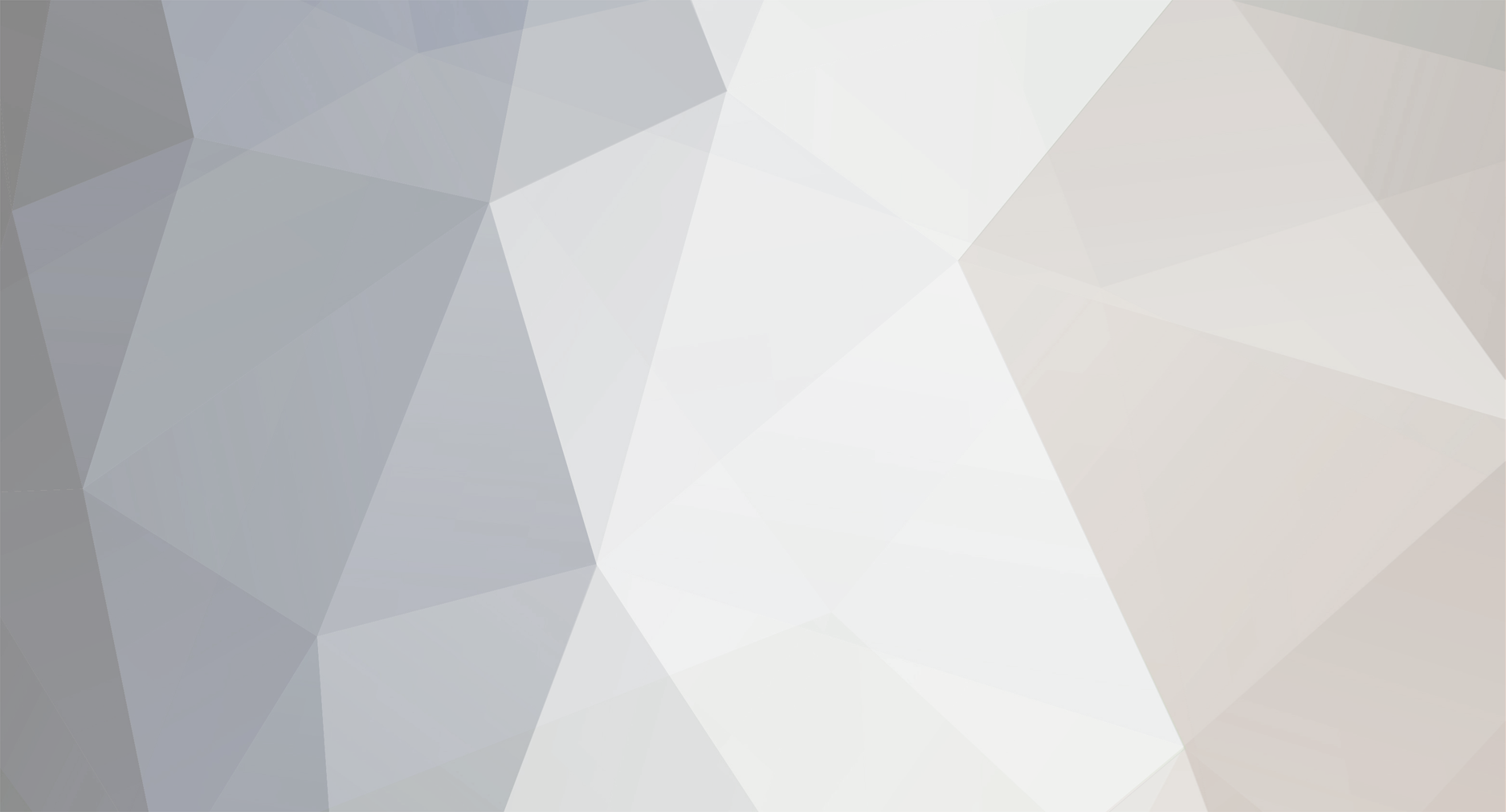
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
How do I call a function which is set in another page?
