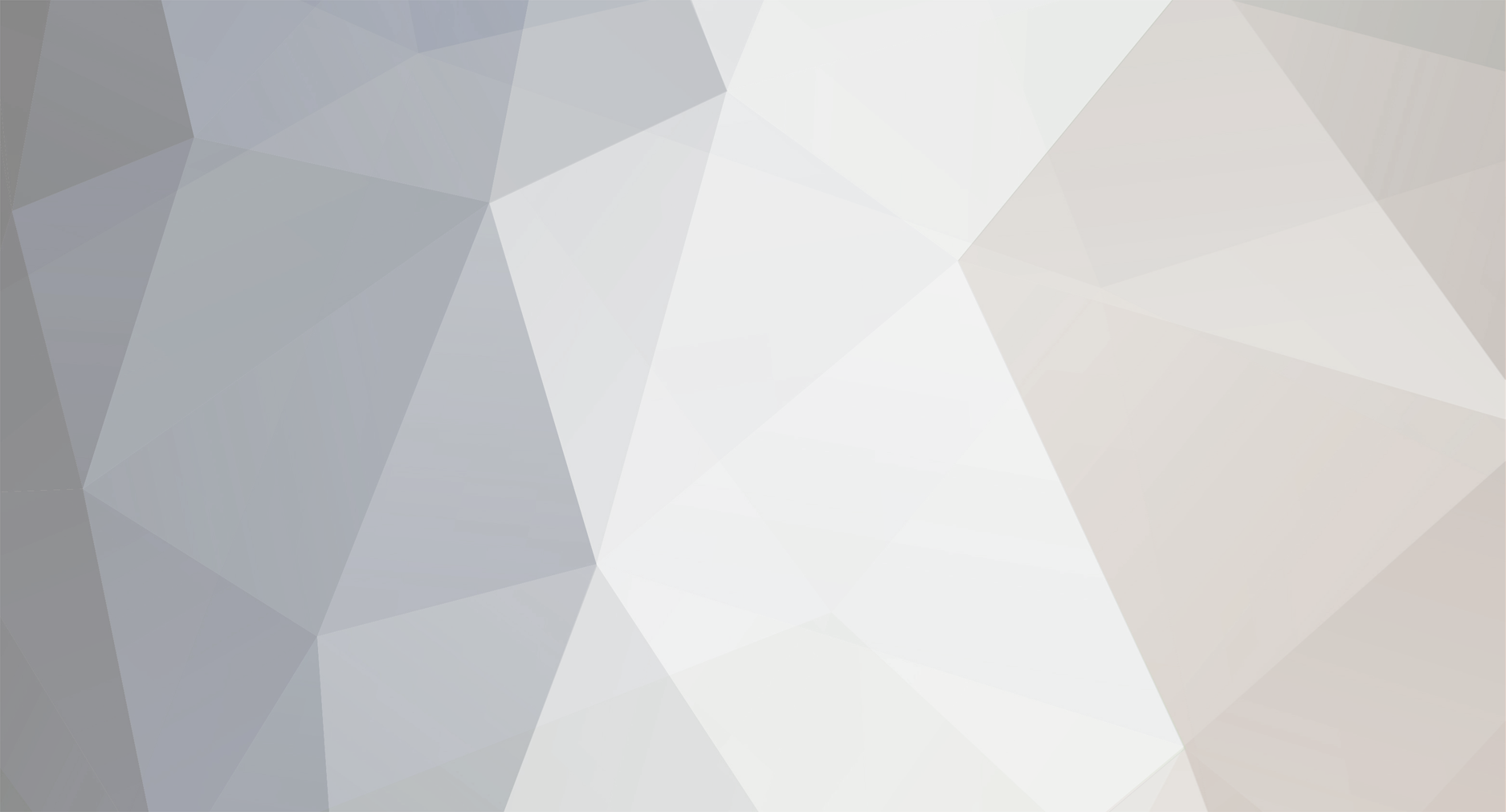
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Never run queries in loops. You handle that in the output logic in PHP. Example: <?php $query = "SELECT country, area FROM calendar GROUP BY country, area ORDER BY country, area"; $result = mysql_query($query); $currentCountry = false; while($row = mysql_fetch_assoc($result)) { //Check of this is a different country than the last record if($currentCountry != $row['country']) { $currentCountry = $row['country']; echo "{$currentCountry}<br>\n"; } echo " - {$row['area']}<br>\n"; } ?>
-
You should NEVER run queries in loops. You need to learn how to use JOINs. For example, the looping query can be replaced with this (i.e. no need for the first query) $query = "SELECT history.account_num, CONCAT(history.prescription,'-',history.dosage) as script FROM ci_prescription_history as history JOIN patient_info as patient ON patient.account_num = history.account_num WHERE history.script IN ({$scripts}) AND patient.dob IN ('1901', '1912', '1940')"; Note; this isn't tested since I don't have your database, but it should be close.
