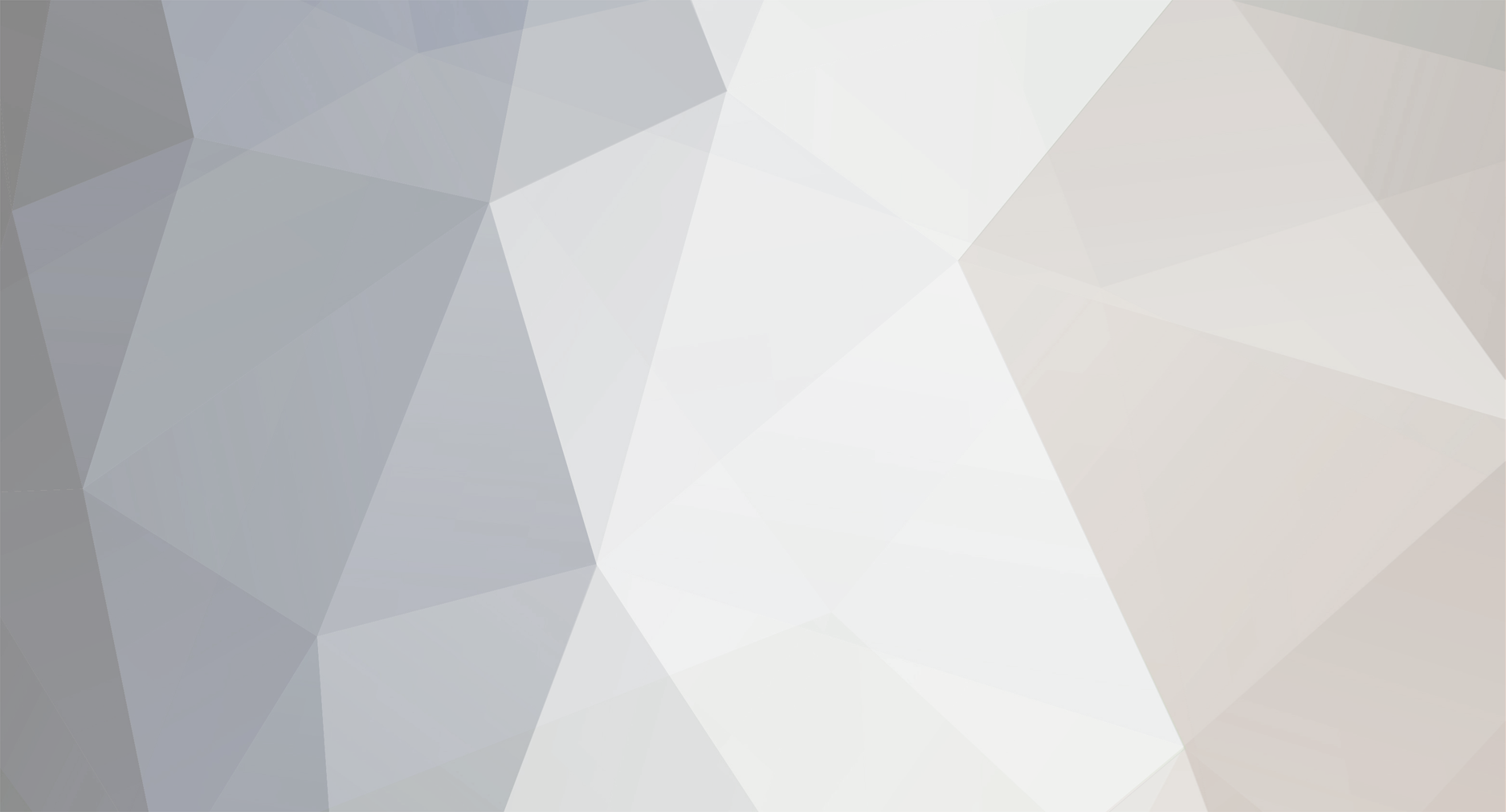
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Here's some PHP code to determine the date range. It's a little odd since you use the lower age to determine the max date and the upper age to determine the min date. Also, for the min date, you have to go back age + 1 years and then increment +1 days. That's because a 40 year old person could be someone who's 40th birthday is today up to someone who is 40 years and 364 days old. $min_age = 20; $max_age = 40; $max_date = strtotime("-{$min_age} years"); $max_date_str = date('Y-m-d', $min_date); $max_age_offset = $max_age +1; $min_date = strtotime("-{$max_age} years"); $min_date = strtotime("+1 day", $min_date); $min_date_str = date('Y-m-d', $min_date); echo "For the user to be from {$min_age} to {$max_age} years old they must have been born between {$min_date_str} and {$max_date_str}";
-
Wouldn't it be simpler to just modify today's date using the age_min and age_max values to determine the date ranges and use a BETWEEN operator? Not sure if it would be more efficient to calculate those dates in PHP or MySQL.