Psycho replied to c-o-d-e's topic in PHP Coding Help
Yes it is just that simple. When you "include" a php file in another it is almost exactly the same as if the code from the included file was written in-line to where it was included. -
No need to do a second query to get the perm value, just grab it with the first query. Try this <?php // Connects to your Database ... //checks cookies to make sure they are logged in if(!isset($_COOKIE['ID_staples_clubs']) || !isset($_COOKIE['Key_staples_clubs'])) { //Cookie values not set, redirect to login header("Location: login.php"); } //Cookie values are set, run query $username = mysql_real_escape_string($_COOKIE['ID_staples_clubs']); $pass = mysql_real_escape_string($_COOKIE['Key_staples_clubs']); $query = "SELECT `perm` FROM `members` WHERE `username`='$username' AND `password`='{$pass}'"; $result = mysql_query($query)or die(mysql_error()); if (mysql_num_rows($result)!=1) { //No match for Username/password, redirect to login header("Location: login.php"); exit(); } //Check for admin $userInfo = mysql_fetch_assoc($result); if ($userInfo['perm']==1) { //Redirect to admin header("Location: admin.php"); exit(); } //Not an admin, show member page echo "{$userInfo['name']} <br />\n"; echo "Welcome, "; echo "{$userInfo['adv']} <br />\n"; echo "<a href=\"edititem.php?id={$userInfo['club']}\">Edit Club</a>"; echo "<br />\n"; echo "<a href=\"changepass.php\">Change password</a><br />\n"; echo "<a href=\"logout.php\">Logout</a>\n"; ?>
-
Disabled form fields and java work in FF not in IE. WHY?
Psycho replied to pioneerx01's topic in Javascript Help
Well, the problem is that you have multiple statements on one line and are separating them with commas. You should be using semi-colons: This if(val=="Elementary"){frm.elementary.disabled=false,frm.junior.disabled=true,frm.senior.disabled=true} Should be if(val=="Elementary"){frm.elementary.disabled=false;frm.junior.disabled=true;frm.senior.disabled=true} -
If you were to provide the code you have it would be easier for us to see what you were already doing and provide an appropriate solution. Here is what I would do: //Array to hold jpeg names and associative text $imageList = array( 'image_1.JPG' => 'Text for image 1.', 'image_2.JPG' => 'Text for image 2.', 'image_3.JPG' => 'Text for image 3.', 'image_4.JPG' => 'Text for image 4.', 'image_5.JPG' => 'Text for image 5.' /// Etc. ); //Create array of just the images $images = array_keys($imageList); //Randomize the image array shuffle($images); //Get 7 random images $randomImages = array_slice($images, 0, 7); //Display the images and the text foreach($randomImages as $image) { echo "<img src=\"folderpath/{$image}\"><br />\n"; echo "{$imageList[$image]}<br />\n"; }
-
The best way to find out would be to test it.
-
Actually, you have no unique keys. The combination of two values must be unique - which ON DUPLICATE cannot work with. But, there is a simple solution - create a new, unique key using those two values. In addition to saving the user ID and the part number as individual fields, create a new column in the table where you save the value "[userID]-[partNo]". Then that field can be set up as a unique column and you can use ON DUPLICATE. Then, as in the examples I provided earlier, use PHP to create one single query using ON DUPLICATE.
-
Your code doesn't work according to your requirments. What? You are contradicting yourself. Does the "displayed" text of the link need to be 46 characters (if so your code fails) or do you want the entire code of the link (including the opening and closing tags) to be 46 characters. If it is the latter, that really makes no sense. If the total length of the text gets too bug, then you will have an empty string as the display text and the actual URL would start to get truncated. If you are only wanting the displayed text to be 46 characters or less, then the code I provided does exactly that. Your 'modified' code makes no sense. EDIT: OK after rereading your posts several times and looking closer at your code, I guess I understand what you wanted now. But, it could have been explained better. There is still a better way to do this.
