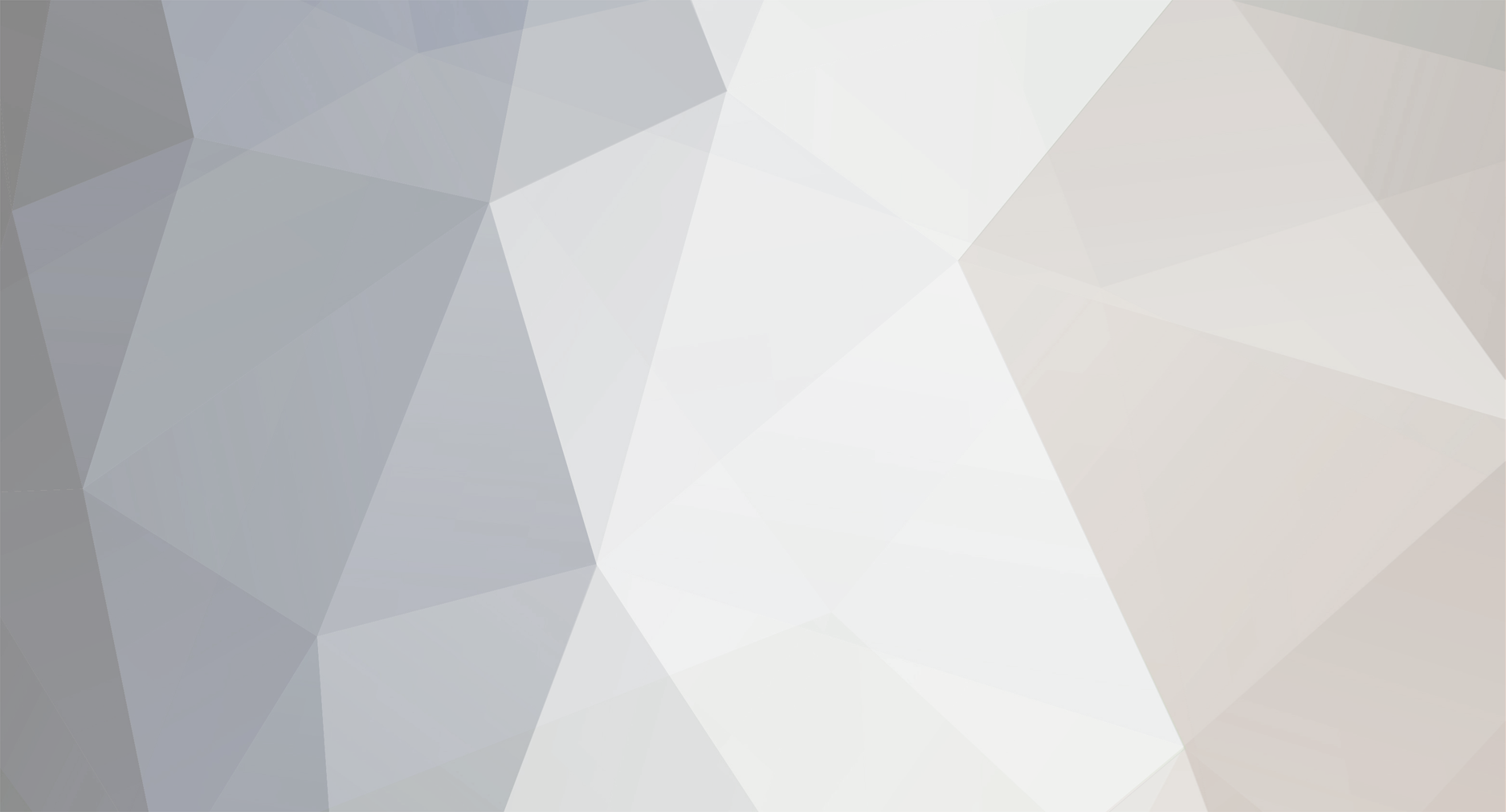
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Which would fail if you had three or more '|' in succession and would not resolve the problem where a '|' is at the beginning or the end of the string.
