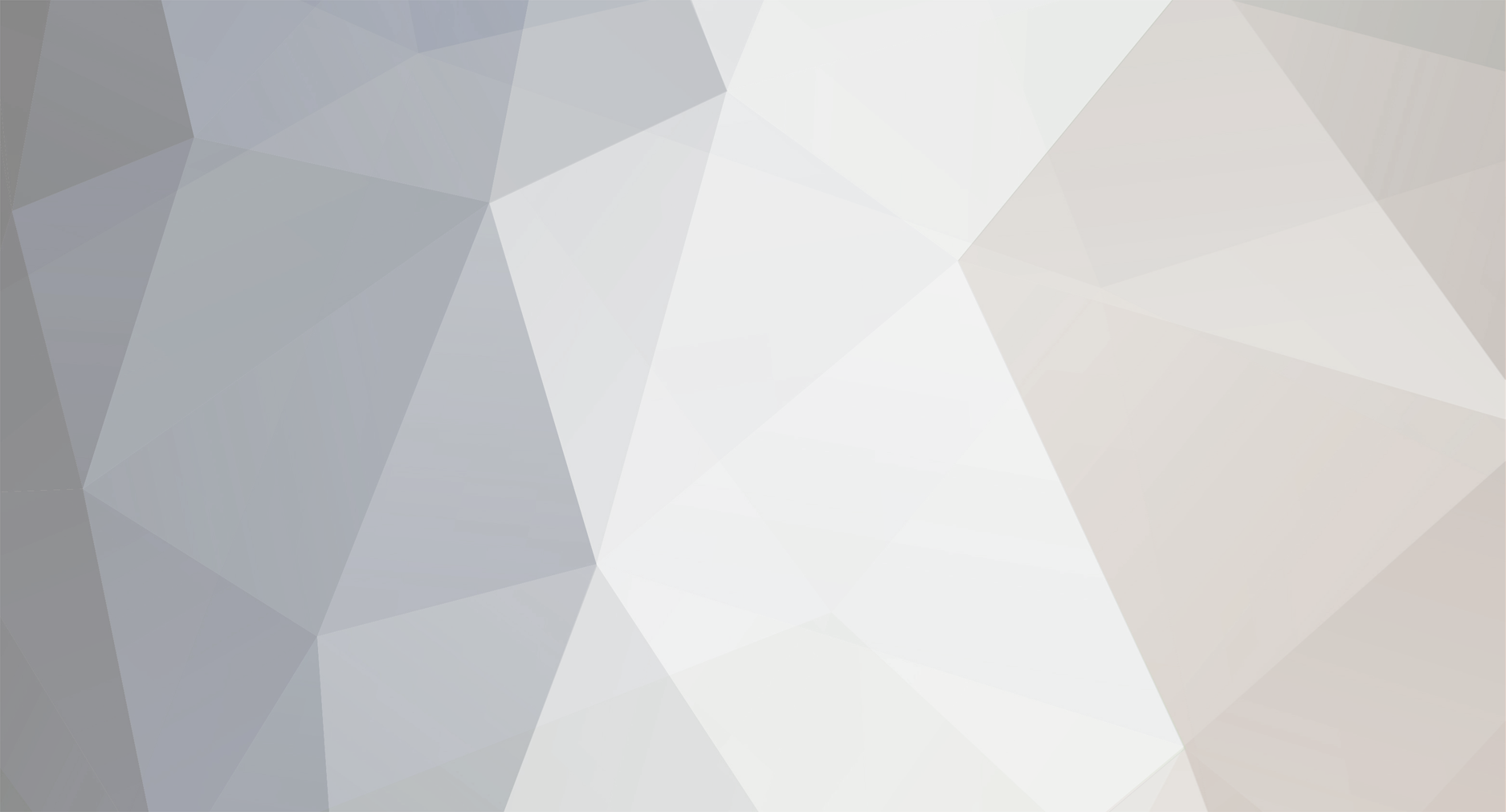
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
More like this $query = "SELECT IF(`premium`>=NOW(), 1, 0) as premium FROM $tbl_name WHERE myusername='$myusername'"; $result = mysql_query($query); $row = mysql_fetch_array($result); if (!$row['premium']) { echo 'Standard <a href="upgrade.php">'; echo 'Upgrade'; echo '</a>'; echo '</td>'; } else ($premium > date(m/d/y) { echo 'Premium'; echo '</td>'; }
-
Well, I just tested it several different ways and it worked for me. SELECT * FROM `scan_jobs` WHERE MONTH(`started`) = 3 SELECT * FROM `scan_jobs` WHERE MONTH(`started`) = '03' SELECT * FROM `scan_jobs` WHERE MONTH(`started`) = "03" The problem must be in your data. Is the field set as a date field and did you validate that the values you expect for those records is correct? if you have a page where you are entering the records you might have a bug that is not saving the dates correctly. EDIT: Scratch that You still need to run a mysql_fetch for EACH record. The first one will only get the first record!!! $sql= $dbc->dbRunSql("select * from points WHERE MONTH(date) = '03' " ); while($record = mysql_fetch_assoc($sql)) { print_r($record); }
-
Well, MONTH() will return the numeric value of the data. So, March would be 3 not "03". Try that. And,. why do you have a month field in the database?
-
So date("m/d/y") + 30 ? There is an easier way. When creating/updating the user's record to set their expiration date you could use something like the following UPDATE users SET expiration = DATE_ADD(NOW(), INTERVAL 30 DAY) WHERE user_id = $user_id Now there is no need to update the expiration value each day for all users. When the user accesses the site, determine if the expiration date is after the current date. If so, the user is a premium member. If not, they are a standard member.
-
Where is your code to display the results. The problem may be in that code. Also, do you really want to show all records from a specific month or do you really want to show the records from a specific MONTH & YEAR? That query above would get records from March of any year.
-
I concur with ignace. To add a little more clarity here are some examples of table structures you could use (fk) = foreign key. Table: Departments Fields: - dept_id - dept_name Table: members - member_id - dept_id (fk) - member_name - member_title - department_head (boolean) Table: hardware - hardware_id - dept_id (fk) - member_id (fk) - asset_tag - hardware_description - cpu - memory - os Table: software - software_id - hardware_id (fk) - dept_id (fk) - software_description Note: for something like software it may make sense to have a separate table to list out the individual software titles and link them using a foreign key to the software table. This ensures you can get a list of all the people/machines running "Office 2010" for example.
-
PHP Post is returning single quote character
Psycho replied to zander1983's topic in PHP Coding Help
