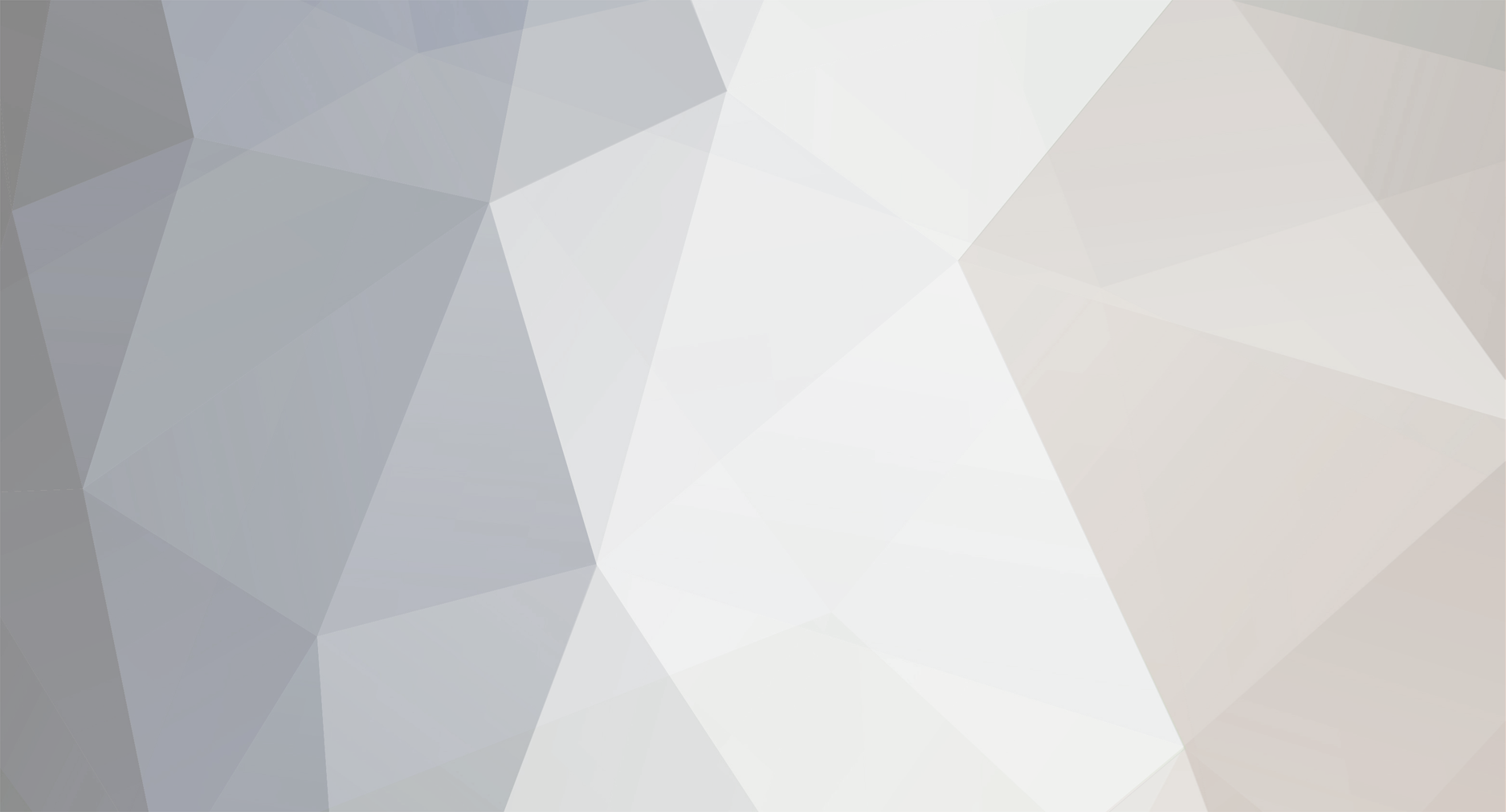
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, I do take offense. The example I provided was a complete, customizable working example. Just copy/paste the code into an HTML document and you caould have verified that the code does replace all instances of the search word. And THAT was based upon the initial code you provided which used the "g" paramater to make the replacements "global".
