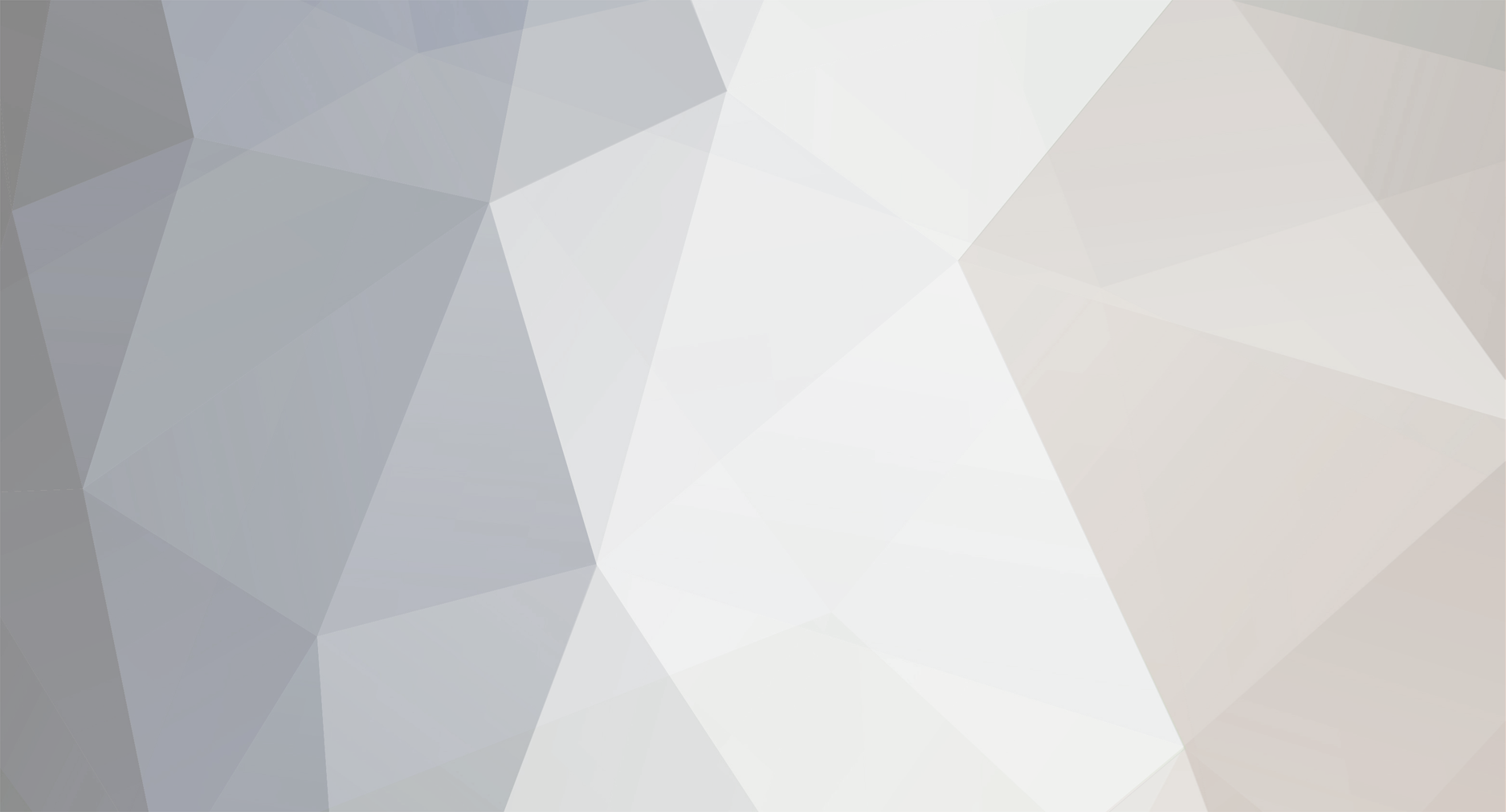
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
The code I provided doesn't print anything to the page. Show the code you used.
