a confirmation message and exit the script echo "You are now Logged In, {$_SESSION['username']}"; exit(); } } } //Validation was not done or failed, unset the session var if it exists if(isset($_SESSION['username']) { unset($_SESSION['username']); } ?> <html> <head></head> <body> <div style="color:red;"><?php echo $errorMsg; ?></div> <h2 style="font-family:verdana;">Login</h2> <form action="" method="post"> Username: <input type="text" name="username" value="<?php echo trim($_POST['username']); ?>"><br> Password: <input type="password" name="password"><br><br> <input type="submit" name="submit" value="Log In"> </form> </body> </html>
-
There are ways to do "push" functionality with a web server, but I am no means an expert on it. Is there some reason you cannot simply increase the polling frequency for your delphi app? If it is a process that take a lot of time/resources I can think of one simple solution with regular "pull" functionality. On the web app allow users to select an option to have new data collected which, in turn, stores a flag in the database. Also, have the delphi app store a timestamp in the database every time it performs the operation to collect & store new data. Lastly, increase the polling of the delphi app to 1 minute (or whatever interval you choose) and instead of it automatically performing the data collecting process, instead have it make a call to the web app. Use that call to a PHP script which will determine if new data need to be collecting using the following logic: if 1) the flag has been set in the database (remember to clear the flag) OR 2) it has been at least 30 minutes since the last time data was collected. Although, the delphi app would be doing a call to the web app every minute it wouldn't perform the data collecting/updating process unless someone requested an update or the data is 30 minutes old. The call it makes to make that check would be minor and shouldn't have any performance/resource issues.
-
No. That is simply declaring the variable that the code later uses to populate with the HTML "output" code for displaying the monster data (if one was selected). Are you getting any errors? You state "it doesnt give me any information about the selected monster..." so I assume that the select list is getting populated (you did leave the code to dynamically populate the select list, right?). There is error handling in case the selected id is not int he database (i.e. the message "Monster not found." should display). Are you getting that message? Are you getting the display table but no data, what? More info please.
-
Ah ok, I had already created a solution for my first interpretation. I really can't think of a solution other than to create a loop. Let me see what I can come up with.
-
So, basically, you need to know how many bits in the value are true, correct?
-
Select results from 2 tables in different databases on same server?
Psycho replied to localtechguy's topic in MySQL Help
Take a look at this link: http://www.dottedidesign.com/node/14 I have not read it entirely, but seems to be what you want to accomplish -
You are right to be concerned. If the script is running on your server it could, potentially, be accessing any files for your site (e.g. the database connection info) as well as accessing the database and then doing who knows what with that data. I would take a two-pronged approach: 1) Ask the author about it. Ask what the purpose of the call is and some details about what is being passed. If the author is legit he/she should be willing to give you that information in a manner that at least makes sense. If the author is malicious chances are they are going to give you a lot of garbage information that "just doesn't seem right". Of course the person could just be terrible at explaining things or just doesn't want to explain them 2) Search the internet for any information regarding the script. Chances are someone has unencrypted the code and knows what it does. If there was malicious code in there I would bet there is information about it somewhere on the net. AFAIK, you can't really "encrypt" the scripts because the PHP interpreted has to understand it. What they are using is obfuscation in converting the code to something that is not readable by humans. This is also done by people wanting to "protect" their JavaScript code. This works for the majority of users looking at the source code, but anyone who really wants to convert the code back to a readable format (and has some basic knowledge) should be able to do so.
-
Yes firewalls exist on servers, but unless you have control over the server you can't modify the firewall (or host) settings. If you do, then you need to find out what port, protocol and location the script is making to determine what you would need to configure in your firewall. But, I suspect that this was the author's attempt at copy protection. So, the "call" is probably sending a piece of information to the author's site (e.g. a confirmation number tied to your purchase) then sending back a response so the script knows whether or not to perform the functions of the script. If there is no corresponding confirmation number in the author's database the script will not work. There might also be some handling to validate that the script isn't being run from multiple servers. So blocking/redirecting the call will likely break the script. So, what can you do? That's tricky and depends on many legal issues and the country you reside. Because PHP is built on Open source technology I think that (for most countries) content authors are limited in what they can demand in the Terms of Use. For example, I don't know if he can prevent you, in the TOS, from unencrypting the code. Even if you are within your right to unencrypt the code, you may or may not be entitled to modify that code. Again, this will be determined by the TOS and what is allowed. Just because someone states something in the TOS - it doesn't mean it is valid. So, you should start by reading the TOS then determine what it allows you to do. If there is something the TOS prevents you from doing that you want to do,then you can research whether that clause in the TOS is valid or not. This isn't so much an issue of what you can technically do as much as it is what you can legally do.
