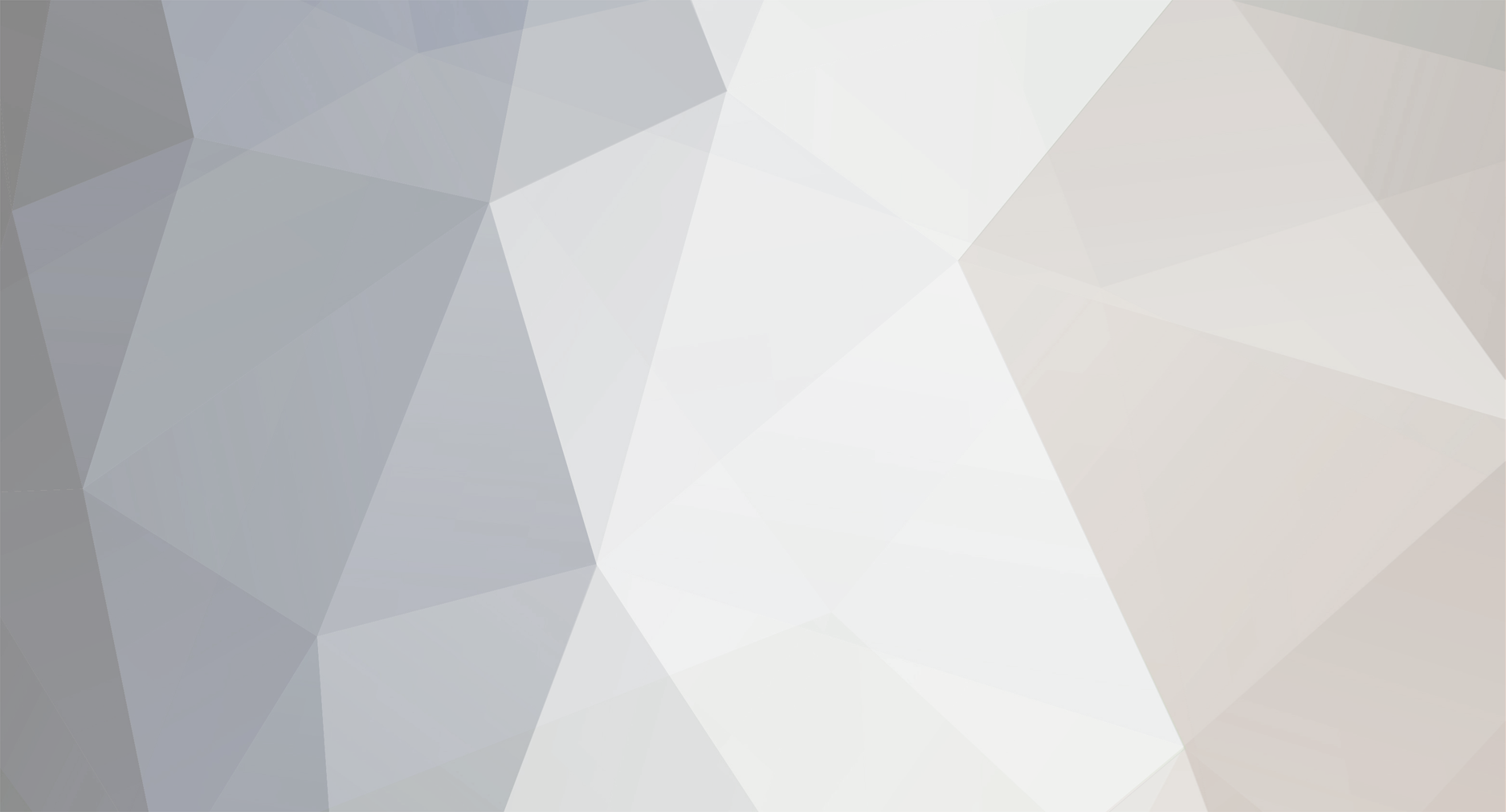
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I think there is a misunderstanding on what ?= does. My understanding is that it is a 'lookaround'. Basically it looks for a match, but then 'gives up' the match. That way it does not move the position forward. So, you can use it to look for a number or a letter and their position won't matter. The way I read the above expression is that the value must be at least 8 characters long. It must have a number. It must have a lower-case letter. It must have an upper-case letter. I think that the ".*" at the beginning and end are unnecessary. But, I'd have to test it to be sure. EDIT: This should do what you want. /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,}$/ - Must contain number - Must contain lower-case letter - Must contain upper-case letter - Must be at least 8 characters long Or, if you also want to include a special character /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.[\W]).{8,}$/
-
A few critiques: 1. That code is trimming the password. I see no logical reason to do that as it would only potentially reduce the strength of the password. A user may add spaces to the beginning or end of a common word to create their password, which would at least prevent a cursory dictionary attack or someone trying values that they know about the user. 2. That code does not trim the 'text' values. Contrary to passwords, I always trim other input from the user unless I have a specific reason not to. You don't appear to have any required field checks, but if you did, not trimming spaces would allow a person to get around those with just spaces. 3. There is a small hole in the logic. In the try block there is an if() check to verify there was a successful result. But, there is no else condition. It is assuming that if there is an error it would be handled by the catch block. If that's the case, then there is no need for the if() condition. But, if there is the possibility that the query does not fail, but does not produce the intended outcome it would produce no results whatsoever. You could consider changing that if() condition to a check of the affected rows to verify it is equal to 1 - then add an else condition to report an 'unknown' error.
