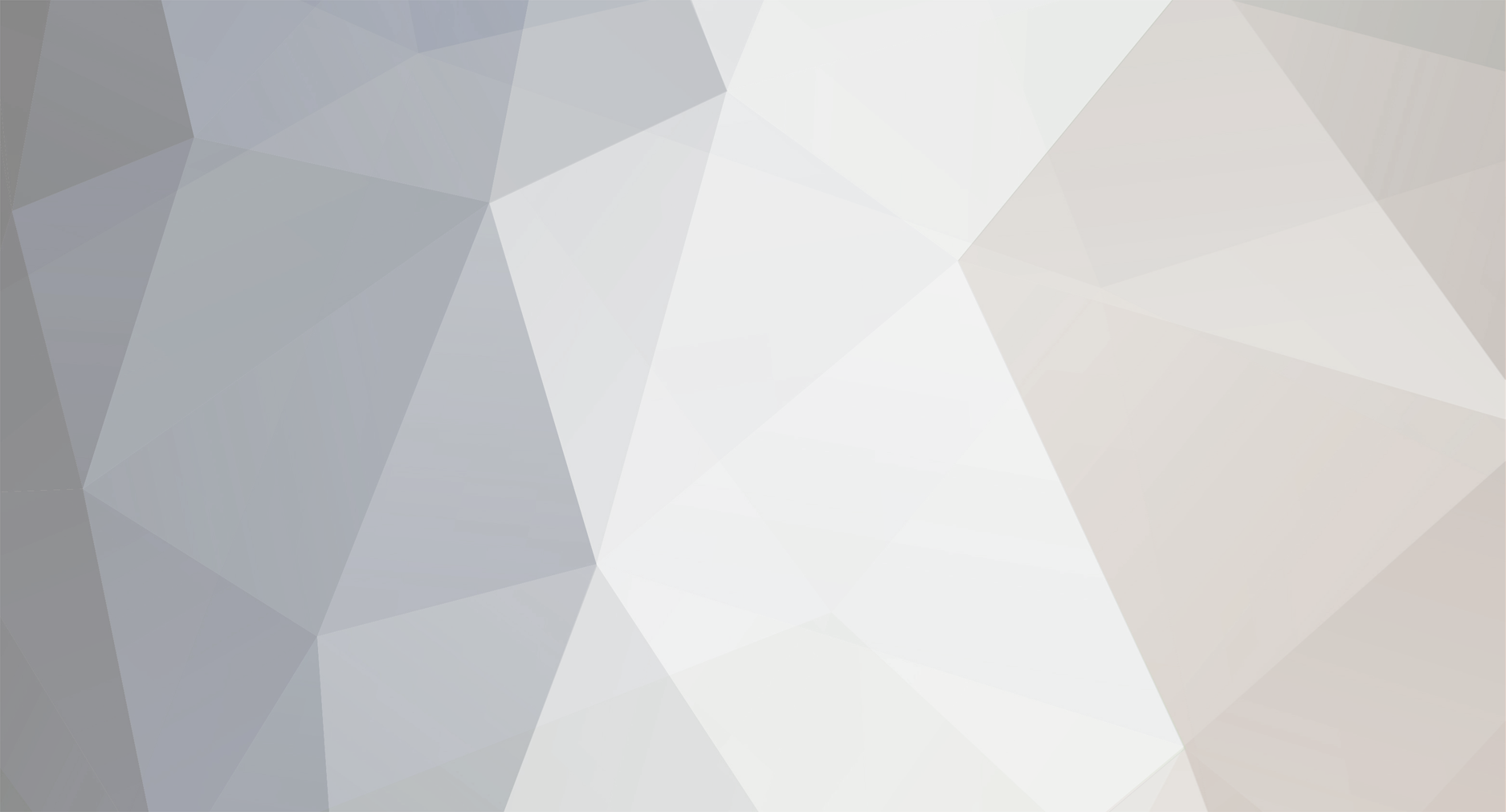
Jenk
Members-
Posts
778 -
Joined
-
Last visited
Never
Everything posted by Jenk
-
You build an application, you don't build patterns.
-
MVC is a pattern. Why oh why does everyone think it is something that can be "built"? where the hell do people get this information?
-
I've not read all of the replies, but what you have described is the functionality of a Service Locator, Factory and Registry. All 3 are separate behaviours. However, it is common (though not everyone agrees good practice, including me) to combine them. I prefer to just use them as intended.. Service Locator example: <?php class ServiceLocator { public function locate ($name, array $args) { if (!class_exists($name)) { include('/some/path/to/class/files/' . $name . '.arbitray_extension'); } $reflect = new ReflectionClass($name); return $reflect->newInstanceArgs($args); } } ?> A registry example: <?php class Registry { private $_registry = array(); public function register ($name, $object) { $this->_registry[$name] = $object; } public function fetch ($name) { if (!isset($this->_registry[$name])) { throw new OutOfRangeException('There is no object registered under ' . $name); } return $this->_registry[$name]; } } ?> Factory: <?php class SomeClassFactory { private $_class = 'SomeClass'; private $_defaultArgs = array('some', 'args'); public function create (array $args = null) { if (is_null($args)) { $args = $this->_defaultArgs; } $reflect = new ReflectionClass($this->_class); return $reflect->newInstanceArgs($args); } } ?> If you want to combine the behaviours, then cascade like so: <?php $someObject = Locator::locate('SomeClassFactory')->create(array('new', 'args')); $registry->register($someObject); ?>
-
Exactly.
-
That's not what I meant. You are overcomplicating the MVC Design Pattern. You are fully justified to have foresight for easy maintenance etc. but that is generic application design, and NOT MVC.
