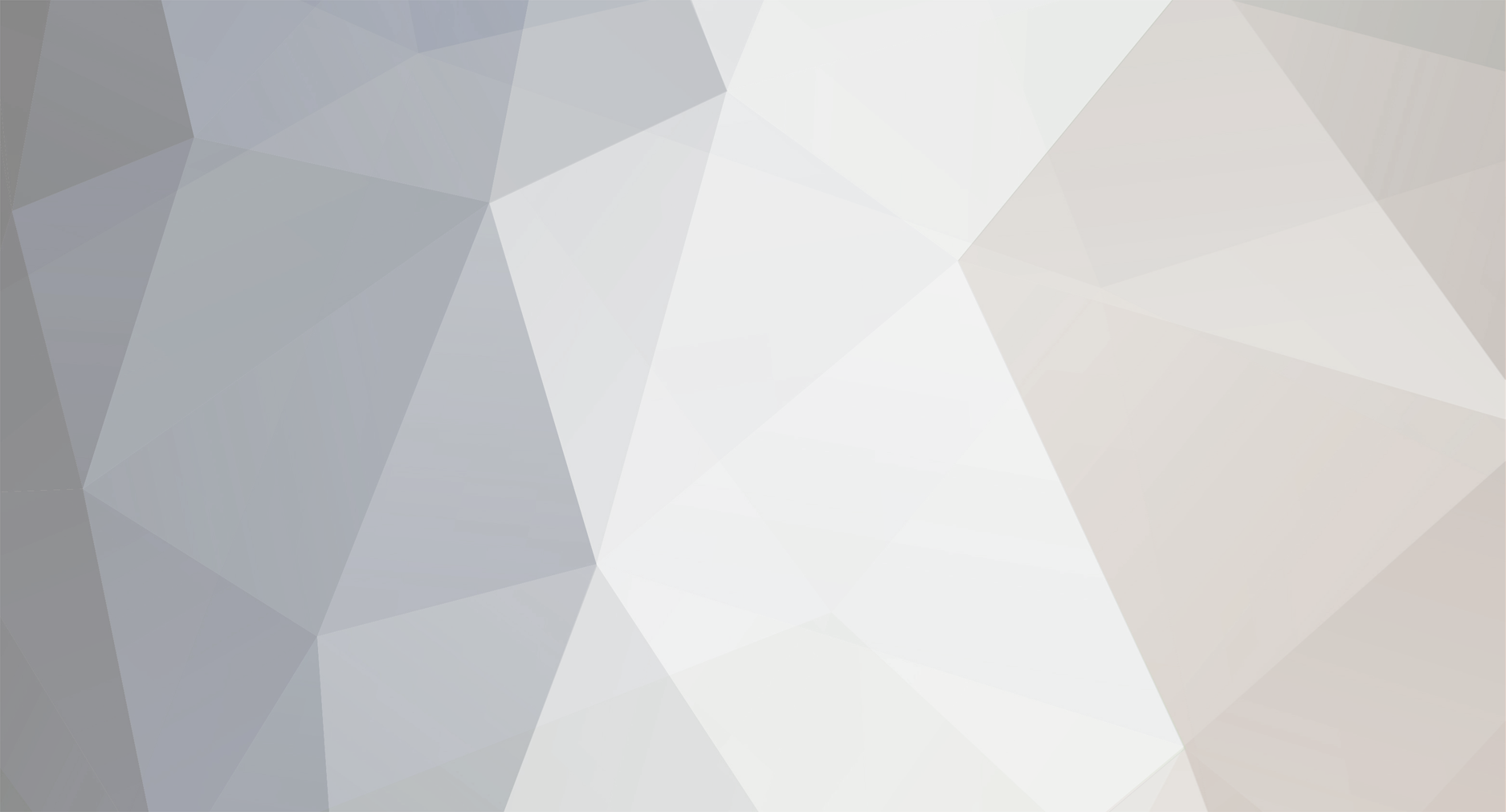
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
I have the following expression to get all "words" from a string using [space], [comma], [period], and [hyphen] as delimiters. It works as expected. preg_match_all("/(\b[^ |\-|,|\.]+)/", $input, $matches); But, I really only want up to the first three occurrences. I tried adding {1, 3} after the parenthesized matching pattern preg_match_all("/(\b[^ |\-|,|\.]+){1,3}/", $input, $matches); but it still returns all the matches. Any ideas what I am doing wrong?
