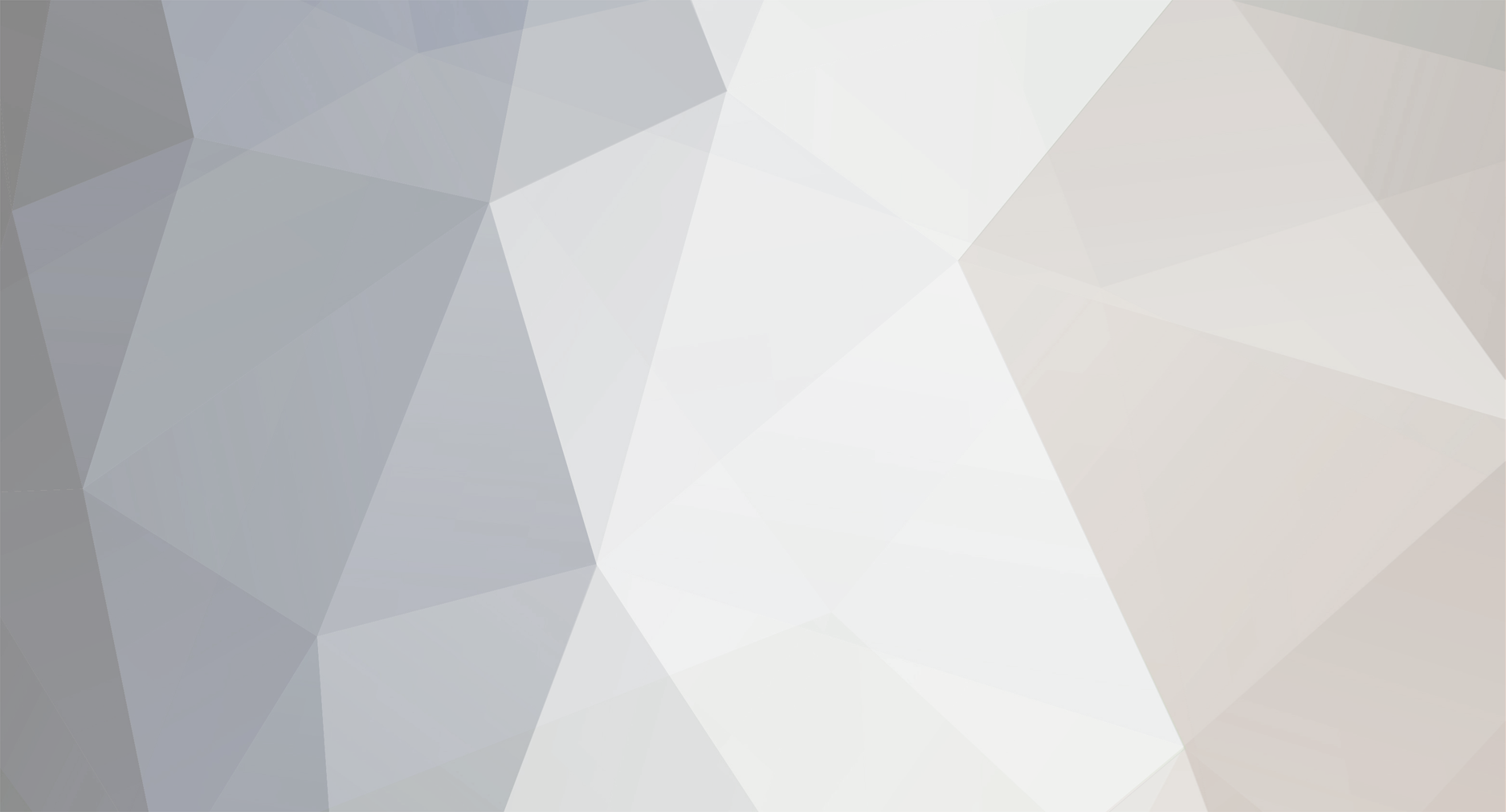
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
You do NOT want to create a new column in your database to store the "elapsed time". That should be calculated in real-time. 1. You should store dates as dates, number as an appropriate number type (in, float, etc) and text as text. There is a date() functions to transform a date value into whatever format you want for displaying the value. Do not store data in the wrong format because of how you want to display it. You modify the value in PHP code after getting it from the database. 2. Yes you could do the calculation in PHP with the varchar value, but you shouldn't be using the varchar for a date to begin with. Even though you should store the date as a date there is a slight hurdle in converting MySQL dates to PHP dates and vice versa. Take a look at this article: http://www.richardlord.net/blog/dates-in-php-and-mysql So first, convert your submission_date field to a datetime field. Then, when saving a date, create a MySQL valid datetime value using $mysqlDate = date( 'Y-m-d H:i:s', $phpdate ); and use that value in your insert query When running a query to get your records you will need to process the value in PHP to convert it to a PHP date using strtotime(). At the same time you can calculate the elapsed time while($row=mysql_fetch_assoc($result)) { $submission_date_ts = strtotime($row['submission_date']); //Timestamp $submission_date_str = date("M-d-Y", $submission_date_ts); //String in format MMM-DD-YYYY $time_elapsed = (time() - $submission_date_ts); //Elapsed time in seconds // }
-
In addition to what you requested, I made other modification to make your code flow in a more logic order. Not tested, so there may be some syntax errors. <?php //Create options for the DAY select list $dayOptions = "<option value="">Day</option>\n";; for($day = 1; $day <= 31; $day++) { $selected = ($day==$_POST['dobd']) ? ' selected="selected"' : ''; $dayOptions .= "<option value=\"{$day}\"{$selected}>".date('d', mktime(0,0,0,0,$day,0))."</option>\n"; } //Create options for the MONTH select list $monthOptions = "<option value="">Month</option>\n"; for($month = 1; $month <= 12; $month++) { $selected = ($month==$_POST['dobm']) ? ' selected="selected"' : ''; $monthOptions .= "<option value=\"{$month}\"{$selected}>".date('M', mktime(0,0,0,$month+1,0,0))."</option>\n"; } //Create options for the YEAR select list $yearOptions = "<option value="">Year</option>\n"; for($year = 1998; $year >= 1911; $year++) { $selected = ($year==$_POST['doby']) ? ' selected="selected"' : ''; $yearOptions .= "<option value=\"{$year}\"{$selected}>{$year}</option>\n"; } ?> <select name="dobd"> <?php echo $dayOptions; ?> </select> <select name="dobm"> <?php echo $monthOptions; ?> </select> <select name="doby"> <?php echo $yearOptions; ?> </select>
-