-
No, as the182guy stated, you cannot unhash a value. The whole point of hashing passwords (vs encrypting them) is that they cannot be unhashed. There are a couple of industry-wide solutions to reset a password (each with a few variations), each has it's benefits and drawbacks 1. Have the site send the user a new password via email, typically after answering a security question. Some sties skip the security question validation with the assumption that only the user would have access to their email. But, it would likely be much easier to break into a user's email than it would their banking site. So, the security question adds additional security. 2. Provide the user the ability to reset their password. This has a few flavors. a) You could have them reset their password directly after answering a security question. Again, this has some security gaps as some security questions are easy to answer. b) you could simply email the user a link to reset their password (as the182guy suggested). This ensures only someone with access to the user's email can do the reset. But this again has a slight security risk. c) Do both, require the user to answer a security question and then email a link to reset their password.
-
You would not create a new PHP file for the invoice. You should store the order information in the database then dynamically create the invoice page using that data. So, you only need one PHP script to display the invoice for any order. I am not going to read through your code above, but the basic implementation would require that you pass the order id to the invoice page. Then use that value to query the database and create the page. Without seeing your exact database structure I can only provide some mock code (and I do not want to see your database structure - I am not going to walk you through the process of creating an order management site via a forum post). But, your database *should* contain at least an "order" table to store the basic info about the order: order id, user, date, shipping costs, etc. Then there should be an associated table of products contained in the order containing the individual product details. Both of those tables should actually have foreign keys to other associative tables (users, product, etc.) but I will leave that out of the example Here is a very rough example script. There would still be a lot to do, but this should give you something to build upon <?php //Get the passed order id $orderID = (isset($_GET['order_id'])) ? intval($_GET['order_id']) : 0; //Create/run query to get the invoice data $query = "SELECT orders.user, orders.date, orders.shipping, order.shipping_address, orders.tax, order_prod.product, order_prod.qty, order_prod.price_per_unit WHERE orders.order_id = {$orderID}"; $result = mysql_query($query); if(!mysql_num_rows($result)) { echo "No order found for order id '{$orderID}'."; } else { //Process the data for generating the invoice $orderUser = false; //Set var as flag to only process common order info once $subtotal = 0; //Var to calculate order subtotal $orderDetails = ''; //Var to stare HTML output of order details while($row = mysql_fetch_assoc($result)) { //Create order specific info from first record if(!$orderUser) { $orderUser = $row['user']; $orderDate = $row['date']; $orderShipCost = $row['shipping']; $orderShipAddr = $row['shipping_address']; $orderTax = $row['tax']; } //Create order details $productTotal = ($row['qty'] * $row['price_per_unit']); $orderDetails .= " <tr>\n"; $orderDetails .= " <td>{$row['product']}</td>\n"; $orderDetails .= " <td>{$row['qty']}</td>\n"; $orderDetails .= " <td>{$row['price_per_unit']}</td>\n"; $orderDetails .= " <td>{$productTotal}</td>\n"; $orderDetails .= " <tr>\n"; //Increase order subtotal $subtotal += $productTotal; } } ?> <html> <head></head> <body> Order date: <?php echo $orderDate; ?> <br><br> Ordered by: <?php echo $orderUser; ?> <br><br> Shipping Address: <?php echo $orderShipAddr; ?> <br><br> Order Details:<br> <table border="1"> <tr> <th>Product name</th> <th>Quantity</th> <th>Per Unit</th> <th>Price</th> </tr> <?php echo $orderDetails; ?> </table> <br><br> Subtotal: <?php echo $orderSubtotal; ?><br> Shipping: <?php echo $orderShipCost; ?><br> Tax: <?php echo $orderTax; ?><br> Total: <?php echo $orderTotal; ?><br> </body> </html>
-
how do I use data in array from a drop down selection list
Psycho replied to futrose's topic in PHP Coding Help
