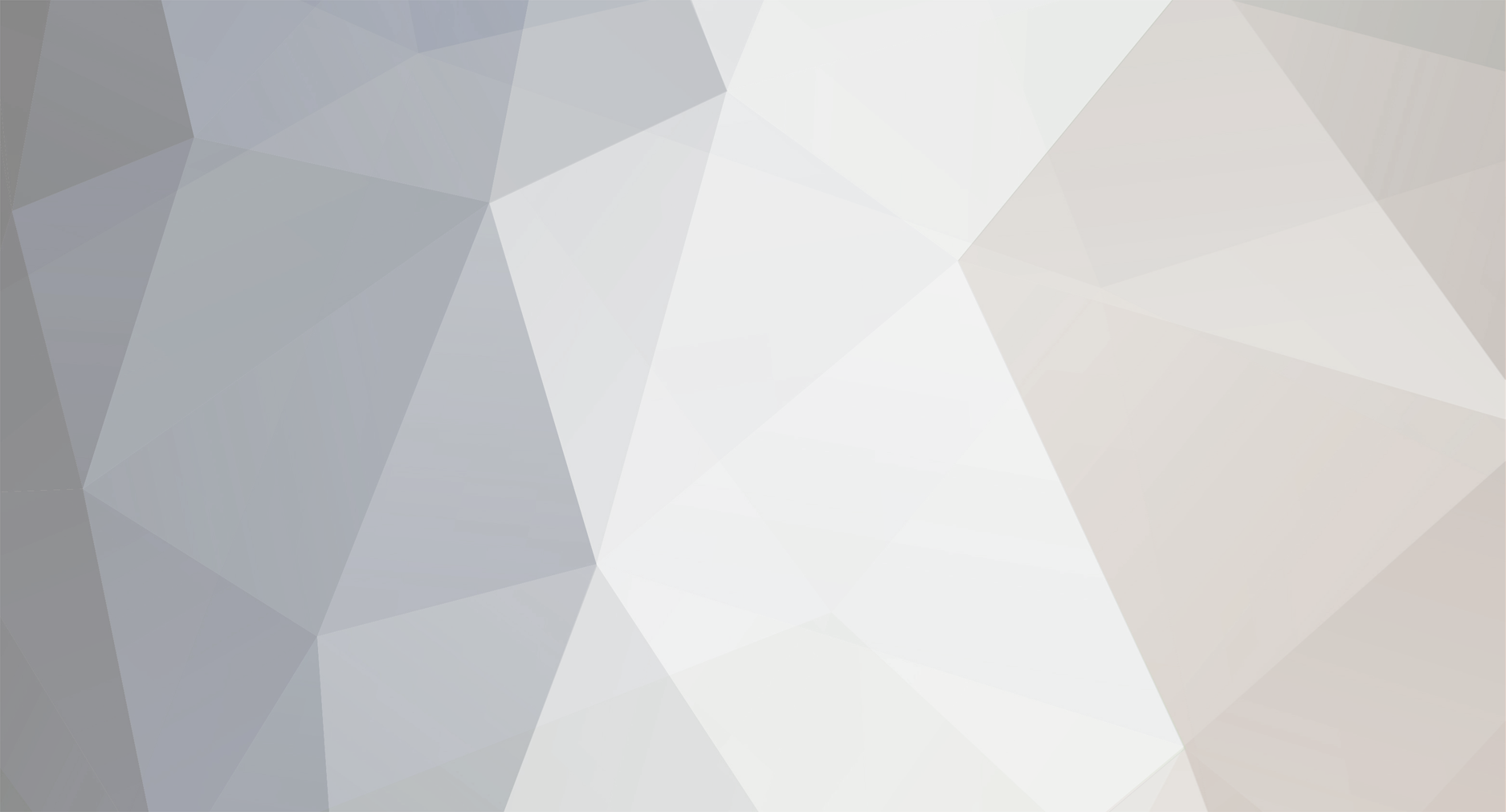
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Your IF logic is backwards. Your regex is close. It needed some delimiters. Plus you should remove the $. Besides that it was looking to see if there are any NON alpha characters. However, that is how you want to do the validation. It is easier to look for any instance of a non-alpha character than to ensure all the characters are alpha characters. You just need to change the IF/ELSE logic. $pattern = "#[^A-Z]#i"; $tom="Tod4sdadasm"; if (preg_match($pattern, $tom)) { echo "One or more non-alpha characters were found"; } else { echo "All the characters where alpha"; } Also modified the regex by adding the "i" parameter to make the test case insensitive. Um, no. That expression only tests to see if the FIRST character is a non a-z character.
