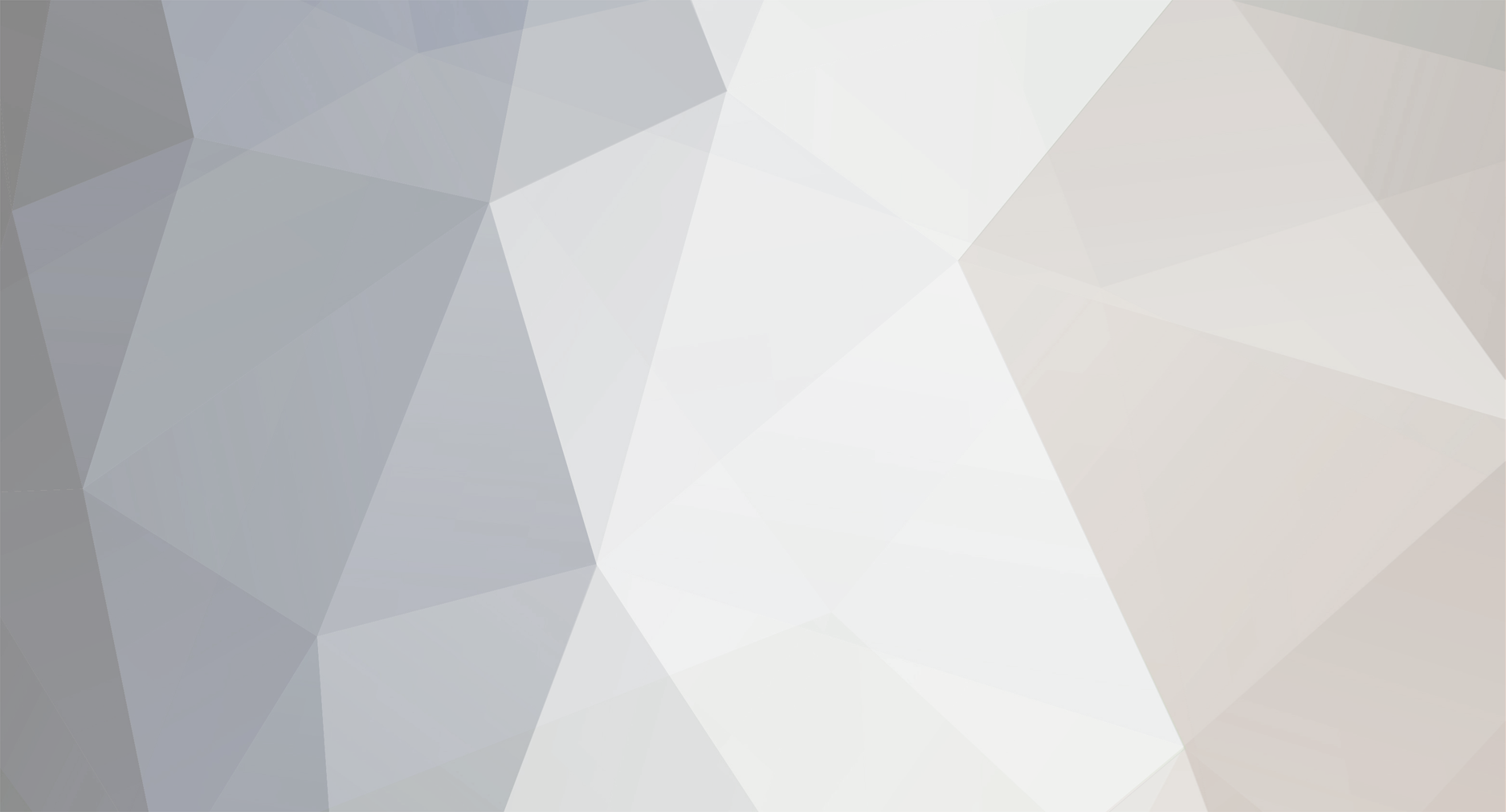
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I really can't follow where the designs come into play in your code - only the types. So, I'll just provide some "mock" code close to what you provided. As long as you don't have a very large number of records, just query all of them. Then use PHP to only show three from each type. Example: <?php function displayTypeDesigns($typeStr, $designsAry) { if (!$typeStr || count($designsAry)==0) { return false; } $typeOutput = "<b><u>{$typeStr}</u></b><br />\n"; $typeOutput .= implode(' | ', $designsAry) . "<br /><br />\n"; return $typeOutput; } $query = "SELECT * FROM design ORDER BY type, series"; $result = mysql_query($query) or die (mysql_error()); $currentType = false; $designList = array(); $output = ''; while ($row = mysql_fetch_array($result)) { extract($row); if ($currentType!=$type) { //Create output for 1st 3 records of last type $output .= displayTypeDesigns($currentType, $designList); //Reset vars $currentType = $type; $designList = array(); } //Add series to current var if less than 3 if (count($designList)<3) { $designList[] = $series; } } //Create output for 1st 3 records of last type $output .= displayTypeDesigns($currentType, $designList); echo $output; ?>
-
Combo Box only sending one variable in multipage form
Psycho replied to hugeness's topic in PHP Coding Help
When you changed the field name to "ts6[]", did you change all the event calls to use the new name? Ex: onClick="objForm.Users.transferFrom('ts6[]'); -
Combo Box only sending one variable in multipage form
Psycho replied to hugeness's topic in PHP Coding Help
Then you need to change the "ts6" field to an array: "ts6[]" -