The magic_quotes_gpc setting is enabled on your server. If you have access to the php.ini file you can turn this off. If you are on a shared server you will not be able to do this. Depending on your situation it is probably better to "cleanse" your user input in a server independent manner - i.e. using a process that will work no matter what the server setting is. there is an example process in the PHP manual for this - along with other useful information on the subject. http://www.php.net/manual/en/function.get-magic-quotes-gpc.php -
Really? If I was a user of your site and you were using my email as a link on the website, I would hunt you down and slash all your tires. Doing so means that spam-bots will pick up those email addresses and put your users on every spam list available. But, since you ask: <td><span class="style10"><a href="mailto:<?php echo $rows['user_login']; ?>"><?php echo $rows['user_login']; ?></a></span></td>
-
Here is a rewrite of your code which fixes some other problems and adds better error handling. Let me know if you have any questions <?php //Parse user input $title = trim(strip_tags($_POST['title'])); $firstname = trim(strip_tags($_POST['firstname'])); $surname = trim(strip_tags($_POST['surname'])); $email = trim(strip_tags($_POST['email'])); $reemail = trim(strip_tags($_POST['reemail'])); $password = $_POST['password']; //Don't need to trim or remove tags $repassword = $_POST['repassword']; $street = trim(strip_tags($_POST['street'])); $city = trim(strip_tags($_POST['city'])); $postcode = trim(strip_tags($_POST['postocde'])); $telephone = trim(strip_tags($_POST['telephone'])); //Check for errors $errors = array(); if(empty($firstname)) { $errors[] = "First name is required."; } if(empty($surname)) { $errors[] = "Surname is required."; } if(empty($email)) { $errors[] = "Email is required."; } if(empty($reemail)) { $errors[] = "Email confirmation is required."; } if(!empty($email) && !empty($reemail)) { if($email!=$reemail) { $errors[] = "Your Emails do not match."; } else { $emailSQL = mysql_real_escape_string($email); $result = mysql_query("SELECT * FROM member WHERE email='$emailSQL'"); if(mysql_num_rows($result)) { $errors[] = "This email is already registered in our database"; } } } if(empty($password)) { $errors[] = "Password is required."; } if(empty($repassword)) { $errors[] = "Password confirmation is required."; } if(!empty($password) && !empty($repassword) && $password!=$repassword) { $errors[] = "Your Passwords do not match."; } if(empty($street)) { $errors[] = "Street is required."; } if(empty($city)) { $errors[] = "City is required."; } if(empty($postcode)) { $errors[] = "Post code is required."; } if(empty($telephone)) { $errors[] = "Telephone is required."; } //Determine if errors occured if(count($errors)>0) { //There were errors - display them echo "The following errors occured:<br />\n"; echo "<ul>\n"; foreach($errors as $error) { echo "<li>{$error}</li>\n"; } echo "</ul>\n"; } else { //No errors occured - insert records $query = sprint_f("INSERT INTO members (title, Firstname, Surname, Street ,City, Postcode, EmailAddress, Password, TelephoneNo, Credit) VALUES ('%s', '%s', '%s', '%s', '%s', '%s', '%s', '%s', '%s', '%d')", mysql_real_escape_string($title), mysql_real_escape_string($firstname), mysql_real_escape_string($surname), mysql_real_escape_string($street), mysql_real_escape_string($city), mysql_real_escape_string($postcode), $emailSQL, md5($password), mysql_real_escape_string($telephone), 0); $submitusers= mysql_query($query); } ?>
