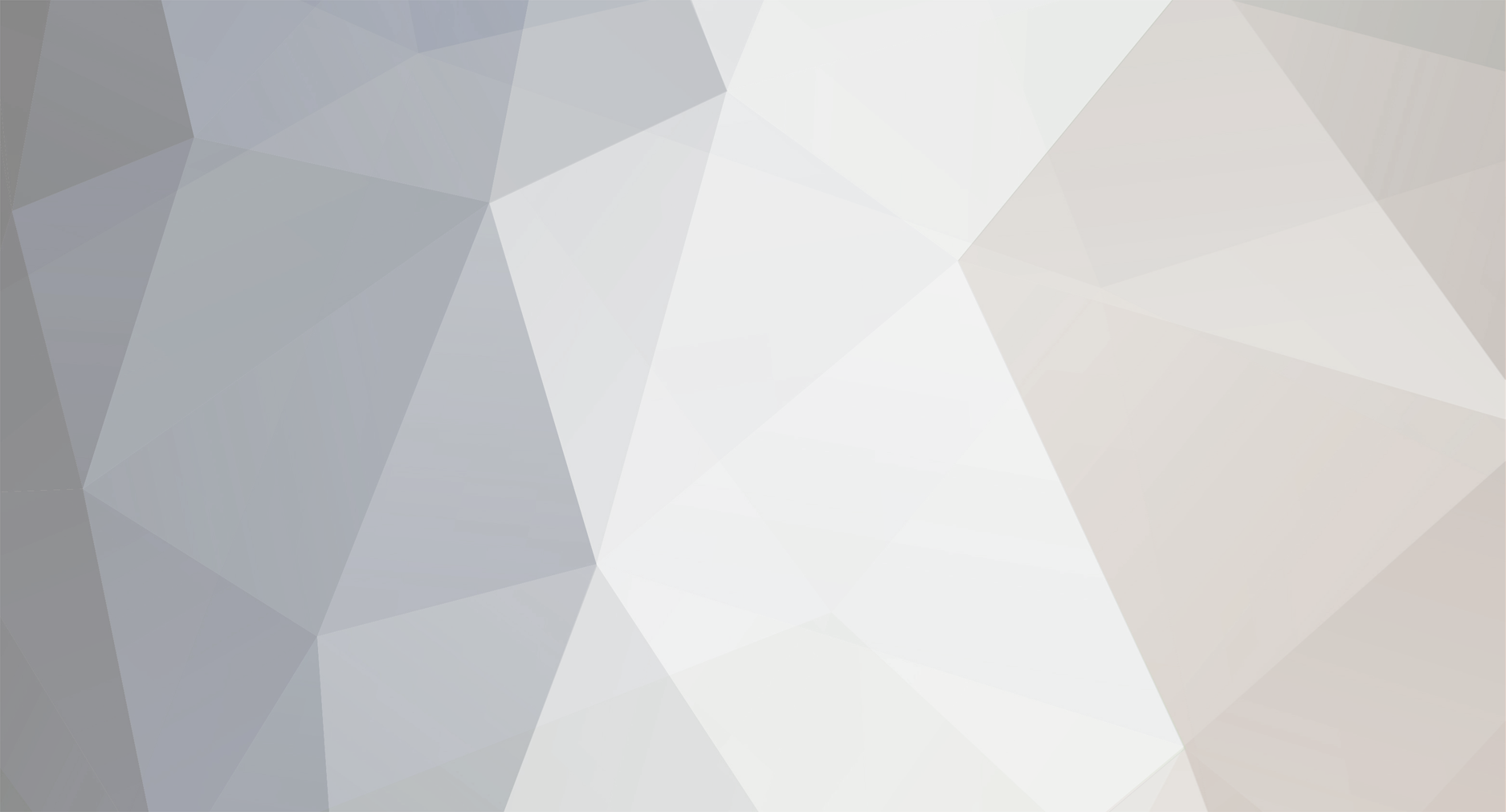
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
I made the comment previously I have no idea what you are setting the value as. If you are setting the value as the boolean true/false then trying to compare with the string values of 'true'/'false' is not correct. Plus, you have TWO conditions on that IF statement. The second is a function call. I have no idea what is being returned from that function call and whether it is what you expect or not. Put these line right before that last IF statement. To see what is being compared: echo "ACCOUNT_COMPANY: "; var_dump(ACCOUNT_COMPANY); echo "<br />\ntep_not_null(company_tax_id): "; var_dump(tep_not_null($company_tax_id));
-
You stated you wanted separate job number lists for each category, but your code shows one table. If you only want to maintain one list (which will be the easiest solution) then just set up the ID field to be an auto-increment field and set the type as a separate field. Then don't specify a job ID when inserting and the DB will create it for you. Very easy solution as the database will do all the work for you. Below is some sample code (I also moved the logic for parsing the post data and connecting to the database. There is no need to do that if nothing was posted since you aren't going to do anything with it) <?php if(isset($_POST['submit'])) { // Connect to server and select database. $host = ""; // Host name $username = ""; // Mysql username $password = ""; // Mysql password $db_name = ""; // Database name mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); //Parse & escape POSTed values $category = mysql_real_escape_string(trim($_POST['category'])); $name = mysql_real_escape_string(trim($_POST['project-name'])); //Create & Run insert query $query = "INSERT INTO job-numbers (category, name) VALUES ('$category', '$name')"; $result = mysql_query($query); if (!$result) { //Query failed, display error echo"Error: <br />\n" . mysql_error() . "<br />\nQuery:<br />\n{$query}\n";; } else { //Display job id of inserted record. $id = mysql_insert_id(); $cat = htmlspecialchars((trim($_POST['category'])); echo"The record {$cat}-{$id} was created."; } } ?