-
I'm not sure how widely accepted the pattern tag is. Also, you definitely wouldn't want to rely upon it since a malicious user could easily circumvent it. But, this seems to work for what you are asking: pattern="[\d]{5}|.{0}" Not sure if this is the most efficient method. It's kind of a hack IMO. Basically looks for 5 digits OR any character matched 0 times. I did some limited testing it out and got the expected results.
-
Making documents only accessible to specific users?
Psycho replied to Kristoff1875's topic in MySQL Help
Not my area of expertise. Suggest you do some research. -
There's a lot of things that need to be fixed int hat code. Here are a few that stand out: 1. Don't suppress errors with the '@' symbol. There are very few scenarios where that really needs to be done. 2. Your query is open to SQL injection. Never, ever trust data from the user 3. The logic is really convoluted. If you have If/Else statements that are nested more than three times, chances are it can be simplified 4. You should be using mysqli_ or PDO for the database. The mysql_ extensions have been deprecated for years. 5. Don't mix and match PHP code within the HTML . Do all the logic first and create the output in variables. Then just have echo statement in the HTML as needed. So, the "HTML" page still needs to be a PHP page so those variables can be echo'd 6. There is a session start was down the page - after content is output. Either that is never executing or it is generating errors. It looks like you are using it for some messages, but I don't think it is really used. As to your original question, the easiest solution is to make the PHP script with the 'logic' as the one that would be accessible to the user. The "HTML" file would only be included by the PHP page. So, you could just leave the 'target' of the form tag blank since it would load the same page by default. And, in this context the 'page' is the one which the user accessed via the URL. It doesn't matter what the actual pages on the server that are executed or loaded. Here is a rewrite of the above into two pages. I didn't test this as I don't have your functions. It includes a lot of changes so there may be some minor errors to resolve. I also did not fix all of the problems I indicated above (e.g. still uses mysql_ extension).. This will take TWO pages. The first is the page the users will access, so name it something you would want the user to see in the browser address bar. The second is the HTML content and would only be included by the first. If you want to put a target in the FORM tag, use the name of the first page. PHP page (i.e. the logic) <?php session_start(); require_once 'phps3integration_lib.php'; $message = ""; //Process form data is submitted if (isset($_FILES["file"]["name"])) { $allowed_ext = array("gif", "jpeg", "jpg", "png", "pdf", "doc", "docs", "zip", "flv", "mp4"); $extension = strtolower(end(explode(".", $_FILES["file"]["name"]))); if (($_FILES["file"]["size"] >= 32428800) || !in_array($extension, $allowed_ext)) { $message.= "Invalid file, Please upload a gif/jpeg/jpg/png/pdf/doc/docs/zip file of maximum size 30 MB."; } elseif($_FILES["file"]["error"] > 0) { //$message.