-
If you added some better error handling to your code (e.g. being able to tell the user "what" fields were empty) it would have been easy to find this error yourself $postcode=strip_tags($_POST['postocde']); Note the name of the POST value.
-
To think that you you tested your queries for every eventuality is ridiculous. I have worked for multiple software companies and I have seen multiple instances where a query passed all initial tests and then some time int he future it fails because of a particular set of circumstances. Or, there is the situation where someone modifies some other code or the database such that previously existing queries fail. It doesn't take a great deal of effort to create a bit of error handling code to make your application a solid product. Typically I create a custom function for running queries to use in place of mysql_query(). That way I can specify different error handling based upon whether the application is in debug mode or production mode. Then you don't have to write error handling for each and every query. I don't see where you used mysql_real_escape_string() on the user input (i.e. "id"). I only see you using it on the message value which you obtained from a previous db query. That is a good idea since it may contain characters that would foul the query, but if "message" contained malicious code it would have been run when the record was inserted. Although I didn't specify this before, you are also not escaping the code you print to the page. echo "<hr>". $e_rep['by'] ." says: ". $e_rep['message'] ."<hr>"; That could lead to a user unintentionally breaking your page if they inserted code that could be interpreted as HTML. Or worse, it opens you up to XSS attacks.
-
OK, if you are using that link above to ACCESS the form, then that value will not be available to the script that processes the form since the form will post to the URL in the "action" parameter of the form tag. If you are already saving the value into a hidden field I still think you should go ahead and access the field via the POST array. You say that the value is not available in the POST data AND the year is resolving to 2007. For the first part you should do a print_r($_POST) on the processing page to validate exactly what is being passed int he post data. As for the year resolving to 2007 - that makes no sense. The code you provide above will determine the current year, regardless of what values are, or are not passed. There must be something else going on that you are not aware of. You may be POSTing to the wrong page, there are IF/ELSE conditions that are causing the code to evaluate differently than you expect, something.