> <html> <body> <form action="#" method="post"> <select id="category" name="category"> <option>Web</option> <option>Print</option> </select> <input type="text" name="project-name" id="project-name" /> <input type="submit" name="submit" id="submit" /> </form> </body> </html> If you do need separate consequtive numbers for each category it can be done, but will be more complex
-
From the manual for str_pad(): Example //Two digit number padded to 6 characters with '0' echo str_pad('12', 6, '0', STR_PAD_LEFT); //Output: 000012 //Five digit number padded to 6 characters with '0' echo str_pad('12345', 6, '0', STR_PAD_LEFT); //Output: 012345
-
Do you know what "pad" means? Also, do you need the sequential numbers to be independanct for each category? If not, then BlueSkyIS's solution would work. But, if they need to be unique for each category you could use three separate tables.
-
There are several ways you could achieve this. Here is one function reKeyArray($inputArray) { if(!is_array($inputArray)) { return false; } $outputArray = array(); foreach($inputArray as $subArray) { if(!is_array($subArray)) { continue; } $outputArray = array_merge($outputArray, $subArray); } return $outputArray; }
-
You must have misunderstood me. You are setting specific text based on gender - whether it is only the first email or both is not relevant. I only provided a more logical means of setting that text.
-
does anyone know a function i could use instead of stristr
Psycho replied to kevinkhan's topic in PHP Coding Help
You need to check the values that YOU THINK are being compared. Most likely you are getting a single character or something like that. By the way, why are you looping through all the values in the array? Use in_array() instead. Just be sure the values in the search string and the array have the same case. For debugging, before this line if($school!="" && stristr($match[2], $school)) { Add: echo "Comparing [{$school}] & [{$match[2]}]<br />\n"; -
Have the function return an array of those three values.
