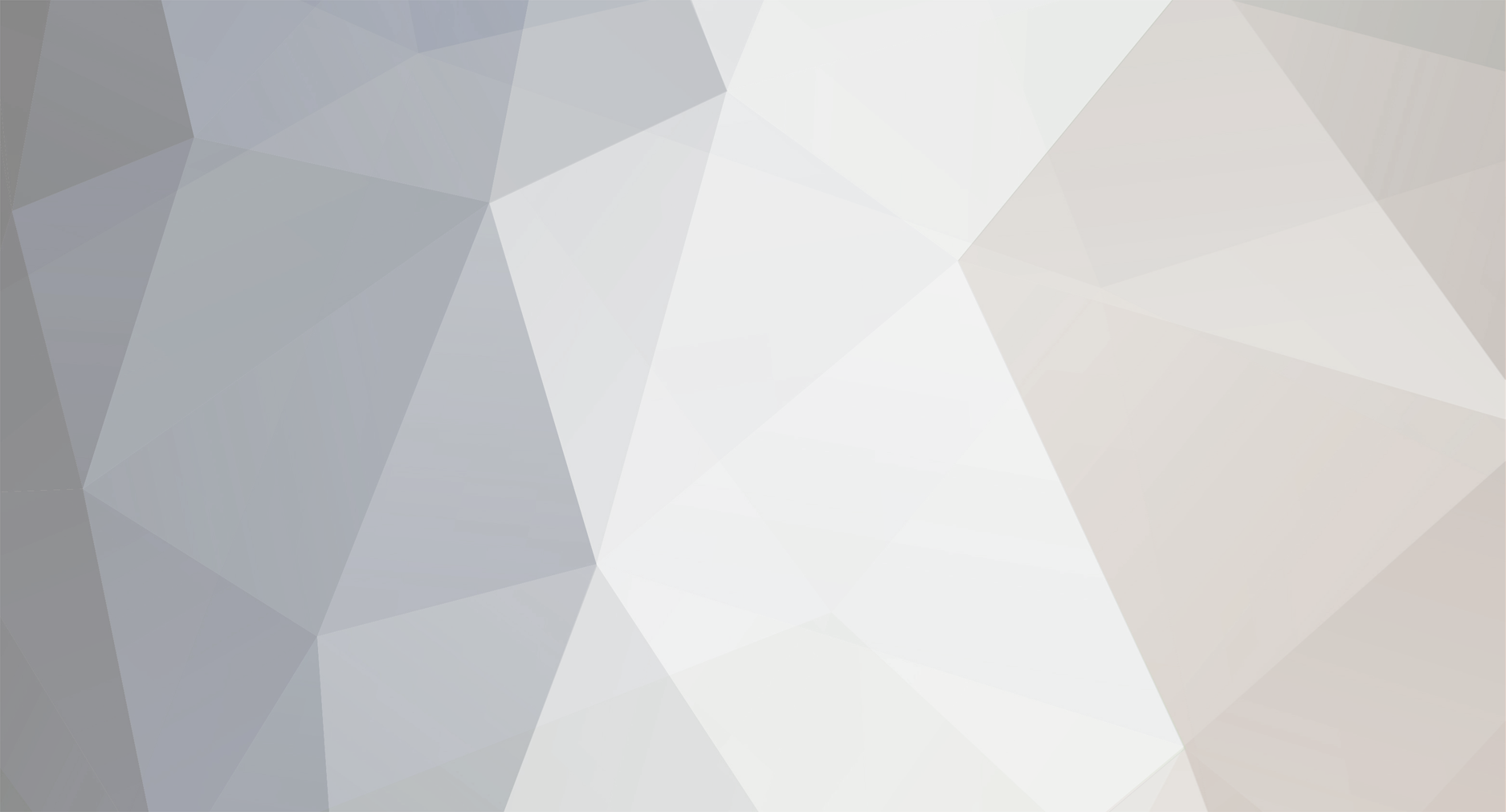
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Did you try the code I provided to see if it generates the results you are after?