-
Still playing around with this, just for my own enjoyment. Here is an improved version that will add a title to links with text that is over 46 characters. It makes the title the entire text. So, if the text is truncated the user can mouse over the link to see the entire text in a popup. $text = preg_replace("/<a([^>]*)>([^<]{46})([^<]*)<\/a>/", '<a\\1 title="\\2\\3">\\2</a>...', $text);
-
OK, it was much simpler than I thought to add the ellipses $text = preg_replace("/<a([^>]*)>([^<]{46})[^<]*<\/a>/", '<a\\1>\\2</a>...', $text);
-
That's easy enough, but I'm not sure how to add the ellipse (i.e. ...) if the length exceeds 46 characters - at least not without making the code more complex. [FYI: The "t" in the second set of letters is the 46th character] $text = 'Joe has posted in <a href="xx">abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyz</a> and also in <a href="xx">abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyz</a>, plus in <a href="xx">abcde</a>'; $new_text = preg_replace("/<a([^>]*)>?([^<]{1,46})[^<]*<\/a>/", '<a\\1>\\2</a>', $text); echo $new_text; //Output // // Joe has posted in <a href="xx">abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrst</a> // and also in <a href="xx">abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrst</a>, plus in // <a href="xx">abcde</a>
-
Help Required on PHP & Mysql Login Security Check Script
Psycho replied to j.smith1981's topic in PHP Coding Help
You've implemented my code backwards. The user should ALWAYS be directed to the logic (i.e. PHP) page login.php. The HTML page login_form.php is called by the logic when it's the first visit to that page or if validation fails. So, in that case, POST is not always set. On the first visit to the page, the logic will bypass all of the validation code and directly load the form. -
This would be much simpler if you were to post your code. Content does not magically get displayed on a web page just becuase you pulled records from a database. There must be some code that actually "prepares" the HTML code for the links. For example, does the value in the database include the entire link, i.e. everything from the opening A tag to the closing A tag? Or, does the value in the DB only include the URL and you are building the HTML anchor? The answer to that will require different solutions.
-
I was thinking of ON DUPLICATE as well, but that only works when you have a single column being used for the duplicate check, whereas it looks like this is using two fields for uniqueness: user and part number. I do see a lot of room for improvement though: First, you have the following code to read the file into a string, then you explode the string into an array. $contents = ''; while (!feof($handle)) { $contents .= fread($handle, 8192); } fclose($handle); if(empty($contents)) { return false; } // grab the items $items = explode("\n", $contents); You can replace all of that with a single line which reads the contents directly into an array using the function file() $items = file($file); Second, and this is minor, you are runnig an is_array() check after creating an array - kind of pointless. Third, you are iterrating through the data several times. Once to validate/cleant the data and then again to put the data in the DB. Do it all in one loop. Finally, and most importantly, as others have noted looping queries is probably the biggest hit inperformance. You are doing two queries for each record - one to check if the record exists and then a second to update or insert the record. Instead, always do an UPDATE query, then use mysql_affected_rows() to determine if the record already existed. If not, then add the values to an array. Once you've gone through every record you have run one query for each and all the updates are done. Then you can insert all the new records in one single query. Doing this will reduce the number of queries needed in half. Here is some modified code I put together. This is not tested though <?php function importFile($user_id, $file, $file_id) { if(!file_exists($file)) { return false; } // grab the items $recordsAry = file($file); if (count($recordsAry)<1) { return false; } foreach($recordsAry as $rKey => $recordStr) { $recordStr = trim($recordStr); if($recordStr=='') { //Remove empty lines unset($recordsAry[$rKey$rKey]); } else { //Create variable to store records to be inserted $insertValues = array(); $recordStr = str_replace("\"", "", $recordStr); $recordStr = mysql_real_escape_string($recordStr); //Split record string into fields vars $delimiter = (strstr($recordStr, ',')!==false) ? ",": "\t"; $fieldsAry = explode($delimiter, $recordStr); list($partNumber, $altPartNumber, $condition, $qty, $description) = $fieldsAry; $partNumber = $this->fixPartNumber($partNumber); $altPartNumber = $this->fixPartNumber($altPartNumber); //Run update script $sql = "UPDATE ".TABLE_PREFIX."inventory SET inventory_alt_part_number='{$altPartNumber}', inventory_condition_code='{$condition}', inventory_quantity='{$qty}', inventory_description='{$description}', last_update = NOW() WHERE user_id = {$user_id} AND inventory_part_number = '{$partNumber}'"; $result = $this->db->query($sql); //Check results if(!$result) { echo "Fail Update: {$partNumber}<br />\n"; } else if (mysql_affected_rows()===0) { //Record doesn't exist - add to INSERT values array $insertValues[$partNumber] = "('{$partNumber}', '{$altPartNumber}', '{$condition}', '{$qty}', '{$description}', NOW(), '{$user_id}')"; } else { //Record was updated echo "Updated: {$partNumber}<br />\n"; } } //Add new records if(count($insertValues)>0) { //Insert records that need to be added $sql = "INSERT INTO ".TABLE_PREFIX."inventory (inventory_part_number, inventory_alt_part_number, inventory_condition_code, inventory_quantity, inventory_description, last_update, user_id) VALUES " implode(', ', $insertValues); $result = $this->db->query($sql); if(!$result) { echo "Fail Insert: " . implode(', ', array_keys($insertValues)) . "<br />\n"; } else { echo "Inserted: " . implode(', ', array_keys($insertValues)) . "<br />\n"; } } } $sql = "UPDATE ".TABLE_PREFIX."file_upload SET file_flag = 1 WHERE file_id={$file_id} AND user_id={$user_id}"; $result = $this->db->query($sql); return true; } ?>
-
Where's your code for creating the links?
