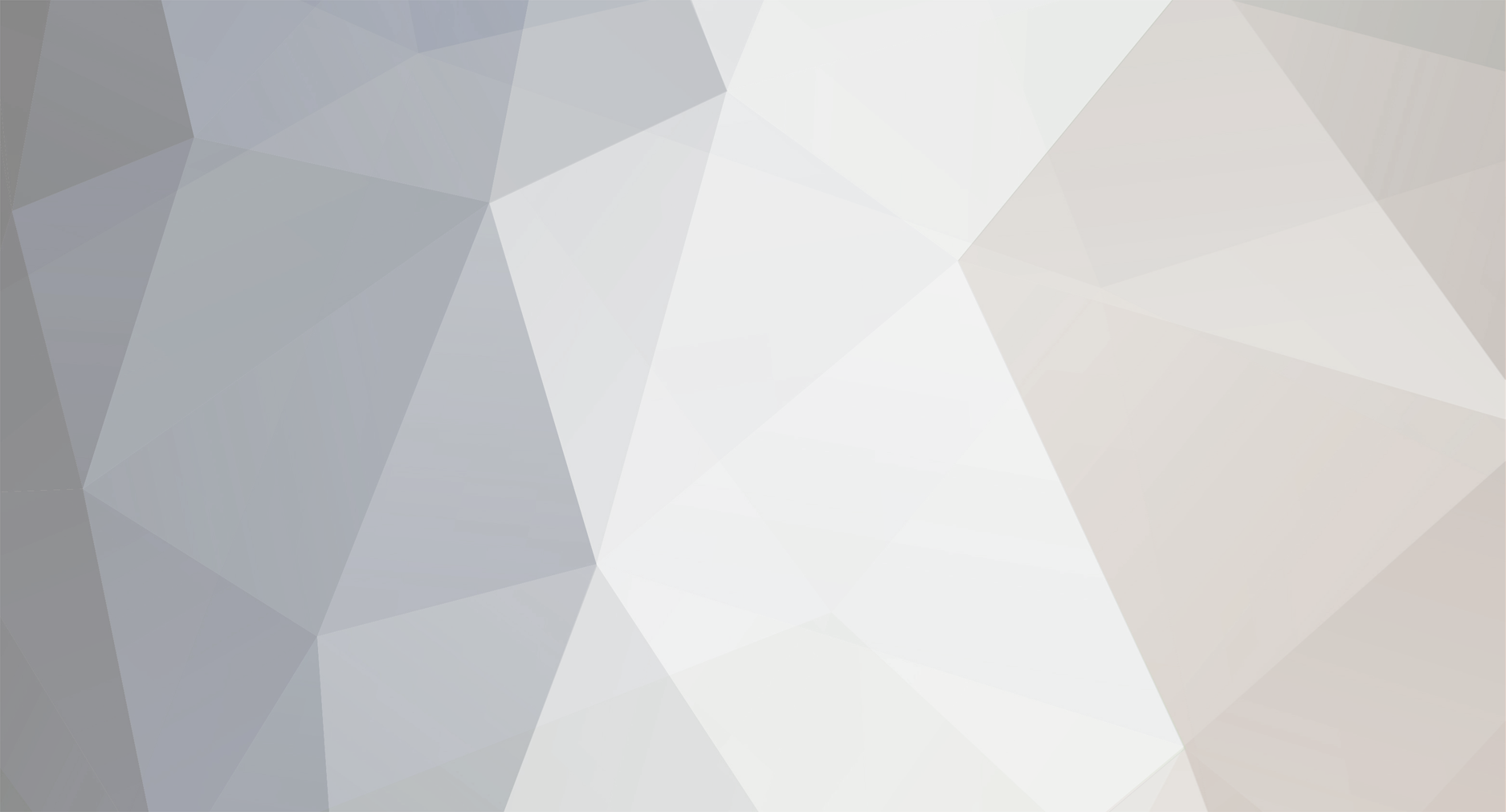
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Save the POSTed data to the session. Then when the page loads you can set the previously selected values using the SESSION data.
