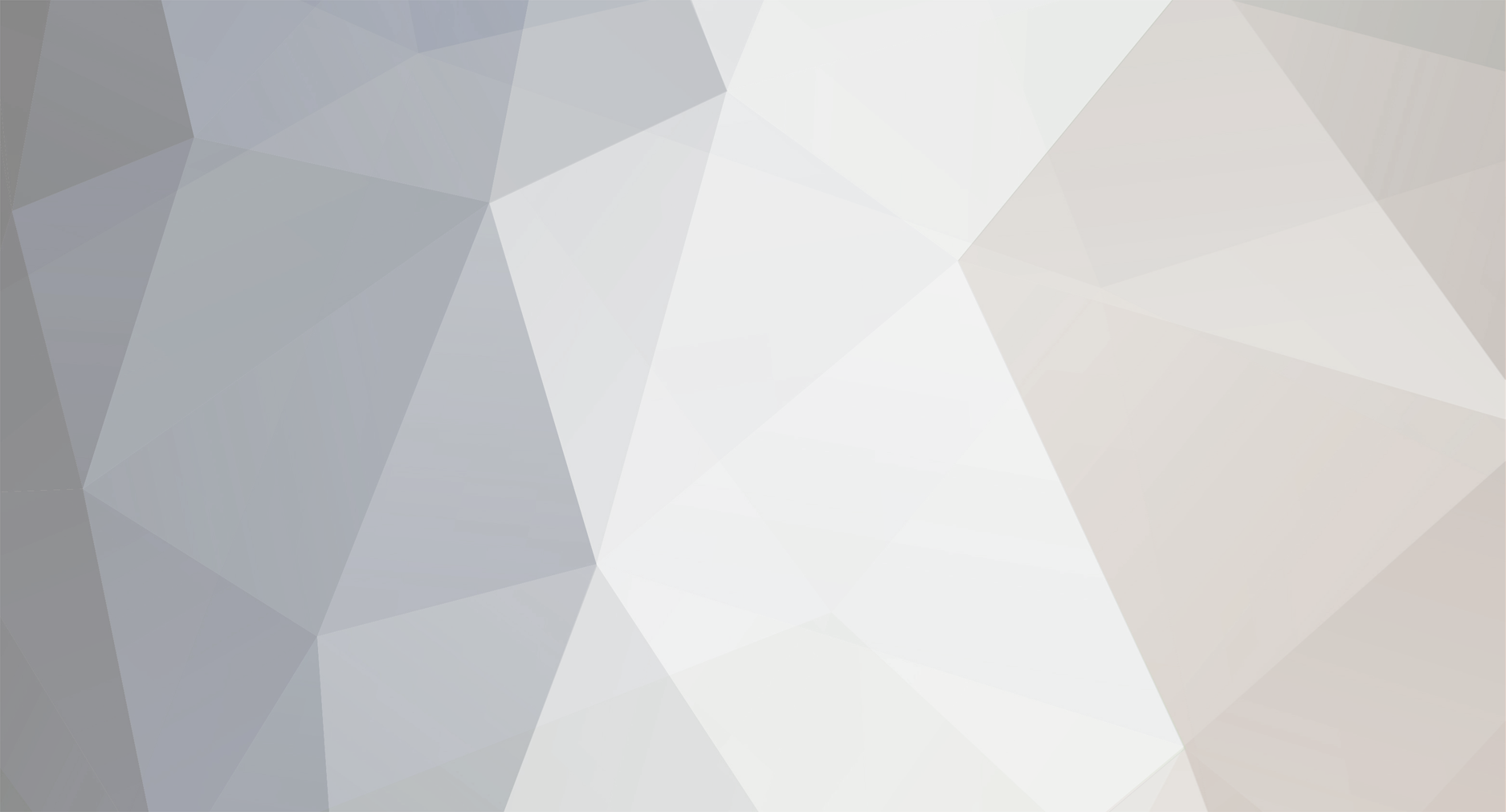
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Struggling w/ PHP/MySQL Role Based Access Control App I'm developing
Psycho replied to jdlev's topic in MySQL Help
Session data is stored on the server and is not available to the user. -
1. Do you really need LEFT JOINS? They are less efficient than a normal INNER JOIN. 2. Instead of using LIKE here, use an equal comparison insead And p.cat like 'Glassware' 3. No need to use a sub-query, just JOIN on the other tables and use group BY on the results
-
Struggling w/ PHP/MySQL Role Based Access Control App I'm developing
Psycho replied to jdlev's topic in MySQL Help
You need to build/find a simple login process. Included in that you should have a field in the user table to define the "type" of user: Admin, Funeral Home account, etc. As part of the login process you would store the User Type as part of the session data. Then whenever the user accesses a page or performs a process that should be secured you will check their type (and possibly their user ID) to determine if they should be allowed to view that page or perform that action. For example, you might have a page to create a new event for a funeral home. You may want to allow anyone of the admin type to add an event for any funeral home. But, for the funeral home accounts you probably want to only allow funeral home users to only add for their assigned funeral home. Pseudo code if($type != admin || ($type != funeral_home && $userHome != current_home) ) { //Error condition } //continue with page And, as I said above you also need to verify access on the "actions". You may have a page to list the current events for a funeral home with a delete link for each. In additional to preventing unauthorized access to that page, you also need to check access on the page that actually processes the deletions. Otherwise, someone could send random IDs on the query (or in POST data) and end up deleting records they shouldn't even be able to see. -
Most likely, your problem is due to the database design and the queries used. Have you indexed the relevant fields? Perhaps you can post the query as well. EDIT: And I really, really hope you are not running any queries in loops.
