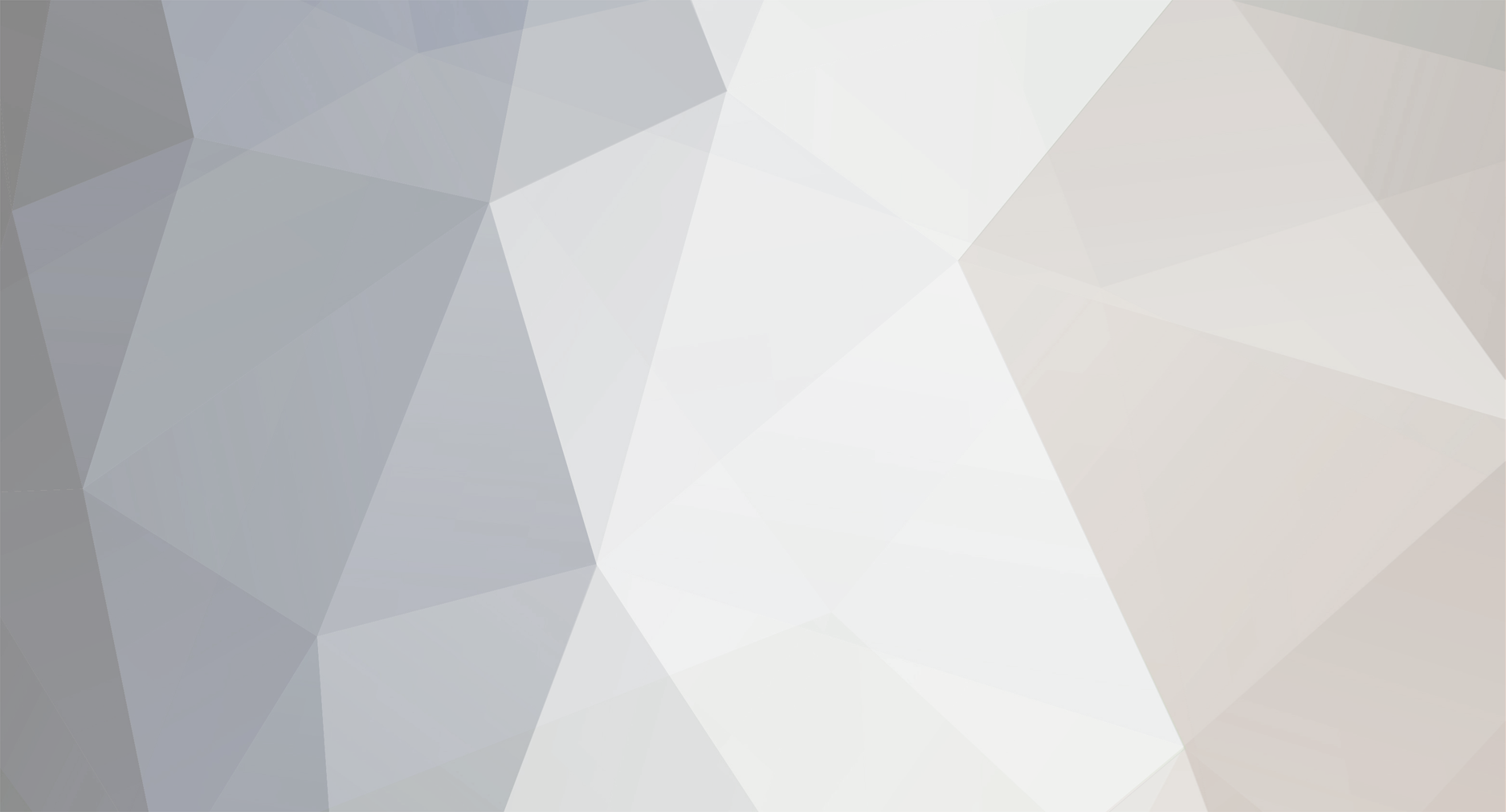
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Warning: mysql_num_rows() expects parameter 1 to be resource
Psycho replied to ZeTutorials101's topic in PHP Coding Help
You are getting an error. Change the line that executes the query to this $query = 'SELECT id, name, description, price FROM products WHERE quantity > 0 ORDER BY id DESC'; $get = mysql_query($query) or die("Query: $query<br>Error: " . mysql_error()); Note: putting the query into a sting variable is useful so you can echo the query to the page for debugging purposes. Here,the query is hard-coded (i.e. no variables) so it isn't really necessary, but it is a good habit to follow. -
You define a variable from an array in the post data $segment = $_POST['segment']; But, you then use that variable here: $email_message .= "Segment: ".clean_string($segment[])."\n"; You are passing $segment[] to the function. Using the brackets at the end of the variable is used to add a new value to the array. Plus, that function is simply returning the results of str_replace(). If you pass it an array, you will get an array as the result. You can't append an array to a string. The simplest solution (based upon my understanding of your code) would be to properly pass the variable to the function (i.e. without the []) and to modify the function to return a string. function clean_string($string) { $bad = array("content-type","bcc:","to:","cc:","href"); $result = str_replace($bad,"",$string); if(is_array($result)) { $result = implode(', ', $result); } return $result; } That will return the cleaned string OR if the value was an array it will return the array values concatenated with a comma and space as a string.