-
Referring to: I would add that there is no need to query the record again after the update has occurred. You already have the updated values to be used for the UPDATE query. Just compare those values to the current values from the first query. In this case I think it would be easier to just iterate through each field instead of using array_diff() since that function doesn't give you a comparison of what changed. Here is a very rough example $record_id = (int) $_POST['id']; $field1Val = mysql_real_escape_string($_POST['field1']); $field2Val = mysql_real_escape_string($_POST['field2']); $field3Val = mysql_real_escape_string($_POST['field3']); //Create array of new values using same key names as in DB fields $new_record = array('field1'=>$_POST['field1'], 'field2'=>$_POST['field2'], 'field3'=>$_POST['field3']); //Get current record vlaues $query = "SELECT field1, field2, field3 FROM table WHERE id = $record_id"; $result = mysql_query($query); $old_record = mysql_fetch_assoc($result); $log_text = ''; foreach($new_record as $key => $new_value) { if($old_record[$key]!=$new_value) { $log_text .= "$key was changed from {$old_record[$key]} to $new_value,"; } } //Run query to UPDATE record $query = "UPDATE table SET field1=$field1Val, field2=$field2Val, =$field3Val WHERE id = $record_id"; $result = mysql_query($query); //Insert log entry if there were changes if($log_text != '') { $query = "INSERT into logs (user_id, date, text) VALUES ($userID, NOW(), '$log_text'"; $result = mysql_query($query); }
-
OK, so where do keys1 and keys2 come from? If you are wanting to know the duration of calls made by a user (I assume the 'source' field), then just do a GROUP BY on the source column. Or, you can get the duration of calls for each source to each destination by using a combined GROUP BY clause on the two fields. You still didn't provide the 2nd half of my request So, I am just assuming all of this. Here is an example of how you would get the combined duration for each unique source/destination combination SELECT source, destination, SUM(CallDuration) as total_duration FROM table GROUP BY source, duration
-
Define a different "time" for purposes of the db query $ctimeSQL = date("h:i:s", strtotime('-29 seconds')); And change your query as I showed above: $sql = "SELECT `value` FROM datatable WHERE time >= '$ctimeSQL' ORDER BY time ASC LIMIT 1"; Also, since you are only getting one record it makes no sense to use a while loop. // Define date and time $cdate = date("d-m-y"); // current server date $ctime = date("h:i:s"); // current server time -29 seconds $ctimeSQL = date("h:i:s", strtotime('-29 seconds')); // current server time -29 seconds // Connect to database $link = mysql_connect("$dbhost","$dbuser","$dbpass") or die(mysql_error()); // Connection starts here mysql_select_db("$dbname") or die(mysql_error()); // Create and run query $sql = "SELECT `value` FROM datatable WHERE time >= '$ctimeSQL' ORDER BY time ASC LIMIT 1"; // simple sql example $re = mysql_query($sql) or die(mysql_error()); // Here's where you'll get an error if the SQL is invalid. $row = mysql_fetch_array($re); //Output HTML echo $cdate; echo "<br>"; echo $ctime; echo "<meta http-equiv='refresh' content='20'>"; include("variables.php"); echo "<br>"; echo $row['value']; // value to be displayed for 30Sec after displayed on site. mysql_close($link);
-
I have no clue what you are wanting. Provide some examples of the data that would be in the database and the output you want generated.
-
I told you what it would do But, to explain further... The WHERE clause will match ALL records where time is >= $ctime. That would include the record you want plus the rest for the remainder of the day. But, we want the lowest of those values. So, we then ORDER the results by the "time" column in ASCending order so the first record is the smales and the last record is the greatest. Lastly, we restrict the result to only the very first record using LIMIT 1 On second though, though, this would not do exactly as you requested. If, the time was exactly 6:00:00 it would get the records that exactly matches 6:00:00. But, at 6:00:01 to 6:00:30 it would get the record for 6:00:30. So, the value would change every 30 seconds, but it wouldn't get the previous record in-between exact matches - it would get the next record. To get the previous record you would need to modify $ctime to be 29 seconds less OR you could modify the query to look for a value 29 seconds less. I would have to see how you are setting $ctime and/or know exactly what type of field "time" is in the database.
