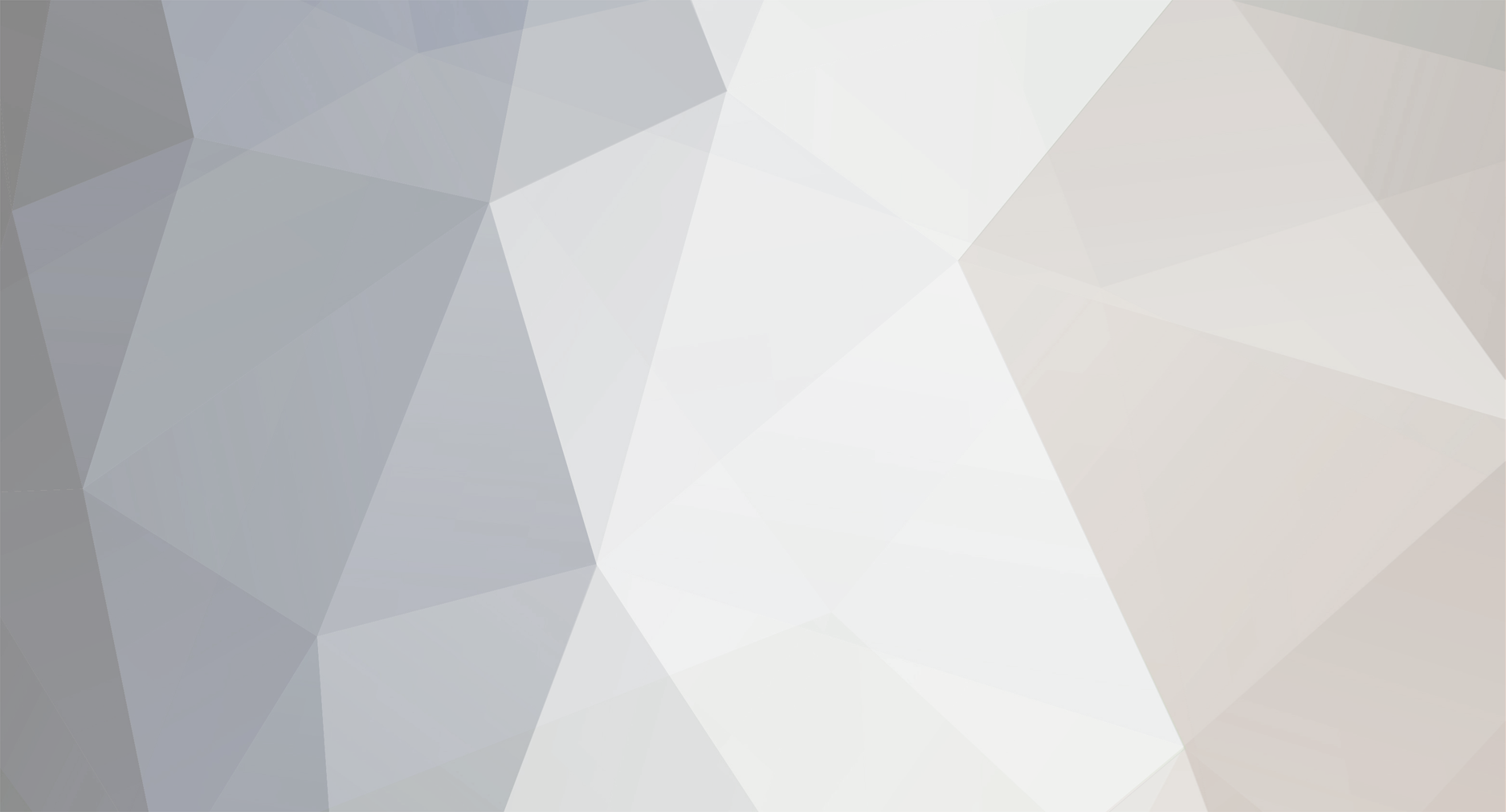
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Tables don't "communicate" or share data with each other. It is up to you, the programmer, to create references between the tables. IF you insert a record into the "Customer" table for a new customer then insert a record into the "Orders" table for that customer the database doesn't "know" that those two records are related. YOU have to create the references and then do JOINS on the tables to get the related data between tables to make it useful. Your tables above have the foreign keys (i.e. references) necessary to do what you need. You just need to make sure you are populating the data correctly AND that you are uaing the proper queries when extracting data. Here is an example. New customer signs up on the site by filling out a form. You would take the POST data and create a new record for that user with a query such as INSERT INTO Customer (first_name, last_name, house, postcode) VALUES ('$fname', '$lname', '$house', '$postcode') I assume that the field cust_id is an auto-increment field. You would then want to "capture" the ID for that customer while they are on the site. At the time you insert the record you could use mysql_insert_id() to get the ID that was just generated. Or, when the customer logs in at a later time you would do a SELECT query to get the ID. You would then, most likely, want to store the ID in a session variable to track with the user while they navigate the site in case you need the ID in a later script. OK, so now you have a customer record created and you have the customer ID stored in a session value. Now we will assume that the user adds some products to their shopping cart and places an order. You now need to create the order record(s). Your order table only has two fields (cust_id & product_id). That doesn't seem sufficient. For one, there is no way to track between mutiple orders for a customer and each order can only have one product. You would want one table to store the order "container" which will include fields such as [(fk) identifies a forein key which is associated to another table] order_id (fk), cust_id (fk), date, status, etc. You would then have an associated table for the products that belong to the order. That way an order can have multiple products. That table (let's call it order_detail) would contain fields such as: order_id (fk), prod_id (fk), qty, price_per_unit. So, you have a shopping cart full of products and you need to create an order for the customer. The process would go something like this: 1. Creat the order record using the cust_id you have stored in the user's session and any other