-
As you may know, when working with strings delimited with double quotes you can put variables within the string and PHP will interpret the variable into it's value. It does not do this with strings delimited with single quotes. Example: $name = "Syrehn"; echo "Hello $name"; //Output = Hello Syrehn echo 'Hello $name'; //Output = Hello $name However, there are times when PHP can't decypher where the string begins or ends or, with array values, the quotes surrounding the index name can cause the string to be terminated instead of being parsed. But, you can enclose variables within curly braces inside double quoted strings to ensure the PHP parser understands exactly where the variable is. Example: $spec = '2.2'; echo "The PC is $specghz"; //Output = The PC is //echo the var $specghz doesn't exist! echo "The PC is {$spec}ghz"; //Output = The PC is 2.2ghz As mrMarcus already stated, if you don't use quotes, PHP will try to fix it. So, it is not good practice to leave them off. As to why I used double quotes in one instance and single quotes in another was just me missing one pair. You can use either, but I typically prefer to use single quotes for text which is not dynamic and use double quotes when I want to use variables to be parsed (as explained above)
-
Please read mrMarcus's post again! Where did you lear to reference array values using $arrayVar $indexName ? In fact, you shouldn't even be getting the values at all since I don't see anywhere that $email, $phone or $job_type have been defined. Looks like extract() is being run against the $_POST variable, but it's not in your code. Are you sure that is all the code? Anyway, try this: <?php date_default_timezone_set('America/Edmonton'); $yourEmail = "shekinteriors@yahoo.ca"; //The Email Address Form Results Will Be Sent To. Change to your email. $subject = "Online Job Requests"; //This will be displayed in your Email Subject line. Edit as needed. $job_description = implode(', ', $_POST["job_description"]); $body = "Name: {$_POST['name']} \n"; $body .= "Email Address: {$_POST['email']} \n"; $body .= " Phone: {$_POST['phone']} \n"; $body .= "Job Type: {$_POST['job_type']} \n"; $body .= "Job Description: {$job_description} \n"; mail($yourEmail, $subject, $body, "From: {$_POST['name']} < {$_POST['email']} >"); $timestamp = date("n/j/Y, \a\\t g:i a T"); $thankyousubject = "Request Submitted Successfully \n on \n $timestamp"; //This will be displayed after a submission. Edit to suit your needs. The \n represent page breaks. $thankyoumsg = "Thank you {$_POST['name']} we have recieved your information. We will contact you soon."; //This will be displayed after a submission. Edit to suit your needs. ?> <html> <body style="background-color:#2c2c2c;color:#FFFFFF"> <center> <b><?php echo $thankyousubject; ?></b> <br /><br /> <?php echo $thankyoumsg; ?> <br /><br /><br /><br /> <a HREF=\"http://www.shekinteriors.com\" TARGET=\"_top\"> <IMG SRC=\"http://www.shekinteriors.com/formbanner.gif\" WIDTH=\"600\" HEIGHT=\"100\" ALT=\"Return to Shek Interiors Ltd\" BORDER=\"0\"> </a> </center> </body> </html>
-
Readability. No functional difference whatsoever. Having it all on one line made it difficult to read because you have to scroll back and forth to see it all. Plus, the modified structure presents it similar to how it would be displayed in the email. Doh! It's perfectly flexible. I've used this method in my own CMS for years without any problems : ) Really? Using RegEx to try and ascertain the correct fields to pull values from assumes that when there are changes to be made later (by the same or different person) that the person knows the logic for ascertaining those fields. It could happen that they want to add a field for the user to enter the number of years that