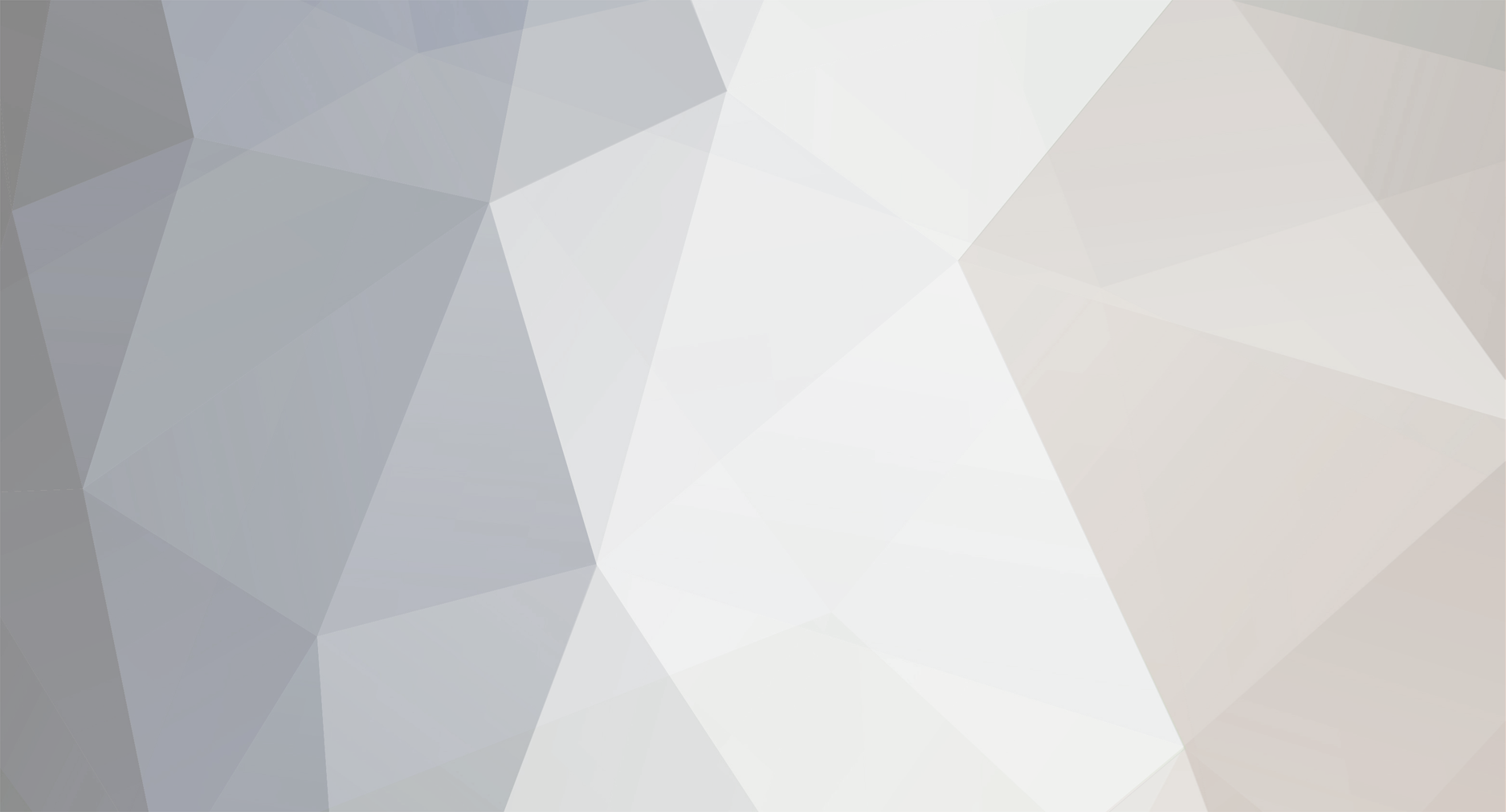
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
[SOLVED] looping throgh arrays with arrays inside them
Psycho replied to nadeemshafi9's topic in PHP Coding Help
You need a recursive function function processAllInArray($array) { foreach ($array as $value) { //Do something //If value is an array then process it as well if(is_array($value)) { processAllInArray($value); } } } -
Is your problem soved? If so, marke the topic solved.
