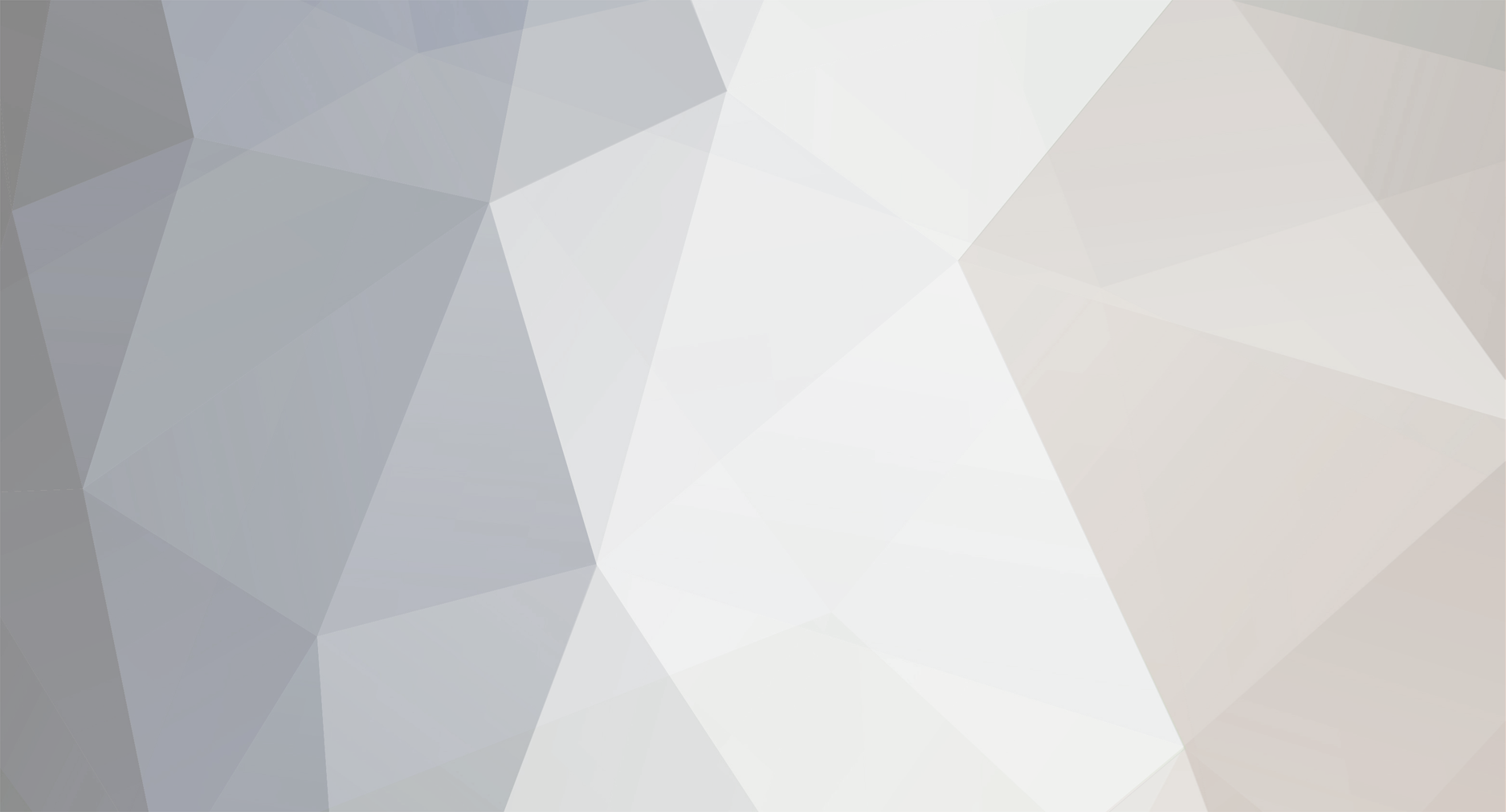
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
is it possible to rename an image after displaying ?