-
Not tested, but should give you an idea on approach //Convert array of new emails so original value is key and escaped value is value $newEmailsAry = array_fill_keys($newEmailsAry, $newEmailsAry); foreach($newEmailsAry as $key => $value) { $newEmailsAry[$key] = "'" . mysql_real_escape_string($value) "'"; } //Query to find existing records $query = "SELECT email FROM table WHERE email IN (" . implode(", ", $newEmailsAry) . ")"; $result = mysql_query($query); //Create array of existing values $existingEmails = array(); while($row = mysql_fetch_assoc($result)) { $existingEmailsAry[] = $row['email']; } //Remove existing values from user entered array $newEmailsAry = array_diff($newEmailsAry, $existingEmailsAry); //Create query to insert only new emails $query = "INSERT INTO table (`email`) VALUES (" . implode("), (", $newEmailsAry) . ")"; $result = mysql_query($query); //Ouput results echo "The following emails already existed in the db<br>\n"; echo "<ul>\n"; foreach($existingEmailsAry as $email) { echo "<li>{$email}</li>\n"; } echo "</ul>\n"; echo "The following emails were added to the db<br>\n"; echo "<ul>\n"; foreach($newEmailsAry as $email) { echo "<li>{$email}</li>\n"; } echo "</ul>\n";
-
I think you are making this more difficult than it needs to be. A simple COUNT and GROUP BY should be all you need. Try this: $query = "SELECT `status`, COUNT(`status`) as `count` FROM ".constant("TBL_USER_REFERRALS")." WHERE `referrer_uid` = '$referrer_uid' GROUP BY `status`"); $result = mysql_query($query); //Put results into array for later use $statusCounts = array(); while($row = mysql_fetch_assoc($result)) { $statusCounts[$row['status']] = $row['count']; } You should have an array structure something like this: array( 'completed' => 12, 'declined' => 14, 'referrred' => 5 )
-
Try $query = "SELECT * FROM items WHERE title LIKE '%{$search_term_esc}%' AND (price = 0 OR (price >= {$min} AND price <= {$max}))";
-
The same way you output anything to the browser with PHP, echo ,print, etc. I only provided some sample code because you failed to show any of the relevant code for me to provide a modification.
-
OK, the code you posted doesn't show anything about how the user selects an amount or how you would determine the value to populate in the text field. Here is a quick example script <html> <head> <script type="text/JavaScript"> function updateField(fieldID, newValue) { var fieldObj = document.getElementById(fieldID); fieldObj.value = newValue; } </script> </head> <body> Amount: <select name="amount" id="amount" onchange="updateField('amount2', this.options[this.selectedIndex].value);"> <option value=""></option> <option value="0">0</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> </select> <br> Amount2: <input type="text" name="amount2" id="amount2" /> </body> </html>
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=330523.0
-
So, why post in the PHP forum? Moving to JS forum.
-
Depends on what is saved when the value is empty. Are you leaving the field as NULL, is it 0, an empty string? Will also depend on the field type in the database. Try the following: $query = "SELECT * FROM items WHERE price <> '' AND price >= {$min} AND price <= {$max} AND title LIKE '%{$search_term_esc}%'";
-
I see several "potential" problems. But, since I can't see into your application/database I am only guessing. 1. The code above does not remove the £ from the price or ensure it is a number. So, trying to use a > or < comparison in the query will not work as you intend. You will need to parse the value from the regex return value to ensure it is a numeric value before storing in the database. 2. Your LIKE values for 'title' have spaces in them and you are using the same value in each of them. You only need to do a LIKE against the value one time - properly formatted. 3. Why do you have a $max value if you aren't using it? 4. You have to be careful with using mutiple AND's and OR's. They will not be interpreted the way you intend if you do not put them in the right order or inclose sections of them in parens. In the situation you have above records would be returned if they matched only the last condition regardless of the price. $query = "SELECT * FROM items WHERE price >= {$min} AND price <= {$max} AND title LIKE '%{$search_term_esc}%'"; $result = mysql_query($query);
-
When you are doing something more than once you should think about creating a funciton <?php //Function to output products for selected subcategory function displaySubcategoryProducts($subcategory) { $query = "SELECT id, product_name FROM products WHERE category='Apparel' AND subcategory='{$subcategory}' ORDER BY date_added DESC LIMIT 1"; $result = mysql_query($query); $htmlOuput .= " <center><h3>{$subcategory}</h3></center>\n"; $htmlOuput .= "<ul>\n"; while($row = mysql_fetch_array($query1)) { $htmlOuput .= "<li>"; $htmlOuput .= "<a href=\"Apparel/{$subcategory}.php\">"; $htmlOuput .= "<img src=\"inventory_images/{$id}_1.jpg\" alt=\"{$product_name}\" width=\"140\" height=\"210\" border=\"0\" />"; $htmlOuput .= "</a>"; $htmlOuput .= "</li>\n"; } $htmlOuput .= "<ul>\n"; return $htmlOutput; } //Execute one line for each subcategory to display echo displaySubcategoryProducts('Dresses'); echo displaySubcategoryProducts('Skirts'); echo displaySubcategoryProducts('Pants'); echo displaySubcategoryProducts('Hats'); ?>
-
The only thing I think I used that you were not already using was the IN statement for the query. Basically it checks for any values in the specified field that match any of the values IN the list. Other than that, I think the code I used should be pretty strait forward. The main thing I did was rearrange the logic to be more elegant. I think it is always better on if/else statements to have the error conditions come first - but that's just my preference. I also added some logic to catch individual errors on the emails. It might be overkill for your needs, but it only takes a few minutes to add something like that and it will save a ton of time later if there are any problems.