-
insert different lines from <textarea> to different mysql rows
Psycho replied to Th3Boss's topic in PHP Coding Help
Try something like this: <?php //Convert text input into lines based upon newline $textLines = explode("\n", $_POST['TextArea']); //Trim all the values $textLines = array_map('trim', $textLines); //Remove empty line $textLines = array_filter('trim', $textLines); //Escape input for query $textLines = array_filter('mysql_real_escape_string', $textLines); //Create parent record in table 1 $query = "INSERT INTO table1 (field1) VALUES ('value1')"; $result = mysql_query($query) or die(mysql_error()); $table1ID = mysql_insert_id(); //Process the lines into separate values for INSERT query $insertValues = array(); foreach($textLines as $line) { $insertValues[] = "('{$table1ID}', '{$line}')"; } //Create INSERT query $query = "INSERT INTO table2 (ID, Name) VALUES " . implode(', ', $insertValues); ?> -
Ok, after reading through your code I see this (which YOU should have pointed out instad of expecting us to do it for you) <input type="hidden" name="date" value="<?php date_default_timezone_set('Asia/Kuala_Lumpur'); echo date('d-m-Y H:i:s');?>" /> If you want to save a value in the database based upon when the form was submitted then don't put a hidden field in the form to store the time when the form was generated. You can simply: 1) Determine the time when the form was submitted in PHP code on the script that processes the form submission or 2) Use the database functionality to do it for you. #2 is definitely the way to go. If this is only for new record creation, then create your time field in the database as a timestamp and set the default value to CURRENT_TIMESTAMP. Then you don't even need to include a timestamp in the INSERT query - it will be saved automatically. If you need to update the value when records are edited you can also set the field to update the value automatically in the database.
-
Well, this forum is for people to get help with code they have written. Although, that doesn't mean we won't help people with code they were provided with, but the assumption is that the person requesting help has enough knowledge to communicate well on the subject at hand and can understand the code provided. If you didn't understand the code you were given you should have looked up the functions provided to understand what it was doing or ask the person who provided it. Per your last response, I can't really say if that is what you "should" do. But, after rereading your original post again, I think the problem is in these two lines: if ($data === $name) $printout .= "<td color=".$color.">".$data."</td>"; else $printout .= "<td class='schedule4'>".$data."</td>"; As I stated previously, you never defined $name (at least in the code you provided). But, what I *think* you want is IF the value is the color you want it to display as shown in the IF condition. But, look at your query and see what the field names will be for the color values - they will be Monday.color, Tuesday.color, etc. Looks like you were trying to have the IF condition be where the field was a "name" field - but again you never defined $name anyway. I really thing you should NOT try and make this too generic. Right now, your code relies upon the data being returned in a specific order (otherwise Tuesday would come before Monday). It is bad form, in my opinion, to create code that "assumes" data is returned in a specific order (there are exceptions). In this case you can write the code to specifically address the data. Give this a try. Not tested, so there may be some syntax errors. <?php $query = "SELECT t1.time, ti.Monday, t1.Tuesday, t1.Wednesday, t1.Thursday, t1.Friday, t1.Saturday, t1.Sunday, Monday.color, Tuesday.color, Wednesday.color, Thursday.color, Friday.color, Saturday.color, Sunday.color FROM Schedule t1 JOIN dj_colors Monday ON t1.Monday = Monday.name, JOIN dj_colors Tuesday ON t1.Tuesday = Tuesday.name, JOIN dj_colors Wednesday ON t1.Wednesday = Wednesday.name, JOIN dj_colors Thursday ON t1.Thursday = Thursday.name, JOIN dj_colors Friday ON t1.Friday = Friday.name, JOIN dj_colors Saturday ON t1.Saturday = Saturday.name, JOIN dj_colors Sunday ON t1.Sunday = Sunday.name"; $DB = new DB(); $result = $DB->select_multi($query); $printout = ''; foreach ($result as $row) { $printout .= "<tr>\n"; $printout .= "<td class='schedule4'>{$row['time']}</td>\n"; $printout .= "<td color=\"{$row['Monday.color']}\">{$row['Monday']}</td>\n"; $printout .= "<td color=\"{$row['Tuesday.color']}\">{$row['Tuesday']}</td>\n"; $printout .= "<td color=\"{$row['Wednesday.color']}\">{$row['Wednesday']}</td>\n"; $printout .= "<td color=\"{$row['Thursday.color']}\">{$row['Thursday']}</td>\n"; $printout .= "<td color=\"{$row['Friday.color']}\">{$row['Friday']}</td>\n"; $printout .= "<td color=\"{$row['Saturday.color']}\">{$row['Saturday']}</td>\n"; $printout .= "<td color=\"{$row['Sunday.color']}\">{$row['Sunday']}</td>\n"; $printout .= "</tr>\n"; } ?>
-
