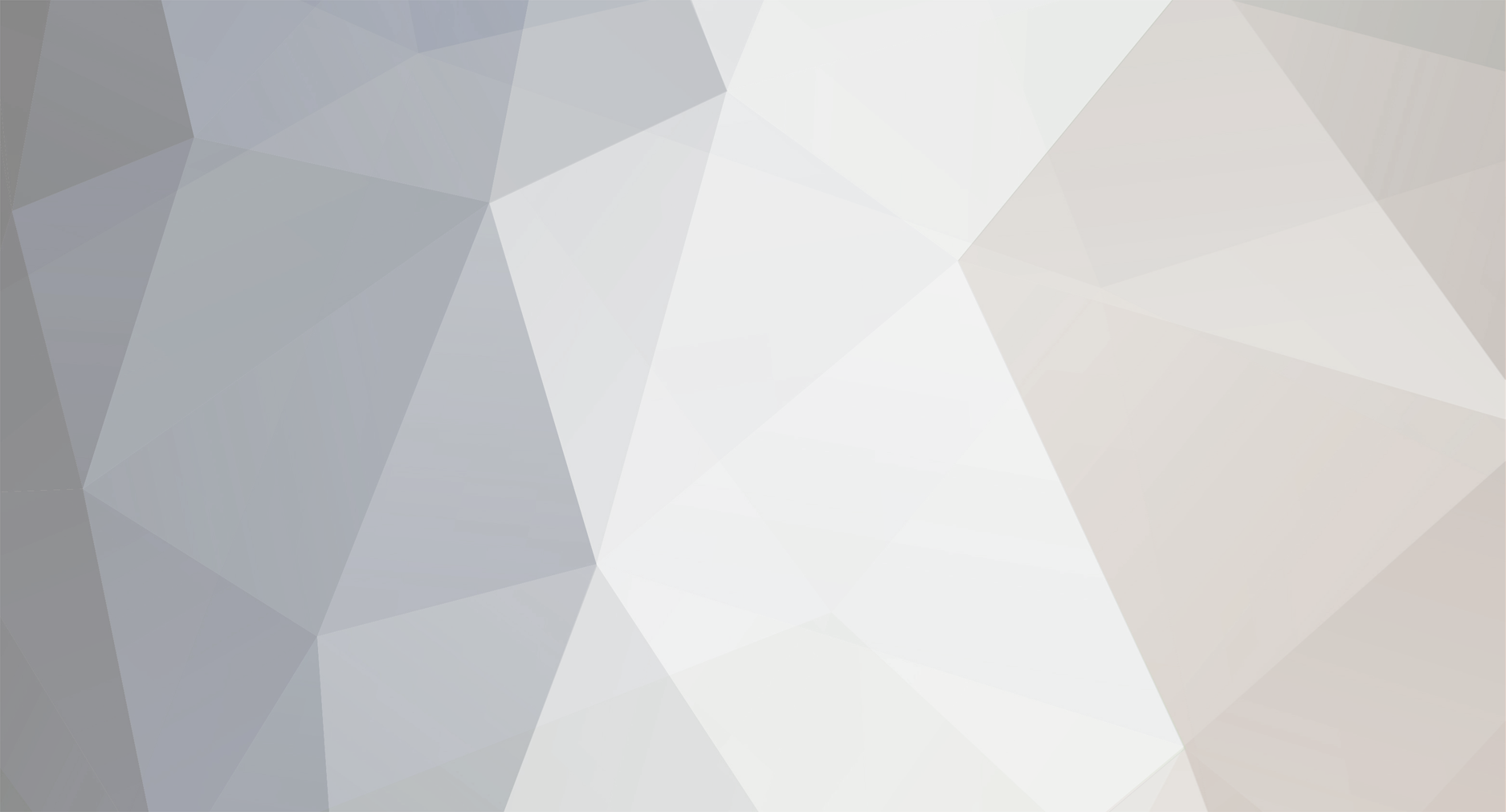
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Dump the results for all three records into an array, then use the array for the output <?php $query = "SELECT * FROM news WHERE published = '1' ORDER by id DESC LIMIT 3"; $row_news = mysql_fetch_array($query); $news = array(); while($row = mysql_fetch_assoc($row_news)) { $news[] = $row; } ?> <div id="myTabs"> <ul> <li><a href="#firsttab"><?php echo $news[0]['shorttitle']; ?></a></li> <li><a href="#secondtab"><?php echo $news[1]['shorttitle']; ?></a></li> <li id="last"><a href="#thirdtab"><?php echo $news[2]['shorttitle']; ?></a></li> </ul> <div id="firsttab" class="tab_content"> <div class="rafat"> <img src="<?php echo $news[0]['image']; ?>" /> <p><?php echo $news[0]['shortstory']; ?></p> </div> </div> <div id="secondtab" class="tab_content"> <div class="rafat"> <img src="<?php echo $news[1]['image']; ?>" /> <p><?php echo $news[1]['shortstory']; ?></p> </div> </div> <div id="thirdtab" class="tab_content"> <div class="rafat"> <img src="<?php echo $news[2]['image']; ?>" /> <p><?php echo $news[2]['shortstory']; ?></p> </div> </div>
-
You have your logic backwards. You have to check if there were results BEFORE processing the results. Although, I have a hard time believing that you are getting no results and no errors. <?php //Connect to DB mysql_connect ("localhost", "John","Harley08") or die (mysql_error()); mysql_select_db ("Customers"); //Parse user input and run query $term = mysql_real_escape_string(trim($_POST['term'])); $query = "SELECT * FROM storage WHERE box_number = '$term'"; $result = mysql_query($query); //Check results of query if (!$result) { //There was an error running the query echo "There was an error running the query<br><br>\n"; echo "Query:<br>{$query}<br><br>\n"; echo "Error:<br>" . mysql_erro(); } else if(mysql_num_rows($sql) < 1) { //No records returned echo 'No Boxes available please use your back button to select a new box.'; } else { //There were records returned, display them while ($row = mysql_fetch_array($sql)) { echo "box_num: {$row['box_number']}<br>\n"; echo "dept: {$row['Department']}<br>\n"; echo "company: {$row['Company']}<br>\n"; echo "status: {$row['status']}<br>\n"; echo "location: {$row['location']}<br>\n"; echo "description: {$row['box_desc']}<br><br>\n"; } } ?>
-