-
Yes. What day of week value are you wanting? Mon - Sun or 1 - 7? You would want to use date() along with strtotime(). After testing it looks like strtotime() can't use that string format as it is. You'll need to remove the comma. $date_string = "20 Dec 2013"; //Convert to timestamp $date_timestamp = strtotime(str_replace(',', '', $date_string)); //Output day of week string echo date('D', $date_timestamp); //Output: Friday //Output day of week number echo date('N', $date_timestamp); //Output: 5
-
If the number of elements is dynamic then you must be creating them using server-side code, correct? Why not just put in a class name on the last element and use that and be done with it.
-
You only want to use htmlspecialchars() on the VALUES for ($i=1; $i<=$num; $i++) { $fieldName = "opt_{$t}"; $fieldValue = isset($_POST[$fieldName]) ? htmlspecialchars(trim($_POST[$fieldName])) : ''; echo "<input type='text' name='{$fieldName}' value='{$fieldValue}'> "; }
-
I do understand you - I don't think you understand me. Right, even if you use numbers an attacker could change the numbers that are passed. But, you are guaranteed that if the number is one that does not exist in your list it can not be used to access other files or be used to interrogate your files. So, if the number is not in the list you simply ignore it or perform whatever error handling you want to. Now, if the file IS in the list and you need to somehow prevent the user from using some files and not others, using an ID does not necessarily provide a method to do that over using the file names. You would still need to implement that validation the same way. But, using an ID (correctly) will 100% guarantee that an attacker cannot pass various file names to use against your own scripts. So, rather than trying to add additional error handling to support file names, do it the right way and use an ID or some other non-descriptive value to identify which file to use.
-
No. You should not provide any information on the client-side regarding your system files. Not knowing what is in $scripts I can't say for sure, but the in_array() part of that condition would "probably" prevent a user from submitting a file that you are not expecting. But, the file_exists() would NOT! The reason is that the user could pass a file name in the format '../../inc/somefile.php' would would cnage the location that file_exists() is looking for the file. If you already have an array of the valid files why not use the index (i.e. ID) when you are passing the value from the client to the server. Bottom line is that using the actual file name to pass between the client and the server is a bad idea
-
Do you really have values in your database that are 50+ characters without any spaces? This is a "problem" I see reported all the time - especially when someone is testing max length restrictions on fields. This is an edge case scenario that doesn't need to be fixed in my opinion. Unless you expect that those types of values are going to be valid it's not worth the time and effort to add logic to handle it. But, to answer your question directly. I don't believe there is any way, with just HTML and CSS, to make a line of characters with no spaces or other "break" characters (dash, period, tab, etc.) break across lines. You would have to do that in server-side code.
-
Using readdir() was just marginally slower than using glob(), but I was able to code it to avoid the bug with folders that begin with a period. So, I guess I'll go with that.
-
Right, the problem is not specific to $_REQUEST - it is with any data sent from the user. You should not, in my opinion, have the file information accessible on the client. You don't need to have a hard-coded JavaScript with the file information. Just use a generic list of options on the client side and then translate those values to their actual value on the server. E.g. the list of "files" on the client might look like this in the JS code var files = new Array(); files[0] = 'fileA'; files[1] = 'fileB'; files[2] = 'file1C'; files[3] = 'fileD'; And when the user makes a selection, send the ID, not the value. Then on the server page take the ID and translate it into the actual file needed $files = array( 0 => 'path/filename.php', 1 => 'anotherpath/anotherfile.php', 2 => 'pathnumber2/differentfile.php', 3 => 'path/image.jpg' ); $fileID = intval($_REQUEST['fileID']); if(!in_array($fileID, $files)) { //User didn't select a valid file } else { //User selected valid file }
-
Hmm . . . file_exists() only returns True/False based upon whether the file exists or not. It doesn't execute any action against the file. But, it is probably a bad idea to allow a user to pass a file name/path to your script anyway as it gives them a way to find out information about your directory structure and file format. If these are files on the server then you should have the user select from a list of options where the file path/name are not exposed. Also, if you are checking if a file exists then I would assume you will be performing some action on it. In that case using $_REQUEST['filename'] would be a big risk.