So, why are you using a varchar to store a date? But, I'm not understanding your request. "what i would like is to have another field which would calculate time elapsed." Time elapsed from when to when? Do you want the time elapsed from 'submission_date' to today, vice versa, or what? You talk about a "new field" but, not sure if you are talking about a new field to store the time elapsed (which makes no sense) or if it is for something else. Once you get the records you can find the time elapsed in PHP with the varchar value using strtotime() and subtracting that from time() to get the time elapsed.
-
Of course it is possible. And, as MrAdam stated, it does not make sense to split the array - it only adds complexity. You don't show the full details of your array, so this is only mock code: <?php $tableOutoput = ''; foreach($grade as $region => $regionUsers) { //calulate totals/averages for the region $userCount = count($regionUsers); $userValuesTotal = array_sum($regionUsers); $userValuesAverage = ($userCount>0) ? $userTotalValues/$userCount : 0; $tableOutoput .= "<tr>\n"; $tableOutoput .= "<td>{$region}</td>\n"; $tableOutoput .= "<td>{$userValuesTotal}</td>\n"; $tableOutoput .= "<td>{$userValuesAverage}</td>\n"; $tableOutoput .= "</tr>\n"; } ?> <table> <tr> <th>Region</th> <th>User Count</th> <th>User Total Values</th> <th>User Average Values</th> </tr> <?php echo $tableOutoput; ?> </table>
-
Which is why there are things such as CRON which can be used WHEN a specific time occurs.
-
It would be interesting to know what, specifically, you want it to do. But, yes, it is possible. Look running "cron" jobs. There's too much to try to explain in a forum post - especially when there are many resources readily available.
-
Yeah, still not following you. Here is what I *think* is going on. You are running some JS against records displayed in the chatbox but you are apparently using the rank from the current user and applying that against each user record in the chatbox. Not really sure if that is correct or not. But, it seems what you need to do is when creating the chatbox in PHP you need to retrieve the relevant variables for each record and store them as JS variables. Since you are apparently referencing the chatbox records by their DOM id, I would store the values accordingly. In your PHP that creates the initial chatbox you could create a JS array that matches the DOM array in the format var ranks = new Array(); ranks[0] = 3; ranks[1] = 1; ranks[2] = 5; //etc The PHP code would look something like this: $jsRanksArray = "var ranks = new Array();\n"; //Loop to create output $index = 0; while($row = mysql_fetch_assoc($result) { //-------------- //Code to produce chatbox output goes here //-------------- //Add to JS array code for current record $jsRanksArray .= "ranks[0] = '" . $row['rank'] . "';"; $index++; } To be honest I am just guessing here as you are leaving information out that is needed to really provide a solution.