-
Well, you don't show the format of the saved values or anything to really go on. But, I would generate two arrays: 1) The array of all possible values for the checkboxes and 2) an array fo the saved values (if any). Then create a procedure for displaying the checkboxes and checking any as needed: $values = array ('1', '2', '3', '4', '5', '6', '7'); $selected = array('1', '4', '5'); foreach ($values as $value) { $checed = (in_array($value, $selected)) ? ' checked="checked"': '' ; echo "<input type=\"checkbox\" name=\"aorgs[]\" value=\"{$value}\"{$checked}>{$value}<br />\n"; }
-
Hmm... You're calling the function like so onsubmit="return validate_form(this);" And, here is the function function validateForm(form1) { //bunch of validation code } I couldn't imagine what could be wrong. Ok, I was just being sarcastic, it happens to the best of us. But, if you still don't see the problem: "validate_form" != "validateForm"
-
If the query is not generating an error then you should check the DB to see if the records were inserted as you expected. However, I think the problem is that when you define the "to" in the values you are appending an additional space (as well as in $from and $date) VALUES ('$to ','$from ','$date ','$subject','$message') // ^ ^ ^ However, having looping queries is terribly inefficient. I suggest you generate one query of all the records to be added and then just run that one query. Besides there is no point in assigning the result of a query to $spm if you aren't going to use it. And you are reassigning the same values over and over to $subject, $message, $from and $date. Why? $subject = $_POST['subject']; $message = $_POST['message']; $from = "Support Staff"; $date = date("F j, Y, g:i a"); $values = array(); $getmembers = mysql_query("SELECT * FROM user"); while($user = mysql_fetch_array($getmembers)) { $values[] = "('{$user['Fname']}', '{$from}', '{$date}', '{$subject}', '{$message}')"; } $query = "INSERT INTO `private_msg` (`to`,`from`,`date`,`subject`,`content`) VALUES " . implode(",\n", $values); mysql_query($query) or die(mysql_error());
-
I have a table where I am storing records that include a file path. I need to determine if a new record to be added has a path which is a child directory of an existing record or a parent directory of an existing directory. To check if the new path is a parent directory of an existing path is simple enough since an existing value will equal the new path with additional path info appended to the end: SELECT * FROM table WHERE path LIKE '$new_path%' However, checking to see if the new path is a sub directory of an existing path requires checking if any exisitng path is LIKE the new path. I came up with the following query which works, but I'm thinking there is probably an easier way that I am not aware of: SELECT * FROM table WHERE '$new_path' LIKE concat(path, '%') So, is there a built in function that will do this that I am not aware of?
-
Switch letters in message (basic cryptology)...
Psycho replied to smartboyathome's topic in PHP Coding Help
I thinkth eproblem is that you are trying to replace the same letters with the other values that you are replacing. So, there is some sort of recursive error that is going on. You replace 'a' with 'n', but you are also replacing 'n' with 'a'. I tried str_replace() and obtained different results - only the first two replacements appeared to be made. But, i suspect that the other replacemetns were made, but they were made back again. Input: "no one at all" Output: "ao oae at all" My suggestion would be to do in intermediary replacement. First, change the letters to something else so you don't have the problem of replacing something with another value that is also being replaced. <?php $text = '"no one at all"'; echo "Input: $text<br>"; $search = array('a', 'n'); $replace1 = array('[a]', '[n]'); $replace2 = array('n', 'a'); $text = str_replace($search, $replace1, $text); $text = str_replace($replace1, $replace2, $text); echo "Output: $text"; //Input: "no one at all" // //Output: "ao oae nt nll" ?> -
Also, most home users have Dynamic IPs. Although they don't change often, they do change.
-
You need to clean up your code and keep it organized. In the functino that is called on submit validateFormOnSubmit(), the second line of code is calling another function: validateUsername(). That function does not exist, so the javascript errors out and the form is sent. Also, you have the function validateForm() defined twice. Overall there is a lot of unnecessary code in there. You shoudl start by implementing validation for one field. Once that works do the next field, and so on. There are other problems as well. In the function validatePassword() you are referencing an object called 'fld', but it is not defined function validatePassword(password) { var error = ""; var illegalChars = /[\W_]/; // allow only letters and numbers if (fld.value == "") {
-
Teynon, did you even read the thread before posting? In my first response I already explained the difference between JavaScript validation and PHP validation, AND I provided a more efficient solution for PHP validation than using regular expression. In my last post I asked for clarification on what he wanted to determine if it was JS or PHP he was asking about and instructed him to create a new thread in JS if that is what he needed. No need to move this thread since only PHP solutions have been dicussed.
-
I'm not understanding what you want. Are you wanting javascript code for the input form? If so, post in the JavaScript forum.
-
From my interpretation of your first post, I think you are saying there will be multiple records with the same 'skey' value and want to know how many times each one appears. If that is the case you will need something a little different: SELECT skey, COUNT(skey) as count FROM titles GROUP BY skey That will return a separate record for each unique value along with the number of times it appears.
-
[SOLVED] Displaying image showing # of players on other sites
Psycho replied to slyte33's topic in PHP Coding Help
You would want the url for the image to point back to your server and it doesn't have to be a url to an actual image. It can be a link to a PHP page that will return an image. So, the image could look something like this: <img src="http://www.mysite.com/playerBanner.php"> Then you would want to create a script for that page which will take the template image and dynamically write the number of players on the image object and then serve the image to the requesting process. Do a google search for "PHP add text to image" to get started -
If you want to prevent the user from actually entering the other characters then you need to use JavaScript. But, you will still want a PHP implementation to handle it in case someone has JS turned off. For the PHP implementation you can use ctype_alnum(): http://us2.php.net/manual/en/function.ctype-alnum.php
-
You could do as suggested above by using preg_replace() or something similar to capture all the text between those custom tags and escape it with one of the PHP functinos for doing so. But, there may be an even easier solution. You could just replace all the [ nohtml ] and [ /nohtml ] tags with < pre > and < /pre >. I think that would give you similar results.