-
You don't need an esle statement - just an IF statement. One question though, what would be the value of $gender if ACCOUNT_GENDER does not equal 'true'. If you were to set $gender to false when ACCOUNT_GENDER is false, it makes your code much simpler. (Also, whay are you comparing ACCOUNT_GENDER to the string 'true' instead of the boolean true???) If $gender will have a false value when gender is not specified. $name = $firstname . ' ' . $lastname; //Determine the appropriate greeting if ($gender == 'm') { $greet = EMAIL_GREET_MR; } elseif ($gender == 'f') { $greet = EMAIL_GREET_MS; } else { $greet = EMAIL_GREET_NONE; } //Compose the message $email_text = sprintf($greet, $lastname); //Send the first email unconditionally $email_text .= EMAIL_WELCOME . EMAIL_TEXT . EMAIL_CONTACT . EMAIL_WARNING; tep_mail($name, $email_address, EMAIL_SUBJECT, $email_text, STORE_OWNER, STORE_OWNER_EMAIL_ADDRESS); //Send the 2nd email IF it meets the condition if (ACCOUNT_COMPANY == 'true' && tep_not_null($company_tax_id)) { $email_text_coupon .= EMAIL_WELCOME . EMAIL_TEXT_COUPON . EMAIL_CONTACT. EMAIL_WARNING; tep_mail($name, $email_address, EMAIL_SUBJECT_COUPON, $email_text_coupon, STORE_OWNER, STORE_OWNER_EMAIL_ADDRESS); }
-
I suspect you will have the same problem with any character outside the "common" character set. When dealing with ASCII vs UTF, vs etc. You can get some odd results. Instead of trying to match the pound symbol, you can take another approach. Before I give a couple of possible solutions, the current pattern is inefficient. Instead of using ".*?", I would asume the data withint he SPAN tags does not have a "<". In tat case you should change the pattern to find all chaacters NOT a "<". Anyway, If the value inside the SPAN tag always has a pound symbol, then just create the pattern to not include the first character. preg_match_all('/<span class="price">.([^<]*)<\/span>/', $html, $prices); Another approach is to find all characters between the span tags that are numerals or the decimal point. But, I'd give that first one a try.
-
//Clean input and prevent sql injection attack $UserNameToCheck = mysql_real_escape_string(trim($_GET['inputUN'])); $query = "SELECT active FROM tableName WHERE Username = '{$UserNameToCheck}'"; $result = mysql_query($query) or die(mysql_error()); if(mysql_num_rows($result)==0) { echo "The user {$UserNameToCheck} does not exist."; } else { $activeStatus = (mysql_result($result, 0)=='1') ? 'active' : 'not active'; echo "The user {$UserNameToCheck} is {$activeStatus}"; }
-
Again, I will ask the same question. Is there a scenario where a tutor would need to have the "Pre-School" level selected BUT not have any of the pre-school subjects selected??? If that is not a valid scenario then, as I have stated multiple times before, you can logically determine the "levels" that a tutor is qualified for based upon the subjects you ahve assigned.
-
OK, here is an article on how to create a single UPDATE query for multiple records needing differnt update values. http://www.karlrixon.co.uk/articles/sql/update-multiple-rows-with-different-values-and-a-single-sql-query/ So, in your loop you will want to create the data for the query. Then after the looping of the data is complete run the one single query to update all the records in one shot. Your performance should drastically improve. Here is a modification of the previous code I posted with example logic that would allow you to run one single update query for all records /Define input file $org = "org-voorraad.txt"; //Read input file into an array $data = file($org); //Create temp arrays for query $barCodes = array(); $whenClauses = array(); //Process the data foreach($data as $line) { //Parse line $partRecord = explode("\t", $line); //Check that it is a valid record if(count($partRecord) != 3) { //Not a valid record, do not process //Add error handling as needed //Proceed to next record continue; } //Record appears valid, continue with processing //Remove any leading/trailing white-space in values array_map('trim', $partRecord); //Populate data array into descriptive variables // to make processing code more intuitive list($barcode, $inStock, $qty) = $partRecord; //Add data to temp arrays $barCodes[] = $barcode; $whenClauses[] = " WHEN {$barcode} THEN {$qty}"; } //Create the single query to update ALL records $query = "UPDATE {$db}.products SET voorraad = CASE barcode" . implode('', $whenClauses) . " END WHERE barcode IN (" . implode(',', $barCodes) . ")";
-
As I said, running queries in loops is really bad - it sucks up server resources like crazy. Think about that for a minute. You are apparently running two queries to see if the value is less than or greater than the current value. Then, after doing that comparison in PHP, you are apparently running a third query to update the value. Why not just update the value no matter what? If the value is the same - so what - it will still be the same after the update. That alone would replace three queries with just one! Plus, you don't have to add additional PHP logic. $q = "UPDATE {$db}.products SET voorraad = {$voorraad} WHERE barcode = {$parts[0]}"; But, that still doesn't solve your problem of running queries in a loop. Trying to update multiple records with different values in one query is a little tricky but is possible. I'll have to research a minute and I'll post back with some sample code.