one-to-one information for the order. Something like INSERT INTO Orders (cust_id, date, status) VALUES ('{$_SESSION['cust_id]}', NOW(), 'placed') 2. Get the order ID of the record you just created so you can add the products to the order $orderID = mysql_insert_id(); 3. Insert the records for the products of the order. I will assume the product details are in a session array. $productInserts = array(); foreach($_SESSION['order'] as $prod) { $productInserts = "('{$orderID}', '{$prod['id']}', '{$prod['qty']}', '{$prod['price']}')\n"; } $query = "INSERT INTO Order_details (order_id, prod_id, qty, price_per_unit) VALUES " . implode(', ', $productInserts); Now, you have your data in separate tables with proper associations, now comes the real benefit of a relational database. Let's say you wanted to provide the customer a list of all the orders he has placed AND show all the products that were in each order. You could get that info by doing a simple JOIN on the Orders and Order_details tables such as SELECT * FROM Orders JOIN Order_details USING (order_id) Where Orders.cust_id = $cust_id If none of this makes sense to you, you need to go read up on some tutorials with using databases. Tizag has some really good stuff
-
You are apparently trying to combine multiple INSERT queries into a single string (separated by semicolons) and attempting to run them all at one. MySQL is NOT PHP - it doesn't work that way. I don't know if you can combine all the queries into a single string. In fact, I highly doubt it, but if you can you don't separate by semi-colons. You should create and run each query separately. The reason why I think it is not possible is when you run an insert query there are certain parameters (such as last insert ID) that are set. If you ran multiple inserts in one then how would you get the insert ID for each insert?
-
You have absolutely no error checking on your current MySQL functions. So, if there is a problem you won't know what it is. Change this $dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME); To This (replace the DB name as necessary) $dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME) or die ("Cant connect to server " . mysql_error()); if (mysqli_connect_errno()) { echo "Connect failed: " . mysqli_connect_error(); exit(); } Then Change this $data = mysqli_query($dbc, $query); To This $data = mysqli_query($dbc, $query); if ($data===false) { echo "Query failed: $query<br />Error: " . mysqli_error($dbc); exit(); }
-
Yeah, but you weren't echoing the QUERY. Had you done that you would have realized that $uid was not set at the time the query was created and you would have resolved your problem much quicker.