-
Actually, I'm not sure how that would be interpreted. You don't need to the '+'. The '+' as a modifier means to match one or more occurrences. But, you are already using '{2}' which means to match exactly two occurrences. So, if you removed the '+', then - yes - it would match 'had [two_digits_number]' Hmm, I thought I explained that. The period is a wild card for any character. The asterisk is a modifier that say match 0 or more occurrences. By default the '*' is greedy which means it will match as many instances as it can. Even digits are "any character4" so the * would make it match everything to the end of the string. But, you only want to match to where the word 'had' starts. So we add the '?' to make the '*' non-greedy, so it will match as few characters as possible. In this case it will match every character up to the word 'had'.
-
How to Know When a Checkbox Has Been Ticked Off?
Psycho replied to chaseman's topic in PHP Coding Help
So, a normalized database is a cluttered database? I guess being able to run efficient queries and do proper joins are just fluff. I don't use WordPress (I guess I know why now), but I can't believe that it doesn't allow you to properly use a database. No need to run a foreach loop, use the functions that PHP makes available to you. $page_id_string = implode(' ', $page_id); -
How to Know When a Checkbox Has Been Ticked Off?
Psycho replied to chaseman's topic in PHP Coding Help
Why would you be saving a list of values in a single field in the database? You should use an associated table with an individual record for each value with a foreign key reference to the parent record. But, let's say you go with the flawed approach above. Why do you need to know which values were unchecked? When the user submits the form, just replace the value with the ones that are still checked. Rough example $excludeVals = implode(' ', $_POST['exclude']); $query = "UPDATE [table_name] SET [field_name] = $excludeVals WHERE user_id = $userID"; -
The problem is that the function is closed with the "}" character BEFORE you have closed the string with the HEREDOC tag. function documentType(){ echo <<<HEREDOC <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" ` ` "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> HEREDOC; } //This goes AFTER you have completed the echo
-
That is a "Regular Expression" I can explain THAT regular expression, but to really understand them will take quite a bit of time for you to research. In my opinion, Regular Expressions are one of the most powerful features in programming, but also one of the hardest to master. In the above regular expression the hash marks (#) are just delimiters and do not define anything to match on. The "(.*?)" searches for ANY characters. The period is a wildcard for any character, the * means to match 0 to many occurrences of any character and the ? makes the match non-greedy. That means it will stop matching as soon as a match is found for the next parameter. The next parameter is "(had \d*)" which will match the letters "had" followed by a space. Immediately following the space there must be o or more numbers (the wildcard d is used to match digits and the * matches 0 or more). On second though I should have used a + which will match one or more occurrences.
-
You contradicted yourself. You said you wanted everything before the word "had", but your first expected result was "«There was a man»". What about the word "who"? But based upon your explanation, what you want is fairly simple: function splitString($string) { if(preg_match("#(.*?)(had \d*)#", $string, $matches) > 0) { unset($matches[0]); return $matches; } //Return false if there was no match return false; } $parts = splitString('There was a man who had 13 legs and looked nice'); print_r($parts); $parts = splitString('My pocket had 41 coins before I spent them'); print_r($parts); Output Array ( [1] => There was a man who [2] => had 13 ) Array ( [1] => My pocket [2] => had 41 )
-
delete javascript generated form fields
Psycho replied to LittleSmilingMonster's topic in Javascript Help
Ok, first off you need to change the field names to be arrays. Otherwise, if you recreate the fields with the exact same name you will only receive the values from the last set of fields. Just add "[]" to the end of the field names. But, because you are using a hidden set of fields to duplicate the field sets you will always have that empty set sicne the user would never see them to be able to enter data. Secondly, to Delete a field set you will need to have some way to reference the container div in order for the delete operation to reference. You could give each DIV a unique ID or you could pass a reference to the Delete link and then traverse up through the parents to the container DIV. Personally, I think that is all a pain in the a**. A question you should ask yourself is if there is any real value in allowing the user to add an unlimited number of field sets. Is there some realistic maximum number that you would expect a user would really need? Let's say you think a user would only ever need to add 15 attributes at most. A simpler solution would be to create the form with 20 field sets with 19 of them hidden. Then allow a user to Add/Delete field sets which would display/hide those field sets. ALSO: Your "forms" are invalid and you would not receive proper data anyway. You have forms within forms. -