-
You had better rethink that approach . . . and quickly. What happens when you have a product with the ID 13 and a scent with the ID 6 - that would also have the unique(?) ID of 136. The proper way to do this would be with three tables. Table one would list the product types (hand cream, lotion, etc.) and each would have a unique ID in that table. The second table would be the scents and again they would each have a unique ID (but they can repeat the IDs in the product table). The third table would be for the products. In that table you would have three values: the unique ID of the product, the product ID and the scent ID. Example: product_types ============= type_id type_name 1 Hand Cream 2 Lotion 3 Deoderant scents ============= scent_id scent_name 1 pomegranate 2 Lemon 3 Musk products ============= prod_id type_id scent_id 1 1 1 2 1 2 3 1 3 4 2 1 5 2 2 6 2 3 7 3 1 8 4 2 9 4 3 In the above example, the product with the ID "6" would be for the type 2 (Lotion) with the scent 3 (Musk)
-
That makes no sense to me. There is no logical reason (I can think of) why session variables would disappear and then magically appear. The only thing I can think of is a problem on the server where it can't find the session data or perhaps a problem in pulling the session ID from your PC to match to the server-side data. Try testing on a different client machine and a different server.
-
OK, so you would parse the other variables from the POST data and the SESSION value as I did with $_POST['myselect'] and add them to the query. //Get & clean values from POST/SESSION data $postVar1 = mysql_real_escape_string(trim($_POST['inputField1'])); $postVar2 = mysql_real_escape_string(trim($_POST['inputField2'])); $postVar3 = mysql_real_escape_string(trim($_POST['inputField3'])); $postVar4 = mysql_real_escape_string(trim($_POST['inputField4'])); $SessionVar1 = mysql_real_escape_string(trim($_SESSION['sessionVar1'])); //Create and run INSERT query $query = "INSERT INTO test (`dbField1`, `dbField2`, `dbField3`, `dbField4`, `dbField5`) VALUES ('{$postVar1}', '{$postVar2}', '{$postVar3}', '{$postVar4}', '{$SessionVar1}')"; $result = mysql_query($query) or die (mysql_error());
-
Well, if you implement appropriate parsing/error handling in the processing logic you don't need to worry about trimming the line break at the end. As I stated above I would personally use file() instead of fgets(). Also, it looks like in your original code you are creating a copy of the input file and then processing that file. Is there a reason for that? Seems unnecessary. I would do something like this: //Define input file $org = "org-voorraad.txt"; //Read input file into an array $data = file($org); //Process the data foreach($data as $line) { //Parse line $partRecord = explode("\t", $line); //Check that it is a valid record if(count($partRecord) != 3) { //Not a valid record, do not process //Add error handling as needed //Proceed to next record continue; } //Record appears valid, continue with processing //Remove any leading/trailing white-space in values array_map('trim', $partRecord); //Populate data array into descriptive variables // to make processing code more intuitive list($partNumber, $inStock, $price) = $partRecord; //Continue with processing script //... //... } }
-
Rather than adding the incorrect solutions to the "root" of the array, you should add them to a subarray element (for example "answers"). In addition you could add the correct solution (while also maintaining the correct in the root) to this sub array element. Then when you want to show the "possible" solutions just randomize that sub-array and display them. Lastly, don't worry about giving the incorrect solutions unique names - just add them to the array and let it index them. Example array structure array( 'text' => "what is 2 + 2", 'question_type' => "math", 'solution' => "4", 'filename' => "math_test.txt", 'author_id' => 1, 'answers' => array ( 0 => "4", 1 => "6", 2 => "3", 3 => "5" ) ) So, to display the possible solutions in a random order you would just need to randomize $data['answers'] using shuffle($data['answers']);
