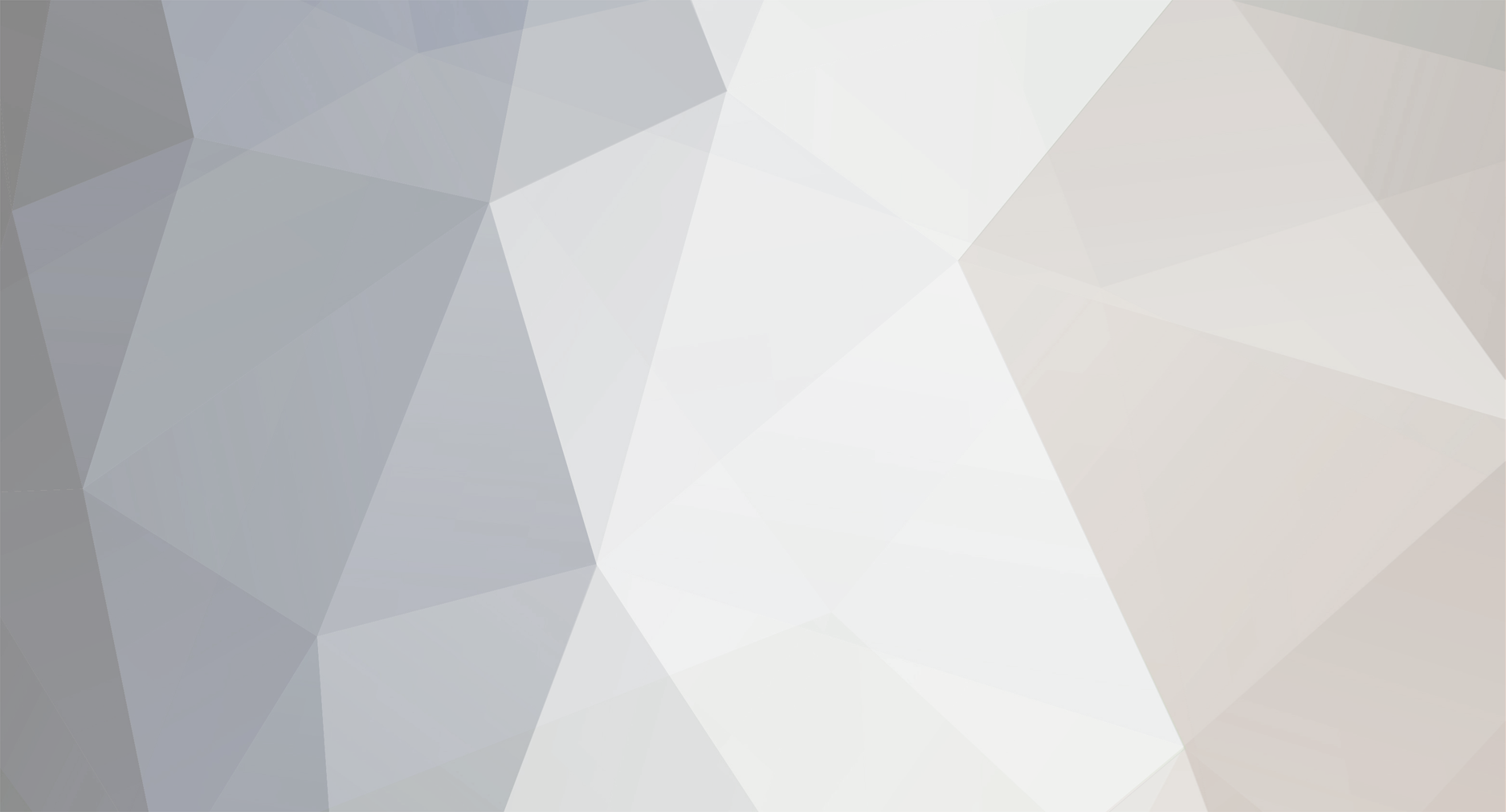
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
Try this SELECT value1, value2, (value1 * value2) as total FROM test_oh WHERE total >= 300
-
$location = $_SERVER['REQUEST_URI']; header("Location: $location");
-
All you need to do is select the information from the database, and use a while loop to display it all. EX <?php //select info from db $query = mysql_query("SELECT * FROM table"); //display it using while loop while ($row = mysql_fetch_assoc($query)){ echo $row['col']; } ?> You just need to add the table in there. Read these tutorials http://www.tizag.com/phpT/fileopen.php http://www.tizag.com/phpT/fileread.php
-
Your going to need to explain a lot more.