="There is some error in upload, see: " . $_FILES["file"]["error"] . "<br>";//Enable this to see actual error $message.="There is some error in upload. Please try after some time."; } elseif(!uploaded_file_to_s3($_FILES["file"], "uploads", true)) { $message.="There is some error in upload. Please try after some time."; } else { $user_name = (isset($_POST['user_name']) ? trim($_POST['user_name']) : "Anonymous"; $form_data = array( 'file' => $uploaded_file, 'user_name' => $user_name, 'type' => 'file' ); $query = "INSERT INTO `phps3files` (`file`, `user_name`, `type`) VALUES ('{$uploaded_file}', '{$user_name}', 'file')"; mysql_query($query) or die(mysql_error()); $message.= "File successfully uploaded in S3 Bucket."; } } //Create the file llist output $file_list_HTML = ''; $query = "SELECT * from `phps3files` WHERE type LIKE 'file' ORDER by id DESC LIMIT 10" $files_result = mysql_query($query) or die(mysql_error()); if(!mysql_num_rows($result)) { $file_list_HTML .= "<tr>\n"; $file_list_HTML .= "<td colspan=\"2\"> No files uploaded yet</td>\n"; $file_list_HTML .= "</tr>\n"; } else { $fileCount = 0; while ($file = mysql_fetch_object($files_result)) { $fileCount++; $fileUrl = site_url_s3("uploads/" . $file->file); $deleteUrl = site_url("delete_file.php?id=" . $file->id); $file_list_HTML .= "<tr>\n"; $file_list_HTML .= "<td>{$fileCount}</td>\n"; $file_list_HTML .= "<td><a href=\"{$fileUrl}\" target=\"_blank\">View/Download</a></td>\n"; $file_list_HTML .= "<td><a href=\"{$deleteUrl}\">Delete file from S3</a></td>\n"; $file_list_HTML .= "<td>Uploaded by: {$file->user_name}</td>\n"; $file_list_HTML .= "</tr>\n"; } } include('the_form.php'); ?> The Content/Output Page, i.e. the_form.php <html> <head></head> <body> <?php require_once 'header.php'; ?> <fieldset> <legend>PHP AWS S3 integration library Demo1</legend> Description: In this demo a file is being upload to an S3 bucket using "PHP AWS S3 integration library". After upload you can check the uploaded file in below table. If you require some manipulation before uploading file to S3 then check <a href="upload_file_manually.php">Demo2</a> <br /> <br /> <form action="" method="post" enctype="multipart/form-data"> <div class="control-group"> <label for="file" class="control-label">Choose a file to upload: <span style="color:red">*</span></label> <div class='controls'> <input id="file" type="file" name="file" /> <?php /*echo form_error('file');*/ ?> </div> </div> <div class="control-group"> <label for="user_name" class="control-label">Your name:</label> <div class='controls'> <input id="user_name" type="text" name="user_name" maxlength="255" value="" /> <?php /*echo form_error('user_name');*/ ?> </div> </div> <div class="control-group"> <div class='controls'> <input type="submit" name="submit" value="Submit" class="btn"> </div> </div> </form> </fieldset> <?php echo "<div class=\"alert alert-success\">{$message}</div>"; ?> <div> <table class="table table-hover"> <caption> <strong>Last 10 user uploaded files</strong> </caption> <?php echo $file_list_HTML; ?> </table> </div> <h4>Source Code Part of Demo</h4> <pre class="prettyprint lang-php linenums"> </pre> <?php require_once 'footer.php'; ?> </body> </html>
-
Making documents only accessible to specific users?