This should work to create variables using the company names, just add to the $company array as many option as you need <?php /*Used to get the key value pair in array*/ function seekKey($array, $key, $value) { $ret = array(); for ($i=0;$i<count($array);$i++) { if ($array[$i][$key]==$value) $ret[] = $array[$i]; } return $ret; } /*Set each company as a vendor_id found in $order->products */ $companies = array(1 => 'ford', 2 => 'bmw', 3 => 'jeep'); foreach ($companies as $key => $company) { $$company = array(0=>array('vendors_id'=>'asd')); seekKey($order->products, 'vendors_id', $key); if (isset(${$company}[0]['vendors_id'])){ print ${$company} . $samething; } } ?>
-
Is there a way to read information on a website
Psycho replied to aeroswat's topic in PHP Coding Help
How about exactly that? a weather report? Say I wanted to say (Such and such news reports the weather as being 68 degrees today) Do you think that would be a big issue? It seems like I could just as easily manually type this in and show people. Doesn't seem like it would be a problem Are you doing your own weather forecasting? If you are using the content from another site you need to get permissions and, possibly, give credit. I have to believe there is some free service available to get current weather info. I'm sure you can find something with a little work. -
Combo Box only sending one variable in multipage form
Psycho replied to hugeness's topic in PHP Coding Help
Where is the field "ts6"? You didn't list it in your code above. -
Combo Box only sending one variable in multipage form
Psycho replied to hugeness's topic in PHP Coding Help
The values need to be passed as an array, therefore you need to give the field a name that will be interpreted as an array. I.e. add square brackets to the field name: <SELECT NAME="Users[]" SIZE="8" MULTIPLE STYLE="width: 250px;" onDblClick="objForm.Users.transferTo('ts6');"> You can then reference the values of the field in the processing page via $_POST['Users'], which will be an array variable -
If the $qty is not correct on the first page load, then there is a problem in how you are setting the value. You haven't provided any code to show how $qty is defined so it's difficult to provide any help.