-
You don't necessarily need to reset a select list if the user chooses to use the other list. Any fieds that are disabled do not post their values. So, you could do something as simple as this: <html> <head> <script type="text/javascript"> function chooseSelect(selectOption) { document.getElementById('select1').disabled = (selectOption!=1); document.getElementById('select2').disabled = (selectOption!=2); } </script> </head> <body> <form action="" method="post"> Select 1 (Letters) <input type="radio" name="selectOpt" value="1" onclick="chooseSelect(this.value);" /><br /> Select 2 (Numbers) <input type="radio" name="selectOpt" value="2" onclick="chooseSelect(this.value);" /><br /> <select name="select1" id="select1" disabled="disabled"> <option value="A">A</option> <option value="B">B</option> <option value="C">C</option> </select><br /> <select name="select2" id="select2" disabled="disabled"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> </select><br /> <br /> <button type="submit">Submit</button> </form> </body> </html> However, if you want to reset the unselected list for aesthetic reasons, I would do this: <html> <head> <script type="text/javascript"> function chooseSelect(selectOption) { select1Obj = document.getElementById('select1'); select2Obj = document.getElementById('select2'); select1Obj.disabled = (selectOption!=1); select1Obj.selectedIndex = (selectOption!=1) ? 0 : select1Obj.selectedIndex; select2Obj.disabled = (selectOption!=2); select2Obj.selectedIndex = (selectOption!=2) ? 0 : select2Obj.selectedIndex; } </script> </head> <body> <form action="" method="post"> Select 1 (Letters) <input type="radio" name="selectOpt" value="1" onclick="chooseSelect(this.value);" /><br /> Select 2 (Numbers) <input type="radio" name="selectOpt" value="2" onclick="chooseSelect(this.value);" /><br /> <select name="select1" id="select1" disabled="disabled"> <option value="A">A</option> <option value="B">B</option> <option value="C">C</option> </select><br /> <select name="select2" id="select2" disabled="disabled"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> </select><br /> <br /> <button type="submit">Submit</button> </form> </body> </html>
-
Here is a function to do what you want. Simply get the entire journal entry from the database and use this function to just display the teaser: //Return a string up to a certain number of characters //but will break on a space function subStringWords($string, $length, $ellipse='...') { if (strlen($string) <= $length) { return $string; } return array_shift(explode("\n", wordwrap($string, $length))) . $ellipse; } Just pass the function the string (obviously) and the max length of characters allows. Example: echo subStringWords($journalText, 200);[code=php:0] Optionally, you can specify the string to be appended at the end of the shortened text. By default the function will use ellipses. But, you could pass anything you want - even an empty string (or a link to the full text) [code=php:0]$linkToFullEntry = " [<a href=\"viewEntry.php?id={$entryID}\">Read Entry</a>]"; echo subStringWords($journalText, 200, $linkToFullEntry);[code=php:0]
-
How do I extract the data from my database query?
Psycho replied to Canadian's topic in PHP Coding Help
Try using fetch_object: $npn_count_query = "SELECT * FROM npn ORDER BY count DESC LIMIT 1"; $npn_count_result = $db->query($npn_count_query); $npn_count_dataObj = $npn_count_result->fetch_object(); echo $npn_count_dataObj->field1; echo $npn_count_dataObj->field2; echo $npn_count_dataObj->field3; Replace field1, field2, field3 with the field names from your database that you need to access. -
I'll be rude. Do you even read your code? There are so many errors in that I don't know where to begin. There are simple syntax errors and there are significant logic problems. Take this example: mysql_select_db("homebudget", $connection) //... $slq = " DROP DATABASE IF EXIST 'homebudget' CREATE TABLE 'expenses' ( Category varchar (10), espenseData DATE, expenditure INT )"; $result=mysql_query (4sql, $connection) You first connect to the database "homebudget", then a little bit later you delete it and try to run another query. To which database would you be running that query since you just deleted the one you connected to? I understand that you are still trying to learn, but I count at least 4 typos/syntax errors that have nothing to do with being new to PHP: $result=@mysqlquery ($sql, $connection) or die (mysql_error()); It is mysql_query() mysql_select_db("homebudget", $connection) Missing a semi-colon at the end of the line $slq = " You meant to use $sql $result=mysql_query (4sql, $connection) or die (mysql_error()); Should be $sql Take your time and check your work. Learn how to debug. Write some code and test it. When you have IF/ELSE conditions, create the necessary parameters to get the results of each condition.