-
Again, still not sure what you are looking for. Do you not know how to process form data? Have you not done a database INSERT or what? Here is an example: //Get & clean value from POST data $postedValue = mysql_real_escape_string(trim($_POST['myselect'])); //Create and run INSERT query $query = "INSERT INTO test (`Sub_ID`) VALUES ({$postedValue})"; $result = mysql_query($query) or die (mysql_error());
-
Did you even try it? I'm thinking he may have forgotten to take out the previous code that removes all the line breaks
-
Did you try it? rtrim() will only trim whitespace from the END of the string. So, it would not be trimming anything before the last non white-space character. Inthe example data you posted above it would trim everything after the last number 5. EDIT: Personally, when processing a file such as that I prefer to use file() to read the file as an array with each line a different item in the array. Then I process each line using appropriate validation. In the case above I would process each line and "break" it into the three variables listed. If the line did not contain all three variables I would skip it (and provide appropriate error handling as needed). So, if there was an extra line break at the end (or many) they would be skipped in the processing.
-
So, what exactly are you having a problem with? Are you wanting someone to just write the code for you? If so, this is the wrong forum.
-
I see a lot wrong with that code, but nothing stands out regarding the problem you state. However, the second block of code you post which is for a "typical" page may be an indication of the problem. You should NOT put code on every page to check login status. Instead you should have ONE page to check login status and call that page at the top of every page that needs it. Otherwise, if you have a typo on just one page it could cause the types of problems you are talking about - and you would have to check each and every page. If you are doing the same thing more than once - don't copy/paste the code. Write the code once and call it multiple times. But, the code you ahve at the top of that typical page is not necessary. You already have $_SESSION['login'] to determine if the user is "logged in". Why do you need to create another variable for that purpose? Also, you are not hashing the password which is a risk
-
finding the last record in array from mysql
Psycho replied to giraffemedia's topic in PHP Coding Help
To add to that, if you want the max booking number for all forms then this should do the trick SELECT booking_form_number, MAX(issue_number) FROM table_name GROUP BY booking_form_number -
I understand you may be new to programming (at least that is what I assume). But, that is not a lot of code. Walk through your code and understand what it is doing. Exactly "HOW" do you expect that code to show/process all the results from the database. You are only grabbing the first result from the db results whith this line $row = mysql_fetch_array($result); You have to continue to use mysql_fetch_array() until you have read all the records from the db result. But, that is the absolute worst way to check if the user exists in the database. Instead of getting all the results and comparing them to the POST values. Use the POST values to see if there is a matching record in the DB! <?php session_start(); m require_once("includes/dbconn.php"); $connection = mysql_select_db('center'); if(!$connection) { echo "couldn't connect to database"; } echo $_SESSION['username']; if(isset($_POST['username'])) { //Login form was posted - try to authenticate //Parse the POSTed data $user = mysql_real_escape_string(trim($_POST['username'])); $pass = mysql_real_escape_string(trim($_POST['password'])); //Create and run query to find user in DB $sql = "SELECT username FROM users WHERE username='{$user}' AND password='{$pass}'"; $result = mysql_query($sql); if(mysql_num_rows($result)>0) { $_SESSION['username'] = trim($_POST['username']); header("Location: home.php"); } else { echo "Invalid Username or Password"; } } ?> <form name="login" method="post"> <input name="username" type="text"></br> <input name="password" type="password"></br> <input name="submit" type="submit" value="Log In"></br> </form>