The code I provided will do exactly that. You should validate 1) the data being sent in the POST variable and the query being generated. As for doign multiple UPDATES, you can't do what you are wanting in that manner. The query you are tying to generate has a single WHERE clause and you are trying to update multiple rows with different values. I believe that you are only wanting to update the associated records for a particular user. So, what you really need to do when that page is submitted is to DELETE all the associated records for that user THEN add the new associations in. The alternative it to check which associations exists, delete the individual ones not in the update list and then add the ones in the update list that do not exist in the database. That is a lot more work. The following script should work no matter if you are adding the associations the first time or if you are updating a users associations. I ahve also added debugging lines to the code. Just set $debug to true to see the debug info //Process each value in the array into a complete INSERT value $values = array(); foreach($_POST['eldercatid'] as $eldercatid) { $eldercatid = intval($eldercatid); $values[] = "('$eldercatid', '$id')"; } //Delete any existing records for the elder $deleteSql = "DELETE FROM `eldercategory` WHERE `elderid` = '$id'"; $result = mysql_query($deleteSql) or die mysql_error()); //Insert new/updated values for the elder $insertSql = "INSERT INTO `eldercategory` (`eldercatid`, `elderid`) VALUES " . implode(', ', $values); $result = mysql_query($insertSql) or die mysql_error()); //Set debug to true to see debug info $debug = false; if($debug) { echo "<b>Debug Info:</b><br>\n"; echo "<pre>\n"; echo "Post['eldercatid'] Data\n"; print_r($_POST['eldercatid']); echo "\nDelete Query:\n{$deleteSql}"; echo "\nInsert Query:\n{$insertSql}"; } -
Yes you *could* do this by making the user install an exe on their computer which could send an update to your site when their IP changes, but do you really think users would be willing to install an exe on their computer from your site? I absolutely would not install an exe on my computer just to be able to access the admin pages from a web site/application. Plus, you would need to create the executable and build the PHP backend scripts to receive those updates. Doi you have the knowledge to do that? And, there are still two very big issues: 1) Let's say you did all that and you now have an exe that users have installed on their computers to update your database when their IPs change. What happens when a users want to access the admin site from a different computer? You are going to lock them out? What if they get a new PC and don't know where to get that exe? 2) This is probable the reason it makes all of this pointless. When the exe is run it is going to have to send the user's new IP and some piece (or pieces) of information so you know which user to associate with the IP. Anyone can easily run the exe and capture the information that is being passed. So, it would be fairly trivial for a malicious user to insert any IPs they wanted into your database.
-
how do I use data in array from a drop down selection list
Psycho replied to futrose's topic in PHP Coding Help
OK, first off you do not need a while loop here since you are only getting one record $row = mysqli_fetch_array($result)) { $id = ($row['id']); } You can just use this: $row = mysqli_fetch_array($result); $id = ($row['id']); Ok, now for your specific issue. You will need to use the "other" format for INSERT queries and "build up" a single insert query to create all the records. Remove this: $eldercatid = mysqli_real_escape_string($link, $_POST['eldercatid']); You don't use mysqli_real_escape_string() on values that should be numeric values. Instead you need to validate the value as a number and/or int value! Replace this: $sql = "Insert into eldercategory set eldercatid = '$eldercatid', elderid = '$id'"; With this: //Process each value in the array into a complete INSERT value $values = array(); foreach($_POST['eldercatid'] as $eldercatid) { $eldercatid = intval($eldercatid); $values[] = "('$eldercatid', '$id')"; } //Create the SQL statemetn using implode on the values $sql = "Insert into eldercategory (`eldercatid`, `elderid`) VALUES " . implode(', ', $values); -
OK, as I said build the page with just mock content as a hard coded HTML file. Once you get the CSS and layout the way you want, then create the PHP code to generate the same way. So, at this point I suggest giving it a go with the hard coded page. Then if you can't get it the way you want post the code you have here and ask for help.
-
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=340008.0