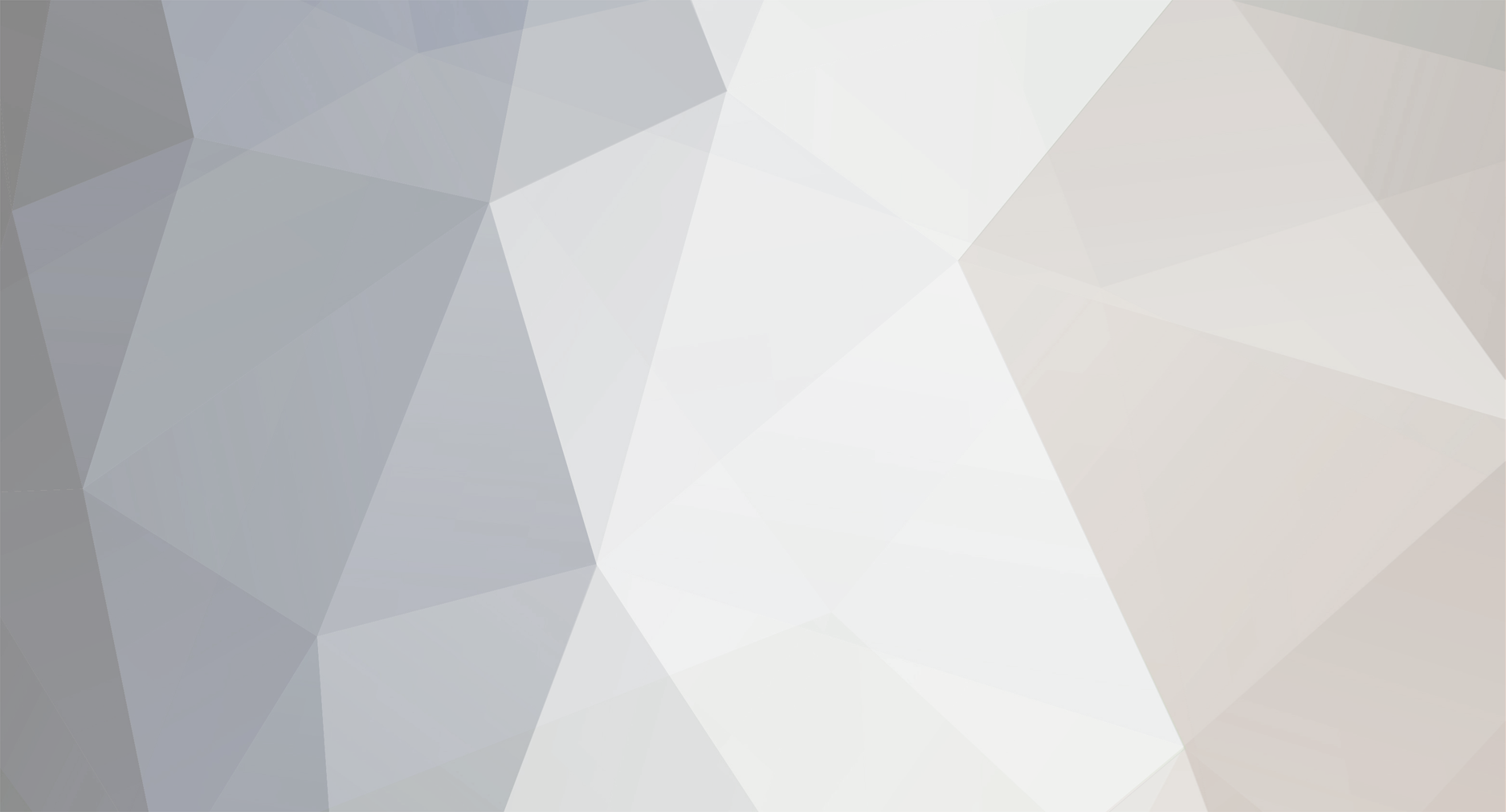
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
email validation function does not allow something@360.com address
Psycho replied to jasonc's topic in Regex Help
Also, if you are using PHP 5.2.0 or later you might want to try using the filter_var() function with the FILTER_VALIDATE_EMAIL flag. http://us.php.net/manual/en/filter.examples.validation.php I have not used it myself to test how comprehensive it is. -
email validation function does not allow something@360.com address
Psycho replied to jasonc's topic in Regex Help
Here is the one I have developed and continued to improve along with a complete explanation of how it does the validation. // NOTES: // // Format test // - Username: // - Can contain the following characters: // - Uppercase and lowercase English letters (a-z, A-Z) // - Digits 0 to 9 // - Characters _ ! # $ % & ' * + - / = ? ^ ` { | } ~ // - May contain '.' (periods), but cannot begin or end with a period // and they may not appear in succession (i.e. 2 or more in a row) // - Must be between 1 and 64 characters // - Domain: // - Can contain the following characters: 'a-z', 'A-Z', '0-9', '-' (hyphen), and '.' (period). // - There may be subdomains, separated by a period (.), but the combined domain may not // begin with a period and they not appear in succession (i.e. 2 or more in a row) // - Domain/Subdomain name parts may not begin or end with a hyphen // - Domain/Subdomain name parts must be between 1-64 characters // - TLD accepts: 'a-z' & 'A-Z' (2 to 6 characters) // // Note: the domain and tld parts must be between 4 and 255 characters total // // Length test // - Username: 1 to 64 characters // - Domain: 4 to 255 character //===================================================== // Function: is_email ( string $email ) // // Description: Finds whether the given string variable // is a properly formatted email. // // Parameters: $email the string being evaluated // // Return Values: Returns TRUE if $email is valid email // format, FALSE otherwise. //===================================================== function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } -
Instead of defining $userClass before the switch, just change the assignments in the switch to "=" instead of ".=" For the second error, I should have used implode() instead of print_r(). $output .= implode("\n"; $parentData['records']);
-
Well, unless the user is changing pages you don't need to pass the page variable. If the user is selecting a different letter or other filter criteria you want to go back to page 1. So your code woul dlook something like this $current_page = (isset($_GET['page']))?$_GET['page']:1; The only place you would need to pass the page variable is when the user selects a next or previous page or selects an actual page to navigate to. So, just modify those links as needed. Example echo "<a href=\"thispage.php?page=".($current_page-1)."\">Next Page</a>";
-