-
<?php $selected_value = false; if (isset($_SESSION['share_prefage']) && ctype_digit($_SESSION['share_prefage'])) { //Session value is number. Set selected value $selected_value = $_SESSION['share_prefage']; } $prefage_options = "<option value='Doesnt Matter'>Doesnt Matter</option>\n"; for ($i=18; $i<=99; $i++) { $selected = ($i == $selected_value) ? ' selected="selected"' : ''; $prefage_options .= "<option value='{$i}'{$selected}>Around {$i}</option>\n"; } ?> <select name="share_prefage" class="textarea" style="width:150px"> <?php echo $prefage_options; ?> </select>
-
This is obviously a homework assignment. At least make an attempt and post what you try.
-
I know that is just an example, but it is an example that can perpetuate bad habits. You should, typically, not use a comparison and then use that comparison to return true/false. Just return the comparison function isLogged($var) { return ($var == 'foo'); }
-
And, have you tried to change it as to how you need it? Just look at the code and follow the logic. It should be very simple to make sure "Doesnt matter" is always displayed. I'm not trying to be an ass, just trying to get you to think through the problem.
-
This would be better $firstTest = ($test == "yes") ? ($textCount > 1000) : ($textCount < 1000); if (($img == "0" && $firstTest) || $img == "") { //do this }
-
<?php $prefage_options = ''; $selected_value = false; if (!isset($_SESSION['share_prefage']) || !ctype_digit($_SESSION['share_prefage'])) { // no session value or session value is text $prefage_options .= "<option value='Doesnt Matter'>Doesnt Matter</option>\n"; } else { //Session value is number. Set selected value $selected_value = $_SESSION['share_prefage']; } for ($i=18; $i<=99; $i++) { $selected = ($i == $selected_value) ? ' selected="selected"' : ''; $prefage_options .= "<option value='{$i}'{$selected}>Around {$i}</option>\n"; } ?> <select name="share_prefage" class="textarea" style="width:150px"> <?php echo $prefage_options; ?> </select>
-
What are you getting output and what are you expecting to be output? EDIT: I ran that code and what I received was Output from first call to myTest(): X = 5, Z = 6, Y = 11 Output from first call to myTest(): X = 6, Z = 7, Y = 11 Output after second call to myTest(): X = 7, Z = 8, Y = 11 Which is correct. No where in that code is the value of $y being modified.
-
Well, $row is an array of a single record from the result set. So you can't echo it. You will need to echo the fields that you want displayed. If you only need rider_name and rider_number, then only include those in your query. Also, only query the fields you need, i.e. don't use "SELECT *" unless you really need all fields. Try this if(isset($_POST['submit'])) { //set variables from form $home = $_POST['home-team']; $away = $_POST['away-team']; $user_id = "2"; $meeting_date = "2013-05-13"; //$meeting_date=date("Y-m-d",strtotim($_POST['meeting-date'])); //create score card $new_card_sql = "INSERT INTO `tbl_card` (`user_id`,`meeting_date`,`home`,`away`) VALUES ('$user_id','$meeting_date','$home','$away')"; //$new_card=mysql_query($new_card_sql); print $new_card_sql; //find rider information $home_riders_query = "SELECT rider_name, rider_number FROM `tbl_riders` where `club_id` = '$home'"; $home_riders_sql = mysql_query("SELECT * FROM `tbl_riders` where `club_id` = '$home'") or die(mysql_error()); if(mysql_num_rows($home_riders_sql)) { while ($row = mysql_fetch_array($home_riders_sql)) { $data[] = $row; } foreach ($data as $row) { echo "{$row['rider_name']}: {$row['rider_number']}<br>\n"; } } //redirect to score-card.php //header("location:score-card.php"); }
-
FYI. This if (isset($_POST[''.$row['id'].'']) === true) { Should be just this if (isset($_POST[$row['id']]) === true) { No need to concatenate empty string to the variable
-
It is not working how? Are you getting errors or what?