Um, you are building a for-pay site and you are having problems with the code. But, you don't give us any specifics about the code in question, just attach a single script with over 2,000 lines of code !!! You seriously expect us to just read through 2,000 lines of code and provide a solution? You need to post the specific section of code that is not working as you expect and explain what it is doing and what you expect it to do. However, you state you want to use page_id=[number] as the parameter in your code. But, I did a FIND in that script and don't see $_GET['page_id'] referenced anywhere.
-
There are plenty of such scripts freely available if you look. below is some code I have laying around which should work: function createThumb($src_file, $dst_w, $dst_h) { //Validate that valid sizes and readable image passed if ($dst_w<1 || $dst_h<1) { return false; } if (!list($src_w, $src_h, $src_type) = @getimagesize($src_file)) { return false; } //Supported image types & the functions to use $createFromSource = array( IMAGETYPE_JPEG => 'imagecreatefromjpeg', IMAGETYPE_GIF => 'imagecreatefromgif', IMAGETYPE_PNG => 'imagecreatefrompng', IMAGETYPE_WBMP => 'imagecreatefromwbmp', IMAGETYPE_XBM => 'imagecreatefromwxbm', ); //Validate that image type is supported if (!$createFromSource[$src_type]) { return false; } //Default values for thumb creation $dst_x=0; $dst_y=0; $src_x=0; $src_y=0; //Resize based upon source/destination ratios $ratio = min(($dst_w/$src_w), ($dst_h/$src_h)); $dst_h = $src_h * $ratio; $dst_w = $src_w * $ratio; //Read the source image $src_image = $createFromSource[$src_type]($src_file); //Create the thumb $dst_image = imagecreatetruecolor($dst_w, $dst_h); imagecopyresampled($dst_image, $src_image, $dst_x, $dst_y, $src_x, $src_y, $dst_w, $dst_h, $src_w, $src_h); return $dst_image; }
-
There isn't one. The code you have is storing the raw data of the image into the database. There isn't even a "file" with the process you are taking. If you want to display the image you will have to read the data from the database and send it as a jpg image. Here is a page that shows the process in a very rudimentary manner: http://digitalpbk.blogspot.com/2007/04/using-php-for-more-than-html.html Of course, you could go the other way. Instead of storing the raw data in the database you could save the actual file on your server and store the path to the file in your database.
