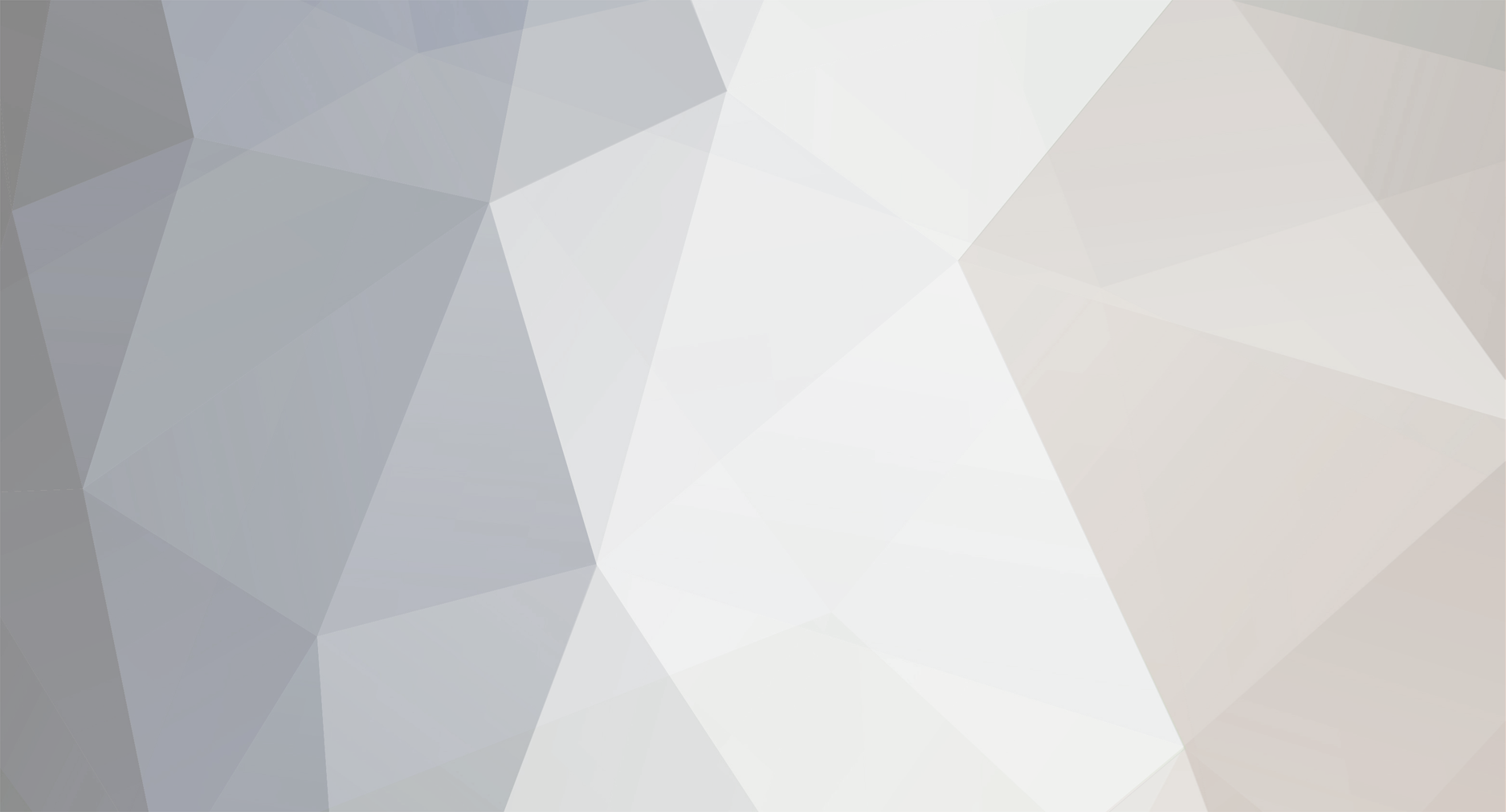
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Use a single set of code along with an array of all the field names. If you add/remove fields later, you only need to modify the array. if (isset($_POST['hidden']) && $_POST['hidden'] == "edit") { //List of input/db fields $fieldList = array('cimage', 'ctext', 'cextra', 'atext', 'aextra', 'aimage'); //Create SET statements for query $setParts = array(); foreach($fieldList as $fieldName) { if(isset($_POST[$fieldName)])) { $value = mysql_prep(trim($_POST[$fieldName)])); $setParts[] = "`{$fieldName)}` = '{$value}'"; } } //If at least one field was passed generate and run query if(count($setParts)>0) { $sql = "UPDATE cluesanswers SET " . implode(', ', $setParts); $result = mysql_query($sql, $connection); if (!$result) { die("Database query failed: " . mysql_error()); } echo "Record updated."; } else { echo "No fields were passed."; } }
-
I'm working on a freshly installed machine and don't have a test server set up yet, so none of this is tested (especially the regex = I think the single quote marks [all three] may need to be escaped with a forward-slash before each one): //Read master category list into array $categoryFile = "path/to/file/filename.txt"; $categoryList = array(); foreach(file($categoryFile) as $line) { preg_match("#(\d+)='([^']*)'#", $line, $match); $categoryList[$match[1]] = str_replace("-", " ", $match[2]); } //Process the current categories $categories = '1,5,23,46'; //Category IDs //Convert to array AND apply trim $categoryIDsAry = array_map('trim', explode("," , $categories)); //Create the HTML ouput $catsHTMLAry = array(); foreach ($categoryIDsAry as $catID) { $catSlug = $categoryList[$catID]; $catName = str_replace("-", " ", $catSlug); $catsHTMLAry[] = "<a href=\"index.php?category=$catSlug\">".strtoupper($catName)."</a>, "; } $catsHTMLStr = implode(', ' $catsAry);
-
Change the JavaScript code to create the date input names so they will be interpreted as a sub-array in the POST data. Create ALL the date input fields with the same name followed by brackets. Example <input type="text" name="dates[]" /> Then in your PHP code you can loop through all of the date inputs as follows: foreach($_POST['dates'] as $dateValue) { //Do something with $dateValue }
-
If you are using PHP version 5.2 or above you can use filter_var(): http://www.php.net/manual/en/function.filter-var.php. If you are on an older server (or want backwards compatibility) you can use a function. I have provided one that I have used below. In your current logic, you would just do an elseif() right after the check to see if email was even entered if( empty($_POST['email'])) { header("Location:Messages.php?msg=12"); exit(); } elseif(!is_email($_POST['email'])) { header("Location:Messages.php?msg=13"); exit(); } Also, you can avoid a lot of duplication in your code with some minor modifications. Instead of doing a header() and exit() for each error condition, just call a function to do that. Otherwise, in the future if you decide on a different process for error handling you have a lot of copying/pasting to do which will lead to errors. Example: function