-
Here is a brief overview of how you can update it to ONLY get new messages on subsequent AJAX requests. All the code below is for illustrative purposes and I do not guarantee it will work without modification. 1. Change the query process to check for a passed timestamp. If none was passed get all messages up to 2 hours (or whatever you choose). This should be a one-time process for each new user. 2. Also add another value to the SELECT list to get the current timestamp from the MySQL DB. (Also need to remove the "*" from the SELECT list and include only the fields you need to be more deficient) 3. Instead of passing back everything as a string (i.e. echo'ing the output), instead create an array with the output and the timestamp (which you can get from the DB results). This can be converted to a JSON format. The AJAX that request the data (assuming you are using JQuery) should set the datatype to JSON as well. Alternatively, you could just append the timestamp and output togetehr with a dash (-) or some other delimiteer and then parse the two values with string manipulation in JavaScript. 4. The receiving JavaScript process should take the "output" portion of the result to append it to the current output of messages. Then take the last_update timestamp from the returned value to update a JavaScript variable. That variable should then be included on the next AJAX request. Then the whole process repeats. Below is a mock up of the PHP code that could support this. I didn't do anything about the JavaScript changes that would be needed as I am short on time. <?php include ("connect.php"); if(isset($_GET['last_update'])) { //Should add validation of this value $last_update = "'{$_GET['last_update']}'"; } else { //Default for first request $last_update = "(NOW() - INTERVAL 2 HOUR)"; } $query = "SELECT function, chatcolor, user, message, CURRENT_TIMESTAMP as last_update FROM messages WHERE `timestamp` >= $last_update ORDER BY TIMESTAMP DESC"; $find = "/me"; $output = ''; while ($row = $result->fetch(PDO::FETCH_ASSOC)) { $last_update = $row['last_update']; //Determine different style properties if($row['function'] == $find) { $recStyle = "font-style:italic;"; $userStyle = "color:tan;font-weight:bold;"; $msgStyle = "color:tan;" } else { $recStyle = ""; $userStyle = "color:{$row['chatcolor']};font-weight:bold;"; $msgStyle = "color:white;"; } //Append output to variable $output .= "<span style='{$recStyle}'>"; $output .= "<span style='{$userStyle}'>{$row['user']} " $output .= "<span style='{$msgStyle}'>{$row['message']}</span>"; $output .= "</span<br>\n"; } //Send the results $result = array( 'last_update' => $last_update, 'output' => $output ) return json_encode($result); ?>
-
The change I provided does not use a LIMIT and instead pulls everything int he last two hours - because that was what you had originally. So, it would be pulling ALL the records int eh last two hours (not just 20 for example), so I would expect it to use more overhead.
-
I already stated that you should post in the JavaScript forum (on this site). What you apparently want to implement is AJAX - which is a combination of JavaScript and a server-side process (such as PHP). I already explained the basic process you would probably want to follow in the 2nd paragraph of my first response. Here's the thing, it is not "difficult" but it requires the person implementing it to be familiar with JavaScript, PHP and how to make them work together. If you don't have that basic knowledge, trying to teach you will not be a trivial task. So, the only other option is for us to write it for you.
-
Lets Play Cards.. Help figuring out what is in your hand
Psycho replied to webdevdea's topic in PHP Coding Help
Yeah, I agree with ginerjm. But, to answer your question in a general sense, I would use session data to store the cards. Whenever there is a shuffle, create all the cards as part of the "deck" in an array in the session. Then, when cards are given to the player(s) remove them from the deck and assign to the player(s) - again in the session. Then, when players decide to exchange cards, remove them from the player array and get additional cards from the deck array. -
I would start with the activities/functions that app is supposed to solve. The obvious one is booking a room. Determine what input/output for that process would be required (which will help in DB design). From there you'll find there are plenty of others you need to consider. Here are some that I can think o Changing the amenities of a room Changing the rates for a room (or maybe it is dynamic based upon amenities) Reserving a room for a guest "Blocking" a room - can't be reserved/booked (repairs) etc. . . Now, you probably don't need an interface to add floors or room numbers. Maybe those are added directly in the DB since they would probably never change. I guess the number of rooms could if there were renovations. However, there is one aspect that you'll need to consider that can be somewhat difficult. When someone "books" a room the hotel does not necessarily book a specific room for that person (unless there is only one room of that type). This is because it could create problems of having certain date ranges being unavailable. For example, if you booked Room 1 from Mon to Wed and Room 2 from Thur to Friday. Then, if someone wants to book a room from Mon to Friday there would be no single room available. Plus, in some instances that will actually overbook to account for cancellations. I'm sure there are some best practices around how that is managed that you will need to research on.