-
Is there a way to read information on a website
Psycho replied to aeroswat's topic in PHP Coding Help
Here's a tutorial: http://www.bradino.com/php/screen-scraping/ -
Is there a way to read information on a website
Psycho replied to aeroswat's topic in PHP Coding Help
Yes, it is very possible. Just use the PHP functions to read a file, but instead of a file path use the URL to the page. You will then need to create logic to extract the information you need. How that is done is dependant upon how the site constructs the page. But, if the site ever changes the format of their page your logic will break. -
You are using mysql_connect() incorrectly. The parameters are: mysql_connect (SERVER, USERNAME, PASSWORD) You are apparently trying to pass the database name as the third parameter. You need to use mysql_select_db() after establishing the connection to select the database
-
If you are running queries within loops like that you will eventually start having pages that will timeout before loading. As for your initial request, I took it to mean you wanted the sum of all the line item totals. But, re-reading your post I think you mean you want to have the total of the number of line item units. If so, just follow the same process as you are using to get the total cost. Here's a change to the code I provided. I did not test the code (as you are apparently using a class of some sort). But, I think I found what was missing. Your code required the use of extract() on the record array (many consider the use of that function as bad practice). function printCart() { global $db; $cart = $_SESSION['cart']; if ($cart) { $contents = array_count_values ( explode(',', $cart) ); $contentIDs = array_keys($contents); $grandTotal = 0; $qtyTotal = 0; $output[] = '<form action="basket.php?action=update" method="post" id="cart">'; $output[] = '<table width="600" align="center">'; $output[] = '<tr><th>Item</th><th>Item Price</th><th>Quantity</th><th>Subtotal</th></tr>'; $sql = "SELECT * FROM products WHERE Product_ID IN (" . implode(', ', $contentIDs) . ")"; $result = $db->query($sql); while($product = $result->fetch()) { extract($product); $lineItemTotal = (($Price) * $qty); $grandTotal += $lineItemTotal; $qtyTotal += $qty; $output[] = '<tr>'; $output[] = "<td align=\"center\">{$Common_Name} ({$Genus})</td>"; $output[] = "<td>£{$Price}</td>"; $output[] = "<td><input type=\"text\" name=\"qty{$id}\" value=\"{$qty}\" size=\"3\" maxlength=\"3\" /></td>"; $output[] = "<td>£{$lineItemTotal}</td>"; $output[] = '</tr>'; } $output[] = '</table>'; $output[] = "<h2 align=\"right\">Total Price: £{$grandTotal}</h2>"; $output[] = "<h2 align=\"right\">Total Qty: £{$qtyTotal}</h2>"; $output[] = '</form>'; } return join('\n', $output); }
-
I suspect the problem is that the "Object doesn't support this property or method" I suspect you are wanting to reference the objects with IDs the same as the values in your array? When you do a For...In within JavaScript, the FOR value is not the value in the array - it is the index of the value. I think what you want to do is this: function UnfoldTree(divArray) { alert(divArray); var myArray = divArray.Split(','); for(index in myArray) { document.getElementById( myArray[index] ).style.display = 'block'; } }
-
is i change the %08s to %16s and add 8 more 9's would that be correct syntax for a 16 digit number? No, it wouldn't - the '0' is the character to be padded. Also, I had a minor typo which I fixed in my previous post. $num = strintf("%016s", (rand(0, 9999999999999999)); OR $num = str_pad(rand(1, 9999999999999999), 16, '0', STR_PAD_LEFT);
-
$num = sprintf("%08s", (rand(0, 99999999)); OR $num = str_pad(rand(1, 99999999), 8, '0', STR_PAD_LEFT);
-
This will give you a result set of the top 5 cities and the number of users associated with each SELECT city, COUNT(city) as count FROM users GROUP BY city ORDER by count DESC LIMIT 0, 5
-