OK, I'm assuming English is not your primary language because I don't understand your variable names which makes it difficult to understand. It looks like you are storing the current page as a session variable, but are passing other parameters for filtering via GET. Not sure why you woul dneed to store the page number as a session variable. You could pass the page variable as an additional GET parameter. If it is set then override the session value or don't use the session variable at all. Alternatively you could save the last used "letter" to a session variable. Then when the script detects that the new letter is different than the last then it should default to page 1.
-
Using the beginning of the text message to determine the recipient would appear to be a weak method due to possible spelling errors. Using separate addresses for each recipient would at least allow the user to save the address for subsequent use. Also, I see no way to do this (easily) by sending the SMS to a phone number. I think you are going to have to have them send it to an email address. So, I think the solution to both of the problems above is . . . drumroll . . . GMail! Create a GMAil account, let's say it is send_to@gmail.com. Now, if someone wants to send a message to john they would use the address send_to+john@gmail.com. To send a message to susy the user would use send_to+suzy@gmail.com. GMail has a feature that allows you to append a tag/label to the username with the plus symbol. BUt, the email will still go to the original address. (There are many uses for this). So, the second part of the equation is how do you recieve those emails. You can use PHP to access those emails: http://www.google.com/search?source=ig&hl=en&rlz=1G1GGLQ_ENUS398&q=php+read+gmail&aq=f&aqi=g1&aql=&oq=&gs_rfai=CxpqntZSjTNvvBYW8NqL3wOYIAAAAqgQFT9CN9BY Then it is just a matter of determining which user was identified in the receiving email address and forwarding to the appropriate person. Of course, you will need to identify each person and what their phone number is and their carrier. Most/all carriers allow SMS messages to be sent to their subscribers via email, e.g. 3124567378@sms.verizon.com (just an example).
-
The specifics of what you are asking (color selection, comparing, etc.) are irrelevant. If you don't have a clue on how to get started I would assume you lack the basic skills needed to start such a project. You will need to learn the basics. I'd suggest finding a tutorial on PHP/MySQL to learn the basics. There are some such tutorials that use a registration/login as the example for the tutorial. Go find one of those and you can use the code you generate from the tutorial as the beginnings of your site. Then work on adding the additional features. If you get stuck on a specific problem post a question here with a description of what you are trying to solve, what code you have and what problems errors you are having.
-
You are referencing the GET values incorrectly. You are missing the "$_". I would suggest also using trim(). $first_name = trim($_GET["first_name"]); $last_name = ($_GET["last_name"]);
-
Yes. But, I'd add a semi-colon and quote marks as well - just to follow good programming standards. <?php echo $row['title']; ?> Which is why we are stating you will need to do a system wide search and replace.