-
I think this is the first post on hashing that didn't get out of hand with a bunch of idiotic responses. There are really two separate issues discussed above. I'll add my 2 cents: First of all it is important to know that hashes are not encryption. They are NOT meant to and cannot be unencrypted (at least not in a real sense). The "collision" problem that was referred to really has no relevancy with passwords. Collisions with hashing IS an issue though. Collisions refers to the frequency of when two separate inputs could result in the same hashed value. Since hashes aren't meant to be decrypted to the original input value it may not seem like a big deal. But, you have probably seen sites that allow you to download executables, pdfs etc. and include a hashed value for the download. That is so you can run a hash on the file after you download it to ensure it matches the one the site provides. This is typically used when a provider allows 3rd party sites host the downloads to ensure one of those third party sites isn't compromised. With a large enough input hashed with MD5 it is possible to create a rogue file that has the same characteristics as the original and will result in the same MD5 hash. But, that is not a problem with passwords. If it was a user could enter the wrong password and still be authenticated. Passwords are finite values that have a limited character set and a limited count of characters. Although not proven (that I am aware) I would bet there are no combinations of strings from 1-32 characters that would generate the same MD5 hash. So, the reason we hash passwords - as stated above - is that if the user database was compromised we don't want a malicious users to know what the passwords are. Many people use the same usernames/passwords on multiple sites - which is a bad, bad idea. If a user's password is compromised on one site a malicious user could try that username/password on multiple other sites (e.g. banking sites) until they found a match. Now, the fact that MD5 has an issue with collisions is irrelevant when hashing passwords. A hacker isn't trying to find a value that will result in the same MD5 hash, they are trying to ascertain the input value. But, as stated above a hash cannot be unhashed, right? That is true, but the same input will always results in the same hashed value. Plus, may users - stupidly - use common words for their password (e.g. "password"). For this, hackers use a dictionary/rainbow table where they has common words/phrases and store the original and hashed values. If they have a hashed value and compare it to their table it could return the original value IF they had included that value in the values they had hashed. That is why users should use strong passwords (upper/lower case letters, numbers, and special characters). To generate a table with all possible values up to the limit of most passwords would take many, many years. If you add a salt - especially if the salt is unique for each user - it is far more difficult. Even if the hacker has the hashed value and the salt, they would have to regenerate all the values in their table using the salt. - - - - - - - - By the way, for the salt I would propose something other than just appending a word as cssfreakie suggested. Use some random text as you apparently intended. Otherwise you could end up with an input value that is two words put together. It would still be "pretty good" but after creating hashes of single words the next thing they would add would be two words (or 1337 text). You could also do somethign such as reversing the letters.
-
I'm not following you. If you have the month in the URL, then why do you have the month in a hidden field. Use one or the other. Regarding the month in the URL is that the URL you use to access the page with the form (i,e. button)? If so, when you POST the form that value is not getting included as part of the url. I suspect you are using the month on the URL to populate the hidden field in the form. I would just use the $_POST['month'] value.