-
http://mikehillyer.com/articles/managing-hierarchical-data-in-mysql/
-
After looking at the output, I think I see what you are doing. I think the logic is backwards. Plus, you should not use Regex for this $keywords = array(0=>"Web Development", 1=>"Online Marketing", 2=>"Australian Web Developers", 3=>"Web Designs", 4=>"Custom Web Design", 5=>"Online Marketing",6=>"Internet Advertising",7=>"Professional Web Design",8=>"Web Designing"); $superlatives = array(0=>"Biggest", 1=>"Leading", 2=>"Best", 3=>"Top", 4=>"Largest", 5=>"Premier"); $suffixes = array(0=>"Agency", 1=>"Provider", 2=>"Service", 3=>"Specialist", 4=>"Experts", 5=>"Company", 6=>"Business", 7=>"Builder", 8=>"Services"); //Iterate over each keyword foreach($keywords as $keyword) { //Iterate over each superlative foreach($superlatives as $superlative)//superlatives { //Check if keyword ends in 'ers' if(substr($keyword, -3)=='ers') { echo $superlative . " " . $keyword . "<br/>"; } else { foreach($suffixes as $suffix)// suffixs { echo $superlative." ". $keyword ." ".$suffix."<br/>"; } } } }
-
I don't understand what it is you are trying to accomplish
-
$filtered_array = array( array('position'=>'1', 'Title' => 'Sanctum', 'URL'=>'http://www.imdb.com/title/tt0881320/'), array('position'=>'2', 'Title' => 'Horrible Bosses', 'URL'=>'http://www.imdb.com/title/tt1499658/'), array('position'=>'3', 'Title' => 'Pirates of the Caribbean: On Stranger Tides', 'URL'=>'http://www.imdb.com/title/tt1298650/'), array('position'=>'4', 'Title' => 'Mission: Impossible - Ghost Protocol', 'URL'=>'http://www.imdb.com/title/tt1229238/'), array('position'=>'123', 'Title' => 'Headhunters', 'URL'=>'http://www.imdb.com/title/tt1614989/') ); $positioinTail = ')'; //Detemine the max lengths $positionLength = 0; $titleLength = 0; foreach($filtered_array as $row) { $positionLength = max($positionLength, strlen($row['position'].$positioinTail)); $titleLength = max($titleLength, strlen($row['Title'])); } //Output fixed width data foreach($filtered_array as $row) { $output .= sprintf("%-{$positionLength}s", $row['position'].$positioinTail) . ' '; $output .= sprintf("%-{$titleLength}s", $row['Title']) . ' '; $output .= '---> ' . $row['URL'] . "\n"; } echo $output; Output 1) Sanctum ---> http://www.imdb.com/title/tt0881320/ 2) Horrible Bosses ---> http://www.imdb.com/title/tt1499658/ 3) Pirates of the Caribbean: On Stranger Tides ---> http://www.imdb.com/title/tt1298650/ 4) Mission: Impossible - Ghost Protocol ---> http://www.imdb.com/title/tt1229238/ 123) Headhunters ---> http://www.imdb.com/title/tt1614989/ NOTE: That is the right output if you are wanting to write to a txt file or provide the user in a download. For it to 'display' on a web page as you wish you will need to wrap the output in PRE tags.