-
Help with logic layout of fields and tables please
Psycho replied to jonnypixel's topic in PHP Coding Help
Just create another field for the listing table to identify if the lead is active or not. Change the status from true to false to remove the active lead. Then change your query as follows: $query = "SELECT * FROM lead WHERE lead_listingID={$urlListing} and active=1"; -
There are a LOT of errors in that bit of code. First, off the reason for your current error is this line: $msg="<P>Database HomeBudget has been created!</P>; You need a double quote at the end of the line. You also have this line $result= mysqlquery ($sql, $connection) or die (mysql_erro()); It should be mysql_query() and mysql_error()
-
When you have a PHP parse error, the line number given is not always the line where the error occurs. It is the line where it can no longer interpret the code in the context given. The line you provided shouldn't cause a PHP error - but it might produce a MySQL error. Post 5-10 lines of code before that line. Most likely you have brackets or quote marks that did not start/end properly. Also, I would change that line above to this: $sql="SELECT players.first, players.last, players.address, players.city, players.postal, players.phone, players.feet, players.inch, players.yyyy, teams.teamname, teams.church, teams.division FROM players, teams WHERE players.teamid = '{$_SESSION['teamid']}' AND teams.teamid = '{$_SESSION['teamid']}'"; Notice th brackets around the variables. I would do that since you have the variables (which have single quotes) enclosed in single quotes as well.
-
When you query a database, a result is created and what is returned is a resource identifier pointing to the result. So, when you run this: $npn_count = $db->query($npn_count_query); You get a pointer to the result - NOT the result itself. You are apparently using a class to do your database functions, so you should look into what methods you should be using to extract the database results.
-
How do I split an array into a string without using foreach ?
Psycho replied to jamesxg1's topic in PHP Coding Help
Isn't that what a loop i supposed to do? Why are you thinking that foreach would be a bad solution? However, based upon the code posted I am assuming you are wanting to do multiple string replacements. If so, then you can use arrays as the search and replacement values. The problem you have with the current setup is that your actual search values are enclosed in brackets. So, you would have to modify the values of your search values. $search = array_keys($parts); foreach($search as $key=>$value) { $search[$key] = "{".$value."}"; } echo str_replace($search, $parts, $file) . '<br /><br />'; -
I don't use JQuery, but I suspect it has the same quirks when dealing with radio groups as when using straight JavaScript. Radio groups are just that - groups. As such the group is referenced as a single opbject with multiple child objects - much like an array. You cannot reference the group to see if any single option is selected. You have to check each option. The easiest solution is to just check one of the optins by default as it is impossible (from the UI perspective) to unselect all th eoptions. You can only select a different option. If you stil lwant to have them all unchecked to begin with, then you will need to iterrate through each of the options to see if one is selected. Here is a javascript function I use to return the selected value of a radio group. If none are selected, the function returns false. You might be able to rewrite that for JQuery. function radioGroupValue(groupObj) { //Check if ther is only on option (i.e. not an array) if (!groupObj.length) { //Only one option in group return (groupObj.checked) ? groupObj.value : false; } //Multiple options, iterate through each option for (var i=0; i<groupObj.length; i++) { //Check if option is checked if (groupObj[i].checked) { //Return value of the checked radio button return groupObj[i].value; } } //No option was selected return false; }
-
http://www.tizag.com/phpT/filewrite.php
-
Here is an all-in-one page. Modify for your needs. Note: I did not include any validation of the user input. <?php $birthdate = explode('-', $_POST['name']); $day = $birthdate[0]; $month = $birthdate[1]; $year = $birthdate[2]; $today = mktime(); $birthDateThisYear = mktime(0,0,0,$month,$day); if ($birthDateThisYear<$today) { $nextBirthDate = mktime(0,0,0,$month,$day,date('Y',$today+1)); } else { $nextBirthDate = $birthDateThisYear; } $daysTillNextBirthday = floor(($nextBirthDate-$today)/60/60/24); ?> tml> <head> <title>Birthdate Generator</Title> </head> <body> <?php echo "Days till next birthday: {$daysTillNextBirthday}"; ?> <form action="birthday.php" method="post"> <h1>The number of days until your birthday!</h1> <p><strong>When is your next birthday dd-mm-yyyy</strong><br> <input type="text" size="25" name="name"></p> <p><input type="submit" value="send"></p> </form> </body> </html>
-
Two things jump out at me: First, on all of your INSERT queries you are not enclosing the value of the title in quotes (you are exiting the quoted sting to append the value). mysql_query("insert into categories (category_name, parent_category) values (".dbquote($cat_name).",$category_id)") or mysql_fail(); Should look something like this mysql_query("insert into categories (category_name, parent_category) values ('".dbquote($cat_name)."',$category_id)") or mysql_fail(); Also, where is the function dbquote()? It may have a flaw in it.