-
Below is a working example. Also, I would add the word boundry to the expression ("\b", but need to use "\\b" to escape the backslash). Otherwise, if you were replacing "car" with "auto" and the word "cartoon" existed in your output, you would end up with "autotoon". <html> <head> <script type='text/javascript'> function replaceWord() { //Get input values var searchWord = document.getElementById('search').value; var replaceWord = document.getElementById('replace').value; var inputText = document.getElementById('input').value; //Create reg ex expression and perform replacement var replaceRegEx = new RegExp('\\b'+searchWord+'\\b', 'g'); var outputText = inputText.replace(replaceRegEx, replaceWord); document.getElementById('output').value = outputText; } </script> </head> <body> Input:<br /> <textarea id="input" style="width:400px;height:100px;">The quick brown fox jumped over the lazy dog</textarea> <br /><br /> Output:<br /> <textarea id="output" style="width:400px;height:100px;" disabled="disabled"></textarea> <br /><br /> Search Word: <input type="text" id="search" value="quick" /><br /> Replace Word: <input type="text" id="replace" value="slow" /> <br /><br /> <button onclick="replaceWord();">Replace</button> </body> </html>
-
You are correct in how you think that statement is interpreted, but it is the comparison that is boolean (true or false) and it is the same comparison either way. The term for the solution I provided is called the ternary operator. Many people refer to it as a shorthand if/esle statement, but that is an understatement in my opinion.
-
I'm not sure I understand. You state you want to change a form field when it has focus, but there is no form field in your code. Plus, I'm not sure what you mean about "when enter is clicked...". Are you taking about the user CLICKING something on the page or when the user PRESSES the Enter key on the keyboard?
-
After furhter review I suspect the root fo the problem is in the form page. You may be getting the same type of notification errors - but they may be masked in the HTML code, thereby corrupting the input data. Kind of hard to know for sure without debugging it. Another possibility is that the host may have enabled/disabled magic quotes which would make the input data different from what you were receiving before. What is the output of the print_r()? Is it what you would expect?
-
Your host has probably modified the default error reporting to show notifications (which is kind of odd in my opinion). You should definitely have error reportingset to show everything in a development environment, but in a production environment notification errors should be suppressed. But, the problem is that on first access of the page the POST values don't exist. So, when you try to set $username = $_POST['username']; you are getting the error because $_POST['username'] doesn't exist. The right way to do that would be $username = isset($_POST['username']) ? $_POST['username'] : false; But, you shoudl rework the logic further. Te current page does a db query regardless if the user submitted login credentials or not. You should only do the authnetication if the user submitted the form.
-
You will need server-side code to build the select list instead of hard-coding it. <?php $statusOptionsList = array('', 'Ongoing', 'Completed'); $statusOptionsHTML =''; foreach($statusOptionsList as $option) { $selected = ($userValueFromDB==$option) ? ' selected="selected"' : ''; $statusOptionsHTML .= "<option value=\"{$option}\"{$selected}>{$option}</option>"; } ?> <select name="status"> <?php echo $statusOptionsHTML; ?> </select>
-
Constructing SQL search strings from multiple html form elements?