Psycho replied to canabatz's topic in PHP Coding Help
Ok, then, this is what I suggest - I assume you will be using a database as this will make this process much, much easier: 1. Have a database table of the images you will be using (i.e. images). 2. Have a secondary table which will be used for each viewing of the images (i.e. temp_images). 3. When a user requests a page with one of these images, use whatever logic you need to determine which image to show and a) add a record to the secondary table with a new unique ID and the ID of the image from the images table. Use the new unique ID in the image src parameter (<img src="showImage.php?id=idFromTempImagesTable"> 4. When the browser loads the image tag it will load the image by calling showImage.php. That page should use the tempImage id to lookup the real image path by JOINing the images and temp_images table and using the passed id in the WHERE clause. 5. After showImage.php serves the image to the user it should delete the record from the temp_images table If the user reloads the page or tries to access the image using the path in the image tag they will get a missing image. Although, the original image *may* show depending on how some browsers cache data. But, you can set certain headers to try and prevent that as well. -
is it possible to rename an image after displaying ?
Psycho replied to canabatz's topic in PHP Coding Help
As I stated in my first post Technically you could delete the image file after including it on a page AND after the user's browser has downloaded it, but it is no trivial task. You have to first finish the page for the user and then wait some time to actually delete the image. The user's browser has to first receive the page and then download it so the user can see the image. Only after that could you delete the image. This is possible, but it would be a lot of work. -
is it possible to rename an image after displaying ?
Psycho replied to canabatz's topic in PHP Coding Help
Yes, as p2grace stated you cannot prevent the user from loading the image independantly (i.e. copying-and-pasting the image url into the browser). I assume you are trying to "protect" your images. If so, stop right now. It is completely impossible to prevent users from getting your images. When a user views a page with images the images have already been downloaded to their PC into the browsers cache. There are many different ways I have seen sites try and prevent people from copying the images and all of them can be easily worked around. I get artwork images all the time for my music collection and not one has prevented my from getting the images I want. The most annoying is where they layer a transparent gif on top of the actual image so when I right-click to copy the image I only get the blank gif. If the "security" they have in place is too much trouble to bother with I just do a screenshot of the page and crop the image out. The only thing you can do is make it difficult for the users to resuse your images as thier own by watermarking them. -
So, just store the results in an array and reuse that array when you need the list of cities: $query = "SELECT C.City FROM Cities AS C INNER JOIN Members_to_Cities AS m2c ON C.CityID = m2c.CityID WHERE m2c.ID = '$ID'"; $citylist = mysql_query($query); if (!$citylist) die("Query to show fields from table failed."); $userCities = array(); while ($cityrow = mysql_fetch_array($citylist)) { $userCities[] = $cityrow['City']; } //Output the cities foreach($userCities as $city) { echo $city; } Additionally, if needed, you can store all the cities of multiple users into a multi-dimensional array and create a function for displaying them as needed. Assuming you need to show the cities for multiple users on a single page. Example: function showUserCities($userID, $cityArray) { foreach($cityArray[$userID] as $city) { echo $city; } } $query = "SELECT m2c.ID, C.City FROM Cities AS C INNER JOIN Members_to_Cities AS m2c ON C.CityID = m2c.CityID"; $citylist = mysql_query($query); if (!$citylist) die("Query to show fields from table failed."); $userCities = array(); while ($cityrow = mysql_fetch_array($citylist)) { $userCities[$cityrow['id']] = $cityrow['City']; } //output cities for user with id 9 showUserCities(9, $userCities);
-
What's the best way to make a multi variable search
Psycho replied to aeroswat's topic in PHP Coding Help
I'm not following your proposals. But what you are asking for is simply a pagination with one record per page. Just create your query and use LIMIT based upon the record you want to view. Also, if you want to allow multiple serach terms for the same field you would just use IN instead of an equals (i.e. =) SELECT * FROM orders WHERE itemName IN ('product1', 'product2', 'preoduct3') ORDER BY orderNumber LIMIT 0, 1 Change the 0 based upon what page you are on (i.e. page-1). Store the query parameters in session variables. -
is it possible to rename an image after displaying ?