A couple notes on your code: if ($field == 'id' || $field == 'TimeFrame') continue; You should only query the fields you want to use. Also, I would write this code to explicitly work with the data instead of a generic loop. The reason why doing both of those (selecting all the records and making the processing code generic) is that it can lead to problems later on. Think about what would happen if someone needed to add a field to that table. It would be displayed in this output by default rather than a decision being made as to whether it should or not. From a security perspective, I think it is a bad idea to have code that would ever show any data by default if things were changed. if ($data === $name) $printout .= "<td color=".$color.">".$data."</td>"; else $printout .= "<td class='schedule4'>".$data."</td>"; I don't see where $name is ever defined. So, I'm not sure what your expectation is on that line. But, I would expect that all records where $data does not equal $name would default to the else condition. Since $name is not changed in the loop, that would only ever occur with one set of records.
-
Can you show the format of your tables? Just looking at your query I think you have built a database design that is going to be difficult to work with and maintain. EDIT: Also provide details on what the values are in "Monday", "Tuesday", etc. in the schedule table and the "name" field from the "colors" table.
-
That is for YOU to decide. I have no clue about the source of the data you are using, the requirements of the data to be stored, etc. etc. But, based upon your statements, you are getting records that should not exist. So you need to clean it up. One way would be to process the data into individual records for the database - removing/skipping any that should be considered "invalid", then running the query to insert all the valid records
-
And you are you processing those import files to create records in the database? If you are using something like MySQL "LOAD DATA" on a file that is not "clean" then you are going to end up with garbage. I'm guessing the same thing as davidannis. You should pre-process the file before creating the records to remove any invalid data.
-
Yes, of course. Simply do a check of the data before inserting.
-
No, I was not suggesting a "temp" table. Just create a new table to store the game sessions. Then associate the records that go into the current data table to the appropriate session.
-
how to use users own IP address while parsing a website
Psycho replied to rakibtg's topic in PHP Coding Help
Use JavaScript to request the external page from the client browser and then send the content to your server via AJAX to be processed and sent back to the client for output.- 6 replies
-
- php
- simple php html dom parser
-
(and 1 more)
Tagged with:
-
It all depends on how you want it to work. Here's a thought off the top of my head. 1. Create a session table with a session_id (auto-increment, start_time (default current_timestamp) and end_time. 2. When a user access the page to input the data, get the last record from the session table. If the session has an end_time, display output that there is no current session and give the user the option (button/link) to create a new session. If the session is still active (no end time) then display the normal form to the user, but also display something like "Enter data for the current session started as mm/dd/yyyy hh:mm.". Then give the user the option to close the previous session.
-
A flat-file shout-box is probably not a good idea as there is too much of an opportunity for multiple read/writes to be attempted concurrently. Some other notes: 1. You have a line of error checking that ends with an else statement to retrieve the new content. I would just have the error code and then close the if/else chain. You can then check if there were any error before getting/setting the messages. On that same note, I would define $errors = false at the top of the page and check for that instead of isset(). Or, better yet - set $error as an empty string. Then you can always display $error in the output without the need for an if condition. Also, I don't like stopping at the first error and instead prefer to find all errors to display to the user. But, what you have will work. 2. The logic for max messages is way over complicated. Never do something in loops that doesn't need to be. In this case, just add the message to the beginning of the array and then use array_slice() to get the max messages. Do this regardless of whether the messages exceeds the max or not. 3. There is no error handling for the reading/writing of the file 4. When there is POST data, at the end of writing the file you appear to do a redirect back to the same page. Why not continue the script and just display the data? I would simply process the post data - if it exists. Then, completely separately, display the data. 5. Your data is stored using a semi-colon as the deliminator. If a user puts a semi-colon in their data it will break the output. Use a character that can not be in the data. Since you are using htmlentities() you can use any character that would be transformed by that process, use a non-printable character (e.g. tab "\t") or something else to prevent that problem. 6. You use ucfirst() on the $name AFTER you saved the data using htmlentities(). This could result in some unexpected results if the character the user entered is transformed by htmlentities(). Either, save the data without htmlentities() and use that during the output or use ucfirst() before you run htmlentities. Personally, I try to store data in it's original state whenever possible and only transform when needed based upon the context.