error_redirect($error_code) { header("Location:Messages.php?msg={$error_code}"); exit(); } if(isset($_POST['Submit'])){ //NEED TO CHECK IF FIELDS ARE FILLED IN if( empty($_POST['email'])){ error_redirect(12); } elseif(!is_email($_POST['email'])){ error_redirect(13); } if( empty($_POST['name'])){ error_redirect(3); } if( empty($_POST['pw1']) && (empty($_POST['pw2']))){ error_redirect(2); } //etc... My is_email() function with full documentation // NOTES: // // Format test // - Username: // - Can contain the following characters: // - Uppercase and lowercase English letters (a-z, A-Z) // - Digits 0 to 9 // - Characters _ ! # $ % & ' * + - / = ? ^ ` { | } ~ // - May contain '.' (periods), but cannot begin or end with a period // and they may not appear in succession (i.e. 2 or more in a row) // - Must be between 1 and 64 characters // - Domain: // - Can contain the following characters: 'a-z', 'A-Z', '0-9', '-' (hyphen), and '.' (period). // - There may be subdomains, separated by a period (.), but the combined domain may not // begin with a period and they not appear in succession (i.e. 2 or more in a row) // - Domain/Subdomain name parts may not begin or end with a hyphen // - Domain/Subdomain name parts must be between 1-64 characters // - TLD accepts: 'a-z' & 'A-Z' (2 to 6 characters) // // Note: the domain and tld parts must be between 4 and 255 characters total // // Length test // - Username: 1 to 64 characters // - Domain: 4 to 255 character //===================================================== // Function: is_email ( string $email ) // // Description: Finds whether the given string variable // is a properly formatted email. // // Parameters: $email the string being evaluated // // Return Values: Returns TRUE if $email is valid email // format, FALSE otherwise. //===================================================== function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); }
-
Without knowing how you are tracking memory usage and at what time there are any number of reasons why you are getting the results you have stated. Besides, memory usage is a tricky thing. Also, just because a function/script ends doesn't mean the memory is instantaneously released. Take a look at this post with some relevant info: http://stackoverflow.com/questions/1145775/why-does-this-simple-php-script-leak-memory
-
Ok, looking at your code there are a heck of a lot more variables than what you state above. Just because your class is returning x number variables doesn't mean that more variables were created (and stored in memory). Case in point: protected function getNormal() { $a0=$this->v[2]->x-$this->v[0]->x; $a1=$this->v[2]->y-$this->v[0]->y; $a2=$this->v[2]->z-$this->v[0]->z; $b0=$this->v[1]->x-$this->v[0]->x; $b1=$this->v[1]->y-$this->v[0]->y; $b2=$this->v[1]->z-$this->v[0]->z; $x=$a1*$b2-$a2*$b1; $y=$a2*$b0-$a0*$b2; $z=$a0*$b1-$a1*$b0; $l=sqrt($x*$x+$y*$y+$z*$z); $x/=$l; $y/=$l; $z/=$l; return array($x,$y,$z); } That function returns three variables, but there are ten total variables generated within that function ($a1, $a2, $a3, etc.). I also suspect that some memory is used as overhead in performing the mathematical operations that isn't necessarily freed up immediately.
