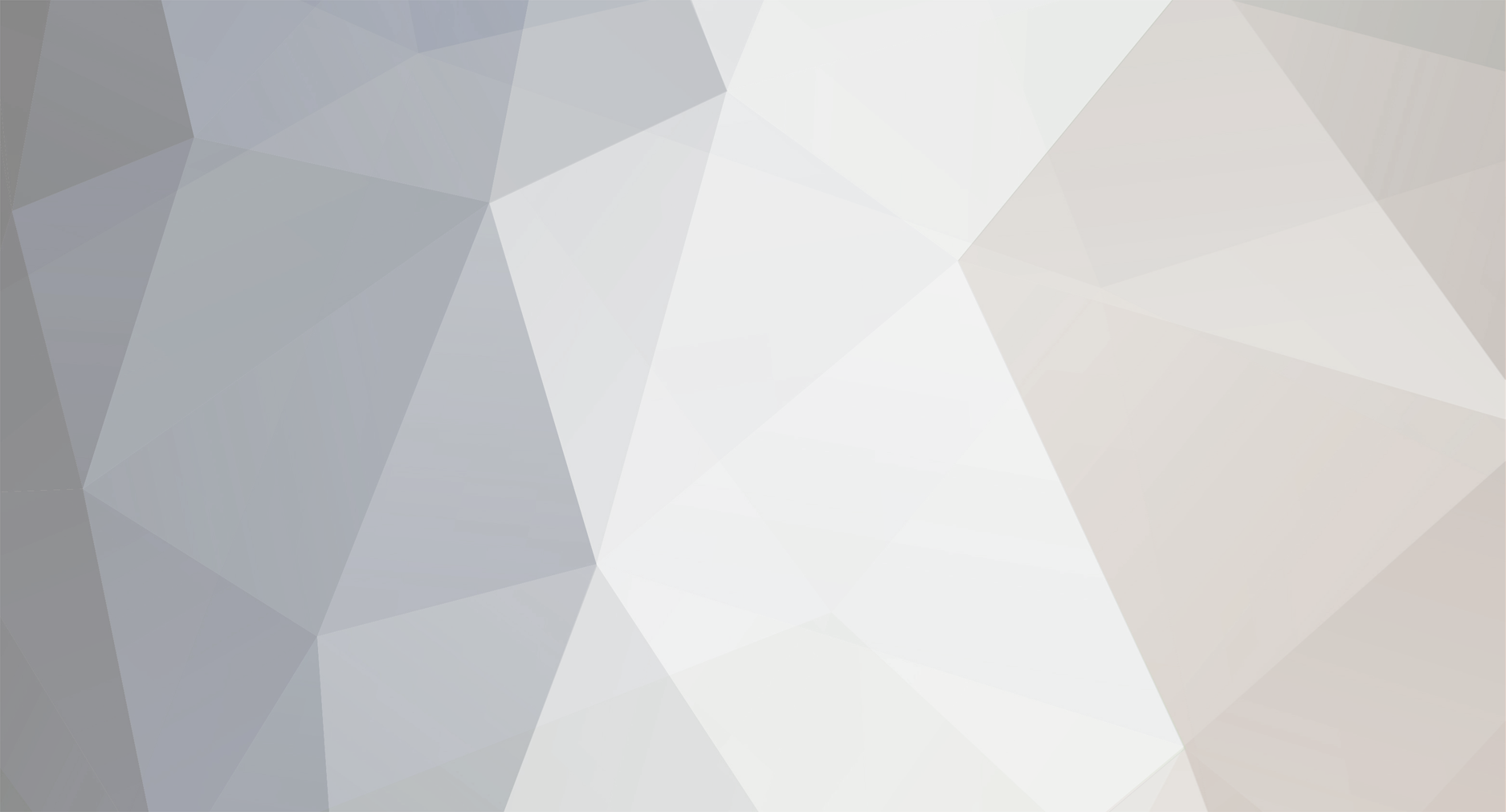
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
You have two options: 1) Reset the internal cursor: mysql_data_seek 2) Copy the results to an array. You possibly also have a third option: 3) Refactor your code to do it in one run. If at all possible, use #3.
-
This is a non-deterministic problem. How is the computer supposed to know if Denial is a mis-spelling Daniel? It sounds like your database is poorly designed if it allows for insertion and update anomalies and if it allows for multiple values to be stored in the same field.
-
Hashing functions do not have an inverse because they're not bijections. It should be quite obvious why for instance MD5 isn't invertible. Its output is always 32-bit, so if you give it 33-bit input (or n-bit input for n > 32) you've lost information. How would you figure out what the last bit(s) should be? Please not that you cannot "reverse" it by brute-force either. You can find input that has the same hash though. Kind of like n^2 = (-n)^2 but -n != n where n is a positive real number.
-
It depends on how you use it.
-
It means what it says: You cannot modify headers when output has been sent. That's just the way HTTP works. Headers are at the top (hence the name "header"). The solution: Don't output anything before you're certain you don't need to send any more headers.
-
As far as I know, C++ doesn't support regular expressions natively. You can just use any regex library, e.g. the ones in Boost/qt/.NET or you could use PCRE. You could possibly also use regex.h from C.
-
I've noticed turning off JScript has some notable benefits.
