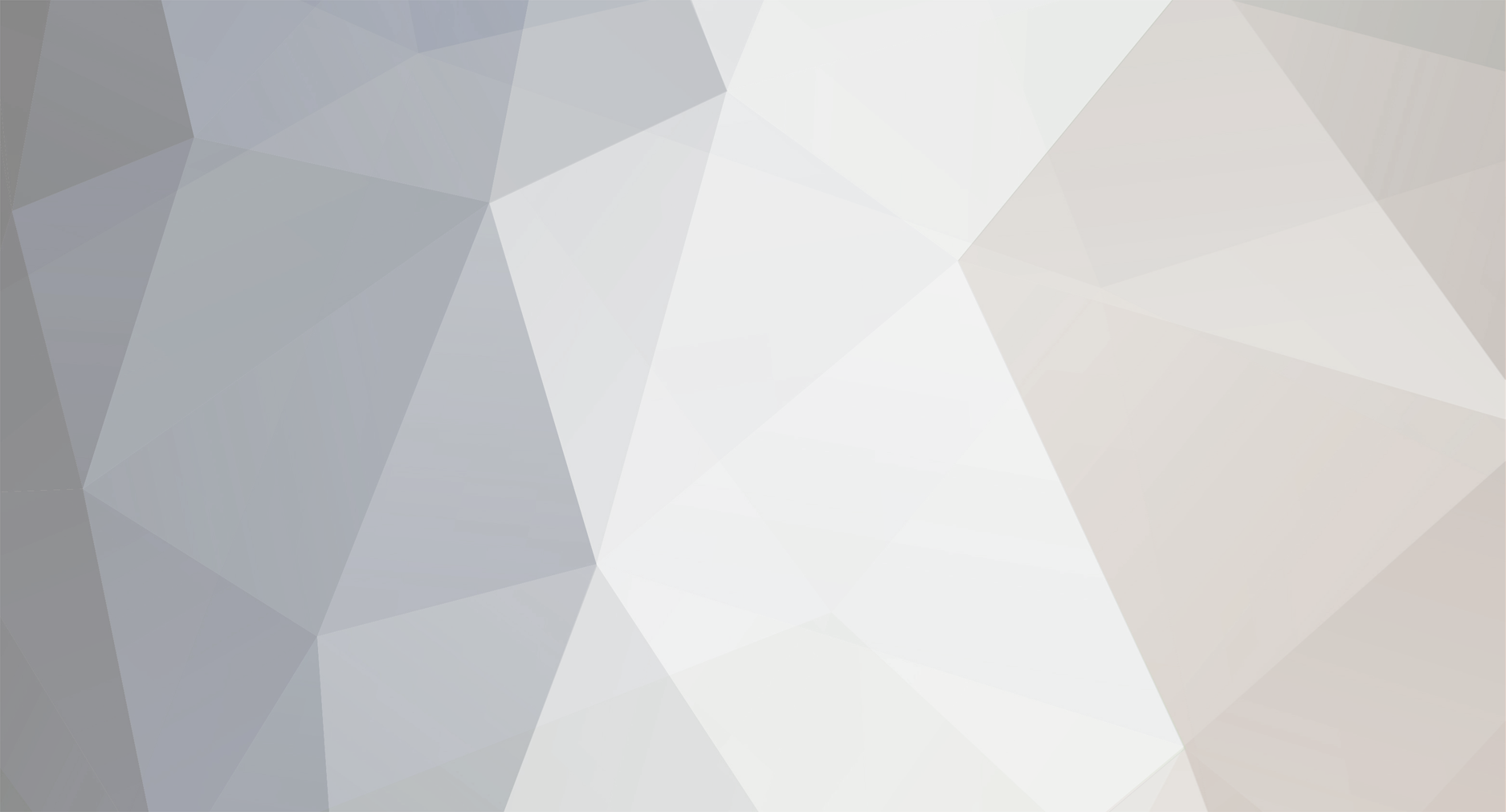
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
The code above is just a function to update the record. You need to look at the code that calls the function and what it does afterward There was a typo in the code I provided, but you also left out the code that would actually update the record. Try this <?php function editUser($userUsername, $userPassword, $userEmail, $userRealname, $id) { //Escape input for DB queries $userUsername = mysql_real_escpae_string(trim($userUsername)); $userPassword = mysql_real_escpae_string(trim($userPassword)); $userEmail = mysql_real_escpae_string(trim($userEmail)); $userRealname = mysql_real_escpae_string(trim($userRealname)); $id = (int) $id; //See if there is ANOTHER user with same username $query = "SELECT * FROM authorized_users WHERE username = '$userUsername' AND id <> '$id'"; $result = mysql_query($query) or die(mysql_error()) ; if (mysql_num_rows($result) > 0) { /* Username already exists */ echo "<p><p><span class='current_admin'>Username already exists</span>"; echo '<p>Please <a href="javascript:history.go(-1)">Go Back</a> and complete the form<br>'; echo '<META HTTP-EQUIV="Refresh" CONTENT="8; URL=users_authorized.php">'; ## START DEBUGGING CODE echo "Query: $query<br>\n"; echo "Results: <pre>"; while($row = mysql_fetch_assoc($query)) { print_r($row); } echo "<pre>\n"; ## END DEBUGGING CODE } //There is not another user with same username (or user didn't select to change username) $query = "UPDATE authorized_users SET username = '$userUsername' password = '$userPassword' email = '$userEmail' realname = '$userRealname' WHERE id = $id"; $result = mysql_query($query) or die(mysql_error()); if(mysql_affected_rows()==0) { //No matching record found echo "The user does not exist"; } else { echo "The record was successfully updated"; } } ?>
-
$tmp[] = "<a href='../profile.php?action=view&username=" . $find_members_online_ro["username"] . "'>$user</a>"; You should really urlencode() the username within the url parameter (and, of course, use urldecode) in the page that processes that value.
-
Using the code I posted previously, I would change the following section as shown //Output results echo "<strong>{$memberCount} members</strong> are online<br><br>\n"; $lastRecord = array_pop($memberAry); echo implode (", ", $memberAry) . "and {$lastRecord}";
-