-
By the way, you can put an If/Else within the query itself. I don't even see what the purpose is of the first query in the code you provided. // connect to the database include('connect-db.php'); // get id value $id = $_GET['id']; echo "$id"; $publish = $_GET['publish']; echo "$publish"; $sqltwo = "UPDATE events SET publish = IF('$publish'='Yes', 'No', 'Yes') WHERE ID='$id'"; $result=mysql_query($sql); Also, I would advise using the values "Yes"/"No". They mean nothing programatically as they are just strings. For boolean values you should be using 1 and 0. Otherwise you are always having to convert the strings too true/false.
-
Your IF condition is using an assignment (single equal sign) not a comparison (double equal). Since it is successfully able to assign the value of 'Yes' to the $publish variable it is always true.
-
Without knowing HOW it is coded this is just a hypothetical discussion. I can write two scripts that produce the exact same results but one uses exponentially more memory. You say you are using a class, right? So just initiating the class will take memory. I have no idea how you are running this or what output is produced. Are you running the client and the server on the same machine? This is the PHP Help forum where people post requests asking for help with their code. You have not provided code nor asked for help. If you are not going to post code, then this should be moved to the "Application Design" forum which includes "Programming theory, database design, performance, etc."
-
You will need to have a web server running on your machine and then have an html link to the file relative to the web server path. So, if the file is in the root of the htdocs, then the URL would look something like http://localhost/index.php. Of course, you can probably leave off the file name since index.php will more than likely be in the list of default file names. By linking directly to the file you are treating it as a file on your PC and Windows is trying to open the file. To get the rendered page of a PHP file you need to request the file through a web server. The webserver will then find the file and process the PHP code before sending the rendered output to the browser.
-
echo repopulating blank data in database on refresh. please help
Psycho replied to bremen1984's topic in PHP Coding Help
No offense, but that IF/THEN statement serves no purpose. If the result is empty then you will get the same results if you used the first echo. Since $result['description'] is a field returned from the DB query it will be defined, but it may not have a value. Typically, you would do something as you showed if the variable may not be set - to prevent errors. -
If there is a one-to-one correlation between shops and addresses, why are you storing the addresses in a separate table? You could store it int he shops table and make your life simpler. Or, if you want the data separated, then you should have a foreign key in the addresses table pointing back to the shop record (since the shop is the parent) - not hte other way around as you have it. Anyway, as GuiltyGear stated, using the structure you already have you can simplify it using a subquery. here is the full version: $query = "UPDATE addresses SET AddressLine1='$AddressLine1', AddressLine2='$AddressLine2', City='$City', Postcode='$Postcode', State='$State' WHERE AddressID = (SELECT DisplayAddressID FROM shops WHERE ShopID='$shopid')"; mysql_query($query);
-
echo repopulating blank data in database on refresh. please help
Psycho replied to bremen1984's topic in PHP Coding Help
What help do you need? You apparently didn't take the time to even really read the initial response I gave you because you completely disregarded the problem I raised. But, I also showed you how you would echo contents inside a textarea. What about the sample code I provided do you not understand? -
There is an awful lot going on in that code. I started reading it line-by-line to understand what was going on but, to be honest, it is very confusing. Not only are there no comments, but many of the variable names have no meaning (e.g. $two_one). Have have a very strong feeling that you are doing much more work and making this more complicated than it should be. For example, I see where you do a query to get an id, then do a subsequent query using that ID to pull records from another table. You should instead be using a JOIN query. I think it might be more advantageous to explain what it is you are trying to accomplish along with a description of your tables structures.