-
You haven't provided enough information. You've posted this in the PHP forum, so either you want the user to make the selection and then submit the page to populate the textarea or you want to do it dynamically and have posted in the wrong forum (should be in JavaScript). Assuming you want it to be static, create an onchange trigger for the second select list. That trigger should call a function that will take the ID of the selected record and make an AJAX call to a page that will take that ID and get the description of the selected product. Alternatively, if you don't have a very large number of products, you can get the descriptions for all the products and create a JavaScript array. Then reference that array whenever the option is changed in the select list to populate the relevant description. This will provide better performance since you won't have to do a call to the server.
-
Error message - undefined index.....when running code
Psycho replied to hance2105's topic in PHP Coding Help
Am I supposed to just read through your files and try to figure out what your second select field is and why it is not being displayed? Look at where you are calling these functions. You aren't calling them within PHP <table width="1000" border="0"> <tr> <td><p align="right">Product Name</p></td> <td> <span id="spryselect3"> <select name="prod_name" id="prod_name" class="prod_name"> getprod_name(); ?> There's a closing PHP tag - but no opening tag. So, the function is treated as HTML In another instance you put PHP code in, but there is no error handling. So it could be the query is returning 0 results. <select name="cat" class="cat"> <option selected="selected">--Select Category--</option> <?php include('db_connect.php'); $sql=mysql_query("select id,cat_name from tblprod_cat ORDER BY id ASC"); while($row=mysql_fetch_array($sql)) { $id=$row['id']; $data=$row['cat_name']; echo '<option value="'.$id.'">'.$data.'</option>'; } ?> You really should revise how you are building your code. Here are my suggestions: 1. Create your functions so the RETURN the output to be displayed instead of echoing it. That is a bad practice. 2. Put all your PHP "logic" at the top of the page (or even in a separate PHP page) and just create variables with the output to be displayed. Here's an example. Change the function getprod_name() to something like this: function getprod_name() { $query = "SELECT DISTINCT prod_name FROM tbl_prodstd"; $result = mysql_query($query) or die(mysql_error()); $output = ''; while($row = mysql_fetch_array( $result )) { $output .= "<option value='{$row['prod_name']}'>{$row['prod_name']}</option>\n"; } return $output; } Note: I use double quotes to define the string so I can put the variables in the string and put a line break (\n) at the end of the string. The latter makes your HTML code readable to assist in debugging. Next. call the function at the top of the page (before any HTML output) where you need that select list and assign the output of that function to a variable. $productOptions = getprod_name(); Lastly, just output that variable within the HTML output of the page <select name="prod_name" id="prod_name" class="prod_name"> <?php echo $productOptions; ?> </select> Following some sort of patter such as this makes your code cleaner and more manageable. -
Look closer at your "src" parameter - something is missing. You need to learn how to work with either type of quote in any context. It shouldn't matter if the output is done inside or outside of an echo statement. I assume you are echoing the string within an echo that is defined using single quotes. There are multiple options to do what you were trying above (even though you can stick with your first attempt after correcting the src parameter). Here is one solution //Escape the single quotes inside the string echo '<input type="submit" name="submit" id="submit" style="background-image:url(\'path_to_image/add_to_cart.jpg\')"/>';
-
Error message - undefined index.....when running code
Psycho replied to hance2105's topic in PHP Coding Help
And what is your question? Do you not understand what the error is? The error is exactly as it states - you are trying to reference the index of an array and that index does not exist. Here is the line in question if($_GET['func'] == "prod_name" && isset($_GET['func'])) { So, the problem here is that unless you will ALWAYS be passing the variable 'func' on the query string every time this page is loaded you will get this error because the FIRST check you do in the if() condition is to test the value of $_GET['func']. So, if that index in the $_GET array is not set it produced the error. But, here's the thing, your SECOND validation checks to see if that variable is set. When you have an AND operator between two conditions the first condition is tested first - if it fails the PHP parser does not attempt to test the second condition (it's smart like that ). So you can fix your problem by simply switching the order of those conditions. if(isset($_GET['func']) && $_GET['func'] == "prod_name") { It will then first test to see if the variable isset() - which would not produce the undefined index error since the whole point of isset() is to check if the variable is set or not. If it isn't set the second check to test the value will not be executed. -
You would create a table in your database that lists ever file type and any information about that file type (e.g. the display icon) tha you want to store. Then you simply query that information as you need it. If this is a document management system I have to assume you are storing the meta data associated with these files in the database. If that is the case you have to already be querying the information about the files to display on the page. If so, you only need to have a filetypes table and do a JOIN query when retrieving the information about the files. Then you will already have the icon to display without creating any logic and a hundred or so if() statements. Example "files" table structure file_id, file_name, file_path, file_type_id, etc. Example "filetypes" table file_type_id, file_type_ext, file_type_icon, etc. Then, when you are going to INSERT a new file you determine the ext and do a SELECT query to get the file_type_id. Then do an INSERT into the files table with the requisite file info and the file_type_id. (note: I would include a default file_type_id to use when there is no matching extension) Then whenever you need to display the files on a page, you can include the file icon to use as part of the query to get the info SELECT * FROM files JOIN filetypes ON files.file_type_id = filetypes.file_type_id
-
Why don't you try testing it? Create a process to run both ways and run it in a loop 100 or 1,000 times and do a timing before and after the loop completes. heck, it might even be faster to just use the database for this. SELECT filtetype FROM types WHERE ext = '$extOfFile' In any case a long string of ifelse() statements is definitely the wrong way to go. With that pattern you should use a switch. But, again, that may or may not be more efficient than an array or using the DB. If you wrote the code for all of that manually you need to approach your coding differently. That is a complete waste of time and energy. I could probably convert your current code to an array or a SQL statement to insert into the DB within 5-10 minutes.
