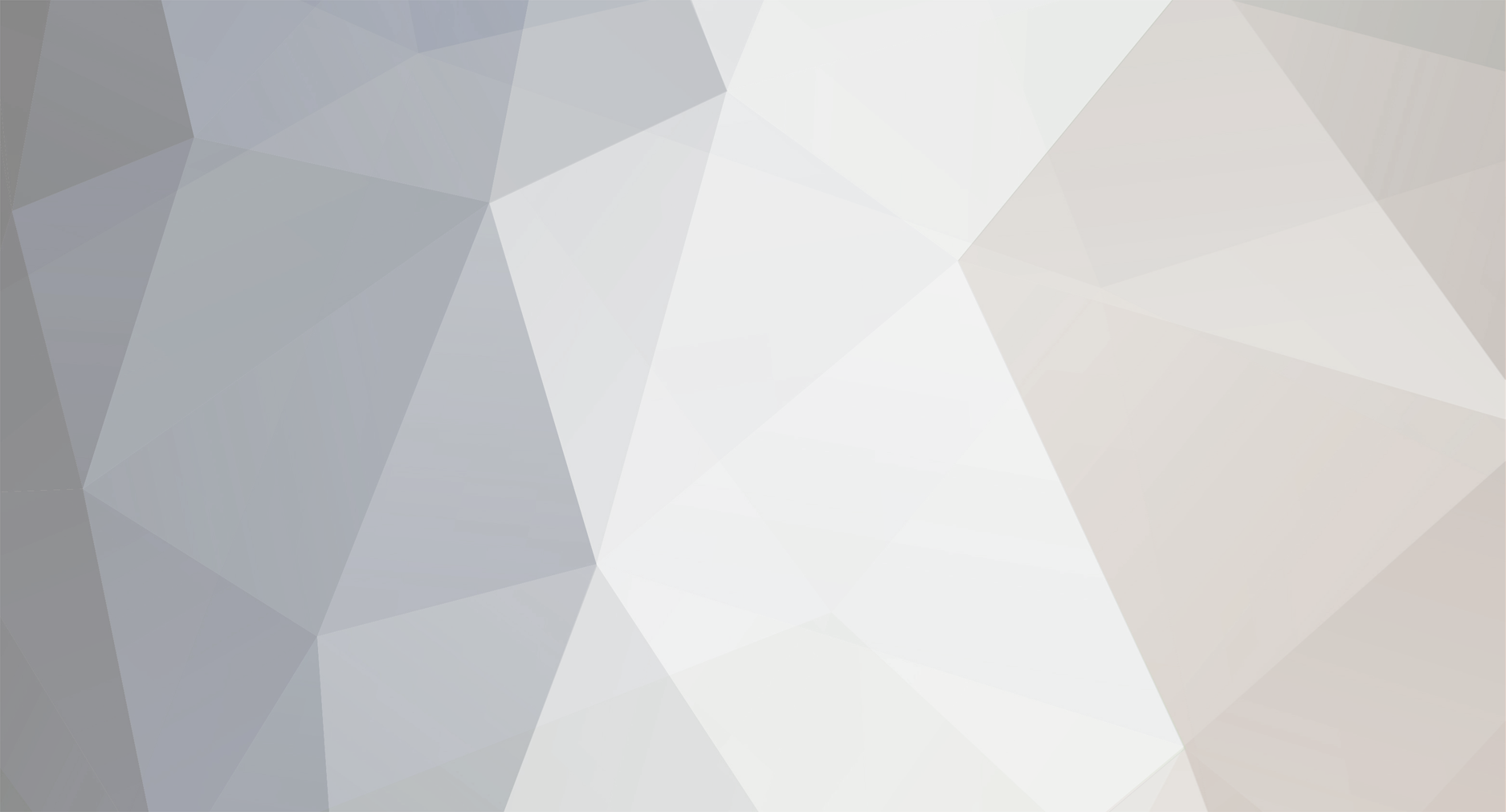
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
Try actually defining the variable $go. It probably was a change in register_globals. <?php $go = $_GET['go']; if ($go == "ga") $action2 = "ga"; elseif ($go == "ga06") $action2 = "ga06"; elseif ($go == "ga05") $action2 = "ga05"; elseif ($go == "ga04") $action2 = "ga04"; elseif ($go == "ga03") $action2 = "ga03"; elseif ($go == "ga07") $action2 = "ga07"; elseif ($go == "ga08") $action2 = "ga08"; else $action2 = "info2"; ?>
-
[SOLVED] Echo HTML after 'X' amount of words
pocobueno1388 replied to epicalex's topic in PHP Coding Help
Okay, here is an example doing what you want. <?php $str = "Sample string of words Sample string of words Sample string of words Sample string of words Sample string of words Sample string of words"; $words = explode(" ", $str); $middle = count($words) / 2; $count = 0; foreach ($words as $word){ echo $word. ' '; if ($count == $middle) echo "<b><u>Some HTML</u></b> "; $count++; } ?> -
[SOLVED] Echo HTML after 'X' amount of words
pocobueno1388 replied to epicalex's topic in PHP Coding Help
EDIT: Sorry, I misread the post. I'll post the new code in a second. -
If you look at the code I supplied above, that will tell you which checkboxes were selected by giving you the value of each one. So try this code and you will see how it works <?php if (isset($_POST['submit'])){ foreach ($_POST['check'] as $key => $val){ //this is where you would build your query echo $val.'<br>'; } } ?> <br> <form method="post"> 1<input type="checkbox" name="check[]" value=1 /><br> 2<input type="checkbox" name="check[]" value=2 /><br> 3<input type="checkbox" name="check[]" value=3 /><br> <input type="submit" name="submit"> </form>
-
Something like this <?php if (isset($_POST['submit'])){ foreach ($_POST['check'] as $key => $val){ //this is where you would build your query echo $key . ' - ' . $val . '<br>'; } } ?> <form> <input type="checkbox" name="check[]" value=1 /> <input type="checkbox" name="check[]" value=2 /> <input type="checkbox" name="check[]" value=3 /> <input type="submit" name="submit"> </form> If you give a little more of an explanation it would be easier to show you how to build the query.