-
There IS an edit button, but it is only available for about 20 minutes after making a post. I can help you, but the problem you need to resolve is NOT the actual query, but how you process the query. You already have an ORDER BY clause and I will assume it is in the correct order that you will present the values. Here is some rough code to show one way to display the records in a "grouped" format. I only added the output for some of the fields since I am lazy. <?php //Run query to get records returned in proper order $query = "SELECT a.id, a.id AS did, a.dates, a.enddates, a.shortdescription, a.max_attendance, a.times, a.endtimes, a.registra, a.unregistra, a.titel, a.locid, a.status, a.shw_attendees, l.club, l.url, l.street, l.please, l.city, l.country, l.locdescription, c.catname, c.id AS catid FROM #__registrationpro_dates AS a LEFT JOIN #__registrationpro_locate AS l ON l.id = a.locid LEFT JOIN #__registrationpro_categories AS c ON c.id = a.catsid WHERE a.published = 1 AND c.access <= 0 ORDER BY c.ordering, a.dates, a.times "; $result = mysql_query($query) or die(mysql_error()); //Create output for the records grouped by month/year $output = ''; //Variable to store HTML output $currentMonthYear = false; //Flag to detect when month/year changes between records while($row = mysql_fetch_assoc($result)) { //Get MM-YYYY value for current record $thisMonthYear = date('F-Y', $row['dates']); //If this Month/Year different than current Month/Year create new header if($currentMonthYear != $thisMonthYear) { $currentMonthYear = $thisMonthYear; $output .= "<tr><th colspan=''><h1>Records for {$currentMonthYear}</h1><th></tr>\n"; $output .= "<tr>\n"; $output .= "<tr><th>Description</th></tr>\n"; $output .= "<tr><th>Max Attendance</th></tr>\n"; $output .= "<tr><th>Times</th></tr>\n"; $output .= "<tr><th>End Times</th></tr>\n"; $output .= "<tr><th>Club</th></tr>\n"; $output .= "<tr><th>City</th></tr>\n"; $output .= "<tr><th>Country</th></tr>\n"; $output .= "<tr><th>Description</th></tr>\n"; $output .= "</tr>\n"; } //Display current record $output .= "<tr>\n"; $output .= "<td>{$row['shortdescription']}</td>\n"; $output .= "<td>{$row['max_attendance']}</td>\n"; $output .= "<td>{$row['times']}</td>\n"; $output .= "<td>{$row['endtimes']}</td>\n"; $output .= "<td>{$row['club']}</td>\n"; $output .= "<td>{$row['city']}</td>\n"; $output .= "<td>{$row['country']}</td>\n"; $output .= "<td>{$row['locdescription']}</td>\n"; $output .= "</tr>\n"; } ?> <table> <?php echo $output; ?> </table>
-
That makes the problem more complex, because now you will need to keep track of which month belongs to which index. In your example above "2011-06-01" is the date for the first record so you would make index 0 to represent "May". Now, when you get to the 2nd record and it has a date of "2011-06-05" how would the code "know" that the index of 0 already exists and that is represents the month of May. You could do it, but it would require creating another array to keep track of the month/index association and additional logic to check of the month of the current record is already in that array or if you need to create one. So, yes it can be done, but you would be making much more complicated than it should be $groupedArray = array(); $monthsArray = array(); foreach($originalArray as $key => $valuesAry) { //Get month of current record $month = date('n', strtotime($valuesAry['dates'])); //Check if month exists in index array if(!in_array($month, $monthsArray)) { //Month does not exist in index array add it $monthsArray[] = $month; } //Get month index value for current month $monthIndex = array_search($month, $monthsArray)); //Add record to new array under month index $groupedArray[$monthIndex][] = $valuesAry; }
-
Where are you getting these values from? If you are getting from a DB you should order the records appropriately there. Although, there is usually no need to physically group them in an array since you can handle that in the processing code. But, if you really need to do this, the only way I can see to do it is to loop over each record and create a new array. Also, I would set the key for the "grouped" elements to the month number for ease of use. $groupedArray = array(); foreach($originalArray as $key => $valuesAry) { $month = date('n', strtotime($valuesAry['dates'])); $groupedArray[$month][] = $valuesAry; } //$groupedArray now holds the data from $originalArray in a grouped format
-
You're really not making this clear. I have a good idea of the general issue you are facing but not sure what code you need because the specifics are clouded in your explanation. But, let's go with your Edit since you state that will make it more clear: echo "chat_div.innerHTML += userN2;"; \\returns "Username" That is impossible. That will echo the exact text between the quotes. This is where it is confusing (at least to me). $var = "chat_div.innerHTML += userN2;"; echo $var; returns chat_div.innerHTML += userN2; Ok, that makes perfect sense. Now, what I think you are having a problem with is that userN2 doesn't have a value in the JS. Is that correct? If so, you just need to set the value using something like this echo "var userN2 = '" . echo getRank($user); . "'; \n"; echo "chat_div.innerHTML += userN2;\n";
-
OK, so you have confirmed that the entire table is getting output, but when using the JavaScript to show/hide the contents of the table you are only getting the first row. So, I think we have ruled out a PHP problem - at least with the logic to produce the output. But, I still think the problem is due to something in the output, such as a character (or characters) in the output content that is screwing up the DOM schema such that the JavaScript is not working correctly. Can you save the rendered page as a flat HTML file and attach to the post?