You know there is a manual for php, right? Having us explain a function to you which has clear documentation with examples and user comments is a useless activity. Now, if you were to have read the manual and still have questions, then by allmeans ask. http://us2.php.net/manual/en/function.implode.php Try this code <?php //Create and run query $query = "SELECT * FROM fans WHERE status = 'Online'"; $result = mysql_query($find_members_online_q); //Process results $memberAry = array(); $baseURL = "../profile.php?action=view&username="; $memberCount = mysql_num_rows($result); while ($row = mysql_fetch_array($result)) { $style = ($row['forum_rank'] == 'Site Owner') ? " style='color: #FF0000'" :'' ; $urlUname = urlencode($row['username']); $memberAry[] = "<a href='{$baseURL}{$urlUname}'{$style}>{$row['username']}</a>"; } //Output results echo "<strong>{$memberCount} members</strong> are online<br><br>\n"; echo implode (", ", $memberAry); ?>
-
Preserving double quotes in an uploaded text file
Psycho replied to Marchand's topic in PHP Coding Help
Yeah, there are more differences than just the double quotes. The leading zero on the date is dropped and the times are converted from AM/PM to military time. There is absolutely no way that the move_uploaded_file() function is doing this. There must be some other processing code that is making the changes. -
Or just view the output in the HTML source code (i.e. right-click View Page Source).
-
It makes it hard to analyze the array when you put it all in one line like that. Could you do a print_r() and post the results. Also, either get the output directly in the HTML source (which will have the line breaks) or put the output inside PRE tags so it will display with line-breaks in the output.
-
mysql resetting password for user and script improvement
Psycho replied to Himself12794's topic in PHP Coding Help
Ah yes, it takes an options parameter for the resource link (i.e. the db connection variable) not the query identifier. -
Your problem is with all the curly braces around the table names - that is invalid code. The only reason to use curly braces in that context would be if you were enclosing variables to be replaced by PHP when parsing the string. But, if that was the case, then the variables would be preceded with a dollar sign. If those are variables, then include the dollar sign. If those are the actual table names then remove the curly braces.