-
Yes, store the data in an array then construct a single INSERT query. I have modified your regular expression and have it working for the sample data you provided. But, with this type of logic it can easily break if unexpected data is encountered or if the site makes any changes. Personally, I would grab the data for each TD element and then break it down from there. Anyway, this seems to be working // get the HTML $url = "http://www.wightbay.com/XCClassifieds/CPSearch.asp?CMD=NEW&SortBy=CADSTARTDESC&VIEW=NORMAL&ipp=150"; $html = file_get_contents($url); //Extract the data $regEx = '#href="CPViewItem\.asp\?ID=([^"]*)">([^<]*)<\/a>.*?<td width="80" align="center">([^<]*)<#is'; preg_match_all($regEx, $html, $posts, PREG_SET_ORDER); //Process the data into multiple VALUES to do the INSERT $valuesAry = array(); foreach($posts as $post) { $id = mysql_real_escape_string(trim($post[1])); $title = mysql_real_escape_string(trim($post[2])); $price = mysql_real_escape_string(trim($post[3])); $valuesAry[] = "('$id', '$title', '$price')"; } //Create the single INSERT query $query = "INSERT INTO items (id, title, price) VALUES \n" . implode(", \n", $valuesAry); mysql_query($query); I ran that code against the actual URL and here is the first 10 lines of the query that was created: INSERT INTO items (id, title, price) VALUES ('3804796', '** £1,250 FIAT PUNTO LONG TAX AND MOT NEW CLUTCH 2001 P/X POSS £1,250 **', '£1,250.00'), ('3804786', 'I OWNER £1,950 FIESTA ZETEC 1.4 LONG T&T 2002 79K ALLOYS A/C E/W £1,950 P/X POSS', '£1,950.00'), ('3804586', 'Man and Very Large Van For Hire', ''), ('3804511', 'Cars and Vans WANTED for cash best prices paid.', ''), ('3804224', 'CAR REPAIR AND BODYSHOP FOR SALE', ''), ('3804200', '1999 (T) Ford Focus 2.0 Ghia Saloon £1295', '£1,295.00'), ('3804106', 'AMAZING 12 MONTHS MOT / 6 MONTHS TAX', '£695.00'), ('3804099', '2 MEN AND VAN FOR HIRE', ''), ('3804094', '2 MEN AND VAN FOR HIRE', ''),
-
Well, before you do that you first need to fix your logic. You should not run queries in a loop - it is terribly inefficient. You should instead generate all the records you need to add - then run one query to add them all. I'll take a look at the code and see what I can come up with to get the prices - if they apply.
