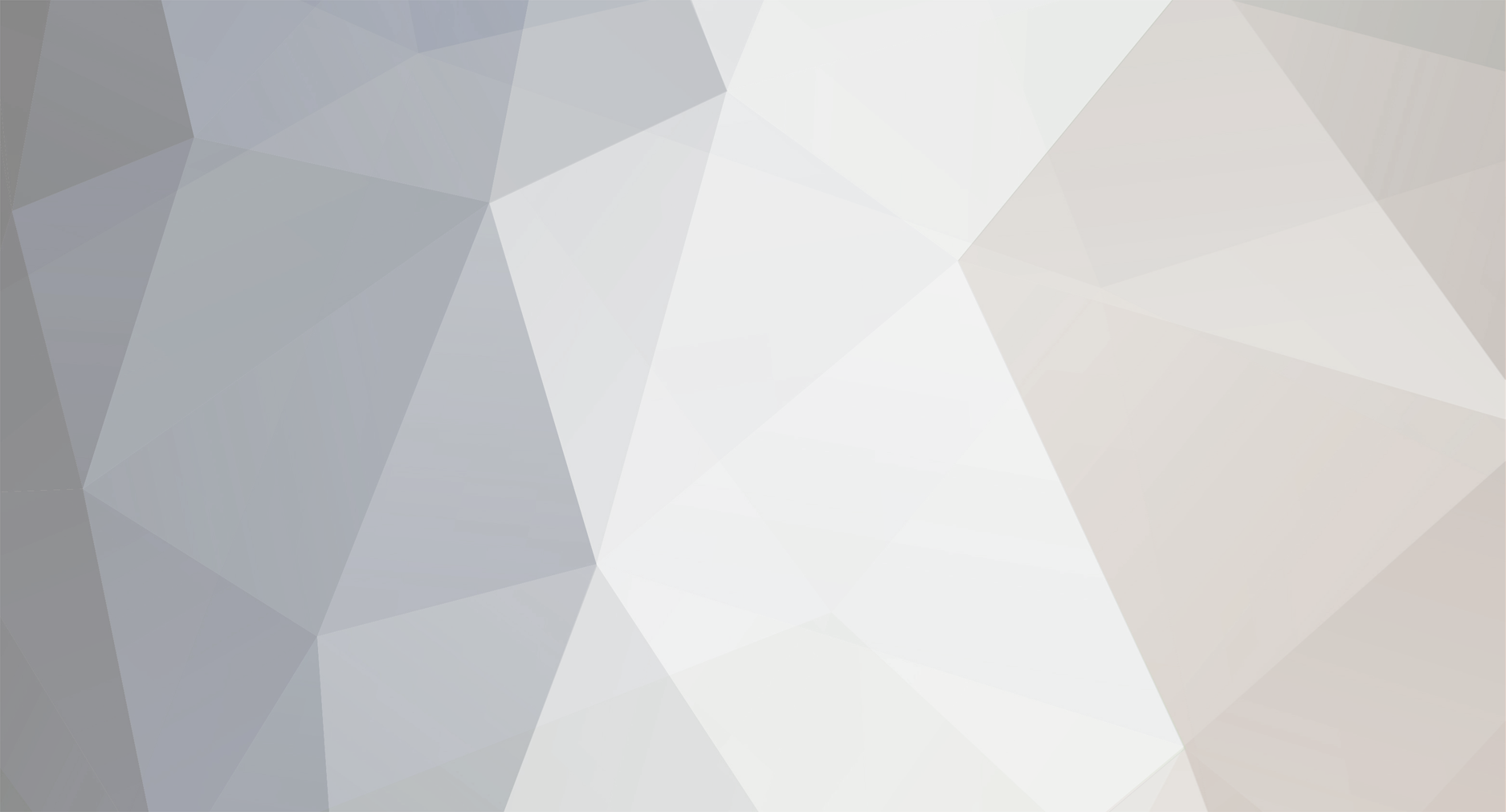
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Then btherl's suggestion would be the easiest to implement
-
$cars = array('ford', 'toyota', 'bmw'); $places =arrray('Orlando','California','Texas'); $combined_array = array_combine($cars, $places); $values = array(); foreach ($combined_array as $car => $place) { $values[] = "('$car', '$place')"; } $query = "INSERT INTO vehicles (cars, places) VALUES " . implode(', ', $values);
-
Why not include a file at the top of the page(s) you want to track to add a "click" for each view?