-
getting weird syntax error when INSERTing query to table
Psycho replied to WhiteRau's topic in PHP Coding Help
I'll also add that for debugging purposes it is always helpful to echo the actual query to the page when it fails. It makes finding/fixing mistakes much easier. For example, sometimes a query will fail because a value is not set which you believe is (maybe there was a typo in the variable name or the value just wasn't set). By echoing the query to the page the error will sometimes be very obvious and allows you to know exactly where to look to fix it. However, you should always provide "friendly" error messages in a production environment that do not give the users DB errors that provide details about the nature of the error. Personally, I typically use a custom function for running my queries that checks to see if the application is in "debug mode". If yes, I will show the actual DB error and query. If not, I provide a friendly error message to the user. -
Sorry, but your code is just way too overcomplicated and too long for me to try and analyze. You need to add some debugging code to validate the values that you expect at each step of the process. For example, if you modify an array, do a print_r() before and after you modify it to ensure it is being modified as you expect. But, as I alluded to previously, you need to know what you are doing and, more importantly, why. By including comments you can provide clarification as the logic flow in your code. Regarding the line you took out, what was the purpose of the line? You obviously thought you needed it for some reason. Then you take it completely out because of my comments.
-
mysql resetting password for user and script improvement
Psycho replied to Himself12794's topic in PHP Coding Help
1. You have two variable: $errmsg_arr & $errflag. In the validation, if an error is found you add an error description to $errmsg_arr and set $errflag to true. Then at the end you use $errflag to determine if errors occurred. $errflag is completely unnecessary. Just use count$errmsg_arr) to determine if any errors occurred. You can then get rid of the line that creates $errflag and all the lines that set it to true. 2. you are running the first query using $login & $email THEN you are validating the values? You should validate that the values are valid before running the query. 3. What is the purpose of the first query, anyway? 4. Don't do separate queries to check login and email. Do just one query searching for a record matching both values. That provides better security. If someone stumbles upon one right value they could continue to try alternates for the second value. 5. You have many, many queries and you only need one. I could go on, but it would just be easier to show you how I might do it <?php //Start session session_start(); //Include database connection details require_once('config.php'); //Connect to mysql server if(!mysql_connect(DB_HOST, DB_USER, DB_PASSWORD)) { die('Failed to connect to server: ' . mysql_error()); } //Select database if(!mysql_select_db(DB_DATABASE)) { die('Unable to select database: ' . mysql_error()); } //Function to sanitize values received from the form. Prevents SQL injection function clean($str) { if(get_magic_quotes_gpc()) { $str = stripslashes($str); } return mysql_real_escape_string(trim($str)); } //Array to store errors $errmsg_arr = array(); //Get form values and clean them $login = clean($_POST['login']); $email = clean($_POST['email']); $newpassword = mt_rand().mt_rand(); //Input Validations if(empty($login)) { $errmsg_arr[] = 'Login ID missing'; } if(empty($email)) { $errmsg_arr[] = 'Email missing'; } //Attempt to set new password value (only run if no previous errors) if(count($errmsg_arr)==0) { $pwHash = md5($newpassword); $qry = "UPDATE members SET passwd='$pwHash' WHERE login='$login' AND email='$email'"; $result = mysql_query($qry); if(!$result) { die("Error running query: " . mysql_error()); } //If there were no affected rows then there was not matching value if(mysql_affected_rows($result)==0) { $errmsg_arr[] = 'That Login ID and/or Email do not exsist. Are you trying to register?'; } else { //Password was uipdated, send new password email. $to = $email; $subject = "New Password"; $message = "New password.\r\r You, or someone using your email address, has requested a new password.\r\r Login: $login\r\r New Password: $newpassword\r\r Regards, Me"; $headers = "From: [email protected]\r\n" . "Reply-To: noreply@ mywebsite.com\r\n" . "X-Mailer: PHP/" . phpversion(); if(!mail($to, $subject, $message, $headers)) { $errmsg_arr[] = 'There was a problem sending the email'; } } } //If there are error, redirect back to the login form if(count($errmsg_arr)>0) { $_SESSION['ERRMSG_ARR'] = $errmsg_arr; session_write_close(); header("location: reset.php"); exit(); } //There were no errors header("location: reset-success.php"); ?> -
Storing product page view settings for specific category
Psycho replied to HDFilmMaker2112's topic in PHP Coding Help
If you want the options to be remembered you will need to store them somewhere. The most logical options would be in cookies or in session data. You can use cookies to have the options persists across sessions or use the session variable to just remember them for the session. You can then use the cookie/session value to implement the appropriate option on each page load. It is an easier solution since you don't have to modify all the links on your pages. So, for the first part to remember the options. When the user selects an option you will detect in on the next page load. Just store that option in the session/cookie as a sub-element of a category element. So, in your example, if the user selected "category "A", and choose Sort By: Name and Products per Page: 24", then you would store the options like this: if(isset($_GET['num_products'])){ $num_products_per_page=$_GET['num_products']; $cookie[$cat]['num_products_per_page'] = $num_products_per_page; //..... if(isset($_GET['sort_by'])){ $sort_by_selected=$_GET['sort_by']; $cookie[$cat]['sort_by_selected'] = $sort_by_selected; Now, if you really want to include the options int eh URLs then you will need to add some logic anywhere that you provide a link to another page where you want the options "remembered". I would suggest creating a function to create the URLs. For example, you create a function that takes the base URL and the category. The function then uses the category to see if there are remembered options for that category and append them to the base URL. -
If you have done some debugging, please share what your findings have been so we don't have to ask about things that you have already ruled out. Go ahead and remove those single quote marks as they are not correct. If you are getting an error it is likely that error is telling you what is wrong. please share what it is.