-
Here are my suggestions: 1. Never create your queries directly in the mysql_query() function. Create them as a variable and use the variable in the mysql_query() function. If you ever have a problem you will be able to quickly debug it be echoing out the query. Or even better you can log the error and the query. That way if a situation ever occurs in production that was accounted for in testing you would be able to trace teh problem 2. Be consistent with your indentations. You do a fairly good job, but there inconsistencies 3. Comment, comment, comment. There's nothing worse than spending a couple hours to come up with some really elegant code to handle a complex scenario. Then coming back to that code weeks/months later to do a bug fix or enhancement and spending another two hours to understand the code you wrote. 4. This code switch ($extract['status']) { case 0: $status = "Unresolved."; break; case 1: $status = "Locked."; break; case 2: $status = "Solved."; break; } Could have been simplified to this: $statuses = array("Unresolved.", "Locked.", "Solved."); $status = $statuses[$extract['status']]; 5. Error handling. You have absolutely no error handling for your queries. 6. Escaping/cleansing user input!!! You are using data sent by the user directly in your database queries. That is a HUGE security risk. 7. This if(!$_SESSION['admin']) { mysql_query("INSERT INTO replies VALUES (null, '$message', 'Guest', '$id')"); } else { mysql_query("INSERT INTO replies VALUES (null, '$message', 'Support Team Member', '$id')"); } Could have been simplified to this $user = (!$_SESSION['admin']) ? 'Guest' : 'Support Team Member'; mysql_query("INSERT INTO replies VALUES (null, '$message', '$user', '$id')");
-
If you want to use txt files you could very easily run into performance issue. On every page load you would have to read the entire txt file to be able to determine the number of pages and to get the content for the current page. And, you could use a database and build an interface for users to modify their own data. If I get some time this afternoon, I'll throw some code together for you to get started with what you have.
-
Well, you need a variable for the current page. Assuming the value is stored in the variable $current_page you would do something like below. Note I change $i to $page - always give your variables descriptive names. $page =1; for ($x=0;$x<$foundnum;$x=$x+$e) { $style = ($page = $current_page) ? 'font-weight:bold;' : ''; echo " <a href='search.php?search=$search&s=$x' style='{$style}'>$page</a> "; $page++; Also, what is the purpose of $x and $e in your code? I would just use a for() loop where the $page variable is incremented as needed.
-
I really have no idea what you are talking about with regard to the two fields. When you have a problem with a query the FIRST thing you should do is look at the entire query. So, just echo $Adds to the page to see what you have. Also, when you are needing to create a query - especially a complex one- don't do it by creating/executing it in a PHP page. Instead start by running the query in the database. In the case of MySQL this is usually done through phpmyadmin (yes, I know that is a PHP page, but not the same as building your own). Once you have validated that the query is generating the results you expect - THEN you move on to creating the query in PHP and making it dynamic. Having said all that, the error was on my part! In the join statement where you specify the "table.field" values, each should be enclosed in backticks not the entire value. WRONG RIGHT
-
Seriously?! Based upon your first post above I "thought" you actually did some coding on the site yourself. I can possibly understand the question about the LEFT JOIN, but you really don't have any idea how you would make the topics bold? Anyway, in a normal JOIN the records from the first table (the left) are joined to the 2nd table (the right) ONLY if there are records to join. That may not make sense as written so let's go over a couple examples. Let's say you have two tables: authors and books. Naturally, the books will be linked to the authors using an author ID. So, if you wanted a list of all the authors and their books you could run the following query SELECT * FROM authors JOIN books ON books.author_id = authors.id But, if there are any authros in the database which don't currently have any books associated with them they would be excluded from the result set because there were no records in the books table to JOIN on them. The solution is a LEFT JOIN which will join all the authors (LEFT) and the associated records from the books (RIGHT) table - but if there are no books to join on a respective author it will still be included in the result set, but the fields for the book data will be NULL. There are many differenent kinds of JOIN and there are plenty of tutorials out there that will do a better job of explaining them than I could. As for making the unread records bold I hope you know the basics on processing a result set from a DB query to display a list. In the while() loop to display the list of articles check the value of the "viewed" field inthe result set (which we dynamically created). If the value is 0 then the user hasn't read the article and you should make it bold. Rough example while($row = mysql_fetch_assoc($result)) { //Create the style for the article link $style = ($row['viewed']==0) ? 'font-weight:bold;' : ''; echo "<a href=\"displayArticle.php?id={$row['id']}\" style=\"{$style}\">$row['title']</a><br />\n"; }