-
What part of the example I provided did you not understand? Put the code to create a row inside that loop. Put anything you need to display before those rows (e.g. the opening table tabs and headers) before the loop. And, put anything you need after those rows (e.g. the closing table tag) after the loop.
-
Your current code is pulling ALL the data in the query and sending it back to the client each time the call is made. Even if you only limit it to 20 records or the ones in the last two hours, it is inefficient and why I suggested a timestamp based approach so you only query and return the messages created since the last request and append them to the chat log. Or, you can implement a PUSH process where the data is sent to the browser automatically when new messages are created. I've never done it myself, but here is a pagewhere someone shows how to implement it: http://webscepter.com/simple-comet-implementation-using-php-and-jquery/
-
Below is a quick update to the chatlog.php script that should significantly help. But, I would still use the approach of passing a timestamp and only querying and returning the new messages since the client last requested an update. Also, here are some other tips: 1. Don't use short tags 2, Don't define a variable inside the loop if it will have the same value on each iteration of the loop 3. There were {} around a section of code with no control structure for it. 4. Don't use FONT tags, they've been deprecated for over a decade! Use style tags (or classes) 5. The output was always adding this to the output. Not only shoudl you not need it - it is invalid <head> <body bgcolor="black"> </head> Try this <?php include ("connect.php"); $query = "SELECT * from messages WHERE `timestamp` >= (NOW() - INTERVAL 2 HOUR) ORDER BY TIMESTAMP DESC"; $result = $db->query($query); $find = "/me"; $output = ''; while ($row = $result->fetch(PDO::FETCH_ASSOC)) { //Determine different style properties if($row['function'] == $find) { $recStyle = "font-style:italic;"; $userStyle = "color:tan;font-weight:bold;"; $msgStyle = "color:tan;" } else { $recStyle = ""; $userStyle = "color:{$row['chatcolor']};font-weight:bold;"; $msgStyle = "color:white;"; } //Append output to variable $output .= "<span style='{$recStyle}'>"; $output .= "<span style='{$userStyle}'>{$row['user']} " $output .= "<span style='{$msgStyle}'>{$row['message']}</span>"; $output .= "</span<br>\n"; } //Send the output echo $output; ?>
-
Well, one of the possible issues is that you are running a DELETE operation on every single call to update the chat log. DELETE's can be resource intensive - plus what is the reason behind deleting old messages? Seems that saving them could prove useful (e.g. someone posting inappropriate comments). You could change the logic to only SELECT the messages that were created in the last two hours rather than deleting the older ones and querying for ALL the messages. Better yet, have a JavaScript pass a timestamp since the last time it checked for messages and only return the new messages and append them to the output.