-
Except he stated he wants to save it as a txt file. @Homer5: You'll also want to take into account the length of the longest position. And if you are saving it as a text file you should use \n instead of the HTML line break - <br>
-
Yep, don't duplicate the First/Middle/Last into a column for Full Name. All you do is make more work and create the potential to get things out of sync. Unless, you have a legitimate business need to have a separate Full Name value. We have this in our application. We auto-populate the Report name field, but the users can put a 'modified' version of the name in the "Report name" field if they wish: FirstName: Robert MiddleName: David LastName: Smith ReportName: Bob Smith If you don't have such a requirement - ditch the Fullname field. Ideally, you would want to set up fulltext searching, but an easy workaround is to split the search string and create multiple LIKE conditions on the concatenated names. Note: I used the mysql_ functions because I'm feeling lazy, you should really use mysqli_ or PDO with prepared statements //Process the input $searchString = $_POST['search']; $searachWords = explode(' ', $searchString); //Create the where condition for each word $WHERE_CONDITIONS = array(); foreach($searachWords as $word) { $word = mysql_real_escape_string($word); $WHERE_CONDITIONS[] = "CONCAT(first_name, middle_name, last_name) LIKE '%$word%'" } $query = "SELECT * FROM table_name WHERE " . implode( " OR ", $WHERE_CONDITIONS);
-
I would propose that the bsmither proposed needs further denormalization. If you have common text in the header or footer of the pages, you would not want to define the same text for every page. I think there needs to be the ability to applyt he same text sections to multiple documents/pages. I would propose something such as this: documents/pages (defines each unique page) doc_id, doc_name text (defines the different sections of text) text_id, text_name doc_text (table to associate which text fields appear on which pages) doc_id, text_id translations (defines the different language values for the sections of text) text_id, language, value
-
convert input text into images based on alphabet
Psycho replied to ajrocha81's topic in PHP Coding Help
You have an error in the logic. The code to output "Character: X" is within the loop, but the code to output the image is not - so only the image for the last letter would display. You should not go in and out of PHP/HTML like that because it becomes hard to find such errors. Have all your PHP code run and create variable to output in the HTML. I found numerous minor issues that were hard to spot because of the poor code formatting. NOTE: The below does not differentiate between 'a' and 'A'. <?php //Get the input from POST data $string = isset($_POST['string']) ? trim($_POST['string']) : false; $output = ''; if(!empty($string)) { $letters = str_split($string); foreach($letters as $letter) { $output .= "Character: {$letter}<br /><br />\n"; if(ctype_alpha($letter)) { $output .= "<img src='{$letter}.png' alt=\"Images for the '{$letter}' character.\" /><br /><br />\n\n"; } } } ?> <html> <body> <?php echo $output; ?> <p style="text-align: center;"> <form action='convert.php' method ='POST'> Convert English into images : <input type="text" name="string" size="20"></input> <br /> <button type="submit">Translate</button> </form> <p> </body> </html> -
Yeah, I'm curious why you want to do this.
-
How do I call a javascript function on remote website
Psycho replied to DeX's topic in Javascript Help
Have you looked into requinix's suggesting of using cURL? -
The format you want is JSON. There is no need to build it yourself, PHP has a built in function to convert an array to JSON. Just run the query and convert the result array to JSON. You'll want to create one array for all the records, the write the entire thing to your output file. Be sure to use the fetch_assoc() method. Also, don't repeat things in loops that don't need to be repeated. For example, why would you need to redefine $myFile in every iteration of the loop? Only do the things in loops that need to be repeated $myFile = "testFile.txt"; $data = array() while($row = mysql_fetch_assoc($qry_result)) { $data[] = $row; } //Open file for writing $fh = fopen($myFile, 'w') or die("can't open file"); //Convert data to JSON format and write to file $stringData = json_encode($data); fwrite($fh, $stringData); //close file fclose($fh);
-
Right, that is why the query to retrieve the value should specify for the value to be returned in UTC. That way there is no dependency on the server being set for any specific timezone. It would work correctly even if the application has to be moved to another server with a different timezone setting.
-
convert input text into images based on alphabet
Psycho replied to ajrocha81's topic in PHP Coding Help
Hmm, is this really necessary?: $image = array( 'a' => 'a.png', 'b' => 'b.png', 'c' => 'c.png', ); Just take the letter from the string and convert to $letter . ".png" -
There is no inherent security risk based upon how you store the data (there are always exceptions). It is the processes of how you store and read the data that add security risk. The problem with what you are doing is that it really limits how you can use the data. I'm not really following your proposal today. If you are going to use a bitwise operator,then just store that in the user table and don't use the other two tables. User Table: username, password, permissions (bitwise values), rest of columns. If you use a bitwise operator then you would need something to define which permission are at which position. You could either store this in PHP as part of a resource file or you could create a table in the DB. But, since you need to have logic in PHP to interpret the permissions anyway and this shoudl rarely, if ever change, I would do it in PHP. The other approach is to simply have one associated table to the user table User Table: userid, username, password, rest of columns. Permissions: userid, createusers, createxxx, dosomething, etc.