-
This is a nice class for this http://htmlpurifier.org/
-
You need to have some sort of way to know whose profile is being viewed. You can do that by either using a session variable for the user that holds their ID or something of the sort, or you could do it through the URL, such as: www.your-site.com/profile.php?userID=5 Then you can use either one of those variables to create your query. Also, there is no need for a while loop when you only expecting one row from the db.
-
My guess is your image input type isn't acting as a submit button, and that $_POST value isn't going through, which would give you a blank page because of the if statement returning false. Try this and see if you get the error message. <?php if (isset($_POST['Register'])){ //check email validity function checkEmail($Email) { if(eregi("^[a-zA-Z0-9_]+@[a-zA-Z0-9\-]+\.[a-zA-Z0-9\-\.]+$]", $Email)) { return FALSE; } list($EmailName, $EmailDomain) = split("@",$Email); if(getmxrr($Domain, $MXHost)) { return TRUE; } else { if(fsockopen($Domain, 25, $errno, $errstr, 30)) { return TRUE; } else { return FALSE; } } } //check captcha input match If($_SESSION['security_code'] != $_POST['security_code'] || empty($_SESSION['security_code'])) { Header("location: Login.php?register&SecurityCodeError"); Die; } //check username $Username = mysql_real_escape_string($_POST['Username']); If(strlen($Username) > 20 OR strlen($Username)< 1 OR !(preg_match("/^\w+$/",$Username))){ Header("location: Login.php?register&UsernameError"); Die; } //check password $Password = mysql_real_escape_string($_POST['Password']); If(strlen($Password) > 20 OR strlen($Password) < 5 OR !(ctype_alnum($Password))){ Header("location: Login.php?register&PasswordError1"); Die; } //check re-entry of password $Password2 = mysql_real_escape_string($_POST['Password2']); If ($Password != $Password2) { Header("location: Login.php?register&PasswordError2"); Die; } //check string length of email $Email = mysql_real_escape_string($_POST['Email']); If(strlen($Email) > 50){ Header("location: Login.php?Register&EmailError"); Die; } //calls function to check email validity if(checkEmail($Email) == FALSE){ Header("location: Login.php?Register&EmailError"); Die; } //username exists check $CheckUsername = mysql_query("SELECT Username FROM `usertable` WHERE `Username` = '$Username'"); If (mysql_num_rows($CheckUsername)>0) { Header("location: Login.php?register&UsernameTakenError"); Die; } // email exists check $CheckEmail = mysql_query("SELECT Email FROM `usertable` WHERE `Email` = '$Email'"); If (mysql_num_rows($CheckEmail)>0) { Header("location: Login.php?register&EmailTakenError"); Die; } $IP = $_SERVER["REMOTE_ADDR"]; $Date = date("Y-m-d H:i:s",time()); $Password = md5($Password); $EmailCode = md5(uniqid(rand(), true)); $Query = "INSERT INTO `usertable` (Username,Password,Email,IP,RegisterDate,ActivateCode) Values ('$Username', '$Password', '$Email', '$Country', '$IP', '$Date', '$EmailCode')"; mysql_query($Query) or die(mysql_error()); Unset($_SESSION['security_code']); Header("location: Login.php?Register&Success"); } else { echo 'ERROR: No such variable as $_POST[register]'; } ?>
-
If I understand what your wanting, try this: <?php $aUsers = array( "Ädams, Egbert", "Zaun, Jillie", ); $sql = "SELECT * FROM `members`"; $show = @mysql_query($sql,$connection) or die(mysql_error()); while ($row = mysql_fetch_array($show)){ $aUsers[] = $row['name']; } //new array with names added echo '<pre>',print_r($aUsers),'</pre>'; ?>
-
Where are you getting the variable $_SESSION['new'] from? Also, you would need to call session_start() at the top of the page in order to maintain the session.