they have conducted those job descriptions. That field might be created with the name "job_description_years" - which would introduce a bug. Of course, I am manufacturing a scenario that would break that code and lead to additional time needed to debug. But, the point is that it is not an efficient method and introduces much more complexity than simply making the fields an array and using a single implode() function in the processing page. Note to OP: you might also consider making an array of the job descriptions and creating a small loop to generate your form code. Somethig like this: $descriptionsAry = ( 'Drywall', 'Insulation', 'Taping', 'Texture', 'Stucco', 'T-Bar', 'Custom Ceilings', 'Steel Stud Framing', Bulk Heads ); $formFields = ''; foreach ($descriptionsAry as $id => $value) { $formFields .= "<div align=\"left\">\n"; $formFields .= "<input type=\"checkbox\" name=\"job_description[]\" value=\"{$value}\" id=\"jobdescription_{$id}\" />\n"; $formFields .= "<label for="jobdescription_{$id}">{$value}</label>\n"; $formFields .= "</div>\n"; } Then in the form: <form method="post" action="" > <td><p>Job Description:</p></td> <td> <?php echo $formFields ; ?> </td> This ensures all the fields are created consistently. There are currently some minor problems with the form code (e.g. empty divs, labels not associated with the fields, etc.). by properly associating a label with the field, the user can click on the label to activate the field.
-
EDIT: Looks like Marcus already addressed the problem, but I'll keep this anyway since the code below may be useful That's just silly. The brackets make the fields an array of values. By hard-coding different names the code is notflexible. I tried your code and the values for the job descriptions were getting handled correctly. Your problem is that you define the $body before you define $job_description! The $job_description variable you define in the foreach() loop is never used. And, instead of a loop for the array, just use implode(). Change the order of the logic and it will work fine. You also have some problems with the HTML. There is a bold and center tags before the body tag. Plus, font tags have been depricated for years. <?php date_default_timezone_set('America/Edmonton'); $yourEmail = "test@yahoo.ca"; //The Email Address Form Results Will Be Sent To. Change to your email. $subject = "Online Job Requests"; //This will be displayed in your Email Subject line. Edit as needed. $job_description = implode(', ', $_REQUEST["job_description"]); $body = "Name: $_REQUEST $name \n"; $body .= "Email Address: $_REQUEST $email\n"; $body .= " Phone: $_REQUEST $phone \n"; $body .= "Job Type: $_REQUEST $job_type \n"; $body .= "Job Description: $job_description \n"; mail($yourEmail, $subject, $body, "From: $_REQUEST[$name] < $_REQUEST[$email] >"); $timestamp = date("n/j/Y, \a\\t g:i a T"); $thankyousubject = "Request Submitted Successfully \n on \n $timestamp"; //This will be displayed after a submission. Edit to suit your needs. The \n represent page breaks. $thankyoumsg = "Thank you $_REQUEST[name] we have recieved your information. We will contact you soon."; //This will be displayed after a submission. Edit to suit your needs. ?> <html> <body style="background-color:#2c2c2c;color:#FFFFFF"> <center> <b><?php echo $thankyousubject; ?></b> <br /><br /> <?php echo $thankyoumsg; ?> <br /><br /><br /><br /> <A HREF=\"http://www.shekinteriors.com\" TARGET=\"_top\"> <IMG SRC=\"http://www.shekinteriors.com/formbanner.gif\" WIDTH=\"600\" HEIGHT=\"100\" ALT=\"Return to Shek Interiors Ltd\" BORDER=\"0\"> </A> </center> </body> </html>
-
Help Required on PHP & Mysql Login Security Check Script
Psycho replied to j.smith1981's topic in PHP Coding Help