-
I thought I already explained that. I'm not going to write the code for you as it would be too involved for a forum post and requires a knowledge of your database schema and architecture. But, here is a more verbose explanation: 1. Create a table to store viewed topics by users (e.g. "viewed_topics". You need two fields: topic_id and user_id. You also need to set both to unique. 2. On the page that displays a topic, in addition to running a SELECT query to get the topic data to display to the user, you need to run an INSERT query to update the "viewed_topics" table with the topic_id and user_id. However, you don't want duplicates, so you could use an ON DUPLICATE KEY UPDATE clause in the query. Example: $query = "INSERT INTO viewed_topics (`topic_id`, `user_id`) VALUES ($topic_id, $user_id) ON DUPLICATE KEY UPDATE `user_id`=`user_id`"; 3. When you pull the list of topics you will join the "viewed_topics" table so you can determine which ones the user has viewed. You would do this with a LEFT JOIN. But, as part of the JOIN condition you would only join those records belonging to the current user. I would put an IF condition in the SELECT statement to generate a dynamic value in the result set for viewed (true/false). You would do this by checking the user_id value from the joined records - if there was a matching record it will equal the user_id. Otherwise, that value would be NULL. Here is an example query $query = "SELECT *.topics, IF(`viewed_topics.user_id`=$user_id, 1, 0) as viewed FROM `topics` LEFT JOIN `viewed_topics` ON `topics.id` = `viewed_topics.topic_id` AND `viewed_topics.user_id` = $user_id";
-
That's a very simple query - don't let the sprintf() scare you. All it does is populate the values (i.e. the $User variables) into the first parameter (i.e. the query). Just add your JOIN right after the FROM as you normally would - along with the appropriate field name to the SELECT clause. By the way you have `cat` listed twice in your select clause. I guessed on the table/field names. $Adds = sprintf("SELECT `name`, `cat`, `area`, `county`, `thumbsup`, `thumbsdown`, `logo`, `members` `cat_name` FROM `clubs` JOIN `categories` ON `clubs.cat` = `categories.cat_id` WHERE `cat` IN('%s', '%s', '%s', '%s', '%s') AND `area` = '%s' OR `county` = '%s' ORDER BY rand() LIMIT 6", $User['fav_1'],$User['fav_2'],$User['fav_3'],$User['fav_4'],$User['fav_5'], $User['area'], $User['county']);
-
Hmm, the example you posted appears to be MySQL, but for PHP usage it is used to suppress error reporting. For example, if your script tries to read a file and the file doesn't exist you wouldn't want to display some ugly PHP error. Instead you would attempt to read the file (suppressing the error if there is one), then display a friendly error to the user: More info in the manual: PHP: Error Control Operators: http://php.net/manual/en/language.operators.errorcontrol.php
-
Use "ORDER BY" on whatever field you want them ordered by using "DESC" to make it in descending order You are going to have to track what topics each user views. So, when a user selects an article, the page that displays the article will do a database insert to save a record identifying the user and the article. Then when pulling articles to display you would need to JOIN the articles table with the "viewed" table to determine which onse are read or not.
-
Your current code for the form page is problematic. You are wanting to allow the user to update ALL the records in one page. That is possible, but it is problematic. The reason is that you can't "know" which values have been updated and which ones haven't, so you would have to update ALL the records whenever that page is submitted. Plus, right now the code you have doesn't format the field names as arrays with an ID reference to each record. So, you would only be updating the last record (when there are input fields with the same name only the last one is sent in the POST data - unless it is configured as an array). A better approach is to provide a page where all the records are listed with a link for each record to go to an edit page. The link will pass the id of the record. Then you want to query the database for the values associated with that record then populate the form fields as appropriate. So, first change your current form page to simply display the data and for each record add an edit link that looks something like this echo "<a href=\"edit.php?id={$_row['id']}\">Edit</a>"; Then create an edit.php page where you query the data for the passed ID and create the form using the db results to populate the values.