Psycho replied to DCM's topic in PHP Coding Help
First, determine what fields to search, THEN add the WHERE and AND clauses if necessary. Also, I would add validation of the select field inputs to ensure the user has submitted a value that is in the list (malicious users can submit data not in your select list). //Construct WHERE clause parts $whereParts = array(); if(isset($_POST['name']) && strlen(trim($_POST['name']))>0) { $whereParts[] = "`name` = '".mysql_real_escape_string(trim($_POST['name']))."'"; } if(isset($_POST['site_location']) && $_POST['site_location'] != 'all') { $whereParts[] = "`site_location` = '".mysql_real_escape_string(trim($_POST['site_location']))."'"; } if(isset($_POST['job_title']) && $_POST['job_title'] != 'all') { $whereParts[] = "`job_title` = '".mysql_real_escape_string(trim($_POST['job_title']))."'"; } if(isset($_POST['manager']) && $_POST['manager'] != 'all') { $whereParts[] = "`manager` = '".mysql_real_escape_string(trim($_POST['manager']))."'"; } if(isset($_POST['email']) && strlen(trim($_POST['email']))>0) { $whereParts[] = "`email` = '".mysql_real_escape_string(trim($_POST['email']))."'"; } //Construct the final WHERE clause $WHERE = (count($whereParts)>0) ? 'WHERE '.implode(' AND ', $whereParts) : ''; //Construct the query $query = "SELECT * FROM employees {$WHERE}"; -
I think you are still not understanding the core issue. Using isset() IS the solution. You DO NOT want to use register globals. Using that opens your site to vulnerabilities. The problem is that it may take a lot of code review to find all the places where you need to implement a fix. Let me give one last example to hopefully illustrate the problem. Again, let's assume that there is an $action variable used to determine what to display on a page. The value may be set usign $_GET or $_POST, or both, and possibly other means. By having register globals turned on you would have to know all the possible ways in which the value could be set. Because of register gloabls there is no direct indication of how the variable is being set. But, to make matters worse, it seems that the value of $action is not required to be set! In the cases where it is not set, the application follows some default logic. So, you might see this in the code. if($action=='admin') { //display admin results } else { //display user results } So, in that case, the else statement will be the default. However, if you are always testing as an admin you would never get the notification error (if register globals was on).
-
need to get 2 words together from explode not just one, how to do it?
Psycho replied to tastro's topic in PHP Coding Help
So, why would you concatentate empty strings at the beginning and end??? Why not just do this: $combinations[] = $pieces[$x].' '.$pieces[$y]; But to explain the {}, when using double quotes to define a string PHP will parse any variables in the string. It makes it much easier, in most instances, and much more readable to just include the variable in the string rather than exiting and entering out of quotes as you did. But, when including variables within double quotes there are times when a variable can be misinterpreted - such as if you want other characters butted up against the string value or with the case of array values using a key enclosed in single quotes. To prevent that you can enclose the variables in curly braces - {}. So, I make it a habit to always enclose my variables in curly braces. -
need to get 2 words together from explode not just one, how to do it?
Psycho replied to tastro's topic in PHP Coding Help
Here you go: $pizza = "piece1 piece2 piece3 piece4"; $pieces = explode(' ', $pizza); $combinations = array(); for($x=0, $pieceCount=count($pieces); $x<$pieceCount; $x++) { for($y=$x+1; $y<$pieceCount; $y++) { $combinations[] = "{$pieces[$x]} {$pieces[$y]}"; } } print_r($combinations); Output: Array ( [0] => piece1 piece2 [1] => piece1 piece3 [2] => piece1 piece4 [3] => piece2 piece3 [4] => piece2 piece4 [5] => piece3 piece4 ) -
need to get 2 words together from explode not just one, how to do it?
Psycho replied to tastro's topic in PHP Coding Help
I misread your first post. I thought you just wanted the first two words, the second two words, etc. I just finished the regex solution for that. Oh well. Anyway, you apparently want each word paired with every other word, right? If so, your original example doesn't show piece1 with piece 3. Your results are different than what I had - you must have changed the code I gave you. Give me a minute to see what I can come up with. -
need to get 2 words together from explode not just one, how to do it?
Psycho replied to tastro's topic in PHP Coding Help
You could either explode it as you are and then loop through the array to concatenate them or you could use a regular expression. Here is how you can do it by using explode and a littel post processing $pizza = "piece1 piece2 piece3 piece4"; $words = explode(' ', $pizza); $twoWords = array(); for($i=0, $wordCount=count($words); $i<$wordCount; $i+=2) { $twoWords[] = $words[$i] . ((isset($words[$i+1])) ? " {$words[$i+1]}" : ''); } -
PHP counting script not including subdirectories, help!