-
And what debugging steps have you performed? You can't just write (or copy-paste) code and just "give up" when it doesn't work right. There are many simple steps you can take to identify the problem. Add some debugging code to the script to the code to echo out variable values, show when certain conditions are met, etc. For eaxample, if you have an IF statement, put an echo right after the condition to display that the condition passed: if (isset($_POST['Add Details'])){ echo "'Add Details' POST value is set."; In this particular case, the script I provided would not have any output if all the conditions and validations passed. Also, if you did not add the code for the form in the last condition or use an include() for the form, then nothing would be output. If you did not include the form code, then the validation might be failing. If the code for the form IS there, then your results make no sense. The only way to get a blank page would mean a record WAS inserted, but you say it isn't. I've modified slightly to add some rudimentary debugging: <?php $debugMode = true; function debug($msg) { if ($debugMode) { echo "<div style=\"background-color:yellow;\">$msg</div><br />\n"; } return; } //Set default state for $show_form $output_form = true; //Form was posted if (isset($_POST['Add Details'])) { debug("Post value for 'Add Details' is set."); //Validate input if (empty($_POST['cartons']) || empty($_POST['areaha']) || empty($_POST['tonnage']) || empty($_POST['domestic_sales']) || empty($_POST['exports']) || count($_POST['facilities'])<1) { debug("Validation failed. One of posted values is empty."); //Validation failed echo 'Please fill out your carton information.<br />'; $output_form = 'yes'; } else { debug("Validation passed. None of the posted values are empty."); // Connect to the database $dbc = mysqli_connect('hostname', 'username', 'password', 'databasename') or die('Error connecting to MySQL server.'); //escape the input $cartons = mysql_real_escape_string(trim($_POST['cartons'])); $areaha = mysql_real_escape_string(trim($_POST['areaha'])); $tonnage = mysql_real_escape_string(trim($_POST['tonnage'])); $domestic_sales = mysql_real_escape_string(trim($_POST['domestic_sales'])); $exports = mysql_real_escape_string(trim($_POST['exports'])); foreach($_POST['facilities'] as $key => $value) { $_POST['facilities'][$key] .= mysql_real_escape_string(trim($value)); } $facilities = implode(', ', $_POST['facilities']); //Create and run the query $query = "INSERT INTO production (cartons, areaha, tonnage, domestic_sales, exports, facilities) VALUES ('$cartons', '$areaha', '$tonnage', '$domestic_sales', '$exports', '$facilities')"; debug("Insert Query: {$query}"); mysqli_query($dbc, $query) or die ('Data not inserted.'); mysqli_close($dbc); } } if ($output_form) { debug("Data not posted or validation failed. Show the form."); //Include the form } ?>
-
It depends on what you mean by "save". I would assume you would want to save it on the server (i.e. using PHP or some other server-side technology). If so, you could simply have a JavaScript function that runs onsubmit of the form and selects all the elements in the list box. Then simply capture the posted values and save in a database, flat-file, etc. However, on the off chance that you aren't doing anything with the values, you could just use JavaScript to save the values to a cookie. You would probably want to modify the current script that adds/removes values to modify the cookie whenever a change is made.