-
There are problems in your script outside of your reported issue: 1. You first check if the user has credentials saved in a cookie and try to authenticate using that. If it fails you send them back to the login page. It is only if they don't have the cookie set that you check if credentials were POSTed. That means if their cookie becomes corrupted the user would be caught in an infinite loop since you would never check their POSTed values. Typically you would always check POSTed values first since they are the most recent. 2. You don't need two sections to check credentials anyway (cookie vs. POST). Jsut determine which credentials to use and hame only ONE query. Otherwise if you need to make a change later you have to remember to change both queries. 3. Why do you have a while() loop when you should only have 1 record returned from the quire and why do you have an if/else statemetn with nothing in the if code block? Just reverse the logic in the if condition to test for failure and don't have an else. 4. This is incorrect. An OR condition is TWO pipes - || if(!$_POST['username'] | !$_POST['pass']) 5. You have a line to addslashes to the email value, but then don't do anything with it. I could go on, but there is a lot wrong here. You need to step back. Plan out th elogic and then build each piece one step at a time. Test it, fix it, then move on.
-
Assuming that you need to get go through all the values in the array multiple times but you want to go through each value before starting over, this will work for you: $arr = array('One', 'Two', 'Three', 'Four', 'Five'); $tmpArray = array(); for($i=0; $i<15; $i++) { if(count($tmpArray)<1) { $tmpArray = $arr; shuffle($tmpArray); } $value = array_shift($tmpArray); echo "{$value}<br />\n"; } Sample output: Five Three Four Two One Three Four Two Five One Five One Three Four Two
-
GuiltyGear's solution is by far the simplest and most efficient.But, I have to ask... In your example you have an array of 5 values and then run a loop 1,000 times to get a random value out of that array. Is that a true example where you need more values than exist in the array. In other words, do you need to be be able to run through (randomly) every value in the array completely and then start over again until you reach some specific number or will you only need each value one time.
-
If it is not working, then debug your code. Just determine the decision points in your logic and echo out the pertinent data to see if what you expect to happen is happening. I was working on adding some debugging code to get you started when I found the problem. Your query to get the data for the page has single quote marks around the $order variable: $sql2 = mysql_query("SELECT * FROM orphans ORDER BY '$order' $limit") or die(mysql_error()); You only put quotes around values in a query. User this $sql2 = mysql_query("SELECT * FROM orphans ORDER BY $order $limit") or die(mysql_error());
-
OK, following your original process I rewrote this to do the exact same thing, but more efficiently. Although it is a lot more code it should run faster due to reducing the queries being done. Right now your script runs an individual query for each and every field of each record. The below script does the following: 1. Gets a list of all the current fields in the table, then while processing the data generates a list of new fieldds to be added to the table. Then ONE query is run to add the fields. 2. Creates an array of all complete records to be added/updated 3. Splits the array into two arrays: records to be added and records to be updated 4. Processes the records to be added array into ONE single query to add all the records 5. Processes the records to be updated into one complete query per record (not per field). With that there are a total of 4 main queries plus one query each for each record update. That is probably less than 10% of the queries you have now. Also, #5 can be further updated into a single query to update all the records in one query. It takes a MySQL function similar to a switch statement in PHP. But, I forget the structure and I'm too lazy to look it up. That would mean only 5 total queries for the entire file. I wrote all this code on-the-fly, so I'm positive there are some syntax errors and I probably have a logic problem or two. I would suggest commenting out the actual mysql_query() calls, add some debugging code to display the queries and other pertinent data, then run it against a small file to validate the results. <?php if($flag == true) { $con = mysql_connect('localhost', 'root', '') or die('cannot connect'); $db = mysql_select_db('translate') or die('cannot select DB'); $table = 'nursinghomes'; //Get list of existing columns from table $query = "SHOW COLUMNS FROM {$table}"; $result = mysql_query($query) or die(mysql_error()); $existingColumns = array(); while($row = mysql_fetch_assoc($result)) { $existingColumns[] = $row['Field']; } //Variables to hold processed data $newColumnsSQL = array(); $data = array(); //Process the file into an array if ($file=fopen($fileloc,"r+")) { while($linearray = fgetcsv($file, 1000, ",") !