Psycho replied to tigertim's topic in PHP Coding Help
It works perfectly fine for me. Are you sure you counted the files and folders correctly? You could add some debugging info to see what it is actually counting. Add the debugging line as shown here { (is_file($object)) ? $fileCount++ : $folderCount++; //Debugging echo "{$object} : " . ((is_file($object)) ? 'FILE' : 'FOLDER' ) . "<br />\n"; if($recursive) { If you can identify a file or folder that is not being counted then we can determine what the problem is. -
Ok, you really don't want to run queries in a loop. It really puts a strain on the server. And that logic is very overcomplicated. Not sure why you are tracking two different totals, one for today and another for total. I assume you have a nightly query that runs to empty the today value. Anyway, set the field 'today' and 'total' to have default values of 1. You only then need three fields: word, today and total. The word field should be the primary field. You can then do what you need with just the following: //process the words into array of query values $valueParts = array(); foreach(explode(' ', trim($var)) as $word) { if($word!='') { $valueParts[] = "('{$word}')"; } } //Create combined query $VALUES = implode(', ', $valueParts) $query = "INSERT INTO searchedwords (`word`) VALUES {$VALUES} ON DUPLICATE KEY UPDATE today=today+1, total=total+1"; $result = mysql_query($query) or die(mysql_error()); The result of that much shorter and efficinet script would be that any word which does not ecurrently exist in the table will be added with values of '1' for today and total. If a word does exist, the values for today and total will be incremented by 1.
-
PHP counting script not including subdirectories, help!
Psycho replied to tigertim's topic in PHP Coding Help
Right now your function simply uses glob() to get a list of ALL the objects in a folder. So, your function is returning the number of files and folders in the directory. So, you will need to iterate over each object returned in glob and increment the file count if it is a file. If an object is a directory, then you call the function again using that directory. That is what is meant by being recursive. Instead of making the function overly complex based upon whether you want to get the count of files, folder or both; I rewrote it to always return both plus the total. Then you can decide which vlaue to display as needed. The second parameter is to specify if you want to count the objects in the subfolders (i.e. be recursive) function directoryCount($directory, $recursive=false) { $objects = glob($directory.'/*'); if($objects === false) { user_error(__FUNCTION__."(): Invalid directory ($directory)"); return false; } $fileCount = 0; $folderCount = 0; foreach($objects as $object) { (is_file($object)) ? $fileCount++ : $folderCount++; if($recursive) { $subFolderCount = directoryCount($object, $recursive); $fileCount += $subFolderCount->files; $folderCount += $subFolderCount->folders; } } $output->files = $fileCount; $output->folders = $folderCount; $output->total = ($fileCount+$folderCount); return $output; } $folderCount = directoryCount('images', true); if($folderCount === false) { echo "<p>Oops....something went wrong.</p>\n"; } else { echo "<p>There are {$folderCount->files} files and {$folderCount->folders} folders in the Image folder. That is a total of {$folderCount->total}</p>\n"; } -
I wasn't knocking your code, just pointing out that the current database design makes this a very resource intensitive tasks. Running a script such as the one you provided once a night to update the results would be one way to mitigate the problems. Although I think by making a small chnage to the database and adding the ability to capture the metrics in real time will provide the best results.
-
The point PFMaBiSmAd was trying to make is that if that variable is relying up REGISTER_GLOBALS to be turned on you won't find that variable being set anywhere. Example: Let's say a user clicks the following link http://www.mysite.com/index.php?action=deleterecord In that script you could access the value of action, as passed through the GET parameter, using $_GET['action']. But, with register globals on, that value is also automatically set in a local variable with the same name: i.e. $action. So, you wouldn't see a $action = $_GET['action'];
-
That is going to be a hugh resource hog over time. Not only do you have to loop through all the records, you also have to explode the records and loop over that result. You shouldn't have to loop through all the records to find the top items - a database can do that dynamically. But, you can't do it with the current database structure. I think you can make this much easier with one small change in the db. Create another table called search_words with the following columns: - search_id (foreign key back to the seach table) - word - count Then whenever anyone performs a search, in addition to adding a record to the search table you would explode the search term and INSERT the words into this secondary table using ON DUPLICATE KEY so new words will be added while existing words will have their count incremented (the word field would be the key). After creating the table you coul dalso create a one time script to add the records from all the current searches. Then, if you need to words most commonly searched you can just do a single query of the search_words table ordering the results according to the count value.