-
I assume you always want to show a value, so one solution would be to simply select the smallest time that is greater than or equal to the current time. SELECT * FROM datatable WHERE time >= '$ctime' ORDER BY time ASC LIMIT 1
-
I would highly recommend taking a more organized approach to your validations. Try to avoid a lot of nested IF/Else statements. I typically do all my validations and then - at the end - do a final check if there were any errors before performing the success scenario. Here is a rewrite of your code in a omre structured format. I did not test it, so there might be some minor syntax errors. <?php if ($_POST['submit']) { //form data $username = strip_tags ($_POST['username']); $email = strip_tags($_POST['email']); $pwd = strip_tags($_POST['pwd']); $confirmpwd = strip_tags($_POST['confirmpwd']); $date = date("Y-m-d"); $errors = array(); //check for required form data if(empty($username)) { $errors[] = "Username is required."; } elseif(strlen($username)>25 || strlen($username)<6) { $errors[] = "Username must be bewteen 6 and 25 characters."; } if(empty($pwd)) { $errors[] = "Password is required."; } elseif(empty($confirmpwd)) { $errors[] = "Password confirmation is required."; } elseif($pwd!=$confirmpwd) { $errors[] = "Your passwords do not match."; } elseif(strlen($pwd)>25 || strlen($pwd)<6) { $errors[] = "Password must be bewteen 6 and 25 characters."; } if(empty($email)) { $errors[] = "Email is required."; } if(count($errors)>0) { //There were errors echo "<p class='warning'>The following errors occured:<br>\n"; foreach ($errors as $error) { echo " - {$error}<br>\n"; } echo "</p>"; } else { //There were no errors, register the user $connect = mysql_connect("xxxxxxxx", "xxxxxxxx", "xxxxxxxx"); mysql_select_db("digital"); //select database $pwdSQL = md5($pwd); $usernameSQL = mysql_real_escape_string($username); $emailSQL = mysql_real_escape_string($email); $query = "INSERT INTO users VALUES ('','$usernameSQL', '$emailSQL', '$pwdSQL')"; $result = mysql_query($query); if(!$result) { echo "<p class='warning'>There was a problem saving your information.</p>"; } else { echo "<p class='success'>Thanks for signing up!</p>"; } } } ?>
-
Please explain what EXACTLY you are trying to accomplish? If you want ALL the records categorized by the key1/key2 values, then you should do just one SELECT query with an appropriate ORDER BY. Then you can display the results categorized by their key1/key2 values in the PHP logic. SELECT * FROM test ORDER BY keys1, keys2
-
The php manual (www.php.net) has great documentation and would do a better job than I of explaining this than I would. You should always look there first and then post here if you have questions. Here is the page for for loops http://us2.php.net/manual/en/control-structures.for.php But to explain it in my terms, the first expression is run when the loop is first encountered, The second expression determines when the loop should run - as long as the expression results in true the loop will execute. The third expression is executed on each iteration of the loop. In your example for ($num = 10; $num >= 10; $num--){ $num is first set to 10 and the loop will run as long as $num >= 10. Also, $num will be decremented by 1 on each iteration. So, the loop will run one time, then the value of $num will be decreased to 9 and will no longer run due to the condition in the 2nd expression. However, in the book's answer for ($num = 10; $num >= 1; $num--) There is the same initial value of 10, but the 2nd expression states the loop should run as long as $num >= 1, so on the first iteration the loop runs and the value is again reduced to 9, but since 9 >= 1 the loop will run again and again - until the value of num is NOT >= 1.