-
You need to create a reference to the window when opening it. You can then use that reference to see if the window was ever opened, is currently open, or has been closed. Here is a quick script that can work with multiple windows (tested in IE and FF) <html> <head> <script type="text/javascript"> var windowObjs = new Array(); function openWindow(windowName) { windowObjs[windowName] = window.open('somepage.htm', windowName); } function windowExist(windowName) { if (!windowObjs[windowName]) { alert('The window "' + windowName + '" has not been opened yet'); } else if(!windowObjs[windowName].closed) { alert('The window "' + windowName + '" is open'); } else { alert('The window "' + windowName + '" has been closed'); } return; } </script> </head> <body> <button onclick="openWindow('test1');">Open Window Test1</button> <button onclick="openWindow('test2');">Open Window Test2</button> <br /><br /><br /> <button onclick="windowExist('test1');">Status of window Test1</button> <button onclick="windowExist('test2');">Status of window Test2</button> </body> </html>
-
Again, what problems are you seeing? Are you getting errors, a blank page, what??? Looking through the code above, there are some things where the logic is flawed: 1. You are using the variable $facilities in several places, but nowhere is it defined! 2. You are checking if $cartoons is empty, but the comments would suggest that any empty field should be an error. 3. You are using $output_form as a trigger (which is good), but you are not using it enough! You should use it to also determine if you should run the query 4. The query is run no matter what. It should be within an IF conditional to ensure there are values to insert and validation passes. Here is a modification of your script based upon my assumptions. Note: Concatenating the 'facilities' values into a comma separated list is a bad process in my opinion. You shoudl save them as separate records associated with the parent record. But, I left it as is because I'm not interested in getting too involved in changing your database structure. <?php //Set default state for $show_form $output_form = true; //Form was posted if (isset($_POST['Add Details'])) { //Validate input if (empty($_POST['cartons']) || empty($_POST['areaha']) || empty($_POST['tonnage']) || empty($_POST['domestic_sales']) || empty($_POST['exports']) || count($_POST['facilities'])<1) { //Validation failed echo 'Please fill out your carton information.<br />'; $output_form = 'yes'; } else { // Connect to the database $dbc = mysqli_connect('hostname', 'username', 'password', 'databasename') or die('Error connecting to MySQL server.'); //escape the input $cartons = mysql_real_escape_string(trim($_POST['cartons'])); $areaha = mysql_real_escape_string(trim($_POST['areaha'])); $tonnage = mysql_real_escape_string(trim($_POST['tonnage'])); $domestic_sales = mysql_real_escape_string(trim($_POST['domestic_sales'])); $exports = mysql_real_escape_string(trim($_POST['exports'])); foreach($_POST['facilities'] as $key => $value) { $_POST['facilities'][$key] .= mysql_real_escape_string(trim($value)); } $facilities = implode(', ', $_POST['facilities']); //Create and run the query $query = "INSERT INTO production (cartons, areaha, tonnage, domestic_sales, exports, facilities) VALUES ('$cartons', '$areaha', '$tonnage', '$domestic_sales', '$exports', '$facilities')"; mysqli_query($dbc, $query) or die ('Data not inserted.'); mysqli_close($dbc); } } if ($output_form) { //Include the form } ?>
-
If I am understanding you correctly, that should work. You might even be able to do a header redirect from server 1 to server 2 to sort of mask it from the user, but I wouldn't guarantee that without validating it. Example: Script on server 1 for downloading files if (isset($_GET['file_id'])) { header("Location: http://www.server2.com/downloadFile.php?file_id={$_GET['file_id']}"); } Then just create the requisite script on server 2
-
In order to "read()" a file it has to be, well, read. That means the server calling the file is reading it from the external server and then serving it to the user. You are, in effect, trippling your overall bandwidth and doubling it on the server where you have limited bandwidth: Example: User requests 10MB file from server 1 Server 1 reads file from server 2 - Server 1 uses 10MB in bandwidth from download - Server 2 uses 10MB in bandwidth from upload Server 1 then serves the file to the user - Server 1 uses 10MB in bandwidth from upload So, that 10MB file just cost you 30MB of bandwidth overall and 20MB is on server 1 (where you have limited bandwidth).
-
What you are asking for and what you really want are two different things. The only way you could read a script file and determine the output of that file would be to create your own PHP parser. Good luck with that! Instead there is a much simpler solution. Just call the file via HTTP so the file is run through the server's PHP parser and you will only be reading the output. For example here is your directory structure on the server for your site: c:\websites\mydomain.com\ Let's say the file in question exists at c:\websites\mydomain.com\subfolder\somefile.php Instead of accessing the file through the directory structure $file = "/somefolder/somefile.php"; reference it via the web $file = "http://www.mydomain.com/somefolder/somefile.php"; That assumes the file is externally accessible.
-
$output = ''; $current_date = ''; while($record = mysql_fetch_assoc($result)) { //Display date ONLY IF NEW if ($current_date != $record['event_date']) { $current_date = $record['event_date']; $output .= "<b>{$current_date}</b><br>\n"; } $output .= "{$record['title']}<br>\n"; } echo $output;
-
You should define the path relative to the server - i.e. not over http. What is the actual path to the file ON THE SERVER? The location of the file shouldn't even be externally accessible over the internet if you are trying to protect it.
-
Was Working, But now it is returning a blank display?