-
Try <?php $query = "SELECT nick, (Monday + Tuesday + Wednesday + Thursday + Friday + Saturday + Sunday) AS added FROM table_name ORDER BY added DESC LIMIT 10"; $result = mysql_query($query)or die(mysql_error()); $i = 0; while ($row = mysql_fetch_assoc($result)){ echo $i .'. '.$row['nick'].'<br />'; $i++; } ?>
-
Here are two pages I have bookmarked http://dryicons.com/free-icons/page/1/ http://speckyboy.com/2008/01/01/top-25-free-icon-resources-for-web-designers/
-
Instead of this line: $id = basename($_SERVER['PHP_SELF']); I think your wanting this: $id = $_GET['id'];
-
The edit link needs to look something like this: www.your-site.com/script_name.php?editID=UNIQUE_ID Then, at the top of your script, your code should look something like this <?php //check if they want to edit something if (isset($_GET['editID'])){ $editID = $_GET['editID']; //select the information from the database that they want to edit $query = mysql_query("SELECT user, os, asset_name, mac, ip FROM inventory WHERE unique_field='$editID'"); $row = mysql_fetch_assoc($query); //put the information in text boxes echo "User: <input type='text' name='user' value='{$row['user']}'>"; } ?> Wherever it says UNIQUE_ID is where you want to use the field from the database table thats unique, that way you know which information to select.
-
-I don't like the header image, it doesn't look very professional -The links under the header image don't look good and are very unorganized looking. A drop down menu seems like it would fit nicely right there. -I would personally take away the "Good Evening" message, that will also allow the middle column to align with the ones to the side of it.
-
Try <?php include 'config.php'; include 'opendb.php'; $pageUR1 = ereg_replace("/(.+)", "", $_SERVER["SERVER_NAME"].$_SERVER["REQUEST_URI"]); $curdomain = str_replace("www.", "", $pageUR1); $query = "SELECT COUNT(*) FROM page WHERE page_url='$curdomain'"; $result = mysql_query($query)or die(mysql_error()); $count = mysql_result($result, 0); if ($count > 0) echo $curdomain; else echo "Domain not in database"; ?>
-
[SOLVED] PHP Sql UPDATE putting data into wrong columns
pocobueno1388 replied to jaxdevil's topic in PHP Coding Help
echo the query out to see if it's coming out how it's expected to. -
Your close the array twice right here: $info_box_contents[] = array('align' => 'left', 'text' => '<font color="' . $font_color . '">' . BOX_HEADING_ASEARCH . '</font>'); ); Just erase the last line -->);
-
session variable set twice on form submit
pocobueno1388 replied to Dragen's topic in PHP Coding Help
It's because your setting the variable every time the page is loaded, not just when the form is submitted. <?php session_start(); if (isset($_POST['go'])){ $_SESSION['bot'][] = date('U'); } print_r($_SESSION['bot']) ?> <form method="post" action="<?php echo $_SERVER['REQUEST_URI']; ?>"> <input type="submit" value="go" name="go" /> </form> -
Removing HTML tags from a database entry.
pocobueno1388 replied to poleposters's topic in PHP Coding Help
for removing HTML tags, use the function strip_tags(). Here is an example of limiting words <?php $str = "This is an example string of words This is an example string of words This is an example string of words"; $str = explode(" ", $str); //Replace "10" with how ever many words you want to be displayed for ($i=0; $i < 10; $i++){ echo $str[$i] .' '; } ?> -
I went through and commented your mistakes. If this doesn't fix it, tell us what the error is. <?php $con=mysql_connect(******) or die ('A problem has occurred'); //You should use quotes around the DB name mysql_select_db("write"); //Your were missing a single quote on the last variable $query="INSERT INTO articles (title, article, author, topic) VALUES ('$_POST[title]','$_POST[article]','$_POST[author]','$_POST[topic]')"; if (!mysql_query($query,$con)) { die('Problem Encountered'); } echo "Thank You"; //Missing a semi-colon after line mysql_close($con); ?>
-
[SOLVED] script to show images in my message :P
pocobueno1388 replied to runnerjp's topic in PHP Coding Help
On this line if ($emoticon == 1) Where is the variable $emoticon coming from? -
[SOLVED] script to show images in my message :P
pocobueno1388 replied to runnerjp's topic in PHP Coding Help
It worked fine for me... <?php $message = "Hello "; echo emoticon($message); function emoticon($msg){ $msg = str_replace("", "<IMG src=\".http://www.website.com/members/shoutbox/images/smile.gif\">", $msg); return $msg; } ?> -
Take a look at this tutorial http://www.tizag.com/phpT/fileappend.php