-
How do you know it = 2??? Where is the code that sets the value? You shouldn't build your queries in the mysql_query() statement as it makes it difficult to debug. Create the query as a string so you can echo it to the page when there are problems. $query = "INSERT INTO `blog_posts` (`title`, `post`, `author_id`, `date_posted`) VALUES ('$btitle', '$bpost', '$uid', CURDATE())"; //Debug code echo $query; $result = mysql_query($query);
-
file_get_contents => PHP Fatal error: Allowed memory exhausted
Psycho replied to kristo5747's topic in PHP Coding Help
Looking at your patterns it appears that the replacements strings would need to fine "newline" characters. So, you wouldn't be able to read a line of data at a time and process it that way. One options would be to create a "buffer". Start by reading five or more lines of data. Process that data. Then remove the first line from the buffer and write it to a new file and get a new line from the input file to add to the buffer. Process the buffer again and start the process over again until you have read all the data from the input file. -
thanks, I just made one like you did with $output , but the double array was confusing me What "double" array? That code is creatng a string in the $ouput variable from the DB results. As I stated before it is dumb to dump the DB results into an array just so you can loop over the array to create the output. It is two processes when you only need one.
-
file_get_contents => PHP Fatal error: Allowed memory exhausted
Psycho replied to kristo5747's topic in PHP Coding Help
Can you provide some information on the operations you need to perform on the data? fread() might be an option depending on how you need to manipulate the data. If this is YOUR server, you can increase the allowed memory limit in the php.ini file -
Why are you looping through the db results to put them in an array just so you can then loop through the array to display the output? Makes no sense. Just create the output while you process the db results $query = "SELECT name, price FROM products"; $result = mysql_query($query)or die(mysql_error()); $ouput = ''; while($DrinkRow = mysql_fetch_assoc($result)) { $output .= "{$DrinkRow['name']} : {$DrinkRow['price']}<br />\n"; } echo $output;
-
Ahh, I was testing using the current folder, i.e. $path = ''; This should work <?php $path = "gallery_files/gallery/"; $columns = 6; $ds = DIRECTORY_SEPARATOR; //Get array of all folders $folders = glob("{$path}*", GLOB_ONLYDIR); //Create ouput for folders $folderList = ''; foreach($folders as $idx => $folder) { //Get images from folder $images = glob("{$folder}{$ds}*.[jJ][pP][gG]"); //Get random picture $image_path = $images[array_rand($images)]; //Open new row as needed if($idx%$columns == 0) { $folderList .= " <tr>\n"; } //Create TD content for folder $folderName = basename($folder); $folderList .= " <td>"; $folderList .= "<a href='pictures/?folder=$folder'>"; $folderList .= "<img src='$image_path' style='height:auto;width:110px;' alt='$folder'>"; $folderList .= "</a>"; $folderList .= "<br /><a href='pictures/?folder=$folderName'>$folderName</a>"; $folderList .= "</td>\n"; //close row as needed if($idx%$columns == $columns-1) { $folderList .= " </tr>\n"; } } //Close last row if needed if($idx%$columns != $columns-1) { $folderList .= "</tr>\n"; } echo "</pre>"; ?> <form name="Gallery" method="post"> <table cellspacing='15'> <?php echo $folderList; ?> </table> </form>
-
This will work if the images are all have the jpg extension <?php $path = "gallery_files/gallery/"; $columns = 6; $ds = DIRECTORY_SEPARATOR; //Get array of all folders $folders = glob("{$path}*", GLOB_ONLYDIR); //Create ouput for folders $folderList = ''; foreach($folders as $idx => $folder) { //Get images from folder $images = glob("{$path}{$folder}{$ds}*.[jJ][pP][gG]"); //Set random picture $image_path = $images[array_rand($images)]; //Open new row as needed if($idx%$columns == 0) { $folderList .= " <tr>\n"; } //Create TD content for folder $folderList .= " <td>"; $folderList .= "<a href='pictures/?folder=$folder'>"; $folderList .= "<img src='$image_path' style='height:auto;width:110px;' alt='$folder'>"; $folderList .= "</a>"; $folderList .= "<br /><a href='pictures/?folder=$folder'>$folder</a>"; $folderList .= "</td>\n"; //close row as needed if($idx%$columns == $columns-1) { $folderList .= " </tr>\n"; } } //Close last row if needed if($idx%$columns != $columns-1) { $folderList .= "</tr>\n"; } ?> <form name="Gallery" method="post"> <table cellspacing='15'> <?php echo $folderList; ?> </table> </form>
-
Where are the images? Are they in the subfolders? What file extensions do they have?