Psycho replied to Modernvox's topic in PHP Coding Help
Corrected code below. Note, I've split the data from the logic to make management much, much easier. Change your current script file to just this: <?php //include the data file include("craiglistURLS.php"); //Set limit for posts per url $post_limit = 60; //Get url for the selected state $urls = (in_array($_POST['state'], array_keys($urlList))) ? $urlList[$_POST['state']]: array(); //Get contents for each url foreach ($urls as $url) { $html = file_get_contents("$url/muc/"); //Extract link data preg_match_all('/<a href="([^"]+)">([^<]+)<\/a>[^<]*<font size="-1">([^"]+)<\/font>/s', $html, $posts, PREG_SET_ORDER); //Limit post resutls $posts = array_slice($posts, 0, $post_limit); //Display the results foreach ($posts as $post) { echo "<a href=\"{$post[1]}\" target=\"_blank\">{$post[2]}<font size=\"3\">{$post[3]}</font></a>\n"; print "<br /><br />\n"; } } ?> Create a separate file to store all the URLs. Call it something like craiglistURLS.php. Just make sure you reference it the same in the above script. <?php $urlList = array( "AL" => array("http://auburn.craigslist.org", "http://bham.craigslist.org"), "auburn" => array("http://auburn.craigslist.org"), "bham" => array("http://bham.craigslist.org"), "columbusga" => array("http://columbusga.craigslist.org"), "dothan" => array("http://dothan.craigslist.org"), "shoals" => array("http://shoals.craigslist.org"), "gadsden" => array("http://gadsden.craigslist.org"), "huntsville" => array("http://huntsville.craigslist.org"), "montgomery" => array("http://montgomery.craigslist.org"), "ALASKA" => array("http://alaska.craigslist.org"), "anchorage" => array("http://anchorage.craigslist.org"), "fairbanks" => array("http://fairbanks.craigslist.org"), "kenai" => array("http://kenai.craigslist.org"), "juneau" => array("http://juneau.craigslist.org"), "ARIZONA" => array("http://arizona.craigslist.org"), "flagstaff" => array("http://flagstaff.craigslist.org"), "mohave" => array("http://mohave.craigslist.org"), "phoenix" => array("http://phoenix.craigslist.org"), "prescott" => array("http://prescott.craigslist.org"), "showlow" => array("http://showlow.craigslist.org"), "sierravista" => array("http://sierravista.craigslist.org"), "tucson" => array("http://tucson.craigslist.org"), "yuma" => array("http://yuma.craigslist.org"), "ARKANSAS" => array("http://arkansas.craigslist.org"), "fayar" => array("http://fayar.craigslist.org"), "fortsmith" => array("http://fortsmith.craigslist.org"), "jonesboro" => array("http://jonesboro.craigslist.org"), "littlerock" => array("http://littlerock.craigslist.org"), "memphis" => array("http://memphis.craigslist.org"), "texarkana" => array("http://texarkana.craigslist.org"), "CALIFORNIA" => array("http://california.craigslist.org"), "sfbay" => array("http://sfbay.craigslist.org"), "bakersfield" => array("http://bakersfield.craigslist.org"), "chico" => array("http://chico.craigslist.org"), "fresno" => array("http://fresno.craigslist.org"), "goldcountry" => array("http://goldcountry.craigslist.org"), "hanford" => array("http://hanford.craigslist.org"), "humboldt" => array("http://humboldt.craigslist.org"), "imperial" => array("http://imperial.craigslist.org"), "inlandempire" => array("http://inlandempire.craigslist.org"), "merced" => array("http://merced.craigslist.org"), "modesto" => array("http://modesto.craigslist.org"), "monterey" => array("http://monterey.craigslist.org"), "orangecounty" => array("http://orangecounty.craigslist.org"), "palmsprings" => array("http://palmsprings.craigslist.org"), "redding" => array("http://redding.craigslist.org"), "reno" => array("http://reno.craigslist.org"), "sacremento" => array("http://sacremento.craigslist.org"), "sandiego" => array("http://sandiego.craigslist.org"), "slo" => array("http://slo.craigslist.org"), "santabarbara" => array("http://santabarbara.craigslist.org"), "santamaria" => array("http://santamaria.craigslist.org"), "siskiyou" => array("http://siskiyou.craigslist.org"), "stockton" => array("http://stockton.craigslist.org"), "susanville" => array("http://susanville.craigslist.org"), "ventura" => array("http://ventura.craigslist.org"), "visalia" => array("http://visalia.craigslist.org"), "yubasutter" => array("http://yubasutter.craigslist.org"), "COLORADO" => array("http://colorado.craigslist.org"), "boulder" => array("http://boulder.craigslist.org"), "cosprings" => array("http://cosprings.craigslist.org"), "denver" => array("http://denver.craigslist.org"), "eastco" => array("http://eastco.craigslist.org"), "fortcollins" => array("http://fortcollins.craigslist.org"), "rockies" => array("http://rockies.craigslist.org"), "pueblo" => array("http://pueblo.craigslist.org"), "westslope" => array("http://westslope.craigslist.org"), "CONNECTICUT" => array("http://connecticut.craigslist.org"), "newlondon" => array("http://newlondon.craigslist.org"), "hartford" => array("http://hartford.craigslist.org"), "newhaven" => array("http://newhaven.craigslist.org"), "nwct" => array("http://nwct.craigslist.org"), "newyork" => array("http://newyork.craigslist.org"), "District of Columbia" => array("http://washingtondc.craigslist.org"), "DELAWARE" => array("http://delaware.craigslist.org"), "FLORIDA" => array("http://florida.craigslist.org"), "daytona" => array("http://daytona.craigslist.org"), "keys" => array("http://keys.craigslist.org"), "fortmyers" => array("http://fortmyers.craigslist.org"), "gainesville" => array("http://gainesville.craigslist.org"), "cfl" => array("http://cfl.craigslist.org"), "jacksonville" => array("http://jacksonville.craigslist.org"), "lakeland" => array("http://lakeland.craigslist.org"), "lakecity" => array("http://lakecity.craigslist.org"), "ocala" => array("http://ocala.craigslist.org"), "okaloosa" => array("http://okaloosa.craigslist.org"), "orlando" => array("http://orlando.craigslist.org"), "panamacity" => array("http://panamacity.craigslist.org"), "pensacola" => array("http://pensacola.craigslist.org"), "sarasota" => array("http://sarasota.craigslist.org"), "miami" => array("http://miami.craigslist.org"), "spacecoast" => array("http://spacecoast.craigslist.org"), "staugustine" => array("http://staugustine.craigslist.org"), "tallahassee" => array("http://tallahassee.craigslist.org"), "tampa" => array("http://tampa.craigslist.org"), "treasure" => array("http://treasure.craigslist.org"), "GEORGIA" => array("http://georgia.craigslist.org"), "albanyga" => array("http://albanyga.craigslist.org"), "atlanta" => array("http://atlanta.craigslist.org"), "augusta" => array("http://augusta.craigslist.org"), "brunswick" => array("http://brunswick.craigslist.org"), "albanyga" => array("http://albanyga.craigslist.org"), "columbusga" => array("http://columbusga.craigslist.org"), "macon" => array("http://macon.craigslist.org"), "nwga" => array("http://nwga.craigslist.org"), "savannah" => array("http://savannah.craigslist.org"), "statesboro" => array("http://statesboro.craigslist.org"), "valdosta" => array("http://valdosta.craigslist.org"), "HAWAII" => array("http://honolulu.craigslist.org"), "IDAHO" => array("http://idaho.craigslist.org"), "boise" => array("http://boise.craigslist.org"), "eastidaho" => array("http://eastidaho.craigslist.org"), "lewiston" => array("http://lewiston.craigslist.org"), "pullman" => array("http://pullman.craigslist.org"), "spokane" => array("http://spokane.craigslist.org"), "twinfalls" => array("http://twinfalls.craigslist.org"), "ILLINOIS" => array("http://bn.craigslist.org", "http://chambana.craigslist.org", "http://chicago.craigslist.org" , "http://decatur.craigslist.org" , "http://lasalle.craigslist.org", "http://mattoon.craigslist.org", "http://peoria.craigslist.org", "http://quadcities.craigslist.org", "http://rockford.craigslist.org", "http://carbondale.craigslist.org" ), "bn" => array("http://bn.craigslist.org"), "chambana" => array("http://chambana.craigslist.org"), "chicago" => array("http://chicago.craigslist.org"), "decatur" => array("http://decatur.craigslist.org"), "lasalle" => array("http://lasalle.craigslist.org"), "mattoon" => array("http://mattoon.craigslist.org"), "peoria" => array("http://peoria.craigslist.org"), "quadcities" => array("http://quadcities.craigslist.org"), "rockford" => array("http://rockford.craigslist.org"), "carbondale" => array("http://carbondale.craigslist.org"), "springfieldil" => array("http://springfieldil.craigslist.org