-
I gave you the answer, I'm sorry if it doesn't fulfill your expectations. dbGetRows is not a PHP function. I can't alter what the function returns. Yes you could insert some inefficient code to keep countries from duplicating. But, you couldn't "remove" any values that don't have associated venues without running a separate query for each country. That just makes no sense. Replace this $countries = dbGetRows("country", "name != ''"); With something like this: $query = "SELECT Country.id, Country.name FROM Country RIGHT JOIN Venues ON Country.id = Venues.Country GROUP BY Country.id"; $countries = mysql_query($query);
-
From what you have showed, all of the content in the container div should display/hide when the toggleDiv() function is run. If that is not happening then there is likely some messed up HTML content inside the div that is causing the problem. For these types of implementations I would write a flat HTML file with static content for a few records. Once I get it to work and am happy with the layout and functionality, I will THEN create the PHP code to dynamically get the output to match my template
-
Here is a rewrite of your code that should produce the results you are after - but I did not test it as I don't have your database. Note, I created it so that you can separate the logic (PHP) from the output (HTML). <?php //selects all the users items to whoever is logged in $sql = "SELECT useritems.quantity, items.name, items.image FROM useritems JOIN items USING(itemid) WHERE userid='{$_SESSION['userid']}' LIMIT {$offset}, {$rowsperpage}"; $result = mysqli_query($cxn, $sql) or die(mysqli_erro($cxn)); $imagecount = 0; $tableOutput = ''; while ($row = mysqli_fetch_assoc($result)) //while there are still results { $imagecount++; $quantity = $row['quantity']; $name = $row['name']; $image = $row['image']; //Open new row if($imagecount%5==1) { $tableOutput .= "<tr>\n"; } //Create TD $tableOutput .= "<td width=\"120px\" align=\"center\">"; $tableOutput .= "<img src=\"http://www.elvonica.com/{$image}\"><br>"; $tableOutput .= "{$name} ({$quantity})"; $tableOutput .= "</td>\n"; //Close current row if ($imagecount%5==0) //after 5 items have been listed, start a new line { $tableOutput .= "</tr>\n"; } } //Close last row if needed if($imagecount%5!=0) { $tableOutput .= "</tr>\n"; } ?> <table cellspacing="0" class="news" align="center"> <?php echo $tableOutput; ?> </table>
-
You don't state what Venues.Country is a foreign key reference for. It SHOULD be the Country.id field and NOT the Country.Name field. But, I have a feeling that isn't the case. Anyway, you just need a simple query to get the list of countries where there are existing venues SELECT Country.id, Country.name FROM Country RIGHT JOIN Venues ON Country.id = Venues.Country GROUP BY Country.id
-
Why are you using extract($row) and then defining variables based upon the variable $row?!!! Assuming $row contains an index named "quantity", the extract function will automatically create a variable called $quantity with the value associated with that index. You then redefine $quantity using $row['quantity']. That makes no sense. Second, why are you running a query in your loop? Just do a join in the first query to get all the records you need at one time. As to your particular issue, I suspect there is a problem with one of the two values you are using on the LIMIT clause. But, you don't show how those are defined, so I can't help you there. But, try echoing the query to the page to ensure it has the values you expect.