-
How are you getting the names now? DB query or what? show the code you are currently using.
-
What is this "life" you speak of?
-
EDIT: I was working on this when the last few posts were made, but I'll submit anyway as the info would be helpful for others debugging a similar issue ---------------------------------------------------------- When you use var_dump() don't just look at what is presented in the browser - check the actual HTML source. I have a suspicion that the "£", or another character is actually a character code in the $POST value. Example: $text = "This is a <string> of text"; var_dump($text); What shows in the browser string(32) "This is a >string< of text" What is in the actual HTML source string(32) "This is a >string< of text"
-
How to order mysql results from most recent entry in while loop
Psycho replied to jdock1's topic in PHP Coding Help
That is a lame excuse. I used to poop my pants when I was a baby, but I learned how not to do that anymore (well, most of the time anyway). You really need to rethink the process you go through when coding. It is completely obvious from walking though that code why ALL the records would be deleted. 1. You run a query to get all the records to display. 2. You run a loop to display all the records and in that loop you also A. Create a Delete button with a value of "Delete message" B. You generate a delete query for each record and run it if ($_POST['delete'] == "Delete message") is passed. Think about that for a moment. If you attempt to delete ANY records then the $_POST value above will be true in every iteration of the loop - so all records are deleted. This isn't hard stuff, just basic logic. Pikachu already explained that you need to use the ID of the record when determining which one to delete. Also, you should NEVER run queries in loops. Lastly - ADD comments to your code. It will help you and others <?php //Check if there was a record selected for deletion if(isset($_POST['delete'])) { $deleteID = (int) $_POST['delete']; $query = "DELETE FROM `messages` WHERE `id` = '{$deleteID}' LIMIT 1"; $result = mysql_query($query, $link2) or die(mysql_error()); echo "<font color='#00FF00'>Message deleted from database</font>"; } //Send email if msg exists in POST data if (isset($_POST['msg'])) { $to = $_POST['email']; $subject = 'Reply from numbergame.com'; $message = "\n{$_POST['msg']}\n"; $headers = "From:[email protected]\r\n"; mail($to, $subject, $message, $headers); } //Get all records and display $query = "SELECT * FROM messages ORDER BY date DESC"; $result = mysql_query($query, $link2); while ($row = mysql_fetch_array($result)) { echo "<fieldset> <legend><div class=\"mhead\">Message from <em>{$row['from']}</em> on <em>{$row['date']}</em></div></legend><br> <div class=\"mbody\">{$row['input']}</div><br> <div class=\"mreply\"><u>Reply</div></u><br> <form action=\"\" method=\"post\"> <input type=\"hidden\" name=\"email\" value=\"{$row['email']}\" /> <textarea name=\"msg\" cols=\"30\" rows=\"15\" style=\"background-color:#6D6968\" /></textarea><br> <input type=\"submit\" name=\"submit\" value=\"Send\" /> </form> <br><br> <form action=\"\" method=\"post\"><input type=\"submit\" name=\"delete\" value=\"{$row['id']}\" /></form> </fieldset>"; echo "<img src='imgs/hr.png' alt='HR' />"; } ?> -
Why not just use range() instead of creating a loop? public function __construct($min=1,$max=49) { if( $max<=$min ) throw new Exception('Max number is less than or equal to min number'); $this->numbers = range($min, $max); }
-
I'm not sure which page is the sending page and which is the receiving page. BUt, I see you are not consistent in the naming ("product" vs. "product_id"). If the first script is posting to the second page, then your problem is that the form action is specified as follows: And the second script is trying to reference the value using
-
Wouldn't that put all the form's values in the URL? I don't want that. I just want the product_id carried in the URL. I did notice I forgot the method attribute and added it as POST. Still the same issue. Yeah, my bad. I see you were appending it to the action value. Do a print_r($_GET) on the receiving page to validate if the value is there and there is a problem in the code.