Psycho replied to Kristoff1875's topic in MySQL Help
Since you are reading the files and pushing them to the user rather than the user downloading them, you really don't need to additional layer of "encryption" of the file name. Just authenticate the user and verify they are allowed to view the file and push it to them. The file name hashing really adds no additional security since you would have to authenticate the user first anyway. If someone has already gotten around the authentication process as another user then the additional hashing of the filename is moot since their "UserID" would be that of the user they are impersonating. If the files are of a very sensitive nature, then you could look at securing them on the server-side from someone who gains access to the server. You could either store the files directly in the database and encrypt them there (as jazzman1 suggested) or you could encrypt the actual files and then decrypt them before sending them to the user. Of course, if you are going to go to that extent then you should be using HTTPS. -
PHP Script randomly restarts itself with blank post data
Psycho replied to holdorfold's topic in PHP Coding Help
That appears to be the Advanced REST client plug-in. Perhaps that is the cause of these mysterious requests. -
Making documents only accessible to specific users?
Psycho replied to Kristoff1875's topic in MySQL Help
I'm pretty sure that is not your directory structure. If it was, then users would not be able to load images or CSS files. All those folders must be in a parent folder that is your actual root. Using my previous example: | |-[my_website_root] | | | |-[images] | |-[style_sheets] | |-[pages] | |-[files_for_download] You would need to configure, on your web host, to point your domain to the [my_website_root] folder. I.e. when a user enters www.mysite.com it points to the [my_website_root] folder. It is impossible for a user to enter a URL to directly access any of the files because they are not publicly available. As for your current approach, I think the logic is more complicated than it needs to be. You already have to authenticate the user, correct? Then there is no need, in my opinion, to use some sort of hash on the file name as part of the security. Instead, you can have database details to determine who can access which files. If all files have one, and only one, user with rights to access the file. Then you can have a column in the files table with the user ID who has rights to the file (you can implement logic that an 'admin' can access any files). Then, when a request is made to a file, you would verify that the logged in user has rights to the file before providing it to them. How you provide the file to them, since it is not publicly accessible, is through a force-download script which will 'read' the file from the file system folder that is accessible on the server, but not from the user, and output to the user. Here is an example of a force download script: http://davidwalsh.name/php-force-download -
Making documents only accessible to specific users?
Psycho replied to Kristoff1875's topic in MySQL Help
If you believe that obfuscation is a form of security, then yes what you propose would work. (Hint: obfuscation is not the same as security). If you want to be sure no one but the authorized user can download the files then you need to first put the files into a directory that is not web accessible. Then, you would put a process in place to provide an authentication mechanism (as described above) when either displaying the list of files or when attempting to "download". The download process would be implemented by passing an id to a download page. The download page would use the ID to find the file (typically stored in a DB) and send it to the user directly. Here is what the directory structure might look like | |-[my_website_root] | | | |-[images] | |-[style_sheets] | |-[pages] | |-[files_for_download] -
PHP Script randomly restarts itself with blank post data
Psycho replied to holdorfold's topic in PHP Coding Help
I've run some tests and cannot even force the problem you are describing. Using the JS function you provided above, I modified it to simply echo the returned value from the AJAX call. I then created the PHP page called by the AJAX request as follows: if($_POST['q']) { sleep(10); echo "TRUE"; } else { echo "FALSE"; } I then loaded the AJAX page and clicked a button to call the function passing a POST value for 'q'. Then, before the 10 seconds expired, on a separate tab I made a browser request directly to the PHP page. That page displayed a 'FALSE'. I then went back to the original tab and when the 10 second sleep expired I saw "TRUE" alerted on the page. In other words, I couldn't hijack the original POST request by making a separate request to the same page in a different browser tab. I also ran a test where I called the same SendPHP() function with two different buttons. One would call the PHP with a 'q' post value and the other would call it without. If I clicked the one with the 'q' value first and then the one without, I would first get the FALSE response (since there is no delay for that) and then I would get the one with the TRUE response after the 10 seconds. What this tells me is that a second request to the same page that occurs after the first request will not prevent the response for the first request. So, as a workaround, have the PHP page simple return an empty result if there is no 'q' in the POST data and change the AJAX success parameter to test for a return value. If empty - do nothing. success: function(msg) { if(!msg){ return; } callback(msg); }, However, I think the problem is as mac_gyver alluded to and there is something on your PC that is doing this. The server can't make the client initiate a request. Have you tried this on a separate PC? -
PHP Script randomly restarts itself with blank post data
Psycho replied to holdorfold's topic in PHP Coding Help
Then add logic to the client-side to handle scenarios where unanticipated return values are appropriately handled. I always try to code processes such as this to elegantly handle scenarios where unanticipated data is returned. -
Who said you had to read the article? I wrote that code using the manual in about 10 minutes.