-
Here is a complete rewrite of that code which, hopefully is more readable. It definitely has improved logic. For example, the previous code was always running the queries to get past/future games even if $showpast or $shownextgame were false. If they were false the code didn't use the queries data. If you are not going to use the data - don't query it. I'm also sure there are some syntax errors since I couldn't test it without an appropriate database. <?php /** * @version $Id: mod_gridiron_game_results.php, v1.5.0 March 2011 01:32:15 * @author Fastball Productions * @package Gridiron * @copyright Copyright (C) 2011 Fastball Productions * @license http://www.gnu.org/licenses/gpl-2.0.html GNU/GPL */ // no direct access defined('_JEXEC') or die('Restricted access'); $db =& JFactory::getDBO(); // get the module parameters $heading = $params->get( 'heading', '' ); $showpast = $params->get( 'showpast', '1' ); $numberpast = $params->get( 'numberpast', '1' ); $linkboxscore = $params->get( 'linkboxscore', '1' ); $shownextgame = $params->get( 'nextgame', '1' ); $numbernext = $params->get( 'numbernext', '1' ); $linknext = $params->get( 'linknext', '1' ); $seasonid = $params->get( 'seasonid', '1' ); $gametypes = $params->get( 'gametypes', '1' ); $teamids = $params->get( 'teamids', '' ); $leagueids = $params->get( 'leagueids', '' ); $divisionids = $params->get( 'divisionids', '' ); if (is_array($gametypes)) { $gametypes = implode(',', $gametypes); } if (is_array($teamids)) { $teamids = implode(',', $teamids); } if (is_array($leagueids)) { $leagueids = implode(',', $leagueids); } if (is_array($divisionids)) { $divisionids = implode(',', $divisionids); } // if there is a league configured, get the teams within the league/division; if ($teamids == '') { if ($divisionids) { // get a listing of all team ID's that belong in the league and division; $sql = "SELECT id FROM #__gridiron_team WHERE (FIND_IN_SET(divisionid, '$divisionids'))"; } elseif ($leagueids) { // get a listing of all team ID's that belong in the league; $sql = "SELECT id FROM #__gridiron_team WHERE (FIND_IN_SET(leagueid, '$leagueids'))"; } else { // get the default team (single team component only); $sql = "SELECT id FROM #__gridiron_team WHERE defaultteam = 1"; } $db->setQuery($sql); $teamids = implode(',', $db->loadResultArray()); } //Generate HTML for past games $pastGamesHTML = ''; if ($showpast && $numberpast > 0) { // get the last x number of games played and the results; $query = "SELECT a.*, a.hometeam AS hometeamid, a.visitingteam AS visitingteamid, DATE_FORMAT(a.gamedatetime, '%a, %M %D') AS gamedate, DATE_FORMAT(a.gamedatetime, '%l:%i %p') As gametime, h.name AS hometeam, v.name AS visitingteam, b.finalv, b.finalh FROM #__gridiron_schedule AS a LEFT JOIN #__gridiron_team AS h ON a.hometeam = h.id LEFT JOIN #__gridiron_team AS v ON a.visitingteam = v.id LEFT JOIN #__gridiron_boxscore AS b ON a.id = b.gameid WHERE (a.scored = 1 AND a.season = {$seasonid} AND (FIND_IN_SET(a.gametype, '$gametypes')) AND (FIND_IN_SET(a.hometeam, '$teamids') OR FIND_IN_SET(a.visitingteam, '$teamids')) AND a.gamedatetime < now()) GROUP BY a.id ORDER BY a.gamedatetime DESC LIMIT 0, {$numberpast}" $db->setQuery($query); $pastgames = $db->loadObjectList(); $pastGamesHTML .