Doing it within the loop makes the most sense. But, that code could be made more efficient. You should never do loops within a query unless there is no other way. Here's an example of changes I would make (not tested) function printCart() { global $db; $cart = $_SESSION['cart']; if ($cart) { $contents = array_count_values ( explode(',', $cart) ); $contentIDs = array_keys($contents); $grandTotal = 0; $output[] = '<form action="basket.php?action=update" method="post" id="cart">'; $output[] = '<table width="600" align="center">'; $output[] = '<tr><th>Item</th><th>Item Price</th><th>Quantity</th><th>Subtotal</th></tr>'; $sql = "SELECT * FROM products WHERE Product_ID IN (" . implode(', ', $contentIDs) . ")"; $result = $db->query($sql); while($product = $result->fetch() { $lineItemTotal = (($Price) * $qty); $grandTotal += $lineItemTotal ; $output[] = '<tr>'; $output[] = '<td align="center">'.$Common_Name.' ('.$Genus.')</td>'; $output[] = '<td>£'.$Price.'</td>'; $output[] = '<td><input type="text" name="qty'.$id.'" value="'.$qty.'" size="3" maxlength="3" /></td>'; $output[] = '<td>£'.$lineItemTotal.'</td>'; $output[] = '</tr>'; } $output[] = '</table>'; $output[] = '<h2 align="right">Total: £'.$grandTotal.'</h2>'; $output[] = '</form>'; } return join('\n', $output); }
-
That is not using AJAX. That code is ...drummroll please... refreshing the page. Which is why you are seeing the page refresh. You need to use a timed event to make an AJAX call. THen use that AJAX call to get the new content and update the contents of the div. A forum is not the appropriate forum in which to teach you how to implement AJAX. There are many tutorials out there on exactly the kind of functionality you are trying to implement. Here is one: http://www.w3schools.com/Ajax/Default.Asp
-
You are echo'ing the wrong values. You are hard codng the vlaues of $image and $folder $folder='foldername'; $image='image_name'; Tghe values you need to be checking are $folderPath and $imagePath. And, if $image and $folder do not have any values then something isn't right. I suspect that they are not displaying because the HTML is fouled up. It is really hard to follow your code because it is constantly going in and out of the PHP and HTML.
-
As Thorpe suggested, you need to look into AJAX. Simply create a JavaScript timed event that makes an AJAX request every n seconds. That request will return back the current content to display.
-
Your form is using the POST method, but you are trying to get the value via the $_GET variable. Either change the form method to GET or use $_POST to obtain the submitted value.
-
Correct, you are forcing the value to be an integer. So, as long as the value passed can be any integer then you don't have to use mysql_real_escape_string(). But, then again, it doesn't hurt either.
-
Most likely the PHP code is not being parsed and is being put into the page literally. The opening and closing PHP tags will prevent the code from being displayed in the browser, but I'll bet you see the code if you look at the source of the HTML page. Because you are working with a CMS application (i.e. phpBB) you need to be aware of how to include new code. The code you posted above is probably from a template file that is set up in a specific manner to be consumed by the CMS application. Look at the first couple lines: <dt>Games</dt>{$C_BLOCK_H_R}<br/> <div class="inner"> There is apparently a variable on the first line but that code is not delimited within double quotes of an echo statement, otherwise the the quotes in the second line would screw up the code. It could be that the contents of that file is read into a heredoc container. I don't think you can include PHP code into that file directly. You will need to review the structure of how the files are processed to determine where you can put PHP code to be run. Then assign the output of that code to a variable and then include in that page just like the other variables.
-
Yeah, are you sure there is no other code on the page - especially JavaScript? I'm thinking there might be something going on where the form is double posting or the page is refreshing. How exactly are you setting those $_GET values? Are you doing it through an AJAX call? Have you tried just typing in the url with the appropriate parameter into the address bar?
-
No, he is referrng to the fact that you are taking input from the user (i.e. $_GET[]) and using it directly in the query. When you do this, a user can craft their input such that they modify the query to do additional things with very bad consequences. For example, if the user entered the search string: foobar'; DELETE FROM table That would delete all the records in that table! You need to use mysql_real_escape_string() on ALL user input to make them safe for use in a query. <?php $name = mysql_real_escape_string($_GET['q']); $query = "SELECT * FROM table WHERE name='$name'"; $result = mysqli_query($connect, $query) ; ?>