-
That looks pretty messy to use mysql_result() for each and every field. You should be using one of the mysql_fetch functions instead. In any event you need to add some debugging code to help find errors. Change $result = mysql_query($query); To $result = mysql_query($query) or die("Query: $query<br>Error: " . mysql_error()); echo "The query "$query" returned " . mysql_num_rows($result) . " records.<br />"; This will tell you if the query is running and, if so, how many records are being returned. As for your display code you REALLY need to stop using FONT tags - those were deprecated YEARS ago. Instead use a defined class name for the font characteristics. Also, your display code is creating invalid HTML. For instance you are creating "</html>" at the end of each record within the loop and you have non-table code within the table. Anyway, the code to display the content could look like this: while ($row = mysql_fetch_assoc($result)) { echo "<tr> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"lead_txt\">{$row['name']}</td>\n <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['email']}</td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['age']}</td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['gender']}</td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['location']}</td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['homephone']}</td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['otherphone']}</td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\"></td> <td align=\"center\" bgcolor=\"#ebf4fb\" class=\"fontClass\">{$row['referrer']}</td> <td bgcolor=\"#01337f\"> <a href=\"db_edit.php?id={$row['id']}\"> <img src=\"images/edit.png\" border=\"0\" width=\"25\" height=\"25\" alt=\"Edit\"> </a> <a href=\"db_remove.php?id={$row['id']}\"> <img src=\"images/delete.png\" border=\"0\" width=\"25\" height=\"25\" alt=\"Delete\"> </a> <a href=\"email_lead.php?id={$row['name']} {$row['email']}\"> <img src=\"images/email.png\" border=\"0\" width=\"25\" height=\"25\" alt=\"E-Mail\"> </a> </td> </tr>"; }
-
Then echo the data to the page to validate your hypothesis. And, as GuiltyGear stated - compare it as a number, not a string $amount = $_POST['amount']; //......... //Only query the field you need $query = mysql_query("SELECT `team_treasure` FROM teams WHERE team_id='$te' ") or die(mysql_error()); $result = mysql_fetch_assoc($query); //Add a debug line: echo "Result['team_treasure']:{$result['team_treasure']}, Amount:{$amount}<br>\n"; if($result['team_treasure'] <= '$amount') { echo "<br><br><div align='center'>Transfer Failed: Not enough credits</div> "; } else { echo "<br><br><div align='center'>Transfer Completed!</div>"; }
-
Supplied argument is not a valid MySQL result resource
Psycho replied to strago's topic in PHP Coding Help
You can't do that in one line. You have to RUN the query THEN get the results. In fact, it is best to create the query as a string, then run it, then get the results. That way, if there is a problem with the query you can echo the query to the page to identify any errors. I'm all for condensed/concise code, but this is not a good example. $query = "SELECT `urlname` FROM `workingurls` ORDER BY RAND() LIMIT 1"; $result = mysql_query() or die("Query: $query<br>Error: " . mysql_error()); $getURL = mysql_fetch_assoc($result); Also, ORDER BY RAND() is a bad solution. Google why and to get a better solution for getting a random value. -
You didn't show the code that displays the results. I *assumed* you were using the DB result contained in $result variable, but apparently you were running another query using the string from the $orderby variable. So, either the query is failing or you are referencing the wrong result variable. Either way you would have had an error on your page and you should have stated that instead of just saying "none of the data is being displayed". Add error handling to your query and ensure you are referencing the correct variable when you output the results
-
This is more efficient. function removeRef(&$value, $key, $searchAry) { $value = str_replace($searchAry, "", $value); } $searchFor = array('REF*19**', 'REF*GG*', 'PID*F*MAC***'); $lineArray = array('REF*19**PHILADELPHIA 02', 'REF*GG*CUT PILE', 'PID*F*MAC***Another name'); array_walk($lineArray, 'removeRef', $searchFor);
-
What you are asking about has nothing to do with download functionality. What you are looking for is a method to control functionality based upon permissions. There is no "name" for what you are asking - other than a permission based functionality. Step #1: is to implement a file download functionality. IMPORTANT: you want to store the files in a folder that is not web accessible. When the user requests the file you would read the file and output directly to the browser. Take a look at any download scripts and they *should* have that functionality. STEP #2: Implement a permission check in the download script. A) user requests file, B) Script checks user's permission c) If user has rights continue with the download otherwise display appropriate errors.