-
The first thing is you need to redo the logic for pulling the records. You should NEVER run queries in loops there is almost always a way to run a single query instead of multiple and when there isn't you are probably trying to do something you shouldn't be. Second, you need to add debugging code so you can actually see what data is being returned so you know where the problem is. Also, don't use $_REQUEST, use $_POST or $_GET instead. Looking at your code I think the problem is that you have a for loop, but the code to run within that loop isn't enclosed in brackets {}, so the loop really isn't running. But, no matter you don't want to do it that way anyway. Your checkbox fields look fine, so try this for the processing page. I don't understand the purpose of all your code because of the language, but I think I understand it enough. <?php //Check if password is correct if($_POST['password'] != "bgabga") { //Password does not match echo "Password ikke udfyldt eller opgivet forkert !!!"; } elseif(!isset($_POST['mailtarget']) || count($_POST['mailtarget'])<1) { //No records were selected echo "Please select one or more recipients"; } else { //Now that we know we are going to do a query we can connect to the DB include "config.php"; $link = mysql_connect($dbhost, $dbuser, $dbpasswd) or die("Couldn't establish connection"); mysql_select_db($dbname); //Clean the user input $gruppeArry = array_map('mysql_real_escape_string', $_POST['mailtarget']); $gruppeList = "'" . implode("', '", $gruppeArry) . "'"; //Create and run query $query = "SELECT email, tilknytning FROM medlemmer WHERE tilknytning IN ($gruppeList)", $result = mysql_query($query) or die("Query:<br>$query<br>Error:<br>".mysql_error()); //Check if there were results if(mysql_num_rows($result)<1) { echo "There were no matching records"; } else { //Define these BEFORE the loop since they are static $EmailSubject = "BGA Nyhedsbrev"; $mailheader = "From: BGA\n"; $mailheader .= "Reply-To: [email protected]\r\n"; $mailheader .= "Content-type: text/html; charset=iso-8859-1\r\n"; $MESSAGE_BODY = " <H2>BGA Nyhedsbrev</H2><br>"; $MESSAGE_BODY .= "".nl2br($_POST["mailtekst"])."<br>"; //Get recipient email address and send email $success = array(); $errors = array(); while ($row = mysql_fetch_object($results)) { $ToEmail = $row->email; $sent = mail($ToEmail, $EmailSubject, $MESSAGE_BODY, $mailheader) or die ("Failure"); if($sent) { $success[] = $row->tilknytning; } else { $errors[] = $row->tilknytning; } } //Output success/error messages if(count($success)>0) { echo "Emails were successfully sent to the following users:<br>\n"; echo "<ul>\n"; foreach($success as $username) { echo "<li>{$username}</li>\n"; } echo "<ul>\n"; } if(count($errors)>0) { echo "There were problems sending emails to the following users:<br>\n"; echo "<ul>\n"; foreach($errors as $username) { echo "<li>{$username}</li>\n"; } echo "<ul>\n"; } echo "<br><br><a href='mail.html'>Tilbage</a>{$bga}"; } } ?>
-
I don't mean to be rude, but I'm curious. Your code actually has some good debugging functionality (which I rarely see), but you seem to have no clue on how to interpret it? Did you just copy paste that code or something? Your error message clearly states that the value for 'usersID' is invalid. Plus, from the query you can see that the value is a null string. Looking at your query construction the variable $useridis supposed to be used as that value. From that we can determine that either that value was not set or it is set as an empty value.So, the next step is to determine if that value was ever set. And we can determine that is is based upon this line earlier in the script: [/color][color=#0000BB]$userid [/color][color=#007700]= [/color][color=#0000BB]$_SESSION[/color][color=#007700][[/color][color=#DD0000]'userID'[/color][color=#007700]][/color][/color] So, finally, we can conclude that either that session value is not set or it has a null string value. Did you start the session at the top of that page? Did you confirm that the value was being set correctly previously?
-
If you want this to happen in real-time (i.e as soon as the user makes a change in the first select box the options in the 2nd immediately change) then you need to use JavaScript (possibly with a PHP back-end script). If you want just a PHP solution then you will have to make the user make a selecting in the first select list and then submit the page before getting the revised list in the 2nd select box. Are you really wanting the options a1, a2, a3, etc. or are those just examples of what you are trying to achieve. If the values you displayed above are really the values you are going to use, then a JS only solution would be simple to implement. But, on second thought why do you need to do this at all? Just have one select list for the user to select the letter and the second to select the number. Then on the receiving page you can combine the two to get the resulting value.