-
The RecursiveIteratorIterator, RecursiveDirectoryIterator, etc. have me going in circles. I'm trying to create a very efficient method of getting a list of all the subfolders in a target folder. Right now, I have a process using glob() with the "GLOB_ONLYDIR" flag that is taking about 1/2 the time of the process I have using the iterator classes (which should be more efficient). I've also run into an issue where "GLOB_ONLYDIR" does not include folders that begin with a period. I believe I may not be using the iterator classes in the most efficient manner for what I need, as I am looping through the results to parse out the files. Here is my current (in progress) code: function getFolderList($startpath) { //Add the target folder to start the list $folders = array($startpath); //Create iterator for directory recursion $dirIterator = new RecursiveIteratorIterator(new RecursiveDirectoryIterator($startpath), RecursiveIteratorIterator::SELF_FIRST); foreach ($dirIterator as $folder) { //Skip any files in the result if(!$folder->isDir()) continue; //Add subfolders to the list $folders[] = $startpath . DIRECTORY_SEPARATOR . $dirIterator->getSubPathname(); } return $folders; } As you can see I am usign a foreach() and skipping the results that are files. In the manual it shows that the RecursiveDirectoryIterator class has the method RecursiveDirectoryIterator::getChildren. But, there is no documentation or examples. I would think there is a way to incorporate that into the instantiation of the iterator object so it only has the directories to begin with so I don't need to run a post-process operation to remove the files.
-
Several things in there don't make sense to me: 1. Why do you do a query using "SELECT *" and then only use the number of rows returned? If you don't need the data, then do a query for the COUNT(). It's also not obvious what the 'login' table represents. Are all logins recorded there or only failures? If all logins are record there then the logic would lock out valid users after 10 logins. 2. You only use the data from the first query if ($_REQUEST["error"] == "1"), so it is a waste of running that query if that condition is not true. 3. In the else condition, you do a second mysql_select_db() to the same database as before. To help further, I think we need to understand what data is stored in the 'login' table and what ($_REQUEST["error"] == "1") is supposed to represent.
-
search form to search two columns from mssql table
Psycho replied to bickyz's topic in PHP Coding Help
You don't have any error handling for the query - it is probably failing. My best guess, on the limited information provided, is that the item_id field may not be a numeric type (e.g. it may be a text type). Using the query $result = mssql_query("SELECT * FROM dbo.userAnswers WHERE item_id = $field AND contents LIKE '%{$search}%' "); would fail because the value for item_id would need to be in quotes. But, you should definitely be sanitizing any data from the user before using in a query (or use a method that handles it natively). I always suggest creating your queries as a string variable so you can echo it to the page for debugging purposes. In this case, you could do that and then run the query directly in the DB manager to see if that is the problem. -
Elements cannot have the same ID. Not sure if this is really the best solution for you since I don['t know what you are really trying to achieve, but . . <script type="text/javascript"> function showDivs(displayBool) { var divObjs = document.getElementsByClassName('answer_list'); var divLength = divObjs.length; for(var i=0; i<divLength; i++) { divObjs[i].style.display = (divObjs[i].style.display=='none') ? 'block' : 'none'; } } </script> <div id="welcomeDiv1" style="display:none;" class="answer_list" > WELCOME</div> <div id="welcomeDiv2" style="display:block;" class="answer_list" > <input type="button" name="answer" value="Show Div" onclick="showDivs();" /></div> <div id="welcomeDiv3" style="display:none;" class="answer_list" > WELCOME2</div> <div id="welcomeDiv4" style="display:block;" class="answer_list" > <input type="button" name="answer" value="Show Div" onclick="showDivs();" /></div> <div id="welcomeDiv5" style="display:none;" class="answer_list" > WELCOME3</div> <div id="welcomeDiv6" style="display:block;" class="answer_list" > <input type="button" name="answer" value="Show Div" onclick="showDivs();" /></div>
-
You need to be more specific when you say "on my site". If the user is clicking a favorite for example, you have no way to add the function that you are asking. As for links that are displayed within your site that point to URLs outside your site, YOU have control of the content on your site. You will need to change all those external links from <a href="http://www.anothersite.com">http://www.anothersite.com</a> To <a href="exit.php?url=http://www.anothersite.com">http://www.anothersite.com</a>
-
Well, it will definitely be slower since you will have to read the data from the database and then output the data appropriately. I'm not sure why you think it would think it is easier than just storing the path to a file. I tend to consider it a more complicated process. As for more secure, that is debatable. If you don't want the files publicly accessible you should be storing the files outside the root directory of the site. You can then use PH to read those files from the file-system and pass on to the user when they request them.