Or, just use strcasecmp()
-
Help With preg_replace adding extra Gaps that arn't in the CSV file
Psycho replied to sniesler08's topic in PHP Coding Help
EDIT: I took a slightly different approach so I will post my code even though it is close to requinix's solution. I think mine has the added advantage of a single array in a logical format. $file = "widget.csv"; @$fp = fopen($file, "r") or die("Could not open file for reading"); $outputArray = array(); while(($line = fgetcsv($fp, 1000, ",")) !== FALSE) { $outputArray[array_shift($line)] = array_filter($line); } print_r($outputArray); Here is the first part of the array where the primary index is the associated letter for each group of records. Array ( [A] => Array ( [0] => Airlie Beach [1] => Andergrove [2] => Alexandra [3] => Armstrong Beach [4] => Alligator Creek ) [b] => Array ( [0] => Bucasia [1] => Blacks Beach [2] => Beaconsfield [3] => Bakers Creek [4] => Balberra [5] => Bloomsbury [6] => Breadalbane [7] => Ball Bay [8] => Belmunda ) [C] => Array ( [0] => Cannonvale [1] => Calen [2] => Crystal Brook [3] => Cremorne [4] => Chelona [5] => Campwin Beach [6] => Cape Hillsborough [7] => Conway ) -
Show 1 Movie frm DBase and give page links Page 1 Page 2 Page 3
Psycho replied to noobtopro's topic in PHP Coding Help
#1: Please enclose your code in [ code ] tags for readability. #2: What is your question? This is obviously a homework assignment and you have attempted the problem on your own. Way to go! Most people just try and pass their homework as a "real" problem and try to get someone to write it all for them. Are you getting any errors or is the code doing something other than you expect it to? -
@cunoodle2: You should be careful when creating loops such as this. while($sCurrentDate < $sEndDate){ The OP didn't state "where" these dates would be coming from but they could be coming from user input. If so, the user could accidentally input the dates backwards. In that case (or if the programmer made a mistake) you would have an infinite loop.
-
I thought I had a function like this before, but can't find it. I found something similar and modified it accordingly. It takes an optional third parameter in case you want the date format differently. function getDateRange($startDate, $endDate, $format="Y-m-d") { //Create output variable $datesArray = array(); //Calculate number of days in the range $total_days = round(abs(strtotime($endDate) - strtotime($startDate)) / 86400, 0) + 1; if($days<0) { return false; } //Populate array of weekdays and counts for($day=0; $day<$total_days; $day++) { $datesArray[] = date($format, strtotime("{$startDate} + {$day} days")); } //Return results array return $datesArray; } $dateRange = getDateRange('2011-05-03', '2011-05-08'); print_r($dateRange); Output: Array ( [0] => 2011-05-03 [1] => 2011-05-04 [2] => 2011-05-05 [3] => 2011-05-06 [4] => 2011-05-07 [5] => 2011-05-08 )
-
Well, it is assumed that each user will have a unique ID since they must log in. I would also assume you have the ability for users to view other user's profiles. So, on a user's profile page include a button (i.e. form) or link which directs the browser to an "add friend" page. In the form or link pass the user ID of the user whose profile was being viewed. Then, on the processing page, use that value to save a record to identify the "friend" link. This all assumes you understand how to build a database and make the php pages work with the database.
-
What, exactly, do you mean by "it seems to be ignoring the 'and' part of the query". Is the query returning ALL records where the first name is a match regardless of whether the last name matches or not? OR, is it returning results where the first name matches or the last name matches? However I suspect your problem is due to a type
-
OK, you are naming your input fields so they will be sent/received as array - that is good. But, you are going to need to provide indexes for the fields such that a checkbox and it's related fields can be associated with one another. You are already using the user ID as the value of the checkbox, so you can use that as the index for the field names. Here are the fields I see in your code and one way you could provide indexes echo '<select name="adminlvl['.$user->id.']">'; // echo '<select name="ban['.$user->id.']">'; Then in your processign code you could do something like this foreach($_POST['check'] as $userID) { //Get the values to be updated for the selected user ID $adminlvl = $_POST['$_POST['check'][$userID]; $ban = $_POST['$_POST['ban'][$userID]; //Create query to update selected record $query = "UPDATE user SET adminlvl='$adminlvl', ban='$ban' WHERE id = $userID"; } NOTE: that is only a preliminary example. I have left all validation/escaping that should be done.