= " <tr>\n"; $pastGamesHTML .= " <td colspan='2' style='text-align:center;'><b>{$heading}</b></td>\n"; $pastGamesHTML .= " </tr>\n"; $pastGamesHTML .= " <tr>\n"; $pastGamesHTML .= " <td width='43%'><b><u>Matchup</u></b></td>\n"; $pastGamesHTML .= " <td width='57%' style='text-align:center;'><b><u>Result</u></b></td>\n"; $pastGamesHTML .= " </tr>\n"; foreach ($pastgames as $past) { $final = "{$past->finalv}<br />{$past->finalh}"; if($linkboxscore) { $href = JRoute::_("index.php?option=com_gridiron&view=boxscore&id=$past->id"); $final = " <a href='{$href}'>$final</a>"; } $pastGamesHTML .= " <tr>\n"; $pastGamesHTML .= " <td>{$past->visitingteam;} vs.<br />{$past->hometeam}</td>\n"; $pastGamesHTML .= " <td style='text-align:center;'>\n"; $pastGamesHTML .= " <td>{$final}</td>"; $pastGamesHTML .= " </tr>\n"; } //Add Separator $pastGamesHTML .= " <tr>\n"; $pastGamesHTML .= " <td colspan='2'></td>\n"; $pastGamesHTML .= " </tr>\n"; } //Generate HTML for past games $nextGamesHTML = ''; if ($shownextgame && $numbernext > 0 && $nextgames) { // get the next x number of games scheduled; $query = "SELECT a.*, a.hometeam AS hometeamid, a.visitingteam AS visitingteamid, DATE_FORMAT(a.gamedatetime, '%a, %M %D') AS gamedate, DATE_FORMAT(a.gamedatetime, '%l:%i %p') As gametime, h.name AS hometeam, v.name AS visitingteam, t.description AS gametype FROM #__gridiron_schedule AS a LEFT JOIN #__gridiron_team AS h ON a.hometeam = h.id LEFT JOIN #__gridiron_team AS v ON a.visitingteam = v.id LEFT JOIN #__gridiron_gametype AS t ON a.gametype = t.id LEFT JOIN #__gridiron_location AS l ON a.location = l.id WHERE (a.scored = 0 AND a.season = {$seasonid} AND (FIND_IN_SET(a.gametype, '$gametypes')) AND (FIND_IN_SET(a.hometeam, '$teamids') OR FIND_IN_SET(a.visitingteam, '$teamids')) AND DATE_ADD(a.gamedatetime, INTERVAL 3 HOUR) > now()) GROUP BY a.id ORDER BY a.gamedatetime ASC LIMIT 0, {$numbernext}" $db->setQuery($query); $nextgames = $db->loadObjectList(); $nextGamesHTML = " <tr>\n"; $nextGamesHTML = " <td colspan='2'><b><u>Next Game</u></b></td>\n"; $nextGamesHTML = " </tr>\n"; foreach ($nextgames as $next) { //Define the visiting team name if($next->visitingteam=='') { $next->visitingteam = 'TBA'; } elseif($linknext) { $href = JRoute::_("index.php?option=com_gridiron&view=schedule&id=$next->visitingteamid"); $next->visitingteam = "<a href='{$href}'>{$next->visitingteam}</a>"; } //Define the home team name if($next->hometeam=='') { $next->hometeam = 'TBA'; } elseif($linknext) { $href = JRoute::_("index.php?option=com_gridiron&view=schedule&id=$next->hometeamid"); $next->hometeam = "<a href='{$href}'>{$next->hometeam}</a>"; } //Create html $nextGamesHTML = " <tr>\n"; $nextGamesHTML = " <td colspan='2'>{$visitingTeam}</td>\n"; $nextGamesHTML = " <td>{$next->visitingteam} vs. {$next->hometeam}</td>\n"; $nextGamesHTML = " </tr>\n"; $nextGamesHTML = " <tr>\n"; $nextGamesHTML = " <td colspan='2'>{$next->gamedate} {$next->gametime}</td>\n"; $nextGamesHTML = " </tr>\n"; } } ?> <table width="100%" border="0" align="center"> <?php echo $pastGamesHTML; ?> <?php echo $nextGamesHTML; ?> </table>