-
Can there be 2 while loops in a single code?
Psycho replied to genzedu777's topic in PHP Coding Help
That code makes no sense. I've alredy provided code in a separate thread. This is the wrong approach to what you are trying to accomplish. -
According to your vardump it is a string. Let's do some debugging to see what may be the problem. Add this line just before the return in the function I provided. $data['string'] = $string; Then do a print_r() of the return value. If the 'string' index has no value then you are passing an empty string into the function. If it shows the expected data, then it may be that some of the "spaces" in that string aren't really spaces. There are several "white-space" characters that may look like spaces in the rendered code. When you did the vardump did you look at the HTML source code or only the result displayed in the browser??? There may be multiple spaces, linebreaks, tabs, etc. in that string. But, when rendered in a browser they are reduced to a single space. Do a vardump again and check the HTML source code and post what is there.
-
Why limit yourself to just those values. Go ahead and parse the entire string. You never know when you may need those other values. Use the following function to return an array of all the parameters (as keys) and their values function getServerData($string) { $data = array( 'hostname' => "~hostname: (.*?) version :~", 'version' => "~version : (.*?) secure udp/ip :~", 'secure ip' => "~secure udp/ip : (.*?) map :~", 'map' => "~map : (.*?) at:~", 'at' => "~at: (.*?) players :~", 'players' => "~players : (.*) #~" ); foreach($data as $varName => $pattern) { preg_match($pattern, $string, $matches); $data[$varName] = $matches[1]; } return $data; } $input = "hostname: [GE] 24/7 OFFICE N00b Training Facility - GangsterElite.com version : 1.0.0.58/15 4426 secure udp/ip : 74.121.47.242:27016 map : fy_dustdm at: 0 x, 0 y, 0 z players : 0 (20 max) # userid name uniqueid connected ping loss state adr"; $serverData = getServerData($input); print_r($serverData); Ouput: Array ( [hostname] => [GE] 24/7 OFFICE N00b Training Facility - GangsterElite.com [version] => 1.0.0.58/15 4426 [secure ip] => 74.121.47.242:27016 [map] => fy_dustdm [at] => 0 x, 0 y, 0 z [players] => 0 (20 max) )
-
First, why are you making this hard on yourself (and us). Don't use the numeric index of the database results - use the field name. It makes it so much easier to understand the code. Plus, why would you create a new table row for a hidden input??? Also, you don't need the hidden fields. You just need to create the checkboxes correctly. 1. Create the checkbox names as an array 2. Set the value of the checkbox as the value you are currently assigning to the hidden input. Only the checked checkboxes and their values will be posted to the processing page. So, you can simply iterrate through all of the POSTED values from the checkboxes using the array you created in the checkbox names Form page: $i = 0; while($arr = mysql_fetch_array($result, MYSQL_NUM)) { $table .= "<tr>\n"; . " <td width='5px';><input type='checkbox' name='transcheck[]' value='{$arr[9]}'></td>\n" . " <td id='parent{$i}A'>{$arr[0]} {$arr[1]}</td>\n" . " <td id='parent{$i}B'>{$arr[2]}</td>\n" . "</tr>\n" } $table .= "</table>"; echo $table; Processing page: $checkedList = ''; foreach($_POST['transcheck'] as $value) { $checkedList .= "<li>{$value}</li>\n"; } echo "The following values were checked:<br>\n"; echo "<ul>{$checkedList}</ul>\n";
-
Assuming the new tables has the fields I described previously you can create all the records (associating every product type with every scent) with a single query. INSERT INTO products_table (type_id, scent_id) SELECT t.type_id, s.scent_id FROM prod_types_table AS t JOIN scents_table AS s
-
OK, I guess I misunderstood the intent of the header level checkboxes. I still don't understand the purpose. Let me ask you, would there ever be a situation where you would want the "Preschool" checkbox selected and the none of the subjects under preschool to be checked? In other words, is that checkbox supposed to represent that the tutor teaches one or more subjects for that level? If so, then that checkbox (and subsequent table) are still not needed since you can ascertain that information according to what subjects they are associated with. Not without understanding the purpose of the level checkboxes. But, if it truely is independant from the subjects, then you would just handle them separately from the code I provided. However, you would need to add an additional section to the SELECT query to get that data. Thenin the update code you would need two additional queries just as I did for subjects. Except in this case you would have one to delete all the current level records for the tutor and then one to insert all the selected levels.