-
Did you LOOK at the HTML source that was produced? You need to write your code so it is readable. I see some problems with how the HTML would be produced, but I'm not going to try and figure out exactly what it is supposed to look like. But, start by making your code readable in the PHP code and in the HTML that is output to the browser. Then work from there. // Build the current users downline and add it to the temporary table $downline = mysql_query("select * from downline order by depth"); $depth = false; while($row = mysql_fetch_assoc($downline)) { if($row['depth'] !== $depth) { $depth_id = $row['depth']; $content .= "<tr id='main_lvl_$depth_id' class='hiddenInfo' onclick='showHide(this);'>\n"; $content .= "<td style='text-align: center;color:#4d53c1;font-weight: bold;' class='w_border'>\n"; $content .= "<span class='signs_s'>".$this->dict['mLevels_Level']." $depth_id : </span>\n"; $content .= "<span class='signs'>".count($down)." ".$this->dict['mLevels_Members']." </span>\n"; $content .= "</td>\n"; $content .= "</tr>\n"; $content .= "<tr id='lvl_$depth_id' style='display:none;'>\n"; $content .= "<td class='w_border' style='text-align: center;'><table style='width:100%;'>\n"; $i = 0; $content .= "<tr> <th>Member ID (Sponsor ID)</th> <th>Member Name</th> <th>Country</th> <th>Member Level</th> </tr>"; } $class = ($depth_row%2) ? 'color1NL' : 'color2NL'; $content .= "<tr id='_{$key}_' class='$class'>\n"; $content .= "<td class='width33'>\n"; $content .= "<a name='_".$row['member_id']."'></a>\n"; $content .= "<a href='?mid={$row['member_id']}'>#{$row['member_id']}</a> \n"; $content .= "(<a href='?mid=".$row['referrer_id']."'>#".$row['referrer_id']."</a>)\n"; $content .= "</td>\n"; $content .= "<td class='width33'>".$row['full_name']."</td>\n"; $content .= "<td>".$row['country']."</td>\n"; $content .= "<td class='width33'>".$row['level']."</td>\n"; $content .= "</tr>\n"; $depth_row++; if($row['depth'] !== $depth && $depth !== false) { $content .= "</table></td></tr>\n"; } $depth = $row['depth']; }
-
Give this a try function removeRef(&$value) { $value = preg_replace("#^REF\*+[^\*]+\*+#", "", $value); } $lineArray = array('REF*19**PHILADELPHIA 02', 'REF*GG*CUT PILE'); array_walk($lineArray, 'removeRef'); print_r($lineArray); Output: Array ( [0] => PHILADELPHIA 02 [1] => CUT PILE ) NOTE: This will remove the text at the beginning of the value if it matches the following criteria: 1. Begins with "REF" 2. followed by one or more asterisks 3. followed by one or more non asterisks 4. followed by one or more asterisks
-
Look at your PHP code - you are always RUNNING the default query. It seems you only create the variables for the other queries based upon the selected sort order - but you don't run them. Plus, your field name is "sort" and you are using $_GET['name'] and $_GET['age'] to test the submitted value. <?php session_start(); if (!isset($_SESSION['username']) || (trim($_SESSION['username'])=='')) { header("location: login.php"); exit(); } //Connect to Database $db_username = "removed_for_this_post"; $password = "removed_for_this_post"; $database = "removed_for_this_post"; mysql_connect("removed_for_this_post", $db_username ,$password); //Connection to Database @mysql_select_db($database) or die("ALERT! Database not found!"); //Selection of Database //Determine sort field switch(strtolower($_GET['sort'])) { case 'name': case 'age': $sort_field = strtolower($_GET['sort']); break; default: $sort_field = 'id'; } //Create and run query $query="SELECT * FROM leads ORDER by {$sort_field} DESC"; $result = mysql_query($query); $num = mysql_numrows($result); //Display results mysql_close(); ?>
-
So, if I understand you correctly, the code you have will display the content correctly but the problem you are having is that the script is timing out because of the volume of records? So, what EXACTLY is your request? If you are trying to prevent the time-out there is only so much you can do to make the code more efficient. But, I think a more important question is what is the value to the user to see 15K+ records on a single page? It would make more sense to me to show only a few levels deep on a page and showing links to display some of the deeper levels. As far as your currently code, one idea to make it more efficient would be to set some of the static text as variables. You are adding a lot of text to the $content variable which is the same. Make that text a variable and add it to the $content variable so the PHP parser doesn't have to interpret the text. I'm not 100% sure that would be more efficient, but it's worth testing. Also, you seem to have a lot of nested tables. That is a killer for the web browser to process. You should consider changing how you compile the HTML.