Psycho replied to canabatz's topic in PHP Coding Help
Not sure what you are "really" trying to accomplish. You have to provide the browser with a real URL to display the image (if you want the user to actually see the image). Whatever output you send to the browser needs to have a valid path for the image and it will be available to the user. You can't change it after sending it to the browser. However, there are ways to "mask" the true image name/location on the server if that is what you are tyring to accomplish. You can hide the true location/path of the image by using an image path that goes to a PHP (or similar page) which will get the right image and read the contents to the browser. For example you might have a page called getImage.php which expects an image ID to be passed on the query string. The page could then use that ID to lookup the image in a database and find the location of the image on the server. Example: $imageID = $_GET['id']; $query = "SELECT path FROM images WHERE id = $imageID"; $result - mysql_query($query); $image = mysql_fetch_assoc($result); //Output the image header('Content-type: image/jpeg'); $imageObj = imagecreatefromjpeg($image['path']); imagejpeg($imageObj); Then in the page where you use an image it would look something like this echo "<img src=\"getImage.php?id=55\">"; -
I'd find the first wednesday on or after the begin date, then create a while loop increasing the date by 7 days until it is greater than the end date.
-
Using php include for header and navigation - bad practice?
Psycho replied to webmaster1's topic in PHP Coding Help
Search engines cannot detect what code was included and what code was not. -
Well, this will do what you ask: if(preg_match('/^\d+ [a-z]/i', $address)<1) { echo "Invalid"; } else { echo "Valid"; } But, that may be too strict. What is the address is "5200 1st Street" To allow the first character after the space to be a letter or a number use this preg_match('/^\d+ [a-z\d]/i', $address)
-
$data = "<?php\n\n"; foreach($info as $name => $value) { $data .= "\$config['{$name}'] = \"{$value}\";\n"; } $data .= "\$config['enable_comments'] = {$comments};\n"; $data .= "\$config['enable_points'] = {$points};\n"; $data .= "\$config['enable_ratings'] = {$ratings};\n"; $data .= "\$config['maintenance_mode'] = {$maintenance};\n"; $data .= "\n\n?>";
-
Here's is a function that will take an amount in any unit and conver to any other unit OR do not specify the output unit and it uses logic to convert it to the most "appropriate" unit. //This function converts untis from one measurement to another. If no output //measurement is specified, the greatest measurement with a whole number is used //PARAMETERS: // // - $outputUnits [Optional]: Will convert the value to the specified unit. // If left blank will convert to the largest possible whole unit. function convert_units($input, $outputUnits='', $precision=2) { $units = array('B', 'KB', 'MB', 'GB', 'TB'); $input = strtoupper($input); $outputUnits = strtoupper($outputUnits); //Determine amount, units and exponent of input (bytes is assumed) $inputParams = preg_split("/([\d.]+)[ ]*(\w*)/", $input, 0, PREG_SPLIT_DELIM_CAPTURE); $inputAmt = $inputParams[1]; $inputUnits = (in_array($inputParams[2], $units))? $inputParams[2] : $units[0]; $inputExp = array_search($inputUnits, $units); $bytes = ($inputAmt * pow(1024, $inputExp)); //Determine the exponent and units for the output $outputExp = (in_array($outputUnits, $units))? array_search($outputUnits, $units) : floor(log($bytes)/log(1024)); $outputUnits = $units[$outputExp]; //Detemine the converted value $outputAmt = number_format(($bytes/pow(1024, $outputExp)), $precision); return "$outputAmt $outputUnits"; } //Usage //Convert MB to KB echo convert_units("2.5MB", "KB"); //Output: 2,560.00 KB //Convert MB to GB echo convert_units("2560 MB", "GB"); //Output: 2.50 GB //Convert GB to KB echo convert_units("1.23GB", "KB"); //Output: 1,289,748.48 KB //Convert units to most appropriate value echo convert_units("2356"); //Output: 2.30 KB echo convert_units("3845475"); //Output: 3.67 MB echo convert_units("65987929 KB"); //Output: 62.93 GB echo convert_units("98566483157"); //Output: 1.80 GB
-
2996 Megabytes does not equal 2.996 Gigabytes. You need to convert using base 2. 2996 MB ~ 2.926 GB I have a function to do the correct conversions, but it relies on the input being in Bytes. Give me a moment to modify for you.