-
There are pros and cons to storing actual files in the database vs storing the files on the file system and just putting a path reference in the database. We cannot answer whether that is a good idea or not without knowing the reasoning behind storing them in the DB. From my experience, storing the files in the filesystem is typically the best approach unless you have some specific reasons not to.
-
Simple, $_SERVER['REQUEST_URI'] returns the value in the URL. If the URL includes the path to the file, then it includes the file. If the URL only includes the path to a folder and the webserver is picking a default file,then the file is not included in the value.
-
That code works for me but, as Jessica stated, it won't be what you think it should be. To make the subkey of the array be the value of $id you could either use double quites when defining the cookie or concatenate the variable setcookie("mycookie[$id]", $id, time()+3600*24); // . . . OR setcookie('mycookie['.$id.']', $id, time()+3600*24); And then you need to remove the variable from inside the single quotes when referencing it as well echo "Saved value: " . $_COOKIE['mycookie'][$id]; But, again, the code you have will work, but the key would just be the string '$id' (i.e. dollar sign, letter 'i', letter 'd'). One reason you may not bee seeing the value is if you are trying to reference the value on the same page load as when you set it. Per the manual: http://php.net/manual/en/function.setcookie.php EDIT: Also, not sure why you are setting the array key and the value using the same variable. Seems like a waste to me.
-
And what are you getting? Errors or not what you expect? I see at least one thing wrong on the basis of these two lines: $today = date("z") + 1; $Quote[0]='Quote goes here'; The 'z' will return "The day of the year (starting from 0) 0 through 365". And you are adding 1 to that value. So, $today will be a value from 1 to 366. But, your array has indexes of 0 to 365. Remove the +1. EDIT: Here's another problem $Quote[100]='Quote goes here'; $Quote[101]='Quote goes here' $Quote[102]='Quote goes here' $Quote[103]='Quote goes here'; Missing the semicolon at the end of those two lines Edit #2: and another two lines missing semi-colons $Quote[300]='Quote goes here'; $Quote[301]='Quote goes here' $Quote[302]='Quote goes here' $Quote[303]='Quote goes here'; Funny how I get errors such as this before I made those corrections. Wonder what that line number is telling me.
-
For an ID field you would normally want to verify/force the value to be an integer instead of mysql_real_escape_string().
- 8 replies
-
- help
- show current users characters
-
(and 2 more)
Tagged with:
-
First off, change your field names so the groups of fields will be in an array Car 1: <input type="text" name="cars[0][fram]" /> <input type="text" name="cars[0][year]" /> <input type="text" name="cars[0][ton]" /> <input type="text" name="cars[0][model]" /> Car 2: <input type="text" name="cars[1][fram]" /> <input type="text" name="cars[1][year]" /> <input type="text" name="cars[1][ton]" /> <input type="text" name="cars[1][model]" /> . . . .etc. Now, you can iterate over each group of fields in a more simple/logical manner foreach($_POST['cars'] as $car) { echo "{$car['fram']}\t{$car['year']}\t{$car['ton']}\t{$car['model']}\t<br>"; } As for limiting the number of records you process - it all depends. If you limit the number of field sets that are created then the foreach() loop will work perfectly. Else, if you will always have 10 fieldsets but only want to process n, then you can use array_slice() to trim the array to the number of elements you want.
-
I think you got some misinformation or have confused two functions. htmlentities() is used to make content safe to display on a web page so the characters are not interpreted as HTML code. When displaying user input on a page, a user could include characters such as '<' that make the browser confused and completely corrupt the output. Or worse, a user could insert malicious JavaScript code that could hijack your visitors. There is no good reason to use htmlentities() on data used in a DB query. You should be using an appropriate function to sanitize the data for use in a DB query, such as mysql_real_escape_string(). However, if you used htmlentities() on the data when you stored it,then you are stuck. You shoudl go back and clean up the existing data and start doing it correctly. As for your most recent query, you should (as Jessica stated) use the user's ID instead of the username. That appears to be the field accounts.id. Also, no need to put "ORDER BY owner ASC" when you only want the results for one owner. So, using the user's ID, this should work - you would need to set $userID beforehand, obviously. $sql = "SELECT accounts.username, characters.owner, characters.name, characters.money FROM characters LEFT JOIN accounts ON accounts.id = characters.owner WHERE accounts.id = '$userID'"; Note: I removed accounts.id from the SELECT clause since you would have to have that information before running the query anyways.
- 8 replies
-
- help
- show current users characters
-
(and 2 more)
Tagged with:
-
And, change all passwords: ftp, database, etc.