== FALSE) { $id = $linearray[0]; $formcolumn = $linearray[1] . "_" . $linearray[2] . "_" . $linearray[3]; $content = $linearray[4]; //Detemine if field exists in table (or has already been added) if(!in_array($formcolumn, $existingColumns)) { $existingColumns[] = $formcolumn; $newColumnsSQL[] = "ADD COLUMN {$formcolumn} CHAR(100)"; } //Add record to data variable $data[(int)$id][$formcolumn] = mysql_real_escape_string($content); } fclose($file); } //Finish processing file, now run queries //Run ONE query to add all new columns if(count($newColumnsSQL) > 0) { $query = "ALTER TABLE $table " . implode(', ', $newColumnsSQL); $result = mysql_query($query) or die(mysql_error()); } //Run query to find all existing records from the processed data $query = "SELECT id FROM {$table} WHERE id IN (" . implode(',', array_keys($data)) . ")"; $result = mysql_query($query) or die(mysql_error()); //Dump results into array $existingIDs = array(); while($row = mysql_fetch_assoc($result)) { $existingIDs[$row['id']] = false; } //Split data array into two arrays (one for new records and one for existing records) $newData = array_diff_key($data, $existingIDs); $existingData = array_diff_key($data, $newData); //Create array of all fields (except ID field) with empty value $columnList = array_fill_keys($existingColumns, ''); //Process insert data $valuesSQL = array(); foreach($newData as $id => $recordData) { //Ensure data is mapped for all fields in correct order $insertData = array_merge($columnList, $recordData); foreach($recordData as $fieldName => $fieldValue) { $valuesSQL[] = "('" . implode("', '", $insertData) . "')"; } } //Create ONE insert query for all new records $query = "INSERT INTO $table (`" . implode('`, `', array_keys($columnList)) . "`) VALUES " . implode(', ', $valuesSQL); $result = mysql_query($query) or die(mysql_error()); //Process update data foreach($existingData as $id => $recordData) { $setValuesSQL = array(); foreach($recordData as $fieldName => $fieldValue) { $setValuesSQL[] = "`{$fieldName}` = '{$fieldValue}'" } $query = "UPDATE {$table} SET " . implode(', ', $setValuesSQL); $result = mysql_query($query) or die(mysql_error()); } echo "Your file has been translated. Click <a href=\"?p=another.php\">here</a> to export the file."; } ?>
-
Never EVER run queries in loops. Instead, in your processing code determine all the DB queries you will need to make and then combine them (as applicable). For example, you could run one query to add multiple columns and you can run one query to add multiple records (both of which will be much more efficient). You can also run one query to update multiple records with different values for each - but it is not strait-forward and I'm not sure how efficient it will be. I'll review the code and give you something to work with.
-
I haven't looked through all your code - especially since it is >600 lines any you didn't bother to at least identify the lines that would have some effect on the problem. But, here is at least one problem: if(isset($_SESSION['cushArray'])){ $_SESSION['cushArray'] = array_values($_SESSION['cushArray']); } What exactly are you trying to do here? That code is basically saying if the session value exists reset it's value with the same values??? Anyway, if you were to check the manual for array_values() you would see this: So, you are replacing the array with named indexes with numerically based indexes. That explains the values being associated with numerically based indexes on the first level. Not sure why you have the same values duplicated in two sub array, but I'm guessing both of those are from the same defect. I tried to see if there were any other apparent problems, but I see no flow/structure to your code. And - ARE YOU KIDDING ME - not one single comment?! Comments should be considered a neccessity of coding.