-
Although, depending on what you need to do on those pages, you could create a PHP page to grab the contents of those pages and build one page with a UI to select the pages to view. Or, another option would be to use frames. Have one frame across the top or left with the list of 150 links and those links are set to change the content of the main frame. I would never propose frames for an actual user facing function, but it would probably work well in this specific case.
-
OK, well, you are still using the wrong variable name of "$total_pages" which actually represents the total_records, but I'll provide some sample code based upon what you have. This uses the variables you defined above: $start, $limit, $total_pages, and $result30 $records_on_page = mysql_num_rows($result30); $start_record = ($start * $limit) + 1; $end_record = $start_record + $records_on_page -1; echo "Showing {$start_record} to {$end_record} out of {$total_pages} products";
-
I'm not understanding the exact problem. Are you saying that the pages where there are less than 20 records are not displaying the records or that the total pages isn't being calculated correctly? Although, based on your variable names I think the problem may be here: $query5 = "SELECT COUNT(*) as num FROM $tbl_name WHERE product_category='$cat'"; $total_pages = mysql_fetch_array(mysql_query($query5)); $total_pages = $total_pages['num']; That would NOT be the total pages, that would be the total records. To get total pages you take the total records divided by the $limit, rounded up. Something like $query = "SELECT COUNT(*) as num FROM $tbl_name WHERE product_category='$cat'"; $result = mysql_query($query); $total_records = mysql_result($result, 0); $total_pages = ceil($total_records/$limit); //Be sure limit is defined before this Also, there is no need to define a bunch of variables such as $query1, $query2, $query3, etc. Once a query is run you can simply redefine the previous variable. I typically use $query and $result. It is very rare that I need to maintain a query or result when I run the second query. If you DO need to maintain those variables, at least give them descriptive names.
-
No need for all that superfluous code. There is a much more elegant and easily maintainable solution. <?php $switchControlsList = array( 'noir' => 'Noir', 'crimson' => 'Crimson', 'forrest' => 'Forrest', 'ocean' => 'Ocean', 'petal' => 'Petal' ); $switchOptions = ''; foreach($switchControlsList as $value => $label) { $selected = (strcmp($value, $_COOKIE['mysheet']) ? ' selected="selected"' : ''; $switchOptions .= "<option value=\"{$value}\"{$selected}>{$label}</option>\n"; } ?> <form id="switchform"> <select name="switchcontrol" size="1" class="topusernav" onChange="chooseStyle(this.options[this.selectedIndex].value, 60)"> <option value="">-----</option> <?php echo $switchOptions; ?> </select> </form> NOTES: 1. Don't use "none" for a "non-selection", just use an empty string - it is a more logical choice 2. No need to have logic on the first option to "select" it since it is supposed to be the default value. if none of the other options are explicitly selected, the first value will be selected by default. 3. The code is built so all you have to do is modify the array of list values. It is built to use the array index as the value and the array value as the select label. But, since you are using the same text for both you could just use array values and not specify the indexes - just use strtolower() when echoing the value for the options. But, I would leave the array in that format as it is more conducive if you are using a database.
-
Checkbox's value is not passing correctly..
Psycho replied to abhi10kumar's topic in PHP Coding Help
Um, you posted this: And that WOULD pass the value of the checkbox - if the checkbox actually had a value! There is no value parameter for that checkbox!!! Looking at the function being called and how that "value" is used I think you meant to pass the checked state of the checkbox - not the value. By the way, why would you pass a variable to a function as str1 only to then to use that value to define another variable "v"? If you want the value in the variable v, then just use that in the function parameters. -
echo repopulating blank data in database on refresh. please help
Psycho replied to bremen1984's topic in PHP Coding Help
Your code makes no sense and I would bet money that there is more to the code than you are showing! You do a DB query and then loop through the results assigning each record to the array variable $result. But, in that loop you are echoing the values from the variable $_POST. So, I am guessing that each time the page loads you also have some code (not shown) that is inserting records based upon the POST data which would explain why you get more entries displayed. I also suspect you aren't checking the POST data properly and you are getting an empty record. If you at least change the $_POST to $result in the while() loop you will at least be seeing the data from the database. To echo your data in a textarea, just do exactly that <textarea name="fieldname"><?php echo $value; ?></textarea> Note, when echoing user entered data to a page, always be sure to apply the appropriate "escaping" mechanism. When displaying as HTML use htmlentities(), in an input field use htmlspecialchars() -
EDIT: reread your post and it is different than I initially thought. Assuming you are hosting multiple sites using the same code-base you would likely have some different static content (i.e. flat-files) and either separate databases or a single database where all the records have a foreign key for each site. So, in your logic you would need to check the domain name used to access the site (check the $_SERVER global var), then find the root of the domain name. Once you have that, use the value to determine the correct folder(s) and the correct databases or site ID to use.