-
The design is simple and clean, which I personally like. But, if you are doing this to promote yourself you may have to add a little more creativity. It all depends on the person who is viewing your site. The only thing that sticks out at me is the content at the bottom. The site is supposedly "your" personal website, yet the content at the bottom is in the third person. If it was your business website that might make more sense. But, as a personal website it seems off. Should be in first person, in my opinion. Also the line about "making many sacrifices along the way" seems like you're wanting some pity. I'd drop it.
-
You put your contact information into a public website and are surprised that people are contacting you. You could have simple asked people to send you a PM on this site. I am surprised that someone who is supposedly in the "web" business would do such a thing. Anyway, to answer your questions: There is no "Marked Solved" option in the Freelancing board because it is not a help forum. The ability to edit a post is only available for a limited time (30 minutes?). After that it cannot be edited by the user. This is so someone doesn't change/remove posts in the future. One of the draws of a help forum is previously answered questions which come up in search results for Google and such. If users could remove or modify the original posts months/years later it would negatively impact that ability. Part of the forum rules that you agreed to when you signed up was that any content you post is the property of PHP Freaks. You can make a request to one of the moderators to see if they are willing to help you.
-
Probably because of this WHERE `8` LIKE '%%' I tested the process of using TIMESTAMP() for a string formatted as a date against a field that was a datetime - so I know it works.
-
Then convert them to timestamps. MySQL has a ton of date/time functions. Just go read up on them to see what one meets your needs. http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html#function_timestamp $sql = "SELECT * FROM $db_tb_name WHERE `$db_tb_atr_name` LIKE '%$query%' AND `6` BETWEEN TIMESTAMP('$mydate1') AND TIMESTAMP('$mydate2')";
-
You have a field named '6'? Interesting. $sql = "SELECT * FROM $db_tb_name WHERE `$db_tb_atr_name` LIKE '%$query%' AND `6` BETWEEN '$mydate1' AND '$mydate2'";
-
Because a BLOB/TEXT field type is meant for a very large amount of data. PHPMyAdmin is trying to help you by not automatically displaying what would likely be a large amount of text in a table output - which would likely make the page unreadable. Let's say you were storing the text for an entire report in that field. It would be stupid to output that in the DB management display. If you are not storing LARGE amounts of text in that field you should by using something like VARCHAR with a limit on the number of characters. http://help.scibit.com/mascon/masconMySQL_Field_Types.html
-
Um, why are you using sprintf() and putting the variables IN the string??? Either don't use sprintf() or take the variables out of the string and use sprintf() as it is meant to be. Anyway, I'm not understanding your query completely, but the solution should be simple. Just query ALL the parts associated with the job (those with I and those with N). Then, in the SELECT statement do a calculation of the quantity based on the I or N to make qty value positive or negative. And, finally, add a GROUP BY on the part ID/No with a sum of the part QTY. Try this $query = "SELECT SUM(IF(Call_Parts_Used.Part_Status='I', Call_Parts_Used.Qty, Call_Parts_Used.Qty * -1)) as qty_used, Call_Parts_Used.Part_No, Call_Parts_Used.Date, Call_Parts_Used.Description, Calls.Order_No, Calls.Call_Ref, LU_Call_Part_Status.Part_Status_Desc FROM { oj (Siclops_Dispense.dbo.Calls Calls INNER JOIN Siclops_Dispense.dbo.Call_Parts_Used Call_Parts_Used ON Calls.Call_Ref = Call_Parts_Used.Link_to_Call) INNER JOIN Siclops_Dispense.dbo.LU_Call_Part_Status LU_Call_Part_Status ON Call_Parts_Used.Part_Status = LU_Call_Part_Status.Part_Status} WHERE Calls.Call_Ref = '$colname_rs_call' GROUP BY Call_Parts_Used.Part_No ORDER BY Call_Parts_Used.Description ASC";