-
What do you mean by "in any of top 6 values in $array1"? Do you mean: $array1['articles'][0], $array1['articles'][1] . . . $array1['articles'][5]? If so, then //Create a random index 0-5 (or max of those avail in array1) $randIdx = rand(0, max(count($array1['articles']), 5)); $array1['articles'][$randIdx] = $array2['articles'][0];
-
I don't see any reason why the code I provided would NOT remove duplicates values, so my *guess* would be that those two records which both show "8 Eyes" are not really duplicates. For example, one may have additional spaces before, after or even between words. When rendered in HTML they will look exactly the same. Or, perhaps a character is displayed using a character code in one value and not the other. Again, they would look the same on the page, but would actually be different. Or, there could be HTML tags within the value which also wouldn't be displayed. You could inspect the HTML source code, but that can be tedious if you have a lot of records to check. One easy way to check if the values are not EXACTLY the same would be to convert everything to HTML entities when displaying it. So, the output on the screed should display what is exactly in the DB, but the actual HTML source would be different. Also, on the initial query you should probably do an ORDER BY ont he name field to help make looking for duplicates easier. 1. Change first query to this $query = "SELECT r_id, r_name FROM roms WHERE r_console = 'NES' ORDER BY r_name"; 2. Change the following two blocks of code that are used to display the data while($row = mysql_fetch_assoc($result)) { if(!in_array($row['r_name'], $saveAry)) { $saveAry[$row['r_id']] = $row['r_name']; $saveHTML .= "<tr><td>{$row['r_id']}</td><td>{$row['r_name']}</td><td><pre>[".htmlenteties($row['r_name'])."]</pre></td></tr>\n"; } else { $deleteAry[$row['r_id']] = $row['r_name']; $deleteHTML .= "<tr><td>{$row['r_id']}</td><td>{$row['r_name']}</td><td><pre>[".htmlenteties($row['r_name'])."]</pre></td></tr>\n"; } } echo "<b>Delete Query:</b><br>$query<br>\n"; echo "<table>\n"; echo "<tr><th colspan=\"3\">SAVED RECORDS</th></tr>\n"; echo "<tr><th>ID</th><th>DB Value</th><th>Escaped Value</th></tr>\n"; echo $saveHTML; echo "<tr><th colspan=\"3\">DELETED RECORDS</th></tr>\n"; echo "<tr><th>ID</th><th>DB Value</th><th>Escaped Value</th></tr>\n"; echo $deleteHTML; echo "</table>\n"; Now, rerun the script and review the results. If you see two similar values in the 2nd column check to see if the values are the same in the third column. If you find that there are two values that are visually identical (but not really identical), Just pick the ID of the one you want removed and run a query DELETE FROM roms WHERE r_id = [THE_ID_YOU_WANT_TO_DELETE]
-
I'm not going to go through all of your code and fix it since I don't have your db to test against and I'm not willing to invest the time. But, I'll provide some suggestions. First off - not related to your question: [code=php:0]$order=$_POST["order"]; if(isset($_POST["order"]) and strlen($order)>0){ $order=$_POST["order"]; } That if() statement serves no purpose. The previous line you already defined $order from the POST value, so if the if condition is true you only redefine it! Anyway: 1. You do not need to use ORDER BY in the first query since it is only used to get the total record count $sql = mysql_query("SELECT * FROM orphans ORDER BY '$order'") or die (mysql_error()); 2. I'm surprised your query isn't failing on the 1st page load. Since you did not submit a sort option your query would be invalid $sql2 = mysql_query("SELECT * FROM orphans ORDER BY '$order' $limit") or die(mysql_error()); When $order is not defined that query would look something like [coed]SELECT * FROM orphans ORDER BY LIMIT 10[/code] 3. OK, the main problem I see is that you are using a form to submit the ORDER BY value through POST data. That's not a problem in itself, and it should work - but only on the first page! The problem is that once you select a new page, you reload the page and there is no POST value to define the ORDER BY value. So, when the user submits an ORDER BY via the POST form, you need to store that value to use on subsequent page loads. You can do this one of three ways: 1: Append to the URL of the page links, 2: store in a cookie, 3: store in a session value (my preference). Then on subsequent page loads you would first check if the ORDER BY was sent in POST data (if so use it). If not, then you have to check the secondary value from the option you chose from the three above. If not set there, then use the default. Here is a sample of how you might do that last part: if(isset($_POST["order"])) { $order = mysql_real_esacpe_string($_POST["order"])); $_SESSION['order'] = $order; } elseif(isset($_SESSION['order'])) { $order = $_SESSION['order']; } else { $order = 'defaultField ASC'; //change as needed }