-
There are two problems with your code: 1) If the user is updating other info, but not their username, the code you have will always fail because there will already be a user with the username passed to the function (the one editing their info). You need to see if there are existing users with the same user name EXCLUDING the current user. 2) Your update query doesn't have a WHERE clause - it is trying to update ALL the records in the table. Update queries should almost always have a WHERE clause. If it doesn't have one you need to be very sure before running it, lest you overwrite all the records in the table. Also, it is good practice to create your queries as variables, then run those variables as the query parameter. That way, if there is a problem, you can echo, log etc. the query to the page for analysis. function editUser($userUsername, $userPassword, $userEmail, $userRealname, $id) { //See if there is ANOTHER user with same username $query = "SELECT * FROM authorized_users WHERE username = '$userUsername' AND id <> $id"; $query = mysql_query($query) or die(mysql_error()) ; if (mysql_num_rows($query) !== 0) { /* Username already exists */ echo "<p><p><span class='current_admin'>Username already exists</span>"; echo '<p>Please <a href="javascript:history.go(-1)">Go Back</a> and complete the form<br>'; echo '<META HTTP-EQUIV="Refresh" CONTENT="8; URL=users_authorized.php">'; } else { /* Username doesn't exist */ $query = "UPDATE authorized_users SET username ='$userUsername', password = '$userPassword', email = '$userEmail', realname = '$userRealname' WHERE id = $id"; $query = mysql_query($query) or die (mysql_error()); echo '<p><p><b> 1 User Added</b>'; echo '<META HTTP-EQUIV="Refresh" CONTENT="4; URL=users_authorized.php">'; } }
-
With that array format, you have no option other than iterating over each of the array values to check if the sub-array has a processing of 0 or 1. However, if this array is coming from a DB query, then you should simply do an array to see if all of the target values have a processing value of 0.
-
I think the OP is wanting to see if there are duplicate values in the array. So, if he is looping through the array and finds that element '2' has a value of 'foo', how would he determine if there is another value 'foo' in the array with a higher index. It would be helpful if you were to explain the process you are trying to accomplish. I can think of a few different solutions, but which one I would go with would be directly tied to what the goal is. But, most likely I would be using array_count_values(): http://php.net/manual/en/function.array-count-values.php
-
Yep, most likely, what you need to be doing is querying ALL the records you need and then handle the differentiation of the output in the processing code. using your example query above where you are interested in age, let's say you want to display lists of people in the database but you want to break it out by age groups. So, separate tables for babies, toddlers, children, teens, young adults, etc. etc. You would do just one query to get all the records and order the results by age. Then, as you process the records you would insert new tables as needed when you detect that the age has changed to the next level.
-
By IP? That means if there are multiple users behind the same NAT router you will only allow one vote for all of them. Not to mention, people can spoof their IP address. Anyway, since you are allowing votes for different articles for the same users, I think you should use a separate table to capture the votes. An example would be a table called "votes" with field for "base_id", "IP", "vote". Since you only appear to let people vote in the affirmative (i.e. vote up but not down) the "vote" field is not really necessary since you could just do a COUNT() of the votes for a particular article. But, I'd use it anyway in case you need more functionality later on. So, on your "voteup.php" page you would change the query as follows: $IP = $_SERVER['REMOTE_ADDR']; $query = "INSERT INTO votes (`base_id`, `IP`, `vote`) VALUES ($id2, '$IP', 1)"; mysql_query($query); To get the votes for an article, you would need to do a join on the votes table. (By the way, you would need to remove the votes field from the "base" table). Example query SELECT base.*, SUM(votes.vote) as votes FROM base JOIN votes ON base.id = votes.base_id GROUP BY base.id
-
I created a function that allows you to determine which words to retain within the quoted text based upon the index. function removeQuotedWords($string, $retainIdxAry) { $matched = preg_match('#"([^"]*)"#', $string, $quotedText); if($matched == 0) { return false; } $quotedWordsAry = explode(' ', $quotedText[1]); $replacWordsAry = array_intersect_key($quotedWordsAry, array_flip($retainIdxAry)); $replaceTextStr = implode(' ', $replacWordsAry); return preg_replace('#"([^"]*)"#', "\"{$replaceTextStr}\"", $string); } $data = 'hello world "this is a test" after text' echo removeQuotedWords($data, array(0,3)); //Output: hello world "this test" after text
-
Kind of hard to really provide a solution based on the information provided. Can there be multiple instances of quoted text in the string? Is there text before AND after the quoted string(s)? I would like to know more information before I invest time in writing any code. But, I think the approach I would take would be to use a regular expression to get the quoted text, split it using explode, remove the unwanted words and implode it back, and finally replace the original value with the modified text.