-
A few things here: 1. The $pred_rate is defined rights after the while loop THEN you include a block of code within curly braces. So, what is happening is that the PHP interpreter think that only that one line applies to the loop. Therefore, $pred_rate is being calculated for each record, then after the loop completes the data for the last record is being displayed. Using properly indented code would have made this problem apparent. 2. You do not have a closing TR tag for the display lines. Also, it would be better (IMHO) if you split out the output into multiple lines so you can see the format easier. 3. You could also change your query to derive the monthly value and not need to do anything in PHP to get the monthly value. I would also suggest using the column names in the $row values. Otherwise any changes to the column order in the database will break your code. 4. Lastly, a good practice is to do the logic first (i.e. PHP) and then do the display. Makes your code cleaner and more flexible. Revised code: <?php //Create table output while($row = mysql_fetch_array($res) ) { $pred_rate=$row[4]/2; $tableOutput = "<tr>\n"; $tableOutput .= "<td valign=\"top\">{$row[0]}</td>\n"; $tableOutput .= "<td valign=\"top\">{$row[1]}</td>\n"; $tableOutput .= "<td valign=\"top\"> {$row[2]} </td>\n"; $tableOutput .= "<td valign=\"top\">{$row[3]}</td>\n"; $tableOutput .= "<td valign=\"top\">{$row[4]}</td>\n"; $tableOutput .= "<td valign=\"top\">{$pred_rate}</td>\n"; $tableOutput .= "<td valign=\"top\">{$row[5]}</td>\n"; $tableOutput .= "<td valign=\"top\">{$row[6]}</td>\n"; $tableOutput .= "<td valign=\"top\">{$row[7]}</td>\n"; $tableOutput .= "</tr>\n"; } ?> <div align="center"> <font color="Green"><b>Search Results...</b></font> <table cellpadding="10"> <tr> <td valign="bottom"><b>Code</b></td> <td valign="bottom"><b>Comcode</b></td> <td valign="bottom"><b>Descript</b></td> <td valign="bottom"><b>Indoor Pred FIT</b></td> <td valign="bottom"><b>Outdoor Pred FIT</b></td> <td valign="bottom"><b>Outdoor Pred Rate</b></td> <td valign="bottom"><b>Pred Ref</b></td> <td valign="bottom"><b>Target FIT</b></td> <td valign="bottom"><b>Target Ref</b></td> </tr> <?php echo $tableOutput; </table>
-
You are asking a lot to be addressed in a forum post. But, if you are new to PHP I would suggest not building your own flat file database from scratch. There are some freely available modules that you can use. Just do a google seach for "php flat file database" and you'll find some good resources. But, a better question is why would you want to use a flat-file database? There are very cheap host (and even free ones I believe) that provide MySQL database support. Doesn't make sense to learn how to use some less than optimal solution when you can do it the right way.
-
Odd. The function works for me. Must be something to do with the character encoding for the page. However, here is a more complete list of character replacements. (found it here: http://www.randomsequence.com/articles/removing-accented-utf-8-characters-with-php/) <?php function friendlyName($name) { $find = explode(","," ,ç,æ,œ,á,é,í,ó,ú,à,è,ì,ò,ù,ä,ë,ï,ö,ü,ÿ,â,ê,î,ô,û,å,e,i,ø,u"); $repl = explode(",",",c,ae,oe,a,e,i,o,u,a,e,i,o,u,a,e,i,o,u,y,a,e,i,o,u,a,e,i,o,u"); return str_replace ($find, $repl, strtolower($name)); } echo friendlyName("La Coruña"); //Output: lacoruna echo friendlyName("León"); //Output: leon ?>
-
What's the point of running substr() twice? $pass = substr($pass, 8, -; //Remove first AND last eight characters
-
Combo Box only sending one variable in multipage form
Psycho replied to hugeness's topic in PHP Coding Help
You could create an onsubmit trigger that fires a function which iterates through all options in the select box and populates those values into a hidden field (delimited with a character such as a comma) Example: function populateSelectValues() { var selectField = getElementById('ts6'); var hiddenField = getElementById('hidden_ts6'); var values = new Array(); for (var i=0; i<selectField.options.length; i++) { values[values.length] = selectField.options[i].value; } hiddenField.value = values.join(); } Personally I think the original problem can be solved. It's just difficult without having all the code to work with. Plus, I just don't have the time to shift through it. -
Really? When I read his original post there is conflicting information. Besides, if the password is encoded, what is the point of putting additional random data on the ends to prevent hackers from getting the info.