-
Strategy for keeping development in synch with test?
Psycho replied to davidannis's topic in MySQL Help
Do realize that when you use the UI within PHP Admin to perform an operation that it runs a query and displays that query? You should copy those queries and save them so you can run them in production when you promote a new release to production. Otherwise, how do you know what changes need to be made to the production DB? So, let's say you have a column in a table called DATA for "Status" and you are currently storing textual values such as "Low", "Medium" and "High". And, as a change for the next release it is decided that the list should be user configurable. So, you need to create a separate table to define the status values and in the DATA table change the "Status" column to hold a foreign key value for the status from the other table. You could do something like this: 1. Create the new table in PHPMyAdmin using the UI and populate with the default values. 2. Perform an export of the table (including values) and save that query 3. Create an update query to change the text values in the DATA table to be the foreign keys to the status ids. Save that query. When you then promote the new functionality to production you would run the same two queries. Likewise, if you take a backup of production to use in your test environment, you just run all of your alters to update it to the current schema. -
Strategy for keeping development in synch with test?
Psycho replied to davidannis's topic in MySQL Help
I assume you are going in an manually updating the database in your test environment using your DB management software. You could create scripts to perform all of the modifications that you make from one release to another. Then, when it is time to move a release to production you just run those scripts to perform the updates. During the development phase you can get a copy of your prod database and execute all of the current scripts to update it to the latest version. However, you really shouldn't be using a production database in a test environment if there is any personal data stored in the production DB. -
Yeah, that a pretty poor way to do that. I would still advocate a separate table to store permissions with a foreign key back to the user. Then have a separate column in the table for each permission. If you really want to have a single column to store the permissions, then you should use a bitwise operator. If you are familiar with binary, number are represented such as 10110 (that would be the number 22). Each digit in the number would represent a different permission. A 1 means the permission is granted and 0 is not
-
You may want to take some time to really understand what prepared statements are any why you should use them, There is NO reason to use a prepared statement for a static query. In fact, even if you have variable data in the query, if you are running it one time - the prepared query would be slower because there is overhead in creating the prepared query before running it. But, that overhead is more than made up for by the fact that it will be much more secure. But, if you are running the query multiple times with different values then you do get a performance increase. But, for a static query, there is no benefit or valid reason to make is a prepared statement. The whole point of prepared statements is to pass them values.
-
I will say that I resisted JQuery for years. Mostly because I felt I would be giving up control and be limited.Plus, I wasn't interested in learning a new 'language' to do things that I could do with vanilla JS. I'm a hobbyist so learning new languages/frameworks is only something I do if I am interested in it. There was a relatively short learning curve and I sometimes struggle on syntax since I have not worked with it as long. But, I have been able to build things very quickly that would have taken me many times longer without JQuery. And it was built to be extremely extensible. So, if I don't like the default behavior for a particular function (or want tit to do something additional) it is typically as simple as creating my own function and "plugging it in".
-
I suppose you could, but why? http://en.wikipedia.org/wiki/Prepared_statement There are no variable values in that query which are substituted. Making that a prepared statement has no purpose.
-
Just being able to speak a language fluently is not enough to build a translation utility. A person who speaks the language could easily translate a specific text from one to the other. But, trying to define all the rules to have a computer do it is a completely different thing. That person would have to have a deep understanding of the semantics and intricacies of the language at the lowest levels. A good example is driving a car. I'm sure you are very proficient at driving a car. But, could you build a program so a car can drive itself? That is something that has been worked on for several years now by very qualified people. It wouldn't just be having the car follow GPS. It needs to determine where other cars are, follow the speed limit, dynamically adjust based upon traffic and other cars. Be able to navigate through detours when there is construction. Etc. Etc. As complicated as that is, building a translation tool is harder. There is much more complexity - but less chance of anyone being fatally injured.
-
Yeah, if he is setting it to NOW() then it should be a timestamp and not a datetime anyway. But, a timestamp will, by default also update the the current timstamp when the record is updated (again, without having to include it in the query). If that's not the behavior needed, then the default property needs to be modified to only populate on creation.
-
Computers will never be able to translate language with any degree of correctness until we have systems that can truly "learn". Computers deal with Black and White - something is either true or false. They cannot figure out "gray" areas. Plus English to Arabic is very difficult since they are so disparate. Something like English to French would be significantly easier as they are both based on Latin. If you think you can build a better translator than Google and you don't have a Masters/Phd in Computer Science, Linguistics and both those languages with years of to devote to the project (or millions of dollars to hire the necessary talent), I think you are fooling yourself.