-
[SOLVED] Checking if variable is 0 as opposed to NULL/Empty...
Psycho replied to PeterJ's topic in PHP Coding Help
You need to do a strinct comparison using three equal signs which checks for an IDENTICAL comparison, not simply an EQUAL comparison. However, you will need to validate EXACTLY what the value is returned from your query when the user doesn't have a value set in the database. I am guessing you will want to compare against 'null'. Examples: if ($access_id === null) {$access_id = '2';} This page further explains how the comparisons are interpreted: http://php.net/manual/en/language.operators.comparison.php -
[SOLVED] Script that runs when the page isn't open
Psycho replied to Peggy's topic in PHP Coding Help
This will tell you everything you need to know about CRON scripts: http://lmgtfy.com/?q=cron+script -
I agree that trying to generate the random numbers in real time as they are needed is problematic. I'd just create a process to geneerate the random numbers and add them to a temporary table if the number in that table is below a predefined threshold. Then pull from that table when you need them. The process to add the numbers to the temp table could be run on a scheduled basis. Granted, creating an algorithym at the start would have been the best case scenario.
-
[SOLVED] regex for quoted text within a string
Psycho replied to abazoskib's topic in PHP Coding Help
That won't work simshaun. The '*' by itself is greedy and will match the text between the very first and very last double quote instead of matching multiple quoted strings when they exist. Plus it REMOVED the quoted text entirely instead of just escaping the double quote marks. $val = 'Here is "quote number one" and here is "quote number two" with a space'; $pattern = '/\s\"(.*?)\"\s/'; $replacement = ' \\\"\1\\\" '; $val = preg_replace($pattern, $replacement, $val); echo $val; //output: // Here is \"quote number one\" and here is \"quote number two\" with a space -
How to integrate PayPal subscriptions with PHP: http://www.justin-cook.com/wp/2007/01/30/how-to-integrate-paypal-subscriptions-with-php/
-
I see at least one siginificant problem. You should really use CODE tags when posting. You are passing 'p' on the query string to determine the current page to display. But, in your logic for determineNumberOfPages() if $_GET['p'] is defined you are returning that value as the number of pages. The number of pages has no bearing on the current page. function determineNumberOfPages($dbc, $display) { if (isset($_GET['p']) && is_numeric($_GET['p'])) { // Already been determined. $pages = $_GET['p']; } else { // Need to determine.
-
Not to be rude, but why should it fix your mistakes? Just because IE makes an assumption of what it thinks you want and it works as you would like doesn't make it valid code. Because it is invalid code there is no standard on how it should be interpreted. The browsers that "work" with your code may have different logic for "fixing" these types of errors and it just happened, in this instance, that they got it right - this time. It could just as well of been that each could have interpreted the invalid code differently resulting in wildly different results. Think about all the time you have wasted on this because you developed in one application that interprets something differently than another application. If they all follow the same standard you don't need to do as much testing between browsers. I for one would rather have an application not work with invalid code - making me fix it - than having it work with my code but not working in the other applications.
-
OK, the problem is how you are referencing your input object. You are not stating it in the appropriate context. It doesn't know where to find the "generatorForm" object. You should use: document.generatorForm.passwdBox.value = random_passwd(); I see no reason why that code would not work with numbers lower than 79. Besides, as I stated you don't need to hard code that value, just use the length of the variable with the characters. Full code that should work: <h3>Secure Password Generator</h3> <script language="javascript"> function random_passwd(min, max) { var chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*'; var length = min + Math.floor(Math.random() * (max-min)) var password = ''; for(var i=0; i<length; i++) { password += chars.charAt(Math.floor(Math.random() * chars.length)); } return password; } </script> <form name="generatorForm"> <input name="passwdBox" type="text" value=""/> <input type="button" value="Generate New Password" onClick="this.form.passwdBox.value = random_passwd(9, 13);" /> </form>
-
It works for me in FF, but there are some problems in the code. You only have a string of 70 characters, yet you are generating random numbers from 0 to 78 (i.e. 79 characters). The function should be dynamic based upon the number of characters in the string. I would also make the length of the password dynamic with a min and max length, say from 9 to 15 characters. Also, you don't need to return false when using a non-submit button. <script language="javascript"> function random_passwd(min, max) { var chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*'; var length = min + Math.floor(Math.random() * (max-min)) var password = ''; for(var i=0; i<length; i++) { password += chars.charAt(Math.floor(Math.random() * chars.length)); } return password; } function formSubmit() { generatorForm.passwdBox.value = random_passwd(9, 15); return false; } </script> <form name="generatorForm"> <input name="passwdBox" type="text" /> <input type="button" value="Generate New Password" onClick="formSubmit()" /> </form>
-
Give the form tag a target parameter. user "_blank" to open a new window each time they submit. <form target="_blank"> Or use a named widow to open all submissions in the same window. A new window window will be opened if it doesn't exist, but subsequent submissions will be opened in the same window. <form target="CustomWindowName"> Having named windows allows you to interact with that window from other pages if needed. For example, if you needed to close the opened window from the original page you could do it by referencing the named window.