-
Your problem is that two of the "folders" returned in a directory are '.' and '..'. Which attempt to traverse the directory tree upwards. You need to exclude those fromthe recursive call. Your function is also overly-complicated. You also shouldn't close the filehandle in the loop/. Otherwise once the first subdirectory is run the process will fail ont he next object in the root directory. function explore($dir) { $handle = opendir($dir); while (false !== ($file = readdir($handle))) { $fullPath = $dir.DIRECTORY_SEPARATOR.$file; if (is_dir($file)) { if($file!='.' && $file!='..') { explore($fullPath); } } else { echo "<a href=\"$fullPath\">$file</a><br />\n"; } } closedir($handle); }
-
You can't put an OR condition is a case statement: case 'ru-md'||'ru': // russia You need a separate case statement for each value, but you don't have to repeat the code to be processed Correct syntax switch ($languages[0]) { case 'ko': // korea echo "do something or set a variable"; break; case 'ru-md': // russia case 'ru': echo "do something or set a variable"; break; case 'zh-cn': // china case 'zh-hk': case 'zh-mo': case 'zh-sg': case 'zh-tw': case 'zh': echo "do something or set a variable"; break; case 'ne': // india echo "do something or set a variable"; break; default: echo "good"; }
-
Here is a "rough" script that may help you. All you need to do is edit four different variables. $image_url: this will be the base URL/path to access the images - I assume it is the same for all the images $rows_per_page: maximim number of rows to be displayed on a page $cols_per_page: maximim number of columns to be displayed on a page $images: this is an array of all the images to be displayed - in the order that they are to be displyed. <?php //Base URL to access the images (set this to your Amazon space) //I have set to a folder on my server for testing purposes $image_url = 'images/'; //User defined variables for page settings $rows_per_page = 2; $cols_per_page = 4; //Master array of ALL the images in the order to be displayed $images = array( 'image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg', 'image5.jpg', 'image6.jpg', 'image7.jpg', 'image8.jpg', 'image9.jpg', 'image10.jpg', 'image11.jpg', 'image12.jpg', 'image13.jpg', 'image14.jpg', 'image15.jpg', 'image16.jpg', 'image17.jpg', 'image18.jpg', 'image19.jpg' ); //END USER DEFINED VARIABLES //System defined variable $records_per_page = $rows_per_page * $cols_per_page; $total_records = count($images); $total_pages = ceil($total_records / $records_per_page); //Get/define current page $current_page = (int) $_GET['page']; if($current_page<1 || $current_page>$total_pages) { $current_page = 1; } //Get records for the current page $page_images = array_splice($images, ($current_page-1)*$records_per_page, $records_per_page); //Create ouput for the records of the current page $ouput = "<table border=\"1\">\n"; for($row=0; $row<$rows_per_page; $row++) { $ouput .= "<tr>\n"; for($col=0; $col<$cols_per_page; $col++) { $imgIdx = ($row * $rows_per_page) + $col; $img = (isset($page_images[$imgIdx])) ? "<img src=\"{$image_url}{$page_images[$imgIdx]}\" />" : ' '; $ouput .= "<td>$img</td>\n"; } $ouput .