-
You will only see the source sent to the browser with the original request. If you are dynamically creating content on the page using JavaScript it will not be included in the HTML source. If you are trying to screen-scrape a page, I don't know of a solution; although I'm sure there are some. If this is YOUR page, then you can either have the output sent to the page with the first request - i.e. not with JS.
-
Hmm... I've reviewed the query a few times now and don't see any reason why that error would be triggered assuming the correct data is getting passed to the function. I have a suspicion that the ID being passed to the function is not correct. Try adding the following code for debugging purposes and see what you get. if (mysql_num_rows($query) !== 0) { /* Username already exists */ echo "<p><p><span class='current_admin'>Username already exists</span>"; echo '<p>Please <a href="javascript:history.go(-1)">Go Back</a> and complete the form<br>'; echo '<META HTTP-EQUIV="Refresh" CONTENT="8; URL=users_authorized.php">'; ## START DEBUGGING CODE echo "Query: $query<br>\n"; echo "Results: <pre>"; while($row = mysql_fetch_assoc($query)) { print_r($row); } echo "<pre>\n"; ## END DEBUGGING CODE }
-
I asked you to "output" your tables (i.e. to a text file) not attach an image. If you want my help, at least take the time to provide the information to me so I don't have to manually enter records. Anyway, after making the correction I had previously asked you to make, the results I get are correct. However, I found one possible problem. If a parent does not have any children associated with it, the parent was not included in the output. I assume that is contrary to what you want. This is easily fixed by making the join a LEFT JOIN. But, that created another issue where a record may not have child data. So, I added another condition to only display the child records if the data isn't empty. Lastly, I added some "tabs" into the HTML code to provide some structure in the output. The code mysql_connect($__DB_SERVER__, $__DB_USER__, $__DB_PASS__) or die(mysql_error()); mysql_select_db($__DB_NAME__) or die(mysql_error()); $query = "SELECT pp.ID, pp.Name, p.pageName, p.pageID FROM parent_pages AS pp LEFT JOIN pages AS p ON p.parentID = pp.ID ORDER BY pp.Name"; $result = mysql_query($query) or die(mysql_query()); //Open the parent UL $menuHTML = "<ul>\n"; $currentParentID = false; while ($row = mysql_fetch_assoc($result)) { if($currentParentID !== $row['ID']) { //This is a new parent page if($currentParentID!==false) { //If not first parent, close last submenu UL and parent LI $menuHTML .= "\t\t</ul>\n"; $menuHTML .= "\t</li>\n"; } $currentParentID = $row['ID']; //Open new parent LI, create link and open submenu UL $menuHTML .= "\t<li class=\"mainnav\">\n"; $menuHTML .= "\t\t<a rel=\"nofollow\" href=\"view.php?id={$row['ID']}\">{$row['Name']}</a>\n"; $menuHTML .= "\t\t<ul>\n"; } //Create submenu LI (if exists) if(!empty($row['pageID'])) { $menuHTML .= "\t\t\t<li class=\"subnav\">"; $menuHTML .= "<a rel=\"nofollow\" class=\"sublink_nav\" href=\"viewpage.php?pageid={$row['pageID']}\">{$row['pageName']}</a>"; $menuHTML .= "</li>\n"; } } //Close last child UL, parent LI and parent UL $menuHTML .= "\t\t</ul>\n"; $menuHTML .= "\t</li>\n"; $menuHTML .= "</ul>\n"; echo $menuHTML; The output <ul> <li class="mainnav"> <a rel="nofollow" href="view.php?id=2">Group</a> <ul> </ul> </li> <li class="mainnav"> <a rel="nofollow" href="view.php?id=3">Spa</a> <ul> </ul> </li> <li class="mainnav"> <a rel="nofollow" href="view.php?id=1">Stre</a> <ul> <li class="subnav"><a rel="nofollow" class="sublink_nav" href="viewpage.php?pageid=1">Site 1</a></li> </ul> </li> <li class="mainnav"> <a rel="nofollow" href="view.php?id=4">Well</a> <ul> <li class="subnav"><a rel="nofollow" class="sublink_nav" href="viewpage.php?pageid=3">Site 3</a></li> <li class="subnav"><a rel="nofollow" class="sublink_nav" href="viewpage.php?pageid=2">Site 2</a></li> </ul> </li> </ul> Here is an image of the rendered output (note I used some slightly different data since you made me type it in).