-
To be honest your code is a little confusing and the comments don't explain what the code is doing. For example, you have a loop to iterrate through all the cart objects in the session. If there is a match on the ID you are using a counter ($i) to remove that item and replace it with what was POSTed. That's way more compicated than it should be. First of all you should use foreach($_SESSION["cart_array"] as $idx => $each_item) Then use $idx (or whatever you want to call it) instead of using an artificial counter. Second, there is no need to use array slice. Simply redefine the array index using the POSTEd values. But, even all that is superfulous. I see no reason to make your cart data so complicated. You should just be saving the cart as a simple array ($_SESSION["cart_array"]) with the product ID as the key and the quantity as the value. The product ID should be to a unique product that contains the scent and "id" (not sure what the difference would be between ID and product ID. At the very least - if you do need the multidimensional array - use the unique ID (whichever one that is) as the index for each cart item. That way you don't have to loop through all the current cart records to find a match. BUt, I think I can see why your code to remove an item is not working if($_POST['item_to_adjust']['quantity'] == 0) { $key_to_remove=$_POST['item_to_adjust']; unset($_SESSION["cart_array"]['$key_to_remove']); } Do you even have a POST value of $_POST['item_to_adjust']['quantity']??? Based upon the variables you define above it seems you have two distinct POST values for 'item_to_adjust' and 'quantity'. So, that statemetn is never returning true. Even if it did the code within wouldn't work based upon my understanding of your structure. You really need to think about your structure and start over. But, I think this may work with your current code /* code to adjust the item quantity in the cart. If the quantity is set to 0, the item will be removed from the cart */ if(isset($_POST['item_to_adjust']) && $_POST['item_to_adjust'] != "") { //Dump POST vars into new array $adjust['id'] = $_POST['item_to_adjust']; $adjust['product_id'] = $_POST['name']; $adjust['quantity'] = $_POST['quantity']; $adjust['scent'] = $_POST['scent']; //Loop through each cart product foreach($_SESSION["cart_array"] as $idx => $item) { //Check if this cart product matches the adjustment product if ($item['product_id'] == $adjust['product_id']) { //Check if adjustment qty is 0 if($adjust['quantity']==0) { //Remove the cart product unset($_SESSION["cart_array"][$idx]) } else { //Change the cart product $_SESSION["cart_array"][$idx] = $adjust; } //Exit the foreach loop break; } } }
-
Displaying master details page links in columns
Psycho replied to wchamber22's topic in PHP Coding Help
<?php $columns = 3; $recCount = 0; $plantDetailsHTML = ''; while ($row = mysql_fetch_assoc($rsBotanicalA)) { $recCount++; //Open row if needed if($recCount%$columns==1) { $plantDetailsHTML .= "<tr>\n"; } //Display record $plantDetailsHTML .= "<td align=\"left\">"; $plantDetailsHTML .= "<a href=\"plantdetails.php?recordID={$row['PlantID']}\">{$row['BotanicalName']}</a>"; $plantDetailsHTML .= "</td>\n"; //Close row if needed if($recCount%$columns==0) { $plantDetailsHTML .= "</tr>\n"; } } //Close last row if needed if($recCount%$columns!=0) { $plantDetailsHTML .= "</tr>\n"; } ?> <table> <?php echo $plantDetailsHTML; ?> </table> -