= "</tr>\n"; } $ouput .= "</table>"; //Create pagination links $first = "First"; $prev = "Prev"; $next = "Next"; $last = "Last"; if($current_page>1) { $prevPage = $current_page - 1; $first = "<a href=\"test.php?page=1\">First</a>"; $prev = "<a href=\"test.php?page={$prevPage}\">Prev</a>"; } if($current_page<$total_pages) { $nextPage = $current_page + 1; $next = "<a href=\"test.php?page={$nextPage}\">Next</a>"; $last = "<a href=\"test.php?page={$total_pages}\">Last</a>"; } ?> <html> <body> <h2>Here are the records for page <?php echo $current_page; ?></h2> <ul> <?php echo $ouput; ?> </ul> Page <?php echo $current_page; ?> of <?php echo $total_pages; ?> <br /> <?php echo "{$first} | {$prev} | {$next} | {$last}"; ?> <img src="/images/button11.jpg" /> </body> </html>
-
You state you want to display the images int he order that you submit them. How are you obtaining that information? If you can't programatically retrieve that information from Amazon then what is the script supposed to use to determine the order for display? You can create a hard coded array that would only require a single update whenever you add an image. Seriously? What good does a variable do without any logic to interpret the variable $foo = 'bar'; There, I set a variable. Now I suppose everythign I want to happen will just magically occur.
-
You want a pagination script. There are many tutorials on this, you don't need a book. I don't know where you are storing the image data at. Well, really I doubt if you are storing it since you say you are using HTML. To do this programatically you need to use somethign to determine the order of the images. Typically this is done in a database. But, again I will assume you are not using a database since you aren't using PHP. So, you probably just have the images stored in a folder and are deciding their order by the HTML pages you are manually editing. But, how are you determining the "date submitted"? Do youhave an upload script that is storing that information somewhere? The "best" approach would be to use a PHP/MySQL approach. But, that would take a lot longer to learn. An easier, albeit, "hacK" approach would be to just use something like the file name or modification date to order the images. I'm really not going to go into muchmore detail at this time becuase I don't know what you need in your situation without more info.
-
One of those days, strange MySQL behavior on mysql_fetch_array
Psycho replied to falcon1620's topic in PHP Coding Help
I don't see any problems. The fact that the loop is runinng (i.e. the labes are displaying) you know that the loop is running that one time. Try doing a print_r() onthe $r variable. Also, to help yourself out - don't construct the query inside the mysql_query() function. It makes it much harder to debug mysql problems. Create the query as a string variable. Then if there are unexpected results you can echo the query to the page. $query = "SELECT * FROM Appointment WHERE TTUNumber = '$TTUNumber' AND ShippingNumber = '$ShippingNumber' LIMIT 1"; $sqlAppt = mysql_query($query) or die('Query: $query<br />Error: ' . mysql_error()); -
The "Solved" button was removed with a recent update to the site.