-
Yes, everything in the form will be passed with the POST data. You could probably implement some JavaScript to run at the time of submission to remove those values, but that's not necessary. What you need to do is implement the solution on the processing page by checking which checkboxes are checked and then only processing the associated values. If you were to show the form and the processing code we could help you further.
-
First of all, that query would be failing since you are trying to group by columns called 'source' and 'duration' and the actual column names are 'Source' and 'CallDuration'. Second, even if you fixed the names it would return an empty result set using the data you had above. The reason is that for the numbers that have no group code they would have a null value in the joined data. By the way, based upon your stated logic, shouldn't that last record be included in your result set since 104 is not in the group list? Anyway, after using that mock data I have a query that I think will work for you SELECT source, destination, SUM(CallDuration) as total_duration FROM calls_table LEFT JOIN group_table as g1 ON calls_table.Source = g1.number LEFT JOIN group_table as g2 ON calls_table.Destination = g2.number WHERE g1.username <> g2.username OR g1.username IS NULL OR g2.username IS NULL GROUP BY Source, destination Results source destination total_duration 101 2564114526 50 1234567890 0101010101 150 1234567890 0201040506 50 1234567890 104 50 If the Source values will always exist in the group table then you don't need the last OR statement.
-
Oh, hell no! Never run queries in loops - it kills the server's resources. All you need to do is run a single query that uses a JOIN on the two tables. Each record in the result will have the document name. You just use logic in the PHP code to only display the document title once. $query = "SELECT d.doctitle, p.party FROM documents as d JOIN parties as p USING(docid)"; $result = mysql_query($query) or die(mysql_error()); //Variable to track change in document title $current_doc = false; while($row = mysql_fetch_array($result)) { if($current_doc != $row['doctitle']) { //New document, show doc title echo "<br><b>{$row['doctitle']}:</b>\n"; $current_doc = $row['doctitle']; } //Display party echo "<b>{$row['party']}:</b>\n"; }
-
Yes, I understand that, read my previous post. The problem that is causing that error is on a line before line 60. The reason the error is showing for line 60 is that is the line where it came upon a character that did not make sense within the context of the previous code! If you were to run this code: <?php echo "this is a test" if($a==$b) { echo "They are equal"; } ?> You will get an error on line 5 (the line with the IF statement) But, the error is actually on line 3 where there is no semi-colon at the end of the echo statement.
-
You could try and google PHP and language file or localization. I typically just build my own solution. Here is a very rough example en.php (english language file) $__TEXT__ = array ( 'name_label' => "Name", 'customer_id_label' => "Customer ID", 'address_label' => "Address" } If you want to support different languages, you would have a separate file for each and you would need to implement logic to determine the appropriate language for each user and choose the right one. Otherwise just hard code the language file you will use. Then in your scripts use a reference to the variable to use. include('language\en.php'); $query = "SELECT * FROM table"; $result = mysql_query($query); $HTMLoutput = ''; while($row = mysql_fetch_assoc($result)) { $HTMLoutput .= "<tr>\n"; $HTMLoutput .= " <th>{$__TEXT__['name_label']}</th>\n"; $HTMLoutput .= " <td>{$row['name']}</td>\n"; $HTMLoutput .= "<tr>\n"; $HTMLoutput .= "<tr>\n"; $HTMLoutput .= " <th>{$__TEXT__['client_id_label']}</th>\n"; $HTMLoutput .= " <td>{$row['client_id']}</td>\n"; $HTMLoutput .= "<tr>\n"; $HTMLoutput .= "<tr>\n"; $HTMLoutput .= " <th>{$__TEXT__['address_label']}</th>\n"; $HTMLoutput .= " <td>{$row['address']}</td>\n"; $HTMLoutput .= "<tr>\n"; $HTMLoutput .= "<tr><td colspan=\"2\"> </td></tr>\n"; }
-