Let's work from the code I last posted. I have it working with the db you provided. The results I was getting with the test data was what I expected - 1) All the levels and their associated levels were displayed with checkboxes and 2) the level/subject combinations where a tutor had a matching record were checked. Further, I also implemented code to update the selections when the page was submitted. I must have missed where you explained the purpose of the tutor_selected_level table. I *think* you are using it to select all subjects for a particular level for a particular tutor. If so, that table is not needed since you can determine if all the subjects in a level are selected for the tutor or not using the data that exists in the other tables. Also - a side note - not every table needs to have an auto-incrementing ID field. For tables that merely associated data between two tables an ID field would typically have no purpose. Your database is way too complex as it is - no need to make it more so. Anyway, after posting the code yesterday I realized there was a way to get all the data in one query. So, I have made that change in the code below. I have also updated the code to check the parent checkbox if all the subjects in the level are checked - without needing the tutor_selected_level table). Int he processing code I parse all the subjects to create their checkboxes THEN I create the level checkbox. That way I can programatically determine if all the subjects were selected. I have no idea what you are referring to here The code I posted yesterday (and below) does not do that. It appears to list the appropriate subjects for each level. NOTE: Although the code below will auto-populate the level checkbox if all the subjects for the level are selected in the DB, the code to process the form submission DOES NOT process the level header checkbox. The code could be chnged to process both the level header and subject checkboxes. But, an easier solution is to simply implement some javascript to select/unselect all subjects when a level checkbox is selected. You would want to do this anyway since it would be possible to check the level header and none of the subjects. That would pe a problematic scenario as you wouldn't know the intention of the user. So, I have also added that functionality as well. So, there may not be any need for the tutor_subject_level table. Please use this code and ask any questions/comments regarding this. As I said I got this working using the db records you provided and it will take me too long to try and step back. <?php $dbc = mysqli_connect('localhost', 'root', '', 'XXX') or die(mysqli_error($dbc)); //THIS SECTION FOR TESTING PURPOSES ONLY if(!isset($_GET['tutor_id']) && !isset($_GET['tutor_id'])) { $query = "SELECT tutor_id FROM tutor_login"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); $tutor_options = ''; while($row = mysqli_fetch_assoc($result)) { $tutor_options .= "<option value=\"{$row['tutor_id']}\">{$row['tutor_id']}</option>\n"; } echo "<html>\n"; echo "<body>\n"; echo "<form action=\"\" method=\"GET\">\n"; echo "Select a tutor:"; echo "<Select name=\"tutor_id\">\n"; echo $tutor_options; echo "</select>\n"; echo "<button type=\"submit\">Submit</button>\n"; echo "</form>\n"; echo "</html>\n"; exit(); } $tutor_id = (isset($_POST['tutor_id'])) ? $_POST['tutor_id'] : $_GET['tutor_id']; if(isset($_POST['tutor_id'])) { $tutor_idSQL = mysqli_real_escape_string($dbc, $tutor_id); //User submitted form process the changes //Delete current level/subjects for tutor $query = "DELETE FROM tutor_overall_level_subject WHERE tutor_id = '$tutor_idSQL'"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); //Add new level/subjects for tutor //Generate VALUE(s) from POST data for the INSERT query if(isset($_POST['subject_level']) && is_array($_POST['subject_level'])) { $values = array(); foreach($_POST['subject_level'] as $subjectLevel) { $subjectLevel = (int) $subjectLevel; $values[] = "('$tutor_idSQL', $subjectLevel)"; } //Create and run query with all the values to be added $query = "INSERT INTO tutor_overall_level_subject (tutor_id, subject_level_id) VALUES " . implode(', ', $values); $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)."\n<br>$query"); } } function createLevelCheckboxes($levelArray, $columns) { if(count($levelArray)==0) { return false; } $subjectsHTML = ''; //Create output for each subject $subjectIDs = array(); $allChecked = true; foreach($levelArray as $data) { //Get vars $subjectID = $data['subject_level_id']; $subjectName = $data['subject_name']; $recordCount = array_push($subjectIDs, $subjectID); //Start new row if needed if ($recordCount % $columns == 1) { $subjectsHTML .