-
Your form doesn't have a method property on it. It my be passing the data as POST values (although I thought the default was GET). Anyway, try setting the method to method="get"
-
How to order mysql results from most recent entry in while loop
Psycho replied to jdock1's topic in PHP Coding Help
Um, why are you referencing $row['id'] in your query?! You are using $row as the array variable for accessing the the DB results. You should be referencing a $_POST value. As Pikachu stated, show the code for creating the form fields and for processing the delete. -
a couple of enhancements I would like to make to my search form
Psycho replied to webguync's topic in PHP Coding Help
To be honest, I read your post yesterday and decided not to respond because you just simply posted all of your code and expected us to read it all to understand the logic and tell you how to do it. I'm not willing to invest the time needed to do that. You need to provide the specific information around a particular problem and make it easier for us volunteers to help you. For example, regarding the highlighting problem I really don't understand what you are doing. You specified a style for an ID (e.g. #search_term). There can only be one element on a page with a particular ID. So, if there were multiple instances of the search term I don't know how you expect to apply that style to each of them. I would think you would want to create a named class (i.e. .search_term). Second, you have some JavaScript which seems to be for applying the style? Not sure, but I see the "#search_term" name in the JavaScript. But, since I see nothing in your PHP code for applying the style property I assume you are trying to do it with JS. So, for the style issue, first create the style as a class. Then implement some functionality in the PHP code to apply the style to the seach term in the output. Personally I would probably do something right at the beginning of the while() loop to go through all of the record values and modify them to highlight the search term if it exists. Here is an example (not sure it is the correct syntax for an object while($row = mysql_fetch_object($result)){ foreach($row as $key -> $value) { $row->$key = str_ireplace($term, "<span class=\"search_term\">{$term}</span>", $value); } $string .= "<tr>"; Note: that is not tested and has a problem with your current code. At the top of your page you apply some parsing to the search term: $term = strip_tags(substr($_POST['search_term'],0, 100)); $term = mysql_escape_string($term); You want to use the first $term (before applying mysql_escape_string()) for the str_ireplace() function. So, you should do something like $term = strip_tags(substr($_POST['search_term'],0, 100)); $termSQL = mysql_escape_string($term); And then use $termSQL in the DB querry -
Do you have queries running within loops? That is the absolutely worst thing you could be doing with regard to efficient programming in reference t DB activities. Post the script from one of the pages that is timing out and we may be able to help you fix the problems.
-
After looking over the original request I see I created the logic to look for a VALID input. btherl's wasn't correct either. In that one an empty $Website would make the first condition true (since it does not match the expression), therefore the parser will not check the other condition because of the OR. You could use the first one I give and wrap it all inside parens with a "!" before it: elseif ( !(preg_match('~^[a-z0-9.-]+\.(com|org|net|edu|co.uk)~i', $Website) || ($Website=='' && $Tickbox=="True")) ) { //Invalid entry } basical the condition is ( [it is a valid address] OR ([address is empty] AND [Tickbox = "True"]) ) which is the validation for correct input, but by putting a "!" before the entire thing it will return TRUE for a false condition.