-
You shuld never run queres in loops. 99% of the time there is a way you can achieve the same results by running one query. The other 1% of the time you are just plain trying to do somethign you shouldn't be. Just looking at those two queries, I don't see why you need both. Why not a single query looking for all records greater than and less than the specified value. But, as I said above you would not want to be runnign queries against each value in the import file. Please explain what you are trying to accomplish and I'll try and provide some code or direction.
-
It would be an alternative. But, I would not consider it a good alternative. You are using a database - at least use it in a manner that will give you the most benefit. By "making up" ids by combining two different IDs you don't have any logical entities in the database for each unique product. If you ever need to store data for each unique product (e.g. inventory) how will you store it? If you used your concatenation method, if you need that data it would be a multi-step process to query the product types and scents. Then in the PHP code you would combine those values to get all the product IDs, then you'd have to run another query to get the data.
-
Well, it seems you have narroed the source down to the server. And, I do understand their position since 99% of problems are most likely due to coding problems. So, you have a few different options. Each may, or may not, be possible in your sitution. In order of preference (for me) would be: Option 1: Get the host to fix the problem. This is going to require you to provide evidence. More on this below. Option 2: Change hosts. I'm guessing this may not be within your control. But, if you can prove it is the host and they will not fix it, then perhaps you can get the person with the authority to make this decision. Option 3: Change the code to work-around the problem. Off the top of my head, one possibility would be to use cookies instead of session variables. Just be sure not to store sensitive data in the cookie. It isn't the best solution for saving login state, but it will work. Option 4: Live with it. So, I would guess your first order of business is to gather the evidence. I'd probably set up a very simple script that records as much info as I would think would be appropriate. Record session variables, cookie values (there is a client side cookie with the session ID), and possibly some server side variables. So, I would have that script record the values to a log file and then set the page up to refresh every xx seconds. Let the page run for 20-30 minutes. For the session values, I'd probably store the timestamp on everey page load and a random number. Then on each page load check if those value are set and logging the results. As I said, there are probably some server-side variables that may be of use, but I don't know. I suggest you do some research as to the backend processes regarding session data as to what might be useful. After you run that script for a while, check the log for any problems. You could then provide that log and the script to the host to illustrate that there is a problem. So, just be sure to keep the script simple. If they have to do any analysis of the code to understand it they are less likely to take the time. Good luck.
-
The manual? http://php.net/manual/en/book.mcrypt.php
-
Didn't I post the code already? Let me state again. You Regex had some minor problems but the way it was written it was supposedly checking to see if there were any NON-ALPHA characters. So, the statements in the IF and ELSE conditions were backwards. IF there was a match, then you want the message that there were non-alpha characters, ELSE the string only contained alpha characters. As, I also stated that is exactly how you want to do that validation - look for any character OTHER THAN alpha characters rather than ensure that all the characters are alpha. It is basically the same check, but programatically it is different. The code I provided should do what you want.