= "<tr>\n"; } //Display the record if($data['checked']=='1') { $checked = ' checked="checked"'; } else { $checked = ''; $allChecked = false; } $subjectsHTML .= " <td>"; $subjectsHTML .= " <input name=\"subject_level[]\" class=\"subject_a\" type=\"checkbox\"{$checked}"; $subjectsHTML .= " id=\"subject_level_{$subjectID}\" value=\"{$subjectID}\"/>\n"; $subjectsHTML .= " <label for=\"subject_level_{$subjectID}\" class=\"subject_1\">{$subjectName}</label>\n"; $subjectsHTML .= "</td>\n"; //Close row if needed if ($recordCount % $columns == 0) { $subjectsHTML .= "</tr>\n"; } } //Close last row if needed if ($recordCount % $columns != 0) { $subjectsHTML .= "</tr>\n"; } //Create level header checkbox $levelHTML = ''; $levelID = $levelArray[0]['level_id']; $levelName = $levelArray[0]['level_name']; $checked = ($allChecked) ? ' checked="checked"' : ''; $levelHTML .= "<tr>\n"; $levelHTML .= "<th colspan=\"{$columns}\" style=\"text-align:left;padding-top:15px;\">"; $levelHTML .= "<input name=\"level[]\" type=\"checkbox\" id=\"level_{$levelID}\" value=\"{$levelID}\" {$checked} "; $levelHTML .= " onclick=\"checkAll(this, '" . implode(',', $subjectIDs) . "');\" \>"; $levelHTML .= "<span class=\"zone_text_enlarge\"><label for=\"level_{$levelID}\">{$levelName}</label></span>"; $levelHTML .= "</th>\n"; $levelHTML .= "</tr>\n"; //Return the output return $levelHTML . $subjectsHTML; } //Run query to get ALL subject/level checkboxes $tutor_idSQL = mysqli_real_escape_string($dbc, $tutor_id); $query = "SELECT tsl.subject_level_id, tl.level_id, tl.level_name, ts.subject_id, ts.subject_name, tols.checked FROM tutor_subject_level AS tsl INNER JOIN tutor_level AS tl USING (level_id) INNER JOIN tutor_subject AS ts USING (subject_id) LEFT JOIN (SELECT subject_level_id, 1 as checked FROM tutor_overall_level_subject WHERE tutor_id = '{$tutor_idSQL}') AS tols USING (subject_level_id) ORDER BY tl.level_id, ts.subject_name"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); //Process the results $checkboxes = ''; //Create variable for output $current_level_id = false; $level_data = array(); while($data = mysqli_fetch_assoc($result)) { //Check if level changed from last if($current_level_id != $data['level_id']) { $checkboxes .= createLevelCheckboxes($level_data, 5); $current_level_id = $data['level_id']; $level_data = array(); } $level_data[] = $data; } $checkboxes .= createLevelCheckboxes($level_data, 5); ?> <html> <head> <script type="text/javascript"> function checkAll(levelObj, idList) { var subIDary = idList.split(','); var idx = 0; for(idx=0, idCount=subIDary.length; idx<idCount; idx++) { document.getElementById('subject_level_'+subIDary[idx]).checked = levelObj.checked; } return; } </script> </head> <body> <b>Level/Subject selections for <?php echo $_GET['tutor_id']; ?></b> <br /> <a href="<?php echo $_SERVER['PHP_SELF']; ?>">Select a different tutor</a> <form action="" method="POST"> <button type="submit">Submit</button> <input type="hidden" name="tutor_id" value="<?php echo $_GET['tutor_id']; ?>" /> <table> <?php echo $checkboxes; ?> </table> <br/> <button type="submit">Submit</button> </form> </body> </html>
-
Can there be 2 while loops in a single code?
Psycho replied to genzedu777's topic in PHP Coding Help
At first glance I thought you were trying to process the results of the same query twice. But, the answer is still the same - you can have two while loops on a page. -
You got the wrong idea. You can set/use global variables so you can use them in different scopes within the same execution. I.e. from one function to another. However, using global variables is typically not the best method of doing that. Instead you can just pass the variables from one function to another. However, in your case, you want a variable created on one page load to be available on another page load. You need to use either session variables (if you only need then for the current session) or cookies (if you need them to persist for multiple sessions).