-
Except for the fact that people do not use gibberish for their passwords. Even if you don't use a delimiter such as a period, the passwords would be plainly visible to anyone looking at the raw data. The values in the database would like look like the following: (d&%^KS*spasswordNs*#]?1% *'@jS7W6Go Raiders&dT$2(@| :+!.D7k#pizza\_=2J3d It wouldn't take a rocket scientist to decypher the real passwords. Hashes have been used to mask passwords in databases for years and are irreversible - i.e. you cannot decypher the real password from the hashed value. Please do not respond that MD5 can be cracked - it can't. But, if users use stupid simple passwords and you do not utilize a SALT then a lookup table can be used, but that is not the same as 'cracking' MD5. Anyway, here is an example of a typical usage //User entered password $password = "Some value by the user"; //First create a salt: append a value specific to the user or manipulate the value in some consistent manner $passWithSalt = strrev($password) . $username; //Second hash the value $hashedValue = md5($passWithSalt); So, when a user creates a password you would follow that process and save it to the database. Then when a user logs in, you follow the same process to get the hashed value and compare that to the value in the database. I will guarntee that neither you or any hacker can ever determine the user's password from what is in the database. At least not without a lot of time and processing power to create a custom lookup table of values using your salt.
-
What? Why are you trying to rebuild the wheel. Use a hash, such as MD5() or SHA() with a salt. I'm assuming the user is not aware of these added values and is just entering their normal password and you are planning to validate that against the database value by stripping off the added values, right? How is that going to thwart a hacker if they were to get into your database? Anyone intelligent enough to get into your database would be able to plainly see the pattern and figure out your 1337 encoding.
-
Create the checkboxes as an array with the value of each set to the id of the relevant record. Example Record 1 <input type="checkbox" name="idList[]" value="1" /><br /> Record 2 <input type="checkbox" name="idList[]" value="2" /><br /> Record 3 <input type="checkbox" name="idList[]" value="3" /><br /> Then on your processing page just check to make sure that the field is passed (i.e. at least one checkbox was checked) and run a single query using the array of values as part of the WHERE clause. Example if (isset($_POST['idList'])) { $idListValues = implode(',', $_POST['idList']); $query = "UPDATE table SET status=0 WHERE id IN ($idListValues)"; mysql_query($query); } Of course you need to add proper validation of the values to prevent sql injection.
-
Date function problem when switching to 2010
Psycho replied to cerebrus189's topic in PHP Coding Help
Why would you change it? The strtotime() function will "Parse about any English textual datetime description into a Unix timestamp". So, when it uses "last month" it will literally return a timestamp for last month. And, that is what you want, right? That must be some really FooBar code if it is creating a different datbase tabe for each month of records. This should work fo rthat line: $tablename = date("FY",mktime(0, 0, 0, date("m")-1, date("d"), date("Y"))); -
Date function problem when switching to 2010
Psycho replied to cerebrus189's topic in PHP Coding Help
Replace these lines //get last month for the filenames $lastMonth = sprintf("%02d", date("m") - 1); $thisYear = date("Y"); $fileLastMonth = $thisYear . $lastMonth; With these lines //get last month for the filenames $fileLastMonth = date("Ym", strtotime("last month")); -
I think the OP is asking for help in paginating the results on screen for the user - not in the actual printing to hard copy. If so, there is a tutorial on this site just for that type of functionality: http://www.phpfreaks.com/tutorial/basic-pagination