-
What do you mean by "try" and what do you mean by "no errors"?
-
How not to display admin when fetching data from mysql user table
Psycho replied to malkocoglu's topic in PHP Coding Help
No, because we have no idea what you may, or may not, have in your database to identify what an ADMIN is. Do you have a column called "admin" with a 0/1 value? SELECT username FROM users WHERE admin = 0 -
If the name of the variable is any indication, I assume $page will be an integer or should at least be forced to be an integer. But, if the value really can be a range from 3 up to just less than 4, you can still use a switch. This is a "pro tip" that a colleague shared with me years ago regarding switch statements. On each case statement within a switch you put the comparison for the switch value. If they are compared as equal (i.e. true) then the code for that case is executed. But, if you want to use a switch statement where each case comparison would be a range or possibly multiple comparisons, you can do it by using the switch in a non-intuitive way. Use the Boolean true as the switch value and the conditions as the case statements switch(true) { case ($page<1): //Perform some action for values 0.0 to 0.9... break; case ($page>=1 && $page<2): //Perform some action for values 1.0 to 1.9... break; case ($page>=2 && $page<3): //Perform some action for values 2.0 to 2.9... break; case ($page>=3 && $page<4): //Perform some action for values 3.0 to 3.9... break; default: //Perform some action for values over 4 break; } This comes in very handy when you have a fixed set of results that are dependent upon multiple conditions as opposed to using a lot of nested if/else statements.
-
What Barand provided was where I was going (but I wasn't aware of the EXTRACT function). But, he has a typo. After looking up the EXTRACT function, the first parameter should be "MONTH_YEAR" not "MONTH-YEAR". Also, I believe in this change the sub query will need to use UNION ALL - otherwise it will only report 1 logged or 1 resolved record in each month for each user That should get you all the results for each user by each month. But, you have another problem to resolve when outputting the data. The query will only return data for each user by month where there is data. So, if a user did not have any logged/resolved issues in a given month, there will not be a record in the result. So, you will need a way in the processing logic to add a zero in the appropriate fields if there was no history for the user in that month. Also, I would make an update to the query to provide a given time period. SELECT member, logged, SUM(log) as logged_count, SUM(resolve) as resolved_count FROM ( SELECT logged_by as member, 1 as log, 0 as resolve EXTRACT(YEAR_MONTH FROM logged) as logged_montYear FROM incidents WHERE logged BETWEEN '2013-01-01' AND '2013-02-29' UNION ALL SELECT resolved_by as member, EXTRACT(YEAR_MONTH FROM logged) as logged, 0 as log, 1 as resolve FROM incidents WHERE logged BETWEEN '2013-01-01' AND '2013-02-29' ) as results GROUP BY logged, member
-
Never run queries in loops. If you intended this report to include more information than calls logged and calls received, you should have mentioned that in your last post. Although you want data to be broken out by month, you don't say if it should be all historical data or just historical data for a specific time period (e.g. last year). The solution will be different. I will assume you need the latter. Even if you don't need it now, you may in the future. So, you will need to have the sub-queries pull ALL the data with no GROUP BY. And then use multiple GROUP BYs on the outer query. I'll see if I can get something together. EDIT: Can you please provide the structure of the two tables. I see you replaced "incident_number" in the query I provided with "item" and not sure why you would have done that. What is the "item" field?