-
I am gonna pull my EYES OUT!!!! What is wrong here???????
Psycho replied to Bl4ckMaj1k's topic in PHP Coding Help
Those are PHP magic constants that return the currently running file name and line number of the file. That way, when you see your debug information, if something seems out of place it gives you an idea of where to look in your code. No idea what you are talking about. The example above is for illustrative purposes. In that example you would make a call to the debug() function any place that makes sense. Just pass whatever text/values that would be helpful for the particular situation along with the magic constants so you can see where the debug was called from. -
I am gonna pull my EYES OUT!!!! What is wrong here???????
Psycho replied to Bl4ckMaj1k's topic in PHP Coding Help
Here is a sample script with a debugging function implemented. You can turn debugging on/off with the first variable. You would want debugging code used in key places in your code, such as: - any branching logic (If/Else or switch statements) - where variables are set/changed to verify the values - when performing certain operations that could fail (DB queries, reading files, etc.) <?php session_start(); //Var to determine if debugging is on or off $debugMode = true; function debug($debugText, $file='Unknown', $line='xx') { global $debugMode; //Do nothing if debug mode is not on if(!$debugMode) { return false; } //Display debug message echo "<b>DEBUG [{$file}: {$line}]:</b><br />\n"; echo "{$debugText}<br /><br />\n"; return; } if($foo == $bar) { debug("\$foo ($foo) equals \$bar ($bar)", __FILE__, __LINE__); $query = "SELECT * FROM table WHERE fieldName = '$somevalue'"; $result = mysql_query($query); if(!result) { debug("Query Succeeded: {$query}", __FILE__, __LINE__); //Do some other stuff } else { debug("Query Failes: {$query}<br />Error".mysql_error(), __FILE__, __LINE__); //Do some other stuff } } else { debug("\$foo ($foo) doe not equals \$bar ($bar)", __FILE__, __LINE__); //Do some other stuff } ?> -
I am gonna pull my EYES OUT!!!! What is wrong here???????
Psycho replied to Bl4ckMaj1k's topic in PHP Coding Help
As ManiacDan was saying you need to incorporate debugging code. In my opinion, this should be done as part of the coding process. It is very easy to implement debugging into your code so that it is not exposed in a production environment. I like to create a debugging function that will echo/not echo the debugging info to the page based upon a switch that I can turn on or off. That way I can leave the debugging code in place even when I *think* I am done with the script. If I run into a problem later I just turn debugging back on. Also, You should always create your queries as a string variable THEN run the mysql_query(). If there are any problems you can echo the query to the page to validate that the values are what you expect. Your query is likely failing. Try the following: <?php session_start(); $successMsg = ''; $staff_id = $_SESSION['sid']; include_once "scripts/connect_to_mysql.php"; $customer_id = $_GET['cid']; $customer_id = mysql_real_escape_string($customer_id ); $customer_id = eregi_replace("`", "", $customer_id); $cust_project_id = $_GET['pid']; $cust_project_id = mysql_real_escape_string($cust_project_id ); $cust_project_id = eregi_replace("`", "", $cust_project_id); if (isset($_POST['billing_contact_fax_form_field_box'])) { $billing_contact_fname_form_field_box = $_POST['billing_contact_fname_form_field_box']; $billing_contact_lname_form_field_box = $_POST['billing_contact_lname_form_field_box']; $billing_contact_address_form_field_box = $_POST['billing_contact_address_form_field_box']; $billing_contact_city_form_field_box = $_POST['billing_contact_city_form_field_box']; $billing_contact_state_form_field_box = $_POST['billing_contact_state_form_field_box']; $billing_contact_zip_form_field_box = $_POST['billing_contact_zip_form_field_box']; $billing_contact_email_form_field_box = $_POST['billing_contact_email_form_field_box']; $billing_contact_phone_form_field_box = $_POST['billing_contact_phone_form_field_box']; $billing_contact_fax_form_field_box = $_POST['billing_contact_fax_form_field_box']; $billing_contact_pref_meth_contact_form_field_box = $_POST['billing_contact_pref_meth_contact_form_field_box']; $billing_contact_cc_billed_form_field_box = $_POST['billing_contact_cc_billed_form_field_box']; $query = "UPDATE billing_info SET contact_fname='$billing_contact_fname_form_field_box', contact_lame='$billing_contact_lname_form_field_box', address='$billing_contact_address_form_field_box', city='$billing_contact_city_form_field_box', state='$billing_contact_state_form_field_box', zip='$billing_contact_zip_form_field_box', email='$billing_contact_email_form_field_box', phone='$billing_contact_phone_form_field_box', fax='$billing_contact_fax_form_field_box', pref_meth_contact='$billing_contact_pref_meth_contact_form_field_box', cc_billed='$billing_contact_cc_billed_form_field_box' WHERE proj_id='$cust_project_id'"; echo "Query:<br />$query<br /><br />"; $sql = mysql_query($query) or die(mysql_error()); $successMsg = '<p style="font-family:Myriad Web Pro" style="font-size:10px" style="color:#666">You have successfully updated the company\'s General Information.<br /><br /> <a href="javascript:window.close();">Close</a></p>'; echo $successMsg; exit(); } ?> -
No, your pattern will work, although it is only going to replace the beginning of the tag as you stated. That is why I questioned what you were really trying to do. If you were trying to remove images and your patten was looking for anchor tags, that would explain why it wasn't working. Try the following: $input = ' Before link text <a href="http://s635.photobucket.com">Link Name</a> after link text Before image text <img src="theimage.jpg"> after image text'; $patterns = array( 'links' => '/<a[^>]*>.*?<\/a>/', 'images' => '/<img[^>]*>/' ); $replacement = "[illegal]"; $output = preg_replace($patterns, $replacement, $input); ?> <pre> <b>Input:</b><br /> <?php echo $input; ?> <br /><br /> <b>Ouput:</b><br /> <?php echo $output; ?> </pre>
-
You do realize that an anchor tag (i.e. the <A> tag for links) is NOT an image tag, right? So, stripping out "<a href" would not remove images (e.g. "<img src=")
-
Damn it! I was thinking there should be a function to do that.
-
This will convert the string into an array (or arrays) based on the name(s) used in the string. In this case it would be an array variable $importance $encodedString = "importance%5B101%5D=50&importance%5B100%5D=50&importance%5B99%5D=50&importance%5B98%5D=50"; $decodedString = urldecode($encodedString); $explodedVars = explode('&', $decodedString); foreach($explodedVars as $nameValuePair) { preg_match("#(.*)\[([^\]]*)\]=(.*)#", $nameValuePair, $matches); ${$matches[1]}[$matches[2]] = $matches[3]; } print_r($importance); /*Output--- Array ( [101] => 50 [100] => 50 [99] => 50 [98] => 50 ) */
-
Select the forum you want to post in and then select the "New Topic" link.
-
OK, now look at the data passed in the POST data and look at the core logic of your page and determine what should happen. Here is the last submission you made with your full code. I took out the "details" of the code and just left the branching structure (i.e. the IF/ELSE statements. I also added comments as to how that post data you just posted would be interpreted by your current logic. <?php $title="Login"; $metakeywords="login, email"; $metadescription="Login to Dating Snap!"; include('header.php'); $email = clean_up($_POST['email']); $pass = clean_up($_POST['password']); if($user && $pass) { //$_POST['password'] was not in the POST data //So this whole branch will not be executed } else { if(!$_GET['step'] && $_POST['newpassword']) { //$_POST['newpassword'] was not in the POST data //So this whole branch will not be executed } //end if email } include('footer.php'); ?> Based upon the code you previously posted and the results of the POST values sent to the page, nothing should happen.