Daniel0 replied to PugJr's topic in Miscellaneous
Of course it'll be faster when you disable things. I'll also use less system resources if I boot into a terminal and don't start any graphical environment on my computer. -
?? You can get this result by playing with the rendering option in IE8's web development window. I'm not booted into Windows right now, but I'm pretty sure that my IE8 cannot do that.
-
It's your encoding that's messed up. You'll have to ensure that you use the same encoding throughout the entire process (in the database, when you connect to the database, strings that are hard coded into your files, on the HTML page, etc.).
-
His link is correct. It's SMF that is parsing the text incorrectly by not figuring out that the ending period part of the sentence and not the URL.
-
[SOLVED] [b]How To Send Email From Local Wamp To Internet [/b]
Daniel0 replied to Gomesh's topic in PHP Coding Help
You need to either a) Install a mail server on your computer. Search for one. There are plenty of free ones available. b) If you have access to an SMTP server you can modify the SMTP settings in php.ini. -
You can increase the maximum allowed memory usage in php.ini. It might be smarter working with chunks of data at a time instead of loading everything immediately.
-
Hmm... I had actually planned on upgrading the forums today. If there are any superstitious people here, maybe I should wait until tomorrow
-
Welcome back. I remember that you were active a couple of years ago. Nice to see you're back.
-
Unless you've profiled your code, you generally shouldn't start optimizing. Of course you should avoid obvious stupidities though.
-
European Commision objects Oracle's take over for sun
Daniel0 replied to nrg_alpha's topic in Miscellaneous
It's released under GPL, so if Oracle started charging for it or discontinuing it someone else could just fork it. -
Well, for the memcache extension in PHP, you'll have to make sure that the PHP development headers are installed on the server. Then you can run pecl install memcache Then you need to download memcached and compile it from source. Then install it.
-
From the manual: Did you take that into account when switching from 2 to 1?
-
Memcached is the daemon/service that is running in the background on the server (hence the 'd' for daemon). Memcache is what you use to interface with the daemon. It's unlikely you'll be able to install that if you're on shared hosting.
-
Well, if you have 1,2,3: Select the first element 1 from the rest 2,3: -- Then get the permutations of 2,3: ---- Select the first element 2 from the rest 3: ------ Then get the permutations of 3: That's just 3. ---- Now you know that (2,3) is a permutation of 2,3. ---- Select the next element 3 from the rest 2: ------ Then get the permutations of 2: That's just 2. ---- Now you know that (3,2) is a permutation of 2,3. ---- There are no more left. Return that ((2,3),(3,2)) are the permutations of 2,3. -- So add 1 to all of them and you know that ((1,2,3),(1,3,2)) are permutations of 1,2,3. -- Select the next element 2 from the rest 1,3: ---- Select the first element 1 from the rest 3: ------ Then get the permutations of 3: That's just 3. ---- Now you know that (1,3) is a permutation of 1,3. ---- Select the next element 3 from the rest 1: ------ Then get the permutations of 1: That's just 1. ---- Now you know that (3,1) is a permutation of 1,3. ---- There are no more left. Return that ((1,3),(3,1)) are the permutations of 1,3. -- So add 2 to all of them and you know that ((2,1,3),(2,3,1)) are permutations of 1,2,3. Combine that with last result and you have ((1,2,3),(1,3,2),(2,1,3),(2,3,1)) so far. -- Select the next element 3 from the rest 1,2: ---- Select the first element 1 from the rest 2: ------ Then get the permutations of 1: That's just 1. ---- Now you know that (1,2) is a permutation of 1,2. ---- Select the next element 2 from the rest 1: ------ Then get the permutations of 2: That's just 2. ---- Now you know that (2,1) is a permutation of 1,2. ---- There are no more left. Return that ((1,2),(2,1)) are the permutations of 2,1. -- So add 3 to all of them and you know that ((3,1,2),(3,2,1)) are permutations of 1,2,3. Combine that with last result and you have ((1,2,3),(1,3,2),(2,1,3),(2,3,1),(3,1,2),(3,2,1)) so far. -- You have no elements left. Return that ((1,2,3),(1,3,2),(2,1,3),(2,3,1),(3,1,2),(3,2,1)) are the permutations of 1,2,3. That's the general idea of the algorithm. I cut some corners obviously.
-
Try executing the function manually using something small like array(1,2) and array(1,2,3). Pretend that you're PHP and have to do this because somebody asked for it. Write down the (sub-)steps and (sub-)results while doing it.
-
You can always remember that by its name. "Greater than or equal to", so it's first > (greater than) and then = (equal to), i.e. >=.
-
If you want the permutations for n elements, you can calculate it for n-1 elements first. This might be easier to understand for you: <?php function getPermutations(array $items, array $acc = array()) { $return = array(); foreach ($items as $i => $item) { // go through our elements $rest = $items; array_splice($rest, $i, 1); // remove the i'th element $perms = getPermutations($rest); // get the permutations for the n-1 elements if (!isset($perms[0])) { // there were none... $return[] = array($item); // just add our item } else { // there were n >= 1 elements left foreach ($perms as $perm) { // go through these... $perm[] = $item; // ... and add our item $return[] = $perm; // then add to our results list } } } return $return; } var_dump(getPermutations(range(1,4))); Beware that it takes a lot of memory though. For just 15 elements there will be 1,307,674,368,000 permutations. For 20 there will be 2,432,902,008,176,640,000.