-
Exactamundo
-
It's important to only have one level deep transparency. That is to say, the main controller will only know/see the sub-controller below it. If that sub-controller needs to start another controller, the main controller does not need to know this. Let's take a url.. foo.php?controller=main&subcontroller=foo&somethingesle=bar. The front controller will only need to see the ver "controller" we can see it has the value of "main" so it will start which ever process is necessary for "main". public function run () { if (isset($_GET['controller']) { switch $_GET['controller'] { case 'main': $sub = new SubController; break; case 'notmain': $sub = new AnotherSubController; break; } $sub->run($_GET); } } So, next the subcontroller checks the value of "subcontroller" and takes appropriate action. public function run ($vars) { switch $vars['subcontroller'] { case 'foo': $this->fooAction(); break; case 'bar': $this->barAction(); break; } } and so forth.. sub controllers are often used for process like I described before, where multiple requests/stages will be used to complete a single objective or functionality. For example, the value of "subcontroller" could be "step1" or "step2" etc.
-
You've over complicated it again MVC is a Design Pattern, "Concept" over glorifies it. It literally is Model, View and Controller separate from each other - that is it. The one file separation still satisfies MVC. The moment you start thinking about anything else other than this separation, you have overcomplicated it, and are in actual fact thinking of generic application design, or everyday OO practices, or whatever.
-
pass mysql connection to an object as reference or doesnt matter?
Jenk replied to arianhojat's topic in PHP Coding Help
Technically that's not exposing anything. A better test would be to use the connection as a connection would be used, then compare scopes. The values are passed by reference, not the variables. There is a big difference there. $connection = mysql_connect(/* blah */); mysql_select_db('db', $connection); function doSomething ($var) { mysql_query('INSERT INTO `table` VALUES(\'a value\')', $var); } echo mysql_insert_id($connection); doSomething($connection); echo '<br />'; echo mysql_insert_id($connection); -
http://api.cakephp.org/controller_8php-source.html You can see some hard coupling in that source. Hard coupling prevents objects from being truly separate, thus you cannot achieve true MVC if you can't separate. CakePHP also has a few singletons, which I frown upon, but that is not relevant to this discussion.
-
CakePHP isn't strictly MVC. There are many "shortcuts" taken that prevent it being so. Hard coupling for one. The nuances I have with MVC include: 1) It's a buzzword, and thrown about like one.. CV/Resume.. marketing (See CakePHP and other frameworks) .. etc. 2) It is vastly over complicated. If your Applications Model, View and Controller are all separate from one another, you have achieved MVC. Separation does not require separate objects, it does not even require separate files. If you have you controller at the "top" of the page, the model in the "middle" of the page, and the View at the "bottom" you have achieved MVC.
-
It is a sub-controller, because it takes a fork of information, then whittles it down further still. I'd imagine you have met this scenario a few times before, you have an admin area of say a forum - so you'll have a controller for the admin area. This admin area has several areas of function for it, user maintenance, etc. A Page Controller would be assigned one of those functions each, as such you have the first action (Select user to maintain), then at least one more action for that function (i.e. make your changes) - all the while, the main controller knows you are using the admin area, the next controller knows you are still in user maintenance, then your page controller knows you have moved to the next stage of user maintenance (or finished.) A Front Controller translates from a raw request into usable data, interprets which action is to be fired, and fires it. The discussion of Front Controllers in PHP is debatable, some argue Apache/IIS is the front controller, others argue that is outside the context of PHP.
-
Why oh why would you want to use anything remotely like Page Controller? Page controllers are very useful. They are nothing more than sub-controllers. http://martinfowler.com/eaaCatalog/pageController.html
-
pass mysql connection to an object as reference or doesnt matter?
Jenk replied to arianhojat's topic in PHP Coding Help
All objects are passed by reference in php5+ -
Template engines are the devil's spawn. It only adds an extra syntax for you to learn, which is pointless.
-
If the value is a value that is shared amongst all objects of that class, and must be the same amongst all instances, or is for use within static methods, then it should be static, or perhaps constant if the value never changes. If the value is individual for each instance, then it must be non-static.
-
That I quite like, it enforces the same principle as I use anyway.
-
I always start my projects with OO.
-
An alternative (and my personal preference) would not be to have an abstract architecure like the above, but to delegate the accessing functionality to a child object, rather than a child class. $session->setAccessor(new FileAccessor('/foo')); or $session->setAccessor(new DBAccessor('mysql://username/pass/db')); We've already had somewhat heated discussions on my views of singletons. So I won't go into detail, but in summary, they break encapsulation, make testing difficult, and are just altogether icky.
-
Making objects from primitive types would probably be too far, yes. But when the rest of your application is well structured objects, you'll never need to actually use a primitive. And the comment regarding OO making it difficult for other developers to jump in is not a valid point. Any OO experienced developer will tell you that jumping into legacy code (i.e. procedural) is a LOT more difficult.
-
It is impossible to go too far with OOP. Seriously, impossible. Other languages are nothing but objects. No such thing as a function or primitive variable.
-
I don't see why you'd want to access private/protected member variables because that is the point of them being private/protected... to be private and/or protected. What are you trying to achieve?
-
Simple, don't let them access the files. Serve them via PHP so they cannot execute, only download as attachments.
-
You do realise "Design Patterns" are simply identifiable/recognised common scenarios, right? They are not their own product, thus OOP is not "useless" without them.. Besides which, your Actor's constructor breaks the whole reason you are using that pattern in the first place.. you should be using a Factory to provide the default speech object, then your Bridge pattern would be complete.