-
I really like helping people, but c'mon could you not take 5 minutes to figure out the problem yourself? You need to learn how to effectively debug code if you are going to program. You didn't even provide enough information for me to reproduce the defect. You should have stated that you set the date to Jan 31st since that was the only date in January that caused the problem. And, I was easily able to discover why by just modifying the $dateValue to $dateValue = date("Y-m-d", strtotime("-{$monthsBack} month")); I then saw the results as 2010-01-31 2009-12-31 2009-12-01 I don't know if this is a PHP bug or not. But, instead of trying to fight it a simple code change would fix it. Just force the base date to be the first day of the current month. <?php $numberOfMonthsToDisplay = 3; $dateOptions = ''; for($monthsBack=0; $monthsBack<$numberOfMonthsToDisplay; $monthsBack++) { $firstDayOfMonth = date("Y-m-1"); $dateValue = date("Y-m", strtotime("$firstDayOfMonth -{$monthsBack} months")); $dateOptions .= " <option value=\"{$dateValue}\">{$dateValue}</option>\n"; } ?> <select name=""> <?php echo $dateOptions; ?> </select>
-
Those are just notifications - not really errors. You are only seeing them because you changed the error reporting to E_ALL. Basically the notification is that you are tying to use/compare a variable that has not been set. It is a notification because you *may* have created a typo and really want to be comparing a different variable. Example: $myVar = 1; //Note V is uppercase if($myvar==1) //Note: V is lowercase { //Do this } You could make a modification of those comparisons to prevent the notification if (isset($action) && $action=="logout") <div align="center"><br><br><?php echo (isset($package))?$package:''; ?><br><br> Or just set $action and $package as '' somewhere at the beginning of the script.
-
Let me pull out my crystal ball so I can see what the error messages are ... that might take a while. You could just tell us what the error messages are.
-
Or, for a better programatical solution you could use the code below. That way you can easily modify how many options are displayed in the list by modifying the value of $numberOfMonthsToDisplay. <?php $numberOfMonthsToDisplay = 3; $dateOptions = ''; for($monthsBack=0; $monthsBack<$numberOfMonthsToDisplay; $monthsBack++) { $dateValue = date("Y-m", strtotime("-{$monthsBack} month")); $dateOptions .= " <option value=\"{$dateValue}\">{$dateValue}</option>\n"; } ?> <select name=""> <?php echo $dateOptions; ?> </select>
-
I suspect the problem is a difference between the date/time settings on your PC vs the Server. For example, in the US Jan. 5th, 2010 would be represented as 1-5-2010, whereas in most European countries it would be represented as 5-1-2010. So, I imagine your PC's settings recognizes "2010-09" as September 2010 and the settings on the Server do not. But, all of that can be solved by removing the overcomplication from what you have. You are converting dates back and forth unneccessarily. Try this: <select name=""> <option><?php echo date("Y-m"); ?></option> <option><?php echo date("Y-m", strtotime("-1 month")); ?></option> <option><?php echo date("Y-m", strtotime("-2 months")); ?></option> </select>
-
I thought you were changing the current page assignment to use a GET variable instead of a SESSION variable. But, I still see this in your code $current_page = (isset($_SESSION['pg'])) ? (int) $_SESSION['pg'] : 1; What is happening is that when you open the other page, that page is starting at page 1 and resetting the session variable to page 1. So, when you go back to the original window and hit the next page link it is going to page 2 (which is the next page from page 1 as stored in the session variable). I stated previously that I didn't know why you would be using SESSION to store the current page number, but I assumed you had a reason for doing so. You need to think about how stored data should be used and the appropriate method for storing it. If a user is expected to open multiple browser windows with different data from the same site you should not be using SESSION variables to store any parameters for that data. I suppose you could use SESSION variables if you wanted to build it so that each windows/gid had it's own namespace in the session data. But, that would seem to just overcomplicate this more than it needs to be.