-
Change this if ($fd) { while (!feof ($fd)) { $seriestitle = trim(fgets($fd, 1024)); // Series Title To this if ($fd) { $maxCount = 10; //Set to number of records to display $count = 0; while (!feof ($fd) && $count<$maxCount) { $count++; $seriestitle = trim(fgets($fd, 1024)); // Series Title
-
OK, after playing around with this I am going to make a suggestino that you probably won't like. I think you *should* change your database structure. Trying to JOIN the tutor_overall_level_subject table on the rest of the tables is killing my system since you have to JOIN it only where the tutor_id is a specific value. I've tried the queries several different ways and it takes way to long to run. I also found a problem with your database, in the tutor_overall_level_subject table you have two of the ID fields (foreign keys) set as varchars instead of ints. This caused a problem when trying to compare. However, I will give you a solution that works with what you have. It will require two queries. I will run one query to get all the subject/levels for the tutor. Then dump those restults into an array. Then I'll run a second query to get ALL the possible subject/level combinations. Then when processing those results for the HTML ouptu I'll check each subject/level to see if it exists in the tutors list from the first query to determine if the checkbox should be checked or not. I have tested this code against the database you provided and it seems to work as expected. It creates a page with all the levels/subjects available as checkboxes and the ones that correspond to the records in the tutor_overall_level_subject for the given tutor are checked. There is a block of code at the top (and some int he HTML output) for testing purposes only so I could select a tutor. Also, I added the code to update the changes according to the checkboxes selected. However, the "Level" checkboxesd are NOT used in the updating process. You can use JavaScript to handle the checking/unchecking of the child records. Plus, you can add code to the processing to add all the child records if the parent is selected. <?php $dbc = mysqli_connect('localhost', 'root', '', 'XXX') or die(mysqli_error($dbc)); //THIS SECTION FOR TESTING PURPOSES ONLY if(!isset($_GET['tutor_id']) && !isset($_GET['tutor_id'])) { $query = "SELECT tutor_id FROM tutor_login"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); $tutor_options = ''; while($row = mysqli_fetch_assoc($result)) { $tutor_options .= "<option value=\"{$row['tutor_id']}\">{$row['tutor_id']}</option>\n"; } echo "<html>\n"; echo "<body>\n"; echo "<form action=\"\" method=\"GET\">\n"; echo "Select a tutor:"; echo "<Select name=\"tutor_id\">\n"; echo $tutor_options; echo "</select>\n"; echo "<button type=\"submit\">Submit</button>\n"; echo "</form>\n"; echo "</html>\n"; exit(); } $tutor_id = (isset($_POST['tutor_id'])) ? $_POST['tutor_id'] : $_GET['tutor_id']; $tutor_idSQL = mysqli_real_escape_string($dbc, $tutor_id); if(isset($_POST['tutor_id'])) { //User submitted form process the changes //Delete current level/subjects for tutor $query = "DELETE FROM tutor_overall_level_subject WHERE tutor_id = '$tutor_idSQL'"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); //Add new level/subjects for tutor //Generate VALUE(s) from POST data for the INSERT query $values = array(); foreach($_POST['subject_level'] as $subjectLevel) { $subjectLevel = (int) $subjectLevel; $values[] = "('$tutor_idSQL', $subjectLevel)"; } //Create and run query with all the values to be added $query = "INSERT INTO tutor_overall_level_subject (tutor_id, subject_level_id) VALUES " . implode(', ', $values); $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)."\n<br>$query"); } function createLevelCheckboxes($levelArray, $columns) { if(count($levelArray)==0) { return false; } $htmlOutput = ''; //Display level header row $levelID = $levelArray[0]['level_id']; $levelName = $levelArray[0]['level_name']; $htmlOutput .= "<tr>\n"; $htmlOutput .= "<th colspan=\"{$columns}\" style=\"text-align:left;padding-top:15px;\">"; $htmlOutput .= "<input name=\"level[]\" type=\"checkbox\" id=\"level_{$levelID}\" value=\"{$levelID}\">"; $htmlOutput .