-
No. I'm no expert in mysqli_ (I use PDO), but, per the manual, once you bind the "variable" to the query you can set/change the variable before executing the query. Here is an excerpt form the manual. Example 2 $stmt = mysqli_prepare($link, "INSERT INTO CountryLanguage VALUES (?, ?, ?, ?)"); mysqli_stmt_bind_param($stmt, 'sssd', $code, $language, $official, $percent); $code = 'DEU'; $language = 'Bavarian'; $official = "F"; $percent = 11.2; /* execute prepared statement */ mysqli_stmt_execute($stmt); @Joak, Are you checking for DB errors?
-
Which is very different from what you stated in the first post Try this: $stmt = $mysqli->prepare('INSERT INTO prueba (id,nombre) VALUES (?, ?)'); $stmt->bind_param('is',$id,$name); foreach($obj as $assocArray) { $id = $assocArray['id']; $name = $assocArray['name']; $stmt->execute(); // execute the query }
-
What's not valid about this? for($i=0; $i<$config_max_digits;$i++) $newCode = $newCode.rand(0,9); Test: $config_max_digits = 10; $newCode = "CODE"; for($i=0; $i<$config_max_digits;$i++) $newCode = $newCode.rand(0,9); echo $newCode; Output: CODE9094108549
-
PHP Script randomly restarts itself with blank post data
Psycho replied to holdorfold's topic in PHP Coding Help
While this is an odd issue, how much time are you wanting to invest into understanding it rather than just fixing the problem. In fact, this is something you should plan for anyway. If the URL is accessible through AJAX then anyone could try and access it with other data or no data. Your script should prevent errors in those conditions anyway. Why not just exit the script if the POST data you are expecting isn't present: if(!isset($POST['q'])) { exit(); } -
spaxi, Using the DOM, as cyberRobot suggests is definitely the better approach. Using that you are far less tied to hard-coded logic. You could, for example, find the text just looking for rows that include a TD with the class "program_date". It wouldn't matter if it is enclosed in single or double quotes or whether there were additional parameters in the TD tag that you were not expecting. It will take more code, but it will actually be much more efficient. In the code below, I am using loadHTML() since I was using a string to test with. But, if you are referencing a web page you can pass a URL to the function and use loadHTMLFile(). function getPrograms($content) { //Create variable to hold results $programResults = array(); //Create DOM object $dom = new DOMDocument(); //Load content into DOM object $dom->loadHTML($content); //Get ALL TR objects from DOM $rows = $dom->getElementsByTagName('tr'); //Iterate through the rows foreach ($rows as $row) { //Get all TDs in current row $cells = $row->getElementsByTagName('td'); //Set flag for program date $programDate = false; //Iterate through all cells in current row foreach($cells as $cell) { //If programDate false, this is first cell. if(!$programDate) { //Test if this is program row, if not skip to next row if($cell->getAttribute('class') != 'program_date') { break; } //Set programDate for this row $programDate = trim($cell->nodeValue); //Skip to next cell continue; } //Add date to current programDate record $programResults[$programDate][] = trim($cell->nodeValue); } } //Return results return $programResults; } $programs = getPrograms($html); echo "<pre>" . print_r($programs, 1) . "</pre>"; Output (using the sample text you provided) Array ( [Mo, 09.06.2014] => Array ( [0] => 13:45 [1] => 16:15 ) [Di, 10.06.2014] => Array ( [0] => 13:45 ) [Mi, 11.06.2014] => Array ( [0] => 13:45 [1] => 16:16 ) [Do, 12.06.2014] => Array ( [0] => 13:45 [1] => 16:17 ) )
-
$disp->displayTomorrow(); Works for me.