-
I'm really not following your original question. But,if you are saying that the code in your second post works - you need to change it. You should NEVER run queries in loops. It is very inefficient and can cause significant performance problems. You need to learn how to use JOINs in your queries. I'm really confused by the first query inside the loop. The outer query gets a list of records from the deposits table. But, the first query in the loop then uses the ID of the record to find the same record. You can also replace this code if ($row['method'] == "RS07") { $coins = $fetch['coins']; } if ($row['method'] == "EOC") { $coins = $fetch['eoc_coins']; } . . . by creating a dynamic value in the query Some other things: Don't use "SELECT *" unless you really need all the fields in the tables Don't use <FONT> tags. They've been deprecated for about a decade! Use classes or inline styles. Here is a rewrite that should work better. Not guaranteeing it doesn't have an error or two. I can't test since I don't have your DB $query = "SELECT d.id, d.user, d.method, d.value, IF(d.method='RS07', u.coins, u.eoc_coins) AS coins_val FROM deposits AS d JOIN users AS u ON d.user = u.username WHERE d.completed = 5 AND d.host = ? ORDER BY d.id DESC LIMIT 10"; $stmt = $db->prepare($query); $stmt->bindValue(1, $user_data['username']); $stmt->execute(); echo "<table>\n"; while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { echo "<tr>\n"; echo "<td style=\"color:#000000; font-size:10pt;\">\n"; echo "| ID: {$row['id']} |{$row['user']} | {$row['method']} "; echo "| Deposit: {$row['value']}<span style=\"font-size:8pt;\">M/Gp</span> | Now has {$row['coins_val']}<span style=\"font-size:8pt;\">M/Gp</span> |\n"; echo "</td>\n"; echo "</tr>\n"; } echo "</table>\n<br/><br/><br/><br/>";
-
Error using font for title Forum, sub forum and topic title.
Psycho replied to mostone's topic in Javascript Help
Help you how? Are you saying you don't know how to use a style sheet? You will need to put the font on your server and them provide a URL to that font in your style sheet as provided in the example code you found on the first link. FYI: The page you linked to for the font states "Free for personal use". If you are using this font on a website,that may violate that requirement. So, you may need to buy a license for the font. In any event you need to provide alternate fonts for the user to use. Even if downloading of fonts is supported you can't be guaranteed that it will necessarily work for all browsers and operating systems. For example, it may work on a PC but not a MAC. And, I would bet good money that a mobile device isn't going to download fonts. So, even if you can get this to work on your machine, be sure to define alternative fonts for the browser to use if it can't get the spy agency one to work. -
And, where is YOUR code that you are having problems with? And what, exactly, are the problems you are having?
-
Error using font for title Forum, sub forum and topic title.
Psycho replied to mostone's topic in Javascript Help
That is because the HTML page is rendered on the client machine. If you specify a font, the browser will look for that font on the user's machine. If it doesn't find it, it will use a different font. You can specify several different fonts so that, if the primary one you wnat isn't found it will look for an alternative that you choose. But, it will be very unlikely that your users will have the font "spy agency" installed on their PCs If your text MUST be displayed in a specific font, there are two options: 1. There are ways to download a font to the user's machine, but I've never been able to get it to work consistently. You can research this if you wish, but I wouldn't get my hopes up. 2. Create an image of the text using the font. Then use the image on your page. -
php form, only require if another field is filled..
Psycho replied to joetaylor's topic in PHP Coding Help
Well, I assume you are SETTING $mobphone using something like $mobphone = $_POST['mobphone']; So, it would always be set. You should check that it has a value. You can use empty(), but that has some downsides. For example a value of '0' will be considered empty. But, using the same logic as you have now, you could do this if(!empty($mobphone) && empty($texts)) { $error_message .= 'Error: Allow Texts yes or no required'; } -
Unless you want to refresh the page when the user clicks a column, you will need to do this with JavaScript. << Moving to JS forum >>
-
I think she was referring to the latter. Not so much the "newest of newbies", but someone who knows at least the very basics of loop structures. I think you would have gotten a little more sympathy if you had shown at least an attempt.
-
This is basic stuff, but..(multidimensional array count)
Psycho replied to Big_Pat's topic in PHP Coding Help
If the data is in a database, you can just calculate the values using a query. SELECT member, SUM(logged) as logged_count, SUM(resolved) as resolved_count FROM ( SELECT logged_by as member, COUNT(incident_number) as logged, 0 as resolved FROM table_name GROUP BY logged_by UNION SELECT resolved_by as member, 0 as logged, COUNT(incident_number) as resolved FROM table_name GROUP BY resolved_by ) as results GROUP BY member EDIT: Corrected query