= "<span class=\"zone_text_enlarge\"><label for=\"level_{$levelID}\">{$levelName}</label></span>"; $htmlOutput .= "</th>\n"; $htmlOutput .= "</tr>\n"; //Display each subject $recordCount = 0; foreach($levelArray as $data) { //Increment counter $recordCount++; //Start new row if needed if ($recordCount % $columns == 1) { $htmlOutput .= "<tr>\n"; } //Display the record $subjectID = $data['subject_level_id']; $subjectName = $data['subject_name']; $checked = ($data['checked']) ? ' checked="checked"' : ''; $htmlOutput .= " <td>"; $htmlOutput .= " <input name=\"subject_level[]\" class=\"subject_a\" type=\"checkbox\" {$checked}"; $htmlOutput .= " id=\"subject_level_{$subjectID}\" value=\"{$subjectID}\"/>\n"; $htmlOutput .= " <label for=\"subject_level_{$subjectID}\" class=\"subject_1\">{$subjectName}</label>\n"; $htmlOutput .= "</td>\n"; //Close row if needed if ($recordCount % $columns == 0) { $htmlOutput .= "</tr>\n"; } } //Close last row if needed if ($recordCount % $columns != 0) { $htmlOutput .= "</tr>\n"; } return $htmlOutput; } //Run query to get subjects/levels of the selected tutor $query = "SELECT subject_level_id FROM tutor_overall_level_subject AS tols WHERE tols.tutor_id = '$tutor_idSQL'"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); //Put results into an array $tutor_subject_levels = array(); while($row = mysqli_fetch_assoc($result)) { array_push($tutor_subject_levels, $row['subject_level_id']); } //Run query to get ALL subject/level checkboxes $query = "SELECT tsl.subject_level_id, tl.level_id, tl.level_name, ts.subject_id, ts.subject_name FROM tutor_subject_level AS tsl INNER JOIN tutor_level AS tl USING (level_id) INNER JOIN tutor_subject AS ts USING (subject_id) ORDER BY tl.level_id, ts.subject_name"; $result = mysqli_query($dbc, $query) or die(mysqli_error($dbc)); //Process the results $checkboxes = ''; //Create variable for output $current_level_id = false; $level_data = array(); while($data = mysqli_fetch_assoc($result)) { //Set checked parameter if tutor has this subject/level $data['checked'] = (in_array((int)$data['subject_level_id'], $tutor_subject_levels)); if($current_level_id != $data['level_id']) { $checkboxes .= createLevelCheckboxes($level_data, 5); $current_level_id = $data['level_id']; $level_data = array(); } $level_data[] = $data; } $checkboxes .= createLevelCheckboxes($level_data, 5); ?> <html> <body> <b>Level/Subject selections for <?php echo $_GET['tutor_id']; ?></b> <br /> <a href="<?php echo $_SERVER['PHP_SELF']; ?>">Select a different tutor</a> <form action="" method="POST"> <input type="hidden" name="tutor_id" value="<?php echo $_GET['tutor_id']; ?>" /> <table> <?php echo $checkboxes; ?> </table> <br/> <button type="submit">Submit</button> </form> </body> </html>
-
Putting the "structure" (i.e. the HTML output) in both the IF and ELSE conditions is not wise, IMHO. Because if you ever need to change the display you have to remember to change both. This will take care of that as well as make your code a little neater $x = $r + $c * $rows; if ( $x < $cnt) { $value = $partnumbers[$x]; } else { $value = ' '; } echo "<td align=\"left\">{$value}</td>\n";
-
Where Do I Put : in my preg_match to Qualify URL with :
Psycho replied to vanleurth's topic in Regex Help
This should work $preg_match02 = "/^[a-zA-Z]+[:\/\/]+[A-Za-z0-9\-_]+\\.+[A-Za-z0-9\.\/%&=\?\-_\:]+$/i"; -
As I said, it takes me way to long to try and wrap my head around this each time you ask a different question. Backup the tables needed to a file and attach to this post.
-
Can there be 2 while loops in a single code?
Psycho replied to genzedu777's topic in PHP Coding Help
Yes, you can have 2 while loops in the same code - BUT you cannot do a 2nd while loop to fetch the results of a db query if the results have been exhausted by the first while loop unless you reset the pointer to the beginning of the db results. But, there should never be a need to run through the results of a db query twice. Do everything you need to do with the results in the first loop. -
To work on your problem takes a great deal of my time because I have to re-learn your data structure and work without a copy of your database to test against. I will gladly help, but I need you to provide a backup of the database to work with.