-
You wouldn't use a variable $visits - just the field name $connection = mysql_connect("localhost", "username", "password"); mysql_select_db("articles", $connection); $aid = mysql_real_esacpe_string($_GET['aid']); $query = "UPDATE articles_description SET visits = visits + 1 WHERE id = $aid"; $result = mysql_query($query) or die(mysql_error()); echo "Record updated successfully"; mysql_close();
-
That is an entirely different problem. Because instead of following the easy appraoch I provided before you can't simply repopulate the textarea whenever a user makes a change. This is possible - BUT - there are issues to be aware of. If the user makes any changes to an existing record in the teaxtarea the script would not be able to identify it for removal if the user unchecks the checkbox. Plus, if the user selects a couple checkboxes, add some text, and then checks a third checkbox, where does that content go in relation to the existng content? This should get you close to what you want. It will generate an occasional extra line break but should be close to what you want <html> <head> <title>Fruits</title> <script type="text/javascript"> function addToList(checkObj, outputObjID) { var value = checkObj.value; var outputObj = document.getElementById(outputObjID); if(checkObj.checked) { //add value to text area linebreakbefore = (outputObj.value=='') ? '' : '\n'; outputObj.value = outputObj.value + linebreakbefore + value; } else { //remove value from text area and empty lines myregexp = new RegExp('^'+value+'(\\r\\n|\\n|\\r|)', "gm"); outputObj.value = outputObj.value.replace(myregexp, ''); } return; } function checkAllBox(formObj, fieldName, checkedState) { if(formObj[fieldName].length) { var fieldLen = formObj[fieldName].length; for(var i=0; i<fieldLen; i++) { formObj[fieldName][i].checked = checkedState; addToList(formObj[fieldName][i], 'txt1'); } } else { formObj[fieldName].checked = checkedState; addToList(formObj[fieldName], 'txt1'); } return; } </script> </head> <body> <form name="myform"> <input type="checkbox" name="checkAll" value="all" onClick="checkAllBox(this.form, 'fruit[]', this.checked);" /><b>Check All</b><br> <input type="checkbox" name="fruit[]" value="Oranges" onClick="addToList(this, 'txt1');" /><span style="color:#808080">Oranges</span><br> <input type="checkbox" name="fruit[]" value="Apples" onClick="addToList(this, 'txt1');" /><span style="color:#808080">Apples</span><br> <input type="checkbox" name="fruit[]" value="Grapes" onClick="addToList(this, 'txt1');" /><span style="color:#808080">Grapes</span><br> <textarea rows="10" cols="40" name="txt1" id="txt1" style="color:#808080"></textarea> </form> </body> </html>
-
You should NEVER run queries in a loop. You should use a loop to create the code for one single INSERT query to insert all the queries at once. Here is an example: //Create array to store all insert records $insertRecords = array(); foreach ($_POST['spread'] as $weekID => $games) { foreach ($games as $gameID => $values) { $insertRecords[] = "('{$weekID}', '{$gameID}', '{$values['A']}', '{$values['H']}')\n"; } } //Create and run single query to insert all the records $sql = "INSERT INTO change_to_table_name (week_id, game_id, A_pt_spread, H_pt_spread) VALUES " . implode(", ", $insertRecords); mysql_query($sql);
-
It all really depends on how you are going to use the data. You *can* allow the user to input anything and still prevent SQL injection, cross site scripting, and just plain old HTML display issues. When saving the data to the database you should always be escaping the input to prevent SQL injection. For MySQL databases you would use mysql_real_escape_string(). When you display the input (if you don't restring the input) you could use htmlenteties() or htmlspecialcharacters() to escape characters that would cause problems in the rendered HTML. You would use strip tags if you really, really don't want the user to input that data. For example, if I put <b>text</b> in this forum post it will not display as bold text. The tags are still being saved to the database, but it is being escaped so it will not be rendered as HTML tags.
-
Yes and no. As far as your intelligence goes, I can't say - I don't know you well enough EXACTLY! My position is that programming is like art. You can teach anyone the fundamentals of painting a picture, but whether the picture is any good or not is really an innate ability. Humans (most anyway) have the ability to think logically but can't transfer that logic to programming. In the above situation you simply needed to go throughthe process of how YOU would have solved the problem. If you had two numbers that, when divided, resulted in a decmial and you wanted to change the numerator to get to the next highest whole number would you really increate the number by 1, test the result, and then continue that till you found the solution? I wouldn't - which is why I came to the solution above. Here is how I would think it through using an example: 18 / 5 = 3.6 OK, I want to change the numerator so result will be equal to that result rounded up to the next highest whole number (4). So, I need to use ceil() on the result. Now what? Well, since I am going to change the numerator to an unknown value and I have known values for the denominator and the result I know have a simple equation: X / 5 = 4, which can then be converted to x = 5 * 4.
-
Well, for starters, I would suggest you use mysql_real_escape_string() instead of addslashes() Again, what is the collation for the DB field? You should also set the HTML output for UTF-8 encoding. I forget the manner in which to do this, but you can google for solutions.