You are duplicating your form. It appears you have the initial form and then, if login fails, you duplicate the form. There's no need to do that. Also, I would suggest that you do not mix the logic (PHP) and the output (HTML). Do all the loigic and then do the presentation. In other words do not put <HTML> on the page until you've done the logic. I see some minor issue, such as you are saving the escaped string for username to session instead of the "plain" username. Plus, there is some error handling missing. Here is a rewrite with some changes I would make. This is not tested. So there may be some minor typos, but the logic should be sound. login.php (this is the main page that will always be called. It will validate the form, if needed. The user does not call the form directly) <?php //Variable to track any errors $error = ''; //Check if form was posted if (isset($_POST)) { //Validate required data was entered if (!isset($_POST['uname']) || trim($_POST['uname'])=='' || !isset($_POST['pword']) || trim($_POST['pword'])=='') { //Missing data $error = "Required data was not entered."; } else { //Data was submitted, execute db query and validation include ('db_config.php'); //Assign value for submited data (used to repopulate form) $username = trim(stripslashes($_POST['uname'])); $password = trim(stripslashes($_POST['pword'])); //Assign values for query use $sqlUsername = mysql_real_escape_string($username); $sqlPassword = mysql_real_escape_string(md5($password)); //Create and run query $sql = "SELECT id FROM $tbl_name WHERE username='$sqlUsername' AND password='$sqlPassword'"; $result = mysql_query($sql); //Check for errors if(!$result) { //Error running query $error = "An unknown error occured, please try again."; } else if(count($result)!=1) { //No match found $error = "Wrong username or password, please try again."; } else { //Login successful session_register("username"); session_register("password"); setcookie("user_name",$username); header("location:login_success.php"); } } } //Form was not posted or there were errors, show form include('login_form.php'); ?> login_form.php (This is only called from the above script) html> <body> <h1>My Login</h1> <br> <tt><?php echo $error; ?></tt> <br> <form name="login" id="login" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <tt>Username:<input name="uname" id="uname" type="text" value="<?php echo $username; ?>" /></tt> <br> <tt>Password:<input name="pword" id="pword" type="password" /></tt> <br><br> <input type="submit" name="submit" id="submit" value="Login"> <input type="reset" name="reset" id="reset" value="Clear"> </form> </body> </html> -
You could create a function <?php function setValue (&$variable, $default) { return (isset($variable)) ? $variable : $default; } //Set values for testing only $foo['bar1'] = 'Passed Value'; //$foo['bar2'] = 'Passed Value'; //This value not set $bar1 = setValue($foo['bar1'], 'Value not set'); $bar2 = setValue($foo['bar2'], 'Value not set'); echo "bar1 = $bar1"; //Output: bar1 = Passed Value echo "bar2 = $bar2"; //Output: bar2 = Value not set ?>
-
To answer some of your questions, no you do not "have" to put the functions before you call them. It is just good programming etiquette (sp?). You *should* break your code up into logical sections. The logic (PHP), the presentation (CSS) and the output (HTML). For example, in the above I would probably use the function like this: $monthOptions = dateOpt('month', $month); That would be in the head of my document (or even a separate file). Then at the bottom (or output file) I woul have just HTML code with the dynamic output inserted appropriately <select name="month"> <?php echo $monthOptions; ?> </select> This keeps your code very clean and much easier to maintain. Try not to intermingle PHP logic in the HTML.