-
PHP timestamps and MySQL timestamps are not compatible. First though, regarding the 1970 reference, look at how you define the variables $today and $expires $datetoday = date('Y-m-d', $expires); $daystoexpire = '7'; $expires = time() + (86400 * $daystoexpire); $userid = $_SESSION['userID']; $newsimage = $_SESSION['imagelocation']; You first try to define $datetoday using date() with $expires as the timestamp - but you have not yet defined $expires! And timestamps start at, you guessed it, 1-1-1970. Just use date() without a timestamp to have it generate a value based upon current date/time. Ok, as for $expires, you need to convert between PHP and MySQL timestamps. So, when inserting the record you can use the following: $query = "INSERT INTO news (usersID, title, story, image, datetoday, expires) VALUES ('$userid', '$title', '$story', '$image', '$datetoday', FROM_UNIXTIME($expires))"; Then when you retrieve the value from the db you will need to convert back to unit time. $query = "SELECT UNIX_TIMESTAMP(expires) FROM news"; Here is a great article on handling dates and times between PHP and MySQL: http://www.richardlord.net/blog/dates-in-php-and-mysql
-
Updating multiple columns in one table, it wont work...
Psycho replied to Hall of Famer's topic in PHP Coding Help
Well, if you also insert a line break after the "SET" and the first value being set that format also has another benefit. You can comment out any line of the values being set and the query will still be valid. Very handy when debugging but, personally, I like the format that "reads" like a human would. When defining a string using double quotes variables will be interpreted inside the string. And, contrary to what btherl stated they are required in this instance for the $prefix variable. If you didn't use them it would try and interpret the variable $prefixuser - which doesn't exist. I prefer to interpret the variables inside the string since exiting/entering the quotes as the original string did is difficult to read. But, the brackets solve some issues where a variable may not be interpreted correctly. -
Updating multiple columns in one table, it wont work...
Psycho replied to Hall of Famer's topic in PHP Coding Help
Remove that last comma right before the WHERE clause. Also, I propose never writing the query directly within the mysql_query() function. Instead, create the query as a variable. then if there are any problems the first step in debugging is to echo the query to the page. Nine times out of ten you will find your error strait away. Lastly, giving some "structure" to the query with indenting makes it much more readable and less prone to those types of typos. $query = "UPDATE {$prefix}users SET nickname = '{$nickname}', gender = '{$gender}', color = '{$color}', profile = '{$profile}', favpet = '{$favpet}' , about = '{$about}' WHERE username = '{$loggedinname}'"; mysql_query($query) or die("Query:<br />{$query}<br />Error:<br />".mysql_error()); -
Either change the select list to a multi-select list or use checkboxes for each group you want to be able to select. In either case you will name the field (or fields) as an array, i.e. name="fieldName[]"
-
Validate form and change field BGColor if null
Psycho replied to AndrewJ1313's topic in Javascript Help
Take this for a test drive and see what you think. Breaking out the logic makes this more flexible. Couldn't get the radio buttons colored, but y0u could probably change the color of the containing div <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <style> .required { font-weight: bold; } </style> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>test</title> <script language="JavaScript"> <!-- function checkInputObj(inputObj, errorMsg) { if (inputObj) { var isEmpty = false; alert(inputObj.type); switch(inputObj.type) { case "select-one": if (inputObj.selectedIndex == -1 || inputObj.options[inputObj.selectedIndex].text == "") { isEmpty = true; } break; case "select-multiple": if (inputObj.selectedIndex == -1) { isEmpty = true; } break; case "text": case "textarea": if (inputObj.value == "" || inputObj.value == null) { isEmpty = true; } break; case undefined: var blnchecked = false; for (var j = 0; j < inputObj.length; j++) { if (inputObj[j].checked) { blnchecked = true; break; } } if (!blnchecked) { isEmpty = true; } default: break; } if(isEmpty) { errors[errors.length] = errorMsg; if(inputObj.type != undefined) { inputObj.style.backgroundColor = '#cc00cc'; } } } } //Global var to track errors var errors = new Array(); function formCheck(formObj) { //Reset errors array errors = new Array(); //Create var to track errors var formElements = formObj.elements; //Validate required fields checkInputObj(formElements['first_name'], 'First name is required'); checkInputObj(formElements['last_name'], 'Last name is