-
Have you done a print_r($_POST) at the top of the page to validate what is being sent in the post data? If it does contain the value then you test the value of $email after setting it with clean_up(), and so on... Like I was saying, your code is very unorganized which makes debuggin much harder than it should be. FOr example, at the top of the page you are setting variables using the post data: $user = clean_up($_POST['email']); $pass = clean_up($_POST['password']); But, then you do the exact same thing with the same POST values in several places in the code. If you've already set the variables you need, there is not reason to set them again.
-
Debugging code does not mean randomly changing logic in the hopes that it will magically solve the problem. What is the field name that holds the id of the value you are trying to get? You have to test/validate what is/in not set and what the values are. Do a print_r($_POST) to validate what is being passed in the post data. I do see int he code above you are trying to populate that vallue into a hidden field echo "<input type='hidden' name='ida' value='$ide' />"; You named that field "ida".
-
@adamlacombe, you are never going to get anywhere if you don't lear how to properly debug code. Instead of just saying something doesn't work, you need to test the input/output at key steps to ascertain where the problem is. In this case the first thing you should have done was do a print_r($_POST) on the page when it is submitted. You would have seen that $_POST['email'] is getting passed with the literal value of "$email" - NOT the value you assigned to the variable $email. You are writing your hidden field within a single quoted string echo '<table width="100%"><form method="post" action="index.php?action=login&step=2"> <input id="email" type="hidden" name="email" value="$email"> <tr> Variables are NOT interpreted within single quoted strings. To be honest, your code really needs to be cleaned up. There is a ton of duplicity and no logical flow.
-
What is the correct using php to see if a checkbox is checked or not.
Psycho replied to jayhawker's topic in PHP Coding Help
That is incorrect. An unchecked checkbox field will not even exist in the POST data. If you don't believe me, run this test page: <html> <body> <form method="post"> Check/uncheck the checkbox and submit to see what is sent in POST data: <input type="checkbox" name="foo" value="bar" /><br /> <button type="submit">Submit</button> </form> <br /><br /> Post Data:<br /> <pre> <?php print_r($_POST); ?> </pre> </body> </html> -
The problem is likely in the code you use to create the "previous" link. You need to validate that you are passing the expected values. Also, I wouldn't use dd-mm-yyyy with strtotime(). I believe the standard would be mm-dd-yyyy. But, to be safe just use yyyy-mm-dd.
-
Simple, in your database for events have a startdate and enddate. If an event is only for a single day you could leave the enddate null or you could populate it with the same date as startdate. Or, alternatively, you could have startdate and days, where days is the number of days for the event.
-
What is the correct using php to see if a checkbox is checked or not.
Psycho replied to jayhawker's topic in PHP Coding Help
You mean to say if the "NAME" of the checkbox is "checkbox". . . The isset() function doesn't care what the value is - as long as the variable has been initiated (it could even have a NULL value). -
What is the correct using php to see if a checkbox is checked or not.
Psycho replied to jayhawker's topic in PHP Coding Help
A checkbox field is only passed IF the checkbox is checked. So, the isset() status would be the appropriate method to determine if the checkbox was checked or not. -
Yes, using an image as an input will pass the x,y coordinates. You could pass the form submission via POST so it does not get included in the URL.
-
Do NOT run MySQL queries in loops. Use the foreach loop to create the insert record text and then run one query at the end. $values = array(); foreach ($text as $value) { $code = rand(1, 1000000); $values[] = "('$value', '$code')"; } $sql = "INSERT INTO $table (text, code) VALUES " . implode(', ', $values); mysql_query($sql, $conn) or die(mysql_error());
-
if(isset($_POST['email']) && $_POST['email']!='') { $email = $_POST['email']; $_SESSION['email_login'] = $email; } elseif (isset($_SESSION['email_login'])) { $email = $_SESSION['email_login']; } else { $email = false; }
-
I already gave you an example of the UPDATE query in my first response.