-
See if this gives you what you want. SELECT u.u_id , u.d_id, u.f_name, u.l_name, u.d_name, u.email, u.password, u.date_added, u.status, d.d_name FROM users u LEFT JOIN departments d ON d.d_id = u.u_id
-
Yes. Try that out and see if the array comes out how you want it.
-
Can you show us the HTML output of your checkboxes? Basically you want to name all your checkboxes something like "check[]". Then when the form is submitted all the values will already be stored in the array called $_POST['check'].
-
Is this what you want? <?php $num = mysql_num_rows($result); echo '<select name="name">'; for ($i=1; $i<$num; $i++){ echo "<option>$i</option>"; } echo '</select>'; ?>
-
Your going to have to give a lot more detail than that. I don't even see a question.
-
[SOLVED] Help with a php news script..
pocobueno1388 replied to Cory94bailly's topic in PHP Coding Help
Try this for test2 <?php if(isset($_POST['submit'])) { // Make a MySQL Connection mysql_connect("***", "***", "***") or die(mysql_error()); mysql_select_db("***") or die(mysql_error()); $news = mysql_real_escape_string($_POST['news']); // Insert a row of information into the table "example" $query = mysql_query("INSERT INTO news (news) VALUES ('$news')")or die(mysql_error()); echo "News posted!"; } else { ?> <form action="<? $_SERVER['PHP_SELF']; ?>" method="post"> <textarea name="news"></textarea> <input type="submit" name="submit" value="submit"> </form> <?php } ?> EDIT: The problems I fixed were: -You were using $_POST['username'] instead of $_POST['news'] -Your submit button wasn't named "submit", so the insert code would have never executed. -I used mysql_real_escape_string() on your variable from the form, which is the least you could do. -
How to use a submit button to pass vars in a URL
pocobueno1388 replied to $username's topic in PHP Coding Help
Are you sure it's not already? Since you don't have the "method" attribute set on the form it should by default be "get". Try <form action="" class="searchform" method="get"> -
http://www.phpfreaks.com/forums/index.php/topic,95426.0.html
-
You would do it like this: include($_GET['module'].".php"); This isn't the safest thing to do. Make sure you do a check on the file they put in the URL and make sure you want them to have access to it.
-
Look at the MySQL DATE_FORMAT() function.
-
What happens? Do you at least see the "Thank you" message? Add a die() to the end of your query mysql_query("INSERT INTO $table (col_2, col_3, col_4, col_5) VALUES ('$name', '$email', '$subject', '$message')")or die(mysql_error());
-
http://php.about.com/od/advancedphp/ss/gd_library.htm Google will give you plenty of results.
-
It's a library of image functions. http://us2.php.net/gd
-
Check your database and make sure there is a table called "probid_dateformat". You probably spelled it wrong.
-
Change this line: $sql_select_dateformat = mysql_query("SELECT * FROM probid_dateformat"); To: $sql_select_dateformat = mysql_query("SELECT * FROM probid_dateformat")or die(mysql_error()); See if you get an error. I'm guessing you spelled the tables name wrong.
-
<?php //Query database to check if entry already exists $query = mysql_query("SELECT entryID FROM table WHERE {condition}"); //If it does exist if (mysql_num_rows($query) > 0){ $row = mysql_fetch_assoc($query); echo "Entry already exists, the id is {$row['entryID']}"; //if it doesn't exist } else { //insert entry to DB $insert = mysql_query("INSERT INTO table (...) VALUES (...)"); $id = mysql_insert_id(); echo "New entry added, the id of the new entry is $id"; } ?>
-
Thanks, but I fixed it. Here is the final code: <?php if (isset($_POST['update'])){ //make sure all powers are in array $powers = array("news", "forum_addDelCategory", "forum_moderate", "chat"); foreach ($powers as $pow){ if (!isset($_POST['power'][$pow])) $_POST['power'][$pow] = NULL; } $get_mods = mysql_query("SELECT userID FROM moderators"); while ($row = mysql_fetch_assoc($get_mods)){ $update = array(); foreach ($_POST['power'] as $field => $userID){ if(@in_array($row['userID'], $userID)) $update[] = $field.'=1'; else $update[] = $field.'=0'; } $sql = "UPDATE moderators SET " . implode(", ", $update) . " WHERE userID={$row['userID']}"; mysql_query($sql) or die(mysql_error()."<p>$sql<p>"); } } ?>
-
Honestly, I don't see a lot of use for a book with all the web tutorials around. Just look in my signature and check out those sites, they teach you a lot. Plus if your reading tutorials online, then you can follow along and try things yourself. If you prefer a book though, there are a lot of good ones out there. I remember my first post on this forum, I knew barely anything. Now that I've stuck with it and have been programming on a regular basis, I can now help many people on this forum.