required'); checkInputObj(formElements['home_phone'], 'Home phone is required'); checkInputObj(formElements['email_address'], 'Email address is required'); checkInputObj(formElements['select_one'], 'Select One is required'); checkInputObj(formElements['select_many'], 'Select Many is required'); checkInputObj(formElements['radio_grp'], 'Radio group is required'); if (errors.length > 0) { // dialog message var alertMsg = "The following errors occured:\n"; for(errIdx=0; errIdx<errors.length; errIdx++) { alertMsg += ' - ' + errors[errIdx] + '\n'; } alert(alertMsg); return false; } //No errors return true; } // --></script> </head> <body> <div id="header"> <p class="clear"></p> <div class="jg_ea"> <form action="process_form.php" method="post" name="adminForm" id="adminForm" onsubmit="formCheck(this); return false;"> <fieldset><legend>General Information</legend> <div id="personal_information_first_name"> <div class="required">First Name:</div> <input type="text" name="first_name" id="first_name" maxlength="250" value = "" /> </div> <div id="personal_information_middle_name"> <div>Middle Name:</div> <input type="text" name="middle_name" id="middle_name" maxlength="250" value = "" /> </div> <div id="personal_information_last_name"> <div class="required">Last Name:</div> <input type="text" name="last_name" id="last_name" maxlength="250" value = "" /> </div> <div id="personal_information_home_phone"> <div class="required">Home Phone:</div> <input type="text" name="home_phone" id="home_phone" maxlength="20" value = "" /> </div> <div id="personal_information_work_phone"> <div>Work Phone:</div> <input type="text" name="work_phone" id="work_phone" maxlength="20" value = "" /> </div> <div id="personal_information_cell_phone"> <div>Cell Phone:</div> <input type="text" name="cell_phone" id="cell_phone" maxlength="20" value = "" /> </div> <div id="personal_information_email_address"> <div class="required">Email Address:</div> <input type="text" name="email_address" id="email_address" maxlength="250" value = "" /> </div> <div> <div class="required">Select One:</div> <select name="select_one"><option></option><option>one</option><option>two</option><option>three</option></select> </div> <div><br /> <div> <div class="required">Select Many:</div> <select name="select_many" multiple="multiple"><option></option><option>one</option><option>two</option><option>three</option></select> </div> <div><br /> <div> <div class="required">Radio:</div> <input type="radio" name="radio_grp" value="1" /> 1<br /> <input type="radio" name="radio_grp" value="2" /> 2<br /> <input type="radio" name="radio_grp" value="3" /> 3<br /> </div> <div><br /> <input class="submitbutton" type="submit" name="submit" value="Submit" /> </div> </fieldset> </form> </div> </body> </html> -
getting the id param to work so I can delete and edit posts.
Psycho replied to Clandestinex337's topic in PHP Coding Help
I thought I already explained that, but I'll try again. You apparently have a link that looks something like this: <a href="somepage.php?action=delete">Delete Record</a> You need to add an additional parameter to the user to add the ID. I would assume you are using a DB query to display the records, so you should have the ID available to add to the link <a href="somepage.php?action=delete&id=12">Delete Record</a> Then on your page that processes the results you would do something like this: switch ($_GET['action']) { case 'create': echo 'created' . '<br/>'; create_post(); break; case 'delete': echo 'deleted' . '<br/>'; delete_post((int) $_GET['id']); break; } -
Validate form and change field BGColor if null
Psycho replied to AndrewJ1313's topic in Javascript Help
If you could provide that code in a working page with 4-6 sample fields it would be easier for me to provide a solution, -
getting the id param to work so I can delete and edit posts.
Psycho replied to Clandestinex337's topic in PHP Coding Help
Looking at your switch() statement I think you have the right idea, you just need to take it a step further. Based on that second code-block you are passing an "action" value on the URL to determine, well, the actino. But, you also need to pass the ID of the record you want to perform the action on - if the action needs it. A delete action would require the ID. So, let's back up a second. Let's say you have a page that displays all the records. You would perform a DB query to get the information to disoplay the details for each record. Then, next to each record you might have a delete link. The link would be a URL containing two parameters: action=delete&id=[##], where [xx] is the id of the record. Then when you click the link you will use the action value in your switch stement to determine that the delete function should be called. When doing so you would use the $_GET['id'] value to pass to the delete function. Does that make sense?