-
Grabbing Html Table and putting it into Drop down Box!Emergency!
Psycho replied to Deanznet's topic in PHP Coding Help
Um, I don't expect this is what you are looking for, but you didn't do a very good job of explaining your problem: <select> <option value="Yamaha WR450F 2003-2006">Yamaha WR450F 2003-2006</option> <option value="Yamaha WR450F 2003-2006">Yamaha WR450F 2003-2006</option> </select> I assume the values in the table are coming from somehwere, but you don't provide that information. Are you reading it from a database, are you reading a file with the actual HTML above, or what??? -
Just to clarify what PFMaBiSmAd is advising. The first replacement will change all short tags from <? to <?php. But if you have any "regular" tags, they will be changed from <?php to <?phpphp which is why he has the second replacement. Then, of course, the short tags used to echo text are changed from <?= to <?php= which is why the third replacement is listed above. That should work. But, call me a nervous Nellie, but I never do such system-wide search and replaces. I would be concerned that there might be a valid use of "<?" somewhere in the code which has nothing to do with the short tags. So, I would use a search and replace utility but validate each replacement before it is made. Of course, it shouldn't need to be said, but you should only do the replacements on copies of the files so you can revert back if needed.
-
The PHP.ini file is a server configuration file - it is not configured for the site or reside in the site's public directory. If you are on a shared host you will likely be out of luck in getting them to change it since it affects all websites on that server. If you have a dedicated server with this new host they should have no problem changing that setting for you. I don't believe there is a way to change the behavior at run time. But, the best solution is to just remove the short tags anyway. If there is just this one instance of that problem, then change that code from <?= $row[title] ?> To this <?php echo $row[title]; ?> But, I would probably do a search of all the files for short tags. There are many free utilities out there that can do this for you. Search & Replace is one that comes to mind (I think it's still available).
-
Ahh, yes, you have stumbled across the notorious problem of short tags. Instead of using <?php //Code goes here ?> Some programmers would use <? //Code goes here ?> Also, the short tags had the added benefit of easily inserting dynamic text using <?=$variable?> instead of <?php echo $variable; ?> But, short tags are considered bad practice and that setting is no longer turned on by default. With that optioned turned off on your new host, the short tags are not recognized as the start of PHP code and the code inside them is not processed. Check this manual page on how to set that option. Although, it might be better to just find and replace all instances of short tags. Then you never have to worry about this again if you change servers. http://php.net/manual/en/ini.core.php
-
And, just to clarify, you would run that query and simply check if the number of results (i.e. mysql_num_rows()) is 0 (no matches) or >1 (there is a match). Here is some sample code to illustrate: $query ="SELECT `name` FROM `table` WHERE `name` = '$name' OR `email` = '$email' OR `phone` = '$phone' OR `website` = '$website'"; $result = mysql_query($query); if(mysql_num_rows($result)>0) { //ERROR: There are existing records with that data } else { //OK: There are no existing records with that data }
-
adding new field to page. how to specify the width instead of cols of box
Psycho replied to jasonc's topic in Javascript Help
Replace form_field.cols=20; With this (using your preferred value) form_field.style.width = '350px'; -
First, you should not put javascript code directly in an elements event parameter; the event should call a function instead. Specific to your problem though, you should use the onchange event of the select object. The options of a select list do not "officially" have an onclick event. However, each browser manufacturer tweaks the standards to their liking. So what may work in one browser may not work in another. The option objects do have an onchange event, but I would stick to the onchange of the select object. <select name="permission" onchange="hidePermissionTable(this);"> <option value="1">Admin</option> <option value="2">Moderator</option> <option value="3">User</option> <option value="-1">Banned</option> </select> Then have this function function hidePermissionTable(this) { var displayVal = (this.options[this.selectedIndex].value==-1) ? 'none' : ''; document.getElementById('permissions').style.display = displayVal; return; }
-
Well, the proper syntax would be if (in_array("?", $row)) {do something} But, that would only check the first record. You would have to loop through all the records in the result set to see if the record exists. However, you are going about this the wrong way. Instead you should craft the query to find the records with the value directly. Are you searching one field for a value SELECT * FROM table WHERE filed1='$searchValue' Or all fields SELECT * FROM table WHERE field1 = '$searchValue' OR field2 = '$searchValue' OR field3 = '$searchValue' OR field4 = '$searchValue'