-
There is no difference between OR or ||, although I tend to use || most of the time myself. Same with AND and &&. http://php.net/manual/en/language.operators.logical.php
-
elseif (preg_match("~^[a-z0-9.-]+\.(com|org|net|edu|co.uk)~i", $Website) OR ($Website=='' AND $Tickbox=="True"));
-
Weird, works fine for me. I used a txt file with a number on each line from 1 to 100. These were my results: Words: 18 , 62 Words: 58 , 92 Words: 13 , 80 Words: 65 , 95 Words: 80 , 82 Words: 4 , 61 Words: 1 , 79 Words: 3 , 43 Words: 69 , 78 Words: 13 , 82 Note that the ORDER of the results is not random in that for each pair the lower value is always first. So, if it matters if the order of the pair is random you would want to be using shuffle() anyways
-
I really try to refrain from disrespecting people (well, usually) but you really need to pull your head out. That is the same invalid code you posted originally. You have been told several times in this post what the problem is and how to fix it, but you go back to what you originally had. //Create a query string $query = "SELECT * FROM u_data WHERE username='$username'"; //Run the query and put RESULT REFERENCE ID into variable $result = mysql_query($query) or die(mysql_error()); //Check if there are any rows in the RESULT REFERENCE ID if(mysql_num_rows($result)>0) { //User exists echo "Username already in use."; //Add proper error handling } else { //User name does not exist - continue }
-
Difficulty understanding multidimensional form array
Psycho replied to mykmallett's topic in PHP Coding Help
Yes, that is called a ternary operator and acts as a quasi IF/ELSE statement. I use it most frequently when I need to assign one of two values to a variable based on a condition. But, it has other uses as well. Here is the basic structure: ([CONDITION]) ? [TRUE_RESULT] : [FALSE_RESULT]; -
As already stated, you cannot run a function within a query. So, you need to take another approach. From your explanation I am assuming that there is one unique record per user in the 'userkeys' table. Also, your example query above is using a WHERE clause on the field 'keynote', but based on your first explanation I'm guessing that isn't what you really want. I do not understand music whatsoever. But, if the user has a selected keychange of '2' and the user is doing a search for the key 'Eb' is it correct that the only matches would be on songs with an original keynote of 'C#'. If so, then you could use a revised function that takes the users keychange and the target keynote to determine the original keynote. Then do your query based on that.
-
Difficulty understanding multidimensional form array
Psycho replied to mykmallett's topic in PHP Coding Help
Also, I want to advise against doing a separate INSERT query for each record - it is a very bad practice. Here is a more complete script for the processing logic $userID = (int) $_SESSION['user_id']; //Generate insert values for each record $linkValues = array(); foreach($_POST['links'] as $linkID => $linkValue) { //Parse input $linkID = (int) $linkID; $linkValue = mysql_real_escape_string(trim($linkValue)); //Create insert record if value entered if(!empty($linkValue)) { $linkName = ($linkID!=3) ? 'NULL' : mysql_real_escape_string(trim($_POST['link_name'])); $linkValues[] = "($userID, '$linkValue', $linkID, '$linkName')"; } } //Create and run ONE insert query for all entered values $query = "INSERT INTO [table_name] (`USER_ID`, `LINK_URL`, `LINK_TYPE`, `LINK_NAME`) VALUES " . implode(', ', $linkValues); $result = mysql_query($query) or die(mysql_error()); -
Difficulty understanding multidimensional form array
Psycho replied to mykmallett's topic in PHP Coding Help
No need for hidden fields. Create your form fields AS arrays with the link ID as the array index. Something like this: Facebook Link: <input type="text" name="links[1]" /><br> Twitter Link: <input type="text" name="links[2]" /><br> Custom Link: <input type="text" name="links[3]" /><br> Link Name: <input type="text" name="link_name" /> Then in your PHP code you can loop through all the entries using foreach($_POSTS['links'] as $linkID => $linkValue) { //Do something with input }