-
I don't see anything obviously wrong as to why you are not getting output. Your second problem is probably due to how you are including the function in your other code. I will offer some suggestions though. 1) I would advise against using 1, 2, 3 to determine the type of list being created. It makes it more difficult to understand your code. Use values that you will understand - i.e. day, month, year. 2) Each of the different "lists" are doing the exact same thing, the only difference is the set of values. Your code is more complex than it needs to be. You should use a switch statement to define the list then perform the exact same operation of creating the optino values. Here's an example: <?php function dateOpt($listType, $selectedValue) { //Select the values for the list type switch($listType) { case 'day': $optionsAry = range(1, 31); break; case 'month': $optionsAry = array ( 'January','February','March','April','May','June', 'July','August','September','October','November','December' ); break; case 'year': default: $optionsAry = range(date("Y"), date("Y")-5, -1); break; } //Create the select options HTML $optionsHTML = ''; foreach($optionsAry as $optionValue) { $selected = ($optionValue==$selectedValue) ? ' selected="selected"' : ''; $optionsHTML .= "<option value=\"{$optionValue}\"{$selected}>{$optionValue}</option>\n"; } return $optionsHTML; } $month = "March"; echo dateOpt('month', $month); ?> NOTE: You could also put logic in to pass the currently selected month and year as optional values when getting the list for days. That way you can limit the number of days appropriately according the the month/year.
-
Query to SQL database not returning actual values
Psycho replied to muskelmann098's topic in PHP Coding Help
You have no error handling so, if the query fails, you will have no way of knowing. Although I suspect it is because you delineated the field 'oil' with single quotes instead of back quotes in the second query. (Edit: I guess it is something else, but the debugging below should help) Plus, there is no reason to do two queries to the exact same table. If they were two different tables you could use a JOIN, but it's the same table. The queries make no sense to me. The first query gets the value of 'camp' where the username matches, then you get the record wich has the same value for camp. Wouldn't that be the same record from the first query? Try the code below. You should add propper error handling/reporting in your application. I would also suggest passing $name to the function instead of making it a global - and the global $conn is not even used. function totRes($resource) { global $name; $query = "SELECT 'Oil' FROM `Villages` WHERE username = '$name'", $result = mysql_query($query); //Temporary debugging if (!$result) { echo "There was a problem running the query:<br />\n{$query}\n"; echo "<br /><br />Error:<br />" . mysql_error(); exit(); } $totres = mysql_result($result, 0); echo $totres; } -
How to delete MySQL records after a certain time?
Psycho replied to Vince889's topic in PHP Coding Help
First off, make it easy on yourself. Instead of storing the hours before deletion, store the timestamp of deletion (if you absolutely need the time to deletion, then store that too). Then, you do not need to do any calculations when it comes time to delete, simply run a simple query such as: DELETE FROM table WHERE deleteTime < NOW() As ym_chaitu stated above you could do this through a CRON job (google for more info). However, do you really need to delete the records? You could make the delete time an expire time. Then just use the expire time to exclude any expired records when doing the normal queries. -
Helping adding another IF statement Please!!
Psycho replied to andrew_biggart's topic in PHP Coding Help
Adding comments and proper format is key to writing good code. I changed us some of your logic as I prefer to check for the negative/error conditions first and the success conditions are at the end. I also separated the logic (PHP) from the output (HTML) <?php include("config.php"); //Check if a submission was made if(isset($_POST['add_suggestion'])) { //Check if user is logged in if(!isset($_SESSION['myusername'])) { //User is not logged in, redirect to login header("location:registration.php"); } //Set default status color $statusColor = "red"; //User is logged in, check for number of submissions $userName = mysql_real_escape_string($_SESSION['name']); $query = "SELECT COUNT('Suggestion_comment') FROM admin_suggestionst WHERE Suggestion_username='$userName'"; $result = mysql_query($query); if (!$result) { //Problem running query $output = "Oops, please try again !"; } else { //Check for number of submissions $submissionCount = mysql_result($result, 0); if($submissionCount>0) { //User has previous submissions $output = "You already have {$submissionCount} submissions."; } else { //Users first submissions, add it $avatar = mysql_real_escape_string($_SESSION['myavatar']); $comment = mysql_real_escape_string($_POST['Suggestion_comment']); $query="INSERT INTO admin_suggestionst (Suggestion_username, Suggestion_comment, Suggestion_date, Suggestion_avatar) VALUES('{$userName}', '{$comment}', NOW(), '{$avatar}')"; $result=mysql_query($query); if(!$result) { //Problem running query $output = "Oops, please try again !"; } else { //Submission was added successfully $statusColor = "green"; $output = "Thanks for your feedback !"; } } } } mysql_close(); ?> <html> <body> <h1 class="status_<?php echo $statusColor; ?>"><?php echo $output; ?></h1> </body> </html>