"), "stlouis" => array("http://stlouis.craigslist.org"), "quincy" => array("http://quincy.craigslist.org"), "INDIANA" => array("http://bn.craigslist.org", "http://bloomington.craigslist.org", "http://evansville.craigslist.org" , "http://fortwayne.craigslist.org" ,"http://indianapolis.craigslist.org", "http://kokomo.craigslist.org", "http://tippycanoe.craigslist.org", "http://muncie.craigslist.org", "http://richmondin.craigslist.org", "http://southbend.craigslist.org", "http://terrahaute.craigslist.org" ), "bloomington" => array("http://bloomington.craigslist.org"), "evansville" => array("http://evansville.craigslist.org"), "bloomington" => array("http://bloomington.craigslist.org"), "fortwayne" => array("http://fortwayne.craigslist.org"), "indianapolis" => array("http://indianapolis.craigslist.org"), "kokomo" => array("http://kokomo.craigslist.org"), "tippycanoe" => array("http://tippycanoe.craigslist.org"), "muncie" => array("http://muncie.craigslist.org"), "richmondin" => array("http://richmondin.craigslist.org"), "southbend" => array("http://southbend.craigslist.org"), "terrahaute" => array("http://terrahaute.craigslist.org"), "IOWA" => array("http://ames.craigslist.org", "http://bloomington.craigslist.org", "http://cedarrapids.craigslist.org" , "http://desmoines.craigslist.org" ,"http://dubuque.craigslist.org", "http://fortdodge.craigslist.org", "http://iowacity.craigslist.org", "http://masoncity.craigslist.org", "http://omaha.craigslist.org", "http://quadcities.craigslist.org", "http://siouxcity.craigslist.org", "http://ottumwa.craigslist.org", "http://waterloo.craigslist.org"), "ames" => array("http://ames.craigslist.org"), "cedarrapids" => array("http://cedarrapids.craigslist.org"), "desmoines" => array("http://desmoines.craigslist.org"), "dubuque" => array("http://dubuque.craigslist.org"), "fortdodge" => array("http://fortdodge.craigslist.org"), "iowacity" => array("http://iowacity.craigslist.org"), "masoncity" => array("http://masoncity.craigslist.org"), "omaha" => array("http://omaha.craigslist.org"), "quadcities" => array("http://quadcities.craigslist.org"), "siouxcity" => array("http://siouxcity.craigslist.org"), "ottumwa" => array("http://ottumwa.craigslist.org"), "waterloo" => array("http://waterloo.craigslist.org"), "KANSAS" => array("http://kansascity.craigslist.org","http://lawrence.craigslist.org","http://ksu.craigslist.org", "http://nwks.craigslist.org","http://salina.craigslist.org","http://seks.craigslist.org", "http://swks.craigslist.org", "http://topeka.craigslist.org","http://wichita.craigslist.org"), "kansascity" => array("http://kansascity.craigslist.org"), "lawrence" => array("http://lawrence.craigslist.org"), "ksu" => array("http://ksu.craigslist.org"), "nwks" => array("http://nwks.craigslist.org"), "salina" => array("http://salina.craigslist.org"), "seks" => array("http://seks.craigslist.org"), "swks" => array("http://swks.craigslist.org"), "topeka" => array("http://topeka.craigslist.org"), "wichita" => array("http://wichita.craigslist.org"), "KENTUCKY" => array("http://bgky.craigslist.org","http://cincinnati.craigslist.org","http://wichita.craigslist.org", "http://eastky.craigslist.org","http://huntington.craigslist.org","http://lexington.craigslist.org", "http://louisville.craigslist.org","http://owensboro.craigslist.org","http://westky.craigslist.org" ), "bgky" => array("http://bgky.craigslist.org"), "cincinnati" => array("http://cincinnati.craigslist.org"), "eastky" => array("http://eastky.craigslist.org"), "huntington" => array("http://huntington.craigslist.org"), "lexington" => array("http://lexington.craigslist.org"), "louisville" => array("http://louisville.craigslist.org"), "owensboro" => array("http://owensboro.craigslist.org"), "westky" => array("http://westky.craigslist.org") ); ?> -
Was Working, But now it is returning a blank display?
Psycho replied to Modernvox's topic in PHP Coding Help
Well, the problem is that (as I supposed in my first post): Your regular expression is build to expect that the FONT tag immediately follows the closing A tag. However, the pages you are scraping currently have have " - " between the anchor tag and the font tag, thus the preg_match returns no results.