-
$max_rows = 3; for($row=0; $row<$max_rows; $row++) { // Insert code to create a row }
-
Um, I have to completely disagree with dalecosp. The "issue" you are having has nothing to do with a bad database design or with the query at all. When you have a one-to-many relationship in your database between tables and you do a normal JOIN query between those two table you will always get your results like that. There will be multiple records for each child element so the parent values will be duplicated. For example, here is what the results might look like when doing a query on a States table joining a Cities table: state | city CA Los Angeles CA San Diego CA Burbank CA Anaheim TX Austin TX Dallas TX San Antonio This is exactly how the results should be returned. The trick is in knowing how to process those results - not in changing the results. There are many ways to achieve what you want. One option is to create a "flag" variable that is used to check when the parent value is changed. Another is to dump the results into a multidimensional array. The process you should take will be dependent upon exactly what you are doing. I typically use the flag variable method, but when the logic for creating the output becomes more difficult I may rely upon creating an output array first. Here is an example script for illustrative purposes that should get you pointed in the right direction //Get the DB results $query = "SELECT * FROM vehicles JOIN pictures ON pictures.veh_id = vehicles.veh_id WHERE vehicles._sold = 'STOCKED' ORDER BY vehicles._make ASC"; $result = mysql_quer($query); //Process the DB results $current_vehicle = false; //Flag variable while($row = mysql_fetch_assoc($result)) { //If different vehicle from last record, display vehicle info if($current_vehicle != $row['vehicle_id']) { //Display vehicle name only for first record echo "<b>{$row['vehicle_name']}</b><br>\n"; //Set flag $current_vehicle = $row['vehicle_id']; } //Display each vehicle picture echo "<img src=\"$row['image_src']\" />\n"; } EDIT: Note that I used a normal (i.e. INNER) JOIN in that query. If it is possible for vehicles to exist without any associated pictures, then you should use a LEFT JOIN EDIT#2: Just in case someone responds with the suggestion of using GROUP_CONCAT. That MySQL function will allow you to get one record for each vehicle with all the associated images for the vehicle as a single concatenated value. I specifically did not suggest that because it doesn't scale well and, I believe, has a limitation on the total string length (Verified that the default is 1024 characters. It can be increased, but is a server-side configuration which you might not have access to change on a shared host and increasing it can have some negative consequences). Depending on how much of the file path you are including in the images that could be a problem for vehicles for many pictures. But, if your picture values will not be excessively long and vehicles will not have large number of pictures it might be an easier solution.
-
Could it be because you have TWO id references in the relevant input tag? The first one is empty. <input id='' size='40' id='recipient' value='recipient'/>
-
This is possible. No sure what ginerjm is referring to. Please show the code you are using There is no reason the output should be an HTML page - unless you are sending it that way by accident.
-
Undefined variable error message when running code
Psycho replied to hance2105's topic in PHP Coding Help
Since you are using it within a loop that is processing record from the DB, I suggest you include that ID in the SELECT part of your query so it will be available in the loop. -
Sounds like homework to me.
-
No, it's NOT a formatting issue if you are wanting to verify that the value entered may have been missing a digit. That is my whole point. Trying to validate the value as a "formatted" phone number is a waste of time unless you are restricting the user to a specific format for a particular business reason. You are wanting to validate that the value is valid for use as a phone number (verifying that it is an actual phone number is near impossible). So, what do you care if the user enters in "123-456-7890" or "123.456.7890" or any other format with those same 10 digits? Here is an example function that will return false if the value doesn't contain exactly 10 digits, otherwise it just returns the 10 digits with no other characters. You would then store just the 10 digits in the database. Then whenever you need to display the phone numbers on your site, create a formatting function that will display all the phone numbers consistently. It would look unprofessional if all the phone numbers were displayed differently based upon the user who entered them.. function valid_phone($phone) { $phone = preg_replace('#[^\d]#', '', $phone); if(strlen($phone) != 10) { return false; } return $phone; }
- 13 replies
-
- validation
- form
-
(and 2 more)
Tagged with:
-
I have done a lot of validations with respect to phones, names, addresses, etc. Are you really concerned about the format of the phone number entered, or are you really wanting to know that the value they have entered is valid as a phone number? A user may enter a phone number in many different formats, but could still be a "valid" number: 123-456-7890 (123) 456-7890 123.456.7890 123 456 7890 etc. etc. It would be a waste of time trying to build logic to validate on any possible way a human might enter he phone number. Unless you have a specific business requirement to verify the format of the value entered, just verify that the digits entered would make a valid number. Then you can strip-out all non-numeric characters and store that. Then, when you display the phone numbers you can format them in a consistent way for your application. So the logic could be a s simple as: 1. Remove all non-numeric characters 2. Test the length of the remaining characters
- 13 replies
-
- validation
- form
-
(and 2 more)
Tagged with:
-
Users can't, to my knowledge, directly manipulate data stored in session. The exploits exists when the application creates a hole. For example, you would never want to do something like this: $key = $_POST['key']; $value = $_POST['value']; $_SESSION[$key] = $value; the best advise I can give is to validate/escape ALL user input before you use it. Never assume any data is safe. For example, just because you give the user a select list to make a selection, don't assume that value is one that was in the list you created. Verify it is before you do anything with it.