-
mysqli_query() expects parameter 1 to be mysqli
Psycho replied to tobimichigan's topic in PHP Coding Help
function queryUniqueObject($query, $debug = -1) { $query = "$query LIMIT 1"; $this->nbQueries++; $result = mysqli_query($myconnection, $query) or $this->debugAndDie($query); $this->debug($debug, $query, $result); return mysql_fetch_object($result); } The mysqli_query() function is executed within a function/method. The variable $myconnection is not defined inside that function - thus the failure. You can resolve the issue by making 'myconnection' a property of the class: $this->myconnection Use that were you define the value and where you use it. Plus, declare it in the top of the class. -
PHP Script randomly restarts itself with blank post data
Psycho replied to holdorfold's topic in PHP Coding Help
Are you sure no one else is accessing it? There are bots out there (both benign and malicious) that will find pages. You could add some data to that logging to include the IP address. Not saying that is it, but it would help. In fact, I would have that script output a lot of other details such as the POST/GET data, etc. Then you can review the log to see exactly what is happening. It looks to me it is being called separately from the one instance that is running the loop. -
Some examples that may help: http://www.quirksmode.org/dom/domform.html http://jsfiddle.net/jaredwilli/tZPg4/4/ http://www.randomsnippets.com/2008/02/21/how-to-dynamically-add-form-elements-via-javascript/
-
Odd, you originally stated Which indicates you intend to use one at some point. But, now you say you must implement this w/o one. Then use a flat-file database. Once you set up a real database later it would be a simple process to transition the data and update the code and add/change the connection settings. You would already have the queries and logic set up for accessing/modifying the data.
-
Create a database and do it the right way. You are only creating more work and problems by trying to do this through flat files. Yes, there is a learning curve to get started with databases. But, the benefits are huge. For a simple blog it would not be a lot of work once you grasp the basics.
-
Well, I'm surprised how someone could work with MySQL for years and not use GROUP BY. There are many basic features that require it. Well, I guess you could always query all the ungrouped records and then get the results by processing all the individual records in the code. But, my god, what a waste that would be. Anyway, that is why I have a hard time telling you that function "X" is another one you would benefit from. GROUP BY is pretty pedestrian in my opinion. I would still suggest reading the names of the functions and their descriptions in the manual. You would then at least have an idea of what is available. Then, if you find a need for something, you can do the extra research to learn how to use it.
-
Start with what sections/features they want. I would probably look at some different school websites to see what they have available: school calendar, mission statement, location, about us, contact us, list of teachers/administrators, etc. etc. As you review these, think about whether the pages would be static, could be static or dynamic and which ones would be static. For example, a page about the mission statement would likely be static. There's no reason to build an elaborate editing feature for the mission statement page which might consist of various font and images when the mission statement would almost never change. Then there are pages that could be dynamic but don't have to be. That's a cost/benefit decision the client would make. Would it be more cost effective to pay the extra money to make it a page that can be managed through an editing page vs. hiring someone when those changes are needed. Then, there are pages that would almost definitely be dynamic: e.g. monthly calendar. But, even for these you have to consider the cost of building an elaborate process of updating vs. a simple solution. Perhaps the school produces a monthly calendar that gets printed each month. If so, the web page could be updated by the school simply by providing a way for them to upload an image rather than building an elaborate calendar system. So, do a review of various features on similar sights and think about those types of questions and the possible solutions. Then you can sit with the customer and ask them what they want. It's likely they have some ideas but haven't really though about the entire site. Maybe the initial reason they are wanting the site is to provide information in the event of an emergency and haven't thought about anything else. So, ask them what they want. They will likely only give partial or vague information. Using the information and analysis you did above you can then guide them into more refined requirements.