-
OK, here is a rewrite of the functionality to display the records. I hope it is in a logical format to understand. Basically, the code will loop through the records from the query and create the necessary HTML code and add it to an array. Whenever a change in the parent_id is identified the array is passed to a function to create the total output for the parent ID and all the associated records. The array is then reset and the loop continues with compiling the records for the next parent ID. I did this all without testing as I don't have your database, so I am sure there are some typos to fix <?php function createTable($parentData) { //Exit if this is the first record if($parentData['parent_id']===fales) { return false; } $output = "<table class=\"forum_table\" onclick=\"expandCollapseTable(this)\">\n"; $output .= "<tr id=\"tr1\">\n"; $output .= "<th class=\"forum_left_corner\"></th>\n"; $output .= "<th class=\"forum_parent_name\">{$parentData['parent_name']}</th>\n"; $output .= "<th class=\"empty\"></th>\n"; $output .= "<th class=\"empty\"></th>\n"; $output .= "<th class=\"forum_last_post_header\">".LAST_POST."</th>\n"; $output .= "</tr>\n"; $output .= print_r("\n"; $parentData['records']); return $output; } //Create and run query to get all the data $query = "SELECT f.forum_id, f.forum_name, f.forum_description, f.forum_topics, f.forum_posts, f.forum_last_poster, f.forum_last_post_time, f.forum_last_post, p.parent_id, p.parent_name, m.user_id, m.user_username, m.user_group, t.topic_id, t.topic_name, po.post_id, po.post_subject FROM ".DB_PREFIX."forums as f JOIN ".DB_PREFIX."parents as p ON f.parent_id = p.parent_id LEFT JOIN ".DB_PREFIX."members as m ON f.forum_last_poster = m.user_id LEFT JOIN ".DB_PREFIX."topics as t ON f.forum_id = t.forum_id LEFT JOIN ".DB_PREFIX."posts as po ON po.post_id = f.forum_last_post ORDER BY p.parent_id"; $forum_info = $db->query($query) or trigger_error("SQL", E_USER_ERROR); //Loop through records to process data $parentData = array('parent_id' => false); while ($forum_info = mysql_fetch_object($forum_info_query)) { //Detect if this record is in a new parent id from the last if($forum_info->prent_id!=$parentData['parent_id'}) { //Create table output for last parent data echo createTable($parentData); //Set new parent ID/name $parentData['parent_id'] = $forum_info->prent_id; $parentData['parent_name'] = $forum_info->prent_name; //Reset the data array $parentData['records'] = array(); } //Create url string $forum_url_name = str_replace(' ', '_', $forum_info->forum_name); //Create HTML output for current record $recordHTML = " <tr class=\"gradient\">\n"; $recordHTML .= " <td class=\"forum_icon\">\n"; $recordHTML .= " <div class=\"thread_icon\">{$forum_info->forum_id}</div>\n"; $recordHTML .= " </td>\n"; $recordHTML .= " <td class=\"forum_name\">\n"; $recordHTML .= " <p class=\"forum_name\">\n"; $recordHTML .= " <a href=\"index.php?forum=\{$forum_info->forum_id}&name={$forum_url_name}\">{$forum_info->forum_name}</a>\n"; $recordHTML .= " </p>\n"; $recordHTML .= " <p class=\"forum_description\">{$forum_info->forum_description}</p>\n"; $recordHTML .= " </td>\n"; $recordHTML .= " <td class=\"forum_topics\">{$forum_info->forum_topics}<span class=\"small_word\">".TOPICS."</span></td>\n"; $recordHTML .= " <td class=\"forum_posts\">{$forum_info->forum_posts}<span class=\"small_word\">".POSTS."</span></td>\n"; $recordHTML .= " <td class=\"forum_last_post\">\n"; if(!$forum_info->forum_last_poster || $forum_info->forum_last_poster==0) { $recordHTML .= "<p class=\"noposts\">".NO_POSTS."</p><p class=\"be_the_first\">".BE_FIRST."</p>\n"; } else { $forum_last_post_clean = str_replace(' ', '_', $forum_info->user_username); $last_post = date("F j, Y", strtotime($forum_info->forum_last_post_time)); if ($last_post == date('F j, Y')) { $last_post = 'Today at '.date("g:i a", strtotime($forum_info->forum_last_post_time)); } $recordHTML .= "<p class=\"last_post_name\">\n"; $recordHTML .= "<a href=\"index.php?topic={$forum_info->topic_id}&name={$forum_last_post_clean}\">{$forum_info->post_subject}</a>\n"; $recordHTML .= "</p>\n"; switch($forum_info->forum_last_poster) { case 1: $userClass .= 'admin'; break; case 2: $userClass .= 'mod'; break; case 3: case 0: default: $userClass .= 'user'; break; } $recordHTML .= "<p class=\"posted_by\">Posted By - <span class=\"{$userClass}\">{$forum_info->user_username}}</span></p>\n"; $recordHTML .= "<p class=\"last_post_date\">{$last_post}</p>\n"; } $recordHTML .= "</td>\n"; $recordHTML .= "</tr>\n"; //Add the record HTML to records array $parentData['records'][] = $recordHTML; } //Display records for last parent ID echo createTable($parentData);
-