OK, after some further verification it looks like the above expressions were preventing some records to be properly identified. I don't have the time to evaluate all the records to determine the problem. Just comment out the lines identified below and you should get the ID, image URL and name of each user. I can't guarantee it will always work based upon how that website modifies their data $pattern = array(); $patterns[] = "id=\"r4_([^\"]*)\""; $patterns[] = "class=\"userImage\"><img.*?src=\"([^\"]*)\""; $patterns[] = "<\/span> ([^<]*)<"; //$patterns[] = "<strong class=\"fn\">([^<]*)<"; //$patterns[] = ", ([^\n]*)"; //$patterns[] = "(Listening|Last track):"; //$patterns[] = "<a href=\"\/music\/[^\"]*\">([^<]*)<"; //$patterns[] = "<a href=\"\/music\/[^\"]*\">([^<]*)<";
-
I assumed you want more than just the IDs. The following code will provide the id, image url, name(s), bio for each user, plus their listening or last track info. $lastfm = file_get_contents('http://www.last.fm/group/Rishloo/members'); $pattern = array(); $patterns[] = "id=\"r4_([^\"]*)\""; $patterns[] = "class=\"userImage\"><img.*?src=\"([^\"]*)\""; $patterns[] = "<\/span> ([^<]*)<"; $patterns[] = "<strong class=\"fn\">([^<]*)<"; $patterns[] = ", ([^\n]*)"; $patterns[] = "(Listening|Last track):"; $patterns[] = "<a href=\"\/music\/[^\"]*\">([^<]*)<"; $patterns[] = "<a href=\"\/music\/[^\"]*\">([^<]*)<"; $pattern = "#" . implode('.*?', $patterns) . "#sim"; preg_match_all($pattern, $lastfm, $matches, PREG_SET_ORDER); //Remove first element from each record, it contains HTML code that will mess up the output //This is not needed and only included so I can print_r the results to the page foreach($matches as $idx => $value) { unset($matches[$idx][0]); } echo "<pre>\n"; print_r($matches) This is probably not the most efficient way to get that data, but it works. Here is some of the output from the above: Array ( [0] => Array ( [1] => 20770702 [2] => http://userserve-ak.last.fm/serve/64s/60856343.jpg [3] => Alice_in_pains [4] => nermin [5] => 20, Female, Azerbaijan [6] => Last track [7] => Tool [8] => Useful Idiot ) [1] => Array ( [1] => 35133964 [2] => http://userserve-ak.last.fm/serve/64s/55291785.jpg [3] => agostinoo [4] => Agostino [5] => 19, Male, United Kingdom [6] => Last track [7] => Cat Power [8] => Fool ) [2] => Array ( [1] => 38461866 [2] => http://userserve-ak.last.fm/serve/64s/61233861.jpg [3] => maverikgameface [4] => Reece Gerard [5] => 20, United States [6] => Last track [7] => A Perfect Circle [8] => Weak And Powerless )
-
Personally, I would recommend against using the db field names in this manner. You should have the "labels" in the application explicitly defined. Either hard code the values in your code, or even better, use a resource file. A resource file allows you to change the name of a label throughout your application by making one change and it allows you to re-purpose the application for different languages if you so choose. By programatically using the DB names you risk displaying text that may be inappropriate/confusion if a DB field is changed at a later time and you have now boxed yourself into using names that have to be able to be converted to a "friendly" value that may not make sense for your DB structure. But, to answer your question anyway, you can simply replace all underscores with a space and then use ucwords() to upper-case the first letter of every word. You don't need two queries. The field name is available in the query results as long as you use the right mysql_fetch statement. Here is a rough example <?php $query = "SELECT * FROM table"; $result = mysql_query($query); $show_header = true; $HTMLoutput = ''; while($row = mysql_fetch_assoc($result)) { if($show_header) { //Display header row if first record $HTMLoutput .= "<tr>\n"; foreach($row as $label => $value) { $label = ucwords(str_replace('_', '', $label)); $HTMLoutput .= "<th>{$label}</th>\n"; } $HTMLoutput .= "</tr>\n"; $show_header = false; } //Display record row $HTMLoutput .= "<tr>\n"; foreach($row as $value) { echo "<td>{$value}</td>\n"; } $HTMLoutput .= "<tr>\n"; } ?> <table> <?php echo $HTMLoutput; ?> </table>