-
I think the problem you are experiencing is that fgets() will get a line from the file and that line already has a line break on the end. In the IF condition you are rewriting the line and including a line break on the end. But, in the ELSE condition you are appending a line break to the original line (which already had a line break). However, the problem is further exacerbated by the fact that fputs() also put in a line break - but you won't see it in a plain text editor. If you were to open the file using Word or Wordpad you would see the extra line breaks. To be honest, I don't really get it - but I think it boils down to a difference on how Linux/Windows define line breaks. I've run into this problem in other instances and had to fight through to resolve it. But the solution to your problem is just to write better code. 1. It is inefficient to write one line at a time. Instead, generate all the content for the new file THEN, when complete, replace the original file. You don't need a temp file at all. Just use a temp string. 2. The ELSE condition is not necessary. If the IF condition is true then redefine $aline. Then you only need one line to handle the disposition of the content. 3. Remove the "internal" line breaks using trim() then add your line breaks. Personally, I would use file() to break the content into an array, redefine the values that need to be redefined, then implode the result and overwrite the file. But, using your method, I would rewrite as follows: ##-- Loop thruoght the ORIGINAL file while( ! feof($old)) { ##-- Get a line of text $aline = trim(fgets($old)); ##-- We only need to check for "=" if(strpos($aline, "=") > 0 ) { $aline = "{$info[$i]} = {$rslt[$i]}"; $i++; } $new .= $aline . PHP_EOL; }//while eof(old) //Close file handle for reading fclose($old); //Reopen file in write mode $old = @fopen($file, "w"); fputs($old, $new); fclose($old);
-
YOU DO NOT NEED SEPARATE QUERIES. YOU ONLY NEED ONE QUERY. Run this code and see what you get $query = "SELECT `status`, COUNT(`status`) as `count` FROM ".constant("TBL_USER_REFERRALS")." WHERE `referrer_uid` = '$referrer_uid' GROUP BY `status`"); $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo "{$row['status']}: {$row['count']}<br>\n"; }
-
I provided code, you then told me the code I provided was reporting the wrong value, but then it turns out you were still using your original code. If you are going to ask for help at least try the help that is offered.
-
The code I provided doesn't print anything to the page. Show the code you used.
-
Not tested, but should give you an idea on approach //Convert array of new emails so original value is key and escaped value is value $newEmailsAry = array_fill_keys($newEmailsAry, $newEmailsAry); foreach($newEmailsAry as $key => $value) { $newEmailsAry[$key] = "'" . mysql_real_escape_string($value) "'"; } //Query to find existing records $query = "SELECT email FROM table WHERE email IN (" . implode(", ", $newEmailsAry) . ")"; $result = mysql_query($query); //Create array of existing values $existingEmails = array(); while($row = mysql_fetch_assoc($result)) { $existingEmailsAry[] = $row['email']; } //Remove existing values from user entered array $newEmailsAry = array_diff($newEmailsAry, $existingEmailsAry); //Create query to insert only new emails $query = "INSERT INTO table (`email`) VALUES (" . implode("), (", $newEmailsAry) . ")"; $result = mysql_query($query); //Ouput results echo "The following emails already existed in the db<br>\n"; echo "<ul>\n"; foreach($existingEmailsAry as $email) { echo "<li>{$email}</li>\n"; } echo "</ul>\n"; echo "The following emails were added to the db<br>\n"; echo "<ul>\n"; foreach($newEmailsAry as $email) { echo "<li>{$email}</li>\n"; } echo "</ul>\n";