Jeez, what's your problem? I've tried to be helpful and you are the one who became argumentative. I suggest you take a step back and reread this post and see who was being "unfriendly". You stated you had a problem getting the records you wanted and I provided the queries you needed. You then stated that the output was not what you wanted and I stated you would have to modify the code that generates the output for the single query. You then gave a brief explanation of how you wanted the output displayed but didn't povide a specific question. I don't know if you were wanting code to be provided or not. Personally, I don't have a problem if you were. I will routinely provide rewrites of code for people, even when it is not asked for, if I think that trying to explain every change needed will be time consuming. So, I stated I didn't have time to rewrite it and gave a general description of the process that would need to be implemented. I take it from your response of "i never asked you to rewrite the code, i asked for help on my code and why it is behaving as such" that you took my statement negatively. But, I had already stated the code would need to be rewritten and gave a description of how that process would work. And, kickstart has now provide a rewrite which I assume will work. I will admit I was a little peeved when you suggested not once, but twice that you had to run the queries in a loop after I had already explained that was not the case - especially after you verified that the query I provided did in fact return all the records. The fact that I have a Guru badge doesn't mean crap. But, if you are going to continually challenge something at least have some data to back it up.
-
As I already explained, yes, you can get all the data in a single query. And, that is how you should do it. I don't understand why you are asking why this is bad practice. I already stated in a separate thread of yours that Try running a single query with all the data and running the queries in a loop as you have it then add some metrics gathering into your scripts to see how long they take to run. You will find that as the data grows the time to execute will grow at an exponential rate. Not to mention the CPU and memory load that those cycles will consume. If you have a moderately successful site that type of code can bring it to a crawl. You already stated that all the records are returned in the query I gave you. What remains now is to rewrite the logic that formats the output so the records from the same parent are in the same table. That will require a rewrite of your existing logic.
-
No, show the output from the query. Attach as a file if it is large.
-
Well, yeah. That is the pointer to the results of the query. Sorry, but I don't have the time to invest in rewriting the code to work with a single query. It is the best process though. You just need to use a flag when looping through the records to identify when the primary ID changes so you can start/end a table as needed.
-
You're using the second query - and just running it once to get all the records? They should be ordered by the parent ID as specified in the last line of the query. I have no idea what you want the data to look like, but if you have ALL the data in a single query you would just need to modify the code that generates the output. Well, you're wrong. Not trying to be an a$$, but that statement is simply not true. The query may need to be tweaked, but you don't need to run multiple queries in loops for what you are trying to achieve.
-
How to convert jpeg/jpg format to png/gif transparent format
Psycho replied to fifin04's topic in PHP Coding Help
imagecolortransparent(): http://php.net/manual/en/function.imagecolortransparent.php Do you have some logic in mind to determine "which" color should be made transparent? For example, if you decide that white should always be transparent, then that is simple enough. But,the image could have white that is part of the background and white that is part of the image itself. It would be difficult, if not impossible to programatically determine which is which and even if you could it would be difficult to make some portions of the same color transparent while making other portions not transparent. For example, in this image you would want to make the outside white color transparent, but not the white which makes up "Quaker State". You will also have a heck of a time dealing with the vignette -
Need some advice/suggestions on template syntax.
Psycho replied to newbtophp's topic in PHP Coding Help
Since these primarily used as placeholders I don't see why you would have the designer enter in hard coded text. Instead, have the designer define two messages: $logged_in_msg & $not_logged_in_msg Then, assuming there is a "system" variabled called $logged_in which has a value of true or false, the designer could do something like this: [ if ($logged_in) { $logged_in_msg } else { $not_logged_in_msg } ] Although, I would probably modify that after I develop the code to actually make the replacements for the if/else conditions. That process will probably reveal a more elegant way of creating the if/else conditions that will be easy to code for.